Monthly Archives: August 2020
JavaScript || How To Make Change – Display The Total Sales Amount In Dollars & Cents Using Modulus
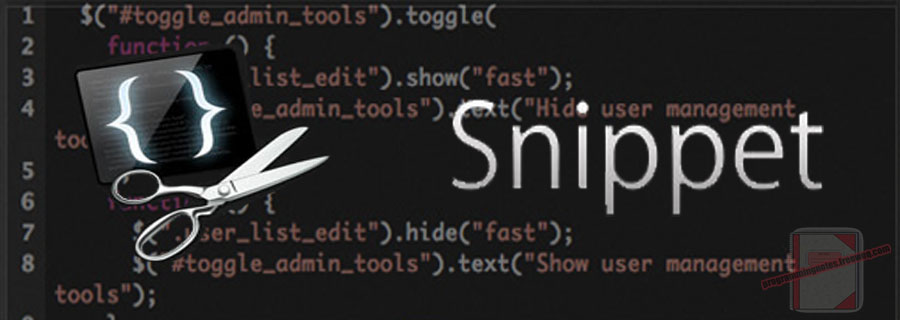
The following is a module with functions which demonstrates the use of the modulus (%) operator to make change.
So for example, given the value of 2.34, this function would return the result of 2 dollars, 1 quarters, 1 nickels, and 4 pennies.
1. Make Change
The example below demonstrates how to make change.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
// Make change <script> (() => { let values = [ 19.87, 11.93, 187.91, 3, 20.19, 1991 ]; values.forEach(value => { // Make change let change = Utils.makeChange(value); console.log(`\n$${value} is made up of: `); for (let amount of change) { console.log(`\t${amount.denomination.description}: x${amount.quantity}`); } }); })(); </script> // expected output: /* $19.87 is made up of: Ten-Dollar Bill: x1 Five-Dollar Bill: x1 One-Dollar Bill: x4 Quarter: x3 Dime: x1 Penny: x2 $11.93 is made up of: Ten-Dollar Bill: x1 One-Dollar Bill: x1 Quarter: x3 Dime: x1 Nickel: x1 Penny: x3 $187.91 is made up of: Hundred-Dollar Bill: x1 Fifty-Dollar Bill: x1 Twenty-Dollar Bill: x1 Ten-Dollar Bill: x1 Five-Dollar Bill: x1 One-Dollar Bill: x2 Quarter: x3 Dime: x1 Nickel: x1 Penny: x1 $3 is made up of: One-Dollar Bill: x3 $20.19 is made up of: Twenty-Dollar Bill: x1 Dime: x1 Nickel: x1 Penny: x4 $1991 is made up of: Hundred-Dollar Bill: x19 Fifty-Dollar Bill: x1 Twenty-Dollar Bill: x2 One-Dollar Bill: x1 */ |
2. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 29, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: makeChange * USE: Makes change out of a given dollar amount. * @param amount: The dollar amount of the change to make. * @return: An array of Denomination info that makes up the given amount. */ exposed.makeChange = (amount) => { let denominations = exposed.Denomination.get(); let remainingCents = exposed.Denomination.convert(amount); let change = []; for (const denomination of denominations) { let quantity = Math.floor(remainingCents / denomination.cents()); remainingCents %= denomination.cents(); if (quantity > 0) { change.push({ quantity: quantity, denomination: denomination, }); } } return change } exposed.Denomination = class { constructor(dollars, description) { this.dollars = dollars; this.description = description; } cents() { return exposed.Denomination.convert(this.dollars); } static convert(dollars) { return dollars * 100; } static get() { let denominations = [ new exposed.Denomination(100, 'Hundred-Dollar Bill'), new exposed.Denomination(50, 'Fifty-Dollar Bill'), new exposed.Denomination(20, 'Twenty-Dollar Bill'), new exposed.Denomination(10, 'Ten-Dollar Bill'), new exposed.Denomination(5, 'Five-Dollar Bill'), new exposed.Denomination(1, 'One-Dollar Bill'), new exposed.Denomination(.25, 'Quarter'), new exposed.Denomination(.10, 'Dime'), new exposed.Denomination(.05, 'Nickel'), new exposed.Denomination(.01, 'Penny'), ]; return denominations; } } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || Swap Two HTML Element Locations In Dom Tree Using Vanilla JavaScript
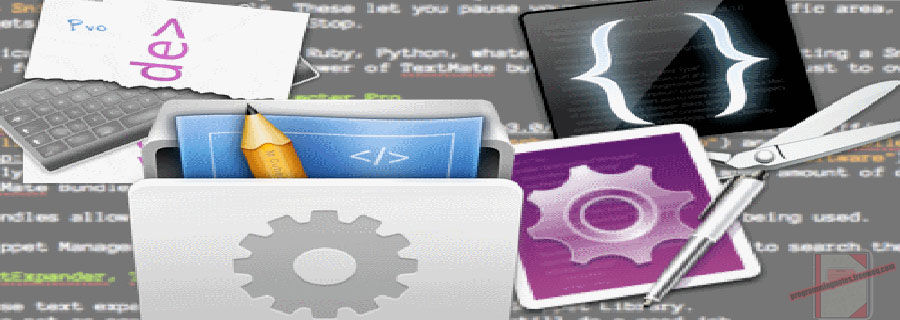
The following is a module with functions that demonstrates a simple way how to swap two HTML element locations in the Dom tree using JavaScript.
This method retains any existing event listeners.
1. Swap Element Locations
The example below demonstrates how to swap two HTML element locations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
<!-- // Swap Element Locations. --> <div class="container"> <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="first">I'm first</p> <div> <p class="second">I'm second</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> </div> <script> (() => { let first = document.querySelector('.first'); let second = document.querySelector('.second'); let container = document.querySelector('.container'); console.log('=== Before Swap ==='); console.log(container.innerHTML); // Swap the two locations Utils.swapLocations(first, second); console.log('=== After Swap ==='); console.log(container.innerHTML); })(); </script> <!-- // expected output: /* === Before Swap === <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="first">I'm first</p> <div> <p class="second">I'm second</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> === After Swap === <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="second">I'm second</p> <div> <p class="first">I'm first</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> */ --> |
2. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 28, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: swapLocations * USE: Swaps two Javascript element locations in the DOM tree. * @param elementX: The first Javascript element to swap. * @param elementY: The second Javascript element to swap. * @return: N/A. */ exposed.swapLocations = (elementX, elementY) => { let parentY = elementY.parentNode; let nextY = elementY.nextSibling; if (nextY === elementX) { parentY.insertBefore(elementX, elementY); } else { elementX.parentNode.insertBefore(elementY, elementX); if (nextY) { parentY.insertBefore(elementX, nextY); } else { parentY.appendChild(elementX); } } } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || Check If An Element Comes Before Or After Another Element In Dom Tree Using Vanilla JavaScript
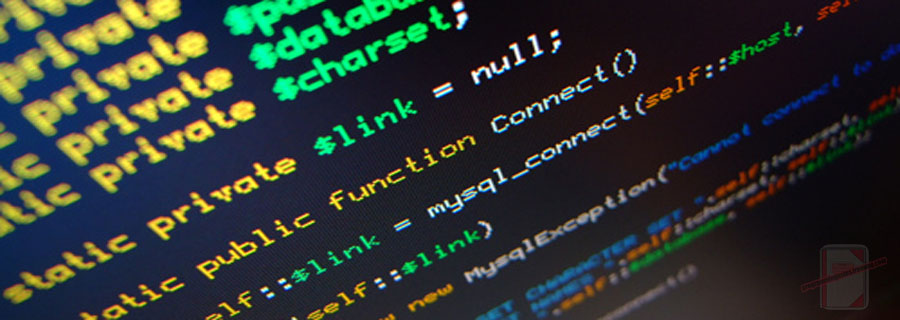
The following is a module with functions that demonstrates a simple way how to check if an element comes before or after another element in the Dom tree using JavaScript.
1. Check If Before / After
The example below demonstrates how to check if an element is before or after another element.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
<!-- // Check before / after. --> <div class="container"> <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="first">I'm first</p> <div> <p class="second">I'm second</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> </div> <script> (() => { let first = document.querySelector('.first'); let second = document.querySelector('.second'); console.log(Utils.isBefore(first, second)); console.log(Utils.isAfter(first, second)); })(); </script> <!-- // expected output: /* true false */ --> |
2. Refined Search
The example below demonstrates how to check if an element is before or after another element by refining the search by a specific container.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
<!-- // Refined Search. --> <div class="container"> <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="first">I'm first</p> <div> <p class="second">I'm second</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> </div> <script> (() => { let first = document.querySelector('.first'); let second = document.querySelector('.second'); let container = document.querySelector('.container'); console.log(Utils.isBefore(first, second, container)); console.log(Utils.isAfter(first, second, container)); })(); </script> <!-- // expected output: /* true false */ --> |
3. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 28, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: isBefore * USE: Determines if 'elementX' comes before 'elementY' in the DOM tree. * @param elementX: The first Javascript element to compare. * @param elementY: The second Javascript element to compare. * @param container: Optional. The container to limit the search. * @return: True if 'elementX' comes before 'elementY', false otherwise. */ exposed.isBefore = (elementX, elementY, container = null) => { container = container || (elementX.parentNode === elementY.parentNode ? elementX.parentNode : null) || document; let items = container.querySelectorAll('*'); let result = false; for (const item of items) { if (item === elementX) { result = true; break; } else if (item === elementY) { result = false; break; } } return result; } /** * FUNCTION: isAfter * USE: Determines if 'elementX' comes after 'elementY' in the DOM tree. * @param elementX: The first Javascript element to compare. * @param elementY: The second Javascript element to compare. * @param container: Optional. The container to limit the search. * @return: True if 'elementX' comes after 'elementY', false otherwise. */ exposed.isAfter = (elementX, elementY, container = null) => { return !exposed.isBefore(elementX, elementY, container); } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || How To Get The Next & Previous Multiple Of A Number Using Vanilla JavaScript
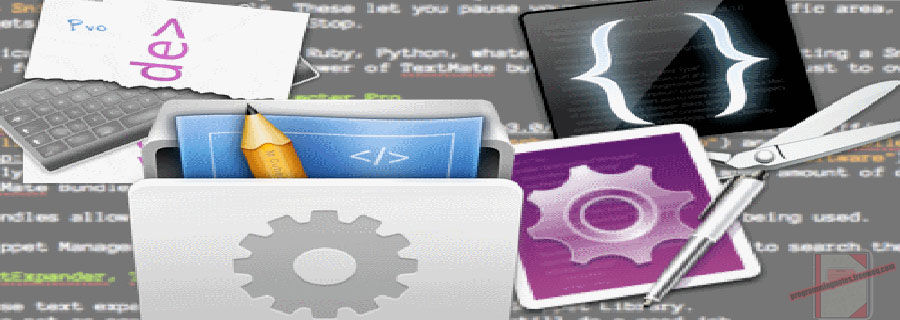
The following is a module with functions that demonstrates how to get the next and previous multiple of a number using Vanilla JavaScript.
If a number is already a multiple, there is a parameter that allows you to specify if it should be rounded or not.
1. Get Next Multiple – Include Existing
The example below demonstrates how to round up a number to the next multiple. In this example, if a number is already a multiple, it will not be rounded up to the next multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Get next multiple - include if already a multiple. <script> (() => { let multiple = 5; for (let index = 1; index <= 10; ++index) { console.log(index, '=>', Utils.getNextMultiple(index, multiple)); } })(); </script> // expected output: /* 1 => 5 2 => 5 3 => 5 4 => 5 5 => 5 6 => 10 7 => 10 8 => 10 9 => 10 10 => 10 */ |
2. Get Next Multiple – Skip Existing
The example below demonstrates how to round up a number to the next multiple. In this example, if a number is already a multiple, it will be rounded up to the next multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Get next multiple - skip if already a multiple. <script> (() => { let multiple = 5; // Set 3rd parameter to true to skip numbers that are already a // multiple to the next value for (let index = 1; index <= 10; ++index) { console.log(index, '=>', Utils.getNextMultiple(index, multiple, true)); } })(); </script> // expected output: /* 1 => 5 2 => 5 3 => 5 4 => 5 5 => 10 6 => 10 7 => 10 8 => 10 9 => 10 10 => 15 */ |
3. Get Previous Multiple – Include Existing
The example below demonstrates how to round down a number to the previous multiple. In this example, if a number is already a multiple, it will not be rounded down to the previous multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Get previous multiple - include if already a multiple. <script> (() => { let multiple = 5; for (let index = 10; index >= 1; --index) { console.log(index, '=>', Utils.getPreviousMultiple(index, multiple)); } })(); </script> // expected output: /* 10 => 10 9 => 5 8 => 5 7 => 5 6 => 5 5 => 5 4 => 0 3 => 0 2 => 0 1 => 0 */ |
4. Get Previous Multiple – Skip Existing
The example below demonstrates how to round down a number to the previous multiple. In this example, if a number is already a multiple, it will be rounded down to the previous multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Get previous multiple - skip if already a multiple. <script> (() => { let multiple = 5; // Set 3rd parameter to true to skip numbers that are already a // multiple to the previous value for (let index = 10; index >= 1; --index) { console.log(index, '=>', Utils.getPreviousMultiple(index, multiple, true)); } })(); </script> // expected output: /* 10 => 5 9 => 5 8 => 5 7 => 5 6 => 5 5 => 0 4 => 0 3 => 0 2 => 0 1 => 0 */ |
5. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 8, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: getNextMultiple * USE: Returns the next multiple of a given number. Unless specified, * it will not round up numbers which are already multiples * @param number: The given number to find the next multiple * @param multiple: The multiple number * @param skipAlreadyMultiple: Boolean that specifies if input numbers which * are already multiples should be skipped to the next multiple or not * @return: The next multiple of the given number */ exposed.getNextMultiple = (number, multiple, skipAlreadyMultiple = false) => { let result = 0; if (multiple !== 0) { let remainder = (Math.abs(number) % multiple); if (!skipAlreadyMultiple && remainder === 0) { result = number; } else if (number < 0 && remainder !== 0) { result = -(Math.abs(number) - remainder); } else { result = number + (multiple - remainder); } } return result; } /** * FUNCTION: getPreviousMultiple * USE: Returns the previous multiple of a given number. Unless specified, * it will not round down numbers which are already multiples * @param number: The given number to find the previous multiple * @param multiple: The multiple number * @param skipAlreadyMultiple: Boolean that specifies if input numbers which * are already multiples should be skipped to the previous multiple or not. * @return: The previous multiple of the given number */ exposed.getPreviousMultiple = (number, multiple, skipAlreadyMultiple = false) => { return exposed.getNextMultiple(number, multiple, !skipAlreadyMultiple) - multiple; } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript || How To Scroll To An Element & Add Automatic Anchor Scroll Using Vanilla JavaScript
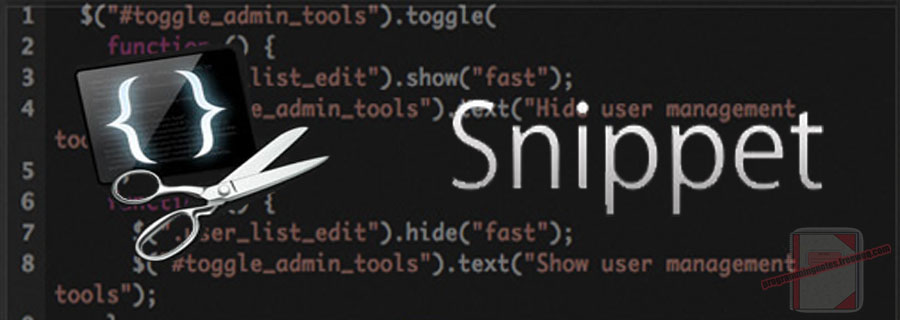
The following is a module with functions that demonstrates how to scroll to a specific HTML element, as well as setting anchors to have a smooth scroll effect on button click.
1. Scroll To Element
The example below demonstrates how to scroll to a specific element.
1 2 3 4 5 6 7 |
// Scroll to a specific element <script> (() => { Utils.scrollToElement("#examples"); })(); </script> |
The ‘Utils.scrollToElement‘ function accepts either a JavaScript element or a string selector of the element to scroll to.
2. Add Scroll To Anchor Target
The example below demonstrates how to automatically add a scrolling effect to an anchor target.
1 2 3 4 5 6 7 |
// Scroll to anchor target <script> (() => { Utils.registerAnchorScroll(); })(); </script> |
The ‘Utils.registerAnchorScroll‘ function gets all the anchor links on a page and registers smooth scrolling on button click using ‘Utils.scrollToElement‘.
3. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 7, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: registerAnchorScroll * USE: Gets all the anchor links on a page and registers smooth scrolling * on button click. * @return: N/A. */ exposed.registerAnchorScroll = () => { let anchorLinks = document.querySelectorAll('a[href^="#"]'); for (let link of anchorLinks) { link.addEventListener('click', (e) => { let hashval = link.getAttribute('href'); let target = document.querySelector(hashval) || document.querySelector(`[name='${hashval.slice(1)}']`); if (target) { exposed.scrollToElement(target); history.pushState(null, null, hashval); e.preventDefault(); } }); } } /** * FUNCTION: scrollToElement * USE: Scrolls the page to the given element. * @param target: A JavaScript element or a string selector of the * element to scroll to. * @param behavior: The scroll behavior. Defaut is 'smooth' * @return: N/A. */ exposed.scrollToElement = (target, behavior = 'smooth') => { if (typeof target === 'string') { target = document.querySelector(target); } target.scrollIntoView({ behavior: behavior, block: 'start' }); } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of ‘Utils.js‘. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Aug 7, 2020 // Taken From: http://programmingnotes.org/ // File: scrollDemo.html // Description: Demonstrates the use of Utils.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes Utils.js Demo</title> <style> .space {margin-top: 1000px;} </style> <!-- // Include module --> <script type="text/javascript" src="./Utils.js"></script> </head> <body> <blockquote><code> <a href="#basicUsage">1. Basic Usage</a> <a href="#examples">2. Examples</a> </code></blockquote> <div class="space"></div> <h3 id="basicUsage"> 1. Basic Usage </h3> <div class="space"></div> <h3 id="examples"> 2. Examples </h3> <div class="space"></div> <script> document.addEventListener("DOMContentLoaded", function(eventLoaded) { Utils.registerAnchorScroll(); //Utils.scrollToElement("#examples"); }); </script> </body> </html> <!-- http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
JavaScript/CSS/HTML || TablePagination.js – Simple Add Pagination To Any Table Using Vanilla JavaScript
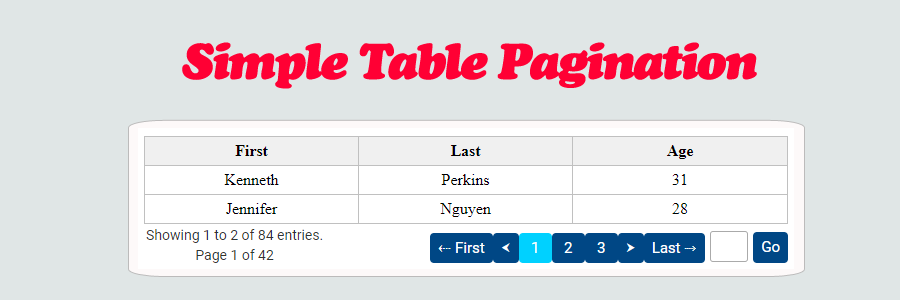
The following is a module which allows for simple yet fully customizable table pagination in vanilla JavaScript.
With multiple options, as well as multiple callback functions to modify the pagination buttons and information text, almost all the ways you can think of pagination is supported by this module. It’s look and feel can also be adjusted via CSS.
Contents
1. Basic Usage
2. Available Options
3. Only Show Page Numbers
4. Mini - Only Show Arrow Buttons
5. Show All Pages
6. Only Show Go Input
7. Format Navigation Text
8. Button Click Event
9. Navigation Position
10. Specify Initial Page
11. Navigation Binding Area
12. Remove Pagination
13. TablePagination.js & CSS Source
14. More Examples
Syntax is very straightforward. The following demonstrates adding pagination to a table.
Calling ‘TablePagination.page‘ with no options will add pagination with all of the default options applied to it. It accepts one or more HTMLTableElement.
1 2 3 4 5 6 7 8 |
// Add table pagination. <script> (() => { // Adds table pagination to the table elements with the default options TablePagination.page(document.querySelectorAll('table')); })(); </script> |
2. Available Options
The options supplied to the ‘TablePagination.page‘ function is an object that is made up of the following properties.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
// Available options TablePagination.page({ table: document.querySelector('table'), // One or more Javascript elements of the tables to page. rowsPerPage: 4, // Optional. The number of rows per page. Default is 5 initialPage: null, // Optional. The initial page to display. Possible values: Numeric value or 'first/last'. Default is page 1 navigationPosition: 'bottom', // Optional. The navigation position. Possible values: 'top/bottom'. Default is 'bottom' showFirstPageButton: true, // Optional. Specifies if the first page button is shown. Default is true showLastPageButton: true, // Optional. Specifies if the last page button is shown. Default is true showPreviousPageButton: true, // Optional. Specifies if the previous page button is shown. Default is true showNextPageButton: true, // Optional. Specifies if the next page button is shown. Default is true showPageNumberButtons: true, // Optional. Specifies if the page number buttons are shown. Default is true showNavigationInput: true, // Optional. Specifies if the 'Go' search functionality is shown. Default is true showNavigationInfoText: true, // Optional. Specifies if the navigation info text is shown. Default is true visiblePageNumberButtons: 4, // Optional. The maximum number of visible page number buttons. Default is 3. Set to null to show all buttons onButtonClick: (pageNumber, event) => { // Optional. Function that allows to do something on button click //window.location.href = "#page=" + pageNumber; }, onButtonTextRender: (text, desc) => { // Optional. Function that allows to format the button text //console.log(`Button Text: ${text}`); //console.log(`Button Description: ${desc}`); return text; }, onButtonTitleRender: (title, desc) => { // Optional. Function that allows to format the button title //console.log(`Button Text: ${text}`); //console.log(`Button Description: ${desc}`); return title; }, onNavigationInfoTextRender: (text, rowInfo) => { // Optional. Function that allows to format the navigation info text //console.log(`Navigation Text: ${text}`); //console.log(`Row Info:`, rowInfo); return text; }, navigationBindTo: null, // Optional. Javascript element of the container where the navigation controls are bound to. // If not specified, default destination is above or below the table element, depending on // the 'navigationPosition' value }); |
Supplying different options to ‘TablePagination.page‘ can change its appearance. The following examples below demonstrate this.
3. Only Show Page Numbers
The following example demonstrates pagination with only page numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// Pagination with only page numbers. <script> (() => { // Only show page numbers TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, showFirstPageButton: false, showLastPageButton: false, showNavigationInput: false, }); })(); </script> |
4. Mini – Only Show Arrow Buttons
The following example demonstrates pagination with only arrow buttons.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Pagination with only arrow buttons. <script> (() => { // Only show arrow buttons TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, showFirstPageButton: false, showLastPageButton: false, showPageNumberButtons: false, showNavigationInput: false, }); })(); </script> |
5. Show All Pages
The following example demonstrates pagination showing all page numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Pagination showing all page numbers. <script> (() => { // Show all page numbers TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 8, showFirstPageButton: false, showLastPageButton: false, showPreviousPageButton: false, showNextPageButton: false, showNavigationInput: false, visiblePageNumberButtons: null }); })(); </script> |
6. Only Show Go Input
The following example demonstrates pagination with only showing the “Go” user input option.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
// Pagination with only showing go input. <script> (() => { // Only showing go input TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, showFirstPageButton: false, showLastPageButton: false, showPreviousPageButton: false, showNextPageButton: false, showPageNumberButtons: false, }); })(); </script> |
7. Format Navigation Text
The following example demonstrates pagination formatting the navigation text. This allows to alter the navigation text without having to modify the pagination code!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
// Pagination formatting the navigation text. <script> (() => { // Pagination formatting the navigation text TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, onButtonTextRender: (text, desc) => { switch (desc) { case TablePagination.settings.onRenderDescFirstPage: break case TablePagination.settings.onRenderDescPrevPage: break case TablePagination.settings.onRenderDescPageNumber: text += ' - Test'; break; case TablePagination.settings.onRenderDescNextPage: break case TablePagination.settings.onRenderDescLastPage: break case TablePagination.settings.onRenderDescGoInput: break } return text; }, onButtonTitleRender: (title, desc) => { switch (desc) { case TablePagination.settings.onRenderDescFirstPage: break case TablePagination.settings.onRenderDescPrevPage: break case TablePagination.settings.onRenderDescPageNumber: title += ' - Test'; break; case TablePagination.settings.onRenderDescNextPage: break case TablePagination.settings.onRenderDescLastPage: break case TablePagination.settings.onRenderDescGoInput: break } return title; }, onNavigationInfoTextRender: (text, rowInfo) => { text += ' - Test' return text; }, }); })(); </script> |
8. Button Click Event
The following example demonstrates pagination with the button click callback. This allows you to do something on button click
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Pagination onButtonClick callback. <script> (() => { // onButtonClick callback allows you to do something on button click TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, onButtonClick: (pageNumber, event) => { alert(pageNumber); window.location.href = "#page=" + pageNumber; }, }); })(); </script> |
9. Navigation Position
The following example demonstrates formatting the navigation position. The position options can be on top, or bottom. Bottom is the default position.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Pagination navigation position. <script> (() => { // Navigation position - top TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, navigationPosition: 'top' }); })(); </script> |
10. Specify Initial Page
The following example demonstrates pagination setting the initial default page.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Pagination with initial page set. <script> (() => { // Set initial page TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, initialPage: 3 }); })(); </script> |
11. Navigation Binding Area
By default, the navigation controls are placed either above or below the table element, depending on the ‘navigationPosition’ value. You can override this placement by specifying the container where the controls should be bound to.
The following example demonstrates specifying the element where the controls are bound to.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// Navigation Binding Area. <script> (() => { // Navigation Binding Area TablePagination.page({ table: document.querySelectorAll('table'), rowsPerPage: 2, navigationPosition: 'bottom', navigationBindTo: document.querySelector('#binding-section') }); })(); </script> |
12. Remove Pagination
The following example demonstrates how to remove pagination from a table.
‘TablePagination.remove‘ will remove pagination. It accepts one or more HTMLTableElement.
1 2 3 4 5 6 7 8 |
// Remove table pagination. <script> (() => { // Removes table pagination from the table elements TablePagination.remove(document.querySelectorAll('table')); })(); </script> |
13. TablePagination.js & CSS Source
The following is the TablePagination.js Namespace & CSS Source. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 609 610 611 612 613 614 615 616 617 618 619 620 621 622 623 624 625 626 627 628 629 630 631 632 633 634 635 636 637 638 639 640 641 642 643 644 645 646 647 648 649 650 651 652 653 654 655 656 657 658 659 660 661 662 663 664 665 666 667 668 669 670 671 672 673 674 675 676 677 678 679 680 681 682 683 684 685 686 687 688 689 690 691 692 693 694 695 696 697 698 699 700 701 702 703 704 705 706 707 708 709 710 711 712 713 714 715 716 717 718 719 720 721 722 723 724 725 726 727 728 729 730 731 732 733 734 735 736 737 738 739 740 741 742 743 744 745 746 747 748 749 750 751 752 753 754 755 756 757 758 759 760 761 762 763 764 765 766 767 768 769 770 771 772 773 774 775 776 777 778 779 780 781 782 783 784 785 786 787 788 789 790 791 792 793 794 795 796 797 798 799 800 801 802 803 804 805 806 807 808 809 810 811 812 813 814 815 816 817 818 819 820 821 822 823 824 825 826 827 828 829 830 831 832 833 834 835 836 837 838 839 840 841 842 843 844 845 846 847 848 849 850 851 852 853 854 855 856 857 858 859 860 861 862 863 864 865 866 867 868 869 870 871 872 873 874 875 876 877 878 879 880 881 882 883 884 885 886 887 888 889 890 891 892 893 894 895 896 897 898 899 900 901 902 903 904 905 906 907 908 909 910 911 912 913 914 915 916 917 918 919 920 921 922 923 924 925 926 927 928 929 930 931 932 933 934 935 936 937 938 939 940 941 942 943 944 945 946 947 948 949 950 951 952 953 954 955 956 957 958 959 960 961 962 963 964 965 966 967 968 969 970 971 972 973 974 975 976 977 978 979 980 981 982 983 984 985 986 987 988 989 990 991 992 993 994 995 996 997 998 999 1000 1001 1002 1003 1004 1005 1006 1007 1008 1009 1010 1011 1012 1013 1014 1015 1016 1017 1018 1019 1020 1021 1022 1023 1024 1025 1026 1027 1028 1029 1030 1031 1032 1033 1034 1035 1036 1037 1038 1039 1040 1041 1042 1043 1044 1045 1046 1047 1048 1049 1050 1051 1052 1053 1054 1055 1056 1057 1058 1059 1060 1061 1062 1063 1064 1065 1066 1067 1068 1069 1070 1071 1072 1073 1074 1075 1076 1077 1078 1079 1080 1081 1082 1083 1084 1085 1086 1087 1088 1089 1090 1091 1092 1093 1094 1095 1096 1097 1098 1099 1100 1101 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 5, 2020 // Taken From: http://programmingnotes.org/ // File: TablePagination.js // Description: Namespace which handles html table pagination // Example: // // Add Pagination to a table // TablePagination.page({ // table: document.querySelector('table'), // rowsPerPage: 10 // }); // ============================================================================ /** * NAMESPACE: TablePagination * USE: Handles html table pagination. */ var TablePagination = TablePagination || {}; (function(namespace) { 'use strict'; // -- Public data -- // Property to hold public variables and functions let exposed = namespace; // Set class names and other shared data const settings = { // Misc settings defaultRowsPerPage: 5, defaultPosition: 'bottom', defaultVisibleButtons: 3, onRenderDescFirstPage: 'first_page', onRenderDescPrevPage: 'previous_page', onRenderDescPageNumber: 'page_number', onRenderDescNextPage: 'next_page', onRenderDescLastPage: 'last_page', onRenderDescGoInput: 'go_input', buttonOptionFirst: 'first', buttonOptionPrevious: 'previous', buttonOptionNext: 'next', buttonOptionLast: 'last', navigationPositionTop: 'top', navigationPositionBottom: 'bottom', onTextRender: (text) => text, // Element class names classNameHide: '.pagination-hide', classNameButton: 'pagination-btn', classNameButtonActive: 'active', classNameButtonFirst: 'first', classNameButtonPrevious: 'previous', classNameButtonPageNumber: 'page-number', classNameButtonNext: 'next', classNameButtonLast: 'last', classNameButtonGo: 'go', classNameButtonHide: 'hide', classNameNavigation: 'pagination-navigation', classNameNavigationTop: 'top', classNameNavigationBottom: 'bottom', classNameNavigationInfoSection: 'pagination-navigation-info-section', classNameNavigationControlSection: 'pagination-navigation-control-section', classNameNavigationButtonSection: 'pagination-navigation-button-section', classNameNavigationInputSection: 'pagination-navigation-input-section', classNameNavigationInput: 'pagination-navigation-input', // Element data names dataNamePageNumber: 'data-pageNumber', dataNameTotalPages: 'data-totalPages', dataNameRowsPerPage: 'data-rowsPerPage', dataNameCurrentPageNumber: 'data-currentPageNumber', dataNameNavigationId: 'data-navigationId', dataNameNavigationInfoId: 'data-navigationInfoId', dataNameNavigationInputId: 'data-navigationInputId', dataNameNavigationButtonsId: 'data-navigationButtonsId', dataNameVisiblePageButtons: 'data-visiblePageButtons', cleanClassName: (str) => { return str ? str.trim().replace('.', '') : ''; }, }; exposed.settings = settings; /** * FUNCTION: page * USE: Initializes & renders pagination for the given table elements * @param options: An object of initialization options. * Its made up of the following properties: * { * table: One or more Javascript elements of the tables to page. * rowsPerPage: Optional. The number of rows per page. Default is page 5 * initialPage: Optional. The initial page to display. Posible values: Numeric value * or 'first/last'. Default is page 1 * navigationPosition: Optional. The navigation position. Posible values: 'top/bottom'. * Default is 'bottom' * showFirstPageButton: Optional. Boolean that indicates if the first page * button should be displayed. Default is true * showLastPageButton: Optional. Boolean that indicates if the last page * button should be displayed. Default is true * showPreviousPageButton: Optional. Boolean that indicates if the previous page * button should be displayed. Default is true * showNextPageButton: Optional. Boolean that indicates if the next page * button should be displayed. Default is true * showPageNumberButtons: Optional. Boolean that indicates if the page number buttons * should be displayed. Default is true * showNavigationInput: Optional. Specifies if the 'Go' search functionality is shown. * Default is true * showNavigationInfoText: Optional. Specifies if the navigation info text is shown. * Default is true * visiblePageNumberButtons: Optional. The maximum number of visible page number buttons. * Default is 3. Set to null to show all buttons * onButtonClick(pageNumber, event): Optional. Function that allows to do something on * button click * onButtonTextRender(text, desc): Optional. Function that allows to format the button text * onButtonTitleRender(title, desc): Optional. Function that allows to format the button title * onNavigationInfoTextRender(text, rowInfo): Optional. Function that allows to format * the navigation info text * navigationBindTo: Optional. Javascript element of the container where the navigation controls * are bound to. If not specified, default destination is above or below the table element, * depending on the 'navigationPosition' value * } * @return: N/A. */ exposed.page = (options) => { // Make sure the required options are valid if (isNull(options)) { // Check to see if there are options throw new TypeError('There are no options specified.'); } else if (typeof options !== 'object' || isElement(options) || isArrayLike(options)) { // Check to see if a table is specified let table = options; options = {}; options.table = table; } // Make sure the table is an array if (!isArrayLike(options.table)) { options.table = [options.table]; } // Make sure additional options are valid if (!isNull(options.rowsPerPage) && !isNumeric(options.rowsPerPage)) { // Check to see if a rowsPerPage is valid throw new TypeError(`Unable to process rowsPerPage of type: ${typeof options.rowsPerPage}. Reason: '${options.rowsPerPage}' is not a numeric value.`); } else if (!isNull(options.navigationBindTo) && !isElement(options.navigationBindTo)) { // Check to see if the navigation bind to element is an HTMLElement throw new TypeError(`Unable to process navigationBindTo of type: ${typeof options.navigationBindTo}. Reason: '${options.navigationBindTo}' is not an HTMLElement.`); } else if (!isNull(options.onButtonTitleRender) && !isFunction(options.onButtonTitleRender)) { // Check to see if callback is a function throw new TypeError(`Unable to call onButtonTitleRender of type: ${typeof options.onButtonTitleRender}. Reason: '${options.onButtonTitleRender}' is not a function.`); } else if (!isNull(options.onButtonTextRender) && !isFunction(options.onButtonTextRender)) { // Check to see if callback is a function throw new TypeError(`Unable to call onButtonTextRender of type: ${typeof options.onButtonTextRender}. Reason: '${options.onButtonTextRender}' is not a function.`); } else if (!isNull(options.onButtonClick) && !isFunction(options.onButtonClick)) { // Check to see if callback is a function throw new TypeError(`Unable to call onButtonClick of type: ${typeof options.onButtonClick}. Reason: '${options.onButtonClick}' is not a function.`); } else if (!isNull(options.visiblePageNumberButtons) && !isNumeric(options.visiblePageNumberButtons)) { // Check to see if a visiblePageNumberButtons is valid throw new TypeError(`Unable to process visiblePageNumberButtons of type: ${typeof options.visiblePageNumberButtons}. Reason: '${options.visiblePageNumberButtons}' is not a numeric value.`); } else if (!isNull(options.onNavigationInfoTextRender) && !isFunction(options.onNavigationInfoTextRender)) { // Check to see if callback is a function throw new TypeError(`Unable to call onNavigationInfoTextRender of type: ${typeof options.onNavigationInfoTextRender}. Reason: '${options.onNavigationInfoTextRender}' is not a function.`); } // Get the tables and remove the property from the object let tables = options.table; delete options.table; // Page the tables for (let index = 0; index < tables.length; ++index) { // Get the table and make sure its valid let table = tables[index]; if (!isTable(table)) { // Check to see if the table is an HTMLTableElement throw new TypeError(`Unable to process ${getTableDisplayName(table)} of type: ${typeof table}. Reason: '${table}' is not an HTMLTableElement.`); } // Build the table navigation controls buildNavigation(table, options) // Add click events for the navigation controls addClickEvents(table, options); // Show the initial page showPage(table, {pageNumber: getInitialPage(table, options.initialPage), pageOptions: options}); } } /** * FUNCTION: remove * USE: Removes the rendered table pagination * @param table: JavaScript elements of the paged tables. * @return: True if table pagination controls were removed from all tables, false otherwise. */ exposed.remove = (tables) => { if (isNull(tables)) { return false; } else if (!isArrayLike(tables)) { tables = [tables]; } let allRemoved = true; for (let index = 0; index < tables.length; ++index) { let table = tables[index]; if (!isTable(table)) { // Check to see if the table is an HTMLTableElement throw new TypeError(`Unable to process ${getTableDisplayName(table)} of type: ${typeof table}. Reason: '${table}' is not an HTMLTableElement.`); } if (!removeNavigation(table)) { allRemoved = false; } } return allRemoved; } // -- Private data -- let getRows = (table) => { let rows = table.rows; let results = []; // Only return data rows for (let indexRow = 0; indexRow < rows.length; ++indexRow) { let row = rows[indexRow]; let isTDRow = true; for (let indexCell = 0; indexCell < row.cells.length; ++indexCell) { let cell = row.cells[indexCell]; if (cell.tagName.toLowerCase() !== 'td') { isTDRow = false; break; } } if (isTDRow) { results.push(row); } } return results; } let buildNavigation = (table, options) => { // Remove the previous navigation removeNavigation(table); // Set the max rows per page let rowsPerPage = options.rowsPerPage || settings.defaultRowsPerPage; setRowsPerPage(table, rowsPerPage); // Calculate the number of pages needed and set its value let totalPages = calculateTotalPagesRequired(table); setTotalPages(table, totalPages); if (totalPages < 1) { throw new Error(`${totalPages} pages calculated in order to page the table. Exiting...`); } // Get the current options let position = (options.navigationPosition || settings.defaultPosition).trim().toLowerCase(); let showNavigationInfoText = !isNull(options.showNavigationInfoText) ? options.showNavigationInfoText : true; let navigationBindTo = options.navigationBindTo || table.parentNode; let isCustomBinding = !isNull(options.navigationBindTo); // Add the navigation controls to the page let navigationContainer = attachNavigation({ table: table, container: navigationBindTo, isCustomBinding: isCustomBinding, position: position, classes: getNavigationClasses(position), data: [{key: 'tabindex', value: 0}] }); // Show page info if (showNavigationInfoText) { attachNavigationInfo({ table: table, container: navigationContainer, classes: [settings.classNameNavigationInfoSection] }); } // Add navigation buttons attatchNavigationButtons({ table: table, pageOptions: options, container: navigationContainer, classes: [settings.classNameNavigationControlSection] }); } let addClickEvents = (table, options) => { let paginationButtons = getNavigationButtons(table); let inputButton = getInputButton(table); let inputTextbox = getInputTextbox(table); let navigation = document.querySelector(`#${getNavigationId(table)}`); // Make sure there are visible navigation buttons let navigationVisible = (!isNull(paginationButtons) && paginationButtons.length > 0) || (!isNull(inputButton) && !isNull(inputTextbox)); // Throw an error if there are no visible navigation buttons if (!navigationVisible) { throw new Error(`The settings chosen on ${getTableDisplayName(table)} do not allow for any visible navigation buttons!`); } // Function to go to a page let navigateToPage = (pageNumber, event) => { pageNumber = translatePageNumber(table, pageNumber); if (!isNumeric(pageNumber)) { return false; } // Show the page showPage(table, {pageNumber: pageNumber, pageOptions: options}); // Call the on click function if specified if (options.onButtonClick) { options.onButtonClick.call(this, pageNumber, event); } return true; } // Add click events for the navigation buttons if (!isNull(paginationButtons)) { paginationButtons.forEach((paginationButton, index) => { // Add button click paginationButton.addEventListener('click', (event) => { event.preventDefault(); let pageNumber = getButtonPageNumber(paginationButton); navigateToPage(pageNumber, event); navigation.focus({preventScroll:true}); }); }); } // Add click event for the input button if (!isNull(inputButton)) { inputButton.addEventListener('click', (event) => { event.preventDefault(); // Get the input textbox let pageNumber = getInputValue(table, inputTextbox); navigateToPage(pageNumber, event); }); } // Add click event for the input textbox if (!isNull(inputTextbox)) { inputTextbox.addEventListener('keyup', (event) => { event = event || window.event; let keyCode = event.key || event.keyCode; // Check to see if the enter button was clicked switch (String(keyCode)) { case 'Enter': case '13': inputButton.click(); break; } }); } // Add click events for the left/right keyboard buttons if (!isNull(navigation)) { navigation.addEventListener('keydown', (event) => { event = event || window.event; let keyCode = event.key || event.keyCode; let pageNumber = getCurrentPageNumber(table); // Check to see if an arrow key was clicked switch (String(keyCode)) { case 'ArrowLeft': case 'Left': case '37': --pageNumber; break; case 'ArrowRight': case 'Right': case '39': ++pageNumber; break; default: return; break; } navigateToPage(pageNumber, event); }); } } let getTableId = (table) => { let tableId = !isNull(table.id) && table.id.length > 0 ? table.id : null; return tableId; } let getTableDisplayName = (table) => { let tableId = getTableId(table); let tableName = 'Table' + (tableId ? ' id: "' + tableId + '"' : ''); return tableName; } let getInitialPage = (table, initialPage) => { let initialActivePage = 1; if (!isNull(initialPage)) { let possiblePageNumber = translatePageNumber(table, initialPage); if (isNumeric(possiblePageNumber)) { initialActivePage = possiblePageNumber; } } return initialActivePage; } let translatePageNumber = (table, pageNumber) => { if (!isNull(pageNumber) && !isNumeric(pageNumber)) { pageNumber = String(pageNumber).trim().toLowerCase(); switch (pageNumber) { case settings.buttonOptionFirst.trim().toLowerCase(): pageNumber = 1; break; case settings.buttonOptionLast.trim().toLowerCase(): pageNumber = getTotalPages(table); break; case settings.buttonOptionPrevious.trim().toLowerCase(): pageNumber = getCurrentPageNumber(table) - 1; break; case settings.buttonOptionNext.trim().toLowerCase(): pageNumber = getCurrentPageNumber(table) + 1; break; } } return pageNumber; } let getNavigationClasses = (position) => { let navigationClasses = [] navigationClasses.push(settings.classNameNavigation); if (position == settings.navigationPositionTop.trim().toLowerCase()) { navigationClasses.push(settings.classNameNavigationTop); } else { navigationClasses.push(settings.classNameNavigationBottom); } return navigationClasses; } let hideAllRows = (table) => { let totalPages = getTotalPages(table); for (let pageNumber = 1; pageNumber <= totalPages; ++pageNumber) { hidePage(table, {pageNumber: pageNumber}); } } let hidePage = (table, options) => { let pageNumber = options.pageNumber; let rowInfo = getRowInfo(table, pageNumber); let rowIndexStart = rowInfo.rowIndexStart; let rowIndexEnd = rowInfo.rowIndexEnd; hideRows(table, rowIndexStart, rowIndexEnd); } let hideRows = (table, rowIndexStart, rowIndexEnd) => { let tableRows = getRows(table); for (let index = rowIndexStart; index <= rowIndexEnd && index < tableRows.length; ++index) { let tableRow = tableRows[index]; hideRow(tableRow); } } let hideRow = (tableRow) => { addClass(tableRow, settings.classNameHide); } let showAllRows = (table) => { let totalPages = getTotalPages(table); for (let pageNumber = 1; pageNumber <= totalPages; ++pageNumber) { showPage(table, { pageNumber: pageNumber, hidePreviousRows: false }); } } let showPage = (table, options) => { if (isNull(options.hidePreviousRows) || options.hidePreviousRows) { hideAllRows(table); } let rowInfo = getRowInfo(table, options.pageNumber); let rowIndexStart = rowInfo.rowIndexStart; let rowIndexEnd = rowInfo.rowIndexEnd; let pageNumber = rowInfo.pageNumber; showRows(table, rowIndexStart, rowIndexEnd); setCurrentPageNumber(table, pageNumber); highlightButton(table, pageNumber); showPageButtons(table, pageNumber); updateInfoText(table, rowInfo, options.pageOptions); clearInputValue(table); } let showRows = (table, rowIndexStart, rowIndexEnd) => { let tableRows = getRows(table); for (let index = rowIndexStart; index <= rowIndexEnd && index < tableRows.length; ++index) { let tableRow = tableRows[index]; showRow(tableRow); } } let showRow = (tableRow) => { removeClass(tableRow, settings.classNameHide); } let clearInputValue = (table, inputTextbox = null) => { inputTextbox = inputTextbox || getInputTextbox(table); if (!isNull(inputTextbox)) { inputTextbox.value = null; } } let getInputValue = (table, inputTextbox = null) => { inputTextbox = inputTextbox || getInputTextbox(table); return !isNull(inputTextbox) ? inputTextbox.value : null; } let updateInfoText = (table, rowInfo, pageOptions) => { let navigationInfo = getNavigationInfo(table); if (isNull(navigationInfo)) { return; } let text = `Showing ${rowInfo.itemCountStart} to ${rowInfo.itemCountEnd} of ${rowInfo.totalItems} entries.`; text += `<br />`; text += `Page ${rowInfo.pageNumber} of ${rowInfo.totalPages}`; let onNavigationInfoTextRender = settings.onTextRender; if (!isNull(pageOptions) && !isNull(pageOptions.onNavigationInfoTextRender)) { onNavigationInfoTextRender = pageOptions.onNavigationInfoTextRender; } navigationInfo.innerHTML = onNavigationInfoTextRender.call(this, text, rowInfo); } let showPageButtons = (table, pageNumber) => { let visibleButtons = getTotalVisiblePageButtons(table); if (!isNumeric(visibleButtons)) { return; } let totalPages = getTotalPages(table); let firstVisiblePage = Math.max(0, getPreviousMultiple(pageNumber, visibleButtons)) + 1; let lastVisiblePage = Math.min(totalPages, getNextMultiple(pageNumber, visibleButtons)); // Make sure there are at least 'visibleButtons' total buttons shown let difference = (visibleButtons - 1) - (lastVisiblePage - firstVisiblePage); if (difference > 0) { firstVisiblePage -= difference; } getNavigationButtons(table).forEach((btn, index) => { let buttonPageNumber = getButtonPageNumber(btn); if (isNumeric(buttonPageNumber)) { buttonPageNumber = Number(buttonPageNumber); addClass(btn, settings.classNameButtonHide); if (buttonPageNumber >= firstVisiblePage && buttonPageNumber <= lastVisiblePage) { removeClass(btn, settings.classNameButtonHide); } } }); } let getRowInfo = (table, pageNumber) => { let totalPages = getTotalPages(table); let rows = getRows(table); // Make sure the page number is within valid range [1 to last page number] pageNumber = Math.max(1, Math.min(pageNumber, totalPages)); let totalItems = rows.length; let rowsPerPage = getRowsPerPage(table); let rowIndexStart = (pageNumber - 1) * rowsPerPage; let rowIndexEnd = Math.min(rowIndexStart + (rowsPerPage - 1), totalItems - 1); let rowInfo = { rowIndexStart: rowIndexStart, rowIndexEnd: rowIndexEnd, pageNumber: pageNumber, totalPages: totalPages, totalItems: totalItems, rowsPerPage: rowsPerPage, itemCountStart: rowIndexStart + 1, itemCountEnd: rowIndexEnd + 1, }; return rowInfo; } let getCurrentPageNumber = (table) => { let pageNumber = getData(table, settings.dataNameCurrentPageNumber); return isNull(pageNumber) ? 0 : Number(pageNumber); } let setCurrentPageNumber = (table, pageNumber) => { addData(table, {key: settings.dataNameCurrentPageNumber, value: pageNumber}); } let highlightButton = (table, pageNumber) => { let paginationButton = null; let paginationButtons = getNavigationButtons(table); if (isNull(paginationButtons)) { return; } // Reset the previous page button colors resetButtonColors(paginationButtons); // Mark the selected button as active paginationButtons.forEach((btn, index) => { let buttonPageNumber = getButtonPageNumber(btn); if (isNumeric(buttonPageNumber) && Number(pageNumber) === Number(buttonPageNumber)) { paginationButton = btn; return false; } }); if (!isNull(paginationButton)) { addClass(paginationButton, settings.classNameButtonActive); } } let resetButtonColors = (paginationButtons) => { if (isNull(paginationButtons)) { return; } paginationButtons.forEach((paginationButton, index) => { let buttonPageNumber = getButtonPageNumber(paginationButton); if (isNumeric(buttonPageNumber)) { removeClass(paginationButton, settings.classNameButtonActive); } }); } let getButtonPageNumber = (btn) => { let pageNumber = getData(btn, settings.dataNamePageNumber); return String(!isNull(pageNumber) ? pageNumber : 0); } let setNavigationId = (table, navigationId) => { addData(table, {key: settings.dataNameNavigationId, value: navigationId}); } let getNavigationId = (table) => { return getData(table, settings.dataNameNavigationId); } let setNavigationInfoId = (table, navigationInfoId) => { addData(table, {key: settings.dataNameNavigationInfoId, value: navigationInfoId}); } let getNavigationInfoId = (table) => { return getData(table, settings.dataNameNavigationInfoId); } let setNavigationInputId = (table, navigationInputId) => { addData(table, {key: settings.dataNameNavigationInputId, value: navigationInputId}); } let getNavigationInputId = (table) => { return getData(table, settings.dataNameNavigationInputId); } let getNavigationButtons = (table) => { let selector = `#${getNavigationButtonsId(table)} a`; return document.querySelectorAll(selector); } let getInputButton = (table) => { let selector = `#${getNavigationInputId(table)} a`; return document.querySelector(selector); } let getInputTextbox = (table) => { let selector = `#${getNavigationInputId(table)} input`; return document.querySelector(selector); } let getNavigationInfo = (table) => { let selector = `#${getNavigationInfoId(table)}`; return document.querySelector(selector); } let setNavigationButtonsId = (table, navigationButtonsId) => { addData(table, {key: settings.dataNameNavigationButtonsId, value: navigationButtonsId}); } let getNavigationButtonsId = (table) => { return getData(table, settings.dataNameNavigationButtonsId); } let getTotalVisiblePageButtons = (table) => { let value = getData(table, settings.dataNameVisiblePageButtons); return !isNull(value) ? Number(value) : value; } let setTotalVisiblePageButtons = (table, visiblePageButtons) => { addData(table, {key: settings.dataNameVisiblePageButtons, value: visiblePageButtons}); } let setRowsPerPage = (table, rowsPerPage) => { addData(table, {key: settings.dataNameRowsPerPage, value: rowsPerPage}); } let getRowsPerPage = (table) => { let value = getData(table, settings.dataNameRowsPerPage); return !isNull(value) ? Number(value) : value; } let setTotalPages = (table, totalPages) => { addData(table, {key: settings.dataNameTotalPages, value: totalPages}); } let getTotalPages = (table) => { let value = getData(table, settings.dataNameTotalPages); return !isNull(value) ? Number(value) : value; } let calculateTotalPagesRequired = (table) => { let rowsPerPage = getRowsPerPage(table); let totalRows = getRows(table).length; let totalPages = totalRows / rowsPerPage; if (totalRows % rowsPerPage !== 0) { totalPages = Math.floor(++totalPages); } return totalPages; } let addClass = (element, cssClass) => { cssClass = settings.cleanClassName(cssClass); let modified = false; if (cssClass.length > 0 && !hasClass(element, cssClass)) { element.classList.add(cssClass) modified = true; } return modified; } let removeClass = (element, cssClass) => { cssClass = settings.cleanClassName(cssClass); let modified = false; if (cssClass.length > 0 && hasClass(element, cssClass)) { element.classList.remove(cssClass); modified = true; } return modified; } let hasClass = (element, cssClass) => { cssClass = settings.cleanClassName(cssClass); return element.classList.contains(cssClass); } let isNull = (item) => { return isUndefined(item) || null === item; } let isUndefined = (item) => { return undefined === item; } let isFunction = (item) => { return 'function' === typeof item } let isTable = (item) => { return isElement(item) && item instanceof HTMLTableElement; } let isElement = (item) => { let value = false; try { value = item instanceof HTMLElement || item instanceof HTMLDocument; } catch (e) { value = (typeof item==="object") && (item.nodeType===1) && (typeof item.style === "object") && (typeof item.ownerDocument ==="object"); } return value; } let randomFromTo = (from, to) => { return Math.floor(Math.random() * (to - from + 1) + from); } let isNumeric = (n) => { return !isNaN(parseFloat(n)) && isFinite(n); } let generateNavigationId = (table) => { let tableId = getTableId(table); return 'tablePaginationNavigation_' + (tableId ? tableId : randomFromTo(1271991, 7281987)); } let removeNavigation = (table) => { let modified = false; let previousNavigationId = getNavigationId(table); // Remove the previous table navigation if (!isNull(previousNavigationId)) { let previousElement = document.querySelector(`#${previousNavigationId}`); if (!isNull(previousElement)) { previousElement.parentNode.removeChild(previousElement); showAllRows(table); modified = true; } } return modified; } let attachNavigation = (options) => { // Create the table navigation control div let navigationContainer = document.createElement('div'); navigationContainer.id = generateNavigationId(options.table); // Determine the navigation position let position = options.position.trim().toLowerCase(); if (!options.isCustomBinding) { if (position === settings.navigationPositionTop) { // Insert navigation before the table options.container.insertBefore(navigationContainer, options.table); } else { // Insert navigation after the table options.container.insertBefore(navigationContainer, options.table.nextSibling); } } else { if (position === settings.navigationPositionTop) { // Insert navigation at the beginning of the container options.container.insertBefore(navigationContainer, options.container.firstChild); } else { // Insert navigation ar end of the container options.container.insertBefore(navigationContainer, options.container.lastChild); } } // Add classes and data addClasses(navigationContainer, options.classes); addData(navigationContainer, options.data); // Set the current table navigation control id setNavigationId(options.table, navigationContainer.id); setNavigationInfoId(options.table, null); return navigationContainer; } let attachButton = (options) => { let btn = document.createElement('a'); btn.href = '#'; addClasses(btn, options.classes); addData(btn, options.data); btn.insertAdjacentHTML('beforeend', options.text); btn.title = options.title; options.container.appendChild(btn); return btn; } let attatchNavigationButtons = (options) => { let pageOptions = options.pageOptions; let table = options.table; // Create the table navigation info control div let navigationButtonSection = document.createElement('div'); navigationButtonSection.id = options.container.id + '_control_section'; options.container.appendChild(navigationButtonSection); // Add classes and data addClasses(navigationButtonSection, options.classes); addData(navigationButtonSection, options.data); let showFirstPageButton = !isNull(pageOptions.showFirstPageButton) ? pageOptions.showFirstPageButton : true; let showLastPageButton = !isNull(pageOptions.showLastPageButton) ? pageOptions.showLastPageButton : true; let showPreviousPageButton = !isNull(pageOptions.showPreviousPageButton) ? pageOptions.showPreviousPageButton : true; let showNextPageButton = !isNull(pageOptions.showNextPageButton) ? pageOptions.showNextPageButton : true; let showPageNumberButtons = !isNull(pageOptions.showPageNumberButtons) ? pageOptions.showPageNumberButtons : true; let showNavigationInput = !isNull(pageOptions.showNavigationInput) ? pageOptions.showNavigationInput : true; let onButtonTitleRender = pageOptions.onButtonTitleRender || settings.onTextRender; let onButtonTextRender = pageOptions.onButtonTextRender || settings.onTextRender; let defaultVisibleButtons = !isUndefined(pageOptions.visiblePageNumberButtons) ? pageOptions.visiblePageNumberButtons : settings.defaultVisibleButtons; if (isNumeric(defaultVisibleButtons) && defaultVisibleButtons < 1) { showPageNumberButtons = false; } // Set the total visible page number buttons setTotalVisiblePageButtons(table, defaultVisibleButtons); // Create the buttons div let navigationButtons = document.createElement('div'); navigationButtons.id = navigationButtonSection.id + '_buttons'; navigationButtonSection.appendChild(navigationButtons); // Set the current table navigation butons id setNavigationButtonsId(table, navigationButtons.id); addClasses(navigationButtons, [settings.classNameNavigationButtonSection]); // Add the first page button if (showFirstPageButton) { attachButton({ container: navigationButtons, classes: [settings.classNameButton, settings.classNameButtonFirst], data: {key: settings.dataNamePageNumber, value: settings.buttonOptionFirst}, text: onButtonTextRender.call(this, '⇠ First', settings.onRenderDescFirstPage), title: onButtonTitleRender.call(this, 'First Page', settings.onRenderDescFirstPage), }); } // Add the previous page button if (showPreviousPageButton) { attachButton({ container: navigationButtons, classes: [settings.classNameButton, settings.classNameButtonPrevious], data: {key: settings.dataNamePageNumber, value: settings.buttonOptionPrevious}, text: onButtonTextRender.call(this, '⮜', settings.onRenderDescPrevPage), title: onButtonTitleRender.call(this, 'Previous Page', settings.onRenderDescPrevPage), }); } // Add the page number buttons if (showPageNumberButtons) { let totalPages = getTotalPages(table); for (let index = 1; index <= totalPages; ++index) { attachButton({ container: navigationButtons, classes: [settings.classNameButton, settings.classNameButtonPageNumber], data: {key: settings.dataNamePageNumber, value: index}, text: onButtonTextRender.call(this, String(index), settings.onRenderDescPageNumber), title: onButtonTitleRender.call(this, 'Page ' + index, settings.onRenderDescPageNumber), }); } } // Add the next page button if (showNextPageButton) { attachButton({ container: navigationButtons, classes: [settings.classNameButton, settings.classNameButtonNext], data: {key: settings.dataNamePageNumber, value: settings.buttonOptionNext}, text: onButtonTextRender.call(this, '⮞', settings.onRenderDescNextPage), title: onButtonTitleRender.call(this, 'Next Page', settings.onRenderDescNextPage), }); } // Add the last page button if (showLastPageButton) { attachButton({ container: navigationButtons, classes: [settings.classNameButton, settings.classNameButtonLast], data: {key: settings.dataNamePageNumber, value: settings.buttonOptionLast}, text: onButtonTextRender.call(this, 'Last ⤑', settings.onRenderDescLastPage), title: onButtonTitleRender.call(this, 'Last Page', settings.onRenderDescLastPage), }); } // Add navigation input if (showNavigationInput) { attachNavigationInput({ table: table, pageOptions: pageOptions, container: navigationButtonSection, classes: [settings.classNameNavigationInputSection] }); } return navigationButtonSection; } let attachNavigationInfo = (options) => { // Create the table navigation info control div let navigationInfo = document.createElement('div'); navigationInfo.id = options.container.id + '_info_section'; options.container.appendChild(navigationInfo); // Add classes and data addClasses(navigationInfo, options.classes); addData(navigationInfo, options.data); // Set the current table navigation info id setNavigationInfoId(options.table, navigationInfo.id); return navigationInfo; } let attachNavigationInput = (options) => { let pageOptions = options.pageOptions; let onButtonTitleRender = pageOptions.onButtonTitleRender || settings.onTextRender; let onButtonTextRender = pageOptions.onButtonTextRender || settings.onTextRender; // Create the table navigation input control div let navigationInputContainer = document.createElement('div'); navigationInputContainer.id = options.container.id + '_input'; options.container.appendChild(navigationInputContainer); // Add classes and data addClasses(navigationInputContainer, options.classes); addData(navigationInputContainer, options.data); // Add the input textbox let navigationGoInput = document.createElement('input'); navigationGoInput.type = 'text'; navigationInputContainer.appendChild(navigationGoInput); // Add input classes addClasses(navigationGoInput, [settings.classNameNavigationInput]); // Add the Go button attachButton({ container: navigationInputContainer, classes: [settings.classNameButton, settings.classNameButtonGo], text: onButtonTextRender.call(this, 'Go', settings.onRenderDescGoInput), title: onButtonTitleRender.call(this, 'Go To Page', settings.onRenderDescGoInput), }); // Set the current table navigation input id setNavigationInputId(options.table, navigationInputContainer.id); return navigationInputContainer; } let addClasses = (element, classes) => { if (isNull(classes)) { return; } else if (!Array.isArray(classes)) { classes = [classes]; } classes.forEach(item => { addClass(element, item); }); } let removeClasses = (element, classes) => { if (isNull(classes)) { return; } else if (!Array.isArray(classes)) { classes = [classes]; } classes.forEach(item => { removeClass(element, item); }); } let addData = (element, data) => { if (isNull(data)) { return; } else if (!Array.isArray(data)) { data = [data]; } data.forEach(item => { if (!isNull(item.value)) { element.setAttribute(item.key, item.value); } else { removeData(element, item); } }); } let removeData = (element, data) => { if (isNull(data)) { return; } else if (!Array.isArray(data)) { data = [data]; } data.forEach(item => { let key = item.key || item; element.removeAttribute(key); }); } let getData = (element, data) => { if (isNull(data)) { return null; } else if (!Array.isArray(data)) { data = [data]; } let results = []; data.forEach(item => { let key = item.key || item; results.push(element.getAttribute(key)); }); return results.length == 1 ? results[0] : results; } let getNextMultiple = (number, multiple, skipAlreadyMultiple = false) => { let retVal = 0; if (multiple !== 0) { let remainder = (number % multiple); if (!skipAlreadyMultiple && remainder === 0) { retVal = number; } else { retVal = number + (multiple - remainder); } } return retVal; } let getPreviousMultiple = (number, multiple, skipAlreadyMultiple = false) => { return getNextMultiple(number, multiple, !skipAlreadyMultiple) - multiple; } // see if it looks and smells like an iterable object, and do accept length === 0 let isArrayLike = (item) => { return ( Array.isArray(item) || (!!item && typeof item === "object" && typeof (item.length) === "number" && (item.length === 0 || (item.length > 0 && (item.length - 1) in item) ) ) ); } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(TablePagination)); // http://programmingnotes.org/ |
The following is TablePagination.css.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 |
/* // ============================================================================ // Author: Kenneth Perkins // Date: Aug 5, 2020 // Taken From: http://programmingnotes.org/ // File: TablePagination.css // Description: CSS for the table pagination // ============================================================================ */ @import url('https://fonts.googleapis.com/css?family=Roboto'); .pagination-btn { padding: 6px 8px; font-weight: normal; font-size: 15px; border-radius: 4px; display: inline-block; text-align: center; white-space: nowrap; vertical-align: middle; touch-action: manipulation; cursor: pointer; user-select: none; text-decoration: none; transition: background-color 400ms; min-width: 10px; height: 18px; line-height: 18px; font-family: "Roboto",sans-serif, Marmelad,"Lucida Grande",Arial,"Hiragino Sans GB",Georgia,"Helvetica Neue",Helvetica; color: white; border-color: #5a3291; background-color: #064685; } .pagination-btn:focus, .pagination-btn:hover, .pagination-btn:active, .pagination-btn:active:hover, .pagination-btn:active:focus { color: white; border-color: #784cb5; background-color: #376a9d; } .pagination-btn.first { } .pagination-btn.previous { font-size: 12px; } .pagination-btn.next { font-size: 12px; } .pagination-btn.last { } .pagination-btn.page-number { min-width: 17px; } .pagination-btn.go { height: 19px; } .pagination-btn.hide { display: none; } .pagination-btn.active { color: white; background-color: #3eceff; border-color: #3eceff; } .pagination-btn.active:focus, .pagination-btn.active:hover, .pagination-btn.active:active { color: white; background-color: #00b4f0; border-color: #00b4f0; } .pagination-btn:disabled, .pagination-btn[disabled], .pagination-btn.disabled:hover, .pagination-btn[disabled]:hover, fieldset[disabled] .pagination-btn:hover, .pagination-btn.disabled:focus, .pagination-btn[disabled]:focus, fieldset[disabled] .pagination-btn:focus, .pagination-btn.disabled.focus, .pagination-btn[disabled].focus, fieldset[disabled] .pagination-btn.focus, .pagination-btn.active:disabled, .pagination-btn.active[disabled], .pagination-btn.active.disabled:hover, .pagination-btn.active[disabled]:hover, fieldset[disabled] .pagination-btn.active:hover, .pagination-btn.active.disabled:focus, .pagination-btn.active[disabled]:focus, fieldset[disabled] .pagination-btn.active:focus, .pagination-btn.active.disabled.focus, .pagination-btn.active[disabled].focus, fieldset[disabled] .pagination-btn.active.focus { color: #e4e4e4; border-color: #9d80c4; background-color: #6990b5; } .pagination-hide { display: none; } .pagination-navigation { width: 100%; box-sizing: border-box; display: flex; align-items: center; justify-content: space-between; outline: none; } .pagination-navigation.top { top: 0; } .pagination-navigation.bottom { bottom: 0; } .pagination-navigation-info-section { text-align: center; padding: 2px; box-sizing: border-box; display: inline-block; font-family: "Roboto",sans-serif, Marmelad,"Lucida Grande",Arial,"Hiragino Sans GB",Georgia,"Helvetica Neue",Helvetica; font-size: 14px; line-height: 1.42857143; color: #333; margin-right: auto; margin-left: 0; } .pagination-navigation-control-section { display: inline-block; margin-left: auto; margin-right: 0; } .pagination-navigation-button-section { display: inline-block; } .pagination-navigation-input-section { display: inline-block; margin-left: 5px; padding-top: 2px; box-sizing: border-box; } .pagination-navigation-input { height: 29px; width: 36px; font-size: 18px; background: #fff; border-radius: 3px; border: 1px solid #aaa; padding: 0; font-size: 14px; text-align: center; vertical-align: baseline; outline: 0; box-shadow: none; margin-right: 5px; } /* // http://programmingnotes.org/ */ |
14. More Examples
Below are more examples demonstrating the use of ‘TablePagination.js‘. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 609 610 611 612 613 614 615 616 617 618 619 620 621 622 623 624 625 626 627 628 629 630 631 632 633 634 635 636 637 638 639 640 641 642 643 644 645 646 647 648 649 650 651 652 653 654 655 656 657 658 659 660 661 662 663 664 665 666 667 668 669 670 671 672 673 674 675 676 677 678 679 680 681 682 683 684 685 686 687 688 689 690 691 692 693 694 695 696 697 698 699 700 701 702 703 704 705 706 707 708 709 710 711 712 713 714 715 716 717 718 719 720 721 722 723 724 725 726 727 728 729 730 731 732 733 734 735 736 737 738 739 740 741 742 743 744 745 746 747 748 749 750 751 752 753 754 755 756 757 758 759 760 761 762 763 764 765 766 767 768 769 770 771 772 773 774 775 776 777 778 779 780 781 782 783 784 785 786 787 788 789 790 791 792 793 794 795 796 797 798 799 800 801 802 803 804 805 806 807 808 809 810 811 812 813 814 815 816 817 818 819 820 821 822 823 824 825 826 827 828 829 830 831 832 833 834 835 836 837 838 839 840 841 842 843 844 845 846 847 848 849 850 851 852 853 854 855 856 857 858 859 860 861 862 863 864 865 866 867 868 869 870 871 872 873 874 875 876 877 878 879 880 881 882 883 884 885 886 887 888 889 890 891 892 893 894 895 896 897 898 899 900 901 902 903 904 905 906 907 908 909 910 911 912 913 914 915 916 917 918 919 920 921 922 923 924 925 926 927 928 929 930 931 932 933 934 935 936 937 938 939 940 941 942 943 944 945 946 947 948 949 950 951 952 953 954 955 956 957 958 959 960 961 962 963 964 965 966 967 968 969 970 971 972 973 974 975 976 977 978 979 980 981 982 983 984 985 986 987 988 989 990 991 992 993 994 995 996 997 998 999 1000 1001 1002 1003 1004 1005 1006 1007 1008 1009 1010 1011 1012 1013 1014 1015 1016 1017 1018 1019 1020 1021 1022 1023 1024 1025 1026 1027 1028 1029 1030 1031 1032 1033 1034 1035 1036 1037 1038 1039 1040 1041 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Aug 5, 2020 // Taken From: http://programmingnotes.org/ // File: tablePaginationDemo.html // Description: Demonstrates the use of TablePagination.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes TablePagination.js Demo</title> <style> .main { text-align:center; margin-left:auto; margin-right:auto; } .inline { display:inline-block; } #pagination-demo { border:1px solid #C0C0C0; border-collapse:collapse; padding:5px; margin: auto; width: 100%; } #pagination-demo th { border:1px solid #C0C0C0; padding:5px; background:#F0F0F0; width: 100px; } #pagination-demo td { border:1px solid #C0C0C0; padding:5px; text-align: center; } .tableContainer { min-height: 50px; max-height: 500px; overflow:auto; width: 100%; } #navigationSection { margin-top: 10px; } </style> <!-- // Include module --> <link type="text/css" rel="stylesheet" href="./TablePagination.css"> <script type="text/javascript" src="./TablePagination.js"></script> </head> <body> <div class="main"> My Programming Notes TablePagination.js Demo <div id="navigationSection"> <div class="inline tableContainer"> <table id="pagination-demo"> <tr> <th>First</th> <th>Last</th> <th>Age</th> </tr> <tr> <td>Kenneth</td> <td>Perkins</td> <td>31</td> </tr> <tr> <td>Jennifer</td> <td>Nguyen</td> <td>28</td> </tr> <tr> <td>Reynalda</td> <td>Bahr</td> <td>87</td> </tr> <tr> <td>Marilou</td> <td>Bower</td> <td>91</td> </tr> <tr> <td>Santina</td> <td>Rinaldi</td> <td>65</td> </tr> <tr> <td>Sherita</td> <td>Deskins</td> <td>41</td> </tr> <tr> <td>Flora</td> <td>Galusha</td> <td>52</td> </tr> <tr> <td>Yun</td> <td>Aucoin</td> <td>55</td> </tr> <tr> <td>Booker</td> <td>Freeland</td> <td>12</td> </tr> <tr> <td>Rhonda</td> <td>Fenimore</td> <td>25</td> </tr> <tr> <td>Rebecka</td> <td>Sickler</td> <td>22</td> </tr> <tr> <td>Aurora</td> <td>Coolbaugh</td> <td>24</td> </tr> <tr> <td>Jordon</td> <td>Finck</td> <td>40</td> </tr> <tr> <td>Renate</td> <td>Allred</td> <td>55</td> </tr> <tr> <td>Juana</td> <td>Rickerson</td> <td>16</td> </tr> <tr> <td>Elsie</td> <td>Hebb</td> <td>5</td> </tr> <tr> <td>Solomon</td> <td>Stookey</td> <td>42</td> </tr> <tr> <td>Collin</td> <td>Kuhlman</td> <td>17</td> </tr> <tr> <td>Siobhan</td> <td>Harville</td> <td>46</td> </tr> <tr> <td>Luigi</td> <td>Moates</td> <td>70</td> </tr> <tr> <td>Charlena</td> <td>Sebree</td> <td>24</td> </tr> <tr> <td>Fairy</td> <td>Pompa</td> <td>74</td> </tr> <tr> <td>Nita</td> <td>Yerkes</td> <td>44</td> </tr> <tr> <td>Marisa</td> <td>Caldwell</td> <td>10</td> </tr> <tr> <td>Tyrone</td> <td>Rodi</td> <td>8</td> </tr> <tr> <td>Irmgard</td> <td>Welker</td> <td>4</td> </tr> <tr> <td>Cristen</td> <td>Tank</td> <td>26</td> </tr> <tr> <td>Hildred</td> <td>Lautenschlage</td> <td>45</td> </tr> <tr> <td>Ranae</td> <td>Mccright</td> <td>45</td> </tr> <tr> <td>Myung</td> <td>Hemsley</td> <td>21</td> </tr> <tr> <td>Carlie</td> <td>Heyer</td> <td>18</td> </tr> <tr> <td>Clorinda</td> <td>Couch</td> <td>35</td> </tr> <tr> <td>Marcel</td> <td>Galentine</td> <td>39</td> </tr> <tr> <td>Zenobia</td> <td>Alford</td> <td>12</td> </tr> <tr> <td>Yuko</td> <td>Nolasco</td> <td>2</td> </tr> <tr> <td>Mertie</td> <td>Zimmer</td> <td>66</td> </tr> <tr> <td>Jeanne</td> <td>Odwyer</td> <td>90</td> </tr> <tr> <td>Suzie</td> <td>Gourley</td> <td>29</td> </tr> <tr> <td>Idella</td> <td>Gauvin</td> <td>60</td> </tr> <tr> <td>Sharyl</td> <td>Hydrick</td> <td>6</td> </tr> <tr> <td>Major</td> <td>Lacey</td> <td>1</td> </tr> <tr> <td>Mercedez</td> <td>Claiborne</td> <td>42</td> </tr> <tr> <td>Antonietta</td> <td>Zehnder</td> <td>70</td> </tr> <tr> <td>Normand</td> <td>Hittle</td> <td>63</td> </tr> <tr> <td>Benita</td> <td>Lineberry</td> <td>44</td> </tr> <tr> <td>Carroll</td> <td>Calfee</td> <td>75</td> </tr> <tr> <td>Theda</td> <td>Winters</td> <td>63</td> </tr> <tr> <td>Ella</td> <td>Hegarty</td> <td>85</td> </tr> <tr> <td>Jule</td> <td>Shanahan</td> <td>22</td> </tr> <tr> <td>Catherine</td> <td>Ver</td> <td>55</td> </tr> <tr> <td>Anabel</td> <td>Difranco</td> <td>33</td> </tr> <tr> <td>Virgen</td> <td>Ruffner</td> <td>81</td> </tr> <tr> <td>Crystle</td> <td>Buschman</td> <td>27</td> </tr> <tr> <td>Alyse</td> <td>Happel</td> <td>68</td> </tr> <tr> <td>Sharla</td> <td>Hammes</td> <td>5</td> </tr> <tr> <td>Eliana</td> <td>Sippel</td> <td>33</td> </tr> <tr> <td>Elise</td> <td>Hoxie</td> <td>82</td> </tr> <tr> <td>Keshia</td> <td>Arceo</td> <td>11</td> </tr> <tr> <td>Leslee</td> <td>Jablonski</td> <td>22</td> </tr> <tr> <td>Marcos</td> <td>Chien</td> <td>18</td> </tr> <tr> <td>Rosalyn</td> <td>Dahl</td> <td>37</td> </tr> <tr> <td>Elmo</td> <td>Mcglade</td> <td>46</td> </tr> <tr> <td>Bernita</td> <td>Mcdavis</td> <td>85</td> </tr> <tr> <td>Myung</td> <td>Marquardt</td> <td>77</td> </tr> <tr> <td>Kalyn</td> <td>Lichtenberger</td> <td>88</td> </tr> <tr> <td>Sophia</td> <td>Warrior</td> <td>99</td> </tr> <tr> <td>Carri</td> <td>Basile</td> <td>10</td> </tr> <tr> <td>Daria</td> <td>Patridge</td> <td>44</td> </tr> <tr> <td>Kory</td> <td>Eifert</td> <td>63</td> </tr> <tr> <td>Ahmed</td> <td>Vore</td> <td>71</td> </tr> <tr> <td>Erlinda</td> <td>Dias</td> <td>36</td> </tr> <tr> <td>Magdalene</td> <td>Hokanson</td> <td>56</td> </tr> <tr> <td>Loren</td> <td>Haun</td> <td>84</td> </tr> <tr> <td>Arlie</td> <td>Garren</td> <td>32</td> </tr> <tr> <td>Fernande</td> <td>Styron</td> <td>63</td> </tr> <tr> <td>Lizabeth</td> <td>Richerson</td> <td>75</td> </tr> <tr> <td>Jessica</td> <td>Ferrara</td> <td>52</td> </tr> <tr> <td>Shin</td> <td>Philson</td> <td>64</td> </tr> <tr> <td>Jackelyn</td> <td>Lafayette</td> <td>2</td> </tr> <tr> <td>Tambra</td> <td>Pantano</td> <td>7</td> </tr> <tr> <td>Tomiko</td> <td>Nicols</td> <td>6</td> </tr> <tr> <td>Cordelia</td> <td>Mask</td> <td>55</td> </tr> <tr> <td>Shellie</td> <td>Duffer</td> <td>10</td> </tr> <tr> <td>Melina</td> <td>Fluitt</td> <td>19</td> </tr> </table> </div> </div> </div> <div id="binding-section"> Custom Binding Section </div> <table id="test" cellspacing="0" style="width: 100%; border: 1px solid black; text-align: left;"> <thead> <tr> <th class="th-sm">Name </th> <th class="th-sm">Position </th> <th class="th-sm">Office </th> <th class="th-sm">Age </th> <th class="th-sm">Start date </th> <th class="th-sm">Salary </th> </tr> </thead> <tbody> <tr> <td>Tiger Nixon</td> <td>System Architect</td> <td>Edinburgh</td> <td>61</td> <td>2011/04/25</td> <td>$320,800</td> </tr> <tr> <td>Garrett Winters</td> <td>Accountant</td> <td>Tokyo</td> <td>63</td> <td>2011/07/25</td> <td>$170,750</td> </tr> <tr> <td>Ashton Cox</td> <td>Junior Technical Author</td> <td>San Francisco</td> <td>66</td> <td>2009/01/12</td> <td>$86,000</td> </tr> <tr> <td>Cedric Kelly</td> <td>Senior Javascript Developer</td> <td>Edinburgh</td> <td>22</td> <td>2012/03/29</td> <td>$433,060</td> </tr> <tr> <td>Airi Satou</td> <td>Accountant</td> <td>Tokyo</td> <td>33</td> <td>2008/11/28</td> <td>$162,700</td> </tr> <tr> <td>Brielle Williamson</td> <td>Integration Specialist</td> <td>New York</td> <td>61</td> <td>2012/12/02</td> <td>$372,000</td> </tr> <tr> <td>Herrod Chandler</td> <td>Sales Assistant</td> <td>San Francisco</td> <td>59</td> <td>2012/08/06</td> <td>$137,500</td> </tr> <tr> <td>Rhona Davidson</td> <td>Integration Specialist</td> <td>Tokyo</td> <td>55</td> <td>2010/10/14</td> <td>$327,900</td> </tr> <tr> <td>Colleen Hurst</td> <td>Javascript Developer</td> <td>San Francisco</td> <td>39</td> <td>2009/09/15</td> <td>$205,500</td> </tr> <tr> <td>Sonya Frost</td> <td>Software Engineer</td> <td>Edinburgh</td> <td>23</td> <td>2008/12/13</td> <td>$103,600</td> </tr> <tr> <td>Jena Gaines</td> <td>Office Manager</td> <td>London</td> <td>30</td> <td>2008/12/19</td> <td>$90,560</td> </tr> <tr> <td>Quinn Flynn</td> <td>Support Lead</td> <td>Edinburgh</td> <td>22</td> <td>2013/03/03</td> <td>$342,000</td> </tr> <tr> <td>Charde Marshall</td> <td>Regional Director</td> <td>San Francisco</td> <td>36</td> <td>2008/10/16</td> <td>$470,600</td> </tr> <tr> <td>Haley Kennedy</td> <td>Senior Marketing Designer</td> <td>London</td> <td>43</td> <td>2012/12/18</td> <td>$313,500</td> </tr> <tr> <td>Tatyana Fitzpatrick</td> <td>Regional Director</td> <td>London</td> <td>19</td> <td>2010/03/17</td> <td>$385,750</td> </tr> <tr> <td>Michael Silva</td> <td>Marketing Designer</td> <td>London</td> <td>66</td> <td>2012/11/27</td> <td>$198,500</td> </tr> <tr> <td>Paul Byrd</td> <td>Chief Financial Officer (CFO)</td> <td>New York</td> <td>64</td> <td>2010/06/09</td> <td>$725,000</td> </tr> <tr> <td>Gloria Little</td> <td>Systems Administrator</td> <td>New York</td> <td>59</td> <td>2009/04/10</td> <td>$237,500</td> </tr> <tr> <td>Bradley Greer</td> <td>Software Engineer</td> <td>London</td> <td>41</td> <td>2012/10/13</td> <td>$132,000</td> </tr> <tr> <td>Dai Rios</td> <td>Personnel Lead</td> <td>Edinburgh</td> <td>35</td> <td>2012/09/26</td> <td>$217,500</td> </tr> <tr> <td>Jenette Caldwell</td> <td>Development Lead</td> <td>New York</td> <td>30</td> <td>2011/09/03</td> <td>$345,000</td> </tr> <tr> <td>Yuri Berry</td> <td>Chief Marketing Officer (CMO)</td> <td>New York</td> <td>40</td> <td>2009/06/25</td> <td>$675,000</td> </tr> <tr> <td>Caesar Vance</td> <td>Pre-Sales Support</td> <td>New York</td> <td>21</td> <td>2011/12/12</td> <td>$106,450</td> </tr> <tr> <td>Doris Wilder</td> <td>Sales Assistant</td> <td>Sidney</td> <td>23</td> <td>2010/09/20</td> <td>$85,600</td> </tr> <tr> <td>Angelica Ramos</td> <td>Chief Executive Officer (CEO)</td> <td>London</td> <td>47</td> <td>2009/10/09</td> <td>$1,200,000</td> </tr> <tr> <td>Gavin Joyce</td> <td>Developer</td> <td>Edinburgh</td> <td>42</td> <td>2010/12/22</td> <td>$92,575</td> </tr> <tr> <td>Jennifer Chang</td> <td>Regional Director</td> <td>Singapore</td> <td>28</td> <td>2010/11/14</td> <td>$357,650</td> </tr> <tr> <td>Brenden Wagner</td> <td>Software Engineer</td> <td>San Francisco</td> <td>28</td> <td>2011/06/07</td> <td>$206,850</td> </tr> <tr> <td>Fiona Green</td> <td>Chief Operating Officer (COO)</td> <td>San Francisco</td> <td>48</td> <td>2010/03/11</td> <td>$850,000</td> </tr> <tr> <td>Shou Itou</td> <td>Regional Marketing</td> <td>Tokyo</td> <td>20</td> <td>2011/08/14</td> <td>$163,000</td> </tr> <tr> <td>Michelle House</td> <td>Integration Specialist</td> <td>Sidney</td> <td>37</td> <td>2011/06/02</td> <td>$95,400</td> </tr> <tr> <td>Suki Burks</td> <td>Developer</td> <td>London</td> <td>53</td> <td>2009/10/22</td> <td>$114,500</td> </tr> <tr> <td>Prescott Bartlett</td> <td>Technical Author</td> <td>London</td> <td>27</td> <td>2011/05/07</td> <td>$145,000</td> </tr> <tr> <td>Gavin Cortez</td> <td>Team Leader</td> <td>San Francisco</td> <td>22</td> <td>2008/10/26</td> <td>$235,500</td> </tr> <tr> <td>Martena Mccray</td> <td>Post-Sales support</td> <td>Edinburgh</td> <td>46</td> <td>2011/03/09</td> <td>$324,050</td> </tr> <tr> <td>Unity Butler</td> <td>Marketing Designer</td> <td>San Francisco</td> <td>47</td> <td>2009/12/09</td> <td>$85,675</td> </tr> <tr> <td>Howard Hatfield</td> <td>Office Manager</td> <td>San Francisco</td> <td>51</td> <td>2008/12/16</td> <td>$164,500</td> </tr> <tr> <td>Hope Fuentes</td> <td>Secretary</td> <td>San Francisco</td> <td>41</td> <td>2010/02/12</td> <td>$109,850</td> </tr> <tr> <td>Vivian Harrell</td> <td>Financial Controller</td> <td>San Francisco</td> <td>62</td> <td>2009/02/14</td> <td>$452,500</td> </tr> <tr> <td>Timothy Mooney</td> <td>Office Manager</td> <td>London</td> <td>37</td> <td>2008/12/11</td> <td>$136,200</td> </tr> <tr> <td>Jackson Bradshaw</td> <td>Director</td> <td>New York</td> <td>65</td> <td>2008/09/26</td> <td>$645,750</td> </tr> <tr> <td>Olivia Liang</td> <td>Support Engineer</td> <td>Singapore</td> <td>64</td> <td>2011/02/03</td> <td>$234,500</td> </tr> <tr> <td>Bruno Nash</td> <td>Software Engineer</td> <td>London</td> <td>38</td> <td>2011/05/03</td> <td>$163,500</td> </tr> <tr> <td>Sakura Yamamoto</td> <td>Support Engineer</td> <td>Tokyo</td> <td>37</td> <td>2009/08/19</td> <td>$139,575</td> </tr> <tr> <td>Thor Walton</td> <td>Developer</td> <td>New York</td> <td>61</td> <td>2013/08/11</td> <td>$98,540</td> </tr> <tr> <td>Finn Camacho</td> <td>Support Engineer</td> <td>San Francisco</td> <td>47</td> <td>2009/07/07</td> <td>$87,500</td> </tr> <tr> <td>Serge Baldwin</td> <td>Data Coordinator</td> <td>Singapore</td> <td>64</td> <td>2012/04/09</td> <td>$138,575</td> </tr> <tr> <td>Zenaida Frank</td> <td>Software Engineer</td> <td>New York</td> <td>63</td> <td>2010/01/04</td> <td>$125,250</td> </tr> <tr> <td>Zorita Serrano</td> <td>Software Engineer</td> <td>San Francisco</td> <td>56</td> <td>2012/06/01</td> <td>$115,000</td> </tr> <tr> <td>Jennifer Acosta</td> <td>Junior Javascript Developer</td> <td>Edinburgh</td> <td>43</td> <td>2013/02/01</td> <td>$75,650</td> </tr> <tr> <td>Cara Stevens</td> <td>Sales Assistant</td> <td>New York</td> <td>46</td> <td>2011/12/06</td> <td>$145,600</td> </tr> <tr> <td>Hermione Butler</td> <td>Regional Director</td> <td>London</td> <td>47</td> <td>2011/03/21</td> <td>$356,250</td> </tr> <tr> <td>Lael Greer</td> <td>Systems Administrator</td> <td>London</td> <td>21</td> <td>2009/02/27</td> <td>$103,500</td> </tr> <tr> <td>Jonas Alexander</td> <td>Developer</td> <td>San Francisco</td> <td>30</td> <td>2010/07/14</td> <td>$86,500</td> </tr> <tr> <td>Shad Decker</td> <td>Regional Director</td> <td>Edinburgh</td> <td>51</td> <td>2008/11/13</td> <td>$183,000</td> </tr> <tr> <td>Michael Bruce</td> <td>Javascript Developer</td> <td>Singapore</td> <td>29</td> <td>2011/06/27</td> <td>$183,000</td> </tr> <tr> <td>Donna Snider</td> <td>Customer Support</td> <td>New York</td> <td>27</td> <td>2011/01/25</td> <td>$112,000</td> </tr> </tbody> <tfoot> <tr> <th>Name </th> <th>Position </th> <th>Office </th> <th>Age </th> <th>Start date </th> <th>Salary </th> </tr> </tfoot> </table> <script> document.addEventListener("DOMContentLoaded", function(eventLoaded) { // Available options TablePagination.page({ table: document.querySelector('#pagination-demo'), // One or more Javascript elements of the tables to page. rowsPerPage: 4, // Optional. The number of rows per page. Default is 5 initialPage: null, // Optional. The initial page to display. Possible values: Numeric value or 'first/last'. Default is page 1 navigationPosition: 'bottom', // Optional. The navigation position. Possible values: 'top/bottom'. Default is 'bottom' showFirstPageButton: true, // Optional. Specifies if the first page button is shown. Default is true showLastPageButton: true, // Optional. Specifies if the last page button is shown. Default is true showPreviousPageButton: true, // Optional. Specifies if the previous page button is shown. Default is true showNextPageButton: true, // Optional. Specifies if the next page button is shown. Default is true showPageNumberButtons: true, // Optional. Specifies if the page number buttons are shown. Default is true showNavigationInput: true, // Optional. Specifies if the 'Go' search functionality is shown. Default is true showNavigationInfoText: true, // Optional. Specifies if the navigation info text is shown. Default is true visiblePageNumberButtons: 4, // Optional. The maximum number of visible page number buttons. Default is 3. Set to null to show all buttons onButtonClick: (pageNumber, event) => { // Optional. Function that allows to do something on button click //window.location.href = "#page=" + pageNumber; }, onButtonTextRender: (text, desc) => { // Optional. Function that allows to format the button text //console.log(`Button Text: ${text}`); //console.log(`Button Description: ${desc}`); return text; }, onButtonTitleRender: (title, desc) => { // Optional. Function that allows to format the button title //console.log(`Button Text: ${text}`); //console.log(`Button Description: ${desc}`); return title; }, onNavigationInfoTextRender: (text, rowInfo) => { // Optional. Function that allows to format the navigation info text //console.log(`Navigation Text: ${text}`); //console.log(`Row Info:`, rowInfo); return text; }, navigationBindTo: null, // Optional. Javascript element of the container where the navigation controls are bound to. // If not specified, default destination is above or below the table element, depending on // the 'navigationPosition' value }); //TablePagination.remove(document.querySelector('#pagination-demo')); TablePagination.page({table: document.querySelector('#test'), rowsPerPage: 10}); }); </script> </body> </html><!-- // http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.