C++ || How To Filter & Select Items In An Array/Vector/Container & Get The Results Using C++
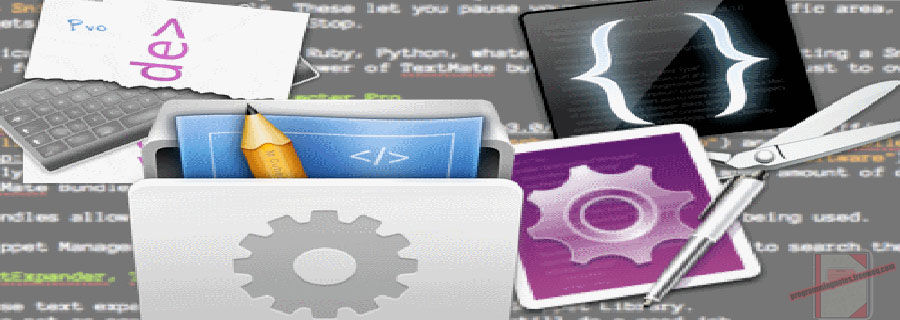
The following is a module with functions which demonstrates how to filter and select items in an array/vector/container, and get the results using C++.
The function demonstrated on this page is a template, so it should work on containers of any type. It also uses a predicate to determine the items to select.
1. Filter – Integer Array
The example below demonstrates the use of ‘Utils::filter‘ to filter an integer array based on a predicate and return its results.
The predicate determines the items to filter and select.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Filter - Integer Array // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Declare predicate auto predicate = [](const auto& x) { return x == 1987 || x == 1991; }; // Get results auto results = Utils::filter(numbers, numbers + size, predicate); // Display the results for (const auto& number : results) { std::cout << "Number: " << number << std::endl; } // expected output: /* Number: 1987 Number: 1991 */ |
2. Filter – String Vector
The example below demonstrates the use of ‘Utils::filter‘ to filter a sequence of values based on a predicate and return its results.
The predicate determines the items to filter and select.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Filter - String Vector // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate = [](const auto& x) { return x == "Kenneth" || x == "Jennifer"; }; // Get the results auto results = Utils::filter(names.begin(), names.end(), predicate); // Display the results for (const auto& name : results) { std::cout << "Name: " << name << std::endl; } // expected output: /* Name: Kenneth Name: Jennifer */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <vector> #include <algorithm> #include <iterator> namespace Utils { /** * FUNCTION: filter * USE: Filters a sequence of values in the given range [first, last) * based on a predicate and returns its results * @param first: The first position of the sequence * @param last: The last position of the sequence * @param predicate: A function to test each element for a condition * @return: A collection that contains elements from the input * sequence that satisfies the condition */ template<typename InputIt, typename T = typename std::iterator_traits<InputIt>::value_type, typename Pred> std::vector<T> filter(InputIt first, InputIt last, const Pred& predicate) { std::vector<T> result; std::copy_if(first, last, std::back_inserter(result), predicate); return result; } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 8, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include <vector> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Declare predicate auto predicate = [](const auto& x) { return x == 1987 || x == 1991; }; // Get results auto results = Utils::filter(numbers, numbers + size, predicate); // Display the results for (const auto& number : results) { display("Number: " + std::to_string(number)); } display(""); // Declare vector std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Declare predicate auto predicate2 = [](const auto& x) { return x == "Kenneth" || x == "Jennifer"; }; // Get the results auto results2 = Utils::filter(names.begin(), names.end(), predicate2); // Display the results for (const auto& name : results2) { display("Name: " + name); } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply