Monthly Archives: July 2020
JavaScript || FileIO.js – Open, Save, & Read Local & Remote Files Using Vanilla JavaScript
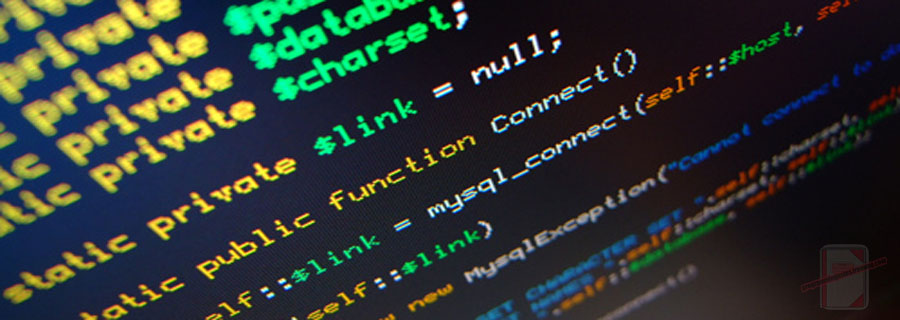
The following is a module that handles opening, reading, and saving files in JavaScript. This allows for file manipulation on the client side.
Contents
1. Open File Dialog
2. Reading Files
3. Saving Files
4. Reading Remote URL Files
5. Saving Remote URL Files
6. FileIO.js Namespace
7. More Examples
1. Open File Dialog
Syntax is very straightforward. The following demonstrates opening a file dialog to get files from the user.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
// Open a file dialog prompt to select files. <script> (async () => { // An array of File objects is returned. let files = await FileIO.openDialog({ contentTypes: '.json, text/csv, image/*', // Optional. The accepted file types allowMultiple: true, // Optional. Allows multiple file selection. Default is false }); // ... Do something with the selected files files.forEach((file, index) => { console.log(`File #${index + 1}: ${file.name}`); }); })(); </script> |
‘FileIO.openDialog‘ returns an array of File objects that contains the selected files after the user hits submit. You can specify the accepted file types, as well as if selecting multiple files is allowed or not.
2. Reading Files
The following example demonstrates how to read a file. This allows to read file contents.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Read file contents. <script> (async () => { // By default, file data is read as text. // To read a file as a Uint8Array, // set 'readAsTypedArray' to true let contents = await FileIO.read({ data: file, // File/Blob encoding: 'ISO-8859-1', // Optional. If reading as text, set the encoding. Default is UTF-8 readAsTypedArray: false, // Optional. Specifies to read the file as a Uint8Array. Default is false onProgressChange: (progressEvent) => { // Optional. Fires periodically as the file is read let percentLoaded = Number(((progressEvent.loaded / progressEvent.total) * 100).toFixed(2)); console.log(`Percent Loaded: ${percentLoaded}`); } }); // ... Do something with the file contents console.log(contents); })(); </script> |
‘FileIO.read‘ accepts either a File, or a Blob as acceptable data to be read.
By default, ‘FileIO.read‘ will read the file contents as text. To read file contents as an array, set the property ‘readAsTypedArray‘ to true. A Uint8Array is returned when this option is set to true.
3. Saving Files
The following example demonstrates how to create and save a file. This allows to save contents to a file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
// Save data to a file. <script> (() => { let text = 'My Programming Notes is awesome!'; let filename = 'myProgrammingNotes.txt' // The data to be saved can be a String/Blob/File/Uint8Array let data = text; // Example demonstrating the other datatypes that can be saved //data = new Blob([text], {type: "text/plain;charset=utf-8"}); //data = new File([text], filename, {type: "text/plain;charset=utf-8"}); //data = FileIO.stringToTypedArray(text); // Save the data to a file FileIO.save({ data: data, // String/Blob/File/Uint8Array filename: filename, // Optional if data is a File object decodeBase64Data: false, // Optional. If data is a base64 string, specifies if it should be decoded before saving. Default is false. }); // ... The file is saved to the client })(); </script> |
‘FileIO.save‘ accepts either a String, Blob, File, or a Uint8Array as acceptable data to be saved.
If the data to be saved is a base64 string, set the property ‘decodeBase64Data‘ to true in order to decode the string before saving. If the bease64 string is a file, this will allow the actual file to be decoded and saved to the client. Note: This action is only performed if the data to be saved is a string.
4. Reading Remote URL Files
The following example demonstrates how ‘FileIO.read‘ can be used to read downloaded file contents from a remote URL.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// Read file contents from a remote URL. <script> (async () => { // Remote file url let url = 'https://www.w3.org/TR/PNG/iso_8859-1.txt'; // Other example files //url = 'http://samplecsvs.s3.amazonaws.com/Sacramentorealestatetransactions.csv'; //url = 'http://dummy.restapiexample.com/api/v1/employees'; // Fetch the URL let response = await fetch(url); // Get the response file data let blob = await response.blob(); // Read the remote file as text let contents = await FileIO.read({ data: blob }); // ... Do something with the file contents console.log(contents); })(); </script> |
The example above uses fetch to retrieve data from the remote URL. Once the data is received, ‘FileIO.read‘ can be used to read its file contents.
5. Saving Remote URL Files
The following example demonstrates how ‘FileIO.save‘ can be used to save downloaded file contents from a remote URL to the client.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// Save file contents from a remote URL. <script> (async () => { // Remote file url let url = 'https://www.w3.org/TR/PNG/iso_8859-1.txt'; // Other example files //url = 'https://www.nba.com/lakers/sites/lakers/files/lakers_logo_500.png'; //url = 'http://www.africau.edu/images/default/sample.pdf'; //url = 'https://gahp.net/wp-content/uploads/2017/09/sample.pdf' //url = 'http://samplecsvs.s3.amazonaws.com/Sacramentorealestatetransactions.csv'; //url = 'https://file-examples-com.github.io/uploads/2017/02/file-sample_1MB.docx'; // Fetch the URL let response = await fetch(url); // Get the response file data let blob = await response.blob(); // Save the remote file to the client FileIO.save({ data: blob, filename: FileIO.getFilename(url) }); // ... The file is saved to the client })(); </script> |
The example above uses fetch to retrieve data from the remote URL. Once the data is received, ‘FileIO.save‘ can be used to save its file contents.
6. FileIO.js Namespace
The following is the FileIO.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 17, 2020 // Taken From: http://programmingnotes.org/ // File: FileIO.js // Description: Namespace which handles opening, reading and saving files // Example: // // 1. Save data to a file // FileIO.save({ // data: 'My Programming Notes is awesome!', // filename: 'notes.txt', // decodeBase64Data: false, // optional // }); // // // 2. Get files // let files = await FileIO.openDialog({ // contentTypes: '.txt, .json, .doc, .png', // optional // allowMultiple: true, // optional // }); // // // 3. Read file contents // let contents = await FileIO.read({ // data: file, // encoding: 'UTF-8', // optional // readAsTypedArray: false, // optional // }); // ============================================================================ /** * NAMESPACE: FileIO * USE: Handles opening, reading and saving files. */ var FileIO = FileIO || {}; (function(namespace) { 'use strict'; // -- Public data -- // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: save * USE: Exports data to be saved to the client * @param options: An object of file save options. * Its made up of the following properties: * { * data: The data (String/Blob/File/Uint8Array) to be saved. * filename: Optional. The name of the file * decodeBase64Data: Optional. If the data to be saved is not * a Blob type, it will be converted to one. If the data * being converted is a base64 string, this specifies * if the string should be decoded or not when converting * to a Blob. * } * @return: N/A. */ exposed.save = (options) => { if (isNull(options)) { throw new TypeError('There are no options specified'); } else if (isNull(options.data)) { throw new TypeError('There is no data is specified to save'); } let blob = options.data; let decodeBase64Data = options.decodeBase64Data || false; // Check to see if the file data is a blob. // Try to convert it if not if (!isBlob(blob)) { blob = exposed.convertToBlob(blob, decodeBase64Data); } let filename = options.filename || blob.name || 'FileIO_Download'; let urlCreator = window.URL || window.webkitURL; let url = urlCreator.createObjectURL(blob); let a = document.createElement('a'); a.download = filename; a.href = url; a.style.display = 'none'; a.rel = 'noopener'; a.target = '_blank'; document.body.appendChild(a); elementClick(a); setTimeout(() => { document.body.removeChild(a); urlCreator.revokeObjectURL(url) }, 250); } /** * FUNCTION: openDialog * USE: Opens a file dialog box which enables the user to select a file * @param options: An object of file dialog options. * Its made up of the following properties: * { * contentTypes: Optional. A comma separated string of * acceptable content types. * allowMultiple: Optional. Boolean indicating if the user * can select multple files. Default is false. * } * @return: A promise that returns the selected files when the user hits submit. */ exposed.openDialog = (options) => { return new Promise((resolve, reject) => { try { if (isNull(options)) { options = {}; } // Check to see if content types is an array let contentTypes = options.contentTypes || ''; if (!isNull(contentTypes) && !Array.isArray(contentTypes)) { contentTypes = contentTypes.split(','); } if (!isNull(contentTypes)) { contentTypes = contentTypes.join(','); } let input = document.createElement('input'); input.type = 'file'; input.multiple = options.allowMultiple || false; input.accept = contentTypes; input.addEventListener('change', (e) => { // Convert FileList to an array let files = Array.prototype.slice.call(input.files); resolve(files); }); input.style.display = 'none'; document.body.appendChild(input); elementClick(input); setTimeout(() => { document.body.removeChild(input); }, 250); } catch (e) { let message = e.message ? e.message : e; reject(`Failed to open dialog. Reason: ${message}`); } }); } /** * FUNCTION: read * USE: Reads a file and gets its file contents * @param options: An object of file read options. * Its made up of the following properties: * { * data: The data (File/Blob) to be read. * encoding: Optional. String that indicates the file encoding * when opening a file while reading as text. Default is UTF-8. * readAsText is the default behavior. * readAsTypedArray: Optional. Boolean that indicates if the file should * be read as a typed array. If this option is specified, a Uint8Array * object is returned on file read. * onProgressChange(progressEvent): Function that is fired periodically as * the FileReader reads data. * } * @return: A promise that returns the file contents after successful file read. */ exposed.read = (options) => { return new Promise((resolve, reject) => { try { if (!(window && window.File && window.FileList && window.FileReader)) { throw new TypeError('Unable to read. Your environment does not support File API.'); } else if (isNull(options)) { // Check to see if there are options throw new TypeError('There are no options specified.'); } else if (isNull(options.data)) { // Check to see if a file is specified throw new TypeError('Unable to read. There is no data specified.'); } else if (!isBlob(options.data)) { // Check to see if a file is specified throw new TypeError(`Unable to read data of type: ${typeof options.data}. Reason: '${options.data}' is not a Blob/File.`); } else if (!isNull(options.onProgressChange) && !isFunction(options.onProgressChange)) { // Check to see if progress change callback is a function throw new TypeError(`Unable to call onProgressChange of type: ${typeof options.onProgressChange}. Reason: '${options.onProgressChange}' is not a function.`); } let file = options.data; let encoding = options.encoding || 'UTF-8'; let readAsTypedArray = options.readAsTypedArray || false; // Setting up the reader let reader = new FileReader(); // Tell the reader what to do when it's done reading reader.addEventListener('load', (e) => { let content = e.target.result; if (readAsTypedArray) { content = new Uint8Array(content) } resolve(content); }); // Return the error reader.addEventListener('error', (e) => { reject(reader.error); }); // Call the on progress change function if specified if (options.onProgressChange) { let events = ['loadstart', 'loadend', 'progress']; events.forEach((eventName) => { reader.addEventListener(eventName, (e) => { options.onProgressChange.call(this, e); }); }); } // Begin reading the file if (readAsTypedArray) { reader.readAsArrayBuffer(file); } else { reader.readAsText(file, encoding); } } catch (e) { let message = e.message ? e.message : e; reject(`Failed to read data. Reason: ${message}`); } }); } /** * FUNCTION: convertToBlob * USE: Converts data to a Blob * @param data: Data to be converted to a Blob. * @param decodeBase64Data: Indicates if the data should be base64 decoded. * @return: The data converted to a Blob. */ exposed.convertToBlob = (data, decodeBase64Data = false) => { let arry = data; if (!isTypedArray(arry)) { arry = decodeBase64Data ? exposed.base64ToTypedArray(data) : exposed.stringToTypedArray(data); } let blob = new Blob([arry]); return blob; } /** * FUNCTION: stringToTypedArray * USE: Converts a string to a typed array (Uint8Array) * @param data: String to be converted to a typed array. * @return: The data converted to a typed array. */ exposed.stringToTypedArray = (data) => { let arry = new Uint8Array(data.length); for (let index = 0; index < data.length; ++index) { arry[index] = data.charCodeAt(index); } return arry; } /** * FUNCTION: typedArrayToString * USE: Converts a typed array to a string * @param data: Typed array to be converted to a string. * @return: The data converted to a string. */ exposed.typedArrayToString = (data) => { let str = ''; for (let index = 0; index < data.length; ++index) { str += String.fromCharCode(data[index]); } return str; } /** * FUNCTION: base64ToTypedArray * USE: Converts a base64 string to a typed array * @param data: Base64 string to be converted to a typed array. * @return: The data converted to a typed array. */ exposed.base64ToTypedArray = (data) => { let str = atob(data); let arry = exposed.stringToTypedArray(str); return arry; } /** * FUNCTION: typedArrayToBase64 * USE: Converts a typed array to a base64 string * @param data: Typed array to be converted to a base64 string. * @return: The data converted to a base64 string. */ exposed.typedArrayToBase64 = (data) => { let str = exposed.typedArrayToString(data); return btoa(str); } /** * FUNCTION: getFilename * USE: Returns the filename of a url * @param url: The url. * @return: The filename of a url. */ exposed.getFilename = (url) => { let filename = ''; if (url && url.length > 0) { filename = url.split('\\').pop().split('/').pop(); // Remove any querystring if (filename.indexOf('?') > -1) { filename = filename.substr(0, filename.indexOf('?')); } } return filename; } /** * FUNCTION: getExtension * USE: Returns the file extension of the filename of a url * @param url: The url. * @return: The file extension of a url. */ exposed.getExtension = (url) => { let filename = exposed.getFilename(url); let ext = filename.split('.').pop(); return (ext === filename) ? '' : ext; } // -- Private data -- let isTypedArray = (item) => { return item && ArrayBuffer.isView(item) && !(item instanceof DataView); } let isBlob = (item) => { return item instanceof Blob; } let isNull = (item) => { return undefined === item || null === item } let isFunction = (item) => { return 'function' === typeof item } let elementClick = (element) => { try { element.dispatchEvent(new MouseEvent('click')) } catch (e) { let evt = document.createEvent('MouseEvents') evt.initMouseEvent('click', true, true, window, 0, 0, 0, 80, 20, false, false, false, false, 0, null) element.dispatchEvent(evt) } } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(FileIO)); // http://programmingnotes.org/ |
7. More Examples
Below are more examples demonstrating the use of ‘FileIO.js‘. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Jul 17, 2020 // Taken From: http://programmingnotes.org/ // File: fileIODemo.html // Description: Demonstrates the use of FileIO.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes FileIO.js Demo</title> <style> .main { text-align:center; margin-left:auto; margin-right:auto; } .button { padding: 5px; background-color: #d2d2d2; height:100%; text-align:center; text-decoration:none; color:black; display: flex; justify-content: center; align-items: center; flex-direction: column; border-radius: 15px; cursor: pointer; width: 120px; } .button:hover { background-color:#bdbdbd; } .inline { display:inline-block; } .image-section, .text-section, .video-section { width: 60%; vertical-align: text-top; margin: 15px; } .imageContainer, .textContainer, .videoContainer { text-align: left; min-height: 100px; max-height: 350px; overflow: auto; } .text-section { width: 30%; } .textContainer { border: 1px solid black; padding: 10px; } .video-section { width: 100%; } .videoContainer { text-align: center; } .fileStatus { margin: 10px; display: none; } </style> <!-- // Include module --> <script type="text/javascript" src="./FileIO.js"></script> </head> <body> <div class="main"> My Programming Notes FileIO.js Demo <div style="margin: 10px;"> <div id="btnOpenFile" class="inline button"> Open File </div> <div class="fileStatus"> </div> </div> <hr /> <div class="inline image-section" > Images: <div class="imageContainer"></div> </div> <div class="inline text-section"> Text: <div class="textContainer"></div> <div style="margin: 10px;"> <div id="btnSaveText" class="inline button"> Save Text </div> </div> </div> <div class="video-section"> Video: <div class="videoContainer"></div> </div> </div> <script> // Open a file dialog prompt to select files. (async () => { return; // Opens a file dialog prompt. // An array of File objects is returned. let files = await FileIO.openDialog({ contentTypes: '.json, text/csv, image/*', // Optional. The accepted file types allowMultiple: true, // Optional. Allows multiple file selection. Default is false }); // ... Do something with the selected files files.forEach((file, index) => { console.log(`File #${index + 1}: ${file.name}`); }); })(); </script> <script> // Read file contents. (async () => { return; // By default, file data is read as text. // To read a file as a Uint8Array, // set 'readAsTypedArray' to true let contents = await FileIO.read({ data: file, // File/Blob encoding: 'ISO-8859-1', // Optional. If reading as text, set the encoding. Default is UTF-8 readAsTypedArray: false, // Optional. Specifies to read the file as a Uint8Array. Default is false onProgressChange: (progressEvent) => { // Optional. Fires periodically as the file is read let percentLoaded = Number(((progressEvent.loaded / progressEvent.total) * 100).toFixed(2)); console.log(`Percent Loaded: ${percentLoaded}`); } }); // ... Do something with the file contents console.log(contents); })(); </script> <script> // Save data to a file. (() => { return; let text = 'My Programming Notes is awesome!'; let filename = 'myProgrammingNotes.txt' // The data to be saved can be a String/Blob/File/Uint8Array let data = text; // Example demonstrating the other datatypes that can be saved //data = new Blob([text], {type: "text/plain;charset=utf-8"}); //data = new File([text], filename, {type: "text/plain;charset=utf-8"}); //data = FileIO.stringToTypedArray(text); // Save the data to a file FileIO.save({ data: data, // String/Blob/File/Uint8Array filename: filename, // Optional if data is a File object decodeBase64Data: false, // Optional. If data is a base64 string, specifies if it should be decoded before saving. Default is false. }); // ... The file is saved to the client })(); </script> <script> // Read a remote file (async () => { return; // Remote file url let url = 'https://www.w3.org/TR/PNG/iso_8859-1.txt'; // Other example files //url = 'http://samplecsvs.s3.amazonaws.com/Sacramentorealestatetransactions.csv'; //url = 'http://dummy.restapiexample.com/api/v1/employees'; //url = 'file:///C:/Users/Jr/Desktop/html%20projects/fileIOExample.html'; // Fetch the URL let response = await fetch(url); // Get the response file data let blob = await response.blob(); // Read the remote file as text let contents = await FileIO.read({ data: blob }); // ... Do something with the file contents console.log(contents); })(); </script> <script> // Save a remote file (async () => { return; // Remote file url let url = 'https://www.nba.com/lakers/sites/lakers/files/lakers_logo_500.png'; // Other example files //url = 'https://www.w3.org/TR/PNG/iso_8859-1.txt'; //url = 'http://www.africau.edu/images/default/sample.pdf'; //url = 'https://gahp.net/wp-content/uploads/2017/09/sample.pdf' //url = 'http://samplecsvs.s3.amazonaws.com/Sacramentorealestatetransactions.csv'; //url = 'https://file-examples-com.github.io/uploads/2017/02/file-sample_1MB.docx'; // Fetch the URL let response = await fetch(url); // Get the response file data let blob = await response.blob(); // Save the remote file to the client FileIO.save({ data: blob, filename: FileIO.getFilename(url) }); // ... The file is saved to the client })(); </script> <script> document.addEventListener("DOMContentLoaded", function(eventLoaded) { // Open file document.querySelector('#btnOpenFile').addEventListener('click', async (e) => { // Open a file dialog prompt to select a file let files = await FileIO.openDialog({ contentTypes: '.txt, .json, text/plain, text/csv, image/*, video/*', allowMultiple: true, }); // Go through each file and read them for (let index = 0; index < files.length; ++index) { let file = files[index]; console.log(`Reading File: ${file.name}`, `, Type: ${file.type}`); // Read the file as a Uint8Array let contents = await FileIO.read({ data: file, readAsTypedArray: true, onProgressChange: (progressEvent) => { let percentLoaded = Number(((progressEvent.loaded / progressEvent.total) * 100).toFixed(2)); updateProgress(file, percentLoaded); } }); console.log(`Finished Reading File: ${file.name}`, `, Type: ${file.type}`); // Show the file showFile(file, contents); } // Hide the status info after a certain amount of time setTimeout(() => updateProgress(null, 0), 5000); }); // Save file document.querySelector('#btnSaveText').addEventListener('click', (e) => { let data = getTextContainer().innerHTML; let filename = 'FileIO_OutputExample.txt'; // Make sure there is data to save if (data.trim().length < 1) { alert('Please load a text file before saving output!'); return; } // Save the text that is in the output div to a file FileIO.save({ data: data, filename: filename, }); }); }); let showFile = (file, data) => { if (isImage(file)) { showImage(file, data); } else if (isVideo(file)) { showVideo(file, data); } else { showText(file, data); } } let showImage = (file, data) => { let output = getImageContainer(); let url = 'data:image/png;base64,' + FileIO.typedArrayToBase64(data); // You could also do this below to create a virtual url //let blob = FileIO.convertToBlob(data); //let url = URL.createObjectURL(blob); let divElementContainer = createElementContainerDiv(); let img = document.createElement("img"); img.src = url; img.height = 150; img.title = file.name; divElementContainer.appendChild(img); divElementContainer.appendChild(createFilenameDiv(file)); output.appendChild(divElementContainer); } let showText = (file, data) => { let output = getTextContainer(); let content = ''; try { content = new TextDecoder('UTF-8').decode(data); } catch (e) { content = FileIO.typedArrayToString(data); } let currentText = output.innerHTML; let newText = ''; newText += ` <div style='text-align: center; margin-bottom: 20px;'> File Name: <span style='font-style: italic; text-decoration: underline;'> ${file.name} </span> </div>`; newText += content; if (currentText.length > 0) { newText += '<br />=========================================<br />'; } newText += currentText; output.innerHTML = newText; } let showVideo = (file, data) => { let output = getVideoContainer(); let blob = FileIO.convertToBlob(data); let divElementContainer = createElementContainerDiv(); let video = document.createElement('video'); video.width = 400; video.controls = true; divElementContainer.appendChild(video); let source = document.createElement('source'); source.src = URL.createObjectURL(blob); source.type = file.type; video.appendChild(source); divElementContainer.appendChild(createFilenameDiv(file)); output.appendChild(divElementContainer); } let isImage = (file) => { try { return isType(file, 'image.*'); } catch (e) { let ext = FileIO.getExtension(file.name); return ['jpg', 'gif', 'bmp', 'png'].indexOf(ext) > -1; } } let isVideo = (file) => { try { return isType(file, 'video.*'); } catch (e) { let ext = FileIO.getExtension(file.name); return ['m4v', 'avi','mpg','mp4', 'webm'].indexOf(ext) > -1; } } let isType = (file, typeCheck) => { return file.type.match(typeCheck) != null; } let getImageContainer = () => { return document.querySelector('.imageContainer'); } let getTextContainer = () => { return document.querySelector('.textContainer'); } let getVideoContainer = () => { return document.querySelector('.videoContainer'); } let getFileStatusContainer = () => { return document.querySelector('.fileStatus'); } let createElementContainerDiv = () => { let divElementContainer = document.createElement("div"); divElementContainer.style.display = 'inline-block'; divElementContainer.style.textAlign = 'center'; divElementContainer.style.margin = '10px'; return divElementContainer; } let createFilenameDiv = (file) => { let divFileName = document.createElement("div"); divFileName.innerHTML = `${file.name}`; //divFileName.style.fontSize = '12.5px'; divFileName.style.width = '150px'; divFileName.style.margin = 'auto'; divFileName.style.wordWrap = 'break-word'; return divFileName; } let updateProgress = (file, percentLoaded) => { let output = getFileStatusContainer(); if (file) { let status = `File: ${file.name} <br />Percent Loaded: ${percentLoaded}`; output.style.display = 'block'; output.innerHTML = status; console.log(status.replace('<br />', '\n')); } else { output.style.display = null; } } </script> </body> </html><!-- // http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || Deserialize & Parse JSON Date To A Date Object & Convert Date String To Date Object Using Vanilla JavaScript
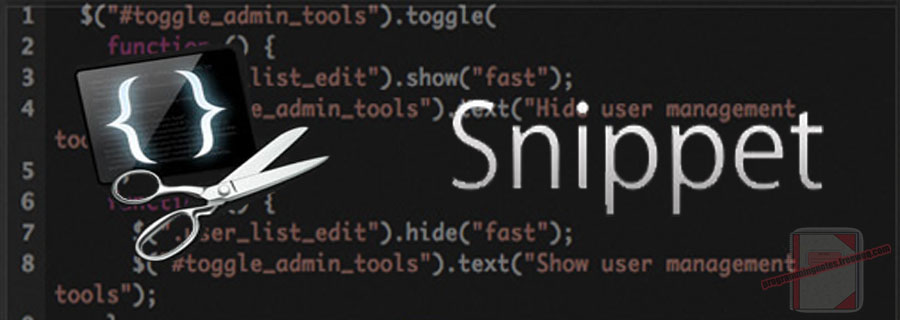
The following is a module which demonstrates how to parse a JSON date string to a date object, as well as converting a date string to a date object.
1. Convert Date String To Date Object
Using ‘Utils.parseDate‘, the example below demonstrates how to convert a date string to a date object.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
// Convert string to date <script> (() => { // Convert the date strings to a date object let dates = [ '2020-01-28T00:45:08.118Z', '2020-07-29', '/Date(1238540400000)/', '/Date(1594368487704)/', '2020-11-28 03:20:35', `/Date(${Date.now()})/`, '7/14/2020 03:20:35 PM', ]; dates.forEach((dateString, index) => { // Convert the date string to a date object let date = Utils.parseDate(dateString); console.log(`\n${index + 1}. ${dateString}`); console.log('Is Date: ', date instanceof Date); console.log(date.toLocaleString()); }); })(); </script> // expected output: /* 1. 2020-01-28T00:45:08.118Z Is Date: true 1/27/2020, 4:45:08 PM 2. 2020-07-29 Is Date: true 7/28/2020, 5:00:00 PM 3. /Date(1238540400000)/ Is Date: true 3/31/2009, 4:00:00 PM 4. /Date(1594368487704)/ Is Date: true 7/10/2020, 1:08:07 AM 5. 2020-11-28 03:20:35 Is Date: true 11/28/2020, 3:20:35 AM 6. /Date(1594774590773)/ Is Date: true 7/14/2020, 5:56:30 PM 7. 7/14/2020 03:20:35 PM Is Date: true 7/14/2020, 3:20:35 PM */ |
2. Parse JSON Date String To Date Object
Using ‘Utils.parseDate‘, the example below demonstrates how to automatically parse a JSON string to a date object via a reviver function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// Parse JSON date string to date <script> (() => { // Convert Json date in object let personJson = ` { "firstName": "Kenneth", "lastName": "P", "time": "2020-07-15T00:28:11.920Z" } `; // Deserialize json and automatically convert date string to date object let deserialized = JSON.parse(personJson, (key, value) => Utils.parseDate(value) ); console.log('Is Date: ', deserialized.time instanceof Date); console.log(deserialized.time.toLocaleString()); })(); </script> // expected output: /* Is Date: true 7/14/2020, 5:28:11 PM */ |
3. Utils.parseDate Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 14, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // -- Public data -- // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: parseDate * USE: Converts a date string to a date object. If the expression to * convert is not a valid date string, no conversion takes place and * the original value is returned. * @param value: The date value to be converted. * @return: The converted value. */ exposed.parseDate = (value) => { if (typeof value === 'string') { // Check ISO-formatted string let reISO = /^\d{4}-(0[1-9]|1[0-2])-([12]\d|0[1-9]|3[01])([T\s](([01]\d|2[0-3])\:[0-5]\d|24\:00)(\:[0-5]\d([\.,]\d+)?)?([zZ]|([\+-])([01]\d|2[0-3])\:?([0-5]\d)?)?)?$/; let a = reISO.exec(value); if (a) { // if so, Date() can parse it return new Date(value); } // Check Microsoft-format string let reMsAjax = /^\/Date\((d|-|.*)\)[\/|\\]$/; a = reMsAjax.exec(value); if (a) { // Parse for relevant portions let b = a[1].split(/[-+,.]/); return new Date(b[0] ? +b[0] : 0 - +b[1]); } // Check forward slash date let reDate = /\d{1,2}\/\d{1,2}\/\d{4} \d{1,2}:\d{2}|^\d{1,2}\/\d{1,2}\/\d{4}$/; a = reDate.exec(value); if (a) { // Parse for relevant portions return new Date(value); } } return value; } // -- Private data -- (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || Convert & Deserialize JSON & Simple Object To A Class Type Using Vanilla JavaScript
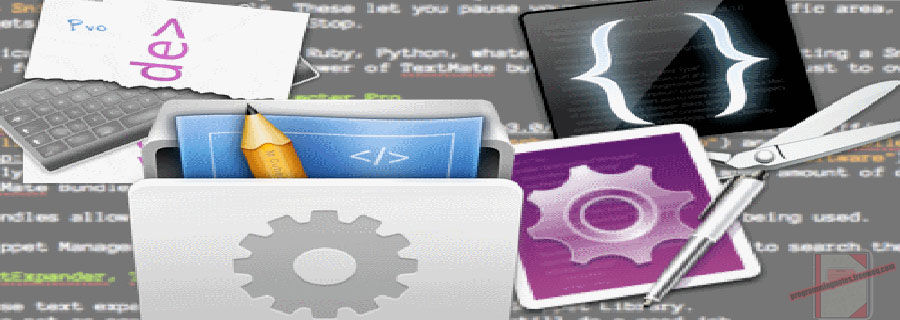
The following is a module which demonstrates how to convert a simple object to a class type, as well as parsing a JSON serialized object back to its class type.
1. Convert Simple Object To Typed Class
The example below demonstrates how to convert a simple object to its class type. Converting the simple object to its class type allows us to use its class functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// Convert Simple Object To Class <script> class Person { constructor(firstName, lastName, address) { this.firstName = firstName; this.lastName = lastName; this.address = address } getFullName() { return `${this.firstName} ${this.lastName}`; } } (() => { // Convert simple object to class object let personObj = { firstName: 'Kenneth', lastName: 'P' }; // Convert to the class type 'Person' allowing // us to use the functions from that class let personType = Utils.ctype(personObj, Person); console.log(personType.getFullName()); })(); </script> // expected output: /* Kenneth P */ |
The ‘Utils.ctype‘ function converts the object to a class, which allows to use the functions from that class.
2. Deserialize Simple JSON Object To Typed Class
Parsing a simple JSON string and converting it to a typed class can be achieved in a similar way. The example below demonstrates this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
// Parse Simple JSON Object To Class <script> class Person { constructor(firstName, lastName, address) { this.firstName = firstName; this.lastName = lastName; this.address = address } getFullName() { return `${this.firstName} ${this.lastName}`; } } (() => { // Convert Json to class object let personJson = ` { "firstName": "Kenneth", "lastName": "P" } `; // Convert to the class type 'Person' allowing // us to use the functions from that class let personType = Utils.ctype(JSON.parse(personJson), Person); console.log(personType.getFullName()); })(); </script> // expected output: /* Kenneth P */ |
3. Deserialize Simple Nested JSON Object To Typed Class
Parsing a multi-leveled, nested JSON string and converting it to a typed class is done a little differently. We have to map the JSON structure with the values we want to convert. This is done using ‘Utils.ctypeMap‘.
The example below demonstrates this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
// Parse Nested JSON Object To Class <script> class Person { constructor(firstName, lastName, address) { this.firstName = firstName; this.lastName = lastName; this.address = address } getFullName() { return `${this.firstName} ${this.lastName}`; } } class Address { constructor(street, city, zip) { this.street = street; this.city = city; this.zip = zip; } getFullAddress() { return `${this.street} - ${this.city}, ${this.zip}`; } } (() => { // Convert Json to class object let personJson = ` { "firstName": "Kenneth", "lastName": "P", "address": { "street": "Valley Hwy", "city": "Valley Of Fire", "zip": "89040" } } `; // Create a conversion type map let conversionTypeMap = { '': Person, // Convert the entire object to type 'Person' 'address': Address, // Convert the address property to type 'Address' }; // Deserialize the json string and convert the types let personType = Utils.ctypeMap(JSON.parse(personJson), conversionTypeMap); console.log(personType.getFullName()); console.log(personType.address.getFullAddress()); })(); </script> // expected output: /* Kenneth P Valley Hwy - Valley Of Fire, 89040 */ |
4. Deserialize Complex Nested JSON Object To Typed Class
Parsing a complex JSON string and converting it to a typed class can be achieved in a similar way. The example below demonstrates this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 |
// Parse Nested JSON Object To Class <script> class Address { constructor(street, city, zip) { this.street = street; this.city = city; this.zip = zip; } getFullAddress() { return `${this.street} - ${this.city}, ${this.zip}`; } } class Person { constructor(firstName, lastName, address) { this.firstName = firstName; this.lastName = lastName; this.address = address } getFullName() { return `${this.firstName} ${this.lastName}`; } } class Album { constructor(artist, title, released) { this.artist = artist; this.title = title; this.released = released } getDescription() { return `${this.artist}: ${this.title} - ${this.released}`; } } (() => { // Convert Json object array to class object let personJson = `{ "people": [ { "firstName": "Kenneth", "lastName": "P", "address": { "street": "Valley Hwy", "city": "Valley Of Fire", "zip": "89040" }, "albums": [ { "artist": "System of a Down", "title": "Toxicity", "released": 2001 }, { "artist": "Kanye West", "title": "The College Dropout", "released": 2004 } ] }, { "firstName": "Jennifer", "lastName": "N", "address": { "street": "Boulder Ln", "city": "Yosemite Valley", "zip": "95389" }, "albums": [ { "artist": "Coldplay", "title": "Parachutes", "released": 2000 }, { "artist": "Alicia Keys", "title": "Songs in A Minor", "released": 2001 } ] } ], "nested": { "albums": [ { "artist": "Coldplay", "title": "Parachutes", "released": 2000 }, { "artist": "Alicia Keys", "title": "Songs in A Minor", "released": 2001 }, { "artist": "System of a Down", "title": "Toxicity", "released": 2001 }, { "artist": "Kanye West", "title": "The College Dropout", "released": 2004 } ] }, "nested2": { "album": { "artist": "Coldplay", "title": "Parachutes", "released": 2000 } } }`; // Create a conversion type map let conversionTypeMap = { 'people.albums': Album, // Convert all the albums in the person class array to type 'Album' 'people[0].address': Address, // Convert only the first address object of the people array to type 'Address' 'people.address': Address, // Convert all address objects of the people array to type 'Address' 'people': Person, // Convert all the objects in the people array to type 'Person' 'nested.albums': Album, // Convert all the albums objects in the nested object to type 'Album' 'nested2.album': Album, // Convert all the albums in the nested2 object to type 'Album' }; // Deserialize the json string and convert the types let deserializedData = Utils.ctypeMap(JSON.parse(personJson), conversionTypeMap); // Print the converted addresses using the class function console.log('\nPeoples Albums:'); deserializedData.people.forEach((person, index) => { console.log((index + 1) + '. ' + person.getFullName() + '\'s Albums:'); person.albums.forEach((album, index) => { console.log(`\t${index + 1}. ${album.getDescription()}`); }); }); // Print the first address object console.log('\nFirst address object'); console.log(deserializedData.people[0].address.getFullAddress()); // Print the converted people using the class function console.log('\nPeople:'); deserializedData.people.forEach((person, index) => { console.log(`${index + 1}. ${person.getFullName()}`); }); // Print the converted albums using the class function console.log('\nNested Albums:'); deserializedData.nested.albums.forEach((album, index) => { console.log(`${index + 1}. ${album.getDescription()}`); }); // Print the converted addresses using the class function console.log('\nPeoples Address:'); deserializedData.people.forEach((person, index) => { console.log(`${index + 1}. ${person.address.getFullAddress()}`); }); console.log('\nNested 2'); console.log(deserializedData.nested2.album.getDescription()); })(); </script> // expected output: /* Peoples Albums: 1. Kenneth P's Albums: 1. System of a Down: Toxicity - 2001 2. Kanye West: The College Dropout - 2004 2. Jennifer N's Albums: 1. Coldplay: Parachutes - 2000 2. Alicia Keys: Songs in A Minor - 2001 First address object Valley Hwy - Valley Of Fire, 89040 People: 1. Kenneth P 2. Jennifer N Nested Albums: 1. Coldplay: Parachutes - 2000 2. Alicia Keys: Songs in A Minor - 2001 3. System of a Down: Toxicity - 2001 4. Kanye West: The College Dropout - 2004 Peoples Address: 1. Valley Hwy - Valley Of Fire, 89040 2. Boulder Ln - Yosemite Valley, 95389 Nested 2 Coldplay: Parachutes - 2000 */ |
5. Utils.ctype/ctypeMap Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 14, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // -- Public data -- // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: ctype * USE: Returns the result of explicitly converting an expression * to a specified data type or class * @param expression: The object to be converted. * @param typename: The data type or class to be converted to. * @return: The converted value. */ exposed.ctype = (expression, typename) => { let value = expression; if (!exposed.isType(expression, typename)) { value = Object.create(typename.prototype, Object.getOwnPropertyDescriptors(expression)); } return value; } /** * FUNCTION: ctypeMap * USE: Returns the result of explicitly converting an expression * to a specified data type or class * @param expression: The object to be converted. * @param conversionTypeMap: An object that specifies the data type * or class to be converted to. * @return: The converted value. */ exposed.ctypeMap = (expression, conversionTypeMap) => { // Go through each property and convert // objects to the class specific types for (const prop in conversionTypeMap) { let type = conversionTypeMap[prop]; if (prop.trim().length < 1) { expression = exposed.ctype(expression, type); continue; } let items = getSetDescendantProp(expression, prop); if (!items) { continue; } else if (!Array.isArray(items)) { items = [items]; } items.forEach((obj, index) => { if (exposed.isType(obj, type)) { return; } getSetDescendantProp(expression, prop, obj, exposed.ctype(obj, type)); }); } return expression; } exposed.isType = (expression, typename) => { return (expression instanceof typename); } // -- Private data -- let getSetDescendantProp = (obj, desc, prevValue, newValue) => { let arr = desc ? desc.split('.') : []; // Go through the item properties and try to // find the item that matches 'desc' while (arr.length && obj) { let comp = arr.shift(); // Handle full arrays let target = obj[comp]; if (target && ((target.length && target.forEach) || Array.isArray(target))) { let remainder = arr.join('.'); let results = []; for (let index = 0; index < target.length; ++index){ let x = getSetDescendantProp(target[index], remainder, prevValue, newValue); if (x) { results = results.concat(x); } if (remainder.length < 1 && typeof newValue !== 'undefined') { if (prevValue === target[index]) { target[index] = newValue; } } } return results; } else { // Handle indexed arrays let match = new RegExp('(.+)\\[([0-9]*)\\]').exec(comp); if ((match !== null) && (match.length == 3)) { let arrayData = { arrName: match[1], arrIndex: match[2] }; if (obj[arrayData.arrName] !== undefined) { if (typeof newValue !== 'undefined' && arr.length === 0 && prevValue === obj[arrayData.arrName][arrayData.arrIndex]) { obj[arrayData.arrName][arrayData.arrIndex] = newValue; } // Move to the next item obj = obj[arrayData.arrName][arrayData.arrIndex]; } else { obj = undefined; } } else { // Handle regular items if (typeof newValue !== 'undefined') { if (obj[comp] === undefined) { obj[comp] = {}; } if (arr.length === 0 && prevValue === obj[comp]) { obj[comp] = newValue; } } // Move to the next item obj = obj[comp]; } } } return obj; } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || Promise – Resolve Promises In Order Of Completion Using Vanilla JavaScript
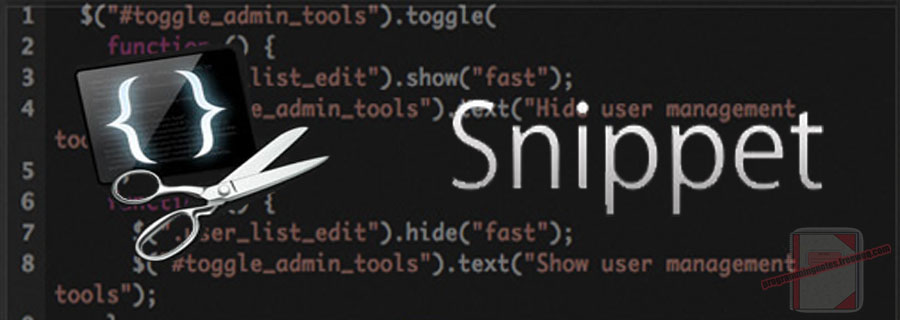
The return values of Promise.all are returned in the order that the Promises were passed in, regardless of completion order. Sometimes, it is nice to know which promise completed first.
The following is sample code which demonstrates how to resolve an array of promises and return the results in order of completion.
Contents
1. Resolve By Completion
2. Fail-Fast Behavior
3. Timeout
4. Utils.resolveByCompletion
5. More Examples
The examples below demonstrate the return values of Promise.all compared to ‘resolve by completion‘.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Promise all example <script> (() => { let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve) => setTimeout(() => resolve('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // Promise all Promise.all(promises).then(values => { console.log(values); }).catch(error => { console.log(error); }); })() </script> // expected output: /* [ "A (slow)","B (slower)","C (fast)" ] */ |
‘Resolve by completion’ returns the completed values from fastest to slowest.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Resolve By Completion <script> (() => { let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve) => setTimeout(() => resolve('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // Promise values are returned in the order of completion Utils.resolveByCompletion(promises).then(values => { console.log(values); }).catch(error => { console.log(error); }); })() </script> // expected output: /* [ "C (fast)","A (slow)","B (slower)" ] */ |
2. Fail-Fast Behavior
Promise.all is rejected if any of the elements are rejected. For example, if you pass in multiple promises that will resolve, and one promise that rejects, then Promise.all will reject immediately.
Similar to Promise.allSettled, ‘Resolve by completion’ allows returning the promises rejected value to the result list.
The following example demonstrates this behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Promise all example <script> (() => { let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve, reject) => setTimeout(() => reject('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // Rejected value is returned to the 'catch' function Promise.all(promises).then(values => { console.log(values); }).catch(error => { console.log('Rejected: ', error); }); })() </script> // expected output: /* Rejected: B (slower) */ |
‘Resolve by completion’ returns the rejected value as apart of the result list, depending on the boolean ‘rejectOnError’ parameter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Resolve By Completion <script> (() => { let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve, reject) => setTimeout(() => reject('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // Rejected promise values are returned when the parameter 'rejectOnError' is set to false. // Setting the parameter to true will reject the operation like normal. Utils.resolveByCompletion(promises, false).then(values => { console.log(values); }).catch(error => { console.log('Rejected: ', error); }); })() </script> // expected output: /* [ "C (fast)","A (slow)","B (slower)" ] */ |
3. Timeout
By default, ‘resolve by completion’ has no timeout. If setting a timeout is desired, it can be done like so.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Resolve By Completion - Timeout <script> (() => { let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve) => setTimeout(() => resolve('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // The operation will reject when timeout period expires. // The operation will reject on timeout, regardless of the 'rejectOnError' parameter Utils.resolveByCompletion(promises, false, 500).then(values => { console.log(values); }).catch(error => { console.log(error); }); })() </script> // expected output: /* Error: Timeout of 500ms expired */ |
4. Utils.resolveByCompletion
The following is Utils.resolveByCompletion. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 8, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // -- Public data -- // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: resolveByCompletion * USE: Returns a promise that will resolve when all of the input's * promises have resolved, in order of completion. * @param promises: An array of promises. * @param rejectOnError: Optional. Boolean that indicates if the operation * should reject if any of the input promises reject or throw an error. * If set to true, the operation is rejected if any of the input * promises reject or throw an error. * If set to false, the promises rejected value is added to the result list. * @param timeout: Optional. Integer that indicates how long to wait * (in milliseconds) for the promise group to complete. * @return: A promise that will contain the input promises results on completion. */ exposed.resolveByCompletion = (promises, rejectOnError = true, timeout = null) => { return new Promise(async (resolve, reject) => { try { let results = [] let promiseMap = new Map(); Array.prototype.forEach.call(promises, (promise, index) => { let promiseResult = { index: index, value: null, error: null }; let mapValue = null; if (promise instanceof Promise) { mapValue = promise .then(value => {promiseResult.value = value; return promiseResult}) .catch(error => {promiseResult.error = error; return promiseResult}) } else { mapValue = promiseResult; promiseResult.value = promise; } promiseMap.set(index, mapValue); }); let start = Date.now(); let isTimedOut = () => { let result = false; if (timeout) { let elapsed = (Date.now() - start); result = elapsed >= timeout; } return result; } while (promiseMap.size > 0) { let promiseResult = await Promise.race(promiseMap.values()); if (!promiseMap.delete(promiseResult.index)) { throw new Error('Error occurred processing values'); } if (promiseResult.error) { if (rejectOnError) { reject(promiseResult.error); return; } results.push(promiseResult.error); } else { results.push(promiseResult.value); } if (isTimedOut()) { throw new Error(`Timeout of ${timeout}ms expired`); } } resolve(results); } catch (e) { reject(e); } }); } // -- Private data -- (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of ‘Utils.resolveByCompletion’. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Jul 8, 2020 // Taken From: http://programmingnotes.org/ // File: utils.html // Description: Demonstrates the use of functions in Utils.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes Utils.resolveByCompletion Demo</title> <style> .main { text-align:center; margin-left:auto; margin-right:auto; } .output { text-align: left; } pre {outline: 1px solid #ccc; padding: 5px; margin: 5px; } .string { color: green; } .number { color: darkorange; } .boolean { color: blue; } .null { color: magenta; } .key { color: red; } </style> <!-- // Include module --> <script type="text/javascript" src="./Utils.js"></script> </head> <body> <div class="main"> My Programming Notes Utils.resolveByCompletion Demo <pre><code><div class="output"></div></code></pre> </div> <script> (() => { return; let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve) => setTimeout(() => resolve('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // Promise values are returned in the order of completion Utils.resolveByCompletion(promises).then(values => { console.log(values); print('Promise values are returned in the order of completion', values); }).catch(error => { console.log(error); }); })() </script> <script> (() => { return; let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve, reject) => setTimeout(() => reject('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // Rejected promise values are returned when the parameter 'rejectOnError' is set to false Utils.resolveByCompletion(promises, false).then(values => { console.log(values); print('Rejected promise values are returned when the parameter "rejectOnError" is set to false', values); }).catch(error => { console.log('Rejected: ', error); }); })() </script> <script> (() => { return; let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve) => setTimeout(() => resolve('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; // The operation will reject when timeout period expires Utils.resolveByCompletion(promises, false, 500).then(values => { console.log(values); print('The operation will reject when timeout period expires', values); }).catch(error => { console.log(error); print('', error.message); }); })() </script> <script> (async () => { //return; // Using promise all let promiseAllResult1 = await Promise.all(example1()); print('1. Resolved promises', promiseAllResult1, 4); // Using resolve by completion let resolveByResult1 = await Utils.resolveByCompletion(example1()); print('1. Resolved promises ordered by completion', resolveByResult1, 4); // Using promise all let promiseAllResult2 = await Promise.all(example2()); print('2. Resolved promises', promiseAllResult2, 4); // Using resolve by completion let resolveByResult2 = await Utils.resolveByCompletion(example2()); print('2. Resolved promises ordered by completion', resolveByResult2, 4); // Using promise all let promiseAllResult3 = await Promise.all(example3()); print('3. Resolved promises', promiseAllResult3, 4); // Using resolve by completion let resolveByResult3 = await Utils.resolveByCompletion(example3()); print('3. Resolved promises ordered by completion', resolveByResult3, 4); // Using promise all let promiseAllResult4 = await Promise.all(example4()); print('4. Resolved promises', promiseAllResult4, 4); // Using resolve by completion let resolveByResult4 = await Utils.resolveByCompletion(example4()); print('4. Resolved promises ordered by completion', resolveByResult4, 4); // Using promise all let promiseAllResult5 = Promise.all(example5()).catch((error) => { print('5. Promise.all rejected item', error, 4); }); // Using resolve by completion let resolveByResult5 = await Utils.resolveByCompletion(example5(), false); print('5. resolveByCompletion including rejected item', resolveByResult5, 4); })() function example1() { let promises = [ new Promise((resolve) => setTimeout(() => resolve('A (slow)'), 1000)), new Promise((resolve) => setTimeout(() => resolve('B (slower)'), 2000)), new Promise((resolve) => setTimeout(() => resolve('C (fast)'), 10)) ]; return promises; } function example2() { let promises = [ new Promise(resolve => {setTimeout(resolve, 200, 'slow');}), 'instant', new Promise(resolve => {setTimeout(resolve, 50, 'quick');}) ]; return promises; } function example3() { let promises = [ Promise.resolve(3), 42, new Promise((resolve, reject) => { setTimeout(resolve, 100, 'foo');}) ]; return promises; } function example4() { let promises = [1,2,3, Promise.resolve(444)]; return promises; } function example5() { let promises = [1,2,3, Promise.reject(555)]; return promises; } </script> <script> function print(desc, obj, indent = 0) { let text = (desc.length > 0 ? '<br />' : '') + desc + (desc.length > 0 ? '<br />' : ''); let objText = obj || ''; if (obj && typeof obj != 'string') { objText = syntaxHighlight(JSON.stringify(obj, null, indent)); } text += objText; let pageText = document.querySelector('.output').innerHTML; pageText += (pageText.length > 0 ? '<br />' : '') + text; document.querySelector('.output').innerHTML = pageText; } function syntaxHighlight(json) { if (typeof json != 'string') { json = JSON.stringify(json, undefined, 2); } json = json.replace(/&/g, '&').replace(/</g, '<').replace(/>/g, '>'); return json.replace(/("(\\u[a-zA-Z0-9]{4}|\\[^u]|[^\\"])*"(\s*:)?|\b(true|false|null)\b|-?\d+(?:\.\d*)?(?:[eE][+\-]?\d+)?)/g, function (match) { let cls = 'number'; if (/^"/.test(match)) { if (/:$/.test(match)) { cls = 'key'; } else { cls = 'string'; } } else if (/true|false/.test(match)) { cls = 'boolean'; } else if (/null/.test(match)) { cls = 'null'; } return '<span class="' + cls + '">' + match + '</span>'; }); } </script> </body> </html><!-- // http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || Resources.js – JavaScript & CSS Parallel File Loader To Dynamically Import & Load Files To Page Document Using Vanilla JavaScript
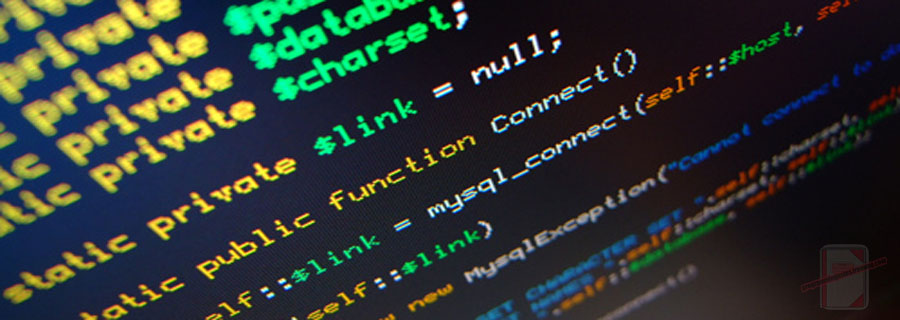
The following is a module that handles loading JavaScript and CSS files into a document in parallel, as fast as the browser will allow. This module also allows to ensure proper execution order if you have dependencies between files.
Contents
1. Load Files One By One
2. Load Multiple Files In Parallel
3. Load Multiple Files In Parallel With Dependencies
4. Load A JavaScript Function From Another JS File
5. Resources.js Namespace
6. More Examples
Syntax is very straightforward. The following demonstrates loading single files one by one:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// Load JavaScript and CSS files one by one. <script> (async () => { // Load files one by one await Resources.load('/file1.js'); await Resources.load('/file2.js'); await Resources.load('/file3.css'); await Resources.load('/file4.css'); await Resources.load('https://code.jquery.com/jquery-3.5.1.js'); await Resources.load('https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js'); })(); </script> |
The ‘Resources.load‘ function returns an object that contains information about the load status. It can let you know if a file was loaded successfully or not.
To get the load status in order to determine if the file was loaded correctly, it can be done like so:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Load JavaScript and CSS files one by one and verify. <script> (async () => { // Load files one by one console.log(await Resources.load('/file1.js')); console.log(await Resources.load('/file2.js')); console.log(await Resources.load('/file3.css')); console.log(await Resources.load('/file4.css')); console.log(await Resources.load('https://code.jquery.com/jquery-3.5.1.js')); console.log(await Resources.load('https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js')); })(); </script> // expected output: /* [ { url: "/file1.js", loaded: false, notes: "*** /file1.js failed to load ***" } ] [ { url: "/file2.js", loaded: false, notes: "*** /file2.js failed to load ***" } ] [ { url: "/file3.css", loaded: false, notes: "*** /file3.css failed to load ***" } ] [ { url: "/file4.css", loaded: false, notes: "*** /file4.css failed to load ***" } ] [ { url: "https://code.jquery.com/jquery-3.5.1.js", loaded: true, notes: "https://code.jquery.com/jquery-3.5.1.js loaded" } ] [ { url: "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js", loaded: true, notes: "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js loaded" } ] */ |
In the example above, the load results can let you know which files were loaded into the document, and the reason for failure.
2. Load Multiple Files In Parallel
In the examples above, files were added sequentially, one by one.
Ideally, you would want to load all of your files at once in parallel. This makes it so you are able to add multiple files as fast as the browser will allow. Parallel loading also allows you to set if there are any file dependencies.
Using the files from the examples above, this can be done like so.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
// Load JavaScript and CSS files in parallel. <script> (async () => { // Load files in parallel let loadResult = await Resources.load({ files: [ { url: '/file1.js', type: 'js', wait: false }, { url: '/file2.js', type: 'js', wait: false }, { url: '/file3.css', type: 'css', wait: false }, { url: '/file4.css', type: 'css', wait: false }, { url: 'https://code.jquery.com/jquery-3.5.1.js', type: 'js', wait: false }, { url: 'https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', type: 'js', wait: false }, ], logResults: true, rejectOnError: false, timeout: null }); console.log(loadResult); })(); </script> // possile output: /* [ { "url": "/file3.css", "loaded": false, "notes": "*** /file3.css failed to load ***" }, { "url": "/file4.css", "loaded": false, "notes": "*** /file4.css failed to load ***" }, { "url": "/file1.js", "loaded": false, "notes": "*** /file1.js failed to load ***" }, { "url": "/file2.js", "loaded": false, "notes": "*** /file2.js failed to load ***" }, { "url": "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js", "loaded": true, "notes": "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js loaded" }, { "url": "https://code.jquery.com/jquery-3.5.1.js", "loaded": true, "notes": "https://code.jquery.com/jquery-3.5.1.js loaded" } ] */ |
For parallel loading, the parameter supplied to the ‘Resources.load‘ function is an object that is made up of the following properties.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
{ • files: An object array of 'File Info' of files to load (See below). • logResults: Optional. Boolean that indicates if the load status should be displayed to console.log(). Default is false. • rejectOnError: Optional. Boolean that indicates if the load operation should reject if a loading error occurs for any file in the group. Default is false. • timeout: Optional. Integer that indicates how long to wait (in milliseconds) for the file group to load before timeout occurs. Default is null. // ** 'File Info': This is made up of the following properties { • url: The source url to load. • type: Optional. String indicating the file type to load. Options are 'css' or 'js'. Default type is determined by the file extension if not supplied. • wait: Optional. Boolean that indicates if this file should be completely loaded before loading subsequent files in the group. • If set to true, other urls are not loaded until this one finishes loading. After loading this file, other files continue loading in parallel • If set to false, no wait occurs and files load in parallel. This makes it so dependent files dont get loaded until the required files are loaded first. Files with this property set to true are loaded before any other file in the group. Default is false. } } |
3. Load Multiple Files In Parallel With Dependencies
The ‘wait‘ property is a great way to manage file dependencies. When the ‘wait‘ property is set to true, that indicates that the file should be completely loaded before loading subsequent files in the group.
This makes it so loading files in parallel does not cause files to be loaded before its dependent files.
The following examples demonstrates the ‘wait‘ functionality when loading files in parallel.
The example below demonstrates what happens when ‘wait‘ is not used. Load order behavior is undefined because files are loaded as they become available. This could cause an issue if the second file depends on the first file, but loads before it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// Load JavaScript and CSS files in parallel. // Load order behavior is undefined because files // are loaded as they become available <script> (async () => { // Load files in parallel let loadResult = await Resources.load({ files: [ { url: 'https://code.jquery.com/jquery-3.5.1.js', type: 'js', wait: false }, { url: 'https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', type: 'js', wait: false }, ], logResults: true, rejectOnError: false, timeout: null }); console.log(loadResult); })(); </script> /* // possible output: [ { "url": "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js", "loaded": true, "notes": "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js loaded" }, { "url": "https://code.jquery.com/jquery-3.5.1.js", "loaded": true, "notes": "https://code.jquery.com/jquery-3.5.1.js loaded" } ] */ |
In the example below, the ‘wait‘ property is set to true for the first file, ensuring that it will always be loaded before any subsequent files.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Load JavaScript and CSS files in parallel. // Load behavior is managed using wait <script> (async () => { // Load files in parallel let loadResult = await Resources.load({ files: [ { url: 'https://code.jquery.com/jquery-3.5.1.js', type: 'js', wait: true }, { url: 'https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', type: 'js', wait: false }, ], logResults: true, rejectOnError: false, timeout: null }); console.log(loadResult); })(); </script> /* // expected output: [ { "url": "https://code.jquery.com/jquery-3.5.1.js", "loaded": true, "notes": "https://code.jquery.com/jquery-3.5.1.js loaded" }, { "url": "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js", "loaded": true, "notes": "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js loaded" } ] */ |
4. Load A JavaScript Function From Another JS File
‘Resources.load‘ could be used to call a JavaScript function in another js file. The example below demonstrates this.
In the following example, main.html is the ‘driver’ file. It loads ‘fullName.js‘ to get the functions located in that file. ‘fullName.js‘ then uses ‘Resources.load‘ to load the files ‘display.js‘, ‘log.js‘ and ‘date.js‘, and calls the functions from those files to display information.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
<!-- // main.html // This file loads functions from fullName.js --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes Resources.js Demo</title> <style> .main { text-align:center; margin-left:auto; margin-right:auto; } </style> <!-- Include Resources.js --> <script type="text/javascript" src="./Resources.js"></script> </head> <body> <div class="main"> My Programming Notes Resources.js Demo </div> <script> (async () => { // Load fullname.js to get the 'fullname' functions await Resources.load({ files: [ './fullName.js' ], logResults: true }); // Fucntions from fullname.js let fullName = getFullName('Kenneth', 'P'); // This calls a function in display.js via fullname.js with no return value await displayFullName(fullName).catch((error) => { // Log if any errors occur console.log('Failed:', error); }); // This calls a function in log.js via fullname.js with no return value await logFullName(fullName).catch((error) => { // Log if any errors occur console.log('Failed:', error); }); // This calls a function in date.js via fullname.js that rerturns a value try { alert(await getFullNameWithDate(fullName)); } catch (error) { // Log if any errors occur console.log('Failed:', error); } })(); </script> </body> </html> <!-- // expected output: *** Resources.js Load Status *** ./fullName.js loaded *** Resources.js Load Status *** ./display.js loaded ./log.js loaded ./date.js loaded [ALERT: Kenneth P] Kenneth P [ALERT: Name: Kenneth P Date: 7/7/2020, 12:09:44 PM] --> |
Below is fullName.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
// fullName.js // Load the 'display', 'log' and 'getDateString' functions // from the files below. let dependencies = Resources.load({ files: [ './display.js', './log.js', './date.js' ], logResults: true }); let getFullName = (firstName, lastName) => { return `${firstName} ${lastName}`; } // Uses the 'display' function from display.js let displayFullName = (fullName) => { return new Promise(async (resolve, reject) => { try { // Make sure the dependencies have loaded. // The load status is logged to the console. let status = await dependencies; //console.log(status); display(fullName); resolve(); } catch (error) { // Log if any errors occur //console.log('Failed:', error); reject(error); } }); } // Uses the 'log' function from log.js let logFullName = (fullName) => { return new Promise(async (resolve, reject) => { try { // Make sure the dependencies have loaded. // The load status is logged to the console. let status = await dependencies; //console.log(status); log(fullName); resolve(); } catch (error) { // Log if any errors occur //console.log('Failed:', error); reject(error); } }); } // Uses the 'getDateString' function from date.js that returns a value let getFullNameWithDate = (fullName) => { return new Promise(async (resolve, reject) => { try { // Make sure the dependencies have loaded. // The load status is logged to the console. let status = await dependencies; //console.log(status); let date = getDateString(new Date()); resolve(`Name: ${fullName}\nDate: ${date}`); } catch (error) { // Log if any errors occur //console.log('Failed:', error); reject(error); } }); } |
Below is display.js
1 2 3 4 5 |
// display.js let display = (data) => { alert(data); } |
Below is log.js
1 2 3 4 5 |
// log.js let log = (data) => { console.log(data); } |
Below is date.js
1 2 3 4 5 |
// date.js let getDateString = (date) => { return date.toLocaleString(); } |
5. Resources.js Namespace
The following is the Resources.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 7, 2020 // Taken From: http://programmingnotes.org/ // File: Resources.js // Description: Javascript that loads Javascript and CSS files // into a document in parallel, as fast as the browser will allow. // This allows to ensure proper execution order if you have dependencies // between files. // Example: // // Load JavaScript and CSS files and wait for them to load // const dependencies = await Resources.load({ // files: [ // { url: '/file1.js', type: 'js', wait: false }, // { url: '/file2.js', type: 'js', wait: false }, // { url: '/file3.css', type: 'css', wait: false }, // { url: '/file4.css', type: 'css', wait: false }, // { url: 'https://code.jquery.com/jquery-3.5.1.js', type: 'js', wait: true }, // { url: 'https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', type: 'js', wait: false }, // ], // logResults: true, // rejectOnError: false, // timeout: null // }); // ============================================================================ /** * NAMESPACE: Resources * USE: Handles loading Javascript and CSS files in parallel. */ var Resources = Resources || {}; (function(namespace) { 'use strict'; // -- Public data -- // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: load * USE: Loads Css and/or Javascript files into a document in * parallel, depending on the options * @param options: An object of file loading options. * Its made up of the following properties: * { * files: An object array of File info of files to load (See below). * logResults: Optional. Boolean that indicates if the load * status should be displayed to console.log(). * Default is false. * rejectOnError: Optional. Boolean that indicates if the load * operation should reject if a loading error occurs for * any file in the group. * Default is false. * timeout: Optional. Integer that indicates how long to wait * (in milliseconds) for the file group to load before timeout occurs. * Default is null. * } * * ** File info: This is made up of the following properties * { * url: The source url to load. * type: Optional. String indicating the file type to load. * Options are 'css' or 'js'. * Default type is determined by the file extension. * wait: Optional. Boolean that indicates if this file should be * completely loaded before loading subsequent files in the group. * If set to true, other urls are not loaded until this one * finishes loading. After loading this file, other files * continue loading in parallel. * If set to false, no wait occurs and files load in parallel. * This makes it so dependent files dont get loaded until the * required files are loaded first. * Files with this property set to true are loaded before * any other file in the group. * Default is false. * } * @return: A promise that completes when all resources are loaded, which * contains an array of each files loading status. */ exposed.load = (options) => { return new Promise(async (resolve, reject) => { try { // Load files and wait for the results options = verifyOptions(options); let results = await loadFiles(options); if (options.logResults) { console.log('*** Resources.js Load Status ***') let notes = results.map((x) => x.notes); console.log(notes.join('\n')); } resolve(results); } catch (e) { reject(e); } }); } /** * FUNCTION: loadJs * USE: Loads a Javascript file into a document * @param url: The javascript url to load. * @return: A promise that completes when the url is loaded. */ exposed.loadJs = (url) => { return new Promise((resolve, reject) => { // Load file and attach it to the document let status = createFileStatus(url); try { if (fileExists(url)) { status.loaded = true; status.notes = `${url} already loaded`; resolve(status); return; } let script = document.createElement("script"); script.src = url; script.type = "text/javascript" script.id = getId(url); script.onload = script.onreadystatechange = function() { if ((!this.readyState || this.readyState == "loaded" || this.readyState == "complete") ) { status.loaded = true; status.notes = `${url} loaded`; resolve(status); } } script.onerror = () => { removeFile(url); status.notes = `*** ${url} failed to load ***`; reject(status); } document.getElementsByTagName("head")[0].appendChild(script); addFile(url); } catch (e) { let message = e.message ? e.message : e; status.notes = `*** ${url} failed to load. Reason: ${message} ***`; reject(status); } }); } /** * FUNCTION: loadCss * USE: Loads a Css file into a document * @param url: The css url to load. * @return: A promise that completes when the url is loaded. */ exposed.loadCss = (url) => { return new Promise((resolve, reject) => { // Load file and attach it to the document let status = createFileStatus(url); try { if (fileExists(url)) { status.loaded = true; status.notes = `${url} already loaded`; resolve(status); return; } let link = document.createElement('link'); link.type = 'text/css'; link.rel = 'stylesheet'; link.onload = () => { status.loaded = true; status.notes = `${url} loaded`; resolve(status); } link.onerror = () => { removeFile(url); status.notes = `*** ${url} failed to load ***`; reject(status); } link.href = url; link.id = getId(url); document.getElementsByTagName("head")[0].appendChild(link); addFile(url); } catch (e) { let message = e.message ? e.message : e; status.notes = `*** ${url} failed to load. Reason: ${message} ***`; reject(status); } }); } /** * FUNCTION: getFilename * USE: Returns the filename of a url * @param url: The url. * @return: The filename of a url. */ exposed.getFilename = (url) => { let filename = ''; if (url && url.length > 0) { filename = url.split('\\').pop().split('/').pop(); // Remove any querystring if (filename.indexOf('?') > -1) { filename = filename.substr(0, filename.indexOf('?')); } } return filename; } /** * FUNCTION: getExtension * USE: Returns the file extension of the filename of a url * @param url: The url. * @return: The file extension of a url. */ exposed.getExtension = (url) => { let filename = exposed.getFilename(url); let ext = filename.split('.').pop(); return (ext === filename) ? '' : ext; } // -- Private data -- let loadFiles = (options) => { return new Promise(async (resolve, reject) => { try { if (!options.files) { throw new Error('There are no files specified to load.'); } let files = verifyArray(options.files); // Order the files putting the critical ones first prioritizeFiles(files); // Load files to the document let results = []; let promises = []; for (let file of files) { file = verifyFile(file); let promise = getCallback(file).call(this, file.url); if (file.wait) { let value = await resolveByCompletion([promise], options.rejectOnError, options.timeout); results.push(value[0]); } else { promises.push(promise); } } // Get the results let completed = await resolveByCompletion(promises, options.rejectOnError, options.timeout); results = [...results, ...completed]; resolve(results); } catch (e) { let message = e.message ? e.message : e.notes ? e.notes : e; reject(new Error(`Unable to load resources. Reason: ${message}`)); } }); } let prioritizeFiles = (files) => { files.sort((x, y) => { let conditionX = x.wait || false; let conditionY = y.wait || false; return conditionY - conditionX; }); return files; } let getCallback = (file) => { let types = { css: exposed.loadCss, js: exposed.loadJs } let callback = types[file.type.toLocaleLowerCase()]; if (!callback) { throw new Error(`Unknown file type/file extension (${file.type}) for the following url: ${file.url}`); } return callback; } let getId = (str) => { return `${str}_added_resource` } let fileExists = (url) => { return loadedResources.has(url) || (document.querySelector(`script[src='${url}']`) != null); } let addFile = (url) => { loadedResources.set(url, true); } let removeFile = (url) => { return loadedResources.delete(url); } let createFileStatus = (url) => { let status = { url: url, loaded: false, notes: '', } return status; } let verifyOptions = (options) => { if (!options) { throw new Error('There are no options specified'); } if (typeof options !== 'object' || Array.isArray(options)) { let files = options; options = {}; options.files = files; } options.rejectOnError = options.rejectOnError || false; options.timeout = options.timeout|| null; return options; } let verifyArray = (arry) => { if (!arry) { arry = []; } if (!Array.isArray(arry)) { arry = [arry]; } return arry; } let verifyFile = (file) => { if (typeof file !== 'object') { let url = file; file = {}; file.url = url; } file.url = file.url || ''; file.wait = file.wait || false; file.type = file.type || exposed.getExtension(file.url); return file } /** * FUNCTION: resolveByCompletion * USE: Returns a promise that will resolve when all of the input's * promises have resolved, in order of completion. * @param promises: An array of promises. * @param rejectOnError: Optional. Boolean that indicates if the operation * should reject if any of the input promises reject or throw an error. * If set to true, the operation is rejected if any of the input * promises reject or throw an error. * If set to false, the promises rejected value is added to the result list. * @param timeout: Optional. Integer that indicates how long to wait * (in milliseconds) for the promise group to complete. * @return: A promise that will contain the input promises results on completion. */ let resolveByCompletion = (promises, rejectOnError = true, timeout = null) => { return new Promise(async (resolve, reject) => { try { let results = [] let promiseMap = new Map(); Array.prototype.forEach.call(promises, (promise, index) => { let promiseResult = { index: index, value: null, error: null }; let mapValue = null; if (promise instanceof Promise) { mapValue = promise .then(value => {promiseResult.value = value; return promiseResult}) .catch(error => {promiseResult.error = error; return promiseResult}) } else { mapValue = promiseResult; promiseResult.value = promise; } promiseMap.set(index, mapValue); }); let start = Date.now(); let isTimedOut = () => { let result = false; if (timeout) { let elapsed = (Date.now() - start); result = elapsed >= timeout; } return result; } while (promiseMap.size > 0) { let promiseResult = await Promise.race(promiseMap.values()); if (!promiseMap.delete(promiseResult.index)) { throw new Error('Error occurred processing values'); } if (promiseResult.error) { if (rejectOnError) { reject(promiseResult.error); return; } results.push(promiseResult.error); } else { results.push(promiseResult.value); } if (isTimedOut()) { throw new Error(`Timeout of ${timeout}ms expired`); } } resolve(results); } catch (e) { reject(e); } }); } let loadedResources = new Map(); (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Resources)); // http://programmingnotes.org/ |
6. More Examples
Below are more examples demonstrating the use of ‘Resources.load‘. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Jul 7, 2020 // Taken From: http://programmingnotes.org/ // File: resourcesDemo.html // Description: Demonstrates the use of Resources.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes Resources.js Demo</title> <style> .main { text-align:center; margin-left:auto; margin-right:auto; } .output { text-align: left; } pre {outline: 1px solid #ccc; padding: 5px; margin: 5px; } .string { color: green; } .number { color: darkorange; } .boolean { color: blue; } .null { color: magenta; } .key { color: red; } </style> <!-- // Include module --> <script type="text/javascript" src="./Resources.js"></script> </head> <body> <div class="main"> My Programming Notes Resources.js Demo <pre><code><div class="output"></div></code></pre> </div> <script> (async () => { // Load files in parallel with undefined loading order let loadResult = await Resources.load({ files: [ { url: 'https://code.jquery.com/jquery-3.5.1.js', type: 'js' , wait: false }, { url: 'https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', type: 'js' , wait: false }, ], logResults: true, rejectOnError: false, timeout: null }); console.log(loadResult); print('Resources.load Status Result with undefined loading order', loadResult, 4); })(); </script> <script> (async () => { return; // Load files in parallel with defined loading order let loadResult = await Resources.load({ files: [ { url: 'https://code.jquery.com/jquery-3.5.1.js', type: 'js' , wait: true }, { url: 'https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', type: 'js' , wait: false }, ], logResults: true, rejectOnError: false, timeout: null }); console.log(loadResult); print('Resources.load Status Result with defined loading order', loadResult, 4); })(); </script> <script> (async () => { return; // Load fullname.js to get the 'fullname' functions await Resources.load({ files: ['./fullName.js'], logResults: true, }); let fullName = getFullName('Kenneth', 'P'); displayFullName(fullName); logFullName(fullName); })(); </script> <script> (async () => { return; // Load files one by one console.log(await Resources.load('/file1.js')); console.log(await Resources.load('/file2.js')); console.log(await Resources.load('/file3.css')); console.log(await Resources.load('/file4.css')); console.log(await Resources.load('https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js')); })(); </script> <script> function print(desc, obj, indent = 0) { let text = (desc.length > 0 ? '<br />' : '') + desc + (desc.length > 0 ? '<br />' : ''); let objText = obj || ''; if (obj && typeof obj != 'string') { objText = syntaxHighlight(JSON.stringify(obj, null, indent)); } text += objText; let pageText = document.querySelector('.output').innerHTML; pageText += (pageText.length > 0 ? '<br />' : '') + text; document.querySelector('.output').innerHTML = pageText; } function syntaxHighlight(json) { if (typeof json != 'string') { json = JSON.stringify(json, undefined, 2); } json = json.replace(/&/g, '&').replace(/</g, '<').replace(/>/g, '>'); return json.replace(/("(\\u[a-zA-Z0-9]{4}|\\[^u]|[^\\"])*"(\s*:)?|\b(true|false|null)\b|-?\d+(?:\.\d*)?(?:[eE][+\-]?\d+)?)/g, function (match) { let cls = 'number'; if (/^"/.test(match)) { if (/:$/.test(match)) { cls = 'key'; } else { cls = 'string'; } } else if (/true|false/.test(match)) { cls = 'boolean'; } else if (/null/.test(match)) { cls = 'null'; } return '<span class="' + cls + '">' + match + '</span>'; }); } </script> </body> </html><!-- // http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.