C++ || How To Search For & Find A Specific Element In A Vector Using C++
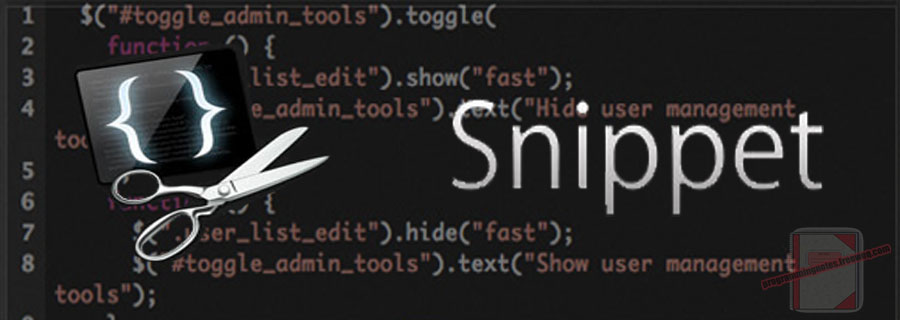
The following is a module with functions which demonstrates how to search for and find a specific element in a vector using C++.
The function demonstrated on this page is a template, so it should work on vectors of any type. It also uses a predicate to determine the item to select.
1. Find
The example below demonstrates the use of ‘Utils::find‘ to find the first element in a sequence of values based on a predicate.
The predicate determines the item to find.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Find // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate = [](const auto& x) { return x.size() >= 8; }; // Get the result auto name = Utils::find(names, predicate); // Display the result std::cout << "Name: " << (*name); // expected output: /* Name: Jennifer */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <algorithm> #include <iterator> namespace Utils { /** * FUNCTION: find * USE: Returns the first element in the given range [first, last) based on a predicate * @param first: The first position of the sequence * @param last: The last position of the sequence * @param predicate: A function to test each element for a condition * @return: A Pointer to the first element that satisfies the predicate, nullptr otherwise */ template<typename RandomIt, typename T = typename std::iterator_traits<RandomIt>::value_type, typename Pred> T* find(RandomIt first, RandomIt last, const Pred& predicate) { T* result = nullptr; auto iter = std::find_if(first, last, predicate); if (iter != last) { result = &(*iter); } return result; } /** * FUNCTION: find * USE: Returns the first element in the collection based on a predicate * @param source: The collection to find items * @param predicate: A function to test each element for a condition * @return: A Pointer to the first element that satisfies the predicate, nullptr otherwise */ template<typename T, typename Pred> T* find(std::vector<T>& source, const Pred& predicate) { return find<typename std::vector<T>::iterator, T, Pred>(source.begin(), source.end(), predicate); } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate = [](const auto& x) { return x.size() >= 8; }; // Get the result auto name = Utils::find(names, predicate); // Display the result display("Name: " + (*name)); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply