C# || How To Remove Stones To Minimize The Total Using C#
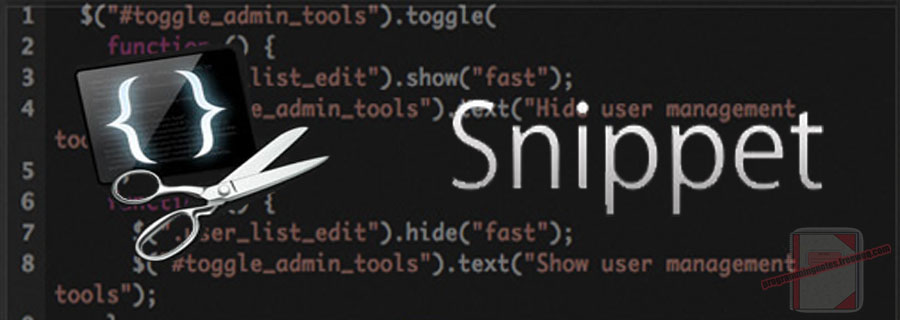
The following is a module with functions which demonstrates how to remove stones to minimize the total using C#.
1. Min Stone Sum – Problem Statement
You are given a 0-indexed integer array piles, where piles[i] represents the number of stones in the ith pile, and an integer k. You should apply the following operation exactly k times:
- Choose any piles[i] and remove floor(piles[i] / 2) stones from it.
Notice that you can apply the operation on the same pile more than once.
Return the minimum possible total number of stones remaining after applying the k operations.
floor(x) is the greatest integer that is smaller than or equal to x (i.e., rounds x down).
Example 1:
Input: piles = [5,4,9], k = 2
Output: 12
Explanation: Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are [5,4,5].
- Apply the operation on pile 0. The resulting piles are [3,4,5].
The total number of stones in [3,4,5] is 12.
Example 2:
Input: piles = [4,3,6,7], k = 3
Output: 12
Explanation: Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are [4,3,3,7].
- Apply the operation on pile 3. The resulting piles are [4,3,3,4].
- Apply the operation on pile 0. The resulting piles are [2,3,3,4].
The total number of stones in [2,3,3,4] is 12.
2. Min Stone Sum – Solution
The following is a solution which demonstrates how to remove stones to minimize the total.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 1, 2023 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to remove stones to minimize the total // ============================================================================ public class Solution { public int MinStoneSum(int[] piles, int k) { var heap = new PriorityQueue<int, int>(Comparer<int>.Create((a, b) => b - a)); int totalSum = 0; foreach (int num in piles) { heap.Enqueue(num, num); totalSum += num; } for (int index = 0; index < k; ++index) { int currentValue = heap.Dequeue(); int removeFromTotal = currentValue / 2; int newValue = currentValue - removeFromTotal; heap.Enqueue(newValue, newValue); totalSum -= removeFromTotal; } return totalSum; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
12
12
Leave a Reply