Tag Archives: Total
Java || Searching An Integer Array For A Target Value
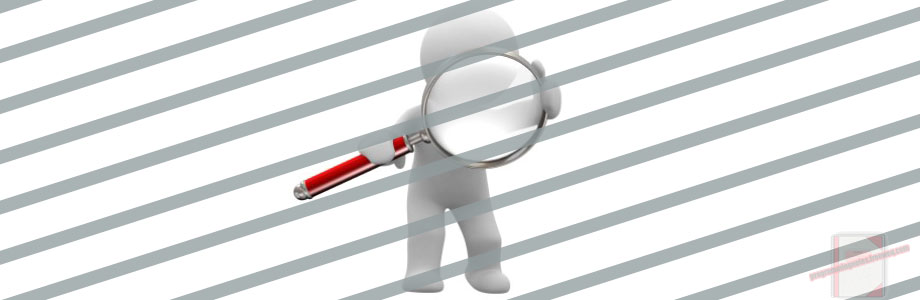
Here is another actual homework assignment which was presented in an intro to programming class which was used to introduce more practice using integer arrays.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Integer Arrays
For Loops
Methods (A.K.A "Functions") - What Are They?
Final Variables
If/Else Statements
This is a small and simple program which demonstrates how to search for a target value which is stored in an integer array. This program first prompts the user to enter five values into an int array. After the user enters all the values into the system, it then displays a prompt asking the user for a search value. Once it has a search value, the program searches through the array looking for the target value; and wherever the value is found, the program display’s the current array index in which that target value is located. After it displays all the locations where the target value resides, it display’s the total number of occurrences the search value was found within the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
import java.util.Scanner; public class ArraySearch { // global variable declaration static Scanner cin = new Scanner(System.in); static final int NUM_INTS = 5; public static void main(String[] args) { // declare variables int searchValue = 0; int numOccurences = 0; int[] numValues = new int[NUM_INTS]; // int array size is initialized with a const value // display message to screen System.out.println("Welcome to My Programming Notes' Java Program.n"); // get data from user via a 'for loop' System.out.println("Please enter "+ NUM_INTS +" integer values:"); for (int index=0; index < NUM_INTS; ++index) { System.out.print("#" + (index + 1) + ": "); numValues[index] = cin.nextInt(); } // get a search value from the user System.out.print("Please enter a search value: "); searchValue = cin.nextInt(); System.out.println(""); // finds the number of occurences the search value was found in the array numOccurences = SearchArray(numValues, searchValue); // display data to user System.out.println("nThe total occurrences of value "+ searchValue + " within the array is: " + numOccurences); }// end of main // ==== SearchArray =========================================================== // // This method will take as input the array, the number of array // elements, and the target value to search for. The function will traverse // the array looking for the target value, and when it finds it, display the // index location within the array. // // Input: // limit [IN] -- the array, the number of array // elements, and the target value // // Output: // The total number of occurrences of the target value in the array // // ============================================================================ public static int SearchArray(int[] numValues, int searchValue) { int numFound=0; for (int index=0; index < NUM_INTS; ++index) { if (numValues[index] == searchValue) { System.out.println("t" + searchValue + " was found at array " + "index #" + index); ++numFound; // if the search values was found, // increment the variable by 1 } } return numFound; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 3 separate times to display the different outputs its able to produce
====== RUN 1 ======
Welcome to My Programming Notes' Java Program.
Please enter 5 integer values:
#1: 25
#2: 25
#3: 25
#4: 25
#5: 25
Please enter a search value: 2525 was found at array index #0
25 was found at array index #1
25 was found at array index #2
25 was found at array index #3
25 was found at array index #4The total occurrences of value 25 within the array is: 5
====== RUN 2 ======
Welcome to My Programming Notes' Java Program.
Please enter 5 integer values:
#1: 8
#2: 19
#3: 97
#4: 56
#5: 8
Please enter a search value: 88 was found at array index #0
8 was found at array index #4The total occurrences of value 8 within the array is: 2
====== RUN 3 ======
Welcome to My Programming Notes' Java Program.
Please enter 5 integer values:
#1: 78
#2: 65
#3: 3
#4: 45
#5: 89
Please enter a search value: 12The total occurrences of value 12 within the array is: 0
C++ || Input/Output – Find The Average of The Numbers Contained In a Text File Using an Array/Bubble Sort
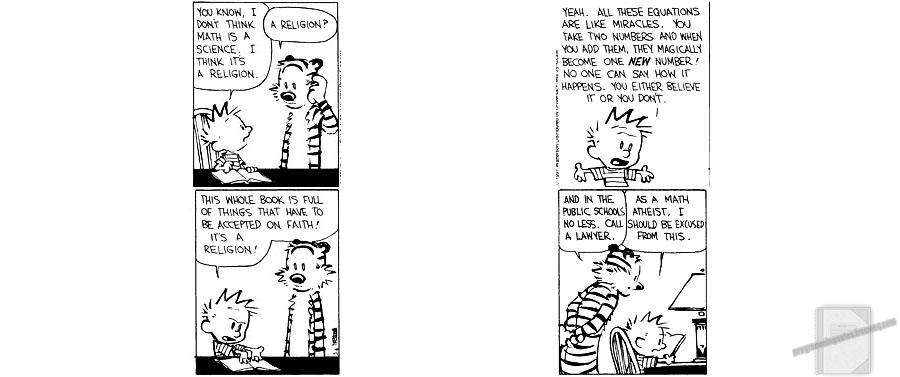
This program highlights more practice using text files and arrays. This program is very similar to one which was previously discussed on this site, but unlike that program, this implementation omits the highest/lowest values which are found within the array via a sort.
The previously discussed program works almost as well as the current implementation, but where it fails is when the data which is being entered into the program contains multiple values of the same type. For example, using the previously discussed method to obtain the average by omitting the highest/lowest entries found within an array, if the array contained the numbers:
1, 2, 3, 3, 3, 2, 2, 1
The previous implementation would mark 1 as being the lowest number (which is correct) and it would mark 3 as being the highest number (which is also correct). The area where it fails is when it omits the highest and lowest scores found within the array. The program will skip over ALL of the numbers contained within the array which equal to 1 and 3, thus resulting in the program obtaining the wrong answer.
To illustrate, before the previous program computes its adjusted average scores, it will not only omit just 1 and 3 from the array, but it will also omit all of the 1’s and 3’s from the list, resulting in our array looking like this:
2, 2, 2
When you are finding the average of a list of numbers by omitting the highest/lowest scores, you don’t want to omit ALL of the values which may equal said numbers, but merely just the highest (last element in the array) and lowest (first element in the array) scores.
So if the previous implementation has subtle issues, why is it on this site? The previous program illustrates very well the process of finding the highest/lowest integers found within an array. It also works flawlessly for data in which there is non repeating values found within a list (i.e 1,2,3,4,5,23,6). So if you know you are reading in from a file in which there are non repeating values, the previous implementation works well. Often times though, developers do not know what type of data the incoming files will contain, so this current implementation is a better way to go, especially if it is not known exactly how many numbers are contained within a file.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Fstream
Ifstream
Ofstream
Working With Files
While Loops
For Loops
Bubble Sort
Basic Math - Finding The Average
The data file that is used in this example can be downloaded here.
Note: In order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 |
#include <iostream> #include <fstream> using namespace std; // function prototype float FindOmittedAverage(int numbers[], int counter); int main() { // declare variables ifstream infile; ofstream outfile; float average=0; float sum =0; int highestNumber = -999999; int lowestNumber = 99999999; int numbers[100]; // open the input file in which you will read data from infile.open("INPUT_Numbers_ programmingnotes_freeweq_com.txt"); if(infile.fail()) { cout<<"nInput file not found!n"; exit(1); // there was an error, program exits } // open the output file in which the compiled data will be saved to outfile.open("OUTPUT_Averages_ programmingnotes_freeweq_com.txt"); if(outfile.fail()) { cout <<"There was an error creating the output file, press enter to terminate program."; exit(1); // there was an error, program exits } // display data to screen via cout cout <<"The numbers countained in the input file are: "; // saves data to the outfile. Notice the declaration to // save data to the outfile is the same as the ^ above cout // statment outfile <<"The numbers countained in the input file are: "; // incoming data from the file will be stored in an int array // so this counter will increment the array index every time the // program finds a new value inside the file to store inside the array int counter =0; // this while loop will read in data from the file until it reaches the end of the file // (i.e until there are no more number found within the file) // this process also stored the numbers inside the "numbers" array while(infile >> numbers[counter]) { // 'sum' variable adds the incoming numbers together // computing the sum sum += numbers[counter]; // finds the highest number contained within the incoming file if( numbers[counter] > highestNumber) { highestNumber = numbers[counter]; } // finds the lowest number contained within the incoming file if (numbers[counter] < lowestNumber) { lowestNumber = numbers[counter]; } // displays the currently found number from the file to stdout cout << numbers[counter] << ", "; // saves the currently found number from the file to the output file outfile << numbers[counter]<< ", "; ++counter; } // always close your file after your done using them infile.close(); // display the highest found number to stdout cout<< "nnThe highest and lowest numbers contained in the file are: nHighest: " << highestNumber<<"nLowest: "<<lowestNumber<<endl; // saves the highest found number to the output file outfile<< "nnThe highest and lowest numbers contained in the file are: nHighest: " << highestNumber<<"nLowest: "<<lowestNumber<<endl; // using the sum found from the above while loop, we compute the average average = sum/(counter); // display/save the average of the numbers which were contained in the // file to stdout and the output file cout<<"nThe average of the "<<counter<<" numbers contained in the file is: "<<average<<endl; outfile<<"nThe average of the "<<counter<<" numbers contained in the file is: "<<average<<endl; // function declaration which finds the omitted average of the found numbers // from the file average = FindOmittedAverage(numbers,counter); // display/save the omitted average of the numbers which were contained in the // file to stdout and the output file cout<<"nThe average of the "<<counter<<" numbers contained in the file omitting the highest and lowest scores is: "<<average<<endl; outfile<<"nThe average of the "<<counter<<" numbers contained in the file omitting the highest and lowest scores is: "<<average<<endl; // closes the outfile once we are done using it outfile.close(); return 0; } // function takes in the 'numbers' array, and 'counter' variable from // the main function as parameters, and computes the average, omitting // the highest and lowest numbers found within the array float FindOmittedAverage(int numbers[], int counter) { float sum = 0; float avg = 0; // this is a 'bubble sort' which will sort the numbers // contained within the array, from lowest to the highest // i.e (1,2,3,4,5,6,7,8) for(int iteration = 1; iteration < counter; iteration++) { for(int index = 0; index < counter - iteration; index++) { // if the previous value in the array is bigger than the next, then swap them if(numbers[index]> numbers[index+1]) { int temp = numbers[index]; numbers[index] = numbers[index+1]; numbers[index+1]= temp; } } }// end of sort // after the sorting is complete, set the highest // and lowest elements in the array to zero numbers[0]=0; numbers[counter-1]=0; // find the sum of the newly sorted array // with the highest and lowest entries being deleted (set to zero) for(int i = 0; i < counter; i++) { sum += numbers[i]; } // compute the average avg = sum / (counter-2); // return the average back to main return avg; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Remember to include the input file)
The numbers countained in the input file are: 12, 45, 23, 46, 11, -5, 23, 33, 50, 17, 13, 25, 15, 50,
The highest and lowest numbers contained in the file are:
Highest: 50
Lowest: -5The average of the 14 numbers contained in the file is: 25.5714
The average of the 14 numbers contained in the file omitting the highest and lowest scores is: 26.0833
C++ || Searching An Integer Array For A Target Value
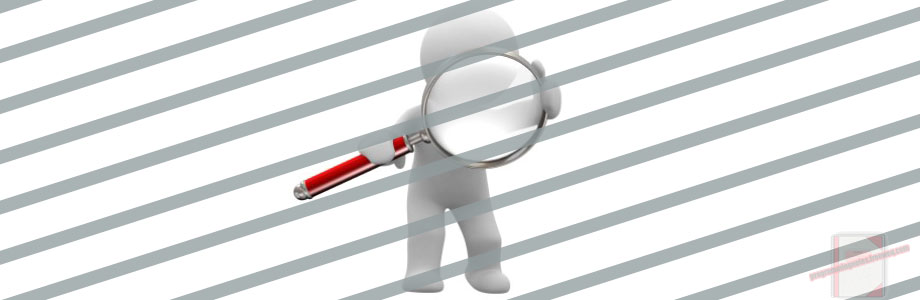
Here is another actual homework assignment which was presented in an intro to programming class.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
This is a small and simple program which demonstrates how to search for a target value that is stored in an integer array. This program prompts the user to enter five values into an int array. After the user has entered all the values, it displays a prompt asking the user for a search value. Once it has the search value, the program will search through the array looking for the target; wherever the value is found, it will display the index location. After it displays all the locations where the value is found, it will display the total number of occurrences the search value was found within the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // File: ArraySearch.cpp // ============================================================================ // // Description: // This program allows the user to populate an array of integers with five // values. After the user has entered all the values, it displays a prompt // asking the user for a search value. Once it has the search value, the // program will search through the array looking for the target; wherever the // value is found, it will display the index location. After it displays all // the locations where the value is found, it will display the total number // of occurrences the search value was found within the array. // // ============================================================================ #include <iostream> using namespace std; // constant variable const int NUM_INTS = 5; // function prototypes int SearchArray(int numValues[], int searchValue); // ==== main ================================================================== // // ============================================================================ int main() { // declare variables int searchValue; int numOccurences = 0; int numValues[NUM_INTS]; // int array size is initialized with a const value // get data from user via a 'for loop' cout << "Please enter "<< NUM_INTS <<" integer values:nn"; for (int index=0; index < NUM_INTS; ++index) { cout << "#" << index + 1 << ": "; cin >> numValues[index]; cout <<endl; } // get a search value from the user cout << "Please enter a search value: "; cin >> searchValue; cout <<endl; // finds the number of occurences the search value was found in the array numOccurences = SearchArray(numValues, searchValue); // display data to user cout << "nThe total occurrences of value " << searchValue << " within the array is: " << numOccurences; cout << endl << endl; return 0; }// end of main // ==== SearchArray =========================================================== // // This function will take as input the array, the number of array // elements, and the target value to search for. The function will traverse // the array looking for the target value, and when it finds it, display the // index location within the array. // // Input: // limit [IN] -- the array, the number of array // elements, and the target value // // Output: // The total number of occurrences of the target in the array // // ============================================================================ int SearchArray(int numValues[], int searchValue) { int numFound=0; for (int index=0; index < NUM_INTS; ++index) { if (numValues[index] == searchValue) { cout << "t" << searchValue << " was found at array index #" << index << endl; ++numFound; // if the search values was found, // increment the variable by 1 } } return numFound; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 3 separate times to display the different outputs its able to produce
Please enter 5 integer values:
#1: 12
#2: 12
#3: 12
#4: 12
#5: 12
Please enter a search value: 1212 was found at array index #0
12 was found at array index #1
12 was found at array index #2
12 was found at array index #3
12 was found at array index #4The total occurrences of value 12 within the array is: 5
-------------------------------------------------------------------------Please enter 5 integer values:
#1: 12
#2: 87
#3: 45
#4: 87
#5: 33
Please enter a search value: 8787 was found at array index #1
87 was found at array index #3The total occurrences of value 87 within the array is: 2
-------------------------------------------------------------------------Please enter 5 interger values:
#1: 54
#2: 67
#3: 98
#4: 45
#5: 98
Please enter a search value: 123The total occurrences of value 123 within the array is: 0
C++ || Input/Output Text File Manipulation – Find Highest, Lowest, Average & Total Sum
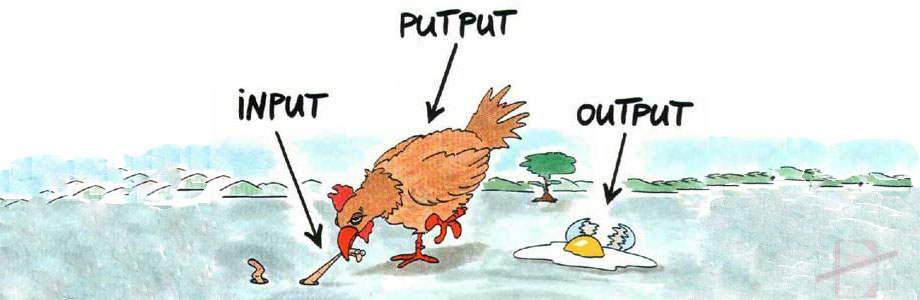
This is a program which will utilize fstream, specifically ifstream and ofstream, to read in data from one .txt file, and it will then output selected data into an entirely new separate .txt file.
The input data file has 8 different rows, with each row containing 7 numbers on each line. The program will take in each line one at a time, manipulating the 7 numbers to receive the desired output. This program will find the highest/lowest number in each selected line, along with the total sum of all the numbers contained in that line, and the average of all the numbers. So at the end of the program, There will be 8 different sets of data compiled for each row, with the output file looking like this:
SAMPLE RUN:
- Input File -
3 5 7 3 4 5 6- Output File -
The dataset for input line #1 is: 3 5 7 3 4 5 6
The highest number is: 7
The lowest number is: 3
The total of the numbers is: 33
The average of the numbers is: 4.71
The data file that is used in this example can be downloaded here
Note: In order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio > Projects > [Your project name] > [Your project name]
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 6, 2012 // Taken From: http://programmingnotes.org/ // File: fileInput.cpp // Description: Demonstrates how to read and write data to a file // ============================================================================ #include <iostream> #include <fstream> using namespace std; int main () { // declare variables ifstream infile; ofstream outfile; int inputNumber = 0; int highestNum = -999999; int lowestNum = 999999; double sum = 0; double average = 0; int currentLineNum = 1; // This opens the input file infile.open("INPUT_programmingnotes_freeweq_com.txt"); if(infile.fail()) //there was an error on open, file not found { cout << "Cannot find input file, press enter to terminate program." << endl; exit (1); // there was an error, program exits } // This opens the output file outfile.open("OUTPUT_programmingnotes_freeweq_com.txt", ios::app); if(outfile.fail()) { cout <<"There was an error opening the output file, press enter to terminate program."; exit(1); // there was an error, program exits } do { cout << "The dataset for input line #" << currentLineNum << " is: "; outfile << "The dataset for input line #" << currentLineNum << " is: "; // loops thru file until we get to the last number // from the selected line // (theres 7 numbers total in each line, see input file for clarification) for(int counter = 1; counter <= 7; counter++) { infile >> inputNumber; sum += inputNumber; // calculates the total sum of #'s for each line // checks to see which number is highest/lowest from // each selected line if(inputNumber > highestNum) { highestNum = inputNumber; } if(inputNumber < lowestNum) { lowestNum = inputNumber; } // displays current number to output screen // and saves to file cout << inputNumber << "\t"; outfile << inputNumber << "\t"; }// end for loop average = sum / 7; // finds the total avg for each line // this displays data to the output screen cout << endl; cout << "The highest number is: " << highestNum << endl; cout << "The lowest number is: " << lowestNum << endl; cout << "The total of the numbers is: " << sum << endl; cout << "The average of the numbers is: " << average << endl; // outfile section here, saves data to file outfile << endl; outfile << "The highest number is: " << highestNum << endl; outfile << "The lowest number is: " << lowestNum << endl; outfile << "The total of the numbers is: " << sum << endl; outfile << "The average of the numbers is: " << average << endl << endl; // outfile section end // resets variables back to default values highestNum = -999999; lowestNum = 999999; sum = 0; average = 0; currentLineNum++; // places data on new line cout << endl << endl; } while(!infile.eof()); // loop stops once it reaches the end of file cout << endl << endl <<"\tWe have reached the end of the file!"<< endl; outfile << endl << endl <<"\tWe have reached the end of the file!"<< endl << endl; outfile.close(); // closes outfile infile.close(); // closes infile return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
LOOPS
This program utilizes one do/while loop on lines 39-97 which loops thru the input file until it reaches the end of the file. This program also uses a for loop, which is noted on line 46.
CALCULATING THE SUM
Line 50 contains the assignment operator “+=“, which will calculate a running total for all the values of each selected line.
READING IN DATA FROM FILE
This is noted in line 48, and works just like a cin statement.
OPENING FILES
File declarations, and the opening of files are highlighted on lines: 14-15, 24-25, 32-33. On line 32, the term “ios::app” means the file will append new data to the text file, instead of overwriting the old data contained within that file.
OUTPUT DATA TO FILE
This is highlighted on lines 80-84, and as you can see, the output statements are exactly the same as cout statements.
CLOSE FILES
Remember to close the files you open, as highlighted on lines 101 and 102.
Once compiling the above code, you should receive this as your output (for the 8 selected lines contained within the input text file)
The dataset for input line #1 is: 346 130 982 90 656 117 595
The highest number is: 982
The lowest number is: 90
The total of the numbers is: 2916
The average of the numbers is: 416.571The dataset for input line #2 is: 415 948 126 4 558 571 87
The highest number is: 948
The lowest number is: 4
The total of the numbers is: 2709
The average of the numbers is: 387The dataset for input line #3 is: 42 360 412 721 463 47 119
The highest number is: 721
The lowest number is: 42
The total of the numbers is: 2164
The average of the numbers is: 309.143The dataset for input line #4 is: 441 190 985 214 509 2 571
The highest number is: 985
The lowest number is: 2
The total of the numbers is: 2912
The average of the numbers is: 416The dataset for input line #5 is: 77 81 681 651 995 93 74
The highest number is: 995
The lowest number is: 74
The total of the numbers is: 2652
The average of the numbers is: 378.857The dataset for input line #6 is: 310 9 995 561 92 14 288
The highest number is: 995
The lowest number is: 9
The total of the numbers is: 2269
The average of the numbers is: 324.143The dataset for input line #7 is: 466 664 892 8 766 34 639
The highest number is: 892
The lowest number is: 8
The total of the numbers is: 3469
The average of the numbers is: 495.571The dataset for input line #8 is: 151 64 98 813 67 834 369
The highest number is: 834
The lowest number is: 64
The total of the numbers is: 2396
The average of the numbers is: 342.286We have reached the end of the file!