Monthly Archives: May 2013
Python || Count The Total Number Of Characters, Vowels, & UPPERCASE Letters Contained In A Sentence Using A ‘For Loop’
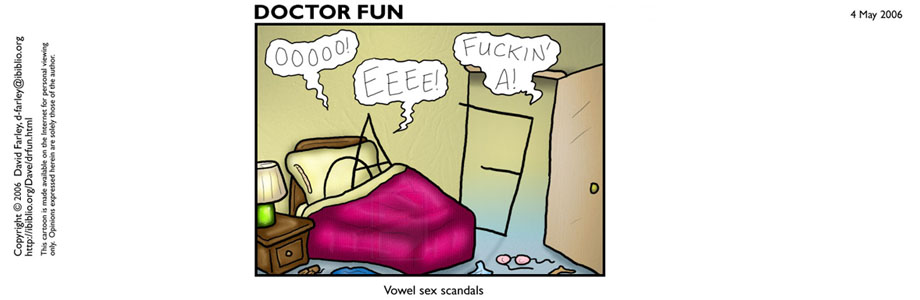
Here is a simple program, which demonstrates more practice using the input/output mechanisms which are available in Python.
This program will prompt the user to enter a sentence, and then display the total number of uppercase letters, vowels and characters contained within that sentence. This program is very similar to an earlier project, this time, utilizing a for loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
def main(): # declare variables numUpperCase = 0 numVowel = 0 numChars = 0 sentence = "" # get data from user sentence = input("Enter a sentence: ") # use a for loop, iterating over each item in the sentence for character in sentence: # do nothing, we dont care about whitespace if(character == ' '): continue # check to see if entered data is UPPERCASE if((character >= 'A') and (character <= 'Z')): numUpperCase += 1 # increment total number of uppercase by one # check to see if entered data is a vowel if((character == 'a')or(character == 'A')or(character == 'e')or (character == 'E')or(character == 'i')or(character == 'I')or (character == 'o')or(character == 'O')or(character == 'u')or (character == 'U')or(character == 'y')or(character == 'Y')): numVowel += 1 # increment Total number of vowels by one numChars += 1 # increment total number of chars being read # display data print("nTotal number of UPPERCASE letters: t%d" %(numUpperCase)) print("Total number of vowels: tt%d" %(numVowel)) print("Total number of characters: tt%d" %(numChars)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
QUICK NOTES:
The highlighted portions are areas of interest.
Notice line 12 contains the for loop declaration. Note that each statement within the for loop block must be uniformly indented, otherwise the code will fail to compile.
Once compiling the above code, you should receive this as your output
Enter a sentence: My Programming Notes Is Awesome.
Total number of UPPERCASE letters: 5
Total number of vowels: 11
Total number of characters: 28
Python || Using If Statements & String Variables
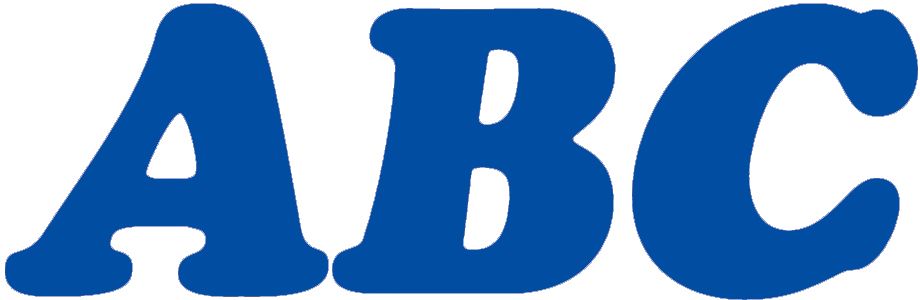
As previously mentioned, you can use “int” and “float” to represent numbers, but what if you want to store letters? Strings help you do that.
==== SINGLE CHAR ====
This example will demonstrate a simple program using strings, which checks to see if the user entered the correctly predefined letter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: char.py # Description: Demonstrates using char variables # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string letter = '' # this holds the individual character # get data from user userInput = input("Please try to guess the letter I am thinking of: ") # we only want to check the first character in the string, # so we get the letter at index zero and save it into its # own variable letter = userInput[0] # use an if statement to check equality. if ((letter == 'a') or (letter == 'A')): print("\nYou have guessed correctly!") else: print("\nSorry, that was not the correct letter I was thinking of..") if __name__ == "__main__": main() # http://programmingnotes.org/ |
Notice in line 11 I declare the string data type, naming it “userInput.” I also initialized it as an empty variable. In line 23 I used an “If/Else Statement” to determine if the user entered value matches the predefined letter within the program. I also used the “OR” operator in line 23 to determine if the letter the user entered into the program was lower or uppercase. Try compiling the program simply using this
if (letter == 'a')
as your if statement, and notice the difference.
The resulting code should give this as output
Please try to guess the letter I am thinking of: A
You have guessed correctly!
==== CHECK IF LETTER IS UPPER CASE ====
This example is similar to the previous one, and will check if a user entered letter is uppercase.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: uppercase.py # Description: Demonstrates checking if a char variable is uppercase # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string letter = '' # this holds the individual character # get data from user userInput = input("Please enter an UPPERCASE letter: ") # get the 1st character in the string letter = userInput[0] # check to see if entered data falls between uppercase values if ((letter >= 'A') and (letter <= 'Z')): print("\n'%c' is an is an uppercase letter!" % (letter)) else: print("\nSorry, '%c' is not an uppercase letter.." % (letter)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
Notice in line 21, an If statement was used, which checks to see if the user entered data falls between letter A and letter Z. We did that by using the “AND” operator. So that IF statement is basically saying (in plain english)
IF ('letter' is equal to or greater than 'A') AND ('letter' is equal to or less than 'Z')
THEN it is an uppercase letter
The resulting code should give this as output
Please enter an UPPERCASE letter: g
Sorry, 'g' is not an uppercase letter..
==== CHECK IF LETTER IS A VOWEL ====
This example will utilize more if statements, checking to see if the user entered letter is a vowel or not. This will be very similar to the previous example, utilizing the OR operator once again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: vowel.py # Description: Demonstrates checking if a char variable is a vowel # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string letter = '' # this holds the individual character # get data from user userInput = input("Please enter a vowel: ") # get the 1st character in the string letter = userInput[0] # check to see if entered data is A,E,I,O,U,Y if ((letter == 'a')or(letter == 'A')or(letter == 'e')or (letter == 'E')or(letter == 'i')or(letter == 'I')or (letter == 'o')or(letter == 'O')or(letter == 'u')or (letter == 'U')or(letter == 'y')or(letter == 'Y')): print("\nCorrect, '%c' is a vowel!" % (letter)) else: print("\nSorry, '%c' is not a vowel.." % (letter)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
This program should be very straight forward, and its basically checking to see if the user entered data is the letter A, E, I, O, U or Y.
The resulting code should give the following output
Please enter a vowel: K
Sorry, 'K' is not a vowel..
==== HELLO WORLD v2 ====
This last example will demonstrate using the string data type to print the line “Hello World!” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: string.py # Description: Demonstrates using string variables # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string # get data from user userInput = input("Please enter a sentence: ") # display string print("\nYou Entered: '%s'" % (userInput)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
The following is similar to the other examples listed on this page, except we display the entire string instead of just simply the first character.
The resulting code should give following output
Please enter a sentence: Hello World!
You Entered: 'Hello World!'
Python || Simple Math Using Int & Float
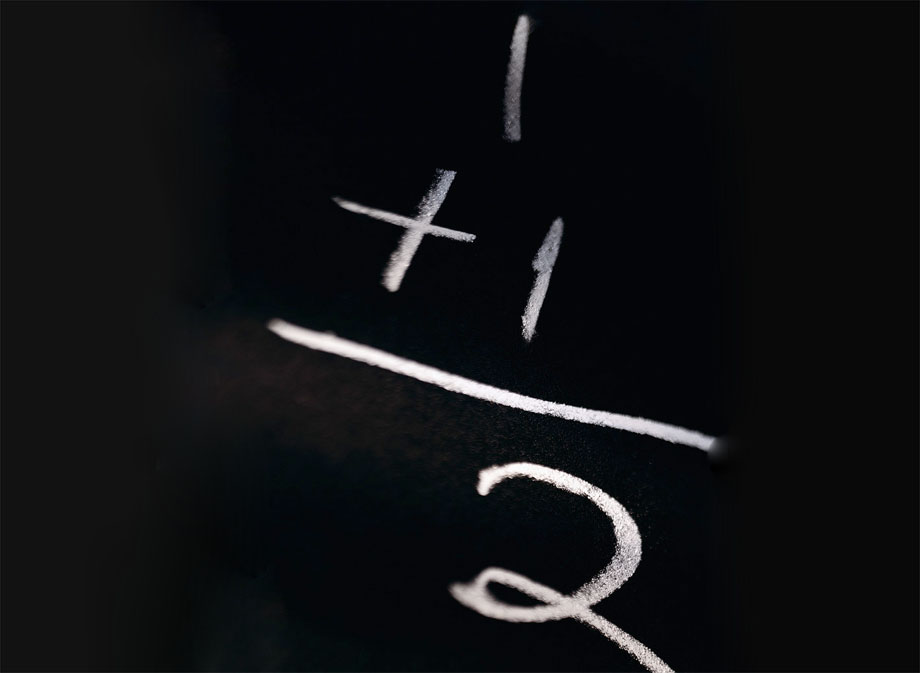
This page will display the use of int and float data types. Since python is a flexible programming language, variable data types do not need to be explicitly declared like in C/C++/Java, but they still exist within the grand scheme of things.
==== ADDING TWO NUMBERS TOGETHER ====
To add two numbers together, you will have to first declare your variables by doing something like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: addition.py # Description: Demonstrates adding numbers together # ============================================================================= def main(): # declare variables # NOTE: data types do not need to be declared num1 = 0; num2 = 0; total = 0; # in Python, input is automatically cast as strings # so we convert everything to integers in order to do math num1 = int(input("Please enter the first number: ")) num2 = int(input("Please enter the second number: ")) # calculate the sum of the two numbers here total = num1 + num2 # display data to the screen. The argument list is # similar to that of the "printf" function in C/C++ print("The sum of %d and %d is: %d" % (num1, num2, total)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
Notice in lines 12-14, I declared my variables, giving them a name. You can name your variables anything you want, with a rule of thumb as naming them something meaningful to your code (i.e avoid giving your variables arbitrary names like “x” or “y”). In line 22 the actual math process is taking place, storing the sum of “num1” and “num2” in a variable called “total.” I also initialized my variables to zero. You should always initialize your variables before you use them.
The above code should give you the following output:
Please enter the first number: 25
Please enter the second number: 1
The sum of 25 and 1 is: 26
==== SUBTRACTING TWO NUMBERS ====
Subtracting two numbers works the same way as the above code, and we would only need to edit the above code in one place to achieve that. In line 22, replace the addition symbol with a subtraction sign, and you should have something like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: subtraction.py # Description: Demonstrates subtracting numbers together # ============================================================================= def main(): # declare variables # NOTE: data types do not need to be declared num1 = 0; num2 = 0; total = 0; # in Python, input is automatically cast as strings # so we convert everything to integers in order to do math num1 = int(input("Please enter the first number: ")) num2 = int(input("Please enter the second number: ")) # calculate the difference of the two numbers here total = num1 - num2 # display data to the screen. print("The difference between %d and %d is: %d" % (num1, num2, total)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
The above code should give you the following output:
Please enter the first number: 25
Please enter the second number: 1
The difference between 25 and 1 is: 24
==== MULTIPLYING TWO NUMBERS ====
This can be achieved the same way as the 2 previous methods, simply by editing line 22, and replacing the designated math operator with the star symbol “*”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: multiplication.py # Description: Demonstrates multiplying numbers together # ============================================================================= def main(): # declare variables # NOTE: data types do not need to be declared num1 = 0; num2 = 0; total = 0; # in Python, input is automatically cast as strings # so we convert everything to integers in order to do math num1 = int(input("Please enter the first number: ")) num2 = int(input("Please enter the second number: ")) # calculate the product of the two numbers here total = num1 * num2 # display data to the screen. print("The product of %d and %d is: %d" % (num1, num2, total)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
The above code should give you the following output:
Please enter the first number: 8
Please enter the second number: 24
The product of 8 and 24 is: 192
==== DIVIDING TWO NUMBERS TOGETHER ====
The resulting code will basically be the same as the other previous three, only instead of our variables being of type int within the print statement, they will be of type float.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: division.py # Description: Demonstrates dividing numbers together # ============================================================================= def main(): # declare variables # NOTE: data types do not need to be declared num1 = 0; num2 = 0; total = 0; # in Python, input is automatically cast as strings # so we convert everything to integers in order to do math num1 = int(input("Please enter the first number: ")) num2 = int(input("Please enter the second number: ")) # calculate the quotient of the two numbers here total = num1 / num2 # display data to the screen. print("The quotient of %d and %d is: %f" % (num1, num2, total)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
The above code should give you the following output:
Please enter the first number: 1
Please enter the second number: 25
The quotient of 1 and 25 is: 0.040000
==== MODULUS ====
If you wanted to capture the remainder of the quotient you calculated from the above code, you would use the modulus operator (%).
From the above code, you would only need to edit line 22, from division, to modulus.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: modulus.py # Description: Demonstrates performing modulus on numbers # ============================================================================= def main(): # declare variables # NOTE: data types do not need to be declared num1 = 0; num2 = 0; total = 0; # in Python, input is automatically cast as strings # so we convert everything to integers in order to do math num1 = int(input("Please enter the first number: ")) num2 = int(input("Please enter the second number: ")) # calculate the mod of the two numbers here total = num1 % num2 # display data to the screen. print("%d mod %d is: %d" % (num1, num2, total)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
The above code should give you the following output:
Please enter the first number: 1
Please enter the second number: 25
1 mod 25 is: 1
Python || Hello World!
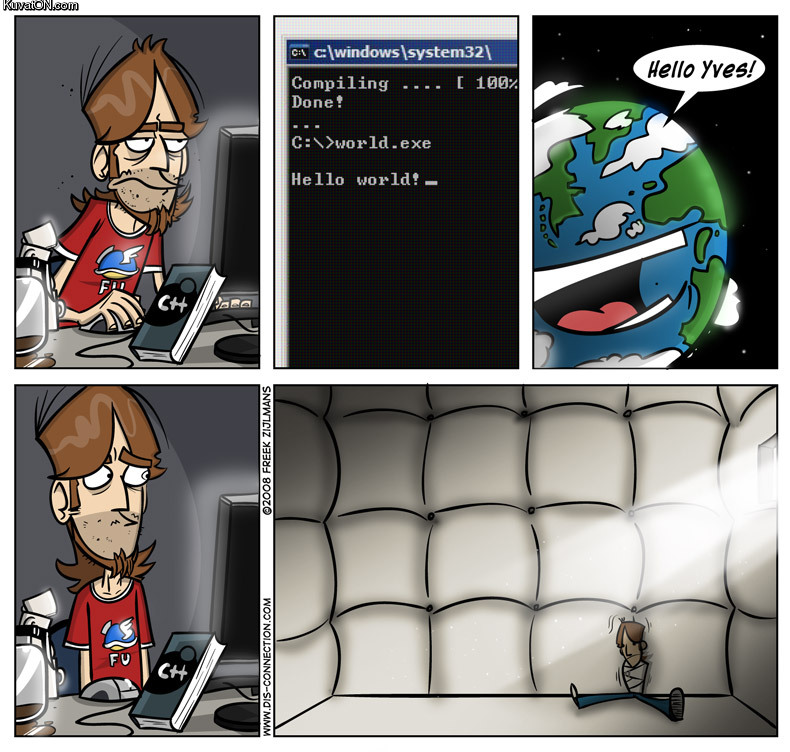
This page will consist of creating the typical “hello world!” application. First, open a text editor and save the file as “HelloWorld.py“. The “.py” is the file extension for python applications.
When you have everything loaded up, you will start your code off by declaring a “main” function like so. This should be placed at the bottom of your source code.
1 2 |
if __name__ == "__main__": main() |
In simple terms, line #1 from above tells the python interpreter to check to see if the current source code thats being executed is the main module; and if it is, line #2 tells the python interpreter to look for the function within the source code whose name is “main.”
NOTE: Main functions are not needed in python, but it is worth noting that without the main sentinel, the entire source code will be executed even if the script was imported as a module.
Next, we will define the main function. This should be placed at the top of your source code.
1 2 |
def main(): # YOUR CODE GOES HERE |
Unlike most programming languages, brackets “{}” are not needed when defining a function, but statements following each function block must be uniformly indented throughout the code. Also, an important fact to remember is, functions must be declared before they are used.
We are halfway finished, now all we have to do is say hello to the world! You will do that by adding this line of code to your program, which basically outputs data to the screen
1 2 |
def main(): print("Hello World!") |
So when you add everything together, your full code will look something like this
1 2 3 4 5 6 |
# This is the main function def main(): print("Hello World!") if __name__ == "__main__": main() |
To execute the above code, simply hit the “run” button in whichever IDE you are using. If you are using a unix system along with a text editor, enter the command below in the terminal.
python3 HelloWorld.py
And there you have it. After executing the above code, you should get this as your output
Hello World!
Python || Which Interpreter To Use?
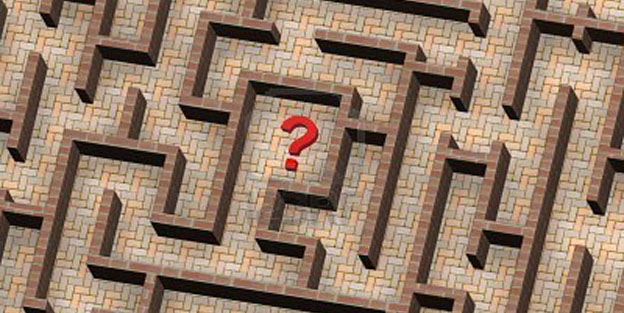
Unlike compiled programming languages such as C, C++ or Java, Python is an interpreted language. Since this is the case, the type of interpreter you use will probably be the same no matter which programming environment you select. You can download the official Python interpreter here. Python version 3 will be featured throughout this site.
If you are using Windows, download the file named “Python 3.X.X Windows x86 MSI Installer“. If you are using Ubuntu, type the following command into the terminal:
sudo apt-get install python3
In terms of which IDE to use, there are a few choices, and your choice should probably be based on what you intend to write with it.
If you intend to program on Windows, the path of least resistance is to try the IDLE which comes bundled with the Windows installation of Python. This provides you with a straightforward IDE with (obviously) good integration with the Windows platform. This IDE is free, but is very basic and is almost like a simple text editor. So if you wish to go this route, there are other alternatives which are more beneficial and practical for use, such as Notepad++ or Gedit.
As far as IDE’s go, Eclipse grouped with PyDev is a popular alternative, as is PyScripter. Both of these will also give you a visual debugger and a build environment.
Ultimately, my opinion is, if you’re just starting out, go with Notepad++ or Gedit. Then when you’re more comfortable with what you’re doing – explore the alternatives.
Personally, I use PyScripter, and all the code that is posted on this site was created using that IDE