C++ || How To Replace All Occurrences Of A String With Another String Using C++
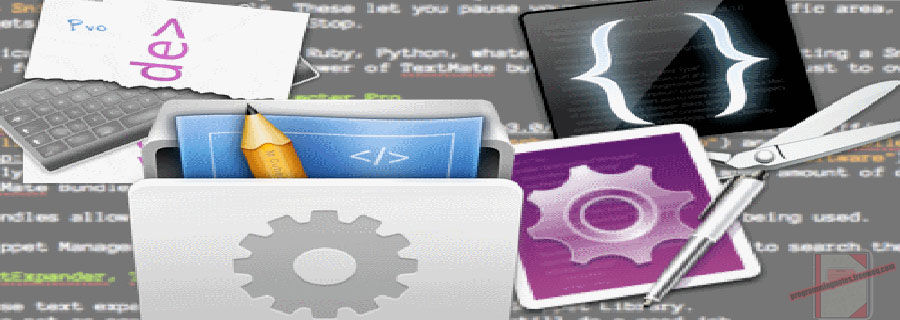
The following is a module with functions which demonstrates how to replace all occurrences of a substring with another substring using C++.
1. Replace All
The example below demonstrates the use of ‘Utils::replaceAll‘ to replace all occurrences of an ‘oldValue’ string with a ‘newValue’ string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Replace All // Declare string std::string numbers = "1987 19 22 2009 2019 1991 28 31"; // Replace whitespace with a comma std::string replaced = Utils::replaceAll(numbers, " ", ", "); // Display the result std::cout << "Original: " << numbers; std::cout << "Replaced: " << replaced; // expected output: /* Original: 1987 19 22 2009 2019 1991 28 31 Replaced: 1987, 19, 22, 2009, 2019, 1991, 28, 31 */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> namespace Utils { /** * FUNCTION: replaceAll * USE: Replaces all occurrences of the 'oldValue' string with the * 'newValue' string * @param source: The source string * @param oldValue: The string to be replaced * @param newValue: The string to replace all occurrences of oldValue * @return: A new string with all occurrences replaced */ std::string replaceAll(const std::string& source , const std::string& oldValue, const std::string& newValue) { if (oldValue.empty()) { return source; } std::string newString; newString.reserve(source.length()); std::size_t lastPos = 0; std::size_t findPos; while (std::string::npos != (findPos = source.find(oldValue, lastPos))) { newString.append(source, lastPos, findPos - lastPos); newString += newValue; lastPos = findPos + oldValue.length(); } newString += source.substr(lastPos); return newString; } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 7, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare string std::string numbers = "1987 19 22 2009 2019 1991 28 31"; // Replace whitespace with a comma std::string replaced = Utils::replaceAll(numbers, " ", ", "); // Display the result display("Original: " + numbers); display("Replaced: " + replaced); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply