Monthly Archives: November 2022
C# || How To Design A Time Based Key-Value Store To Retrieve Timestamps Using C#
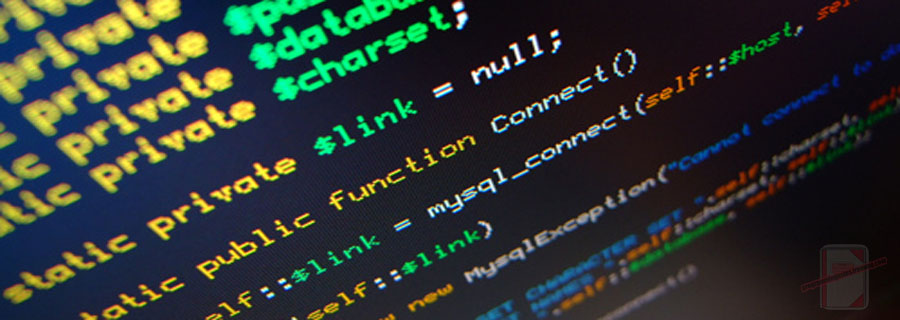
The following is a module with functions which demonstrates how to design a time based key-value store to retrieve timestamps using C#.
1. Time Map – Problem Statement
Design a time-based key-value data structure that can store multiple values for the same key at different time stamps and retrieve the key’s value at a certain timestamp.
Implement the TimeMap class:
- TimeMap() Initializes the object of the data structure.
- void set(String key, String value, int timestamp) Stores the key key with the value value at the given time timestamp.
- String get(String key, int timestamp) Returns a value such that set was called previously, with timestamp_prev <= timestamp. If there are multiple such values, it returns the value associated with the largest timestamp_prev. If there are no values, it returns “”.
Example 1:
Input
["TimeMap", "set", "get", "get", "set", "get", "get"]
[[], ["foo", "bar", 1], ["foo", 1], ["foo", 3], ["foo", "bar2", 4], ["foo", 4], ["foo", 5]]
Output
[null, null, "bar", "bar", null, "bar2", "bar2"]Explanation
TimeMap timeMap = new TimeMap();
timeMap.set("foo", "bar", 1); // store the key "foo" and value "bar" along with timestamp = 1.
timeMap.get("foo", 1); // return "bar"
timeMap.get("foo", 3); // return "bar", since there is no value corresponding to foo at timestamp 3 and timestamp 2, then the only value is at timestamp 1 is "bar".
timeMap.set("foo", "bar2", 4); // store the key "foo" and value "bar2" along with timestamp = 4.
timeMap.get("foo", 4); // return "bar2"
timeMap.get("foo", 5); // return "bar2"
2. Time Map – Solution
The following is a solution which demonstrates how to design a time based key-value store to retrieve timestamps.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
// ============================================================================ // Author: Kenneth Perkins // Date: Nov 1, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to design a time map // ============================================================================ /** * Your TimeMap object will be instantiated and called as such: * TimeMap obj = new TimeMap(); * obj.Set(key,value,timestamp); * string param_2 = obj.Get(key,timestamp); */ public class TimeMap { private class Data { public string value; public int timestamp; public Data(string value, int timestamp) { this.value = value; this.timestamp = timestamp; } } private Dictionary<string, List<Data>> map; public TimeMap() { map = new Dictionary<string, List<Data>>(); } public void Set(string key, string value, int timestamp) { if (!map.ContainsKey(key)) { map.Add(key, new List<Data>()); } map[key].Add(new Data(value, timestamp)); } public string Get(string key, int timestamp) { if (!map.ContainsKey(key)) { return ""; } return BinarySearch(map[key], timestamp); } string BinarySearch(List<Data> bucket, int target) { int low = 0, high = bucket.Count; while (low < high) { int mid = low + (high - low) / 2; if (bucket[mid].timestamp <= target) { low = mid + 1; } else { high = mid; } } if (high == 0) { return ""; } return (bucket[high - 1].timestamp <= target) ? bucket[high - 1].value : ""; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[null,null,"bar","bar",null,"bar2","bar2"]