Tag Archives: c++
C++ || How To Get Distinct & Unique Values In An Array/Vector/Container & Remove Duplicates Using C++
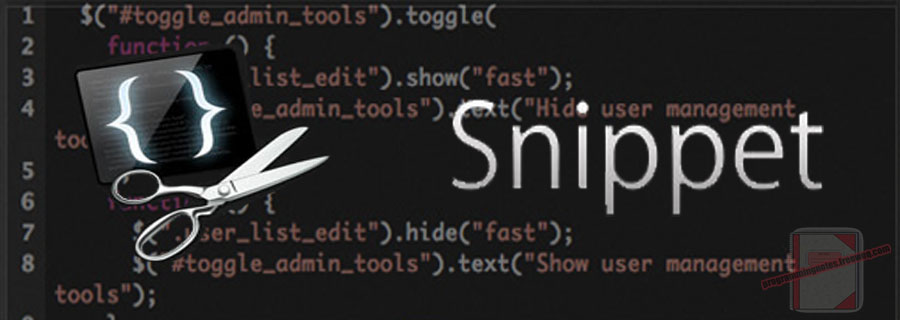
The following is a module with functions which demonstrates how to get distinct and unique values in an array/vector/container and remove duplicates using C++.
The function demonstrated on this page is a template, so it should work on containers of any type.
The function also does not require a sort, it preserves the original order of the vector, uses the equality operator (operator==), and allows for a custom predicate comparison function to determine whether the arguments are equal.
1. Distinct – String Array
The example below demonstrates the use of ‘Utils::distinct‘ to get the distinct elements from an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Distinct - String Array // Declare array std::string names[] = { "Kenneth", "Jennifer", "Lynn", "Sole", "Kenneth", "Jennifer" }; // Get array size int size = sizeof(names) / sizeof(names[0]); // Get distinct values auto results = Utils::distinct(names, names + size); // Display results for (const auto& name : results) { std::cout << "Name: " << name << std::endl; } // expected output: /* Name: Kenneth Name: Jennifer Name: Lynn Name: Sole */ |
2. Distinct – Object Vector
The example below demonstrates the use of ‘Utils::distinct‘ to get the distinct elements from an object vector.
The object in this example overloads the equality operator to determine whether arguments are equal.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// Distinct - Object Vector // Declare object struct Person { int id; std::string name; bool operator==(const Person& rhs) const {return name == rhs.name;} }; // Declare data std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Get distinct values using object equality operator auto results = Utils::distinct(people.begin(), people.end()); // Display results for (const auto& person : results) { std::cout << "ID: " << person.id << ", Name: " << person.name << std::endl; } // expected output: /* ID: 31, Name: Kenneth ID: 28, Name: Jennifer ID: 87, Name: Lynn ID: 91, Name: Sole */ |
3. Distinct – Object Vector Predicate
The example below demonstrates the use of ‘Utils::distinct‘ to get the distinct elements from an object vector.
In this example, a predicate is used to determine whether arguments are equal.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
// Distinct - Object Vector Predicate // Declare object struct Person { int id; std::string name; }; // Declare data std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Declare custom comparison predicate auto pred = [](const auto& lhs, const auto& rhs) {return lhs.name == rhs.name;}; // Get distinct values using predicate auto results = Utils::distinct(people.begin(), people.end(), pred); // Display results for (const auto& person : results) { std::cout << "ID: " << person.id << ", Name: " << person.name << std::endl; } // expected output: /* ID: 31, Name: Kenneth ID: 28, Name: Jennifer ID: 87, Name: Lynn ID: 91, Name: Sole */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 22, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <algorithm> #include <iterator> namespace Utils { /** * FUNCTION: distinct * USE: Returns distinct elements in the given range [first, last) * @param first: The first position of the sequence * @param last: The last position of the sequence * @param predicate: A comparison function to check whether arguments are equal * @return: A container with distinct elements from the given range */ template<typename InputIt, typename T = typename std::iterator_traits<InputIt>::value_type, typename Pred> std::vector<T> distinct(InputIt first, InputIt last, const Pred& predicate) { std::vector<T> results; std::copy_if(first, last, std::back_inserter(results), [&](const T& lhs) { return std::find_if(results.begin(), results.end(), [&](const T& rhs) { return predicate(lhs, rhs); }) == results.end(); } ); return results; } /** * FUNCTION: distinct * USE: Returns distinct elements in the given range [first, last) * @param first: The first position of the sequence * @param last: The last position of the sequence * @return: A container with distinct elements from the given range */ template<typename InputIt, typename T = typename std::iterator_traits<InputIt>::value_type> std::vector<T> distinct(InputIt first, InputIt last) { auto pred = [](const T& x, const T& y) { return x == y; }; return distinct(first, last, pred); } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 22, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare array std::string names[] = { "Kenneth", "Jennifer", "Lynn", "Sole", "Kenneth", "Jennifer" }; // Get array size int size = sizeof(names) / sizeof(names[0]); // Get distinct values auto results = Utils::distinct(names, names + size); // Display results for (const auto& name : results) { display("Name: " + name); } display(""); // Declare object struct Person { int id; std::string name; bool operator==(const Person& rhs) const { return name == rhs.name; } }; // Declare data std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Get distinct values using object equality operator auto results2 = Utils::distinct(people.begin(), people.end()); // Display results for (const auto& person : results2) { display("ID: " + std::to_string(person.id) + ", Name: " + person.name); } display(""); // Declare custom comparison predicate auto pred = [](const auto& lhs, const auto& rhs) { return lhs.name == rhs.name; }; // Get distinct values using predicate auto results3 = Utils::distinct(people.begin(), people.end(), pred); // Display results for (const auto& person : results3) { display("ID: " + std::to_string(person.id) + ", Name: " + person.name); } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Create A Simple Predictive Spell Checker Using C++
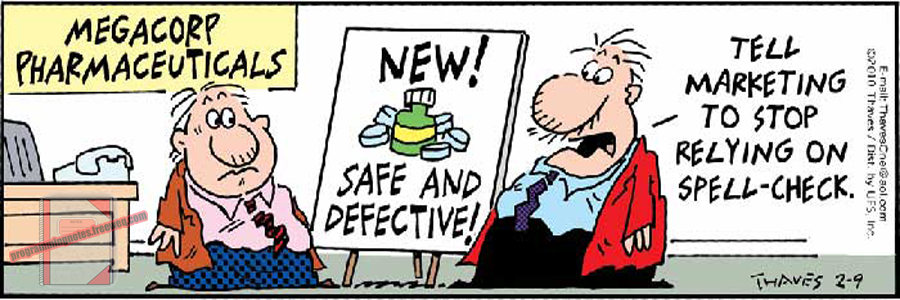
The following is a module with functions which demonstrates how to create a simple predictive spell checker using C++.
The module demonstrated on this page is an implementation based on the Norvig Spelling Corrector.
1. Overview
In its simplest form, this process works by first telling the spelling corrector the valid words in our language. This is called “training” the corrector. Once the corrector is trained to know the valid words in our language, it tries to choose the word that is the most likely spelling correction.
This process is done by choosing the candidate with the highest probability to show up in our language. For example, should “lates” be corrected to “late” or “latest” or “lattes” or …? This choice is determined by the word which has the highest probability to appear in our language, according to the training text we provide.
Traditionally, spell checkers look for four possible errors: a wrong letter (“wird”), also knows as alteration. An inserted letter (“woprd”), a deleted letter (“wrd”), or a pair of adjacent transposed letters (“wrod”). This process is used when generating probable spelling correction candidates.
The training text used in the example on this page can be downloaded here. This file consists of 80891 distinct words, which is used to train the spelling corrector.
2. Spell Check – Basic Usage
The example below demonstrates the use of the ‘SpellChecker‘ class to load the training file and correct the spelling of a word.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Spell Check - Basic Usage // Declare spell checker SpellChecker spellchecker; // Declare training text path std::string path = "INPUT_Dictionary_programmingnotes_org.txt"; // Add training text from a file spellchecker.trainFromFile(path); // Optional: Add training text from an std::string //spellchecker.train(); // Correct a word and display results std::cout << spellchecker.correct("KENNtH"); // example output: /* KENNetH */ |
3. Spell Check – Unit Tests
The example below demonstrates the use of the ‘SpellChecker‘ class to load the training file and correct the spelling of a word.
In this example, words are added to a set to determine if the most likely spelling correction is returned by the spelling corrector. The first word in the set is the word to test against (some are spelled correctly, some incorrectly). The second word in the set is the expected result that should be returned by the spelling corrector.
If no spelling suggestion is found, an empty string is returned by the spelling corrector. This is true for one of the words in the test cases below. Even though the word is spelled correctly, it is not defined in our training text, and no correction can be found.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 |
// Spell Check - Unit Tests // Declare spell checker SpellChecker spellchecker; // Declare training text path std::string path = "INPUT_Dictionary_programmingnotes_org.txt"; // Add training text from a file spellchecker.trainFromFile(path); // Declare words to check spelling. // The first word in the set is the current spelling, the second word // is the expected corrected spell checker result std::pair<std::string, std::string> cases[] = { {"KENNtH", "KENNetH"}, {"Jennierr", "Jennifer"}, {"LYnNn", "Lynn"}, {"Soole", "Sole"}, {"speling", "spelling"}, {"korrectud", "corrected"}, {"bycycle", "bicycle"}, {"inconvient", "inconvenient"}, {"arrainged", "arranged"}, {"peotry", "poetry"}, {"peotryy", "poetry"}, {"word", "word"}, {"quintessential", "quintessential"}, {"transportibility", "transportability"}, {"addresable", "addressable"}, {"auxiliaryy", "auxiliary"}, {"WirD", "WorD"}, {"prplee", "purple"}, {"Succesfuil", "Successful"}, {"AMEIRICUA", "AMERICA"}, {"Langauege", "Language"} }; // Correct the words in the test cases for (const auto& pair : cases) { // Correct word auto correction = spellchecker.correct(pair.first); // Check to see if correction matches the expected result bool casePassed = (strcasecmp(correction.c_str(), pair.second.c_str()) == 0); // Display results if (casePassed) { std::cout << "Passed - Original: " + pair.first + ", Correction: " + correction << std::endl; } else { std::cout << " *** Failed - Original: " + pair.first + ", Correction: " + correction + ", Expected: " + pair.second << std::endl; } } // example output: /* Passed - Original: KENNtH, Correction: KENNetH Passed - Original: Jennierr, Correction: Jennifer Passed - Original: LYnNn, Correction: LYNn Passed - Original: Soole, Correction: Sole Passed - Original: speling, Correction: spelling Passed - Original: korrectud, Correction: corrected Passed - Original: bycycle, Correction: bicycle Passed - Original: inconvient, Correction: inconvenient Passed - Original: arrainged, Correction: arranged Passed - Original: peotry, Correction: poetry Passed - Original: peotryy, Correction: poetry Passed - Original: word, Correction: word *** Failed - Original: quintessential, Correction: , Expected: quintessential Passed - Original: transportibility, Correction: transportability Passed - Original: addresable, Correction: addressable Passed - Original: auxiliaryy, Correction: auxiliary Passed - Original: WirD, Correction: WorD Passed - Original: prplee, Correction: purple Passed - Original: Succesfuil, Correction: Successful Passed - Original: AMEIRICUA, Correction: AMERICA Passed - Original: Langauege, Correction: Language */ |
4. SpellChecker Class
The following is the SpellChecker Class. Include this in your project to start using!
The following is the header file ‘SpellChecker.h‘.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 18, 2020 // Taken From: http://programmingnotes.org/ // File: SpellChecker.h // Description: Header file for a predictive spell checker class that // generates spelling corrections // ============================================================================ #pragma once #include <string> #include <unordered_set> #include <utility> #include <unordered_map> #include <cctype> #ifdef _MSC_VER #include <string.h> #define strncasecmp _strnicmp #define strcasecmp _stricmp #else #include <strings.h> #endif class SpellChecker { public: SpellChecker() {} /** * FUNCTION: correct * USE: Corrects the spelling of a word. If the word is spelled incorrectly, * a spelling suggestion is returned. If it is spelled correctly, the * initial word is returned. If no spelling suggestion is found, an empty * string is returned * @param word: The word to correct * @return: The spelling correction of the word */ std::string correct(const std::string& word); /** * FUNCTION: train * USE: Adds training text to the spell checker * @param text: The training text * @return: N/A */ void train(const std::string& text); /** * FUNCTION: trainFromFile * USE: Reads a file containing training text and adds it to the spell checker * @param path: The path of the file containing training text * @return: N/A */ void trainFromFile(const std::string& path); /** * FUNCTION: exists * USE: Determines if a word is a valid word in the spell checker * @param word: The word to test against * @return: True if the word exists in the spell checker, false otherwise */ bool exists(const std::string& word); ~SpellChecker() { NWORDS.clear(); } private: // Makes map keys case insensitive struct case_insensitive { struct comp { bool operator() (const std::string& lhs, const std::string& rhs) const { return strcasecmp(lhs.c_str(), rhs.c_str()) == 0; } }; struct hash { std::size_t operator() (std::string str) const { for (std::size_t index = 0; index < str.size(); ++index) { auto ch = static_cast<unsigned char>(str[index]); str[index] = static_cast<unsigned char>(std::tolower(ch)); } return std::hash<std::string>{}(str); } }; }; typedef std::unordered_map<std::string, unsigned , case_insensitive::hash, case_insensitive::comp> dictionary; std::unordered_set<std::string> edit(const std::unordered_set<std::string>& words); std::unordered_set<std::string> known(const std::unordered_set<std::string>& words); std::string max(const std::unordered_set<std::string>& candidates, const std::string& word); std::unordered_set<std::string> getCandidates(const std::string& word); dictionary NWORDS; const std::string alphabet = "abcdefghijklmnopqrstuvwxyz"; };// http://programmingnotes.org/ |
The following is the implementation file ‘SpellChecker.cpp‘.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 18, 2020 // Taken From: http://programmingnotes.org/ // File: SpellChecker.cpp // Description: Implementation file for a predictive spell checker class // that generates spelling corrections // ============================================================================ #include "SpellChecker.h" #include <string> #include <sstream> #include <unordered_set> #include <fstream> #include <stdexcept> #include <algorithm> #include <cmath> #include <cctype> void clean(std::string& word) { auto isInvalidChar = [](unsigned char c) { return std::ispunct(c) && c != '\'' && c != '-'; }; for (int index = (int)word.size() - 1; index >= 0; --index) { if (isInvalidChar(word[index])) { word.erase(index, 1); } } } std::string SpellChecker::correct(const std::string& word) { std::string suggestion = ""; // Get spelling suggestions for the word auto candidates = getCandidates(word); if (!candidates.empty()) { suggestion = max(candidates, word); } return suggestion; } void SpellChecker::trainFromFile(const std::string& path) { std::ifstream infile; infile.open(path); if (infile.fail()) { throw std::invalid_argument{ "Unable to read file at path: '" + path + "'" }; } infile.seekg(0, std::ios::end); auto size = infile.tellg(); std::string contents((std::size_t)size, ' '); infile.seekg(0); infile.read(&contents[0], size); infile.close(); train(contents); } void SpellChecker::train(const std::string& text) { std::stringstream strStream(text); while (strStream) { std::string word; strStream >> word; clean(word); if (word.empty()) { continue; } NWORDS[word] += 1; } } std::unordered_set<std::string> SpellChecker::getCandidates(const std::string& word) { auto candidates = known({ word }); // Edits 1 std::unordered_set<std::string> firstEdit; if (candidates.empty()) { firstEdit = edit({ word }); candidates = known(firstEdit); } // Edits 2 if (candidates.empty()) { candidates = known(edit(firstEdit)); } return candidates; } std::string SpellChecker::max(const std::unordered_set<std::string>& candidates, const std::string& word) { std::string maxKey; const double nullValue = -1987199120102019; auto maxValue = nullValue; for (const auto& candidate : candidates) { int currentValue = NWORDS[candidate]; // Add a penalty to the candidate based on the length change auto lengthChange = std::abs((int)(candidate.size() - word.size())); currentValue -= lengthChange; if (maxValue == nullValue || currentValue > maxValue) { maxKey = candidate; maxValue = currentValue; } } return maxKey; } bool SpellChecker::exists(const std::string& word) { return !NWORDS.empty() && NWORDS.find(word) != NWORDS.end(); } std::unordered_set<std::string> SpellChecker::edit(const std::unordered_set<std::string>& words) { std::unordered_set<std::string> edits; for (const auto& word : words) { for (std::size_t index = 0; index <= word.size(); ++index) { auto a = word.substr(0, index); auto b = word.substr(index); auto c = b.size() > 1 ? b.substr(1) : ""; auto d = b.size() > 2 ? b.substr(2) : ""; if (!b.empty()) { // Deletes edits.insert(a + c); // Transposes if (b.size() > 1) { edits.insert(a + b[1] + b[0] + d); } // Alteration & Inserts for (const auto& letter : alphabet) { // Alteration edits.insert(a + letter + c); // Inserts edits.insert(a + letter + b); } } else { // Inserts (remaining set at the end) for (const auto& letter : alphabet) { edits.insert(a + letter); } } } } return edits; } std::unordered_set<std::string> SpellChecker::known(const std::unordered_set<std::string>& words) { std::unordered_set<std::string> set; for (const auto& word : words) { if (exists(word)) { set.insert(word); } } return set; }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘SpellChecker‘ class. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 18, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the SpellChecker class // ============================================================================ #include <iostream> #include <string> #include <exception> #include <utility> #include "SpellChecker.h" void display(const std::string& message); int main() { try { // Declare spell checker SpellChecker spellchecker; // Declare training text path std::string path = "INPUT_Dictionary_programmingnotes_org.txt"; // Add training text from a file spellchecker.trainFromFile(path); // Optional: Add training text from an std::string //spellchecker.train(); // Correct a word and display results display(spellchecker.correct("KENNtH")); display(""); // Declare words to check spelling. // The first word in the set is the current spelling, the second word // is the expected corrected spell checker result std::pair<std::string, std::string> cases[] = { {"KENNtH", "KENNetH"}, {"Jennierr", "Jennifer"}, {"LYnNn", "Lynn"}, {"Soole", "Sole"}, {"speling", "spelling"}, {"korrectud", "corrected"}, {"bycycle", "bicycle"}, {"inconvient", "inconvenient"}, {"arrainged", "arranged"}, {"peotry", "poetry"}, {"peotryy", "poetry"}, {"word", "word"}, {"quintessential", "quintessential"}, {"transportibility", "transportability"}, {"addresable", "addressable"}, {"auxiliaryy", "auxiliary"}, {"WirD", "WorD"}, {"prplee", "purple"}, {"Succesfuil", "Successful"}, {"AMEIRICUA", "AMERICA"}, {"Langauege", "Language"} }; // Correct the words in the test cases for (const auto& pair : cases) { // Correct word auto correction = spellchecker.correct(pair.first); // Check to see if correction matches the expected result bool casePassed = (strcasecmp(correction.c_str(), pair.second.c_str()) == 0); // Display results if (casePassed) { display("Passed - Original: " + pair.first + ", Correction: " + correction); } else { display(" *** Failed - Original: " + pair.first + ", Correction: " + correction + ", Expected: " + pair.second); } } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Remember to include the training file.
C++ || How To Execute & Get Regex Match Results Using C++
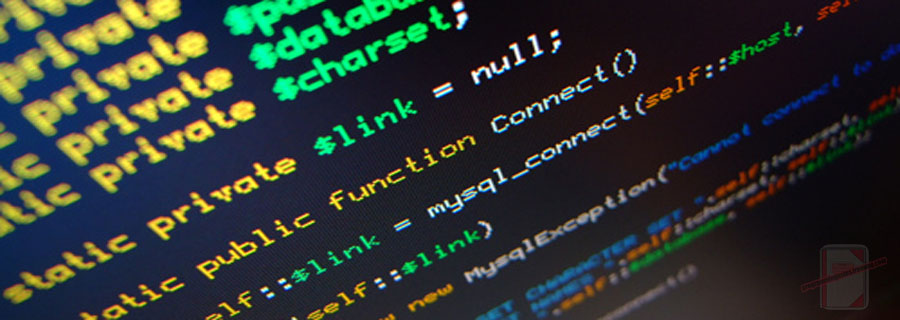
The following is a module with functions which demonstrates how to execute and get regex match results using C++.
The function demonstrated on this page uses regex to search a specified input string for all occurrences of a specified regular expression. The function returns a collection of std::smatch objects found by the search.
1. Get Regex Matches
The example below demonstrates the use of ‘Utils::getRegexMatches‘ to search an input string for all occurrences of a regular expression.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Get Regex Matches // Declare source std::string input = "Kenneth, Jennifer, Lynn, Sole"; // Declare pattern std::string pattern = R"(\w+)"; // Find matches auto matches = Utils::getRegexMatches(pattern, input); // Display results for (const auto& match : matches) { // Display full match std::cout << "Match: " << match.str() << std::endl; } // example output: /* Match: Kenneth Match: Jennifer Match: Lynn Match: Sole */ |
2. Get Regex Matches – Sub Match
The example below demonstrates the use of ‘Utils::getRegexMatches‘ to search an input string for all occurrences of a regular expression.
In this example, sub matches are expected and displayed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// Get Regex Matches - Sub Match // Declare source std::string input = "212-555-6666 906-932-1111 415-222-3333 425-888-9999"; // Declare pattern std::string pattern = R"((\d{3})-(\d{3}-\d{4}))"; // Find matches auto matches = Utils::getRegexMatches(pattern, input); // Display results for (const auto& match : matches) { // Display full match std::cout << "Full Match: " << match.str() << std::endl; // Display any submatches for (unsigned index = 1; index < match.size(); ++index) { std::cout << " Sub Match #" << index << ": " << match.str(index) << std::endl; } } // example output: /* Full Match: 212-555-6666 Sub Match #1: 212 Sub Match #2: 555-6666 Full Match: 906-932-1111 Sub Match #1: 906 Sub Match #2: 932-1111 Full Match: 415-222-3333 Sub Match #1: 415 Sub Match #2: 222-3333 Full Match: 425-888-9999 Sub Match #1: 425 Sub Match #2: 888-9999 */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 11, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <regex> #include <vector> namespace Utils { /** * FUNCTION: getRegexMatches * USE: Searches the specified input string for all occurrences of a * specified regular expression * @param pattern: The regular expression pattern to match * @param input: The string to search for a match * @return: A collection of std::smatch objects found by the search. If * no matches are found, the method returns an empty collection */ auto getRegexMatches(const std::string& pattern, const std::string& input) { std::vector<std::smatch> results; std::regex rgx(pattern); for (std::sregex_iterator it(input.begin(), input.end(), rgx), end; it != end; ++it) { results.push_back(*it); } return results; } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 11, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare source std::string input = "Kenneth, Jennifer, Lynn, Sole"; // input = "212-555-6666 906-932-1111 415-222-3333 425-888-9999"; // Declare pattern std::string pattern = R"(\w+)"; // pattern = R"((\d{3})-(\d{3}-\d{4}))"; // Find matches auto matches = Utils::getRegexMatches(pattern, input); // Display results for (const auto& match : matches) { // Display full match std::cout << "Full Match: " << match.str() << std::endl; // Display any submatches for (unsigned index = 1; index < match.size(); ++index) { std::cout << " Sub Match #" << index << ": " << match.str(index) << std::endl; } } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Trim & Remove The Leading & Trailing Whitespace From A String Using C++
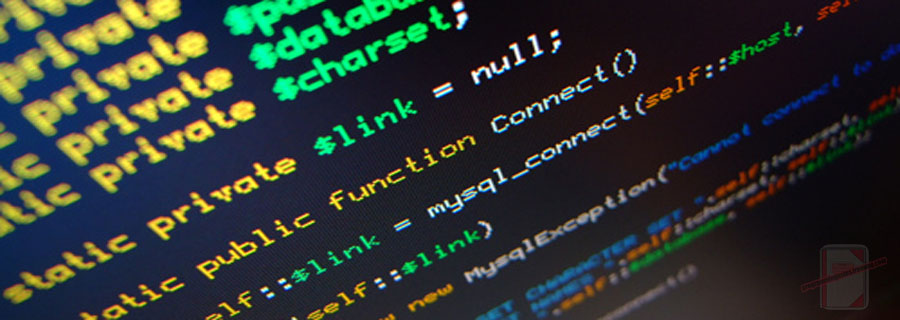
The following is a module with functions which demonstrates how to trim and remove the leading and trailing whitespace from a string using C++.
1. Trim
The example below demonstrates the use of ‘Utils::trim‘ to remove all the leading and trailing whitespace characters from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Trim // Declare string std::string original = " <-Kenneth-> "; // Trim the string auto trim = Utils::trim(original); // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Trim: '" << trim << "'" << std::endl; // expected output: /* Original: ' <-Kenneth-> ' Trim: '<-Kenneth->' */ |
2. Trim Start
The example below demonstrates the use of ‘Utils::trimStart‘ to remove all leading whitespace characters from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Trim Start // Declare string std::string original = " <-Kenneth-> "; // Trim the start of string auto ltrim = Utils::trimStart(original); // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Trim Start: '" << ltrim << "'" << std::endl; // expected output: /* Original: ' <-Kenneth-> ' Trim Start: '<-Kenneth-> ' */ |
3. Trim End
The example below demonstrates the use of ‘Utils::trimEnd‘ to remove all trailing whitespace characters from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Trim End // Declare string std::string original = " <-Kenneth-> "; // Trim the end of string auto rtrim = Utils::trimEnd(original); // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Trim End: '" << rtrim << "'" << std::endl; // expected output: /* Original: ' <-Kenneth-> ' Trim End: ' <-Kenneth->' */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 10, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> #include <algorithm> #include <cctype> namespace Utils { /** * FUNCTION: trimEnd * USE: Returns a new string with all trailing whitespace characters removed * @param source: The source string * @return: A new string with all the trailing whitespace characters removed */ std::string trimEnd(std::string source) { source.erase(std::find_if(source.rbegin(), source.rend(), [](char c) { return !std::isspace(static_cast<unsigned char>(c)); }).base(), source.end()); return source; } /** * FUNCTION: trimStart * USE: Returns a new string with all leading whitespace characters removed * @param source: The source string * @return: A new string with all the leading whitespace characters removed */ std::string trimStart(std::string source) { source.erase(source.begin(), std::find_if(source.begin(), source.end(), [](char c) { return !std::isspace(static_cast<unsigned char>(c)); })); return source; } /** * FUNCTION: trim * USE: Returns a new string with all the leading and trailing whitespace * characters removed * @param source: The source string * @return: A new string with all the leading and trailing whitespace * characters removed */ std::string trim(std::string source) { return trimEnd(trimStart(source)); } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 10, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare string std::string original = " <-Kenneth-> "; // Trim the string auto trim = Utils::trim(original); // Display result display("Original: '" + original + "'"); display("Trim: '" + trim + "'"); display(""); // Trim the start of string auto ltrim = Utils::trimStart(original); // Display result display("Original: '" + original + "'"); display("Trim Start: '" + ltrim + "'"); display(""); // Trim the end of string auto rtrim = Utils::trimEnd(original); // Display result display("Original: '" + original + "'"); display("Trim End: '" + rtrim + "'"); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Check If A String Starts & Ends With A Certain String Using C++
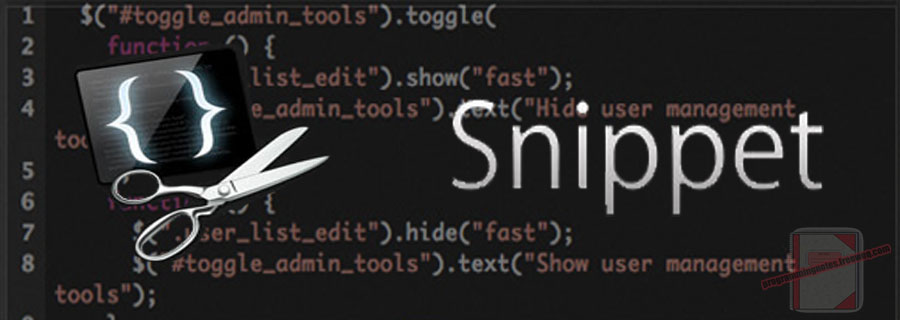
The following is a module with functions which demonstrates how to determine if a string starts and ends with a certain substring using C++.
1. Starts With
The example below demonstrates the use of ‘Utils::startsWith‘ to determine whether the beginning of a string instance matches a specified string value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Starts With // Declare values std::string values[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search = "Th"; // Check if items starts with search value for (const auto& value : values) { std::cout << "'" << value << "' starts with '" << search << "': " << (Utils::startsWith(value, search) ? "True" : "False") << std::endl; } // example output: /* 'This is a string.' starts with 'Th': True 'Hello!' starts with 'Th': False 'Nothing.' starts with 'Th': False 'Yes.' starts with 'Th': False 'randomize' starts with 'Th': False */ |
2. Ends With
The example below demonstrates the use of ‘Utils::endsWith‘ to determine whether the end of a string instance matches a specified string value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Ends With // Declare values std::string values[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search = "."; // Check if items ends with search value for (const auto& value : values) { std::cout << "'" << value << "' ends with '" << search << "': " << (Utils::endsWith(value, search) ? "True" : "False") << std::endl; } // example output: /* 'This is a string.' ends with '.': True 'Hello!' ends with '.': False 'Nothing.' ends with '.': True 'Yes.' ends with '.': True 'randomize' ends with '.': False */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 9, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> namespace Utils { /** * FUNCTION: startsWith * USE: Determines whether the beginning of a string instance matches * a specified string value * @param source: The source string * @param value: The string to compare * @return: True if 'value' matches the beginning of 'source', * False otherwise */ bool startsWith(const std::string& source, const std::string& value) { return source.size() >= value.size() && source.compare(0, value.size(), value) == 0; } /** * FUNCTION: endsWith * USE: Determines whether the end of a string instance matches * a specified string value * @param source: The source string * @param value: The string to compare to the substring at the end * @return: True if 'value' matches the end of 'source', * False otherwise */ bool endsWith(const std::string& source, const std::string& value) { return source.size() >= value.size() && source.compare(source.size() - value.size(), value.size(), value) == 0; } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 9, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare values std::string values[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search = "Th"; // Check if items starts with search value for (const auto& value : values) { std::cout << "'" << value << "' starts with '" << search << "': " << (Utils::startsWith(value, search) ? "True" : "False") << std::endl; } display(""); // Declare values std::string values2[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search2 = "."; // Check if items ends with search value for (const auto& value : values2) { std::cout << "'" << value << "' ends with '" << search2 << "': " << (Utils::endsWith(value, search2) ? "True" : "False") << std::endl; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Read An Entire File Into A String & Write A String To A File Using C++
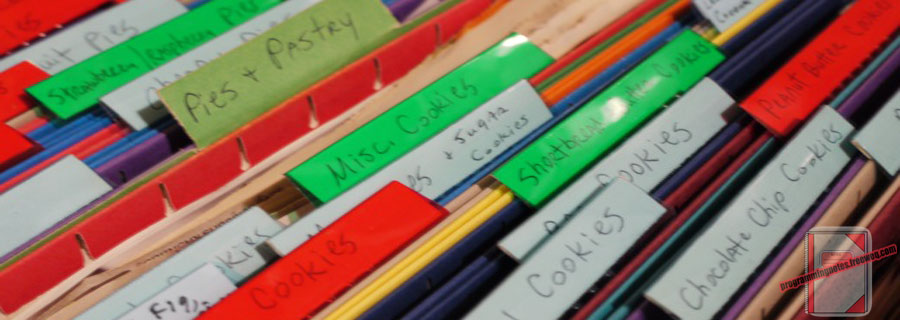
The following is a module with functions which demonstrates how to write a string to a file and read an entire file to a string using C++.
The functions demonstrated on this page uses fstream to read and write data to and from a file.
1. Read All Text
The example below demonstrates the use of ‘Utils::readAllText‘ to open a text file, read all the text in the file into a string, and then close the file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Read All Text // Declare path std::string path = "C:\\Users\\Name\\Desktop\\text.txt"; // Read the contents of the file at the specified path std::string contents = Utils::readAllText(path); // Display contents std::cout << contents; // example output: /* ... The contents of the file */ |
2. Write All Text
The example below demonstrates the use of ‘Utils::writeAllText‘ to create a new file, write a specified string to the file, and then close the file. If the target file already exists, it is overwritten.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Write All Text // Declare path std::string path = "C:\\Users\\Name\\Desktop\\text.txt"; // Declare file contents std::string contents = "Kenneth, Jennifer, Lynn, Sole"; // Save contents to the specified path Utils::writeAllText(path, contents); // ... Contents are written to the file |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 9, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> #include <fstream> #include <limits> #include <stdexcept> namespace Utils { /** * FUNCTION: readAllText * USE: Opens a text file, reads all the text in the file into a string, * and then closes the file * @param path: The file to open for reading * @return: A string containing all text in the file */ std::string readAllText(const std::string& path) { std::ifstream infile(path); if (infile.fail()) { throw std::invalid_argument{ "Unable to read file at path: '" + path + "'" }; } infile.ignore(std::numeric_limits<std::streamsize>::max()); std::streamsize size = infile.gcount(); infile.clear(); infile.seekg(0, infile.beg); std::string contents(size, ' '); infile.read(&contents[0], size); return contents; } /** * FUNCTION: writeAllText * USE: Creates a new file, writes the specified string to the file, * and then closes the file. If the target file already exists, it * is overwritten * @param path: The file to write to * @param contents: The string to write to the file * @return: N/A */ void writeAllText(const std::string& path, const std::string& contents) { std::ofstream outfile(path); if (outfile.fail()) { throw std::invalid_argument{ "Unable to write file at path: '" + path + "'" }; } outfile << contents; } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 9, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare path std::string path = "C:\\Users\\Name\\Desktop\\text.txt"; // Declare file contents std::string contents = "Kenneth, Jennifer, Lynn, Sole"; // Save contents to the specified path Utils::writeAllText(path, contents); // Read the contents of the file at the specified path std::string contents2 = Utils::readAllText(path); // Display contents display(contents2); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Get A List Of Files At A Given Path Directory Using C++
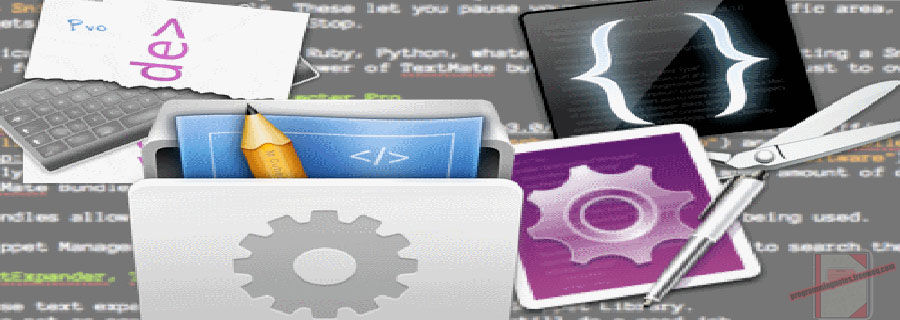
The following is a module with functions which demonstrates how to get a list of files at a given directory path using C++.
The function demonstrated on this page returns a list of std::filesystem::directory_entry, which contains information about the files in the given directory.
1. Get Files In Directory
The example below demonstrates the use of ‘Utils::getFilesInDirectory‘ to get a list of files at a given path directory.
The optional function parameter lets you specify the search option. Set the search option to True to limit the search to just the current directory. Set the search option to False to expand the search to the current directory and all subdirectories when searching for files.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Get Files In Directory // Declare path std::string directory = "C:\\Users\\Name\\Desktop"; // Get files at the specified directory auto files = Utils::getFilesInDirectory(directory); // Display info about the files for (const auto& file : files) { std::cout << "File: " << file.path().u8string() << std::endl; } // example output: /* File: C:\Users\Name\Desktop\text.txt File: C:\Users\Name\Desktop\image.png File: C:\Users\Name\Desktop\document.docx */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <filesystem> namespace Utils { /** * FUNCTION: getFilesInDirectory * USE: Returns a 'directory_entry' list of the files in the given directory * @param directory: The relative or absolute path to the directory to search * @param topDirectoryOnly: If True, limits the search to just the current * directory. If False, expands the search to the current directory * and all subdirectories * @return: A list of 'directory_entry' in the given directory */ auto getFilesInDirectory(const std::string& directory, bool topDirectoryOnly = true) { namespace fs = std::filesystem; std::vector<fs::directory_entry> results; auto fill = [&results](const auto& iter) { for (const fs::directory_entry& entry : iter) { if (!entry.is_regular_file()) { continue; } results.push_back(entry); } }; if (topDirectoryOnly) { fill(fs::directory_iterator(directory)); } else { fill(fs::recursive_directory_iterator(directory)); } return results; } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare path std::string directory = "C:\\Users\\Name\\Desktop"; // Get files at the specified directory auto files = Utils::getFilesInDirectory(directory); // Display info about the files for (const auto& file : files) { std::cout << "File: " << file.path().u8string() << std::endl; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Make Map & Unordered Map Keys Case Insensitive Using C++
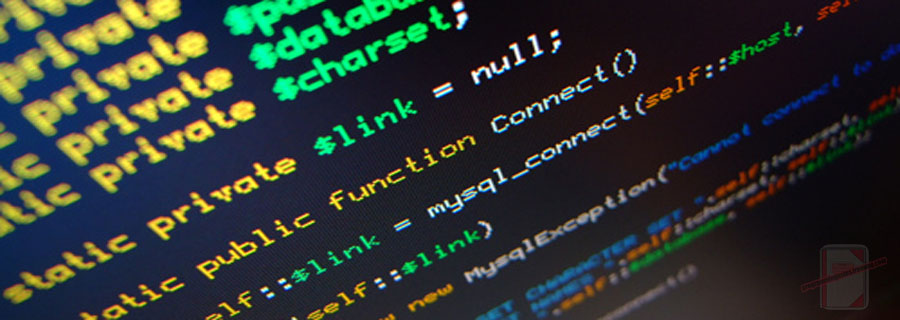
The following is a module with functions which demonstrates how to make map and unordered_map keys case insensitive using C++.
1. Case Insensitive – Map
The example below demonstrates how to make a map with a string key case insensitive.
To do this, the comparison function needs to be overridden.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// Case Insensitive - Map #include <string> #include <map> // Makes map keys case insensitive struct case_insensitive_map { struct comp { bool operator() (const std::string& lhs, const std::string& rhs) const { // On non Windows OS, use the function "strcasecmp" in #include <strings.h> return _stricmp(lhs.c_str(), rhs.c_str()) < 0; } }; }; // Declare map std::map<std::string, unsigned, case_insensitive_map::comp> map = { {"KENNetH", 2019}, {"Jennifer", 2010}, {"Lynn", 28}, {"SOLe", 31} }; // Display results std::cout << map["kenneth"] << ", " << map["JeNniFer"] << ", " << map["LYNN"] << ", " << map["sole"]; // expected output: /* 2019, 2010, 28, 31 */ |
2. Case Insensitive – Unordered Map
The example below demonstrates how to make an unordered map with a string key case insensitive.
To do this, the comparison and the hash function needs to be overridden.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// Case Insensitive - Unordered Map #include <string> #include <unordered_map> #include <utility> #include <cctype> // Makes unordered map keys case insensitive struct case_insensitive_unordered_map { struct comp { bool operator() (const std::string& lhs, const std::string& rhs) const { // On non Windows OS, use the function "strcasecmp" in #include <strings.h> return _stricmp(lhs.c_str(), rhs.c_str()) == 0; } }; struct hash { std::size_t operator() (std::string str) const { for (std::size_t index = 0; index < str.size(); ++index) { auto ch = static_cast<unsigned char>(str[index]); str[index] = static_cast<unsigned char>(std::tolower(ch)); } return std::hash<std::string>{}(str); } }; }; // Declare unordered map std::unordered_map<std::string, unsigned , case_insensitive_unordered_map::hash , case_insensitive_unordered_map::comp> unorderedMap = { {"KENNetH", 2019}, {"Jennifer", 2010}, {"Lynn", 28}, {"SOLe", 31} }; // Display results std::cout << unorderedMap["kenneth"] << ", " << unorderedMap["JeNniFer"] << ", " << unorderedMap["LYNN"] << ", " << unorderedMap["sole"]; // expected output: /* 2019, 2010, 28, 31 */ |
3. Full Examples
Below is a full example demonstrating the concepts on this page.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates case insensitive maps. // ============================================================================ #include <iostream> #include <string> #include <exception> #include <map> #include <unordered_map> #include <utility> #include <cctype> void display(const std::string& message); // Makes map keys case insensitive struct case_insensitive_map { struct comp { bool operator() (const std::string& lhs, const std::string& rhs) const { // On non Windows OS, use the function "strcasecmp" in #include <strings.h> return _stricmp(lhs.c_str(), rhs.c_str()) < 0; } }; }; // Makes unordered map keys case insensitive struct case_insensitive_unordered_map { struct comp { bool operator() (const std::string& lhs, const std::string& rhs) const { // On non Windows OS, use the function "strcasecmp" in #include <strings.h> return _stricmp(lhs.c_str(), rhs.c_str()) == 0; } }; struct hash { std::size_t operator() (std::string str) const { for (std::size_t index = 0; index < str.size(); ++index) { auto ch = static_cast<unsigned char>(str[index]); str[index] = static_cast<unsigned char>(std::tolower(ch)); } return std::hash<std::string>{}(str); } }; }; int main() { try { // Declare map std::map<std::string, unsigned, case_insensitive_map::comp> map = { {"KENNetH", 2019}, {"Jennifer", 2010}, {"Lynn", 28}, {"SOLe", 31} }; // Display results std::cout << map["kenneth"] << ", " << map["JeNniFer"] << ", " << map["LYNN"] << ", " << map["sole"]; display(""); // Declare unordered map std::unordered_map<std::string, unsigned , case_insensitive_unordered_map::hash , case_insensitive_unordered_map::comp> unorderedMap = { {"KENNetH", 2019}, {"Jennifer", 2010}, {"Lynn", 28}, {"SOLe", 31} }; // Display results std::cout << unorderedMap["kenneth"] << ", " << unorderedMap["JeNniFer"] << ", " << unorderedMap["LYNN"] << ", " << unorderedMap["sole"]; } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Search For & Find A Specific Element In A Vector Using C++
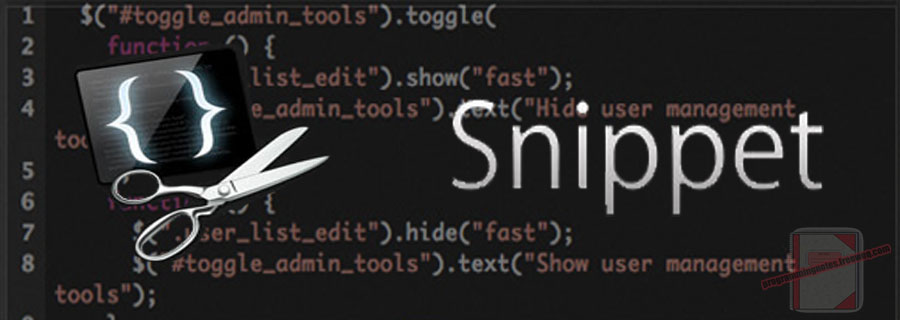
The following is a module with functions which demonstrates how to search for and find a specific element in a vector using C++.
The function demonstrated on this page is a template, so it should work on vectors of any type. It also uses a predicate to determine the item to select.
1. Find
The example below demonstrates the use of ‘Utils::find‘ to find the first element in a sequence of values based on a predicate.
The predicate determines the item to find.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Find // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate = [](const auto& x) { return x.size() >= 8; }; // Get the result auto name = Utils::find(names, predicate); // Display the result std::cout << "Name: " << (*name); // expected output: /* Name: Jennifer */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <algorithm> #include <iterator> namespace Utils { /** * FUNCTION: find * USE: Returns the first element in the given range [first, last) based on a predicate * @param first: The first position of the sequence * @param last: The last position of the sequence * @param predicate: A function to test each element for a condition * @return: A Pointer to the first element that satisfies the predicate, nullptr otherwise */ template<typename RandomIt, typename T = typename std::iterator_traits<RandomIt>::value_type, typename Pred> T* find(RandomIt first, RandomIt last, const Pred& predicate) { T* result = nullptr; auto iter = std::find_if(first, last, predicate); if (iter != last) { result = &(*iter); } return result; } /** * FUNCTION: find * USE: Returns the first element in the collection based on a predicate * @param source: The collection to find items * @param predicate: A function to test each element for a condition * @return: A Pointer to the first element that satisfies the predicate, nullptr otherwise */ template<typename T, typename Pred> T* find(std::vector<T>& source, const Pred& predicate) { return find<typename std::vector<T>::iterator, T, Pred>(source.begin(), source.end(), predicate); } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate = [](const auto& x) { return x.size() >= 8; }; // Get the result auto name = Utils::find(names, predicate); // Display the result display("Name: " + (*name)); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Filter & Select Items In An Array/Vector/Container & Get The Results Using C++
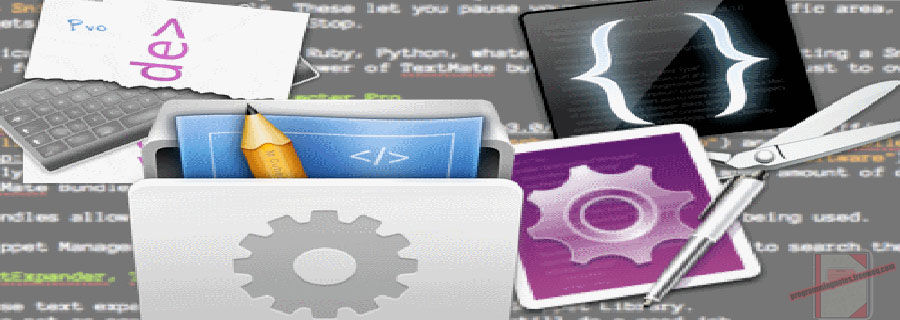
The following is a module with functions which demonstrates how to filter and select items in an array/vector/container, and get the results using C++.
The function demonstrated on this page is a template, so it should work on containers of any type. It also uses a predicate to determine the items to select.
1. Filter – Integer Array
The example below demonstrates the use of ‘Utils::filter‘ to filter an integer array based on a predicate and return its results.
The predicate determines the items to filter and select.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Filter - Integer Array // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Declare predicate auto predicate = [](const auto& x) { return x == 1987 || x == 1991; }; // Get results auto results = Utils::filter(numbers, numbers + size, predicate); // Display the results for (const auto& number : results) { std::cout << "Number: " << number << std::endl; } // expected output: /* Number: 1987 Number: 1991 */ |
2. Filter – String Vector
The example below demonstrates the use of ‘Utils::filter‘ to filter a sequence of values based on a predicate and return its results.
The predicate determines the items to filter and select.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Filter - String Vector // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate = [](const auto& x) { return x == "Kenneth" || x == "Jennifer"; }; // Get the results auto results = Utils::filter(names.begin(), names.end(), predicate); // Display the results for (const auto& name : results) { std::cout << "Name: " << name << std::endl; } // expected output: /* Name: Kenneth Name: Jennifer */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <algorithm> #include <iterator> namespace Utils { /** * FUNCTION: filter * USE: Filters a sequence of values in the given range [first, last) * based on a predicate and returns its results * @param first: The first position of the sequence * @param last: The last position of the sequence * @param predicate: A function to test each element for a condition * @return: A collection that contains elements from the input * sequence that satisfies the condition */ template<typename InputIt, typename T = typename std::iterator_traits<InputIt>::value_type, typename Pred> std::vector<T> filter(InputIt first, InputIt last, const Pred& predicate) { std::vector<T> result; std::copy_if(first, last, std::back_inserter(result), predicate); return result; } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Declare predicate auto predicate = [](const auto& x) { return x == 1987 || x == 1991; }; // Get results auto results = Utils::filter(numbers, numbers + size, predicate); // Display the results for (const auto& number : results) { display("Number: " + std::to_string(number)); } display(""); // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate2 = [](const auto& x) { return x == "Kenneth" || x == "Jennifer"; }; // Get the results auto results2 = Utils::filter(names.begin(), names.end(), predicate2); // Display the results for (const auto& name : results2) { display("Name: " + name); } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Check If An Item Exists In A Vector And Get Its Position Index Using C++
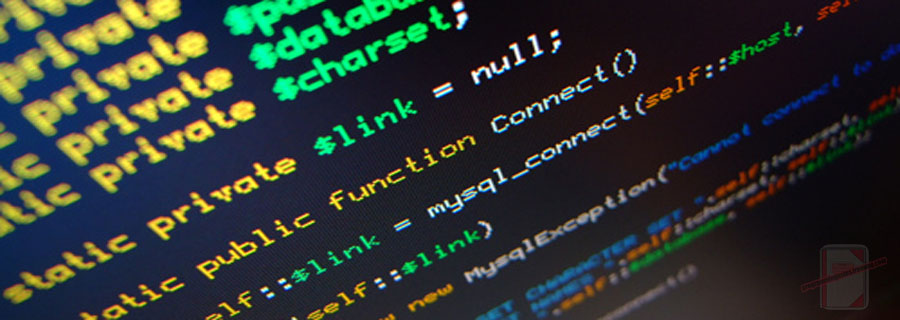
The following is a module with functions which demonstrates how to find the position index of an item in a vector, and make sure the item exists using C++.
The functions demonstrated on this page are wrappers for std::find_if function, and are templates, so they should work on vectors/containers of any type.
1. Contains
The example below demonstrates the use of ‘Utils::contains‘ to determine whether an element is contained in the collection.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Contains // Declare vector std::vector<std::string> names = {"Kenneth", "Jennifer", "Lynn", "Sole"}; // Declare names to search std::string searches[] = {"Lynn", "Jessica"}; // Display results for (const auto& name : searches) { std::cout << "Name: " << name << ", Contains Item: " << (Utils::contains(names, name) ? "True" : "False") << std::endl; } // expected output: /* Name: Lynn, Contains Item: True Name: Jessica, Contains Item: False */ |
2. Index Of
The example below demonstrates the use of ‘Utils::indexOf‘ to get the zero based index of the first occurrence of a search value in the collection.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Index Of // Declare vector std::vector<std::string> names = {"Kenneth", "Jennifer", "Lynn", "Sole"}; // Declare names to search std::string searches[] = {"Lynn", "Jessica"}; // Display results for (const auto& name : searches) { std::cout << "Name: " << name << ", Index: " << Utils::indexOf(names, name) << std::endl; } // expected output: /* Name: Lynn, Index: 2 Name: Jessica, Index: -1 */ |
3. Index Of – Predicate
The example below demonstrates the use of ‘Utils::indexOf‘ to get the zero based index of the first occurrence of a search value in the collection.
In this example, a predicate is used to determine the item to search for. This allows you to customize how an item should be searched.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// Index Of - Predicate // Declare vector std::vector<std::string> names = {"Kenneth", "Jennifer", "Lynn", "Sole"}; // Declare names to search std::string searches[] = {"Lynn", "Jessica"}; // Display results for (const auto& name : searches) { auto pred = [name](const auto& x) { return x == name; }; std::cout << "Name: " << name << ", Index: " << Utils::indexOf(names, pred) << std::endl; } // expected output: /* Name: Lynn, Index: 2 Name: Jessica, Index: -1 */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <algorithm> #include <iterator> namespace Utils { /** * FUNCTION: indexOf * USE: Returns the zero-based index of the first occurrence of a value in * the given range [first, last) * @param first: The first position of the sequence * @param last: The last position of the sequence * @param predicate: A function to test each element for a condition * @return: The zero-based index of the first occurrence, or -1 otherwise */ template<typename InputIt, typename Pred> int indexOf(InputIt first, InputIt last, const Pred& predicate) { auto pos = std::find_if(first, last, predicate); return pos != last ? pos - first : -1; } /** * FUNCTION: indexOf * USE: Returns the zero-based index of the first occurrence of a value * in the collection * @param source: The collection to find items * @param predicate: A function to test each element for a condition * @return: The zero-based index of the first occurrence, or -1 otherwise */ template<typename T, typename Pred> int indexOf(const std::vector<T>& source, const Pred& predicate) { return indexOf(source.begin(), source.end(), predicate); } /** * FUNCTION: indexOf * USE: Returns the zero-based index of the first occurrence of a value * in the collection * @param source: The collection to search * @param search: The item to search for * @return: The zero-based index of the first occurrence, or -1 otherwise */ template<typename T> int indexOf(const std::vector<T>& source, const T& search) { auto pred = [&](const T& x) { return x == search; }; return indexOf(source, pred); } /** * FUNCTION: contains * USE: Determines whether an element is in the given range [first, last) * @param first: The first position of the sequence * @param last: The last position of the sequence * @param predicate: A function to test each element for a condition * @return: True if the item is inside the collection, false otherwise */ template<typename InputIt, typename Pred> bool contains(InputIt first, InputIt last, const Pred& predicate) { return indexOf(first, last, predicate) > -1; } /** * FUNCTION: contains * USE: Determines whether an element is in the collection * @param source: The collection to search * @param predicate: A function to test each element for a condition * @return: True if the item is inside the collection, false otherwise */ template<typename InputIt, typename T = typename std::iterator_traits<InputIt>::value_type, typename Pred> bool contains(const std::vector<T>& source, const Pred& predicate) { return indexOf(source.begin(), source.end(), predicate) > -1; } /** * FUNCTION: contains * USE: Determines whether an element is in the collection * @param source: The collection to search * @param search: The item to search for * @return: True if the item is inside the collection, false otherwise */ template<typename T> bool contains(const std::vector<T>& source, const T& search) { return indexOf(source, search) > -1; } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare vector std::vector<std::string> names = {"Kenneth", "Jennifer", "Lynn", "Sole"}; // Declare names to search std::string searches[] = {"Lynn", "Jessica"}; // Display results for (const auto& name : searches) { display("Name: " + name + ", Contains Item: " + (Utils::contains(names, name) ? "True" : "False")); } // Display results for (const auto& name : searches) { display("Name: " + name + ", Index: " + std::to_string(Utils::indexOf(names, name))); } // Display results for (const auto& name : searches) { auto pred = [name](const auto& x) { return x == name; }; display("Name: " + name + ", Index: " + std::to_string(Utils::indexOf(names, pred))); } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Merge & Concatenate Two Vectors Using C++
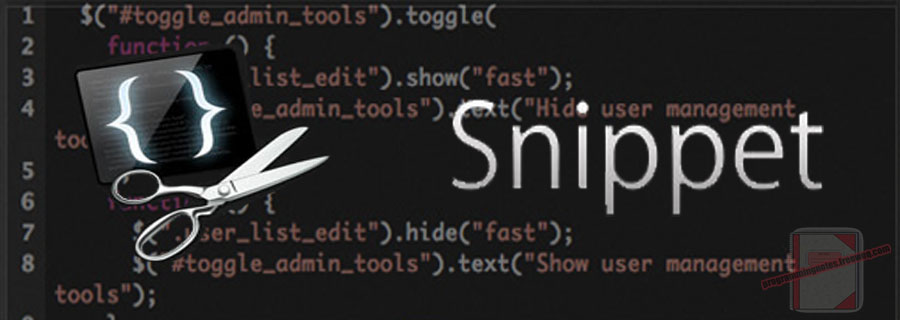
The following is a module with functions which demonstrates how to merge, concatenate and append two vectors using C++.
The function demonstrated on this page is a template, so it should work on vectors of any type.
1. Add Range
The example below demonstrates the use of ‘Utils::addRange‘ add elements to the end of the collection.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// Add Range // Declare vector std::vector<std::string> names = {"Kenneth"}; // Add more names Utils::addRange(names, {"Jennifer", "Lynn", "Sole"}); // Display results for (const auto& name : names) { std::cout << "Name: " + name << std::endl; } // expected output: /* Name: Kenneth Name: Jennifer Name: Lynn Name: Sole */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <iterator> namespace Utils { /** * FUNCTION: addRange * USE: Adds elements to the end of the collection * @param source: The collection that will have items added to it * @param addition: The items that should be added to the end of the collection * @return: N/A */ template<typename T> void addRange(std::vector<T>& source, const std::vector<T>& addition) { source.reserve(source.size() + addition.size()); source.insert(source.end() , std::make_move_iterator(addition.begin()) , std::make_move_iterator(addition.end())); } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare vector std::vector<std::string> names = {"Kenneth"}; // Add more names Utils::addRange(names, {"Jennifer", "Lynn", "Sole"}); // Display results for (const auto& name : names) { display("Name: " + name); } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Replace All Occurrences Of A String With Another String Using C++
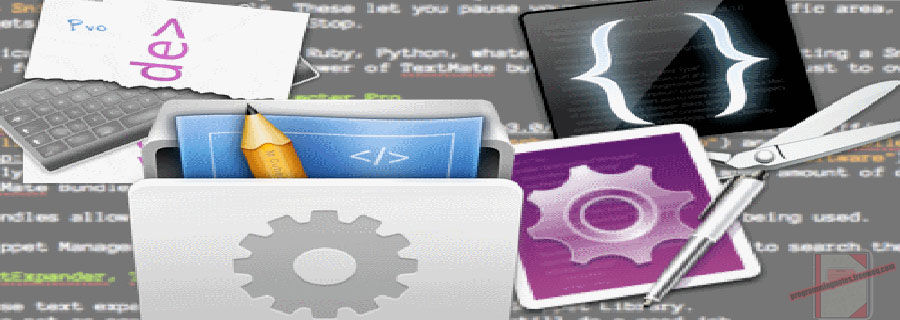
The following is a module with functions which demonstrates how to replace all occurrences of a substring with another substring using C++.
1. Replace All
The example below demonstrates the use of ‘Utils::replaceAll‘ to replace all occurrences of an ‘oldValue’ string with a ‘newValue’ string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Replace All // Declare string std::string numbers = "1987 19 22 2009 2019 1991 28 31"; // Replace whitespace with a comma std::string replaced = Utils::replaceAll(numbers, " ", ", "); // Display the result std::cout << "Original: " << numbers; std::cout << "Replaced: " << replaced; // expected output: /* Original: 1987 19 22 2009 2019 1991 28 31 Replaced: 1987, 19, 22, 2009, 2019, 1991, 28, 31 */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> namespace Utils { /** * FUNCTION: replaceAll * USE: Replaces all occurrences of the 'oldValue' string with the * 'newValue' string * @param source: The source string * @param oldValue: The string to be replaced * @param newValue: The string to replace all occurrences of oldValue * @return: A new string with all occurrences replaced */ std::string replaceAll(const std::string& source , const std::string& oldValue, const std::string& newValue) { if (oldValue.empty()) { return source; } std::string newString; newString.reserve(source.length()); std::size_t lastPos = 0; std::size_t findPos; while (std::string::npos != (findPos = source.find(oldValue, lastPos))) { newString.append(source, lastPos, findPos - lastPos); newString += newValue; lastPos = findPos + oldValue.length(); } newString += source.substr(lastPos); return newString; } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare string std::string numbers = "1987 19 22 2009 2019 1991 28 31"; // Replace whitespace with a comma std::string replaced = Utils::replaceAll(numbers, " ", ", "); // Display the result display("Original: " + numbers); display("Replaced: " + replaced); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Check If A String Is Empty Or Only Contains Whitespace Using C++
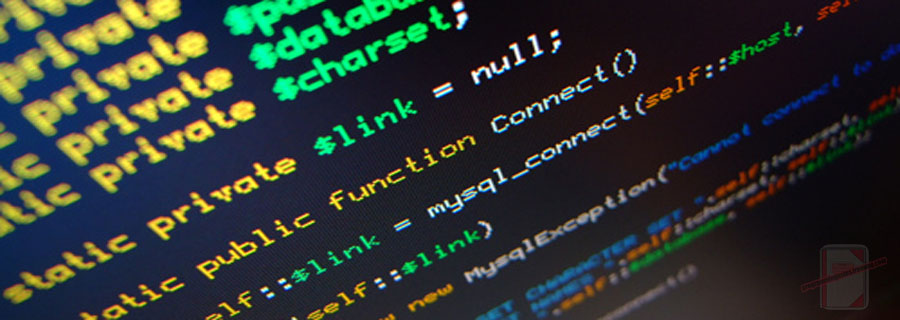
The following is a module with functions which demonstrates how to determine whether a string is empty, or consists only of white-space characters using C++.
1. Is Null Or Whitespace
The example below demonstrates the use of ‘Utils::isNullOrWhitespace‘ to determine whether a string is empty, or consists only of white-space characters.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Is Null Or Whitespace // Declare values std::string words[] = { "Kenneth", "", "Jennifer", " ", "Lynn", "\n\t", "Sole" }; // Check to see which is empty for (auto& word: words) { bool isEmpty = Utils::isNullOrWhitespace(word); std::cout << "Word: " << word << ", Is Empty: " << (isEmpty ? "True" : "False") << std::endl; } // expected output: /* Word: Kenneth, Is Empty: False Word: , Is Empty: True Word: Jennifer, Is Empty: False Word: , Is Empty: True Word: Lynn, Is Empty: False Word: , Is Empty: True Word: Sole, Is Empty: False */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 5, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> #include <cctype> #include <algorithm> namespace Utils { /** * FUNCTION: isNullOrWhitespace * USE: Indicates whether a specified string is empty, or consists only * of whitespace characters * @param str: The source string * @return: True if str is Empty, or consists of only whitespace characters, * False otherwise */ bool isNullOrWhitespace(const std::string& str) { return str.empty() || std::all_of(str.begin(), str.end(), [](char c) { return std::isspace(static_cast<unsigned char>(c)); }); } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 5, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare values std::string words[] = { "Kenneth", "", "Jennifer", " ", "Lynn", "\n\t", "Sole" }; // Check to see which is empty for (auto& word: words) { bool isEmpty = Utils::isNullOrWhitespace(word); display("Word: " + word + ", Is Empty: " + (isEmpty ? "True" : "False")); } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.