C++ || Snippet – How To Display The Size Of A File Using Stat
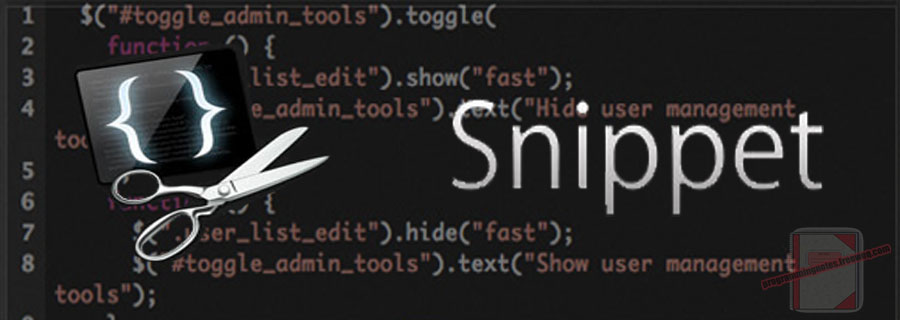
The following is sample code which demonstrates the use of the “stat” function calls on Unix based systems.
The stat function returns information about a file. No permissions are required on the file itself, but-in the case of stat() and lstat() – execute (search) permission is required on all of the directories in path that lead to the file.
The “stat” system call returns a stat structure, which contains the following fields:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
struct stat { dev_t st_dev; /* ID of device containing file */ ino_t st_ino; /* inode number */ mode_t st_mode; /* protection */ nlink_t st_nlink; /* number of hard links */ uid_t st_uid; /* user ID of owner */ gid_t st_gid; /* group ID of owner */ dev_t st_rdev; /* device ID (if special file) */ off_t st_size; /* total size, in bytes */ blksize_t st_blksize; /* blocksize for file system I/O */ blkcnt_t st_blocks; /* number of 512B blocks allocated */ time_t st_atime; /* time of last access */ time_t st_mtime; /* time of last modification */ time_t st_ctime; /* time of last status change */ }; |
The following example demonstrates the use of the “st_size” struct parameter to display the size of a file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// ============================================================================ // Author: K Perkins // Date: Oct 4, 2013 // Taken From: http://programmingnotes.org/ // File: Stat.cpp // Description: Demonstrate the use of the "stat" function to display the // size of a file // ============================================================================ #include <iostream> #include <cstdlib> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> using namespace std; int main(int argc, char* argv[]) { // declare variables struct stat fileInfo; // the buffer to store file information // check to see if theres enough commandline args if(argc < 2) { cerr<<"n** ERROR - NOT ENOUGH ARGUMENTS!n" <<"nUSAGE: "<<argv[0]<<" <FILE NAME>n"; exit(1); } // get the file information if(stat(argv[1], &fileInfo) < 0) { cerr<<"nstat failed (file not found)n"; exit(1); } // display info to the screen cerr<<"nThe file ""<<argv[1]<<"" is "<<(int)fileInfo.st_size<<" bytesnn"; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
The following is sample output:
./Stat Stat.cpp
The file "Stat.cpp" is 1170 bytes
Leave a Reply