Monthly Archives: October 2022
C# || How To Return The Top K Frequent Words In Array Of Strings Using C#
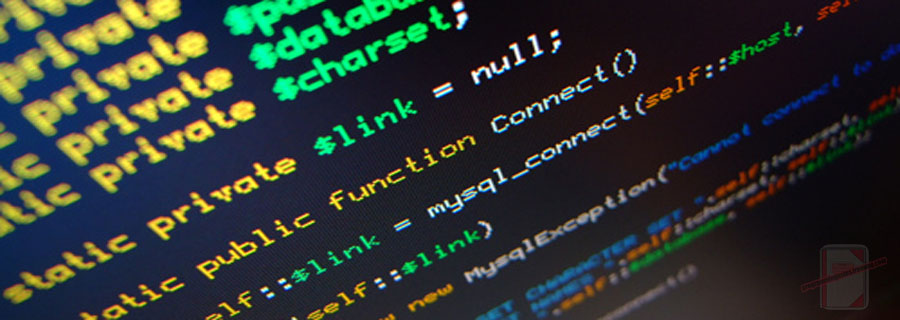
The following is a module with functions which demonstrates how to get the top K frequent words in an array of strings using C#.
1. Top K Frequent – Problem Statement
Given an array of strings words and an integer k, return the k most frequent strings.
Return the answer sorted by the frequency from highest to lowest. Sort the words with the same frequency by their lexicographical order.
Example 1:
Input: words = ["i","love","leetcode","i","love","coding"], k = 2
Output: ["i","love"]
Explanation: "i" and "love" are the two most frequent words.
Note that "i" comes before "love" due to a lower alphabetical order.
Example 2:
Input: words = ["the","day","is","sunny","the","the","the","sunny","is","is"], k = 4
Output: ["the","is","sunny","day"]
Explanation: "the", "is", "sunny" and "day" are the four most frequent words, with the number of occurrence being 4, 3, 2 and 1 respectively.
2. Top K Frequent – Solution
The following are two solutions which demonstrates how to get the top K frequent words in an array of strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 23, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Determines how to get the top K frequent words in array // ============================================================================ public class Solution { public IList<string> TopKFrequent(string[] words, int k) { // Use a map to get the frequency of each word var frequency = new Dictionary<string, int>(); foreach (var word in words) { frequency[word] = frequency.GetValueOrDefault(word, 0) + 1; } // Get the frequency map as a list and sort var frequencyList = frequency.ToList(); frequencyList.Sort((a, b) => { // If words have the same frequency, sort // by their lexicographical order if (a.Value == b.Value) { return string.Compare(a.Key, b.Key); } // Sort by the frequency from highest to lowest return b.Value - a.Value; }); // Get the results var result = new List<string>(); for (int index = 0; index < frequencyList.Count; ++index) { result.Add(frequencyList[index].Key); if (result.Count == k) { break; } } return result; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
["i","love"]
["the","is","sunny","day"]
C# || Two Sum IV – How To Get Two Numbers In Binary Search Tree Equal To Target Value Using C#
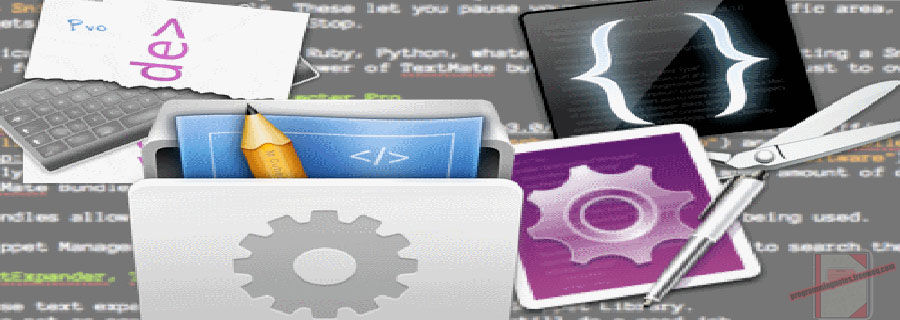
The following is a module with functions which demonstrates how to get two numbers in a binary search tree equal to target value using C#.
1. Find Target – Problem Statement
Given the root of a Binary Search Tree and a target number k, return true if there exist two elements in the BST such that their sum is equal to the given target.
Example 1:
Input: root = [5,3,6,2,4,null,7], k = 9
Output: true
Example 2:
Input: root = [5,3,6,2,4,null,7], k = 28
Output: false
2. Find Target – Solution
The following are two solutions which demonstrates how to get two numbers in a binary search tree equal to target value.
Both solutions use a set to keep track of the items already seen.
Each time a new node is encountered, we subtract the target value from the current node value. If the difference amount from subtracting the two numbers exists in the set, a 2 sum combination exists in the tree
1. Recursive
The following solution uses Depth First Search when looking for the target value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 9, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to get two numbers equal to target value // ============================================================================ public class Solution { public bool FindTarget(TreeNode root, int k) { return Traverse(root, k, new HashSet<int>()); } private bool Traverse(TreeNode node, int k, HashSet<int> seen) { if (node == null) { return false; } // Get remaining value var remaining = k - node.val; // Check if remaining value has been seen if (seen.Contains(remaining)) { return true; } // Add current node value to items seen seen.Add(node.val); // Keep traversing left and right looking for 2 sum return Traverse(node.left, k, seen) || Traverse(node.right, k, seen); } }// http://programmingnotes.org/ |
2. Iterative
The following solution uses Breadth First Search when looking for the target value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 9, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to get two numbers equal to target value // ============================================================================ public class Solution { public bool FindTarget(TreeNode root, int k) { if (root == null) { return false; } // Declare stack var stack = new Stack<TreeNode>(); // Keep track of values seen var seen = new HashSet<int>(); // Add root to stack stack.Push(root); // Loop through items on the stack while (stack.Count > 0) { // Get current node var node = stack.Pop(); // Get remaining value var remaining = k - node.val; // Check if remaining value has been seen if (seen.Contains(remaining)) { return true; } else { // Keep traversing left and right looking for 2 sum if (node.left != null) { stack.Push(node.left); } if (node.right != null) { stack.Push(node.right); } } // Add current node value to items seen seen.Add(node.val); } return false; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
true
false
C# || Counting Bits – How To Return The Number Of 1’s In Binary Representation Of X Using C#
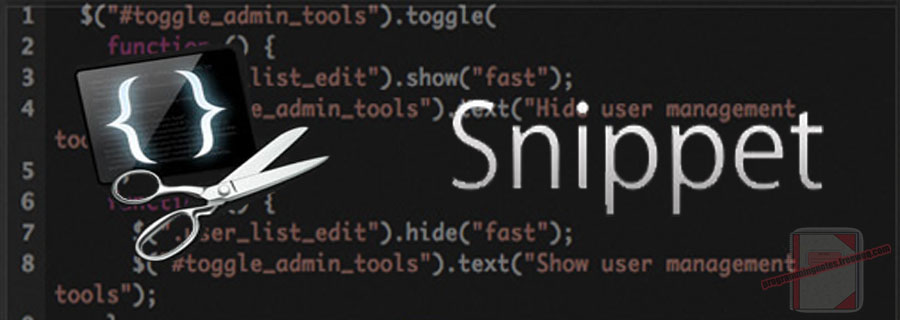
The following is a module with functions which demonstrates how to return the number of 1’s in binary representation of X using C#.
1. Count Bits – Problem Statement
Given an integer n, return an array ans of length n + 1 such that for each i (0 <= i <= n), ans[i] is the number of 1‘s in the binary representation of i.
Example 1:
Input: n = 2
Output: [0,1,1]
Explanation:
0 --> 0
1 --> 1
2 --> 10
Example 2:
Input: n = 5
Output: [0,1,1,2,1,2]
Explanation:
0 --> 0
1 --> 1
2 --> 10
3 --> 11
4 --> 100
5 --> 101
2. Count Bits – Solution
The following is a solution which demonstrates how return the number of 1’s in binary representation of X.
In this problem, we can see a pattern start to form.
When the current n is even, we can get the answer by dividing by 2 (e.g result[n] = result[n / 2])
When the current n is odd, we can get the answer by getting the result at previous index and adding 1 (e.g result[n] = result[n – 1] + 1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 2, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to return binary representation of X // ============================================================================ public class Solution { public int[] CountBits(int n) { var result = new int[n + 1]; result[0] = 0; for (int index = 1; index < result.Length; ++index) { if (index % 2 == 0) { result[index] = result[index / 2]; } else { result[index] = result[index - 1] + 1; } } return result; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[0,1,1]
[0,1,1,2,1,2]