Monthly Archives: June 2012
Java || Find The Prime, Perfect & All Known Divisors Of A Number Using A For, While & Do/While Loop
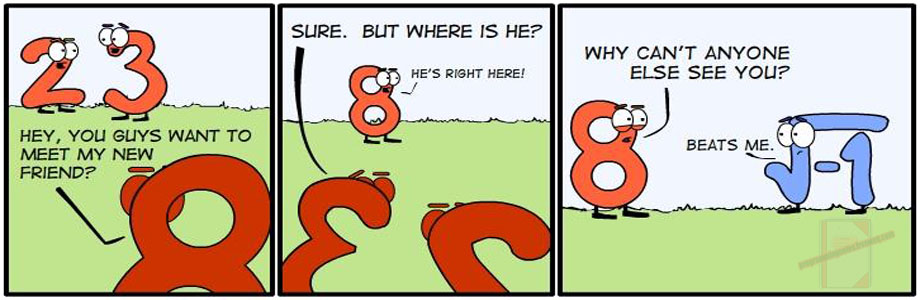
This program was designed to better understand how to use different loops which are available in Java, as well as more practice using objects with classes.
This program first asks the user to enter a non negative number. After it obtains a non negative integer from the user, the program determines if the user obtained number is a prime number or not, aswell as determining if the user obtained number is a perfect number or not. After it obtains its results, the program will display to the screen if the user inputted number is prime/perfect number or not. The program will also display a list of all the possible divisors of the user obtained number via stdout.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Class Objects - How TO Use
Constructors - What Are They?
Do/While Loop
While Loop
For Loop
Modulus
Basic Math - Prime Numbers
Basic Math - Perfect Numbers
Basic Math - Divisors
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 |
import java.util.Scanner; public class PrimePerfectNums { // global variable declaration int userInput = 0; static Scanner cin = new Scanner(System.in); public PrimePerfectNums(int input) {// this is the constructor // give the global variable a value userInput = input; }// end of PrimePerfectNums public void CalcPrimePerfect() { // declare variables int divisor = 0; int sumOfDivisors = 0; System.out.print("nInput number: " + userInput); // for loop adds sum of all possible divisors for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0) { // this will repeatedly add the found divisors together sumOfDivisors += counter; } } System.out.println(""); // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is prime if(userInput == (sumOfDivisors - 1)) { System.out.print(userInput + " is a prime number."); } else { System.out.print(userInput + " is not a prime number."); } System.out.println(""); // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is a perfect number if (userInput == (sumOfDivisors - userInput)) { System.out.print(userInput + " is a perfect number."); } else { System.out.print(userInput + " is not a perfect number."); } System.out.print("nDivisors of " + userInput + " are: "); // for loop lists all the possible divisors for the // 'userInput' variable by using the modulus operator for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0 && counter !=userInput) { System.out.print(counter + ", "); } } System.out.print("and " + userInput); }// end of CalcPrimePerfect public static void main(String[] args) { // declare variables int input = 0; char response ='n'; do{ // this is the start of the do/while loop System.out.print("Enter a number: "); input = cin.nextInt(); // if the user inputs a negative number, do this code while(input < 0) { System.out.print("ntSorry, but the number entered is less " + "than the allowable limit.ntPlease try again....."); System.out.print("nnEnter an number: "); input = cin.nextInt(); } // entry point to class declaration PrimePerfectNums myClass = new PrimePerfectNums(input); // function call to obtain data using the number // which was passed from main to the constructor myClass.CalcPrimePerfect(); // asks user if they want to enter new data System.out.print("nntDo you want to input another number?(Y/N): "); response = cin.next().toLowerCase().charAt(0); System.out.println("---------------------------" + "---------------------------------"); }while(response =='y'); // ^ End of the do/while loop. As long as the user chooses // 'Y' the loop will keep going. // It stops when the user chooses the letter 'N' System.out.println("BYE!"); }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Enter a number: 41
Input number: 41
41 is a prime number.
41 is not a perfect number.
Divisors of 41 are: 1, and 41Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 496Input number: 496
496 is not a prime number.
496 is a perfect number.
Divisors of 496 are: 1, 2, 4, 8, 16, 31, 62, 124, 248, and 496Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 1858Input number: 1858
1858 is not a prime number.
1858 is not a perfect number.
Divisors of 1858 are: 1, 2, 929, and 1858Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: -9Sorry, but the number entered is less than the allowable limit.
Please try again.....Enter an number: 12
Input number: 12
12 is not a prime number.
12 is not a perfect number.
Divisors of 12 are: 1, 2, 3, 4, 6, and 12Do you want to input another number?(Y/N): n
------------------------------------------------------------
BYE!
Java || Find The Average Using an Array – Omit Highest And Lowest Scores
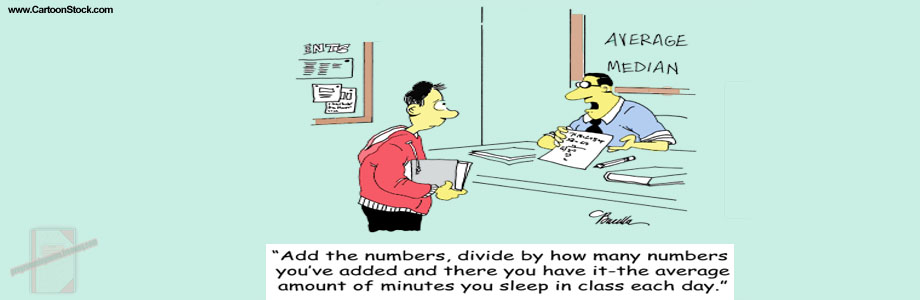
This page will consist of two programs which calculates the average of a specific amount of numbers using an array.
REQUIRED KNOWLEDGE FOR BOTH PROGRAMS
Double Data Type
Final Variables
Arrays
For Loops
Assignment Operators
Basic Math - How To Find The Average
====== FIND THE AVERAGE USING AN ARRAY ======
The first program is fairly simple, and it was used to introduce the array concept. The program prompts the user to enter the total amount of numbers they want to find the average for, then the program displays the answer to them via stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
import java.util.Scanner; public class FindTheAverage { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables int numElems = 0; double sum = 0; double[] average = new double[100]; // declare array which has the ability to hold 100 elements // display message to screen System.out.println("Welcome to My Programming Notes' Java Program.n"); // determine how many numbers the user wants in the array System.out.print("How many numbers do you want to find the average for?: "); numElems = cin.nextInt(); System.out.println(""); // user enters data into array using a for loop // you can also use a while loop, but for loops are more common // when dealing with arrays for(int index=0; index < numElems; ++index) { System.out.print("Enter value #" +(index+1)+ ": "); average[index] = cin.nextDouble(); sum += average[index]; } // find the average. // Note: the expression below literally // means: sum = sum / numElems; sum /= numElems; System.out.println("nThe average of the " + numElems + " numbers is " + sum); }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
ARRAY
Notice the array declaration on line #13. The type of array being used in this program is a dynamic array, which has the ability to store up to 100 integer elements in the array. You can change the number of elements its able to store to a higher or lower number if you wish.
FOR LOOP
Lines 27-32 contains a for loop, which is used to actually store the data inside of the array. Without some type of loop, it is virtually impossible for the user to input data into the array; that is, unless you want to add 100 different println statements into your code asking the user to input data. Line 31 uses the assignment operator “+=” which gives us a running total of the data that is being inputted into the array. Note the loop only stores as many elements as the user so desires, so if the user only wants to input 3 numbers into the array, the for loop will only execute 3 times.
Once compiled, you should get this as your output:
Welcome to My Programming Notes' Java Program.
How many numbers do you want to find the average for?: 4
Enter value #1: 21
Enter value #2: 24
Enter value #3: 19
Enter value #4: 17
The average of the 4 numbers is 20.25
====== FIND THE AVERAGE – OMIT HIGHEST AND LOWEST SCORES ======
The second program is really practical in a real world setting. We were asked to create a program for a fictional competition which had 6 judges. The 6 judges each gave a score of the performance for a competitor in a competition, (i.e a score of 1-10), and we were asked to find the average of those scores, omitting the highest/lowest results. The program was to store the scores into an array, display the scores back to the user via stdout, display the highest and lowest scores among the 6 obtained, display the average of the 6 scores, and finally display the average adjusted scores omitting the highest and lowest result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
import java.util.Scanner; public class FindOmittedAverage { // global variable declaration static Scanner cin = new Scanner(System.in); static final int NUM_JUDGES = 6; public static void main(String[] args) { // declare variables double highestScore = -999999; double lowestScore = 999999; double sumOfScores = 0; double avgScores = 0; double[] scores = new double[NUM_JUDGES]; // array is initialized using a final variable // display message to screen System.out.println("Welcome to My Programming Notes' Java Program.n"); System.out.print("Judges, enter one score each for the current competitor: "); // use a for loop to obtain data from user using the final variable for(int index=0; index < NUM_JUDGES; ++index) { // this puts data into the current array index scores[index] = cin.nextDouble(); // this calculates a running total of all the scores // adding each element in the array together sumOfScores += scores[index]; // if current score in the array index is bigger than the current 'highestScore' // value, then set 'highestScore' equal to the current value in the array if(scores[index] > highestScore) { highestScore = scores[index]; } // if current score in the array index is smaller than the current 'lowestScore' // value, then set 'lowestScore' equal to the current value in the array if(lowestScore > scores[index]) { lowestScore = scores[index]; } } System.out.print("nThese are the scores from the " + NUM_JUDGES + " judges: "); // use another for loop to redisplay the data back to the user via stdout for(int index=0; index < NUM_JUDGES; ++index) { System.out.print("nThe score for judge #"+(index+1)+" is: "+scores[index]); } // display the highest/lowest numbers to the screen System.out.print("nnThese are the highest and lowest scores: "); System.out.print("ntHighest: "+ highestScore); System.out.print("ntLowest: "+ lowestScore); // find the average avgScores = sumOfScores/NUM_JUDGES; System.out.print("nThe average score is: "+ avgScores); // reset data back to 0 so we can find the ommitted average sumOfScores = 0; avgScores = 0; System.out.print("nThe average adjusted score omitting the highest and lowest result is: "); // final loop, which calculates a running total, adding each element // in the array together, this time omitting the highest/lowest scores for(int index=0; index < NUM_JUDGES; ++index) { // IF(current score isnt equal to the highest elem) AND (current score isnt equal lowest elem) // THEN create a running total if((scores[index] != highestScore) && (scores[index] != lowestScore)) { sumOfScores += scores[index]; } } // find the average, minus the 2 scores avgScores = sumOfScores/(NUM_JUDGES-2); System.out.print(avgScores); }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
FINAL
A final variable was declared and used to initialize the array (line 7). This was used to initialize the size of the array.
FOR LOOPS
Once again loops were used to traverse the array, as noted on lines 24, 51 and 73. The final variable was also used within the for loops, making it easier to modify the code if its necessary to reduce or increase the number of available judges.
HIGHEST/LOWEST SCORES
This is noted on lines 35-45, and it is really simple to understand the process once you see the code.
OMITTING HIGHEST/LOWEST SCORE
Lines 73-81 highlights this process. The loop basically traverses the array, skipping over the highest/lowest elements.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Judges, enter one score each for
the current competitor: 123 453 -789 2 23345 987These are the scores from the 6 judges:
The score for judge #1 is: 123.0
The score for judge #2 is: 453.0
The score for judge #3 is: -789.0
The score for judge #4 is: 2.0
The score for judge #5 is: 23345.0
The score for judge #6 is: 987.0These are the highest and lowest scores:
Highest: 23345.0
Lowest: -789.0
The average score is: 4020.1666666666665
The average adjusted score omitting the highest and lowest result is: 391.25
Java || Display Today’s Date Using a Switch Statement
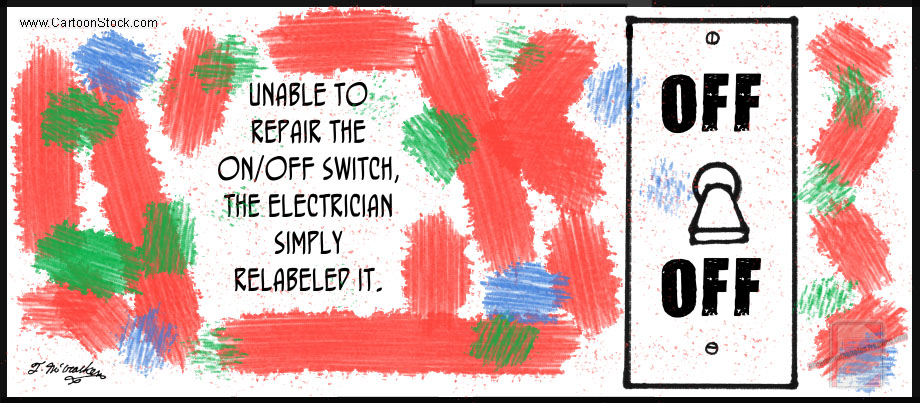
If statements, char’s and strings have been previously discussed, and this page will be more of the same. This program will demonstrate how to use a switch statement to display today’s date, converting from mm/dd/yyyy format (i.e 6/17/12) to formal format (i.e June 17th, 2012).
This same program can easily be done using if statements, but sometimes that is not always the fastest programming method. Switch statements are like literal light switches because the code “goes down a line” to check to see which case is valid or not, just like if statements. You will see why switches are very effective when used right by examining this program.
====== TODAY’S DATE USING A SWITCH ======
So to start our program out, lets define the variables.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import java.util.Scanner; public class TodaysDate { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables int month = 6; int day = 17; int year = 2012; }// end of main }// http://programmingnotes.org/ |
Notice on line 6 there is a variable named “cin.” This program uses the scanner class to obtain data from the user. Click here for various examples demonstrating how to obtain data from the user using the scanner class.
We also declared three other variables, named “month, day, and year.” You should always name your variables something descriptive, as well as initializing them to a starting value.
Next we get data from the user for the month, day, and year variables. This process is demonstrated below:
1 2 3 4 5 6 7 8 9 10 11 |
// get month from user System.out.print("Please enter the current month: "); month = cin.nextInt(); // get day from user System.out.print("Please enter the current day: "); day = cin.nextInt(); // get year from user System.out.print("Please enter the current year: "); year = cin.nextInt(); |
Notice the format that the user will input the data in. They will input data in mm/dd/yyyy format, and using the “cin” variable will make that possible.
So after obtaining data from the user, how will the program convert numbers into actual text? Next comes the switch statements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// determine which month to display switch(month) { case 1: System.out.print("tJanuary "); break; case 2: System.out.print("tFebruary "); break; case 3: System.out.print("tMarch "); break; case 4: System.out.print("tApril "); break; case 5: System.out.print("tMay "); break; case 6: System.out.print("tJune "); break; case 7: System.out.print("tJuly "); break; case 8: System.out.print("tAugust "); break; case 9: System.out.print("tSeptember "); break; case 10: System.out.print("tOctober "); break; case 11: System.out.print("tNovember "); break; case 12: System.out.print("tDecember "); break; default: System.out.print("t" + month +" is not a valid monthn"); System.exit(1); break; }// http://programmingnotes.org/ |
Line 2 contains the switch declaration, and its comparing the variable of “month” to the 12 different cases that is defined within the switch statement. So this piece of code will “go down the line” comparing to see if the user obtained data is any of the numbers from 1 to 12, as defined in the switch statement. If the user chooses a number which does not fall between 1 thru 12, the “default” case will be executed, prompting the user that the data they entered was invalid, which can be seen in line 40. Notice line 42 has an exit code. This program will force an exit whenever the user enters invalid data.
Line 6 is also very important, because that forces the computer to “break” away from the selected case whenever it is done examining that specific piece of code. It is important to add the break statement in there to avoid errors, which may result if the program does not break away from the current statement in which it is examining. Try compiling this code removing the “break” statements and see what happens!
Next we will add another switch statement in order to convert the day of the month to have a number suffix (i.e displaying the number in 1st, 2nd, 3rd format). This is very similar to the previous switch statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
// determine which suffix to display switch(day) { case 1: case 21: case 31: System.out.print(day + "st, "); break; case 2: case 22: System.out.print(day + "nd, "); break; case 3: case 23: System.out.print(day + "rd, "); break; default: System.out.print(day + "th, "); break; } |
This block of code is very similar to the previous one. Line 2 is declaring the variable ‘day’ to be compared with the base cases; line 6 and so forth has the break lines, but line 4, 7 and 10 are different. If you notice, line 4, 7 and 10 are comparing multiple cases in one line. Yes, with switch statements, you can do that. Just like you can compare multiple values in if statements, the same can be done here. So this switch is comparing the number the user entered into the program, with the base cases, adding a suffix to the end of the number.
So far we have obtained data from the user, compared the month and day using switch statements and displayed that to the screen. Now all we have to do is output the year to the user. This is fairly simple, because the year is not being compared, you are just simply using a system print to display data to the user.
1 2 |
// display the current year System.out.println(year); |
So finally, adding all the above snippets together should give us the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 |
import java.util.Scanner; public class TodaysDate { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables int month = 6; int day = 17; int year = 2012; // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); // get month from user System.out.print("nPlease enter the current month: "); month = cin.nextInt(); // get day from user System.out.print("Please enter the current day: "); day = cin.nextInt(); // get year from user System.out.print("Please enter the current year: "); year = cin.nextInt(); System.out.println("nTodays date is: "); // use this switch statement to display the current month // using the information obtained from above switch(month) { case 1: System.out.print("tJanuary "); break; case 2: System.out.print("tFebruary "); break; case 3: System.out.print("tMarch "); break; case 4: System.out.print("tApril "); break; case 5: System.out.print("tMay "); break; case 6: System.out.print("tJune "); break; case 7: System.out.print("tJuly "); break; case 8: System.out.print("tAugust "); break; case 9: System.out.print("tSeptember "); break; case 10: System.out.print("tOctober "); break; case 11: System.out.print("tNovember "); break; case 12: System.out.print("tDecember "); break; default: System.out.print("t" + month +" is not a valid monthn"); System.exit(1); break; } // use this switch statement to display the current day // using the information obtained from above switch(day) { case 1: case 21: case 31: System.out.print(day + "st, "); break; case 2: case 22: System.out.print(day + "nd, "); break; case 3: case 23: System.out.print(day + "rd, "); break; default: System.out.print(day + "th, "); break; } // display the current year System.out.println(year); }// end of main }// http://programmingnotes.org/ |
Once compiled, you should get this as your output:
Welcome to My Programming Notes' Java Program.
Please enter the current month: 7
Please enter the current day: 28
Please enter the current year: 2012Todays date is:
July 28th, 2012
Java || Snippet – Custom Setw & Setfill Sample Code For Java
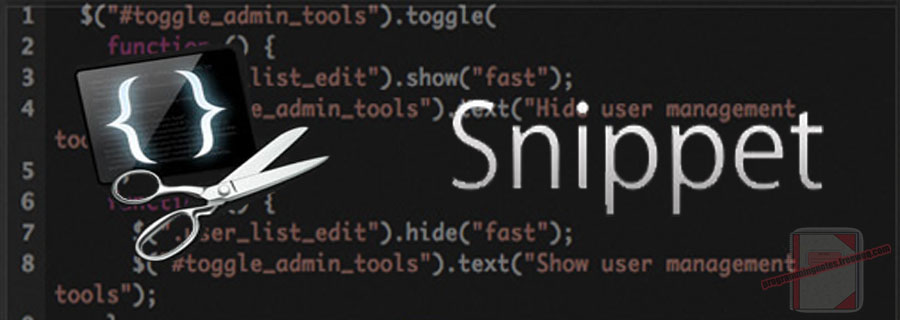
This page will consist of a brief implementation of setw/ios::width and setfill/ios::fill in Java.
If you are a C++ programmer, no doubt you have used the setw and setfill commands many times. It makes formatting output like this very clean and simple (see below).
Ending balance:................. $ 6433.47
Amount of deposits:............. $ 1750.00
Amount of withdrawals:.......... $ 420.00
Amount of interest earned:...... $ 103.47
But to my amazement, I could not find a very suitable replacement for the setw/setfill functions in Java. Using simple for loops, the methods provided on this page has the ability to mimic both functions which are available in C++.
Included in the sample code are the following:
== SETW ==
(1) right - Justifies string data of size "width," filling the width to the start of the string with whitespace
(2) left - Justifies string data of size "width," filling the width to the end of the string with whitespace
== SETFILL ==
(3) right - Justifies string data of size "width," filling the width to the start of the string with a filler character
(4) left - Justifies string data of size "width," filling the width to the end of the string with a filler character
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jun 6, 2012 // Taken From: http://programmingnotes.org/ // File: Setw.java // Description: Demonstrate the use of custom setw/ios::width and // setfill/ios::fill functions for text formatting in Java. // ============================================================================ public class Setw { // ==== right ====================================================================== // // - Set field width right align - // // Justifies string data of size "width," filling the width to the start of // the string with whitespace (' '). // // - USAGE - // right(<String> the text you wish to format, <int> size of width to be formated); // // ================================================================================= public static void right(String str, int width) { right(str, width, ' '); }// end of right // ==== left ====================================================================== // // - Set field width left align - // // Justifies string data of size "width," filling the width to the end of // the string with whitespace (' '). // // - USAGE - // left(<String> the text you wish to format, <int> size of width to be formated); // // ================================================================================= public static void left(String str, int width) { left(str, width, ' '); }// end of left // ==== right ====================================================================== // // - Set field width right align fill - // // Justifies string data of size "width," filling the width to the start of // the string with a filler character. // // Use this method (instead of using 'left/right') when you want so specify the // type of filler you want to use // // - USAGE - // right(<String> the text you wish to format, // <int> size of width to be formated, <char> the type of filler to be displayed); // // ================================================================================= public static void right(String str, int width, char fill) { for (int x = str.length(); x < width; ++x) { System.out.print(fill); } System.out.print(str); }// end of right // ==== left ====================================================================== // // - Set field width left align fill - // // Justifies string data of size "width," filling the width to the end of // the string with a filler character. // // Use this method (instead of using 'left/right') when you want so specify the // type of filler you want to use // // - USAGE - // left(<String> the text you wish to format, // <int> size of width to be formated, <char> the type of filler to be displayed); // // ================================================================================= public static void left(String str, int width, char fill) { System.out.print(str); for (int x = str.length(); x < width; ++x) { System.out.print(fill); } }// end of left }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Also, you must understand minimal object oriented programming to use this code!
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
===== DEMONSTRATION HOW TO USE =====
Use of the above snippet is similar to its C++ counterpart. Here is a sample program demonstrating its use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jun 6, 2012 // Taken From: http://programmingnotes.org/ // File: Main.java // Description: Demonstrate the use of the Setw class // ============================================================================ public class Main { public static void main(String[] args) { // this formats text, printng whitespace to the right of the string System.out.print("1)"); Setw.right("This is text right aligned", 45); System.out.println(""); // this formats text, printng whitespace to the left of the string System.out.print("2)"); Setw.left("This is text left aligned", 45); System.out.print("!"); System.out.println(""); // this formats text, but this time, instead of printng whitespace // to the left of the string, it prints a "filler," which can be anything // you want. Currently, the filler is a exclaimation ('!') System.out.print("3)"); Setw.right("This is text right aligned with filler", 45, '!'); System.out.println(""); // this formats text, but this time, instead of printng whitespace // to the right of the string, it prints a "filler," which can be anything // you want. Currently, the filler is a dollar sign ('$') System.out.print("4)"); Setw.left("This is text left aligned with filler", 45, '$'); System.out.println(""); // you can also send numbers to any of the functions, // provided you first convert them to strings System.out.print("5)"); Setw.left("This is a string with a number " + Integer.toString(1987), 45, '.'); System.out.println("\n"); // you can also use this method to print the famous "triangle" shapes System.out.println("6) This is a triangle printed using the right method"); for(int width = 0; width <= 10; ++width) { Setw.right("", width, '*'); System.out.println(""); } System.out.println(""); // this prints an upside down triangle System.out.println("7) This is an upside down triangle printed " + "using the left method\n"); for(int width = 10; width > 0; --width) { Setw.left("", width, '*'); System.out.println(""); } System.out.println(""); // this is the example receipt System.out.println("8) This is the example receipt\n"); Setw.left("Ending balance:", 32, '.'); System.out.println(" $ " + Double.toString(6433.47)); Setw.left("Amount of deposits:", 32, '.'); System.out.println(" $ " + Double.toString(1750.00)); Setw.left("Amount of withdrawals:", 32, '.'); System.out.println(" $ " + Double.toString(420.00)); Setw.left("Amount of interest earned:", 32, '.'); System.out.println(" $ " + Double.toString(103.47)); }// end of main }// http://programmingnotes.org/ |
Once compiled, you should get this as your output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
1) This is text right aligned 2)This is text left aligned ! 3)!!!!!!!This is text right aligned with filler 4)This is text left aligned with filler$$$$$$$$ 5)This is a string with a number 1987.......... 6) This is a triangle printed using the right method * ** *** **** ***** ****** ******* ******** ********* ********** 7) This is an upside down triangle printed using the left method ********** ********* ******** ******* ****** ***** **** *** ** * 8) This is the example receipt Ending balance:................. $ 6433.47 Amount of deposits:............. $ 1750.0 Amount of withdrawals:.......... $ 420.0 Amount of interest earned:...... $ 103.47 |
Java || Whats My Name? – Practice Using Strings, Methods & Switch Statemens
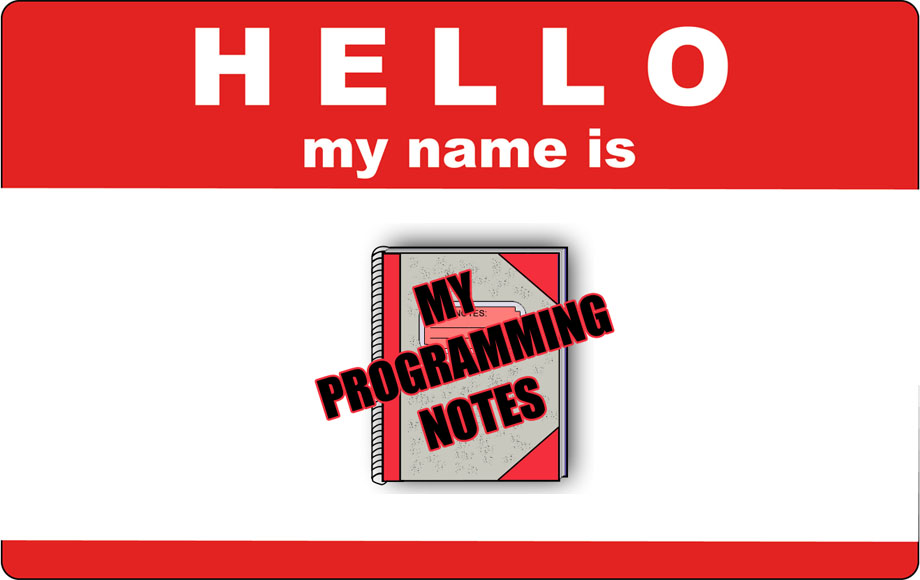
Here is another actual homework assignment which was presented in an intro to programming class. This program highlights more use using strings, modules, and switch statements.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Methods (A.K.A "Functions") - What Are They?
Switch Statements - How To Use Them
Equal - String Comparison
This program first prompts the user to enter their name. Upon receiving that information, the program saves input into a string called “firstName.” The program then asks if the user has a middle name. If they do, the user will enter a middle name. If they dont, the program proceeds to ask for a last name. Upon receiving the first, [middle], and last names, the program will append the first, [middle], and last names into a completely new string titled “fullName.” Lastly, if the users’ first, [middle], or last names are the same, the program will display that data to the screen via stdout. The program will also display to the user the number of characters their full name contains using the built in function “length.”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 |
import java.util.Scanner; public class NameString { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char response = ' '; String firstName= ""; String fullName=""; // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); // get first name from user System.out.print("Please enter your first name: "); firstName = cin.next(); System.out.print("nDo you have a middle name?(Y/N): "); String choice = cin.next(); choice = choice.toUpperCase(); response = choice.charAt(0); // use a switch statement to detrmine which function will be called switch(response) { case 'Y': fullName = FirstMiddleLast(firstName); // method declaration break; case 'N': fullName = FirstLast(firstName); // method declaration break; default: System.out.print("nPlease press either 'Y' or 'N'n" + "Program exiting...n"); System.exit(1); break; } System.out.println("And your full name is "+fullName); }// end of main // ==== FirstMiddleLast ==================================================== // // This module will take as input the first name, and prompt the user for // their middle and last name. Then it will display the total number of // characters in their name. It returns the full name back to main // // ========================================================================= private static String FirstMiddleLast(String firstName) { String middleName=""; String lastName=""; String fullName=""; System.out.print("nPlease enter your middle name: "); middleName = cin.next(); System.out.print("Please enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+middleName+" "+lastName; System.out.println(""); // check to see if the first, middle or last names are the same // if they are, display a message to the user if(firstName.equals(middleName)) { System.out.print("tYour first and middle name are the samen"); } if(middleName.equals(lastName)) { System.out.print("tYour middle and last name are the samen"); } if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the 2 white spaces System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-2)); // return the full name string to main return fullName; }// end of FirstMiddleLast // ==== FirstLast ========================================================== // // This module will take as input the first name, and prompt the user for // their last name. Then it will display the total number of characters in // their name. It returns the full name back to main // // ============================================================================ private static String FirstLast(String firstName) { String lastName=""; String fullName=""; System.out.print("nPlease enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+lastName; System.out.println(""); // check to see if the first or last names are the same // if they are, display a message to the user if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the white space System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-1)); // return the full name string to main return fullName; }// end of FirstLast }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: The code was compiled five separate times to display the different outputs its able to produce
====== RUN 1 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): y
Please enter your middle name: Programming
Please enter your last name: NotesThe total number of characters in your name is: 18
And your full name is My Programming Notes====== RUN 2 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Programming
Do you have a middle name?(Y/N): n
Please enter your last name: NotesThe total number of characters in your name is: 16
And your full name is Programming Notes====== RUN 3 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Notes
Do you have a middle name?(Y/N): y
Please enter your middle name: Notes
Please enter your last name: NotesYour first and middle name are the same
Your middle and last name are the same
Your first and last name are the sameThe total number of characters in your name is: 15
And your full name is Notes Notes Notes====== RUN 4 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): n
Please enter your last name: MyYour first and last name are the same
The total number of characters in your name is: 4
And your full name is My My====== RUN 5 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): zPlease press either 'Y' or 'N'
Program exiting...
Java || Count The Total Number Of Characters, Vowels, & UPPERCASE Letters Contained In A Sentence Using A ‘For Loop’
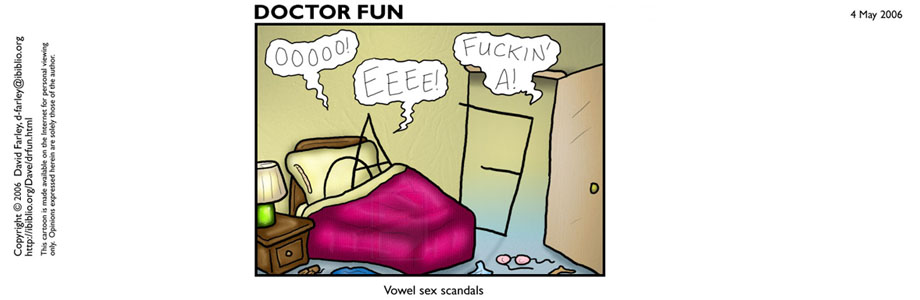
Here is a simple program, which demonstrates more practice using the input/output mechanisms which are available in Java.
This program will prompt the user to enter a sentence, then upon entering an “exit code,” will display the total number of uppercase letters, vowels and characters which are contained within that sentence. This program is very similar to an earlier project, this time, utilizing a for loop, strings, and user defined methods.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
For Loops
Methods (A.K.A "Functions") - What Are They?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
import java.util.Scanner; public class VowelCount { public static void main(String[] args) { // declare variables int numUpperCase = 0; int numVowel = 0; int numChars = 0; char character = ' '; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); System.out.print("Enter a sentence, ending with a period: "); userInput = cin.nextLine(); // loop thru the string until we reach the 'exit code' for(int index=0; userInput.charAt(index) != '.'; ++index) { character = userInput.charAt(index); // do nothing, we dont care about whitespace if(character == ' ') { continue; } // check to see if entered data is UPPERCASE if((character >= 'A')&&(character <= 'Z')) { ++numUpperCase; // Increments Total number of uppercase by one } // check to see if entered data is a vowel if((character == 'a')||(character == 'A')||(character == 'e')|| (character == 'E')||(character == 'i')||(character == 'I')|| (character == 'o')||(character == 'O')||(character == 'u')|| (character == 'U')||(character == 'y')||(character == 'Y')) { ++numVowel; // increment total number of vowels by one } ++numChars; // increment total number of chars by one } setwf("ntTotal number of upper case letters:", 45,'.'); System.out.println(numUpperCase); setwf("tTotal number of vowels:", 44,'.'); System.out.println(numVowel); setwf("tTotal number of characters:", 44,'.'); System.out.println(numChars); }// end of main public static void setwf(String str, int width, char fill) {// this mimics the C++ 'setw' function & formats string output to the screen System.out.print(str); for (int x = str.length(); x < width; ++x) { System.out.print(fill); } }// end of setwf }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted portions are areas of interest.
Notice line 22 contains the for loop declaration. The loop will continually loop thru the string, and will not stop doing so until it reaches an exit character, and the defined exit character in this program is a period (“.”). So, the program will not stop looping thru the string until it reaches a period.
Once compiling the above code, you should receive this as your output
Welcome to My Programming Notes' Java Program.
Enter a sentence, ending with a period:
My Programming Notes Is An Awesome Site.Total number of upper case letters:........7
Total number of vowels:....................14
Total number of characters:................33
Java || Compute The Sum From A String Of Integers & Display Each Number Individually
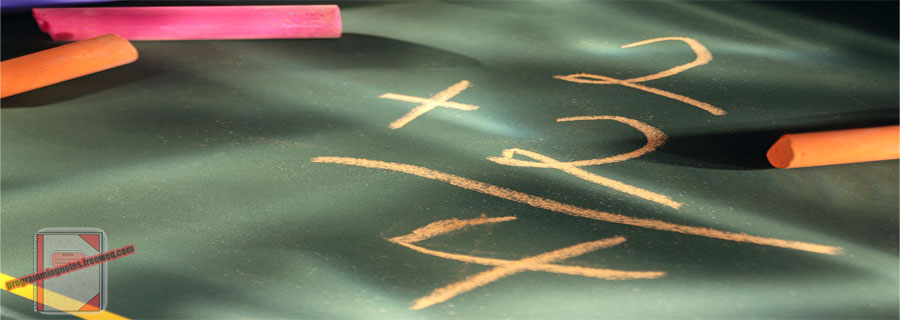
Here is a simple program, which was presented in a Java course. This program was used to introduce the input/output mechanisms which are available in Java. This assignment was modeled after Exercise 2.30, taken from the textbook “Java How to Program” (early objects) (9th Edition) (Deitel). It is the same exercise in both the 8th and 9th editions.
Our class was asked to make a program which prompts the user to enter a non-negative integer into the system. The program was supposed to then extract the digits from the inputted number, displaying them each individually, separated by a white space (” “). After the digits are displayed, the program was then supposed to display the sum of those digits to the screen. So for example, if the user inputted the number “39465,” the program would output the numbers 3 9 4 6 5 individually, and then the sum of 27.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Do/While Loops
For Loops
Methods (A.K.A "Functions") - What Are They?
ParseInt - Convert String To Integer
Substring
Try/Catch
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
import java.util.Scanner; import java.lang.Math; public class SumFromString { public static void main(String[] args) { // declare variables char question = 'n'; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); do{// this is the start of the do/while loop int sum = 0; // get data from the user System.out.print("nEnter a non negative integer: "); userInput = cin.nextLine(); System.out.println(""); // if the string isnt a number, dont compute the sum if(!IsNumeric(userInput)) { System.out.println(userInput+" is not a number..."); System.out.println("Please enter digits only!"); } // if the string is a negative number, dont compute the sum else if(IsNegative(userInput)) { System.out.println(userInput+" is a negative number..."); System.out.println("Please enter positive digits only!"); } // if the string is a decimal number (i.e 19.87), dont compute the sum else if(IsDecimal(userInput)) { System.out.println(userInput+" is a decimal number..."); System.out.println("Please enter positive whole numbers only!"); } // if everything else checks out OK, then compute the sum else { System.out.print("The digits are: "); sum = ComputeSum(userInput); System.out.println("and the sum is " + sum); } // ask user if they want to enter more data System.out.print("nDo you have more data for input? (Y/N): "); String response = cin.nextLine(); response=response.toLowerCase(); question = response.charAt(0); System.out.println("n------------------------------------------------"); }while(question == 'y'); System.out.print("nBYE!n"); }// end of main public static boolean IsNumeric(String str) {// checks to see if a string is a number try { Double.parseDouble(str); return true; } catch(NumberFormatException nfe) { return false; } }// end of IsNumeric public static boolean IsNegative(String str) {// checks to see if string is a negative number return Double.parseDouble(str) < 0; }// end of IsNegative public static boolean IsDecimal(String str) {// checks to see if string is a decimal number return (Math.floor(Double.parseDouble(str))) != Double.parseDouble(str); }// end of IsDecimal public static int ComputeSum(String userInput) {// displays each number individually & computes the sum of the string int sum = 0; String[] temp = new String[userInput.length()]; for(int counter=0; counter < userInput.length(); ++counter) { temp[counter] = userInput.substring(counter,counter+1); System.out.print(temp[counter] + " "); sum = sum + Integer.parseInt(temp[counter]); } return sum; }// end of ComputeSum }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Enter a non negative integer: 0
The digits are: 0 and the sum is 0
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 39465The digits are: 3 9 4 6 5 and the sum is 27
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: H3ll0 W0rldH3ll0 W0rld is not a number...
Please enter digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: -98-98 is a negative number...
Please enter positive digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 19.8719.87 is a decimal number...
Please enter positive whole numbers only!Do you have more data for input? (Y/N): n
------------------------------------------------
BYE!