Monthly Archives: August 2022
C# || How To Validate A Binary Search Tree Using C#
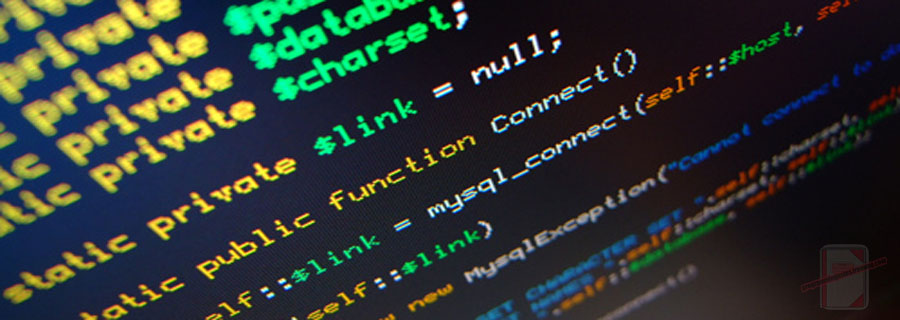
The following is a module with functions which demonstrates how to validate a binary search tree using C#.
1. Is Valid BST – Problem Statement
Given the root of a binary tree, determine if it is a valid binary search tree (BST).
A valid BST is defined as follows:
- The left subtree of a node contains only nodes with keys less than the node’s key.
- The right subtree of a node contains only nodes with keys greater than the node’s key.
- Both the left and right subtrees must also be binary search trees.
Example 1:
Input: root = [2,1,3]
Output: true
Example 2:
Input: root = [5,1,4,null,null,3,6]
Output: false
Explanation: The root node's value is 5 but its right child's value is 4.
2. Is Valid BST – Solution
The following is a solution which demonstrates how to validate a binary search tree.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 12, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to validate a BST // ============================================================================ /** * Definition for a binary tree node. * public class TreeNode { * public int val; * public TreeNode left; * public TreeNode right; * public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) { * this.val = val; * this.left = left; * this.right = right; * } * } */ public class Solution { public bool IsValidBST(TreeNode root) { return Traverse(root, null, null); } private bool Traverse(TreeNode node, TreeNode min, TreeNode max) { if (node == null) { return true; } if (max != null && node.val >= max.val) { return false; } if (min != null && node.val <= min.val) { return false; } return Traverse(node.left, min, node) && Traverse(node.right, node, max); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
true
false