Monthly Archives: March 2013
C++ || Snippet – How To Display The Current Working Directory
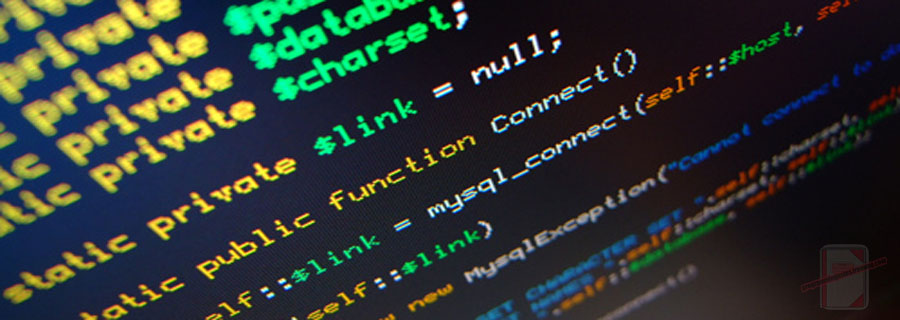
The following is sample code which demonstrates how to display the current working directory of the program when its properties were initialized.
If you are a system admin, this has a similar effect to the “echo %CD%
” Windows Shell command or the “pwd
” Unix/Linux terminal command, which essentially displays the current working directory the program is located at.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// ============================================================================ // Author: K Perkins // Date: Mar 12, 2013 // Taken From: http://programmingnotes.org/ // File: pwd.cpp // Description: Demonstrates how to display the current working directory // the program is located at to the screen. // ============================================================================ #include <iostream> using namespace std; // determine which platform #ifdef _WIN32 #include <direct.h> #define GetCurrentDir _getcwd #else #include <unistd.h> #define GetCurrentDir getcwd #endif int main() { // declare variables char filePath[256]; // get current directory if(!GetCurrentDir(filePath, sizeof(filePath))) { cout<<"** ERROR - Something went wrong, exiting...n"; } else { cout<<"This file is located in directory:nn"<<filePath<<endl; } cout<<"nPlease press ENTER to continue..."; cin.get(); return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: This was compiled using a Windows computer
This file is located in directory:
C:UsersAdminDesktop
Please press ENTER to continue...
C++ || Snippet – How To Convert A Decimal Number Into Binary
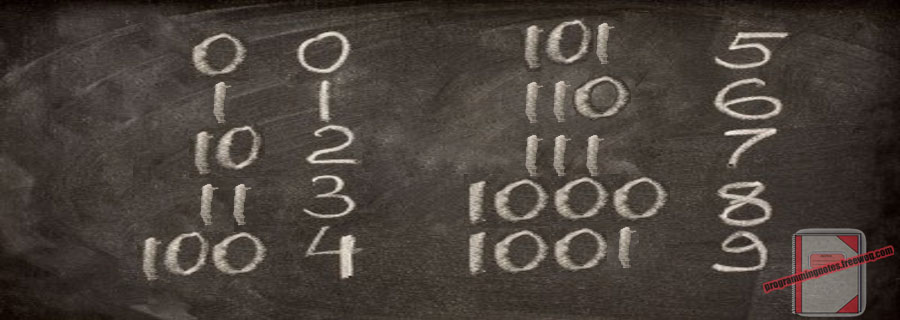
This page will demonstrate how to convert a decimal number (i.e a whole number) into its binary equivalent. So for example, if the decimal number of 26 was entered into the program, it would display the converted binary value of 11010.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
How To Count In Binary
The "Long" Datatype - What Is It?
While Loops
Online Binary to Decimal Converter - Verify For Correct Results
How To Reverse A String
If you are looking for sample code which converts binary to decimal, check back here soon!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
#include <iostream> #include <string> #include <algorithm> using namespace std; // function prototype string DecToBin(long long decNum); int main() { // declare variables long long decNum = 0; string binaryNum=""; // use a string instead of an int to avoid // overflow, because binary numbers can grow large quick cout<<"Please enter an integer value: "; cin >> decNum; if(decNum < 0) { binaryNum = "-"; } // call function to convert decimal to binary binaryNum += DecToBin(decNum); // display data to user cout<<"nThe integer value of "<<decNum<<" = "<<binaryNum<<" in binary"<<endl; return 0; } string DecToBin(long long decNum) { string binary = ""; // use this string to save the binary number if(decNum < 0) // if input is a neg number, make it positive { decNum *= -1; } // converts decimal to binary using division and modulus while(decNum > 0) { binary += (decNum % 2)+'0'; // convert int to char decNum /= 2; } // reverse the string reverse(binary.begin(), binary.end()); return binary; }// http://programmingnotes.org/ |
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: The code was compiled 3 separate times to display different output
====== RUN 1 ======
Please enter an integer value: 1987
The integer value of 1987 = 11111000011 in binary
====== RUN 2 ======
Please enter an integer value: -26
The integer value of -26 = -11010 in binary
====== RUN 3 ======
Please enter an integer value: 12345678910
The integer value of 12345678910 = 1011011111110111000001110000111110 in binary