C# || How To Find Minimum Operations To Reduce X To Zero Using C#
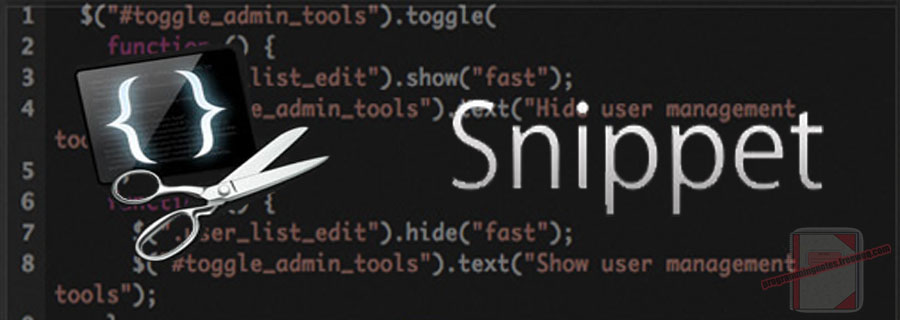
The following is a module with functions which demonstrates how to find the minimum number of operations to reduce X to zero using C#.
1. Min Operations – Problem Statement
You are given an integer array nums and an integer x. In one operation, you can either remove the leftmost or the rightmost element from the array nums and subtract its value from x. Note that this modifies the array for future operations.
Return the minimum number of operations to reduce x to exactly 0 if it is possible, otherwise, return -1.
Example 1:
Input: nums = [1,1,4,2,3], x = 5
Output: 2
Explanation: The optimal solution is to remove the last two elements to reduce x to zero.
Example 2:
Input: nums = [5,6,7,8,9], x = 4
Output: -1
Example 3:
Input: nums = [3,2,20,1,1,3], x = 10
Output: 5
Explanation: The optimal solution is to remove the last three elements and the first two elements (5 operations in total) to reduce x to zero.
2. Min Operations – Solution
The following is a solution which demonstrates how to find the minimum number of operations to reduce X to zero.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 25, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to find the minimum operations reduce X // ============================================================================ public class Solution { public int MinOperations(int[] nums, int x) { var target = -x; foreach (int num in nums) { target += num; } // since all elements are positive, we have to take all of them if (target == 0) { return nums.Length; } var map = new Dictionary<int, int>(); map[0] = -1; var sum = 0; var res = int.MinValue; for (var index = 0; index < nums.Length; ++index) { sum += nums[index]; if (map.ContainsKey(sum - target)) { res = Math.Max(res, index - map[sum - target]); } // no need to check containsKey since sum is unique map[sum] = index; } return res == int.MinValue ? -1 : nums.Length - res; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
2
-1
5
Leave a Reply