JavaScript || How To Scroll To An Element & Add Automatic Anchor Scroll Using Vanilla JavaScript
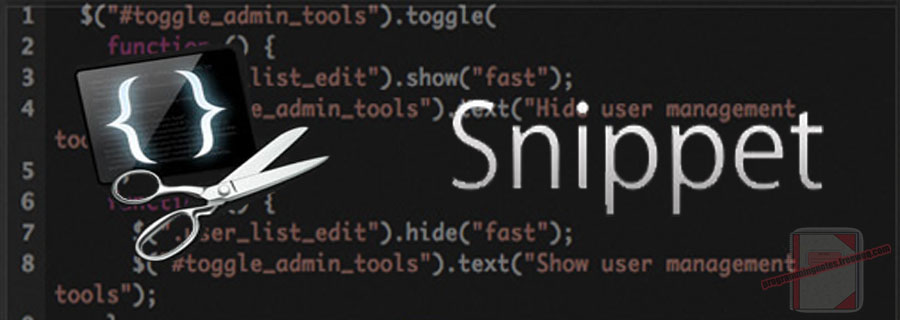
The following is a module with functions that demonstrates how to scroll to a specific HTML element, as well as setting anchors to have a smooth scroll effect on button click.
1. Scroll To Element
The example below demonstrates how to scroll to a specific element.
1 2 3 4 5 6 7 |
// Scroll to a specific element <script> (() => { Utils.scrollToElement("#examples"); })(); </script> |
The ‘Utils.scrollToElement‘ function accepts either a JavaScript element or a string selector of the element to scroll to.
2. Add Scroll To Anchor Target
The example below demonstrates how to automatically add a scrolling effect to an anchor target.
1 2 3 4 5 6 7 |
// Scroll to anchor target <script> (() => { Utils.registerAnchorScroll(); })(); </script> |
The ‘Utils.registerAnchorScroll‘ function gets all the anchor links on a page and registers smooth scrolling on button click using ‘Utils.scrollToElement‘.
3. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 7, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: registerAnchorScroll * USE: Gets all the anchor links on a page and registers smooth scrolling * on button click. * @return: N/A. */ exposed.registerAnchorScroll = () => { let anchorLinks = document.querySelectorAll('a[href^="#"]'); for (let link of anchorLinks) { link.addEventListener('click', (e) => { let hashval = link.getAttribute('href'); let target = document.querySelector(hashval) || document.querySelector(`[name='${hashval.slice(1)}']`); if (target) { exposed.scrollToElement(target); history.pushState(null, null, hashval); e.preventDefault(); } }); } } /** * FUNCTION: scrollToElement * USE: Scrolls the page to the given element. * @param target: A JavaScript element or a string selector of the * element to scroll to. * @param behavior: The scroll behavior. Defaut is 'smooth' * @return: N/A. */ exposed.scrollToElement = (target, behavior = 'smooth') => { if (typeof target === 'string') { target = document.querySelector(target); } target.scrollIntoView({ behavior: behavior, block: 'start' }); } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of ‘Utils.js‘. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Aug 7, 2020 // Taken From: http://programmingnotes.org/ // File: scrollDemo.html // Description: Demonstrates the use of Utils.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes Utils.js Demo</title> <style> .space {margin-top: 1000px;} </style> <!-- // Include module --> <script type="text/javascript" src="./Utils.js"></script> </head> <body> <blockquote><code> <a href="#basicUsage">1. Basic Usage</a> <a href="#examples">2. Examples</a> </code></blockquote> <div class="space"></div> <h3 id="basicUsage"> 1. Basic Usage </h3> <div class="space"></div> <h3 id="examples"> 2. Examples </h3> <div class="space"></div> <script> document.addEventListener("DOMContentLoaded", function(eventLoaded) { Utils.registerAnchorScroll(); //Utils.scrollToElement("#examples"); }); </script> </body> </html> <!-- http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply