VB.NET || How To Send, Post & Process A REST API Web Request Using VB.NET
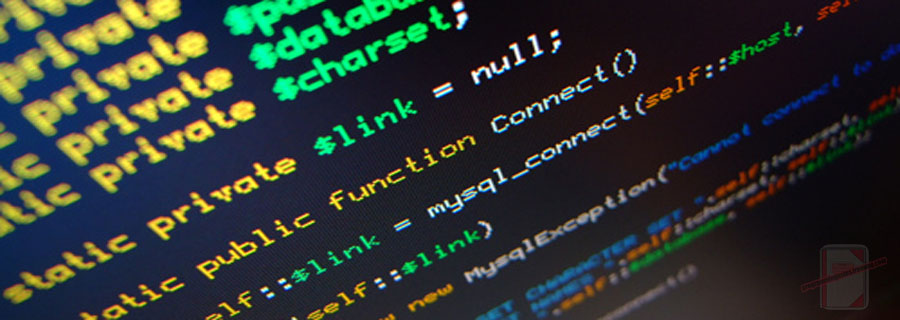
The following is a module with functions which demonstrates how to send and receive a RESTful web request using VB.NET.
Contents
1. Overview
2. WebRequest - GET
3. WebRequest - POST
4. WebRequest - PUT
5. WebRequest - PATCH
6. WebRequest - DELETE
7. Utils Namespace
8. More Examples
1. Overview
The following functions use System.Net.HttpWebRequest and System.Net.HttpWebResponse to send and process requests. They can be called synchronously or asynchronously. This page will demonstrate using the asynchronous function calls.
The examples on this page will call a test API, and the resulting calls will return Json results.
The Json objects we are sending to the API are hard coded in the examples below. In a real world application, the objects would be serialized first before they are sent over the network, and then deserialized once a response is received. For simplicity, those operations are hard coded.
For examples of how to serialize and deserialize objects using Json and XML, see below:
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
2. WebRequest – GET
The example below demonstrates the use of ‘Utils.WebRequest.Get‘ to execute a GET request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 |
' WebRequest - GET ' Declare url Dim url = "https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a get request at the following url Dim response = Await Utils.WebRequest.GetAsync(url) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 5645 ' Body: [ ' { ' "id": 1, ' "name": "Leanne Graham", ' "username": "Bret", ' "email": "[email protected]", ' "address": { ' "street": "Kulas Light", ' "suite": "Apt. 556", ' "city": "Gwenborough", ' "zipcode": "92998-3874", ' "geo": { ' "lat": "-37.3159", ' "lng": "81.1496" ' } ' }, ' "phone": "1-770-736-8031 x56442", ' "website": "hildegard.org", ' "company": { ' "name": "Romaguera-Crona", ' "catchPhrase": "Multi-layered client-server neural-net", ' "bs": "harness real-time e-markets" ' } ' }, ' { ' "id": 2, ' "name": "Ervin Howell", ' "username": "Antonette", ' "email": "[email protected]", ' "address": { ' "street": "Victor Plains", ' "suite": "Suite 879", ' "city": "Wisokyburgh", ' "zipcode": "90566-7771", ' "geo": { ' "lat": "-43.9509", ' "lng": "-34.4618" ' } ' }, ' "phone": "010-692-6593 x09125", ' "website": "anastasia.net", ' "company": { ' "name": "Deckow-Crist", ' "catchPhrase": "Proactive didactic contingency", ' "bs": "synergize scalable supply-chains" ' } ' }, ' { ' "id": 3, ' "name": "Clementine Bauch", ' "username": "Samantha", ' "email": "[email protected]", ' "address": { ' "street": "Douglas Extension", ' "suite": "Suite 847", ' "city": "McKenziehaven", ' "zipcode": "59590-4157", ' "geo": { ' "lat": "-68.6102", ' "lng": "-47.0653" ' } ' }, ' "phone": "1-463-123-4447", ' "website": "ramiro.info", ' "company": { ' "name": "Romaguera-Jacobson", ' "catchPhrase": "Face to face bifurcated interface", ' "bs": "e-enable strategic applications" ' } ' }, ' { ' "id": 4, ' "name": "Patricia Lebsack", ' "username": "Karianne", ' "email": "[email protected]", ' "address": { ' "street": "Hoeger Mall", ' "suite": "Apt. 692", ' "city": "South Elvis", ' "zipcode": "53919-4257", ' "geo": { ' "lat": "29.4572", ' "lng": "-164.2990" ' } ' }, ' "phone": "493-170-9623 x156", ' "website": "kale.biz", ' "company": { ' "name": "Robel-Corkery", ' "catchPhrase": "Multi-tiered zero tolerance productivity", ' "bs": "transition cutting-edge web services" ' } ' }, ' { ' "id": 5, ' "name": "Chelsey Dietrich", ' "username": "Kamren", ' "email": "[email protected]", ' "address": { ' "street": "Skiles Walks", ' "suite": "Suite 351", ' "city": "Roscoeview", ' "zipcode": "33263", ' "geo": { ' "lat": "-31.8129", ' "lng": "62.5342" ' } ' }, ' "phone": "(254)954-1289", ' "website": "demarco.info", ' "company": { ' "name": "Keebler LLC", ' "catchPhrase": "User-centric fault-tolerant solution", ' "bs": "revolutionize end-to-end systems" ' } ' }, ' { ' "id": 6, ' "name": "Mrs. Dennis Schulist", ' "username": "Leopoldo_Corkery", ' "email": "[email protected]", ' "address": { ' "street": "Norberto Crossing", ' "suite": "Apt. 950", ' "city": "South Christy", ' "zipcode": "23505-1337", ' "geo": { ' "lat": "-71.4197", ' "lng": "71.7478" ' } ' }, ' "phone": "1-477-935-8478 x6430", ' "website": "ola.org", ' "company": { ' "name": "Considine-Lockman", ' "catchPhrase": "Synchronised bottom-line interface", ' "bs": "e-enable innovative applications" ' } ' }, ' { ' "id": 7, ' "name": "Kurtis Weissnat", ' "username": "Elwyn.Skiles", ' "email": "[email protected]", ' "address": { ' "street": "Rex Trail", ' "suite": "Suite 280", ' "city": "Howemouth", ' "zipcode": "58804-1099", ' "geo": { ' "lat": "24.8918", ' "lng": "21.8984" ' } ' }, ' "phone": "210.067.6132", ' "website": "elvis.io", ' "company": { ' "name": "Johns Group", ' "catchPhrase": "Configurable multimedia task-force", ' "bs": "generate enterprise e-tailers" ' } ' }, ' { ' "id": 8, ' "name": "Nicholas Runolfsdottir V", ' "username": "Maxime_Nienow", ' "email": "[email protected]", ' "address": { ' "street": "Ellsworth Summit", ' "suite": "Suite 729", ' "city": "Aliyaview", ' "zipcode": "45169", ' "geo": { ' "lat": "-14.3990", ' "lng": "-120.7677" ' } ' }, ' "phone": "586.493.6943 x140", ' "website": "jacynthe.com", ' "company": { ' "name": "Abernathy Group", ' "catchPhrase": "Implemented secondary concept", ' "bs": "e-enable extensible e-tailers" ' } ' }, ' { ' "id": 9, ' "name": "Glenna Reichert", ' "username": "Delphine", ' "email": "[email protected]", ' "address": { ' "street": "Dayna Park", ' "suite": "Suite 449", ' "city": "Bartholomebury", ' "zipcode": "76495-3109", ' "geo": { ' "lat": "24.6463", ' "lng": "-168.8889" ' } ' }, ' "phone": "(775)976-6794 x41206", ' "website": "conrad.com", ' "company": { ' "name": "Yost and Sons", ' "catchPhrase": "Switchable contextually-based project", ' "bs": "aggregate real-time technologies" ' } ' }, ' { ' "id": 10, ' "name": "Clementina DuBuque", ' "username": "Moriah.Stanton", ' "email": "[email protected]", ' "address": { ' "street": "Kattie Turnpike", ' "suite": "Suite 198", ' "city": "Lebsackbury", ' "zipcode": "31428-2261", ' "geo": { ' "lat": "-38.2386", ' "lng": "57.2232" ' } ' }, ' "phone": "024-648-3804", ' "website": "ambrose.net", ' "company": { ' "name": "Hoeger LLC", ' "catchPhrase": "Centralized empowering task-force", ' "bs": "target end-to-end models" ' } ' } ' ] |
3. WebRequest – POST
The example below demonstrates the use of ‘Utils.WebRequest.Post‘ to execute a POST request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
' WebRequest - POST ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to create a new employee Dim payload = " { ""id"": 0, ""name"": ""Kenneth Perkins"", ""username"": null, ""email"": null, ""address"": null, ""phone"": null, ""website"": null, ""company"": null } " ' Execute a post request at the following url Dim response = Await Utils.WebRequest.PostAsync(url, payload, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 201 - Created ' Bytes Received: 154 ' Body: { ' "id": 11, ' "name": "Kenneth Perkins", ' "username": null, ' "email": null, ' "address": null, ' "phone": null, ' "website": null, ' "company": null ' } |
4. WebRequest – PUT
The example below demonstrates the use of ‘Utils.WebRequest.Put‘ to execute a PUT request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
' WebRequest - PUT ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a put request at the following url Dim response = Await Utils.WebRequest.PutAsync(url, payload, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 87 ' Body: { ' "name": "Kenneth Perkins", ' "website": "https://www.programmingnotes.org/", ' "id": 1 ' } |
5. WebRequest – PATCH
The example below demonstrates the use of ‘Utils.WebRequest.Patch‘ to execute a PATCH request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
' WebRequest - PATCH ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a patch request at the following url Dim response = Await Utils.WebRequest.PatchAsync(url, payload, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 526 ' Body: { ' "id": 1, ' "name": "Kenneth Perkins", ' "username": "Bret", ' "email": "[email protected]", ' "address": { ' "street": "Kulas Light", ' "suite": "Apt. 556", ' "city": "Gwenborough", ' "zipcode": "92998-3874", ' "geo": { ' "lat": "-37.3159", ' "lng": "81.1496" ' } ' }, ' "phone": "1-770-736-8031 x56442", ' "website": "https://www.programmingnotes.org/", ' "company": { ' "name": "Romaguera-Crona", ' "catchPhrase": "Multi-layered client-server neural-net", ' "bs": "harness real-time e-markets" ' } ' } |
6. WebRequest – DELETE
The example below demonstrates the use of ‘Utils.WebRequest.Delete‘ to execute a DELETE request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
' WebRequest - DELETE ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a delete request at the following url Dim response = Await Utils.WebRequest.DeleteAsync(url, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 2 ' Body: {} |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 18, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Imports System Imports System.Linq Imports System.Threading.Tasks Namespace Global.Utils Namespace WebRequest Public Module modRequest ''' <summary> ''' Executes a GET request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function [Get](url As String _ , Optional options As Options = Nothing) As Response Return GetAsync(url, options:=options).Result End Function ''' <summary> ''' Executes a GET request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function GetAsync(url As String _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Get, url, payload:=CType(Nothing, Byte()), options:=options) End Function ''' <summary> ''' Executes a POST request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Post(url As String, payload As String _ , Optional options As Options = Nothing) As Response Return Post(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a POST request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Post(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Response Return PostAsync(url, payload:=payload, options:=options).Result End Function ''' <summary> ''' Executes a POST request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PostAsync(url As String, payload As String _ , Optional options As Options = Nothing) As Task(Of Response) Return PostAsync(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a POST request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PostAsync(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Post, url, payload:=payload, options:=options) End Function ''' <summary> ''' Executes a PUT request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Put(url As String, payload As String _ , Optional options As Options = Nothing) As Response Return Put(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PUT request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Put(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Response Return PutAsync(url, payload:=payload, options:=options).Result End Function ''' <summary> ''' Executes a PUT request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PutAsync(url As String, payload As String _ , Optional options As Options = Nothing) As Task(Of Response) Return PutAsync(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PUT request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PutAsync(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Put, url, payload:=payload, options:=options) End Function ''' <summary> ''' Executes a PATCH request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Patch(url As String, payload As String _ , Optional options As Options = Nothing) As Response Return Patch(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PATCH request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Patch(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Response Return PatchAsync(url, payload:=payload, options:=options).Result End Function ''' <summary> ''' Executes a PATCH request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PatchAsync(url As String, payload As String _ , Optional options As Options = Nothing) As Task(Of Response) Return PatchAsync(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PATCH request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PatchAsync(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Patch, url, payload:=payload, options:=options) End Function ''' <summary> ''' Executes a DELETE request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Delete(url As String _ , Optional options As Options = Nothing) As Response Return DeleteAsync(url, options:=options).Result End Function ''' <summary> ''' Executes a DELETE request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function DeleteAsync(url As String _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Delete, url, payload:=CType(Nothing, Byte()), options:=options) End Function ''' <summary> ''' Executes a request method on the given url as an asynchronous operation ''' </summary> ''' <param name="type">The type of request method to execute</param> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to send to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function ExecuteAsync(type As Method _ , url As String _ , Optional payload As String = Nothing _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(type, url, payload:=CType(payload?.GetBytes, Byte()), options:=options) End Function ''' <summary> ''' Executes a request method on the given url as an asynchronous operation ''' </summary> ''' <param name="type">The type of request method to execute</param> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to send to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Async Function ExecuteAsync(type As Method _ , url As String _ , Optional payload As Byte() = Nothing _ , Optional options As Options = Nothing) As Task(Of Response) Dim request = CType(System.Net.WebRequest.Create(url), System.Net.HttpWebRequest) If options IsNot Nothing Then request.CopyProperties(options) End If request.Method = type.ToString.ToUpper If payload IsNot Nothing Then request.ContentLength = payload.Length Using requestStream = request.GetRequestStream Using payloadStream = New System.IO.MemoryStream(payload) payloadStream.CopyTo(requestStream) End Using End Using End If Dim webResponse As System.Net.HttpWebResponse = Nothing Try webResponse = CType(Await request.GetResponseAsync(), System.Net.HttpWebResponse) Catch ex As System.Net.WebException If ex.Response Is Nothing Then Throw End If webResponse = CType(ex.Response, System.Net.HttpWebResponse) Catch ex As Exception Throw End Try Dim result = New Response With { .Result = webResponse, .Bytes = GetBytes(webResponse) } Return result End Function Private Function GetBytes(response As System.Net.HttpWebResponse) As Byte() Dim bytes As Byte() Dim responseStream = response.GetResponseStream() Using memoryStream = New System.IO.MemoryStream responseStream.CopyTo(memoryStream) bytes = memoryStream.ToArray End Using Return bytes End Function <Runtime.CompilerServices.Extension()> Public Function GetBytes(str As String, Optional encode As System.Text.Encoding = Nothing) As Byte() If encode Is Nothing Then encode = GetDefaultEncoding() End If Return encode.GetBytes(str) End Function <Runtime.CompilerServices.Extension()> Public Function GetString(bytes As Byte(), Optional encode As System.Text.Encoding = Nothing) As String If encode Is Nothing Then encode = GetDefaultEncoding() End If Return encode.GetString(bytes) End Function Private Function GetDefaultEncoding() As System.Text.Encoding Static encode As New System.Text.UTF8Encoding Return encode End Function <Runtime.CompilerServices.Extension()> Private Sub CopyProperties(Of T1, T2)(dest As T1, src As T2) Dim srcProps = src.GetType().GetProperties() Dim destProps = dest.GetType().GetProperties() For Each srcProp In srcProps If srcProp.CanRead Then Dim destProp = destProps.FirstOrDefault(Function(x) x.Name = srcProp.Name) If destProp IsNot Nothing AndAlso destProp.CanWrite Then Dim value = srcProp.GetValue(src, If(srcProp.GetIndexParameters.Count = 1, New Object() {Nothing}, Nothing)) destProp.SetValue(dest, value) End If End If Next End Sub End Module ''' <summary> ''' The response result of a <see cref="System.Net.HttpWebRequest"/> ''' </summary> Public Class Response Public Property Result As System.Net.HttpWebResponse = Nothing Public Property Bytes As Byte() = Nothing Public ReadOnly Property Body As String Get If _body Is Nothing AndAlso Bytes IsNot Nothing Then _body = Bytes.GetString() End If Return _body End Get End Property Private _body As String = Nothing End Class ''' <summary> ''' Options for the given <see cref="System.Net.HttpWebRequest"/> ''' </summary> Public Class Options Public Property Headers As New System.Net.WebHeaderCollection Public Property Credentials As System.Net.ICredentials = Nothing Public Property Connection As String = Nothing Public Property KeepAlive As Boolean = True Public Property Expect As String = Nothing Public Property IfModifiedSince As Date Public Property TransferEncoding As String Public Property Accept As String = Nothing Public Property AllowAutoRedirect As Boolean = True Public Property AllowReadStreamBuffering As Boolean = False Public Property AllowWriteStreamBuffering As Boolean = True Public Property MaximumAutomaticRedirections As Integer = 50 Public Property MediaType As String = Nothing Public Property Pipelined As Boolean = True Public Property PreAuthenticate As Boolean = False Public Property Referer As String = Nothing Public Property SendChunked As Boolean = False Public Property UseDefaultCredentials As Boolean = False Public Property UserAgent As String = Nothing Public Property ContentType As String = Nothing Public Property Timeout As Integer = 100000 Public Property ReadWriteTimeout As Integer = 300000 End Class Public MustInherit Class ContentType Public Const ApplicationUrlEncoded As String = "application/x-www-form-urlencoded" Public Const ApplicationJson As String = "application/json" Public Const TextXml As String = "text/xml" End Class Public Enum Method [Get] Post Put Patch Delete End Enum End Namespace End Namespace ' http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 18, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ProcessRequests().Wait() Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Async Function ProcessRequests() As Task ' Declare url Dim url = "https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a get request at the following url Dim response = Await Utils.WebRequest.GetAsync(url) ' Display the status code and response body Display($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") Display("") ' Declare url Dim url2 = $"https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options2 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to get the Json to create a new employee Dim payload = " { ""id"": 0, ""name"": ""Kenneth Perkins"", ""username"": null, ""email"": null, ""address"": null, ""phone"": null, ""website"": null, ""company"": null } " ' Execute a post request at the following url Dim response2 = Await Utils.WebRequest.PostAsync(url2, payload, options2) ' Display the status code and response body Display($" Status: {CInt(response2.Result.StatusCode)} - {response2.Result.StatusDescription} Bytes Received: {response2.Bytes.Length} Body: {response2.Body} ") Display("") ' Declare url Dim url3 = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options3 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload3 = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a put request at the following url Dim response3 = Await Utils.WebRequest.PutAsync(url3, payload3, options3) ' Display the status code and response body Display($" Status: {CInt(response3.Result.StatusCode)} - {response3.Result.StatusDescription} Bytes Received: {response3.Bytes.Length} Body: {response3.Body} ") Display("") ' Declare url Dim employeeId = 1 Dim url4 = $"https://jsonplaceholder.typicode.com/users/{employeeId}" ' Optional: Specify request options Dim options4 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a delete request at the following url Dim response4 = Await Utils.WebRequest.DeleteAsync(url4, options4) ' Display the status code and response body Display($" Status: {CInt(response4.Result.StatusCode)} - {response4.Result.StatusDescription} Bytes Received: {response4.Bytes.Length} Body: {response4.Body} ") Display("") ' Declare url Dim url5 = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options5 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload5 = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a patch request at the following url Dim response5 = Await Utils.WebRequest.PatchAsync(url5, payload5, options5) ' Display the status code and response body Display($" Status: {CInt(response5.Result.StatusCode)} - {response5.Result.StatusDescription} Bytes Received: {response5.Bytes.Length} Body: {response5.Body} ") End Function Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
This has been out here for a while. I would love to use it, but when I add the Utils Namespace to my project, it does not compile. The error is ‘Task’ is not defined.
Hi, thanks for visiting!
‘Task’ is defined in System.Threading.Tasks. I have added the import to the Utils Namespace in case anyone else runs into that issue.
Thanks for pointing that out!
I am testing an application using your utils module. I am making a Post call to an eBay api. Watching the call in Fiddler I can see that it is made successfully, and returns a response, but as soon as I try to get the response body the console app exits. I am using your example Post code to view the response. Any thoughts?
Hi Douglas,
If you are running the example in a console application, it could be that the program is ending before you can see the results displayed in the console window. Click here for some ideas how you can prevent the window from automatically closing, or see the full code snippet example posted on this page under the “More Examples” section.
Thank you for your reply to my previous question. I added the console.writeline statements to my code in the finally portion of the try catch. In stepping through my test console app it closes on the line “webResponse = CType(Await request.GetResponseAsync(), System.Net.HttpWebResponse)” in your function Public Async Function ExecuteAsync(type As Method _
, url As String _
, Optional payload As Byte() = Nothing _
, Optional options As Options = Nothing) As Task(Of Response). I did add the finally statement in the try catch but the app closed without hitting it. The call did return a response as I was watching the network traffic with Fiddler, but for some reason the console app terminates before it returns the response to my code.
Thank you. I finally really did look at your examples and saw the ProcessRequests().Wait() and Public Async Function ProcessRequests() As Task. I was missing the “.Wait” and “Public Async Function ProcessRequests() As Task” Would help if I really looked at what you were doing. My test works now!
Glad everything worked out! Just a note. If your program doesn’t need to run asynchronously, you can use the non async functions in the Namespace. They work the same as the asynchronous versions, only they don’t need to be awaited.
Thanks for visiting!
Hi admin,
I am new in VB .NET, but i love to explore your examples.
Sorry, but i dont know how am i going to add this Utils namespace in my vb application?
THanks a lot.
I’m getting “Unsupported Media Type” error. Please help. Thank you
Hi
The Unsupported Media Type is a response code that indicates that the server refused to accept the request because the payload format is in an unsupported format.
Try setting the content type in the web request to a format that the server is expecting.
Here’s how to do it for a Post request:
Hope this helps