Monthly Archives: January 2012
C++ || Input/Output – Find The Average of The Numbers Contained In a Text File Using an Array/Bubble Sort
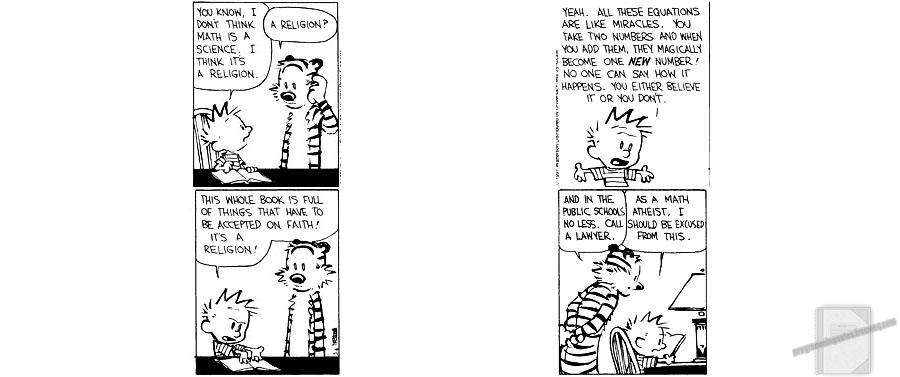
This program highlights more practice using text files and arrays. This program is very similar to one which was previously discussed on this site, but unlike that program, this implementation omits the highest/lowest values which are found within the array via a sort.
The previously discussed program works almost as well as the current implementation, but where it fails is when the data which is being entered into the program contains multiple values of the same type. For example, using the previously discussed method to obtain the average by omitting the highest/lowest entries found within an array, if the array contained the numbers:
1, 2, 3, 3, 3, 2, 2, 1
The previous implementation would mark 1 as being the lowest number (which is correct) and it would mark 3 as being the highest number (which is also correct). The area where it fails is when it omits the highest and lowest scores found within the array. The program will skip over ALL of the numbers contained within the array which equal to 1 and 3, thus resulting in the program obtaining the wrong answer.
To illustrate, before the previous program computes its adjusted average scores, it will not only omit just 1 and 3 from the array, but it will also omit all of the 1’s and 3’s from the list, resulting in our array looking like this:
2, 2, 2
When you are finding the average of a list of numbers by omitting the highest/lowest scores, you don’t want to omit ALL of the values which may equal said numbers, but merely just the highest (last element in the array) and lowest (first element in the array) scores.
So if the previous implementation has subtle issues, why is it on this site? The previous program illustrates very well the process of finding the highest/lowest integers found within an array. It also works flawlessly for data in which there is non repeating values found within a list (i.e 1,2,3,4,5,23,6). So if you know you are reading in from a file in which there are non repeating values, the previous implementation works well. Often times though, developers do not know what type of data the incoming files will contain, so this current implementation is a better way to go, especially if it is not known exactly how many numbers are contained within a file.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Fstream
Ifstream
Ofstream
Working With Files
While Loops
For Loops
Bubble Sort
Basic Math - Finding The Average
The data file that is used in this example can be downloaded here.
Note: In order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 |
#include <iostream> #include <fstream> using namespace std; // function prototype float FindOmittedAverage(int numbers[], int counter); int main() { // declare variables ifstream infile; ofstream outfile; float average=0; float sum =0; int highestNumber = -999999; int lowestNumber = 99999999; int numbers[100]; // open the input file in which you will read data from infile.open("INPUT_Numbers_ programmingnotes_freeweq_com.txt"); if(infile.fail()) { cout<<"nInput file not found!n"; exit(1); // there was an error, program exits } // open the output file in which the compiled data will be saved to outfile.open("OUTPUT_Averages_ programmingnotes_freeweq_com.txt"); if(outfile.fail()) { cout <<"There was an error creating the output file, press enter to terminate program."; exit(1); // there was an error, program exits } // display data to screen via cout cout <<"The numbers countained in the input file are: "; // saves data to the outfile. Notice the declaration to // save data to the outfile is the same as the ^ above cout // statment outfile <<"The numbers countained in the input file are: "; // incoming data from the file will be stored in an int array // so this counter will increment the array index every time the // program finds a new value inside the file to store inside the array int counter =0; // this while loop will read in data from the file until it reaches the end of the file // (i.e until there are no more number found within the file) // this process also stored the numbers inside the "numbers" array while(infile >> numbers[counter]) { // 'sum' variable adds the incoming numbers together // computing the sum sum += numbers[counter]; // finds the highest number contained within the incoming file if( numbers[counter] > highestNumber) { highestNumber = numbers[counter]; } // finds the lowest number contained within the incoming file if (numbers[counter] < lowestNumber) { lowestNumber = numbers[counter]; } // displays the currently found number from the file to stdout cout << numbers[counter] << ", "; // saves the currently found number from the file to the output file outfile << numbers[counter]<< ", "; ++counter; } // always close your file after your done using them infile.close(); // display the highest found number to stdout cout<< "nnThe highest and lowest numbers contained in the file are: nHighest: " << highestNumber<<"nLowest: "<<lowestNumber<<endl; // saves the highest found number to the output file outfile<< "nnThe highest and lowest numbers contained in the file are: nHighest: " << highestNumber<<"nLowest: "<<lowestNumber<<endl; // using the sum found from the above while loop, we compute the average average = sum/(counter); // display/save the average of the numbers which were contained in the // file to stdout and the output file cout<<"nThe average of the "<<counter<<" numbers contained in the file is: "<<average<<endl; outfile<<"nThe average of the "<<counter<<" numbers contained in the file is: "<<average<<endl; // function declaration which finds the omitted average of the found numbers // from the file average = FindOmittedAverage(numbers,counter); // display/save the omitted average of the numbers which were contained in the // file to stdout and the output file cout<<"nThe average of the "<<counter<<" numbers contained in the file omitting the highest and lowest scores is: "<<average<<endl; outfile<<"nThe average of the "<<counter<<" numbers contained in the file omitting the highest and lowest scores is: "<<average<<endl; // closes the outfile once we are done using it outfile.close(); return 0; } // function takes in the 'numbers' array, and 'counter' variable from // the main function as parameters, and computes the average, omitting // the highest and lowest numbers found within the array float FindOmittedAverage(int numbers[], int counter) { float sum = 0; float avg = 0; // this is a 'bubble sort' which will sort the numbers // contained within the array, from lowest to the highest // i.e (1,2,3,4,5,6,7,8) for(int iteration = 1; iteration < counter; iteration++) { for(int index = 0; index < counter - iteration; index++) { // if the previous value in the array is bigger than the next, then swap them if(numbers[index]> numbers[index+1]) { int temp = numbers[index]; numbers[index] = numbers[index+1]; numbers[index+1]= temp; } } }// end of sort // after the sorting is complete, set the highest // and lowest elements in the array to zero numbers[0]=0; numbers[counter-1]=0; // find the sum of the newly sorted array // with the highest and lowest entries being deleted (set to zero) for(int i = 0; i < counter; i++) { sum += numbers[i]; } // compute the average avg = sum / (counter-2); // return the average back to main return avg; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Remember to include the input file)
The numbers countained in the input file are: 12, 45, 23, 46, 11, -5, 23, 33, 50, 17, 13, 25, 15, 50,
The highest and lowest numbers contained in the file are:
Highest: 50
Lowest: -5The average of the 14 numbers contained in the file is: 25.5714
The average of the 14 numbers contained in the file omitting the highest and lowest scores is: 26.0833
C++ || Random Number Guessing Game Using Srand, Rand, & Do/While Loop
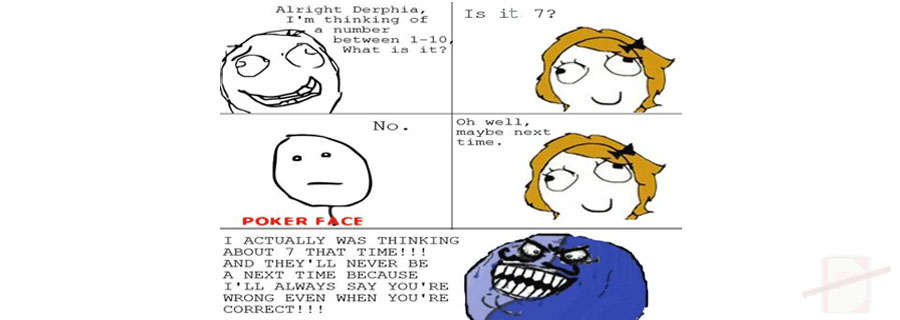
This is a simple guessing game, which demonstrates the use of srand and rand to generate random numbers. This program first prompts the user to enter a number between 1 and 1000. Using if/else statements, the program will then check to see if the user inputted number is higher/lower than the pre defined random number which is generated by the program. If the user makes a wrong guess, the program will re prompt the user to enter in a new number, where they will have a chance to enter in a new guess. Once the user finally guesses the correct answer, using a do/while loop, the program will ask if they want to play again. If the user selects yes, the game will start over, and a new random number will be generated. If the user selects no, the game will end.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 20, 2012 // Taken From: http://programmingnotes.org/ // File: guessingGame.cpp // Description: Demonstrates a simple random number guessing game // ============================================================================ #include <iostream> #include <ctime> #include <iomanip> using namespace std; int main() { // declare & initialize variables char playAgain = 'y'; int userInput = 0; int numGuesses = 0; // Seed the random number generator with the current time so // the numbers will be different every time the program runs srand(time(NULL)); int randomNumber = rand() % 1000+1; // display directions to user cout << "I'm thinking of a number between 1 and 1000. Go ahead and make your first guess.\n\n"; do{ // this is the start of the do/while loop // get data from user cin >> userInput; // increments the 'numGuesses' variable each time the user // gets the guess wrong ++numGuesses; // if user guess is too high, do this code if(userInput > randomNumber) { cout << "Too high! Think lower.\n"; } // if user guess is too low, do this code else if(userInput < randomNumber) { cout << "Too low! Think higher.\n"; } // if user guess is correct, do this code else { // display data to user, prompt if user wants to play again cout << "You got it, and it only took you " << numGuesses <<" trys!\nWould you like to play again (y/n)? "; cin >> playAgain; // if user wants to play again then re initialize the variables if(playAgain == 'y'|| playAgain =='Y') { // creates a line seperator if user wants to enter new data cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; numGuesses = 0; cout << "\n\nMake a guess (between 1-1000):\n\n"; // generate a new random number for the user to try & guess randomNumber = rand() % 1000+1; } } }while(playAgain =='y' || playAgain =='Y'); // ^ do/while loop ends when user doesnt select 'Y' // display data to user cout<<"\n\nThanks for playing!!"<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
I'm thinking of a number between 1 and 1000. Go ahead and make your first guess.
67
Too low! Think higher.
500
Too low! Think higher.
700
Too high! Think lower.
600
Too low! Think higher.
680
Too high! Think lower.
650
Too low! Think higher.
660
Too low! Think higher.
670
You got it, and it only took you 8 trys!
Would you like to play again (y/n)? y------------------------------------------------------------
Make a guess (between 1-1000):
500
Too low! Think higher.
600
Too low! Think higher.
700
Too low! Think higher.
900
Too high! Think lower.
800
Too high! Think lower.
760
Too high! Think lower.
740
Too high! Think lower.
720
Too high! Think lower.
700
Too low! Think higher.
710
Too high! Think lower.
705
Too high! Think lower.
701
Too low! Think higher.
702
Too low! Think higher.
703
Too low! Think higher.
704
You got it, and it only took you 15 trys!
Would you like to play again (y/n)? nThanks for playing!!
C++ || Struct – Add One Day To Today’s Date Using A Struct
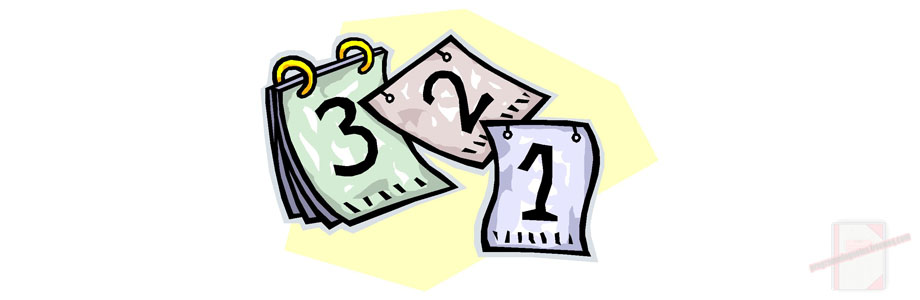
This program displays more practice using the structure data type, and is very similar to another program which was previously discussed here.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Functions
Passing a Value By Reference
Integer Arrays
Structures
Constant Variables
Boolean Expressions
This program utilizes a struct, which is very similar to the class concept. This program first prompts the user to enter the current date in mm/dd/yyyy format. Upon obtaining the date from the user, the program then uses a struct implementation to simply add one day to the date which was entered by the user. If the day that was entered into the program by the user falls on the end of the month, the program will”roll over” the incremented date into the next month. If the user enters 12/31/2012, the program will “roll over” the incremented date into the next calendar year.
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 |
#include <iostream> using namespace std; // struct declaration struct Date { int month; int day; int year; }; // constants which do not change // specifies the max values for each variable const int MAX_DAYS = 31; const int MAX_MONTHS = 12; // function prototypes bool IsDateValid(Date dateVal); void CalcNextDay(Date &dateVal); int main() { // initialize the structure: month, day, year Date dateVal = {1,18,2012}; char backSlash = '/'; // this is a 'placeholder' variable // obtain information from user cout << "Please enter today's date in mm/dd/yyyy format: "; cin >> dateVal.month >> backSlash >> dateVal.day >> backSlash >> dateVal.year; // Checks to see if user entered valid data if (IsDateValid(dateVal) == false) { cout << "Invalid input...nProgram exiting...." << endl; exit(EXIT_FAILURE); } // function declaration which calculates the next day CalcNextDay(dateVal); // display updated data to user cout << "The next day is " << dateVal.month << "/" << dateVal.day << "/" << dateVal.year <<endl; return 0; }// end of main // this function checks to see if the user inputted valid data bool IsDateValid(Date dateVal) { // checks to see if user inputted data falls between the specified // const variables as declared above if ((dateVal.day >= 1 && dateVal.day <= MAX_DAYS) &&(dateVal.month >= 1 && dateVal.month <= MAX_MONTHS)) { return true; } else { return false; } }// end of IsDateValid // this calculates the next day & updates values via reference void CalcNextDay(Date &dateVal) { // int array which holds the max date each month has // from January to December int maxDays[12]={31,28,31,30,31,30,31,31,30,31,30,31}; // bool which lets the program know if the month the // user entered is a max month for the specific month // the user inputted bool maxDay = false; // checks to see if user inputted day is a max date from the // above array for the currently selected month which // was entered by the user if(dateVal.day == maxDays[dateVal.month-1]) { dateVal.day = 1; ++dateVal.month; maxDay = true; } // checks to see if user inputted a valid date // for the currently selected month else if (dateVal.day > maxDays[dateVal.month-1]) { cout << "Invalid day input - There is no such date for the selected month.nProgram exiting...." << endl; exit(EXIT_FAILURE); } // if user didnt enter a max date, and the date is valid, increment the day else { ++dateVal.day; } // if the date is 12/31/yyyy // increment to the next year if ((dateVal.month > 12) && maxDay == true) { dateVal.month = 1; ++dateVal.year; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 6 separate times to display the different outputs its able to produce
Please enter today's date in mm/dd/yyyy format: 1/18/2012
The next day is 1/19/2012
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 7/31/2012
The next day is 8/1/2012
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 2/28/2012
The next day is 3/1/2012
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 13/5/2012
Invalid input...
Program exiting....
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 2/31/2012
Invalid day input - There is no such date for the selected month.
Program exiting....
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 12/31/2012
The next day is 1/1/2013
C++ || Searching An Integer Array For A Target Value
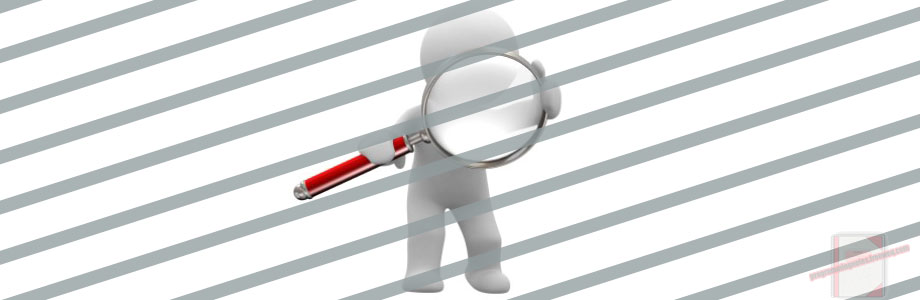
Here is another actual homework assignment which was presented in an intro to programming class.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
This is a small and simple program which demonstrates how to search for a target value that is stored in an integer array. This program prompts the user to enter five values into an int array. After the user has entered all the values, it displays a prompt asking the user for a search value. Once it has the search value, the program will search through the array looking for the target; wherever the value is found, it will display the index location. After it displays all the locations where the value is found, it will display the total number of occurrences the search value was found within the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // File: ArraySearch.cpp // ============================================================================ // // Description: // This program allows the user to populate an array of integers with five // values. After the user has entered all the values, it displays a prompt // asking the user for a search value. Once it has the search value, the // program will search through the array looking for the target; wherever the // value is found, it will display the index location. After it displays all // the locations where the value is found, it will display the total number // of occurrences the search value was found within the array. // // ============================================================================ #include <iostream> using namespace std; // constant variable const int NUM_INTS = 5; // function prototypes int SearchArray(int numValues[], int searchValue); // ==== main ================================================================== // // ============================================================================ int main() { // declare variables int searchValue; int numOccurences = 0; int numValues[NUM_INTS]; // int array size is initialized with a const value // get data from user via a 'for loop' cout << "Please enter "<< NUM_INTS <<" integer values:nn"; for (int index=0; index < NUM_INTS; ++index) { cout << "#" << index + 1 << ": "; cin >> numValues[index]; cout <<endl; } // get a search value from the user cout << "Please enter a search value: "; cin >> searchValue; cout <<endl; // finds the number of occurences the search value was found in the array numOccurences = SearchArray(numValues, searchValue); // display data to user cout << "nThe total occurrences of value " << searchValue << " within the array is: " << numOccurences; cout << endl << endl; return 0; }// end of main // ==== SearchArray =========================================================== // // This function will take as input the array, the number of array // elements, and the target value to search for. The function will traverse // the array looking for the target value, and when it finds it, display the // index location within the array. // // Input: // limit [IN] -- the array, the number of array // elements, and the target value // // Output: // The total number of occurrences of the target in the array // // ============================================================================ int SearchArray(int numValues[], int searchValue) { int numFound=0; for (int index=0; index < NUM_INTS; ++index) { if (numValues[index] == searchValue) { cout << "t" << searchValue << " was found at array index #" << index << endl; ++numFound; // if the search values was found, // increment the variable by 1 } } return numFound; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 3 separate times to display the different outputs its able to produce
Please enter 5 integer values:
#1: 12
#2: 12
#3: 12
#4: 12
#5: 12
Please enter a search value: 1212 was found at array index #0
12 was found at array index #1
12 was found at array index #2
12 was found at array index #3
12 was found at array index #4The total occurrences of value 12 within the array is: 5
-------------------------------------------------------------------------Please enter 5 integer values:
#1: 12
#2: 87
#3: 45
#4: 87
#5: 33
Please enter a search value: 8787 was found at array index #1
87 was found at array index #3The total occurrences of value 87 within the array is: 2
-------------------------------------------------------------------------Please enter 5 interger values:
#1: 54
#2: 67
#3: 98
#4: 45
#5: 98
Please enter a search value: 123The total occurrences of value 123 within the array is: 0
C++ || Find The Day Of The Week You Were Born Using Functions, String, Modulus, If/Else, & Switch
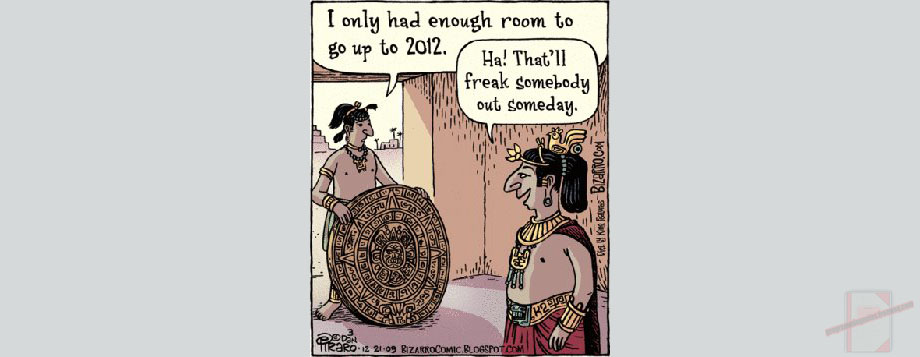
This program displays more practice using functions, modulus, if and switch statements.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Functions
Strings
Modulus
If/Else Statements
Switch Statements
Knowledge of Leap Years
A Calendar
This program prompts the user for their name, date of birth (month, day, year), and then displays information back to them via cout. Once the program obtains selected information from the user, it will use simple math to determine the day of the week in which the user was born, and determine the day of the week their current birthday will be for the current calendar year. The program will also display to the user their current age, along with re-displaying their name back to them.
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 |
#include <iostream> #include <string> using namespace std; // a const variable containing the current year const int CURRENT_YEAR = 2012; // function prototypes string GetNameOfMonth(int numOfMonth); int GetLastTwoDigits(int fourDigitYear); string GetDayOfWeek(int numOfMonth, int lastTwoDigits, int numOfDay, int fourDigitYear); int main () { // declare variables string nameOfMonth = " ", dayOfWeek = " ", userName = " ", dayOfWeekCurrentYear = " " ; int numOfMonth=0, numOfDay=0, fourDigitYear=0, lastTwoDigits=0, lastTwoDigitsCurrentYear; // user inputs data cout << "Please enter your name: "; cin >> userName; cout << "nPlease enter the month in which you were born (between 1 and 12): "; cin >> numOfMonth; // converts the number the user inputted from above ^ // into a literal month name (i.e month #1 = January) nameOfMonth = GetNameOfMonth(numOfMonth); // user inputs data cout << "nPlease enter the day you were born (between 1 and 31): "; cin >> numOfDay; // checks to see if user entered valid data if (numOfDay < 1 || numOfDay > 31) { cout << "nYou have entered an invalid number of day. " << "Please enter a valid number." << endl << endl; exit(1); } // user inputs data cout << "nPlease enter the year you were born (between 1900 and 2099): "; cin >> fourDigitYear; cout << endl << endl; // checks to see if user entered valid data if (fourDigitYear < 1900 || fourDigitYear > 2099) { cout << "You have entered an invalid year. " << "Please enter a valid year." << endl << endl; exit(1); } // gets the las two digits in which the user was born // (i.e 1999: last two digits = 99) lastTwoDigits = GetLastTwoDigits(fourDigitYear); // gets the las two digits of the current year // (i.e 2012: last two digits = 12) lastTwoDigitsCurrentYear = GetLastTwoDigits(CURRENT_YEAR); // finds the day of the week in which the user was born dayOfWeek = GetDayOfWeek(numOfMonth, lastTwoDigits, numOfDay, fourDigitYear); // finds the day of the week the users current birthday will be this year dayOfWeekCurrentYear = GetDayOfWeek(numOfMonth, lastTwoDigitsCurrentYear, numOfDay, CURRENT_YEAR); // display data to user cout << "Hello " << userName <<". Here are some facts about you!" <<endl; cout << "You were born "<< nameOfMonth << numOfDay << " "<< fourDigitYear << endl; cout << "Your birth took place on a " << dayOfWeek << endl; cout << "This year your birthday will take place on a " << dayOfWeekCurrentYear << endl; cout << "You currently are, or will be " << (CURRENT_YEAR - fourDigitYear) << " years old this year!"<<endl; return 0; }// end of main // converts the number the user inputted from above ^ // into a literal month name (i.e month #1 = January) string GetNameOfMonth(int numOfMonth) { string nameOfMonth = " "; // a switch determining what month the user was born switch(numOfMonth) { case 1: nameOfMonth = "January "; break; case 2: nameOfMonth = "February "; break; case 3: nameOfMonth = "March "; break; case 4: nameOfMonth = "April "; break; case 5: nameOfMonth = "May "; break; case 6: nameOfMonth = "June "; break; case 7: nameOfMonth = "July "; break; case 8: nameOfMonth = "August "; break; case 9: nameOfMonth = "September "; break; case 10: nameOfMonth = "October "; break; case 11: nameOfMonth = "November "; break; case 12: nameOfMonth = "December "; break; default: cout << "You have entered an invalid number of month. nPlease enter a valid number.n" << "Program Terminating.."; exit(1); break; }// end of switch return nameOfMonth ; }// end of GetNamOfMonth // gets the las two digits in which the user was born/current year int GetLastTwoDigits(int fourDigitYear) { if (fourDigitYear >= 1900 && fourDigitYear <= 1999) { return (fourDigitYear - 1900); } else { return (fourDigitYear - 2000); } }// end of GetLastTwoDigits // finds the day of the week in which the user was born/current year string GetDayOfWeek(int numOfMonth, int lastTwoDigits, int numOfDay, int fourDigitYear) { string dayOfWeek = " "; int Total = 0, valueOfMonth = 0; if (numOfMonth == 1) valueOfMonth = 1; else if (numOfMonth == 2) valueOfMonth = 4; else if (numOfMonth == 3) valueOfMonth = 4; else if (numOfMonth == 4) valueOfMonth = 0; else if (numOfMonth == 5) valueOfMonth = 2; else if (numOfMonth == 6) valueOfMonth = 5; else if (numOfMonth == 7) valueOfMonth = 0; else if (numOfMonth == 8) valueOfMonth = 3; else if (numOfMonth == 9) valueOfMonth = 6; else if (numOfMonth == 10) valueOfMonth = 1; else if (numOfMonth == 11) valueOfMonth = 4; else if (numOfMonth == 12) valueOfMonth = 6; else cout <<"nError"; Total = lastTwoDigits / 4; Total += lastTwoDigits; Total += numOfDay; Total += valueOfMonth; // uses the 'Total' variable from above to determine the day // of the week in which you were born if ((fourDigitYear > 2000)&&((numOfMonth != 1)&&(numOfMonth != 2))&&(fourDigitYear != 2004)) { Total = Total + 6; } else if ((fourDigitYear < 2000) && ((numOfMonth == 1) || (numOfMonth == 2))) { Total = Total + 7; } else if ((fourDigitYear == 2000) && ((numOfMonth == 1) || (numOfMonth == 2))) { Total = Total -2; } else if ((fourDigitYear == 2004||fourDigitYear == 2000)&&((numOfMonth != 1)&&(numOfMonth != 2))) { Total = Total -1; } else if ((fourDigitYear % 4 == 0) && (fourDigitYear % 100 == lastTwoDigits) && (fourDigitYear % 400 == lastTwoDigits)) { Total = Total-2; } else if ((fourDigitYear > 2000)&&((numOfMonth == 1)||(numOfMonth == 2))&&(fourDigitYear != 2004)) { Total = Total + 6; } // uses the 'Total' variable from above to determine the day // of the week in which you were born/when you birthday will be this year if (Total % 7 == 1) dayOfWeek = "Sunday"; else if (Total % 7 == 2) dayOfWeek = "Monday"; else if (Total % 7 == 3) dayOfWeek = "Tuesday"; else if (Total % 7 == 4) dayOfWeek = "Wednesday"; else if (Total % 7 == 5) dayOfWeek = "Thursday"; else if (Total % 7 == 6) dayOfWeek = "Friday"; else if (Total % 7 == 0) dayOfWeek = "Saturday"; else cout<< "nError"; return dayOfWeek; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled five separate times to display the different outputs its able to produce
Please enter your name: MyProgrammingNotes
Please enter the month in which you were born (between 1 and 12): 1
Please enter the day you were born (between 1 and 31): 1
Please enter the year you were born (between 1900 and 2099): 2012Hello MyProgrammingNotes. Here are some facts about you!
You were born January 1 2012
Your birth took place on a Sunday
This year your birthday will take place on a Sunday
You currently are, or will be 0 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 4
Please enter the day you were born (between 1 and 31): 2
Please enter the year you were born (between 1900 and 2099): 1957Hello Name. Here are some facts about you!
You were born April 2 1957
Your birth took place on a Tuesday
This year your birthday will take place on a Monday
You currently are, or will be 55 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 5
Please enter the day you were born (between 1 and 31): 7
Please enter the year you were born (between 1900 and 2099): 1999Hello Name. Here are some facts about you!
You were born May 7 1999
Your birth took place on a Friday
This year your birthday will take place on a Monday
You currently are, or will be 13 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 8
Please enter the day you were born (between 1 and 31): 4
Please enter the year you were born (between 1900 and 2099): 1983Hello Name. Here are some facts about you!
You were born August 4 1983
Your birth took place on a Thursday
This year your birthday will take place on a Saturday
You currently are, or will be 29 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 6
Please enter the day you were born (between 1 and 31): 7
Please enter the year you were born (between 1900 and 2099): 2987You have entered an invalid year. Please enter a valid year.
Press any key to continue . . .
C++ || Find The Prime, Perfect & All Known Divisors Of A Number Using A For, While & Do/While Loop
This program was designed to better understand how to use different loops which are available in C++.
This program first asks the user to enter a non negative number. After it obtains a non negative integer from the user, the program will determine if the inputted number is a prime number or not, aswell as determine if the user inputted number is a perfect number or not. After it obtains its results, the program will display to the screen if the user inputted number is prime/perfect number or not. The program will also display a list of all the possible divisors of the user inputted number via cout.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Do/While Loop
While Loop
For Loop
Modulus
Basic Math - Prime Numbers
Basic Math - Perfect Numbers
Basic Math - Divisors
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
#include <iostream> #include <iomanip> using namespace std; int main () { int userInput = 0; int divisor = 0; int sumOfDivisors = 0; char response ='n'; do{ // this is the start of the do/while loop cout<<"Enter a number: "; cin >> userInput; // if the user inputs a negative number, do this code while(userInput < 0) { cout<<"ntaSorry, but the number entered is less than the allowable limit. Please try again"; cout<<"nnEnter an number: "; cin >> userInput; } cout << "nInput number: " << userInput; // for loop adds sum of all possible divisors for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0) { // this will repeatedly add the found divisors together sumOfDivisors += counter; } } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is prime if(userInput == (sumOfDivisors - 1)) { cout<<endl; cout << userInput << " is a prime number."; } else { cout<<endl; cout << userInput << " is not a prime number."; } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is a perfect number if (userInput == (sumOfDivisors - userInput)) { cout<<endl; cout << userInput << " is a perfect number."; } else { cout<<endl; cout << userInput << " is not a perfect number."; } cout << "nDivisors of " << userInput << " are: "; // for loop lists all the possible divisors for the // 'userInput' variable by using the modulus operator for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0 && counter !=userInput) { cout << counter << ", "; } } cout << "and "<< userInput; // asks user if they want to enter new data cout << "nntDo you want to input another number?(Y/N): "; cin >> response; // creates a line seperator if user wants to enter new data cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // resets variable back to zero if the user wants to enter new data sumOfDivisors=0; }while(response =='y' || response =='Y'); // ^ End of the do/while loop. As long as the user chooses // 'Y' the loop will keep going. // It stops when the user chooses the letter 'N' return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Enter a number: 8128
Input number: 8128
8128 is not a prime number.
8128 is a perfect number.
Divisors of 8128 are: 1, 2, 4, 8, 16, 32, 64, 127, 254, 508, 1016, 2032, 4064, and 8128Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 6Input number: 6
6 is not a prime number.
6 is a perfect number.
Divisors of 6 are: 1, 2, 3, and 6Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 241Input number: 241
241 is a prime number.
241 is not a perfect number.
Divisors of 241 are: 1, and 241Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 2012Input number: 2012
2012 is not a prime number.
2012 is not a perfect number.
Divisors of 2012 are: 1, 2, 4, 503, 1006, and 2012Do you want to input another number?(Y/N): n
------------------------------------------------------------
Press any key to continue . . .
C++ || Whats My Name? – Using a Char Array, Strcpy, Strcat, Strcmp, & Strlen
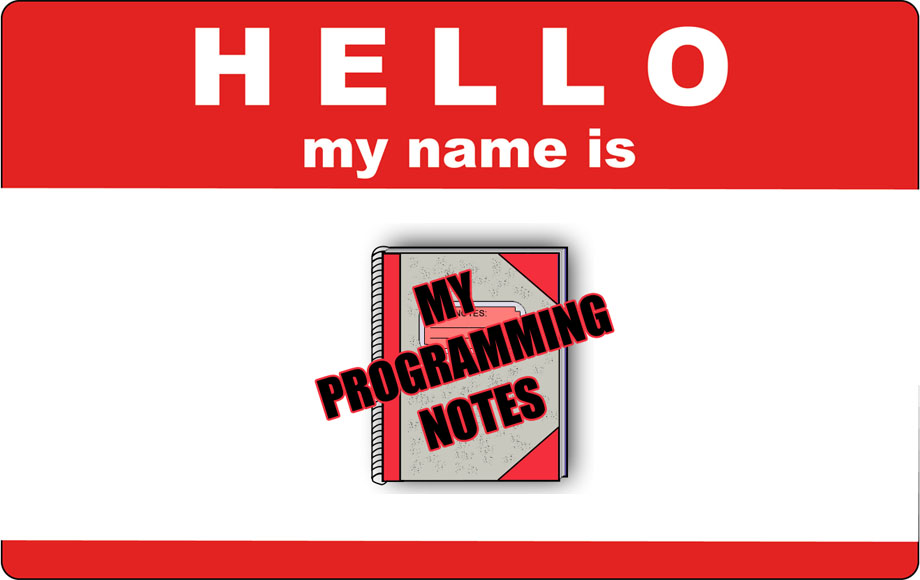
Here is another actual homework assignment which was presented in an intro to programming class.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
This program first prompts the user to enter their name. Upon receiving that information, the program saves input into a character array of size 15. The program then asks if the user has a middle name. If they do, the user will enter a middle name. If they dont, the program proceeds to ask for a last name. Upon receiving the first, [middle], and last names, the program will use strcpy and strcat to copy/append the first, [middle], and last names into a completely new char array titled “fullname.” Lastly, if the users’ first, [middle], or last names are the same, the program will display that data to the screen via cout. The program will also display to the user the number of characters their full name contains using strlen.
NOTE: On some compilers, you may have to add #include < cstring> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 |
// ============================================================================ // File: NameString.cpp // ============================================================================ // // Description: // This program prompts the user for a first name, middle name and last name. // Each name string is to be saved in its own character array. Then a // "full name" string is created by copying the first name to a fourth array, // and concatenating the middle and last names. Once the full name is // in its own buffer, the total number of characters in the full name will // be displayed. // // ============================================================================ #include <iostream> using namespace std; //function prototypes void FirstMiddleLast(char firstname[]); void FirstLast(char firstname[]); // ==== main ================================================================== // // ============================================================================ int main() { char firstname[15]; // char array with ability to hold 15 characters char response = ' '; // get data from user. Notice, you dont need a loop to input data // into the char array cout << "Please enter your first name: "; cin >> firstname; // check to see if user has a middle name cout << "nDo you have a middle name?(Y/N): "; cin >> response; // you could also use a switch statement here if ((response=='y')||(response=='Y')) { FirstMiddleLast(firstname); // function declaration } else if ((response=='n')||(response=='N')) { FirstLast(firstname); // function declaration } else { cout << "nPlease press either 'Y' or 'N'nna" << "Program exiting...n"; } return 0; }// end of main // ==== FirstMiddleLast ======================================================= // // This function will take as input the first name, and prompt the user for // their middle and last name. Then it will display the total number of // characters in their name // // Input: // limit [IN] -- The users first name // // Output: // The users full name, and the total number of characters in their name // // ============================================================================ void FirstMiddleLast(char firstname[]) { char middlename[15]; char lastname[15]; char fullname[30]; cout << "nPlease enter your middle name: "; cin >> middlename; cout <<"nPlease enter your last name: "; cin >> lastname; strcpy(fullname, firstname); // copies data from 'firstname' into 'fullname' array strcat(fullname, " "); // appends 'fullname' with a space strcat(fullname, middlename); // appends 'fullname' to contain the data from 'middlename' array strcat(fullname, " "); // appends 'fullname' with a space strcat(fullname, lastname); // appends 'fullname' to contain the data from 'lastname' array cout <<"ntYour full name is " << fullname << endl; cout << "tThe total number of characters in your name is: "; cout << strlen(fullname)-2 << endl; // gets total num of chars contained in the 'fullname' array, minus the 2 spaces // if strcmp returns 0, the two strings are the same if(strcmp(firstname, middlename) == 0) { cout << "tYour first and middle name are the samen"; } if(strcmp(middlename, lastname) == 0) { cout << "tYour middle and last name are the samen"; } if(strcmp(firstname, lastname) == 0) { cout << "tYour first and last name are the samen"; } }// end of FirstMiddleLast // ==== FirstLast ============================================================= // // This function will take as input the first name, and prompt the user for // their last name. Then it will display the total number of characters in // their name // // Input: // limit [IN] -- The users first name // // Output: // The users full name, and the total number of characters in their name // // ============================================================================ void FirstLast(char firstname[]) { char lastname[15]; char fullname[30]; cout <<"nPlease enter your last name: "; cin >> lastname; strcpy(fullname, firstname); // copies data from 'firstname' into 'fullname' array strcat(fullname, " "); // appends 'fullname' with a space strcat(fullname, lastname); // appends 'fullname' to contain the data from 'lastname' array cout <<"ntYour full name is " << fullname << endl; cout << "tThe total number of characters in your name is: "; cout << strlen(fullname)-1 << endl; // gets total num of chars contained in the 'fullname' array, minus the single space // if strcmp returns 0, the two strings are the same if(strcmp(firstname, lastname) == 0) { cout << "tYour first and last name are the samenn"; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
CHAR ARRAY
Unlike integer arrays, a loop is not necessary in order to input data into a char array. This is highlighted on lines 32, 76, 79, and 125.
FUNCTIONS
Notice lines 18, 19, 69 and 119. When dealing with arrays, in order to pass variables to different functions, you need to include brackets[] letting the compiler know that you are passing an array variable. If you do not declare the functions as so, you will get a compiler error.
STRCPY/STRCAT
These functions are highlighted on lines 81-85, and 127-129. Read the comments within the code on those selected lines, they are helpful.
STRCMP
This compares two strings together to determine if they are the same. This is displayed on lines 92, 96, 100, and 136 when comparing the first, [middle], and last names together for sameness.
STRLEN
This finds the length of the current string, as noted on lines 89 and 133.
Once compiled, you should get this as your output:
Note: The code was compiled five separate times to display the different outputs its able to produce
Please enter your first name: My
Do you have a middle name?(Y/N): y
Please enter your middle name: Programming
Please enter your last name: NotesYour full name is My Programming Notes
The total number of characters in your name is: 18
-----------------------------------------------------------------------Please enter your first name: Programming
Do you have a middle name?(Y/N): N
Please enter your last name: NotesYour full name is Programming Notes
The total number of characters in your name is: 16
-----------------------------------------------------------------------Please enter your first name: Notes
Do you have a middle name?(Y/N): y
Please enter your middle name: Notes
Please enter your last name: NotesYour full name is Notes Notes Notes
The total number of characters in your name is: 15
Your first and middle name are the same
Your middle and last name are the same
Your first and last name are the same
-----------------------------------------------------------------------Please enter your first name: My
Do you have a middle name?(Y/N): N
Please enter your last name: MyYour full name is My My
The total number of characters in your name is: 4
Your first and last name are the same
-----------------------------------------------------------------------Please enter your first name: My
Do you have a middle name?(Y/N): zPlease press either 'Y' or 'N'
Program exiting...
C++ || Find The Average Using an Array – Omit Highest And Lowest Scores
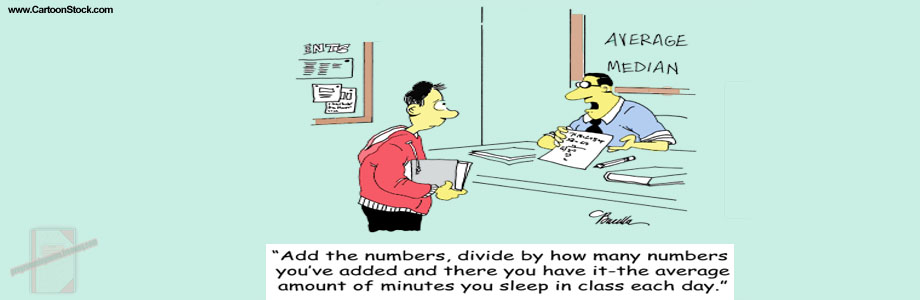
This page will consist of two programs which calculates the average of a specific amount of numbers using an array.
REQUIRED KNOWLEDGE FOR BOTH PROGRAMS
Float Data Type
Constant Values
Arrays
For Loops
Assignment Operators
Basic Math
====== FIND THE AVERAGE USING AN ARRAY ======
The first program is fairly simple, and it was used to introduce the array concept. The program prompts the user to enter the total amount of numbers they want to find the average for, then the program displays the answer to them via cout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
#include <iostream> using namespace std; int main() { // declare variables float total = 0; int numElems = 0; float average[100]; // declare array which has the ability to hold 100 elements cout << "How many numbers do you want to find the average for?: "; cin >> numElems; // user enters data into array using a for loop // you can also use a while loop, but for loops are more common // when dealing with arrays for(int index=0; index < numElems; ++index) { cout << "nEnter #" << index +1<< " : "; cin >> average[index]; total += average[index]; } // find the average. Note: // the expression below literally // means: total = total / numElems; total /= numElems; cout << "nThe average of the " << numElems << " numbers is " << total <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
ARRAY
Notice the array declaration on line #9. The type of array being used in this program is a static array, which has the ability to store up to 100 integer elements in the array. You can change the number of elements its able to store to a higher or lower number if you wish.
FOR LOOP
Notice line 17-22 contains a for loop, which is used to actually store the data inside of the array. Without some type of loop, it is virtually impossible for the user to input data into the array; that is, unless you want to add 100 different cout statements into your code asking the user to input data. Line 21 uses the assignment operator “+=” which gives us a running total of the data that is being inputted into the array. Note the loop only stores as many elements as the user so desires, so if the user only wants to input 3 numbers into the array, the for loop will only execute 3 times.
Once compiled, you should get this as your output:
How many numbers do you want to find the average for?: 5
Enter #1 : 23
Enter #2 : 17
Enter #3 : 29
Enter #4 : 14
Enter #5 : 16
The average of the 5 numbers is 19.8
====== FIND THE AVERAGE – OMIT HIGHEST AND LOWEST SCORES ======
The second program is really practical in a real world setting, specifically when a teacher records test scores into the computer. We were asked to create a program for a fictional competition which had 6 judges. The 6 judges each gave a score of the performance for a competitor in a competition, (i.e a score of 1-10), and we were asked to find the average of those scores, omitting the highest/lowest results. The program was to store the scores into an array, display the scores back to the user via cout, display the highest and lowest scores among the 6 obtained, display the average of the 6 scores, and finally display the average adjusted scores omitting the highest and lowest result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 |
#include <iostream> using namespace std; const int NUM_JUDGES = 6; int main() { int scores[NUM_JUDGES]; // array is initialized using a const variable int highestScore = -999999; int lowestScore = 999999; float sumOfScores = 0; float avgScores = 0; // use a for loop to obtain data from user using the const variable cout<< "Judges, enter one score each for the current competitor: "; for(int counter=0; counter < NUM_JUDGES; ++counter) { cin >> scores[counter]; } // use another for loop to redisplay the data back to the user via cout cout<<"nThese are the scores from the " << NUM_JUDGES << " judges: "; for(int index=0; index < NUM_JUDGES; ++index) { cout<<"nThe score for judge #"<<(index+1)<<" is: "<<scores[index]; } // use a for loop to go thru the array checking to see the highes/lowest element cout<<"nnThese are the highest and lowest scores: "; for(int index=0; index < NUM_JUDGES; ++index) { // if current score in the array is bigger than the current 'highestScore' // element, then set 'highestScore' equal to the current array element if(scores[index] > highestScore) { highestScore = scores[index]; } // if current 'lowestScore' element is bigger than the current array element, // then set 'lowestScore' equal to the current array element if(lowestScore > scores[index]) { lowestScore = scores[index]; } } cout << "ntHighest: "<< highestScore; cout << "ntLowest: "<< lowestScore; cout << "nThe average score is: "; // this calculates a running total, adding each element in the array together for(int counter=0; counter < NUM_JUDGES; ++counter) { sumOfScores += scores[counter]; } avgScores = sumOfScores/NUM_JUDGES; cout<< avgScores; // reset data back to 0 so we dont get wrong answers sumOfScores = 0; avgScores = 0; cout<<"nThe average adjusted score omitting the highest and lowest result is: "; // final loop, which calculates a running total, adding each element // in the array together, this time omitting the highest/lowest elements for(int counter=0; counter < NUM_JUDGES; ++counter) { // IF(current score isnt equal to the highest elem) AND (current score isnt equal lowest elem) // THEN create a running total if ((scores[counter] != highestScore) && (scores[counter] != lowestScore)) { sumOfScores += scores[counter]; } } avgScores = sumOfScores/(NUM_JUDGES-2); cout<< avgScores <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
CONST
A constant variable was declared and used to initialize the array (line 8). Note, when using static arrays, the program has to know how many elements to initialize the program with before the program starts, so creating a constant variable to do that for us is convenient.
FOR LOOPS
Once again loops were used to traverse the array, as noted on lines 16, 23, 30, 53, and 70. The const variable was also used within the for loops, making it easier to modify the code if its necessary to reduce or increase the number of available judges.
HIGHEST/LOWEST SCORES
This is noted on lines 34-44, and it is really simple to understand the process once you see the code.
OMITTING HIGHEST/LOWEST SCORE
Lines 70-78 highlight this process, and the loop basically traverses the array, skipping over the highest/lowest elements
Once compiled, you should get this as your output:
Judges, enter one score each for
the current competitor: 123 453 -789 2 23345 987These are the scores from the 6 judges:
The score for judge #1 is: 123
The score for judge #2 is: 453
The score for judge #3 is: -789
The score for judge #4 is: 2
The score for judge #5 is: 23345
The score for judge #6 is: 987These are the highest and lowest scores:
Highest: 23345
Lowest: -789
The average score is: 4020.17
The average adjusted score omitting the highest and lowest result is: 391.25
C++ || Struct – Add One Second To The Clock Using A Struct
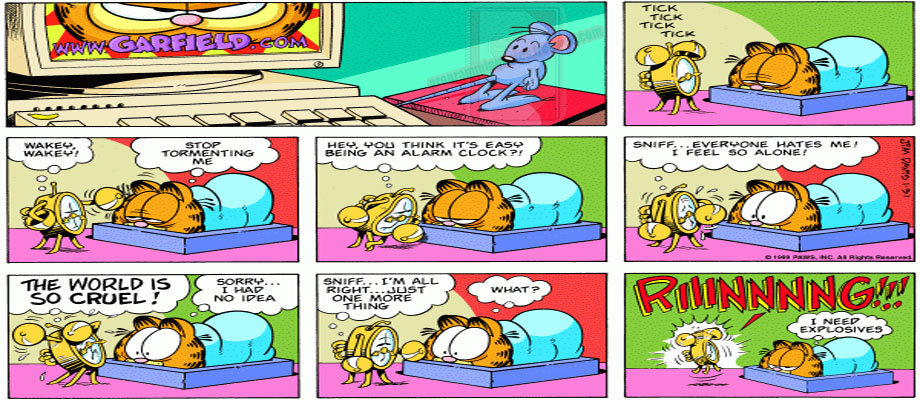
Here is another actual homework assignment which was presented in an intro to programming class. This program utilizes a struct, which is very similar to the class concept. For this assignment, the class was asked to make a program which prompted the user to enter a time in HH:MM:SS (Hours:Minutes:Seconds) format. Upon obtaining the time from the user, our class was asked to use a struct implementation which was to simply add one second to the time that was entered by the user. Seems easy enough, though I initially had afew problems when starting the program.
This page will be very brief in its breakdown of the program’s code, as it is already heavily commented. The code is basically unchanged from the code which was turned in for grading.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Functions
Passing a Value By Reference
Structures
Constant Variables
Boolean Expressions
Setw
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 |
// ============================================================================ // File: AddOneSecond.cpp // ============================================================================ // Description: // This program prompts the user for the input time, then adds // one second to the time -- if any of the time structure fields need to // "rollover" (e.g., the hours, minutes or seconds need to be reset to zero), // this function will handle that. Finally, the incremented time will be // displayed to stdout. // ============================================================================ #include <iostream> #include <iomanip> using namespace std; // structure declaration struct Time { int hours; int min; int sec; }; // defined constants which do not change // specifies the max values for each variable const int MAX_HOURS = 23; const int MAX_MINS = 59; const int MAX_SECS = 59; // function prototypes bool IsTimeValid(Time timeParam); void AddOneSecond(Time &timeParam); void DisplayTime(Time timeParam); // ==== main ================================================================== // // ============================================================================ int main() { Time userTime = {0,0,0}; // Initialize struct members: hrs, min, sec to 0 char colon = ':'; cout << "Please enter the time in HH:MM:SS format: "; cin >> userTime.hours >> colon >> userTime.min >> colon >> userTime.sec; // Checks to see if user entered correct data if (IsTimeValid(userTime) == false) { cout << "Invalid input...n" << endl; exit(EXIT_FAILURE); } cout << "nThe incremented time is "; AddOneSecond(userTime); DisplayTime(userTime); return 0; } // end of main // ==== IsTimeValid =========================================================== // // This function will validate that the time structure contains legitimate // values. If this function determines that the time values are invalid, it // will display an error message to stdout. // // Input: // limit [IN] -- The users time input // // Output: // The Time structure as an argument to the AddOneSecond function // // ============================================================================ bool IsTimeValid(Time timeParam) { // checks to see if user inputted data falls between the specified // const variables as declared above if ((timeParam.hours >= 0 && timeParam.hours <= MAX_HOURS) &&(timeParam.min >= 0 && timeParam.min <= MAX_MINS) &&(timeParam.sec >= 0 && timeParam.sec <= MAX_SECS)) { return true; } else { return false; } } // end of IsTimeValid // ==== AddOneSecond ========================================================== // // This function will add one second to the time // // Input: // limit [IN] -- The users time input // // Output: // The incremented time will be displayed to stdout by calling the // DisplayTime function // // ============================================================================ void AddOneSecond(Time &timeParam) { // this is just a simple bool which checks to see if // the program should increment the max hrs in the // 1st or 2nd if statements, which are located below bool incrementHrsInFirstIf = false; // this is just a simple bool which checks to see if // the program should increment the max mins in the // 2nd or 3rd if statements, which are located below bool incrementMinInSecondIf = false; // ========== if statement #1 - hrs ========== // checks to see if user selected hrs is equal to MAX // if it is, reset time back to 0 if (timeParam.hours == MAX_HOURS && timeParam.min == MAX_MINS) { timeParam.hours = 0; incrementHrsInFirstIf = true; } // ========== if statement #2 - min ========== // checks to see if user selected mins is equal to MAX // if it is, reset time back to 0 if (timeParam.min == MAX_MINS) { timeParam.min = 0; // if mins == 59, we need to increment the hrs by 1 if(incrementHrsInFirstIf == false) { ++timeParam.hours; } incrementMinInSecondIf = true; } // ========== if statement #3 - Secs ========== // checks to see if user selected sec is equal to MAX // if it is, reset time back to 0 if (timeParam.sec == MAX_SECS) { timeParam.sec = 0; // if secs == 59, we need to increment the mins by 1 if(incrementMinInSecondIf == false) { ++timeParam.min; } } else // if time is not at max, increment by 1 { ++timeParam.sec; } } // end of AddOneSecond // ==== DisplayTime =========================================================== // // This function will display the user's time // // Input: // limit [IN] -- The users time input // // Output: // The incremented time will be displayed // // ============================================================================ void DisplayTime(Time timeParam) { cout.fill('0'); cout << setw(2) << timeParam.hours << ":" << setw(2) << timeParam.min << ":" << setw(2) << timeParam.sec << endl; } // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
STRUCT
The structure declaration is placed above the main function, as noted on lines 16-21, containing the 3 variables (Hrs, min, sec) which are defined within the program. Line 39 displays how to access those variables from the main function, having the variable “Time userTime” as the means of access. Note, line 43, the variables can utilize cin for input.
CONST
Lines 25-27 declare the constant variables, which hold the maximum allowable time the clock is able to display. The function IsTimeValid (line 72) checks to see if user defined input is within the maximum allowable limit or not.
FILL
The program will automatically display 2 numbers for hours,mins,sec even if the user only inputted one number, as noted on lines 169-172. This is basically the same as the setfill function.
Once compiling the above code, you should receive this as your output
Note: The code was compiled five separate times to display the different outputs its able to produce
==== SAMPLE RUN #1 ====
Please enter the time in HH:MM:SS format: 0:0:25
The incremented time is 00:00:26==== SAMPLE RUN #2 ====
Please enter the time in HH:MM:SS format: 23:58:59
The incremented time is 23:59:00==== SAMPLE RUN #3 ====
Please enter the time in HH:MM:SS format: 23:59:59
The incremented time is 00:00:00==== SAMPLE RUN #4 ====
Please enter the time in HH:MM:SS format: : :
The incremented time is 00:00:01==== SAMPLE RUN #5 ====
Please enter the time in HH:MM:SS format: 76:09:67
Invalid input...
C++ || Input/Output Text File Manipulation – Find Highest, Lowest, Average & Total Sum
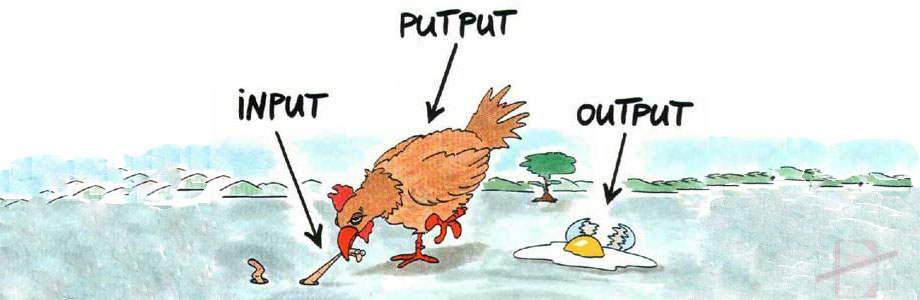
This is a program which will utilize fstream, specifically ifstream and ofstream, to read in data from one .txt file, and it will then output selected data into an entirely new separate .txt file.
The input data file has 8 different rows, with each row containing 7 numbers on each line. The program will take in each line one at a time, manipulating the 7 numbers to receive the desired output. This program will find the highest/lowest number in each selected line, along with the total sum of all the numbers contained in that line, and the average of all the numbers. So at the end of the program, There will be 8 different sets of data compiled for each row, with the output file looking like this:
SAMPLE RUN:
- Input File -
3 5 7 3 4 5 6- Output File -
The dataset for input line #1 is: 3 5 7 3 4 5 6
The highest number is: 7
The lowest number is: 3
The total of the numbers is: 33
The average of the numbers is: 4.71
The data file that is used in this example can be downloaded here
Note: In order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio > Projects > [Your project name] > [Your project name]
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 6, 2012 // Taken From: http://programmingnotes.org/ // File: fileInput.cpp // Description: Demonstrates how to read and write data to a file // ============================================================================ #include <iostream> #include <fstream> using namespace std; int main () { // declare variables ifstream infile; ofstream outfile; int inputNumber = 0; int highestNum = -999999; int lowestNum = 999999; double sum = 0; double average = 0; int currentLineNum = 1; // This opens the input file infile.open("INPUT_programmingnotes_freeweq_com.txt"); if(infile.fail()) //there was an error on open, file not found { cout << "Cannot find input file, press enter to terminate program." << endl; exit (1); // there was an error, program exits } // This opens the output file outfile.open("OUTPUT_programmingnotes_freeweq_com.txt", ios::app); if(outfile.fail()) { cout <<"There was an error opening the output file, press enter to terminate program."; exit(1); // there was an error, program exits } do { cout << "The dataset for input line #" << currentLineNum << " is: "; outfile << "The dataset for input line #" << currentLineNum << " is: "; // loops thru file until we get to the last number // from the selected line // (theres 7 numbers total in each line, see input file for clarification) for(int counter = 1; counter <= 7; counter++) { infile >> inputNumber; sum += inputNumber; // calculates the total sum of #'s for each line // checks to see which number is highest/lowest from // each selected line if(inputNumber > highestNum) { highestNum = inputNumber; } if(inputNumber < lowestNum) { lowestNum = inputNumber; } // displays current number to output screen // and saves to file cout << inputNumber << "\t"; outfile << inputNumber << "\t"; }// end for loop average = sum / 7; // finds the total avg for each line // this displays data to the output screen cout << endl; cout << "The highest number is: " << highestNum << endl; cout << "The lowest number is: " << lowestNum << endl; cout << "The total of the numbers is: " << sum << endl; cout << "The average of the numbers is: " << average << endl; // outfile section here, saves data to file outfile << endl; outfile << "The highest number is: " << highestNum << endl; outfile << "The lowest number is: " << lowestNum << endl; outfile << "The total of the numbers is: " << sum << endl; outfile << "The average of the numbers is: " << average << endl << endl; // outfile section end // resets variables back to default values highestNum = -999999; lowestNum = 999999; sum = 0; average = 0; currentLineNum++; // places data on new line cout << endl << endl; } while(!infile.eof()); // loop stops once it reaches the end of file cout << endl << endl <<"\tWe have reached the end of the file!"<< endl; outfile << endl << endl <<"\tWe have reached the end of the file!"<< endl << endl; outfile.close(); // closes outfile infile.close(); // closes infile return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
LOOPS
This program utilizes one do/while loop on lines 39-97 which loops thru the input file until it reaches the end of the file. This program also uses a for loop, which is noted on line 46.
CALCULATING THE SUM
Line 50 contains the assignment operator “+=“, which will calculate a running total for all the values of each selected line.
READING IN DATA FROM FILE
This is noted in line 48, and works just like a cin statement.
OPENING FILES
File declarations, and the opening of files are highlighted on lines: 14-15, 24-25, 32-33. On line 32, the term “ios::app” means the file will append new data to the text file, instead of overwriting the old data contained within that file.
OUTPUT DATA TO FILE
This is highlighted on lines 80-84, and as you can see, the output statements are exactly the same as cout statements.
CLOSE FILES
Remember to close the files you open, as highlighted on lines 101 and 102.
Once compiling the above code, you should receive this as your output (for the 8 selected lines contained within the input text file)
The dataset for input line #1 is: 346 130 982 90 656 117 595
The highest number is: 982
The lowest number is: 90
The total of the numbers is: 2916
The average of the numbers is: 416.571The dataset for input line #2 is: 415 948 126 4 558 571 87
The highest number is: 948
The lowest number is: 4
The total of the numbers is: 2709
The average of the numbers is: 387The dataset for input line #3 is: 42 360 412 721 463 47 119
The highest number is: 721
The lowest number is: 42
The total of the numbers is: 2164
The average of the numbers is: 309.143The dataset for input line #4 is: 441 190 985 214 509 2 571
The highest number is: 985
The lowest number is: 2
The total of the numbers is: 2912
The average of the numbers is: 416The dataset for input line #5 is: 77 81 681 651 995 93 74
The highest number is: 995
The lowest number is: 74
The total of the numbers is: 2652
The average of the numbers is: 378.857The dataset for input line #6 is: 310 9 995 561 92 14 288
The highest number is: 995
The lowest number is: 9
The total of the numbers is: 2269
The average of the numbers is: 324.143The dataset for input line #7 is: 466 664 892 8 766 34 639
The highest number is: 892
The lowest number is: 8
The total of the numbers is: 3469
The average of the numbers is: 495.571The dataset for input line #8 is: 151 64 98 813 67 834 369
The highest number is: 834
The lowest number is: 64
The total of the numbers is: 2396
The average of the numbers is: 342.286We have reached the end of the file!
C++ || Count The Total Number Of Characters, Vowels, & UPPERCASE Letters Contained In A Sentence Using A ‘While Loop’
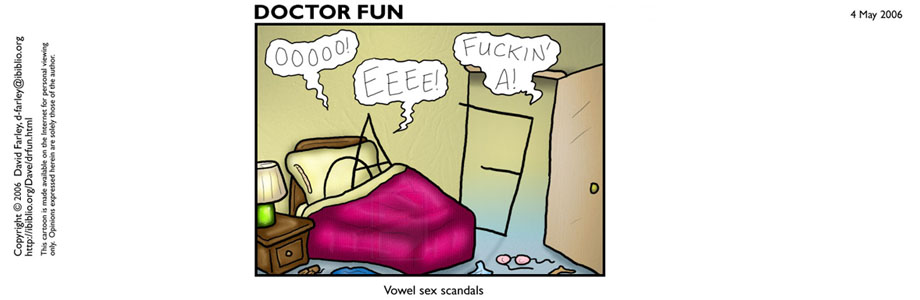
This program will prompt the user to enter a sentence, then upon entering an “exit code,” will display the total number of uppercase letters, vowels and characters contained within that sentence. This program is very similar to an earlier project, this time, utalizing a while loop, the setw and setfill functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
#include <iostream> #include <iomanip> using namespace std; int main() { // declare variables int numUpperCase = 0; int numVowel = 0; int numChars = 0; char character = ' '; cout << "Enter a sentence: "; // Get sentence from user utilizing while loop while(cin >> character && character != '.') { // Checks to see if inputted data is UPPERCASE if ((character >= 'A')&&(character <= 'Z')) { ++numUpperCase; // Increments Total number of uppercase by one } // Checks to see if inputted data is a vowel if ((character == 'a')||(character == 'A')||(character == 'e')|| (character == 'E')||(character == 'i')||(character == 'I')|| (character == 'o')||(character == 'O')||(character == 'u')|| (character == 'U')||(character == 'y')||(character == 'Y')) { ++numVowel; // Increments Total number of vowels by one } ++numChars; // Increments Total number of chars by one } // end loop // display data using setw and setfill cout << setfill('.'); // This will fill any excess white space with period within the program cout <<left<<setw(36)<<"ntTotal number of upper case letters:"<<right<<setw(10) <<numUpperCase<<endl; cout <<left<<setw(36)<<"tTotal number of vowels:"<<right<<setw(10) <<numVowel<<endl; cout <<left<<setw(36)<<"tTotal number of characters:"<<right<<setw(10) <<numChars<<endl; return 0; }// http://programmingnotes.freeweq.com |
QUICK NOTES:
The highlighted portions are areas of interest.
In order to use the setfill and setw functions, remember to #include iomanip, as noted on line 2.
Notice line 17 contains the while loop declaration. The loop will continually ask the user to input data, and will not stop doing so until the user enters an exit character, and the defined exit character in this program is a period (“.”). So, the program will not stop asking the user to enter data until they enter a period.
Once compiling the above code, you should receive this as your output
Enter a sentence: My Programming Notes Is Awesome.
Total number of upper case letters:.........5
Total number of vowels:....................11
Total number of characters:................27
C++ || Display Today’s Date Using a Switch
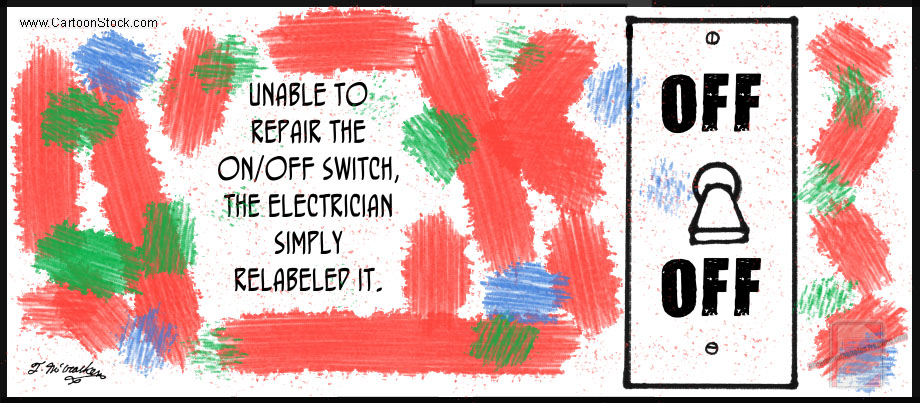
If statements, char’s and strings have been previously discussed, and this page will be more of the same. This program will demonstrate how to use a switch to display today’s date, converting from mm/dd/yyyy format to formal format (i.e January 5th, 2012).
This same program can easily be done using if statements, but sometimes that is not always the fastest programming method. Switch statements are like literal light switches because the code “goes down a line” to check to see which case is valid or not, just like if statements. You will see why switches are very effective when used right by examining this program.
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
====== TODAY’S DATE USING A SWITCH ========
So to start our program out, lets define the variables.
1 2 3 4 5 6 7 8 9 10 |
#include <iostream> using namespace std; int main() { // Declare Variables int month; int day; int year; char backSlash; } |
Notice on line 9 there is a variable named “backslah.” This program was designed to display the date in this format.
Enter today's date: 10/7/2008
October 7th, 2008
So the only way to achieve that was to add a “place holder” variable during the user input process, which is demonstrated below.
1 2 3 |
// Collect Data cout << "Enter today's date: "; cin >> month >> backSlash >> day >> backSlash >> year; |
In line 3, you can see the format that the user will input the data in. They will input data in mm/dd/yyyy format, and having the “backslash” placeholder there will make that possible.
After the user enters in data, how will the program convert numbers into actual text? Next comes the switch statements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
// Check month switch(month) { case 1: { cout << "tJanuary "; break; } case 2: { cout << "tFebruary "; break; } case 3: { cout << "tMarch "; break; } case 4: { cout << "tApril "; break; } case 5: { cout << "May "; break; } case 6: { cout << "tJune "; break; } case 7: { cout << "tJuly "; break; } case 8: { cout << "tAugust "; break; } case 9: { cout << "tSeptember "; break; } case 10: { cout << "tOctober "; break; } case 11: { cout << "tNovember "; break; } case 12: { cout << "tDecember "; break; } default: { cout << "t" << month <<" is not a valid monthn"; exit(1); // this forces the program to quit } } |
Line 2 contains the switch declaration, and its comparing the variable of “month” to the 12 different cases that is defined within the switch statement. So this piece of code will “go down the line” comparing to see if the user inputted data is any of the numbers, from 1 to 12. If the user inputted a number which does not fall between 1 thru 12, the “default” case will be executed, prompting the user that the data they inputted was invalid, which can be seen in line 64. Notice line 67 has an exit code. This program will force an exit whenever the user enters invalid data.
Line 7 is very important, because that forces the computer to “break” away from the selected case whenever it is done examining the piece of code. It is important to add the break in there to avoid errors, which may result in the program giving you wrong output as the answer.
Next we will add another switch statement to convert the day of the month to have a number suffix (i.e displaying the number in 1st, 2nd, 3rd format). This is very similar to the previous switch statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Check Day switch(day) { case 1: case 21: case 31: { cout << day << "st, "; break; } case 2: case 22: { cout << day << "nd, "; break; } case 3: case 23: { cout << day << "rd, "; break; } default: { cout << day << "th, "; break; } } |
This block of code is very similar to the previous one. Line 2 is declaring the variable ‘day’ to be compared with the base cases, line 7 and so forth has the break lines, but line 4, 9 and 14 are different. If you notice, line 4, 9 and 14 are comparing multiple cases in one line. Yes with switch statements, you can do that. Just like you can compare multiple values in if statements, the same cane be done here. So this switch is comparing the number the user inputted, with the base cases, adding a suffix to the end of the number.
So far we have obtained data from the user, compared the month and day using switch statements and displayed that to the screen. Now all we have to do is output the year to the user. This is fairly simple, because the year is not being compared, you are just simply using a cout to display data to the user.
1 2 |
// Display Year cout << year << endl; |
Adding all the code together should give us this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 |
#include <iostream> using namespace std; int main() { // Declare Variables int month; int day; int year; char backSlash; // Collect Data cout << "Enter today's date: "; cin >> month >> backSlash >> day >> backSlash >> year; // Check month switch(month) { case 1: { cout << "tJanuary "; break; } case 2: { cout << "tFebruary "; break; } case 3: { cout << "tMarch "; break; } case 4: { cout << "tApril "; break; } case 5: { cout << "May "; break; } case 6: { cout << "tJune "; break; } case 7: { cout << "tJuly "; break; } case 8: { cout << "tAugust "; break; } case 9: { cout << "tSeptember "; break; } case 10: { cout << "tOctober "; break; } case 11: { cout << "tNovember "; break; } case 12: { cout << "tDecember "; break; } default: { cout << "t" << month <<" is not a valid monthn"; exit(1); } } // Check Day switch(day) { case 1: case 21: case 31: { cout << day << "st, "; break; } case 2: case 22: { cout << day << "nd, "; break; } case 3: case 23: { cout << day << "rd, "; break; } default: { cout << day << "th, "; break; } } // Display Year cout << year << endl; // Terminate return 0; } // http://programmingnotes.freeweq.com |
Once compiled, you should get this as your output
Enter today's date: 1/5/2012
January 5th, 2012
C++ || Using If Statements, Char & String Variables
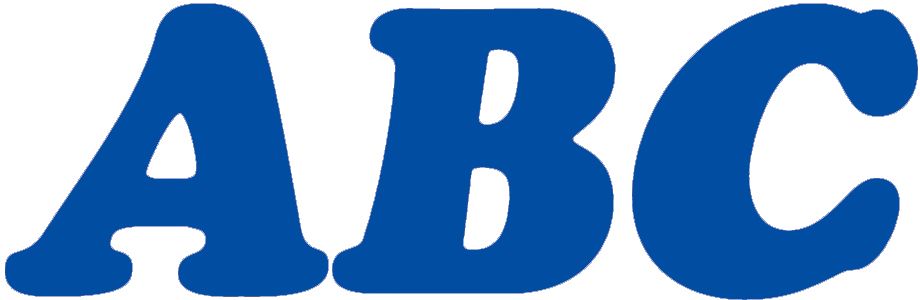
As previously mentioned, you can use the “int/float/double” data type to store numbers. But what if you want to store letters? Char and Strings help you do that.
==== SINGLE CHAR ====
This example will demonstrate a simple program using char, which checks to see if you entered the correctly predefined letter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: char.cpp // Description: Demonstrates using char variables // ============================================================================ #include <iostream> int main() { // Declare empty variable char userInput = ' '; std::cout << "Please try to guess the letter I am thinking of: "; std::cin >> userInput; // Use an If Statement to check equality if (userInput == 'a' || userInput == 'A') { std::cout <<"\nYou have Guessed correctly!"<< std::endl; } else { std::cout <<"\nSorry, that was not the correct letter I was thinking of"<< std::endl; } return 0; }// http://programmingnotes.org/ |
Notice in line 13 I declare the char data type, naming it “userInput.” I also initialized it as an empty variable. In line 19 I used an “If/Else Statement” to determine if the user inputted value matches the predefined letter within the program. I also used the “OR” operator in line 19 to determine if the letter the user inputted was lower or uppercase. Try compiling the program simply using this
if (userInput == 'a')
as your if statement, and notice the difference.
The resulting code should give this as output
Please try to guess the letter I am thinking of: K
Sorry, that was not the correct letter I was thinking of
==== CHECK IF LETTER IS UPPER CASE ====
This example is similar to the previous one, and will check if a letter is uppercase.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: uppercase.cpp // Description: Demonstrates checking if a char variable is uppercase // ============================================================================ #include <iostream> int main() { // Declare empty variable char userInput = ' '; std::cout << "Please enter an UPPERCASE letter: "; std::cin >> userInput; // Checks to see if entered data falls between uppercase values if ((userInput >= 'A') && (userInput <= 'Z')) { std::cout << "\n" << userInput << " is an uppercase letter"<< std::endl; } else { std::cout <<"\nSorry, "<< userInput <<" is not an uppercase letter"<< std::endl; } return 0; }// http://programmingnotes.org/ |
Notice in line 19, an If statement was used, which checked to see if the user entered data fell between letter A and letter Z. We did that by using the “AND” operator. So that IF statement is basically saying (in plain english)
IF ('userInput' is equal to or greater than 'A') AND ('userInput' is equal to or less than 'Z')
THEN it is an uppercase letter
C++ uses ASCII codes to determine letters, so from looking at the table, the letter ‘A’ would equal ASCII code number 65, letter ‘B’ would equal ASCII code number 66 and so forth, until you reach letter Z, which would equal ASCII code number 90. So in literal terms, the program is checking to see if the user input is between ASCII code number 65 thru 90. If it is, then the number is an uppercase letter, otherwise it is not.
The resulting code should give this as output
Please enter an UPPERCASE letter: G
G is an uppercase letter
==== CHECK IF LETTER IS A VOWEL ====
This example will utilize more if statements, checking to see if the user inputted data is a vowel or not. This will be very similar to the previous example, utilizing the OR operator once again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: vowel.cpp // Description: Demonstrates checking if a char variable is a vowel // ============================================================================ #include <iostream> int main() { // Declare empty variable char userInput = ' '; std::cout << "Please enter a vowel: "; std::cin >> userInput; // Checks to see if inputted data is A,E,I,O,U,Y if ((userInput == 'a')||(userInput == 'A')||(userInput == 'e')|| (userInput == 'E')||(userInput == 'i')||(userInput == 'I')|| (userInput == 'o')||(userInput == 'O')||(userInput == 'u')|| (userInput == 'U')||(userInput == 'y')||(userInput == 'Y')) { std::cout <<"\nCorrect, "<< userInput <<" is a vowel!"<< std::endl; } else { std::cout <<"\nSorry, "<< userInput <<" is not a vowel"<< std::endl; } return 0; }// http://programmingnotes.org/ |
This program should be very straight forward, and its basically checking to see if the user inputted data is the letter A, E, I, O, U or Y.
The resulting code should give the following output
Please enter a vowel: O
Correct, O is a vowel!
==== HELLO WORLD v2 ====
This last example will demonstrate using the string data type to print the line “Hello World!” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: string.cpp // Description: Demonstrates using string variables // ============================================================================ #include <iostream> #include <string> int main() { // Declare empty variable std::string userInput = " "; std::cout << "Please enter a sentence: "; std::getline(std::cin, userInput); std::cout << "\nYou Entered: "<<userInput << std::endl; return 0; }// http://programmingnotes.com |
Notice in line 10 we have to add “#include string” in order to use the getline function, which is used on line 17. Rather than just simply using the “cin” function, we used the getline function instead to read in data. That is because cin is unable to read entire sentences as input. So in line 17, the following code reads a line from the user input until a newline is entered.
The resulting code should give following output
Please enter a sentence: Hello World!
You Entered: Hello World!
C++ || Simple Math Using Integer & Double
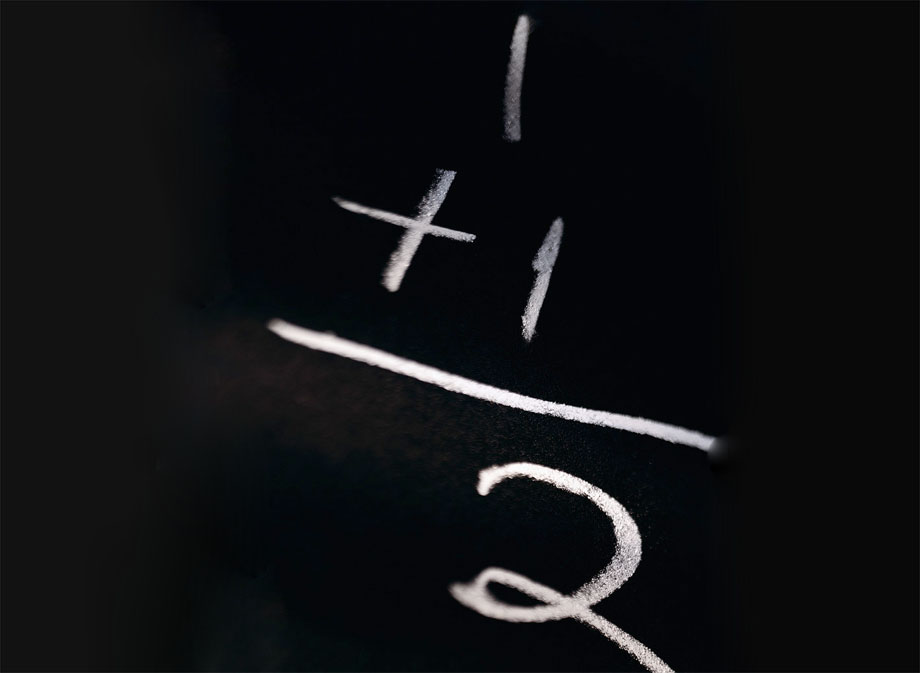
This page will display the use of int and double data types.
==== ADDING TWO NUMBERS TOGETHER ====
To add two numbers together, you will have to first declare your variables by doing something like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 15, 2021 // Taken From: http://programmingnotes.org/ // File: addition.cpp // Description: Demonstrates adding numbers together // ============================================================================ #include <iostream> int main() { int num1 = 0; int num2 = 0; int sum = 0; std::cout << "Please enter the first number: "; std::cin >> num1; std::cout << "\nPlease enter the second number: "; std::cin >> num2; // calculate the sum of the two numbers here sum = num1 + num2; std::cout << "\nThe sum of " <<num1<<" and "<<num2<< " is: "<< sum; return 0; }// http://programmingnotes.org/ |
Notice in lines 12-14, I declared my variables, giving them a name. You can name your variables anything you want, with a rule of thumb as naming them something meaningful to your code (i.e avoid giving your variables arbitrary names like “x” or “y”). In line 22 the actual math process is taking place, storing the sum of “num1” and “num2” in a variable called “sum.” I also initialized my variables to zero. You should always initialize your variables.
The above code should give you the following output:
Please enter the first number: 8
Please enter the second number: 24
The sum of 8 and 24 is: 32
==== SUBTRACTING TWO NUMBERS ====
Subtracting two numbers works the same way as the above code, and we would only need to edit the above code in one place to achieve that. In line 22, replace the addition symbol with a subtraction sign, and you should have something like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 15, 2021 // Taken From: http://programmingnotes.org/ // File: subtraction.cpp // Description: Demonstrates subtracting numbers together // ============================================================================ #include <iostream> int main() { int num1 = 0; int num2 = 0; int sum = 0; std::cout << "Please enter the first number: "; std::cin >> num1; std::cout << "\nPlease enter the second number: "; std::cin >> num2; // calculate the difference of the two numbers here sum = num1 - num2; std::cout << "\nThe difference between " <<num1<<" and "<<num2<< " is: "<< sum; return 0; }// http://programmingnotes.org/ |
The above code should give you the following output:
Please enter the first number: 8
Please enter the second number: 24
The difference between 8 and 24 is: -16
==== MULTIPLYING TWO NUMBERS ====
This can be achieved the same way as the 2 previous methods, simply by editing line 22, and replacing the designated math operator with the star symbol “*”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 15, 2021 // Taken From: http://programmingnotes.org/ // File: multiplication.cpp // Description: Demonstrates multiplying numbers together // ============================================================================ #include <iostream> int main() { int num1 = 0; int num2 = 0; int sum = 0; std::cout << "Please enter the first number: "; std::cin >> num1; std::cout << "\nPlease enter the second number: "; std::cin >> num2; // calculate the product of the two numbers here sum = num1 * num2; std::cout << "\nThe product of " <<num1<<" and "<<num2<< " is: "<< sum; return 0; }// http://programmingnotes.org/ |
The above code should give you the following output:
Please enter the first number: 8
Please enter the second number: 24
The product of 8 and 24 is: 192
==== DIVIDING TWO NUMBERS TOGETHER ====
This one is a little different from the other three. Before we would use integer variables to store our data. In division, when you divide numbers together, sometimes they end in decimals. Integer data types can not store decimal data (try it yourself and see), so here is where we use a floating point data type to store the values.
So the resulting code will basically be the same as the other previous three, only instead of our variables being of type int, they will be of type double.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 15, 2021 // Taken From: http://programmingnotes.org/ // File: division.cpp // Description: Demonstrates dividing numbers together // ============================================================================ #include <iostream> int main() { int num1 = 0; int num2 = 0; double sum = 0; std::cout << "Please enter the first number: "; std::cin >> num1; std::cout << "\nPlease enter the second number: "; std::cin >> num2; // calculate the quotient of the two numbers here sum = (double)num1 / num2; std::cout << "\nThe quotient of " <<num1<<" and "<<num2<< " is: "<< sum; return 0; }// http://programmingnotes.org/ |
The above code should give the following output:
Please enter the first number: 8
Please enter the second number: 24
The quotient of 8 and 24 is: 0.333333
==== MODULUS ====
If you wanted to capture the remainder of the quotient you calculated from the above code, you would use the modulus operator (%).
From the above code, you would only need to edit line 22, from division, to modulus.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 15, 2021 // Taken From: http://programmingnotes.org/ // File: modulus.cpp // Description: Demonstrates performing modulus on numbers // ============================================================================ #include <iostream> int main() { int num1 = 0; int num2 = 0; int remainder = 0; std::cout << "Please enter the first number: "; std::cin >> num1; std::cout << "\nPlease enter the second number: "; std::cin >> num2; // find the remainder of the two numbers here remainder = num1 % num2; std::cout << "\nThe remainder of " <<num1<<" and "<<num2<< " is: "<< remainder; return 0; }// http://programmingnotes.org/ |
The above code should give the following output:
Please enter the first number: 24
Please enter the second number: 8
The remainder of 24 and 8 is: 0