Monthly Archives: February 2021
C++ || How To Round A Number To The Nearest X Using C++
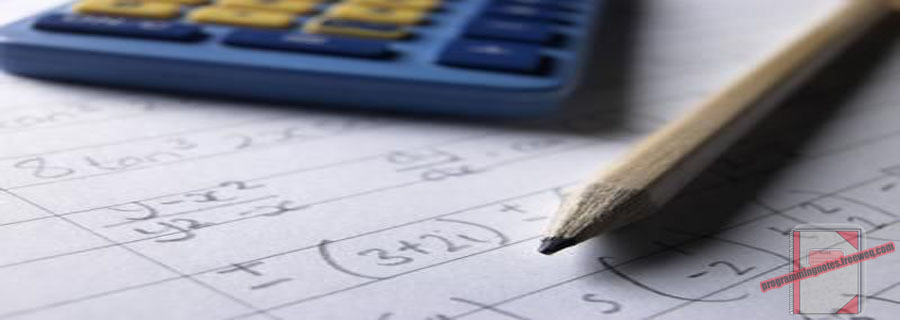
The following is a module with functions which demonstrates how to round a number to the nearest X using C++.
This function has the ability to either round a number to the nearest amount, always round up, or always round down. For example, when dealing with money, this is good for rounding a dollar amount to the nearest 5 cents.
This function can be used to round a number up or down by any step amount.
1. Round – Nearest
The example below demonstrates the use of ‘Utils::roundAmount‘ to round a number to the nearest 5 cents.
The optional function parameter determines they type of rounding to perform.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Round - Nearest // Declare values to round double values[] = { 19.28, 31.22, 19.91, 19.87, 0.05 }; // Declare step amount double stepAmount = 0.05; // Round to nearest amount for (const auto& value : values) { std::cout << "Value: " << value << ", Rounded: " << Utils::roundAmount(value, stepAmount) << std::endl; } // expected output: /* Value: 19.28, Rounded: 19.3 Value: 31.22, Rounded: 31.2 Value: 19.91, Rounded: 19.9 Value: 19.87, Rounded: 19.85 Value: 0.05, Rounded: 0.05 */ |
2. Round – Up
The example below demonstrates the use of ‘Utils::roundAmount‘ to always round a number up to the nearest 5 cents.
The optional function parameter determines they type of rounding to perform.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Round - Up // Declare values to round double values[] = { 19.28, 31.22, 19.91, 19.87, 0.05 }; // Declare step amount double stepAmount = 0.05; // Round up to nearest amount for (const auto& value : values) { std::cout << "Value: " << value << ", Rounded: " << Utils::roundAmount(value, stepAmount, Utils::RoundType::Up) << std::endl; } // expected output: /* Value: 19.28, Rounded: 19.3 Value: 31.22, Rounded: 31.25 Value: 19.91, Rounded: 19.95 Value: 19.87, Rounded: 19.9 Value: 0.05, Rounded: 0.05 */ |
3. Round – Down
The example below demonstrates the use of ‘Utils::roundAmount‘ to always round a number down to the nearest 5 cents.
The optional function parameter determines they type of rounding to perform.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Round - Down // Declare values to round double values[] = { 19.28, 31.22, 19.91, 19.87, 0.05 }; // Declare step amount double stepAmount = 0.05; // Round down to nearest amount for (const auto& value : values) { std::cout << "Value: " << value << ", Rounded: " << Utils::roundAmount(value, stepAmount, Utils::RoundType::Down) << std::endl; } // expected output: /* Value: 19.28, Rounded: 19.25 Value: 31.22, Rounded: 31.2 Value: 19.91, Rounded: 19.9 Value: 19.87, Rounded: 19.85 Value: 0.05, Rounded: 0.05 */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 25, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <cmath> #include <stdexcept> #include <string> namespace Utils { enum RoundType { Nearest, Up, Down }; /** * FUNCTION: roundAmount * USE: Rounds a number to the nearest X * @param value: The value to round * @param stepAmount: The amount to round the value by * @param type: The type of rounding to perform * @return: The value rounded by the step amount and type */ double roundAmount(double value, double stepAmount, RoundType type = RoundType::Nearest) { double inverse = 1 / stepAmount; double dividend = value * inverse; switch (type) { case RoundType::Nearest: dividend = std::round(dividend); break; case RoundType::Up: dividend = std::ceil(dividend); break; case RoundType::Down: dividend = std::floor(dividend); break; default: throw std::invalid_argument{ "Unknown type: '" + std::to_string(type) + "'" }; break; } double result = dividend / inverse; return result; } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 25, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare values to round double values[] = { 19.28, 31.22, 19.91, 19.87, 0.05 }; // Declare step amount double stepAmount = 0.05; // Round to nearest amount for (const auto& value : values) { std::cout << "Value: " << value << ", Rounded: " << Utils::roundAmount(value, stepAmount) << std::endl; } display(""); // Round up to nearest amount for (const auto& value : values) { std::cout << "Value: " << value << ", Rounded: " << Utils::roundAmount(value, stepAmount, Utils::RoundType::Up) << std::endl; } display(""); // Round down to nearest amount for (const auto& value : values) { std::cout << "Value: " << value << ", Rounded: " << Utils::roundAmount(value, stepAmount, Utils::RoundType::Down) << std::endl; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Split & Batch An Array/Vector/Container Into Smaller Sub-Lists Of N Size Using C++
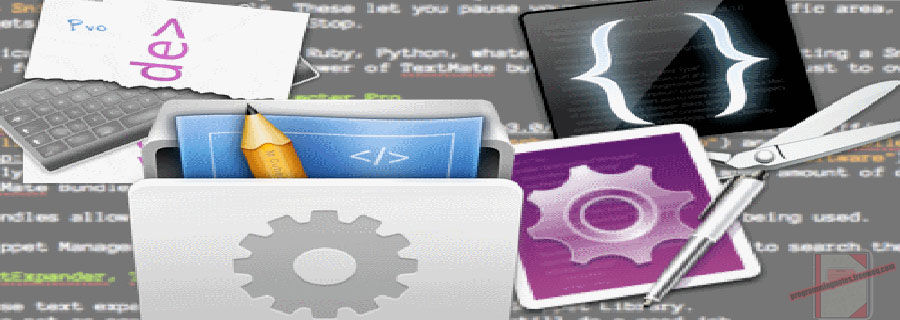
The following is a module with functions which demonstrates how to split/batch an array/vector/container into smaller sublists of n size using C++.
The function demonstrated on this page is a template, so it should work on containers of any type. It uses a simple for loop to group items into batches.
1. Partition – Integer Array
The example below demonstrates the use of ‘Utils::partition‘ to group an integer array into batches.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// Partition - Integer Array // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Split into sub groups auto numbersPartition = Utils::partition(numbers, numbers + size, 3); // Display grouped batches for (unsigned batchCount = 0; batchCount < numbersPartition.size(); ++batchCount) { auto batch = numbersPartition[batchCount]; std::cout << "Batch #" << batchCount + 1 << std::endl; for (const auto& item : batch) { std::cout << " Item: " << item << std::endl; } } // expected output: /* Batch #1 Item: 1987 Item: 19 Item: 22 Batch #2 Item: 2009 Item: 2019 Item: 1991 Batch #3 Item: 28 Item: 31 */ |
2. Partition – String Vector
The example below demonstrates the use of ‘Utils::partition‘ to group a string vector into batches.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Partition - String Vector // Declare data std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Split into sub groups auto namesPartition = Utils::partition(names.begin(), names.end(), 2); // Display grouped batches for (unsigned batchCount = 0; batchCount < namesPartition.size(); ++batchCount) { auto batch = namesPartition[batchCount]; std::cout << "Batch #" << batchCount + 1 << std::endl; for (const auto& item : batch) { std::cout << " Item: " << item << std::endl; } } // expected output: /* Batch #1 Item: Kenneth Item: Jennifer Batch #2 Item: Lynn Item: Sole */ |
3. Partition – Custom Object Vector
The example below demonstrates the use of ‘Utils::partition‘ to group a custom object vector into batches.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// Partition - Custom Object Vector // Declare object struct Person { int id; std::string name; }; // Declare data std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Split into sub groups auto peoplePartition = Utils::partition(people.begin(), people.end(), 4); // Display grouped batches for (unsigned batchCount = 0; batchCount < peoplePartition.size(); ++batchCount) { auto batch = peoplePartition[batchCount]; std::cout << "Batch #" << batchCount + 1 << std::endl; for (const auto& item : batch) { std::cout << " Item: " << item.id << " - " << item.name << std::endl; } } // expected output: /* Batch #1 Item: 31 - Kenneth Item: 28 - Jennifer Item: 87 - Lynn Item: 91 - Sole Batch #2 Item: 22 - Kenneth Item: 19 - Jennifer */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 24, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <iterator> namespace Utils { /** * FUNCTION: partition * USE: Breaks a sequence into smaller sub-lists of a specified size * in the given range [first, last) * @param first: The first position of the sequence * @param last: The last position of the sequence * @param size: The maximum size of each sub-list * @return: A container of the smaller sub-lists of the specified size */ template<typename InputIt, typename T = typename std::iterator_traits<InputIt>::value_type> std::vector<std::vector<T>> partition(InputIt first, InputIt last, unsigned size) { std::vector<std::vector<T>> result; std::vector<T>* batch{}; for (unsigned index = 0, row = 0; first != last; ++first, ++index) { if ((index % size) == 0) { result.resize(++row); batch = &result.back(); batch->reserve(size); } batch->push_back(*first); } return result; } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 24, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" // Declare object struct Person { int id; std::string name; }; void display(const std::string& message); int main() { try { // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Split into sub groups auto numbersPartition = Utils::partition(numbers, numbers + size, 3); // Display grouped batches for (unsigned batchCount = 0; batchCount < numbersPartition.size(); ++batchCount) { auto batch = numbersPartition[batchCount]; std::cout << "Batch #" << batchCount + 1 << std::endl; for (const auto& item : batch) { std::cout << " Item: " << item << std::endl; } } display(""); // Declare data std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Split into sub groups auto namesPartition = Utils::partition(names.begin(), names.end(), 2); // Display grouped batches for (unsigned batchCount = 0; batchCount < namesPartition.size(); ++batchCount) { auto batch = namesPartition[batchCount]; std::cout << "Batch #" << batchCount + 1 << std::endl; for (const auto& item : batch) { std::cout << " Item: " << item << std::endl; } } display(""); // Declare data std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Split into sub groups auto peoplePartition = Utils::partition(people.begin(), people.end(), 4); // Display grouped batches for (unsigned batchCount = 0; batchCount < peoplePartition.size(); ++batchCount) { auto batch = peoplePartition[batchCount]; std::cout << "Batch #" << batchCount + 1 << std::endl; for (const auto& item : batch) { std::cout << " Item: " << item.id << " - " << item.name << std::endl; } } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Shuffle & Randomize An Array/Vector/Container Using C++
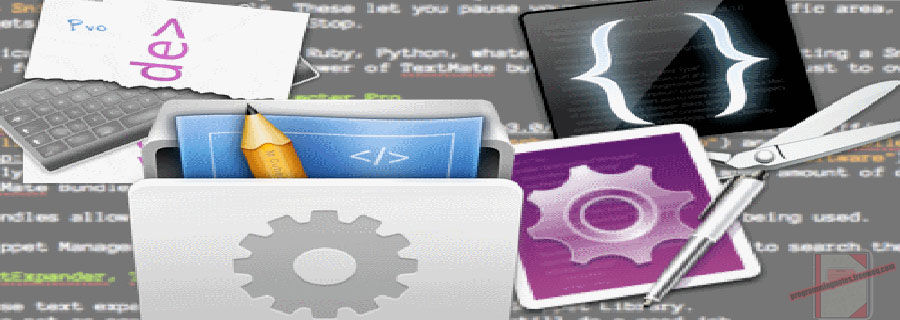
The following is a module with functions which demonstrates how to randomize and shuffle the contents of an array/vector/container using C++.
The following template function is a wrapper for the std::shuffle function.
1. Shuffle – Integer Array
The example below demonstrates the use of ‘Utils::shuffle‘ to randomize an integer array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Shuffle - Integer Array // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Get results Utils::shuffle(numbers, numbers + size); // Display results for (const auto& item : numbers) { std::cout << item << std::endl; } // example output: /* 28 1991 22 1987 19 2019 2009 31 */ |
2. Shuffle – String Vector
The example below demonstrates the use of ‘Utils::shuffle‘ to randomize a string vector.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// Shuffle - String Vector // Declare names std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Get results Utils::shuffle(names.begin(), names.end()); // Display results for (const auto& item : names) { std::cout << item << std::endl; } // example output: /* Jennifer Sole Kenneth Lynn */ |
3. Shuffle – Custom Object Vector
The example below demonstrates the use of ‘Utils::shuffle‘ to randomize a custom object vector.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// Shuffle - Custom Object Vector // Declare object struct Person { int id; std::string name; }; // Declare names std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Get results Utils::shuffle(people.begin(), people.end()); // Display results for (const auto& item : people) { std::cout << item.id << " - " << item.name << std::endl; } // example output: /* 28 - Jennifer 19 - Jennifer 22 - Kenneth 87 - Lynn 91 - Sole 31 - Kenneth */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 9, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <random> #include <chrono> #include <algorithm> namespace Utils { /** * FUNCTION: shuffle * USE: Shuffles a collection in the given range [first, last) * @param first: The first position of the sequence to be sorted * @param last: The last position of the sequence to be sorted * @return: N/A */ template<typename RandomIt> void shuffle(RandomIt first, RandomIt last) { auto seed = static_cast<std::mt19937::result_type> ( std::chrono::system_clock::now().time_since_epoch().count() ); std::mt19937 generator(seed); std::shuffle(first, last, generator); } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 9, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); // Declare object struct Person { int id; std::string name; }; int main() { try { // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Get results Utils::shuffle(numbers, numbers + size); // Display results for (const auto& item : numbers) { std::cout << item << std::endl; } display(""); // Declare names std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Get results Utils::shuffle(names.begin(), names.end()); // Display results for (const auto& item : names) { std::cout << item << std::endl; } display(""); // Declare names std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Get results Utils::shuffle(people.begin(), people.end()); // Display results for (const auto& item : people) { std::cout << item.id << " - " << item.name << std::endl; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Find The Day Of The Week You Were Born Using C++
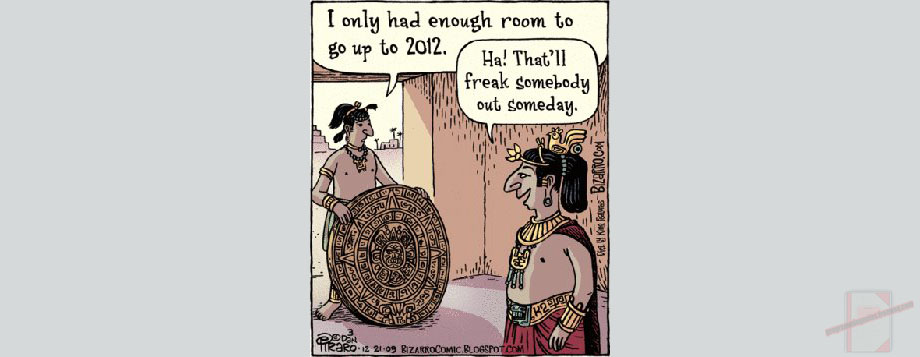
The following is a program which demonstrates how to find the day of week you were born using C++.
The program demonstrated on this page is an updated version of a previous program of the same type.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
1. Overview
This program prompts the user for their name, date of birth (month, day, year), and then displays information back to them via cout. Once the program obtains selected information from the user, it will use simple math to determine the day of the week in which the user was born, and determine the day of the week their current birthday will be for the current calendar year. The program will also display to the user their current age, along with re-displaying their name back to them.
2. When Were You Born?
Note: This program uses functions in a custom .h header file #include “Utils.h”. To obtain the code for that file, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 12, 2012 // Updated: Feb 6, 2021 // Taken From: http://programmingnotes.org/ // File: dateOfWeek.cpp // Description: Simple program to determine the day of week for a date // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" // Function prototypes std::string getMonthName(int month); void validate(int value, int lower, int upper, const std::string& desc); // A const variable containing the current year const int CURRENT_YEAR = 2021; int main() { try { // Declare variables std::string userName = ""; int month = 0; int day = 0; int year = 0; // Get user input std::cout << "Please enter your name: "; std::getline(std::cin, userName); // Get month born std::cout << "\nPlease enter the month in which you were born (between 1 and 12): "; std::cin >> month; // Validate correct month range validate(month, 1, 12, "month"); // Get day born std::cout << "\nPlease enter the day you were born (between 1 and 31): "; std::cin >> day; // Validate correct birth date range validate(day, 1, 31, "birth date"); // Get year born std::cout << "\nPlease enter the year you were born: "; std::cin >> year; // Get the month name auto monthName = getMonthName(month); // Get the week name auto weekdayName = Utils::getWeekdayName(day, month, year); // display data to user std::cout << "\nHello " << userName << ". Here are some facts about you!" << std::endl; std::cout << "You were born " << monthName << " " << day << " " << year << std::endl; std::cout << "Your birth took place on a " << weekdayName << std::endl; std::cout << "This year (" << CURRENT_YEAR << ") your birthday will take place on a " << Utils::getWeekdayName(day, month, CURRENT_YEAR) << std::endl; std::cout << "You currently are, or will be " << (CURRENT_YEAR - year) << " years old this year!" << std::endl; } catch (std::exception& e) { std::cout << "\nAn error occurred: " + std::string(e.what()) << std::endl; } std::cin.get(); return 0; } /** * FUNCTION: getMonthName * USE: Returns the name of the month for the given paremeters * (i.e month #1 = January) * @param month: The month * @return: The name of the month for the given parameters */ std::string getMonthName(int month) { std::string months[] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"}; return months[month - 1]; } void validate(int value, int lower, int upper, const std::string& desc) { if (value < lower || value > upper) { throw std::invalid_argument{ "Invalid " + desc + " entered: '" + std::to_string(value) + "'" }; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled four separate times to display the different outputs its able to produce
Please enter your name: My Programming Notes
Please enter the month in which you were born (between 1 and 12): 1
Please enter the day you were born (between 1 and 31): 1
Please enter the year you were born: 2012Hello My Programming Notes. Here are some facts about you!
You were born January 1 2012
Your birth took place on a Sunday
This year (2021) your birthday will take place on a Friday
You currently are, or will be 9 years old this year!---------------------------------------------------------------------
Please enter your name: Jennifer
Please enter the month in which you were born (between 1 and 12): 1
Please enter the day you were born (between 1 and 31): 27
Please enter the year you were born: 1991Hello Jennifer. Here are some facts about you!
You were born January 27 1991
Your birth took place on a Sunday
This year (2021) your birthday will take place on a Wednesday
You currently are, or will be 30 years old this year!---------------------------------------------------------------------
Please enter your name: Kenneth
Please enter the month in which you were born (between 1 and 12): 7
Please enter the day you were born (between 1 and 31): 28
Please enter the year you were born: 1987Hello Kenneth. Here are some facts about you!
You were born July 28 1987
Your birth took place on a Tuesday
This year (2021) your birthday will take place on a Wednesday
You currently are, or will be 34 years old this year!---------------------------------------------------------------------
Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 12
Please enter the day you were born (between 1 and 31): 35
An error occurred: Invalid birth date entered: '35'
C++ || How To Get The Day Of The Week & The Week Day Name From A Date Using C++
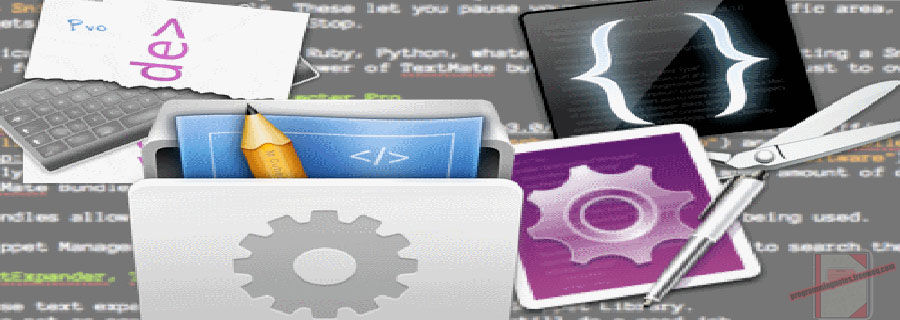
The following is a module with functions which demonstrates how to get the day of the week and the week day name from a given date using C++.
The function demonstrated on this page uses Zeller’s congruence to determine the day of the week from a given date.
1. Week Day
The example below demonstrates the use of ‘Utils::getWeekday‘ to get the day of week for a given date. The following function returns a value from a DayOfWeek enum, which represents the day of the week for the given parameters.
The following are possible values that are returned from this function:
• 0 = Sunday
• 1 = Monday
• 2 = Tuesday
• 3 = Wednesday
• 4 = Thursday
• 5 = Friday
• 6 = Saturday
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Week Day // Declare data int day = 27; int month = 1; int year = 1991; std::cout << "Day = " << day << ", Month = " << month << ", Year = " << year << std::endl; // Get the weekday auto weekday = Utils::getWeekday(day, month, year); // Display result std::cout << "Weekday = " << weekday << std::endl; // expected output: /* Day = 27, Month = 1, Year = 1991 Weekday = 0 */ |
2. Week Day Name
The example below demonstrates the use of ‘Utils::getWeekdayName‘ to get the name of the day of week for the given date.
The following are possible values that are returned from this function:
• Sunday
• Monday
• Tuesday
• Wednesday
• Thursday
• Friday
• Saturday
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Week Day Name // Declare data int day = 27; int month = 1; int year = 1991; std::cout << "Day = " << day << ", Month = " << month << ", Year = " << year << std::endl; // Get the weekday name std::string weekdayName = Utils::getWeekdayName(day, month, year); // Display result std::cout << "Weekday Name = " << weekdayName << std::endl; // expected output: /* Day = 27, Month = 1, Year = 1991 Weekday Name = Sunday */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 5, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> #include <cmath> namespace Utils { // Specifies the day of the week enum DayOfWeek { Sunday = 0, Monday = 1, Tuesday = 2, Wednesday = 3, Thursday = 4, Friday = 5, Saturday = 6 }; /** * FUNCTION: getWeekday * USE: Returns the day of week for the given parameters * @param day: The day * @param month: The month * @param year: The year * @return: The day of week for the given parameters * 0 = Sunday, 1 = Monday, 2 = Tuesday, ... 6 = Saturday */ DayOfWeek getWeekday(int day, int month, int year) { if (month == 1) { month = 13; --year; } if (month == 2) { month = 14; --year; } int q = day; int m = month; int k = year % 100; int j = static_cast<int>(std::floor(year / 100)); int h = static_cast<int>( q + (std::floor((13 * (m + 1)) / 5)) + k + (std::floor(k / 4)) + (std::floor(j / 4)) + (5 * j) ); h = ((h + 6) % 7); return static_cast<DayOfWeek>(h); } /** * FUNCTION: getWeekdayName * USE: Returns the name of the day of week for the given weekday * @param weekday: The day of the week * @return: The name of the day of week for the given weekday */ std::string getWeekdayName(DayOfWeek weekday) { std::string days[] = { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" }; return days[weekday]; } /** * FUNCTION: getWeekdayName * USE: Returns the name of the day of week for the given parameters * @param day: The day * @param month: The month * @param year: The year * @return: The name of the day of week for the given parameters */ std::string getWeekdayName(int day, int month, int year) { return getWeekdayName(getWeekday(day, month, year)); } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 5, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare data int day = 27; int month = 1; int year = 1991; display("Day = " + std::to_string(day) + ", Month = " + std::to_string(month) + ", Year = " + std::to_string(year)); // Get the weekday auto weekday = Utils::getWeekday(day, month, year); display("Weekday = " + std::to_string(weekday)); // Get the weekday name std::string weekdayName = Utils::getWeekdayName(day, month, year); display("Weekday Name = " + weekdayName); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || Simple Multi Digit, Decimal & Negative Number Infix To Postfix Conversion & Evaluation
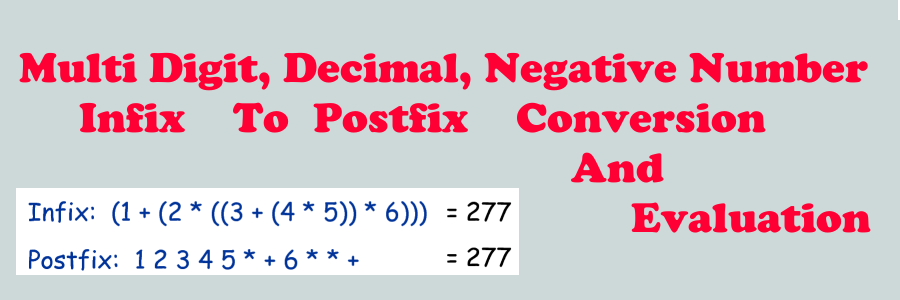
The following is sample code which demonstrates the implementation of a multi digit, decimal, and negative number infix to postfix converter and evaluator using C++.
The program demonstrated on this page has the ability to convert and evaluate a single digit, multi digit, decimal number, and/or negative number infix equation. So for example, if the the infix equation of (19.87 * -2) was entered into the program, the converted postfix expression of 19.87 -2 * would display to the screen, as well as the final evaluated answer of -39.74.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Convert Infix To Postfix
How To Evaluate A Postfix Expression
1. Overview
The program demonstrated on this page is different from a previous implementation of the same type in that this version does not use a Finite State Machine during the conversion process, which simplifies the implemetation!
This program has the following flow of control:
• Get an infix expression from the user
• Convert the infix expression to postfix & isolate all of the math operators, multi digit, decimal, negative and single digit numbers that are found in the postfix expression
• Evaluate the postfix expression by breaking the infix string into tokens found from the above step
• Display the evaluated answer to the screen
The above steps are implemented below.
2. Infix To Posfix Conversion & Evaluation
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 31, 2014 // Updated: Feb 5, 2021 // Taken From: http://programmingnotes.org/ // File: InToPostEval.cpp // Description: The following demonstrates the implementation of an infix to // postfix converter and evaluator. This program has the ability to // convert and evaluate multi digit, decimal, negative and positive values // ============================================================================ #include <iostream> #include <cstdlib> #include <cmath> #include <cctype> #include <string> #include <vector> #include <stack> #include <algorithm> #include <exception> #include <stdexcept> // function prototypes void displayDirections(); std::string convertInfixToPostfix(std::string infix); bool isMathOperator(char token); int orderOfOperations(char token); double evaluatePostfix(const std::string& postfix); double calculate(char mathOperator, double value1, double value2); bool isNumeric(char value); bool isNumeric(std::string value); std::vector<std::string> split(const std::string& source, const std::string& delimiters = " "); std::string replaceAll(const std::string& source , const std::string& oldValue, const std::string& newValue); int main() { // declare variables std::string infix = ""; // display directions to user displayDirections(); try { // get data from user std::cout << "\nPlease enter an Infix expression: "; std::getline(std::cin, infix); // convert infix to postfix std::string postfix = convertInfixToPostfix(infix); std::cout << "\nThe Infix expression = " << infix; std::cout << "\nThe Postfix expression = " << postfix << std::endl; // evaluate the postfix string double answer = evaluatePostfix(postfix); std::cout << "\nFinal answer = " << answer << std::endl; } catch (std::exception& e) { std::cout << "\nAn error occurred: " + std::string(e.what()) << std::endl; } std::cin.get(); return 0; }// end of main void displayDirections() { // this function displays instructions to the screen std::cout << "\n==== Infix To Postfix Conversion & Evaluation ====\n" << "\nMath Operators:\n" << "+ || Addition\n" << "- || Subtraction\n" << "* || Multiplication\n" << "/ || Division\n" << "% || Modulus\n" << "^ || Power\n" << "$ || Square Root\n" << "s || Sine\n" << "c || Cosine\n" << "t || Tangent\n" << "- || Negative Number\n" << "Sample Infix Equation: ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))\n"; // ((sin(-4^5)*1.4)/(sqrt(23+2)--2.8))*(cos(1%2)/(7.28*.1987)^(tan(23))) }// end of displayDirections std::string convertInfixToPostfix(std::string infix) { // this function converts an infix expression to postfix // declare function variables std::string postfix; std::stack<char> charStack; // remove all whitespace from the string infix.erase(std::remove_if(infix.begin(), infix.end(), [](char c) { return std::isspace(static_cast<unsigned char>(c)); }), infix.end()); // negate equations marked with '--' infix = replaceAll(infix, "(--", "("); // automatically convert negative numbers to have the ~ symbol. // this is done so we can distinguish negative numbers and the subtraction symbol for (unsigned x = 0; x < infix.length(); ++x) { if (infix[x] != '-') { continue; } if (x == 0 || infix[x - 1] == '(' || isMathOperator(infix[x - 1])) { infix[x] = '~'; } } // loop thru array until there is no more data for (unsigned x = 0; x < infix.length(); ++x) { // place numbers (standard, decimal, & negative) // numbers onto the 'postfix' string if (isNumeric(infix[x])) { if (postfix.length() > 0 && !isNumeric(postfix.back())) { if (!std::isspace(postfix.back())) { postfix += " "; } } postfix += infix[x]; } else if (std::isspace(infix[x])) { continue; } else if (isMathOperator(infix[x])) { if (postfix.length() > 0 && !std::isspace(postfix.back())) { postfix += " "; } // use the 'orderOfOperations' function to check equality // of the math operator at the top of the stack compared to // the current math operator in the infix string while ((!charStack.empty()) && (orderOfOperations(charStack.top()) >= orderOfOperations(infix[x]))) { // place the math operator from the top of the // stack onto the postfix string and continue the // process until complete if (postfix.length() > 0 && !std::isspace(postfix.back())) { postfix += " "; } postfix += charStack.top(); charStack.pop(); } // push the remaining math operator onto the stack charStack.push(infix[x]); } // push outer parentheses onto stack else if (infix[x] == '(') { charStack.push(infix[x]); } else if (infix[x] == ')') { // pop the current math operator from the stack while ((!charStack.empty()) && (charStack.top() != '(')) { if (postfix.length() > 0 && !std::isspace(postfix.back())) { postfix += " "; } // place the math operator onto the postfix string postfix += charStack.top(); // pop the next operator from the stack and // continue the process until complete charStack.pop(); } // pop '(' symbol off the stack if (!charStack.empty()) { charStack.pop(); } else { // no matching '(' throw std::invalid_argument{ "PARENTHESES MISMATCH" }; } } else { throw std::invalid_argument{ "INVALID INPUT" }; } } // place any remaining math operators from the stack onto // the postfix array while (!charStack.empty()) { if (charStack.top() == '(' || charStack.top() == ')') { throw std::invalid_argument{ "PARENTHESES MISMATCH" }; } if (postfix.length() > 0 && !std::isspace(postfix.back())) { postfix += " "; } postfix += charStack.top(); charStack.pop(); } // replace all '~' symbols with a minus sign postfix = replaceAll(postfix, "~", "-"); return postfix; }// end of convertInfixToPostfix bool isMathOperator(char token) { // this function checks if operand is a math operator switch (std::tolower(token)) { case '+': case '-': case '*': case '/': case '%': case '^': case '$': case 'c': case 's': case 't': return true; break; default: return false; break; } }// end of isMathOperator int orderOfOperations(char token) { // this function returns the priority of each math operator int priority = 0; switch (std::tolower(token)) { case 'c': case 's': case 't': priority = 5; break; case '^': case '$': priority = 4; break; case '*': case '/': case '%': priority = 3; break; case '-': priority = 2; break; case '+': priority = 1; break; } return priority; }// end of orderOfOperations double evaluatePostfix(const std::string& postfix) { // this function evaluates a postfix expression // declare function variables double answer = 0; std::stack<double> doubleStack; // split string into tokens to isolate multi digit, negative and decimal // numbers, aswell as single digit numbers and math operators auto tokens = split(postfix); // display the found tokens to the screen //for (unsigned x = 0; x < tokens.size(); ++x) { // std::cout<< tokens.at(x) << std::endl; //} std::cout << "\nCalculations:\n"; // loop thru array until there is no more data for (unsigned x = 0; x < tokens.size(); ++x) { auto token = tokens[x]; // push numbers & negative numbers onto the stack if (isNumeric(token)) { doubleStack.push(std::atof(token.c_str())); } // if expression is a math operator, pop numbers from stack // & send the popped numbers to the 'calculate' function else if (isMathOperator(token[0]) && (!doubleStack.empty())) { double value1 = 0; double value2 = 0; char mathOperator = static_cast<unsigned char>(std::tolower(token[0])); // if expression is square root, sin, cos, // or tan operation only pop stack once if (mathOperator == '$' || mathOperator == 's' || mathOperator == 'c' || mathOperator == 't') { value2 = 0; value1 = doubleStack.top(); doubleStack.pop(); answer = calculate(mathOperator, value1, value2); doubleStack.push(answer); } else if (doubleStack.size() > 1) { value2 = doubleStack.top(); doubleStack.pop(); value1 = doubleStack.top(); doubleStack.pop(); answer = calculate(mathOperator, value1, value2); doubleStack.push(answer); } } else { // this should never execute, & if it does, something went really wrong throw std::invalid_argument{ "INVALID POSTFIX STRING" }; } } // pop the final answer from the stack, and return to main if (!doubleStack.empty()) { answer = doubleStack.top(); } return answer; }// end of evaluatePostfix double calculate(char mathOperator, double value1, double value2) { // this function carries out the actual math process double ans = 0; switch (std::tolower(mathOperator)) { case '+': std::cout << value1 << mathOperator << value2; ans = value1 + value2; break; case '-': std::cout << value1 << mathOperator << value2; ans = value1 - value2; break; case '*': std::cout << value1 << mathOperator << value2; ans = value1 * value2; break; case '/': std::cout << value1 << mathOperator << value2; ans = value1 / value2; break; case '%': std::cout << value1 << mathOperator << value2; ans = ((int)value1 % (int)value2) + std::modf(value1, &value2); break; case '^': std::cout << value1 << mathOperator << value2; ans = std::pow(value1, value2); break; case '$': std::cout << char(251) << value1; ans = std::sqrt(value1); break; case 'c': std::cout << "cos(" << value1 << ")"; ans = std::cos(value1); break; case 's': std::cout << "sin(" << value1 << ")"; ans = std::sin(value1); break; case 't': std::cout << "tan(" << value1 << ")"; ans = std::tan(value1); break; default: ans = 0; break; } std::cout << " = " << ans << std::endl; return ans; }// end of calculate std::vector<std::string> split(const std::string& source, const std::string& delimiters) { std::size_t prev = 0; std::size_t currentPos = 0; std::vector<std::string> results; while ((currentPos = source.find_first_of(delimiters, prev)) != std::string::npos) { if (currentPos > prev) { results.push_back(source.substr(prev, currentPos - prev)); } prev = currentPos + 1; } if (prev < source.length()) { results.push_back(source.substr(prev, std::string::npos)); } return results; }// end of split std::string replaceAll(const std::string& source , const std::string& oldValue, const std::string& newValue) { if (oldValue.empty()) { return source; } std::string newString; newString.reserve(source.length()); std::size_t lastPos = 0; std::size_t findPos; while (std::string::npos != (findPos = source.find(oldValue, lastPos))) { newString.append(source, lastPos, findPos - lastPos); newString += newValue; lastPos = findPos + oldValue.length(); } newString += source.substr(lastPos); return newString; }// end of replaceAll bool isNumeric(char value) { return std::isdigit(value) || value == '.' || value == '~'; }// end of isNumeric bool isNumeric(std::string value) { for (unsigned index = 0; index < value.length(); ++index) { if (index == 0 && value[index] == '-' && value.length() > 1) { continue; } if (!isNumeric(value[index])) { return false; } } return true; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
The following is sample output.
====== RUN 1 ======
==== Infix To Postfix Conversion & Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square Root
s || Sine
c || Cosine
t || Tangent
- || Negative Number
Sample Infix Equation: ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))Please enter an Infix expression: 12/3*9
The Infix expression = 12/3*9
The Postfix expression = 12 3 / 9 *Calculations:
12/3 = 4
4*9 = 36Final answer = 36
====== RUN 2 ======
==== Infix To Postfix Conversion & Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square Root
s || Sine
c || Cosine
t || Tangent
- || Negative Number
Sample Infix Equation: ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))Please enter an Infix expression: -150.89996 - 87.56643
The Infix expression = -150.89996 - 87.56643
The Postfix expression = -150.89996 87.56643 -Calculations:
-150.9-87.5664 = -238.466Final answer = -238.466
====== RUN 3 ======
==== Infix To Postfix Conversion & Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square Root
s || Sine
c || Cosine
t || Tangent
- || Negative Number
Sample Infix Equation: ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))Please enter an Infix expression: ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))
The Infix expression = ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))
The Postfix expression = -4 5 ^ s 1.4 * 23 2 + $ -2.8 - / 1 2 % c 7.28 .1987 * 23 t ^ / *Calculations:
-4^5 = -1024
sin(-1024) = 0.158533
0.158533*1.4 = 0.221947
23+2 = 25
√25 = 5
5--2.8 = 7.8
0.221947/7.8 = 0.0284547
1%2 = 1
cos(1) = 0.540302
7.28*0.1987 = 1.44654
tan(23) = 1.58815
1.44654^1.58815 = 1.79733
0.540302/1.79733 = 0.300614
0.0284547*0.300614 = 0.00855389Final answer = 0.00855389
====== RUN 4 ======
==== Infix To Postfix Conversion & Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square Root
s || Sine
c || Cosine
t || Tangent
- || Negative Number
Sample Infix Equation: ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))Please enter an Infix expression: (1987 + 1991) * -1
The Infix expression = (1987 + 1991) * -1
The Postfix expression = 1987 1991 + -1 *Calculations:
1987+1991 = 3978
3978*-1 = -3978Final answer = -3978
====== RUN 5 ======
==== Infix To Postfix Conversion & Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square Root
s || Sine
c || Cosine
t || Tangent
- || Negative Number
Sample Infix Equation: ((s(-4^5)*1.4)/($(23+2)--2.8))*(c(1%2)/(7.28*.1987)^(t23))Please enter an Infix expression: (1+(2*((3+(4*5))*6)))
The Infix expression = (1+(2*((3+(4*5))*6)))
The Postfix expression = 1 2 3 4 5 * + 6 * * +Calculations:
4*5 = 20
3+20 = 23
23*6 = 138
2*138 = 276
1+276 = 277Final answer = 277