Monthly Archives: September 2022
C# || How To Traverse N-ary Tree Level Order Using C#
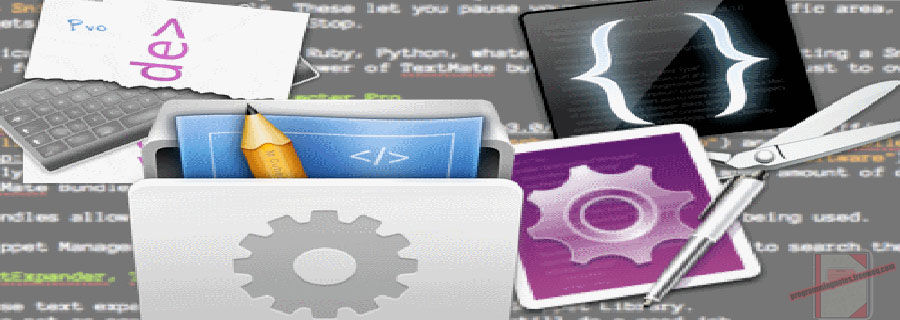
The following is a module with functions which demonstrates how to traverse a N-ary Tree level order using C#.
1. Level Order – Problem Statement
Given an n-ary tree, return the level order traversal of its nodes’ values.
Nary-Tree input serialization is represented in their level order traversal, each group of children is separated by the null value (See examples).
Example 1:
Input: root = [1,null,3,2,4,null,5,6]
Output: [[1],[3,2,4],[5,6]]
Example 2:
Input: root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14]
Output: [[1],[2,3,4,5],[6,7,8,9,10],[11,12,13],[14]]
2. Level Order – Solution
The following is a solution which demonstrates how to traverse a N-ary Tree level order.
This solution uses Breadth First Search to explore items at each level.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
// ============================================================================ // Author: Kenneth Perkins // Date: Sep 5, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to traverse a N-ary Tree Level Order // ============================================================================ /* // Definition for a Node. public class Node { public int val; public IList<Node> children; public Node() {} public Node(int _val) { val = _val; } public Node(int _val, IList<Node> _children) { val = _val; children = _children; } } */ public class Solution { public IList<IList<int>> LevelOrder(Node root) { var result = new List<IList<int>>(); if (root == null) { return result; } var queue = new Queue<Node>(); queue.Enqueue(root); while (queue.Count > 0) { var currentLevel = new List<int>(); for (int count = queue.Count - 1; count >= 0 ; --count) { var currentNode = queue.Dequeue(); currentLevel.Add(currentNode.val); foreach (var child in currentNode.children) { queue.Enqueue(child); } } result.Add(currentLevel); } return result; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[[1],[3,2,4],[5,6]]
[[1],[2,3,4,5],[6,7,8,9,10],[11,12,13],[14]]