Tag Archives: leetcode
C# || How To Design Graph With Shortest Path Calculator Using C#
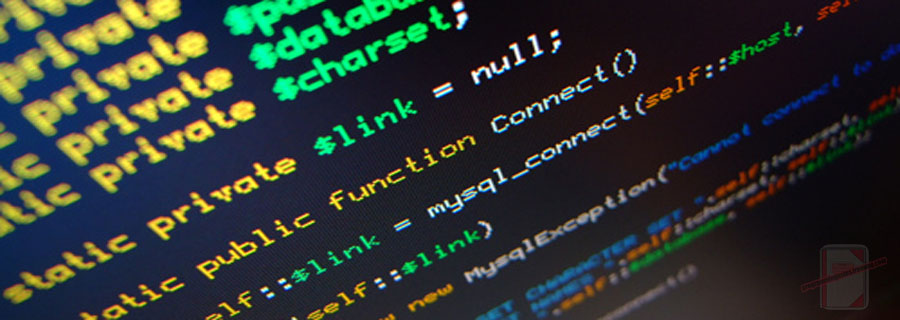
The following is a module with functions which demonstrates how to design graph with shortest path calculator using C#.
1. Graph – Problem Statement
There is a directed weighted graph that consists of n nodes numbered from 0 to n – 1. The edges of the graph are initially represented by the given array edges where edges[i] = [fromi, toi, edgeCosti] meaning that there is an edge from fromi to toi with the cost edgeCosti.
Implement the Graph class:
- Graph(int n, int[][] edges) initializes the object with n nodes and the given edges.
- addEdge(int[] edge) adds an edge to the list of edges where edge = [from, to, edgeCost]. It is guaranteed that there is no edge between the two nodes before adding this one.
- int shortestPath(int node1, int node2) returns the minimum cost of a path from node1 to node2. If no path exists, return -1. The cost of a path is the sum of the costs of the edges in the path.
Example 1:
Input
["Graph", "shortestPath", "shortestPath", "addEdge", "shortestPath"]
[[4, [[0, 2, 5], [0, 1, 2], [1, 2, 1], [3, 0, 3]]], [3, 2], [0, 3], [[1, 3, 4]], [0, 3]]
Output
[null, 6, -1, null, 6]Explanation
Graph g = new Graph(4, [[0, 2, 5], [0, 1, 2], [1, 2, 1], [3, 0, 3]]);
g.shortestPath(3, 2); // return 6. The shortest path from 3 to 2 in the first diagram above is 3 -> 0 -> 1 -> 2 with a total cost of 3 + 2 + 1 = 6.
g.shortestPath(0, 3); // return -1. There is no path from 0 to 3.
g.addEdge([1, 3, 4]); // We add an edge from node 1 to node 3, and we get the second diagram above.
g.shortestPath(0, 3); // return 6. The shortest path from 0 to 3 now is 0 -> 1 -> 3 with a total cost of 2 + 4 = 6.
2. Graph – Solution
The following is a solution which demonstrates how to design graph with shortest path calculator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jun 1, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to design graph shortest path calculator // ============================================================================ /** * Your Graph object will be instantiated and called as such: * Graph obj = new Graph(n, edges); * obj.AddEdge(edge); * int param_2 = obj.ShortestPath(node1,node2); */ public class Graph { List<List<KeyValuePair<int, int>>> adjList; public Graph(int n, int[][] edges) { adjList = new List<List<KeyValuePair<int, int>>>(); for (int i = 0; i < n; i++) { adjList.Add(new List<KeyValuePair<int, int>>()); } foreach (int[] e in edges) { AddEdge(e); } } public void AddEdge(int[] edge) { adjList[edge[0]].Add(new KeyValuePair<int, int>(edge[1], edge[2])); } public int ShortestPath(int node1, int node2) { int n = adjList.Count; var pq = new PriorityQueue<List<int>, List<int>>( Comparer<List<int>>.Create((a, b) => a[0].CompareTo(b[0])) ); int[] costForNode = new int[n]; Array.Fill(costForNode, int.MaxValue); costForNode[node1] = 0; var root = new List<int>() { 0, node1 }; pq.Enqueue(root, root); while (pq.Count > 0) { var curr = pq.Dequeue(); int currCost = curr[0]; int currNode = curr[1]; if (currCost > costForNode[currNode]) { continue; } if (currNode == node2) { return currCost; } foreach (var neighbor in adjList[currNode]) { int neighborNode = neighbor.Key; int cost = neighbor.Value; int newCost = currCost + cost; if (newCost < costForNode[neighborNode]) { costForNode[neighborNode] = newCost; var newPath = new List<int>() { newCost, neighborNode }; pq.Enqueue(newPath, newPath); } } } return -1; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[null,6,-1,null,6]
C# || How To Find Largest 3 Same Digit Number In String Using C#
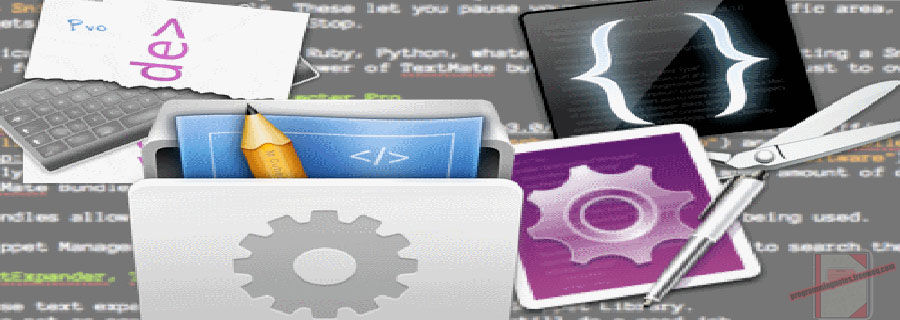
The following is a module with functions which demonstrates how to find the largest 3 same digit number in a string using C#.
1. Largest Good Integer – Problem Statement
You are given a string num representing a large integer. An integer is good if it meets the following conditions:
- It is a substring of num with length 3.
- It consists of only one unique digit.
Return the maximum good integer as a string or an empty string “” if no such integer exists.
Note:
- A substring is a contiguous sequence of characters within a string.
- There may be leading zeroes in num or a good integer.
Example 1:
Input: num = "6777133339"
Output: "777"
Explanation: There are two distinct good integers: "777" and "333".
"777" is the largest, so we return "777".
Example 2:
Input: num = "2300019"
Output: "000"
Explanation: "000" is the only good integer.
Example 3:
Input: num = "42352338"
Output: ""
Explanation: No substring of length 3 consists of only one unique digit. Therefore, there are no good integers.
2. Largest Good Integer – Solution
The following is a solution which demonstrates how to find the largest 3 same digit number in a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 23, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find largest odd number in a string // ============================================================================ public class Solution { public string LargestGoodInteger(string num) { // Assign 'maxDigit' to the NUL character (smallest ASCII value character) char maxDigit = '\0'; // Iterate on characters of the num string. for (int index = 0; index <= num.Length - 3; ++index) { // If 3 consecutive characters are the same, // store the character in 'maxDigit' if it's bigger than what it already stores. if (num[index] == num[index + 1] && num[index] == num[index + 2]) { maxDigit = (char) Math.Max(maxDigit, num[index]); } } // If 'maxDigit' is NUL, return an empty string; otherwise, return a string of size 3 with 'maxDigit' characters. return maxDigit == '\0' ? "" : new string(new char[]{maxDigit, maxDigit, maxDigit}); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
"777"
"000"
""
C# || How To Find Largest Odd Number In String Using C#
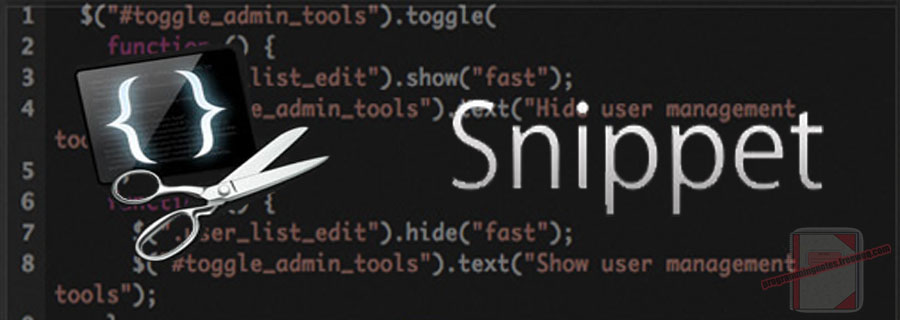
The following is a module with functions which demonstrates how to find the largest odd number in a string using C#.
1. Largest Odd Number – Problem Statement
You are given a string num, representing a large integer. Return the largest-valued odd integer (as a string) that is a non-empty substring of num, or an empty string “” if no odd integer exists.
A substring is a contiguous sequence of characters within a string.
Example 1:
Input: num = "52"
Output: "5"
Explanation: The only non-empty substrings are "5", "2", and "52". "5" is the only odd number.
Example 2:
Input: num = "4206"
Output: ""
Explanation: There are no odd numbers in "4206".
Example 3:
Input: num = "35427"
Output: "35427"
Explanation: "35427" is already an odd number.
2. Largest Odd Number – Solution
The following is a solution which demonstrates how to find the largest odd number in a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// ============================================================================ // Author: Kenneth Perkins // Date: Apr 13, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find largest odd number in a string // ============================================================================ public class Solution { public string LargestOddNumber(string num) { for (int index = num.Length - 1; index >= 0; --index) { if (Convert.ToInt32(num[index]) % 2 != 0) { return num.Substring(0, index + 1); } } return ""; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
"5"
""
"35427"
C# || How To Determine If A Binary Tree Is Even Odd Tree Using C#
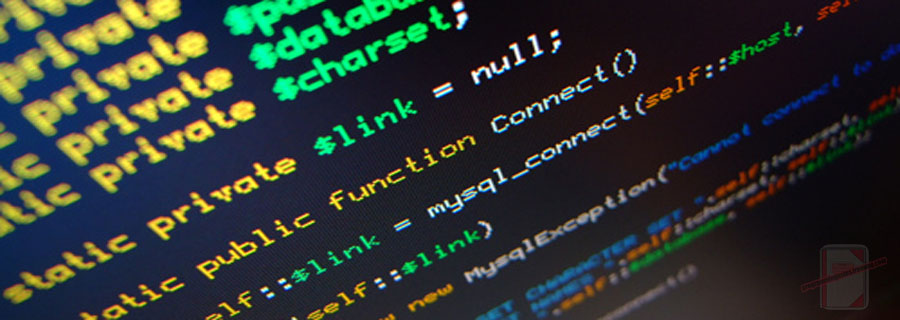
The following is a module with functions which demonstrates how to determine if a binary tree is an even odd tree using C#.
1. Is Even Odd Tree – Problem Statement
A binary tree is named Even-Odd if it meets the following conditions:
- The root of the binary tree is at level index 0, its children are at level index 1, their children are at level index 2, etc.
- For every even-indexed level, all nodes at the level have odd integer values in strictly increasing order (from left to right).
- For every odd-indexed level, all nodes at the level have even integer values in strictly decreasing order (from left to right).
Given the root of a binary tree, return true if the binary tree is Even-Odd, otherwise return false.
Example 1:
Input: root = [1,10,4,3,null,7,9,12,8,6,null,null,2]
Output: true
Explanation: The node values on each level are:
Level 0: [1]
Level 1: [10,4]
Level 2: [3,7,9]
Level 3: [12,8,6,2]
Since levels 0 and 2 are all odd and increasing and levels 1 and 3 are all even and decreasing, the tree is Even-Odd.
Example 2:
Input: root = [5,4,2,3,3,7]
Output: false
Explanation: The node values on each level are:
Level 0: [5]
Level 1: [4,2]
Level 2: [3,3,7]
Node values in level 2 must be in strictly increasing order, so the tree is not Even-Odd.
Example 3:
Input: root = [5,9,1,3,5,7]
Output: false
Explanation: Node values in the level 1 should be even integers.
2. Is Even Odd Tree – Solution
The following is a solution which demonstrates how to determine if a binary tree is an even odd tree.
This solution uses breadth-first search.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
// ============================================================================ // Author: Kenneth Perkins // Date: Mar 1, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to determine an even odd tree // ============================================================================ /** * Definition for a binary tree node. * public class TreeNode { * public int val; * public TreeNode left; * public TreeNode right; * public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) { * this.val = val; * this.left = left; * this.right = right; * } * } */ public class Solution { public bool IsEvenOddTree(TreeNode root) { var queue = new Queue<TreeNode>(); queue.Enqueue(root); var isEvenLevel = true; while (queue.Count > 0) { int previousValue = isEvenLevel ? int.MinValue : int.MaxValue; for (var size = queue.Count - 1; size >= 0; --size) { var currentNode = queue.Dequeue(); if (isEvenLevel) { if (IsEven(currentNode.val) || previousValue >= currentNode.val) { return false; } } else { if (!IsEven(currentNode.val) || previousValue <= currentNode.val) { return false; } } if (currentNode.left != null) { queue.Enqueue(currentNode.left); } if (currentNode.right != null) { queue.Enqueue(currentNode.right); } previousValue = currentNode.val; } isEvenLevel = !isEvenLevel; } return true; } private bool IsEven(int value) { return value % 2 == 0; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
true
false
false
C# || How To Group People Given The Group Size They Belong To Using C#
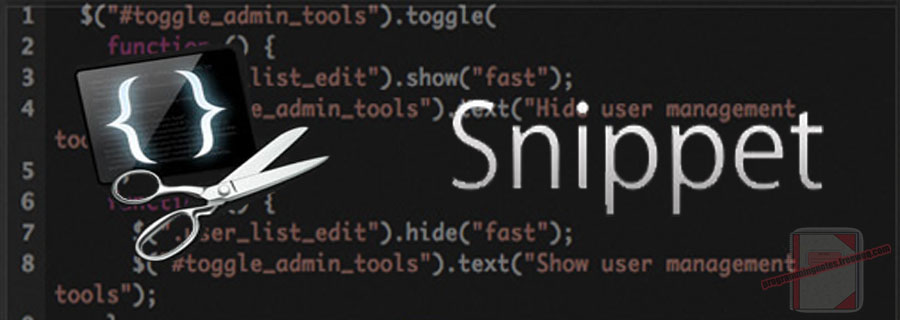
The following is a module with functions which demonstrates how to group people given the group size they belong to using C#.
1. Group The People – Problem Statement
There are n people that are split into some unknown number of groups. Each person is labeled with a unique ID from 0 to n – 1.
You are given an integer array groupSizes, where groupSizes[i] is the size of the group that person i is in. For example, if groupSizes[1] = 3, then person 1 must be in a group of size 3.
Return a list of groups such that each person i is in a group of size groupSizes[i].
Each person should appear in exactly one group, and every person must be in a group. If there are multiple answers, return any of them. It is guaranteed that there will be at least one valid solution for the given input.
Example 1:
Input: groupSizes = [3,3,3,3,3,1,3]
Output: [[5],[0,1,2],[3,4,6]]
Explanation:
The first group is [5]. The size is 1, and groupSizes[5] = 1.
The second group is [0,1,2]. The size is 3, and groupSizes[0] = groupSizes[1] = groupSizes[2] = 3.
The third group is [3,4,6]. The size is 3, and groupSizes[3] = groupSizes[4] = groupSizes[6] = 3.
Other possible solutions are [[2,1,6],[5],[0,4,3]] and [[5],[0,6,2],[4,3,1]].
Example 2:
Input: groupSizes = [2,1,3,3,3,2]
Output: [[1],[0,5],[2,3,4]]
2. Group The People – Solution
The following is a solution which demonstrates how to group people given the group size they belong to.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 14, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to group people given group they belong to // ============================================================================ public class Solution { public IList<IList<int>> GroupThePeople(int[] groupSizes) { var ans = new List<IList<int>>(); // A map from group size to the list of indices that are there in the group. var szToGroup = new Dictionary<int, List<int>>(); for (int i = 0; i < groupSizes.Length; i++) { if (!szToGroup.ContainsKey(groupSizes[i])) { szToGroup.Add(groupSizes[i], new List<int>()); } var group = szToGroup[groupSizes[i]]; group.Add(i); // When the list size equals the group size, empty it and store it in the answer. if (group.Count == groupSizes[i]) { ans.Add(group); szToGroup.Remove(groupSizes[i]); } } return ans; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[[0,1,2],[5],[3,4,6]]
[[1],[2,3,4],[0,5]]
C# || How To Find Minimum Deletions To Make Character Frequencies Unique Using C#
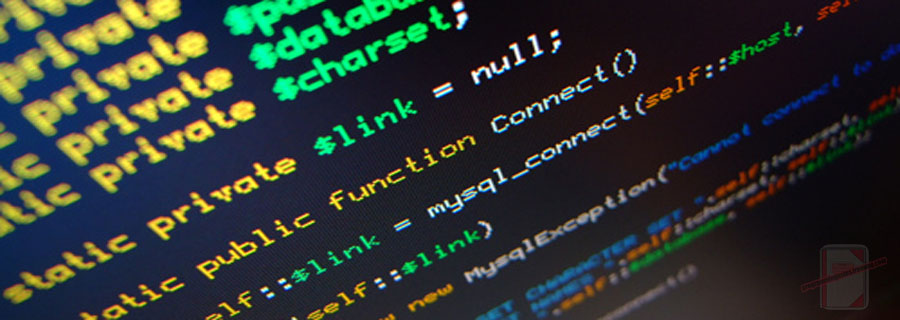
The following is a module with functions which demonstrates how to find the minimum deletions to make character frequencies unique using C#.
1. Min Deletions – Problem Statement
A string s is called good if there are no two different characters in s that have the same frequency.
Given a string s, return the minimum number of characters you need to delete to make s good.
The frequency of a character in a string is the number of times it appears in the string. For example, in the string “aab”, the frequency of ‘a’ is 2, while the frequency of ‘b’ is 1.
Example 1:
Input: s = "aab"
Output: 0
Explanation:s
is already good.
Example 2:
Input: s = "aaabbbcc"
Output: 2
Explanation: You can delete two 'b's resulting in the good string "aaabcc".
Another way it to delete one 'b' and one 'c' resulting in the good string "aaabbc".
Example 3:
Input: s = "ceabaacb"
Output: 2
Explanation: You can delete both 'c's resulting in the good string "eabaab".
Note that we only care about characters that are still in the string at the end (i.e. frequency of 0 is ignored).
2. Min Deletions – Solution
The following is a solution which demonstrates how to find the minimum deletions to make character frequencies unique.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 1, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find minimum deletions to make unique // ============================================================================ public class Solution { public int MinDeletions(string s) { // Store the frequency of each character int[] frequency = new int[26]; for (int i = 0; i < s.Length; i++) { frequency[s[i] - 'a']++; } Array.Sort(frequency); int deleteCount = 0; // Maximum frequency the current character can have int maxFreqAllowed = s.Length; // Iterate over the frequencies in descending order for (int i = 25; i >= 0 && frequency[i] > 0; i--) { // Delete characters to make the frequency equal the maximum frequency allowed if (frequency[i] > maxFreqAllowed) { deleteCount += frequency[i] - maxFreqAllowed; frequency[i] = maxFreqAllowed; } // Update the maximum allowed frequency maxFreqAllowed = Math.Max(0, frequency[i] - 1); } return deleteCount; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
0
2
2
C# || Reconstruct Itinerary – How To Reconstruct Itinerary In Order Using C#
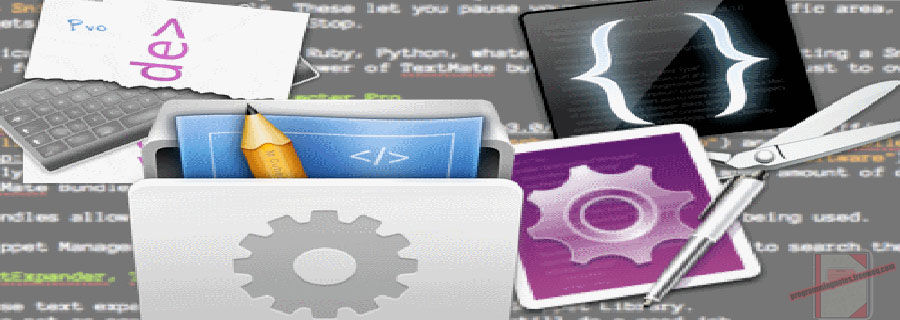
The following is a module with functions which demonstrates how to reconstruct itinerary in order using C#.
1. Find Itinerary – Problem Statement
You are given a list of airline tickets where tickets[i] = [fromi, toi] represent the departure and the arrival airports of one flight. Reconstruct the itinerary in order and return it.
All of the tickets belong to a man who departs from “JFK”, thus, the itinerary must begin with “JFK”. If there are multiple valid itineraries, you should return the itinerary that has the smallest lexical order when read as a single string.
- For example, the itinerary [“JFK”, “LGA”] has a smaller lexical order than [“JFK”, “LGB”].
You may assume all tickets form at least one valid itinerary. You must use all the tickets once and only once.
Example 1:
Input: tickets = [["MUC","LHR"],["JFK","MUC"],["SFO","SJC"],["LHR","SFO"]]
Output: ["JFK","MUC","LHR","SFO","SJC"]
Example 2:
Input: tickets = [["JFK","SFO"],["JFK","ATL"],["SFO","ATL"],["ATL","JFK"],["ATL","SFO"]]
Output: ["JFK","ATL","JFK","SFO","ATL","SFO"]
Explanation: Another possible reconstruction is ["JFK","SFO","ATL","JFK","ATL","SFO"] but it is larger in lexical order.
2. Find Itinerary – Solution
The following is a solution which demonstrates how to reconstruct itinerary in order.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 1, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to reconstruct itinerary in order // ============================================================================ public class Solution { public IList<string> FindItinerary(IList<IList<string>> tickets) { var map = new Dictionary<string, List<string>>(); for (int index = 0; index < tickets.Count; ++index) { List<string> ticket = tickets[index].ToList(); string from = ticket[0]; string to = ticket[1]; if (!map.ContainsKey(from)) { map[from] = new List<string>(); } map[from].Add(to); } foreach (List<string> list in map.Values) { list.Sort(); } var result = new List<string>(); string start = "JFK"; result.Add(start); DFS(map, start, result, tickets.Count); return result; } private bool DFS(Dictionary<string, List<string>> map, string cur, List<string> result, int totalTicket) { if (result.Count == totalTicket + 1) { return true; } if (!map.ContainsKey(cur)) { return false; } List<string> nextList = map[cur]; for (int index = 0; index < nextList.Count; ++index) { string next = nextList[index]; if (next == null) { continue; } nextList[index] = null; result.Add(next); if (DFS(map, next, result, totalTicket)) { return true; } // back track result.RemoveAt(result.Count - 1); nextList[index] = next; } return false; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
["JFK","MUC","LHR","SFO","SJC"]
["JFK","ATL","JFK","SFO","ATL","SFO"]
C# || How To Find Minimum Operations To Reduce X To Zero Using C#
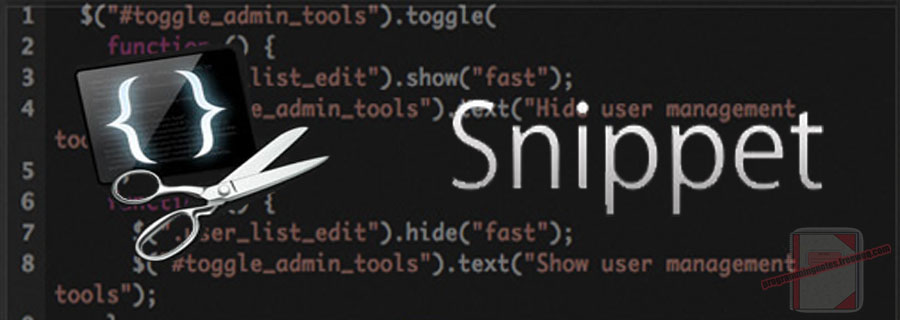
The following is a module with functions which demonstrates how to find the minimum number of operations to reduce X to zero using C#.
1. Min Operations – Problem Statement
You are given an integer array nums and an integer x. In one operation, you can either remove the leftmost or the rightmost element from the array nums and subtract its value from x. Note that this modifies the array for future operations.
Return the minimum number of operations to reduce x to exactly 0 if it is possible, otherwise, return -1.
Example 1:
Input: nums = [1,1,4,2,3], x = 5
Output: 2
Explanation: The optimal solution is to remove the last two elements to reduce x to zero.
Example 2:
Input: nums = [5,6,7,8,9], x = 4
Output: -1
Example 3:
Input: nums = [3,2,20,1,1,3], x = 10
Output: 5
Explanation: The optimal solution is to remove the last three elements and the first two elements (5 operations in total) to reduce x to zero.
2. Min Operations – Solution
The following is a solution which demonstrates how to find the minimum number of operations to reduce X to zero.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 25, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find the minimum operations reduce X // ============================================================================ public class Solution { public int MinOperations(int[] nums, int x) { var target = -x; foreach (int num in nums) { target += num; } // since all elements are positive, we have to take all of them if (target == 0) { return nums.Length; } var map = new Dictionary<int, int>(); map[0] = -1; var sum = 0; var res = int.MinValue; for (var index = 0; index < nums.Length; ++index) { sum += nums[index]; if (map.ContainsKey(sum - target)) { res = Math.Max(res, index - map[sum - target]); } // no need to check containsKey since sum is unique map[sum] = index; } return res == int.MinValue ? -1 : nums.Length - res; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
2
-1
5
C# || How To Sort An Array By Parity Using C#
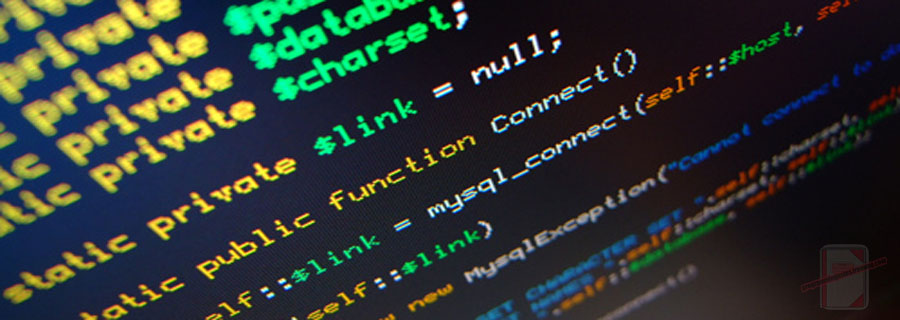
The following is a module with functions which demonstrates how to sort an array by parity using C#.
1. Sort Array By Parity – Problem Statement
Given an integer array nums, move all the even integers at the beginning of the array followed by all the odd integers.
Return any array that satisfies this condition.
Example 1:
Input: nums = [3,1,2,4]
Output: [2,4,3,1]
Explanation: The outputs [4,2,3,1], [2,4,1,3], and [4,2,1,3] would also be accepted.
Example 2:
Input: nums = [0]
Output: [0]
2. Sort Array By Parity – Solution
The following is a solution which demonstrates how to sort an array by parity.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 1, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to sort an array by parity // ============================================================================ public class Solution { public int[] SortArrayByParity(int[] nums) { var startIndex = 0; var endIndex = nums.Length -1; while (startIndex < endIndex) { if (IsOdd(nums[startIndex])) { var temp = nums[startIndex]; nums[startIndex] = nums[endIndex]; nums[endIndex] = temp; --endIndex; } else { ++startIndex; } } return nums; } private bool IsOdd(int num) { return num % 2 != 0; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[4,2,1,3]
[0]
C# || Parallel Courses III – How To Find Minimum Number Months To Complete All Courses Using C#
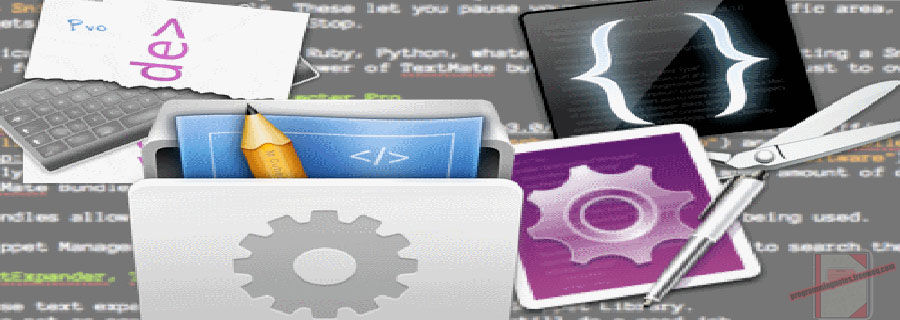
The following is a module with functions which demonstrates how to find the minimum number of months needed to complete all courses using C#.
1. Minimum Time – Problem Statement
You are given an integer n, which indicates that there are n courses labeled from 1 to n. You are also given a 2D integer array relations where relations[j] = [prevCoursej, nextCoursej] denotes that course prevCoursej has to be completed before course nextCoursej (prerequisite relationship). Furthermore, you are given a 0-indexed integer array time where time[i] denotes how many months it takes to complete the (i+1)th course.
You must find the minimum number of months needed to complete all the courses following these rules:
- You may start taking a course at any time if the prerequisites are met.
- Any number of courses can be taken at the same time.
Return the minimum number of months needed to complete all the courses.
Note: The test cases are generated such that it is possible to complete every course (i.e., the graph is a directed acyclic graph).
Example 1:
Input: n = 3, relations = [[1,3],[2,3]], time = [3,2,5]
Output: 8
Explanation: The figure above represents the given graph and the time required to complete each course.
We start course 1 and course 2 simultaneously at month 0.
Course 1 takes 3 months and course 2 takes 2 months to complete respectively.
Thus, the earliest time we can start course 3 is at month 3, and the total time required is 3 + 5 = 8 months.
Example 2:
Input: n = 5, relations = [[1,5],[2,5],[3,5],[3,4],[4,5]], time = [1,2,3,4,5]
Output: 12
Explanation: The figure above represents the given graph and the time required to complete each course.
You can start courses 1, 2, and 3 at month 0.
You can complete them after 1, 2, and 3 months respectively.
Course 4 can be taken only after course 3 is completed, i.e., after 3 months. It is completed after 3 + 4 = 7 months.
Course 5 can be taken only after courses 1, 2, 3, and 4 have been completed, i.e., after max(1,2,3,7) = 7 months.
Thus, the minimum time needed to complete all the courses is 7 + 5 = 12 months.
2. Minimum Time – Solution
The following is a solution which demonstrates how to find the minimum number of months needed to complete all courses.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
// ============================================================================ // Author: Kenneth Perkins // Date: Nov 1, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find the minimum months complete courses // ============================================================================ public class Solution { public int MinimumTime(int n, int[][] relations, int[] time) { var graph = new Dictionary<int, List<int>>(); for (int i = 0; i < n; i++) { graph[i] = new List<int>(); } int[] indegree = new int[n]; foreach (int[] edge in relations) { int x = edge[0] - 1; int y = edge[1] - 1; graph[x].Add(y); indegree[y]++; } var queue = new Queue<int>(); int[] maxTime = new int[n]; for (int node = 0; node < n; node++) { if (indegree[node] == 0) { queue.Enqueue(node); maxTime[node] = time[node]; } } while (queue.Count > 0) { int node = queue.Dequeue(); foreach (int neighbor in graph[node]) { maxTime[neighbor] = Math.Max(maxTime[neighbor], maxTime[node] + time[neighbor]); indegree[neighbor]--; if (indegree[neighbor] == 0) { queue.Enqueue(neighbor); } } } int ans = 0; for (int node = 0; node < n; node++) { ans = Math.Max(ans, maxTime[node]); } return ans; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
8
12
C# || How To Find Largest Value In Each Binary Tree Row Using C#
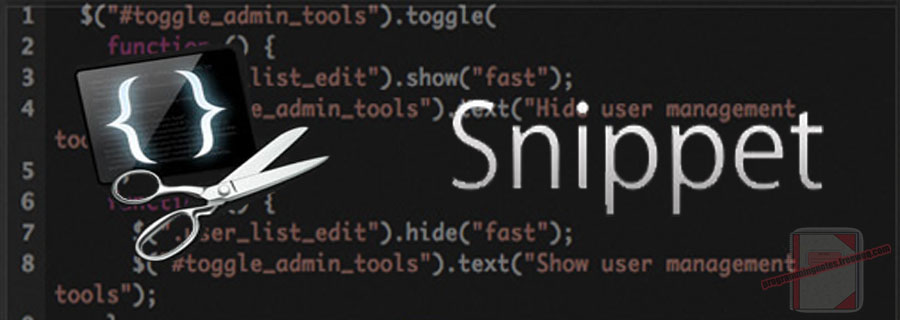
The following is a module with functions which demonstrates how to find the largest value in each binary tree row using C#.
1. Largest Values – Problem Statement
Given the root of a binary tree, return an array of the largest value in each row of the tree (0-indexed).
Example 1:
Input: root = [1,3,2,5,3,null,9]
Output: [1,3,9]
Example 2:
Input: root = [1,2,3]
Output: [1,3]
2. Largest Values – Solution
The following is a solution which demonstrates how to find the largest value in each binary tree row.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 28, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find the largest value binary tree row // ============================================================================ /** * Definition for a binary tree node. * public class TreeNode { * public int val; * public TreeNode left; * public TreeNode right; * public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) { * this.val = val; * this.left = left; * this.right = right; * } * } */ public class Solution { public IList<int> LargestValues(TreeNode root) { if (root == null) { return new List<int>(); } var ans = new List<int>(); var queue = new Queue<TreeNode>(); queue.Enqueue(root); while (queue.Count > 0) { int currMax = int.MinValue; for (int i = queue.Count -1; i >= 0; --i) { var node = queue.Dequeue(); currMax = Math.Max(currMax, node.val); if (node.left != null) { queue.Enqueue(node.left); } if (node.right != null) { queue.Enqueue(node.right); } } ans.Add(currMax); } return ans; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[1,3,9]
[1,3]
C# || How To Find The Minimum Speed To Arrive On Time Using C#
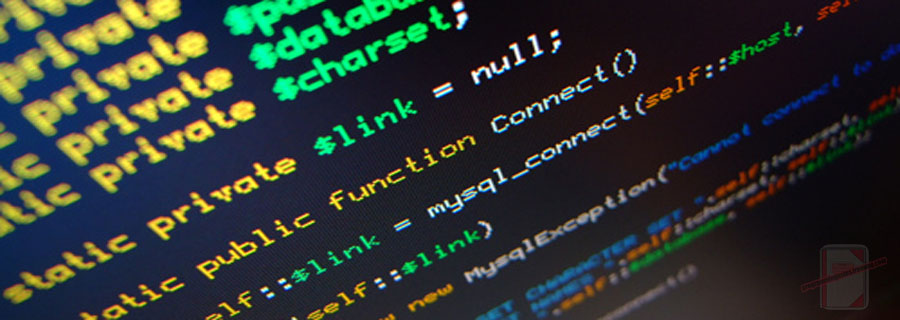
The following is a module with functions which demonstrates how to find the minimum speed to arrive on time using C#.
1. Min Speed On Time – Problem Statement
You are given a floating-point number hour, representing the amount of time you have to reach the office. To commute to the office, you must take n trains in sequential order. You are also given an integer array dist of length n, where dist[i] describes the distance (in kilometers) of the ith train ride.
Each train can only depart at an integer hour, so you may need to wait in between each train ride.
- For example, if the 1st train ride takes 1.5 hours, you must wait for an additional 0.5 hours before you can depart on the 2nd train ride at the 2 hour mark.
Return the minimum positive integer speed (in kilometers per hour) that all the trains must travel at for you to reach the office on time, or -1 if it is impossible to be on time.
Tests are generated such that the answer will not exceed 107 and hour will have at most two digits after the decimal point.
Example 1:
Input: dist = [1,3,2], hour = 6
Output: 1
Explanation: At speed 1:
- The first train ride takes 1/1 = 1 hour.
- Since we are already at an integer hour, we depart immediately at the 1 hour mark. The second train takes 3/1 = 3 hours.
- Since we are already at an integer hour, we depart immediately at the 4 hour mark. The third train takes 2/1 = 2 hours.
- You will arrive at exactly the 6 hour mark.
Example 2:
Input: dist = [1,3,2], hour = 2.7
Output: 3
Explanation: At speed 3:
- The first train ride takes 1/3 = 0.33333 hours.
- Since we are not at an integer hour, we wait until the 1 hour mark to depart. The second train ride takes 3/3 = 1 hour.
- Since we are already at an integer hour, we depart immediately at the 2 hour mark. The third train takes 2/3 = 0.66667 hours.
- You will arrive at the 2.66667 hour mark.
Example 3:
Input: dist = [1,3,2], hour = 1.9
Output: -1
Explanation: It is impossible because the earliest the third train can depart is at the 2 hour mark.
2. Min Speed On Time – Solution
The following is a solution which demonstrates how to find the minimum speed to arrive on time.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Sep 1, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find the minimum speed to arrive on time // ============================================================================ public class Solution { public int MinSpeedOnTime(int[] dist, double hour) { int left = 1; int right = 10000000; int minSpeed = -1; while (left <= right) { int mid = left + (right - left) / 2; // Move to the left half. if (TimeRequired(dist, mid) <= hour) { minSpeed = mid; right = mid - 1; } else { // Move to the right half. left = mid + 1; } } return minSpeed; } double TimeRequired(int[] dist, int speed) { double time = 0.0; for (int i = 0 ; i < dist.Length; i++) { double t = (double) dist[i] / (double) speed; // Round off to the next integer, if not the last ride. time += (i == dist.Length - 1 ? t : Math.Ceiling(t)); } return time; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
1
3
-1
C# || How To Find The Minimum ASCII Delete Sum for Two Strings Using C#
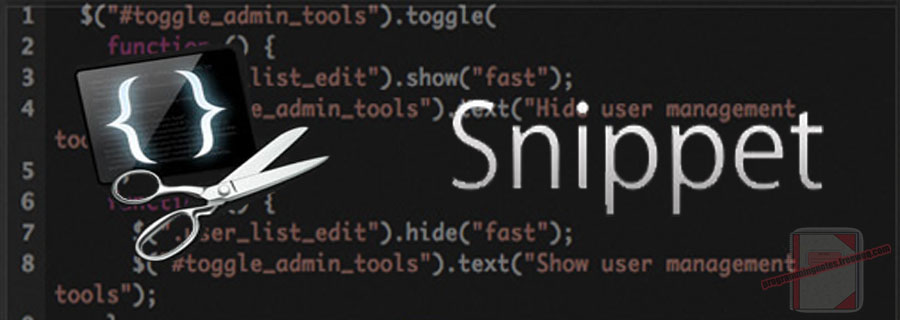
The following is a module with functions which demonstrates how to find the minimum ASCII delete sum for two strings using C#.
1. Minimum Delete Sum – Problem Statement
Given two strings s1 and s2, return the lowest ASCII sum of deleted characters to make two strings equal.
Example 1:
Input: s1 = "sea", s2 = "eat"
Output: 231
Explanation: Deleting "s" from "sea" adds the ASCII value of "s" (115) to the sum.
Deleting "t" from "eat" adds 116 to the sum.
At the end, both strings are equal, and 115 + 116 = 231 is the minimum sum possible to achieve this.
Example 2:
Input: s1 = "delete", s2 = "leet"
Output: 403
Explanation: Deleting "dee" from "delete" to turn the string into "let",
adds 100[d] + 101[e] + 101[e] to the sum.
Deleting "e" from "leet" adds 101[e] to the sum.
At the end, both strings are equal to "let", and the answer is 100+101+101+101 = 403.
If instead we turned both strings into "lee" or "eet", we would get answers of 433 or 417, which are higher.
2. Minimum Delete Sum – Solution
The following is a solution which demonstrates how to find the minimum ASCII delete sum for two strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 28, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find minimum ASCII delete sum two string // ============================================================================ public class Solution { public int MinimumDeleteSum(string s1, string s2) { // Make sure s2 is smaller string if (s1.Length < s2.Length) { return MinimumDeleteSum(s2, s1); } // Case for empty s1 int m = s1.Length, n = s2.Length; int[] currRow = new int[n + 1]; for (int j = 1; j <= n; j++) { currRow[j] = currRow[j - 1] + s2[j - 1]; } // Compute answer row-by-row for (int i = 1; i <= m; i++) { int diag = currRow[0]; currRow[0] += s1[i - 1]; for (int j = 1; j <= n; j++) { int answer; // If characters are the same, the answer is top-left-diagonal value if (s1[i - 1] == s2[j - 1]) { answer = diag; } // Otherwise, the answer is minimum of top and left values with // deleted character's ASCII value else { answer = Math.Min( s1[i - 1] + currRow[j], s2[j - 1] + currRow[j - 1] ); } // Before overwriting currRow[j] with answer, save it in diag // for the next column diag = currRow[j]; currRow[j] = answer; } } // Return the answer return currRow[n]; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
231
403
C# || Fruit Into Baskets – How To Find The Maximum Number Of Fruits Using C#
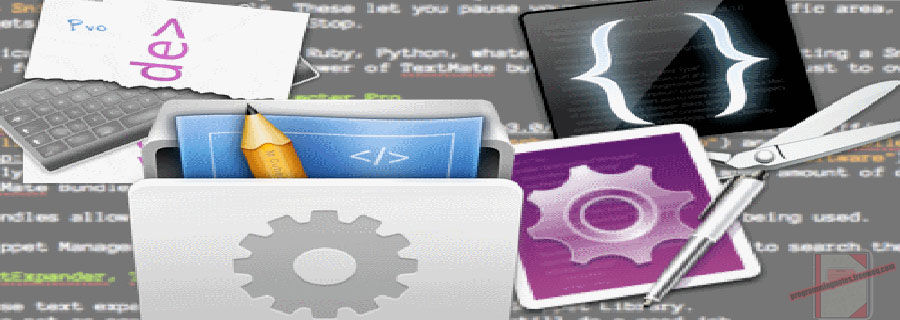
The following is a module with functions which demonstrates how to find the maximum number of fruits using C#.
1. Total Fruit – Problem Statement
You are visiting a farm that has a single row of fruit trees arranged from left to right. The trees are represented by an integer array fruits where fruits[i] is the type of fruit the ith tree produces.
You want to collect as much fruit as possible. However, the owner has some strict rules that you must follow:
- You only have two baskets, and each basket can only hold a single type of fruit. There is no limit on the amount of fruit each basket can hold.
- Starting from any tree of your choice, you must pick exactly one fruit from every tree (including the start tree) while moving to the right. The picked fruits must fit in one of your baskets.
- Once you reach a tree with fruit that cannot fit in your baskets, you must stop.
Given the integer array fruits, return the maximum number of fruits you can pick.
Example 1:
Input: fruits = [1,2,1]
Output: 3
Explanation: We can pick from all 3 trees.
Example 2:
Input: fruits = [0,1,2,2]
Output: 3
Explanation: We can pick from trees [1,2,2].
If we had started at the first tree, we would only pick from trees [0,1].
Example 3:
Input: fruits = [1,2,3,2,2]
Output: 4
Explanation: We can pick from trees [2,3,2,2].
If we had started at the first tree, we would only pick from trees [1,2].
2. Total Fruit – Solution
The following is a solution which demonstrates how to find the maximum number of fruits.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 28, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find the maximum number of fruits // ============================================================================ public class Solution { public int TotalFruit(int[] fruits) { // We use a hash map 'basket' to store the number of each type of fruit. var basket = new Dictionary<int, int>(); int left = 0; int maxPicked = 0; // Add fruit from the right index (right) of the window. for (int right = 0; right < fruits.Length; ++right) { basket[fruits[right]] = basket.GetValueOrDefault(fruits[right], 0) + 1; // If the current window has more than 2 types of fruit, // we remove fruit from the left index (left) of the window, // until the window has only 2 types of fruit. while (basket.Count > 2) { basket[fruits[left]] = basket[fruits[left]] - 1; if (basket[fruits[left]] == 0) { basket.Remove(fruits[left]); } ++left; } // Update maxPicked. maxPicked = Math.Max(maxPicked, right - left + 1); } // Return maxPicked as the maximum number of fruits we can collect. return maxPicked; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
3
3
4