C++ || Searching An Integer Array For A Target Value
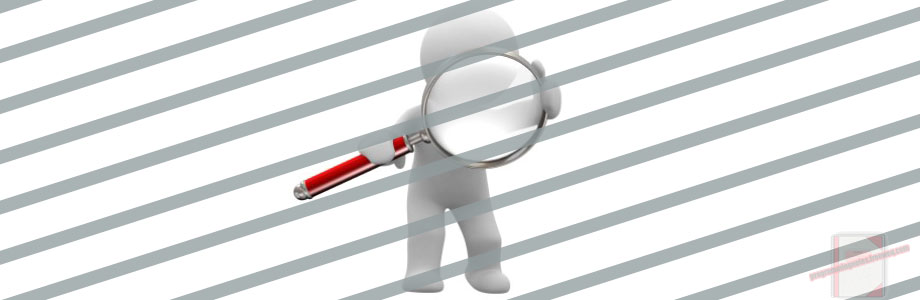
Here is another actual homework assignment which was presented in an intro to programming class.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
This is a small and simple program which demonstrates how to search for a target value that is stored in an integer array. This program prompts the user to enter five values into an int array. After the user has entered all the values, it displays a prompt asking the user for a search value. Once it has the search value, the program will search through the array looking for the target; wherever the value is found, it will display the index location. After it displays all the locations where the value is found, it will display the total number of occurrences the search value was found within the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // File: ArraySearch.cpp // ============================================================================ // // Description: // This program allows the user to populate an array of integers with five // values. After the user has entered all the values, it displays a prompt // asking the user for a search value. Once it has the search value, the // program will search through the array looking for the target; wherever the // value is found, it will display the index location. After it displays all // the locations where the value is found, it will display the total number // of occurrences the search value was found within the array. // // ============================================================================ #include <iostream> using namespace std; // constant variable const int NUM_INTS = 5; // function prototypes int SearchArray(int numValues[], int searchValue); // ==== main ================================================================== // // ============================================================================ int main() { // declare variables int searchValue; int numOccurences = 0; int numValues[NUM_INTS]; // int array size is initialized with a const value // get data from user via a 'for loop' cout << "Please enter "<< NUM_INTS <<" integer values:nn"; for (int index=0; index < NUM_INTS; ++index) { cout << "#" << index + 1 << ": "; cin >> numValues[index]; cout <<endl; } // get a search value from the user cout << "Please enter a search value: "; cin >> searchValue; cout <<endl; // finds the number of occurences the search value was found in the array numOccurences = SearchArray(numValues, searchValue); // display data to user cout << "nThe total occurrences of value " << searchValue << " within the array is: " << numOccurences; cout << endl << endl; return 0; }// end of main // ==== SearchArray =========================================================== // // This function will take as input the array, the number of array // elements, and the target value to search for. The function will traverse // the array looking for the target value, and when it finds it, display the // index location within the array. // // Input: // limit [IN] -- the array, the number of array // elements, and the target value // // Output: // The total number of occurrences of the target in the array // // ============================================================================ int SearchArray(int numValues[], int searchValue) { int numFound=0; for (int index=0; index < NUM_INTS; ++index) { if (numValues[index] == searchValue) { cout << "t" << searchValue << " was found at array index #" << index << endl; ++numFound; // if the search values was found, // increment the variable by 1 } } return numFound; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 3 separate times to display the different outputs its able to produce
Please enter 5 integer values:
#1: 12
#2: 12
#3: 12
#4: 12
#5: 12
Please enter a search value: 1212 was found at array index #0
12 was found at array index #1
12 was found at array index #2
12 was found at array index #3
12 was found at array index #4The total occurrences of value 12 within the array is: 5
-------------------------------------------------------------------------Please enter 5 integer values:
#1: 12
#2: 87
#3: 45
#4: 87
#5: 33
Please enter a search value: 8787 was found at array index #1
87 was found at array index #3The total occurrences of value 87 within the array is: 2
-------------------------------------------------------------------------Please enter 5 interger values:
#1: 54
#2: 67
#3: 98
#4: 45
#5: 98
Please enter a search value: 123The total occurrences of value 123 within the array is: 0
Leave a Reply