VB.NET || How To Shuffle & Randomize An Array/List/IEnumerable Using VB.NET
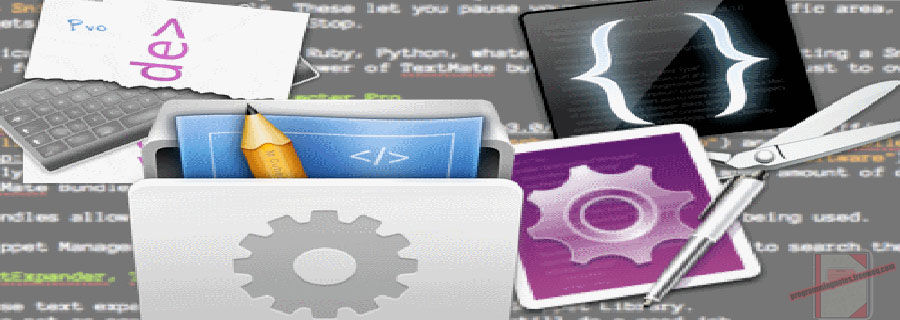
The following is a module with functions which demonstrates how to randomize and shuffle the contents of an Array/List/IEnumerable using VB.NET.
This function shuffles an IEnumerable and returns the results as a new List(Of T).
This function is generic, so it should work on IEnumerables of any datatype.
1. Shuffle – Integer Array
The example below demonstrates the use of ‘Utils.Shuffle‘ to randomize an integer array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
' Shuffle - Integer Array Imports Utils Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} Dim results = numbers.Shuffle For Each item In results Debug.Print($"{item}") Next ' example output: ' 2009 ' 1987 ' 31 ' 22 ' 28 ' 2019 ' 1991 ' 19 |
2. Shuffle – String List
The example below demonstrates the use of ‘Utils.Shuffle‘ to randomize a list of strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
' Shuffle - String List Imports Utils Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } Dim results = names.Shuffle For Each item In results Debug.Print($"{item}") Next ' example output: ' Sole ' Kenneth ' Lynn ' Jennifer |
3. Shuffle – Custom Object List
The example below demonstrates the use of ‘Utils.Shuffle‘ to randomize a list of custom objects.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
' Shuffle - Custom Object List Imports Utils Public Class Part Public Property PartName As String Public Property PartId As Integer End Class Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} Dim results = parts.Shuffle For Each item In results Debug.Print($"{item.PartId} {item.PartName}") Next ' example output: ' 1634 shift lever ' 1444 banana seat ' 1434 regular seat ' 1234 crank arm ' 1334 chain ring ' 1534 cassette |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 3, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Shuffles an IEnumerable and returns the results as a List ''' </summary> ''' <param name="list">The IEnumerable list to shuffle</param> ''' <returns>A List of shuffled items from the IEnumerable</returns> <Runtime.CompilerServices.Extension()> Function Shuffle(Of T)(list As IEnumerable(Of T)) As List(Of T) Dim r = New Random Dim shuffled = list.ToList For index = 0 To shuffled.Count - 1 Dim randomIndex = r.Next(index, shuffled.Count) If index <> randomIndex Then Dim temp = shuffled(index) shuffled(index) = shuffled(randomIndex) shuffled(randomIndex) = temp End If Next Return shuffled End Function End Module End Namespace ' http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 3, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Imports Utils Module Program Public Class Part Public Property PartName As String Public Property PartId As Integer End Class Sub Main(args As String()) Try Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} Dim results = numbers.Shuffle For Each item In results Display($"{item}") Next Display("") Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } Dim results1 = names.Shuffle For Each item In results1 Display($"{item}") Next Display("") Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} Dim results2 = parts.Shuffle For Each item In results2 Display($"{item.PartId} {item.PartName}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply