C# || How To Find Largest 3 Same Digit Number In String Using C#
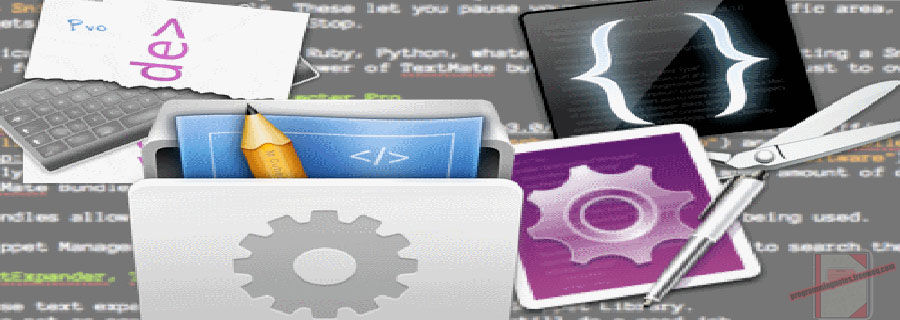
The following is a module with functions which demonstrates how to find the largest 3 same digit number in a string using C#.
1. Largest Good Integer – Problem Statement
You are given a string num representing a large integer. An integer is good if it meets the following conditions:
- It is a substring of num with length 3.
- It consists of only one unique digit.
Return the maximum good integer as a string or an empty string “” if no such integer exists.
Note:
- A substring is a contiguous sequence of characters within a string.
- There may be leading zeroes in num or a good integer.
Example 1:
Input: num = "6777133339"
Output: "777"
Explanation: There are two distinct good integers: "777" and "333".
"777" is the largest, so we return "777".
Example 2:
Input: num = "2300019"
Output: "000"
Explanation: "000" is the only good integer.
Example 3:
Input: num = "42352338"
Output: ""
Explanation: No substring of length 3 consists of only one unique digit. Therefore, there are no good integers.
2. Largest Good Integer – Solution
The following is a solution which demonstrates how to find the largest 3 same digit number in a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 23, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find largest odd number in a string // ============================================================================ public class Solution { public string LargestGoodInteger(string num) { // Assign 'maxDigit' to the NUL character (smallest ASCII value character) char maxDigit = '\0'; // Iterate on characters of the num string. for (int index = 0; index <= num.Length - 3; ++index) { // If 3 consecutive characters are the same, // store the character in 'maxDigit' if it's bigger than what it already stores. if (num[index] == num[index + 1] && num[index] == num[index + 2]) { maxDigit = (char) Math.Max(maxDigit, num[index]); } } // If 'maxDigit' is NUL, return an empty string; otherwise, return a string of size 3 with 'maxDigit' characters. return maxDigit == '\0' ? "" : new string(new char[]{maxDigit, maxDigit, maxDigit}); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
"777"
"000"
""
Leave a Reply