Tag Archives: if/else
Java || Find The Prime, Perfect & All Known Divisors Of A Number Using A For, While & Do/While Loop
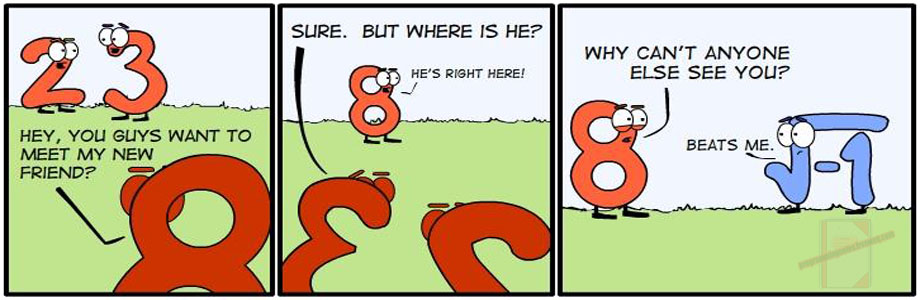
This program was designed to better understand how to use different loops which are available in Java, as well as more practice using objects with classes.
This program first asks the user to enter a non negative number. After it obtains a non negative integer from the user, the program determines if the user obtained number is a prime number or not, aswell as determining if the user obtained number is a perfect number or not. After it obtains its results, the program will display to the screen if the user inputted number is prime/perfect number or not. The program will also display a list of all the possible divisors of the user obtained number via stdout.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Class Objects - How TO Use
Constructors - What Are They?
Do/While Loop
While Loop
For Loop
Modulus
Basic Math - Prime Numbers
Basic Math - Perfect Numbers
Basic Math - Divisors
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 |
import java.util.Scanner; public class PrimePerfectNums { // global variable declaration int userInput = 0; static Scanner cin = new Scanner(System.in); public PrimePerfectNums(int input) {// this is the constructor // give the global variable a value userInput = input; }// end of PrimePerfectNums public void CalcPrimePerfect() { // declare variables int divisor = 0; int sumOfDivisors = 0; System.out.print("nInput number: " + userInput); // for loop adds sum of all possible divisors for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0) { // this will repeatedly add the found divisors together sumOfDivisors += counter; } } System.out.println(""); // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is prime if(userInput == (sumOfDivisors - 1)) { System.out.print(userInput + " is a prime number."); } else { System.out.print(userInput + " is not a prime number."); } System.out.println(""); // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is a perfect number if (userInput == (sumOfDivisors - userInput)) { System.out.print(userInput + " is a perfect number."); } else { System.out.print(userInput + " is not a perfect number."); } System.out.print("nDivisors of " + userInput + " are: "); // for loop lists all the possible divisors for the // 'userInput' variable by using the modulus operator for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0 && counter !=userInput) { System.out.print(counter + ", "); } } System.out.print("and " + userInput); }// end of CalcPrimePerfect public static void main(String[] args) { // declare variables int input = 0; char response ='n'; do{ // this is the start of the do/while loop System.out.print("Enter a number: "); input = cin.nextInt(); // if the user inputs a negative number, do this code while(input < 0) { System.out.print("ntSorry, but the number entered is less " + "than the allowable limit.ntPlease try again....."); System.out.print("nnEnter an number: "); input = cin.nextInt(); } // entry point to class declaration PrimePerfectNums myClass = new PrimePerfectNums(input); // function call to obtain data using the number // which was passed from main to the constructor myClass.CalcPrimePerfect(); // asks user if they want to enter new data System.out.print("nntDo you want to input another number?(Y/N): "); response = cin.next().toLowerCase().charAt(0); System.out.println("---------------------------" + "---------------------------------"); }while(response =='y'); // ^ End of the do/while loop. As long as the user chooses // 'Y' the loop will keep going. // It stops when the user chooses the letter 'N' System.out.println("BYE!"); }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Enter a number: 41
Input number: 41
41 is a prime number.
41 is not a perfect number.
Divisors of 41 are: 1, and 41Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 496Input number: 496
496 is not a prime number.
496 is a perfect number.
Divisors of 496 are: 1, 2, 4, 8, 16, 31, 62, 124, 248, and 496Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 1858Input number: 1858
1858 is not a prime number.
1858 is not a perfect number.
Divisors of 1858 are: 1, 2, 929, and 1858Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: -9Sorry, but the number entered is less than the allowable limit.
Please try again.....Enter an number: 12
Input number: 12
12 is not a prime number.
12 is not a perfect number.
Divisors of 12 are: 1, 2, 3, 4, 6, and 12Do you want to input another number?(Y/N): n
------------------------------------------------------------
BYE!
Java || Find The Average Using an Array – Omit Highest And Lowest Scores
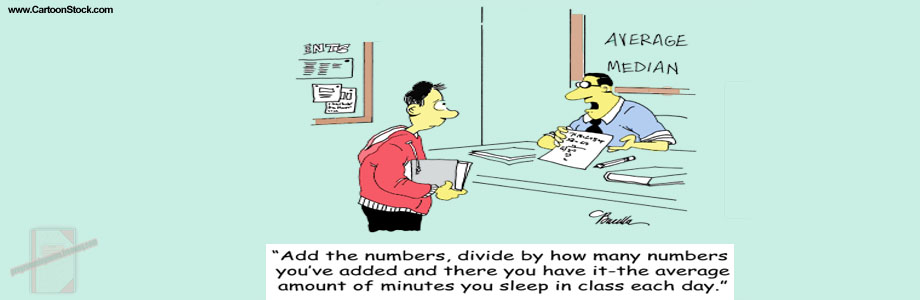
This page will consist of two programs which calculates the average of a specific amount of numbers using an array.
REQUIRED KNOWLEDGE FOR BOTH PROGRAMS
Double Data Type
Final Variables
Arrays
For Loops
Assignment Operators
Basic Math - How To Find The Average
====== FIND THE AVERAGE USING AN ARRAY ======
The first program is fairly simple, and it was used to introduce the array concept. The program prompts the user to enter the total amount of numbers they want to find the average for, then the program displays the answer to them via stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
import java.util.Scanner; public class FindTheAverage { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables int numElems = 0; double sum = 0; double[] average = new double[100]; // declare array which has the ability to hold 100 elements // display message to screen System.out.println("Welcome to My Programming Notes' Java Program.n"); // determine how many numbers the user wants in the array System.out.print("How many numbers do you want to find the average for?: "); numElems = cin.nextInt(); System.out.println(""); // user enters data into array using a for loop // you can also use a while loop, but for loops are more common // when dealing with arrays for(int index=0; index < numElems; ++index) { System.out.print("Enter value #" +(index+1)+ ": "); average[index] = cin.nextDouble(); sum += average[index]; } // find the average. // Note: the expression below literally // means: sum = sum / numElems; sum /= numElems; System.out.println("nThe average of the " + numElems + " numbers is " + sum); }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
ARRAY
Notice the array declaration on line #13. The type of array being used in this program is a dynamic array, which has the ability to store up to 100 integer elements in the array. You can change the number of elements its able to store to a higher or lower number if you wish.
FOR LOOP
Lines 27-32 contains a for loop, which is used to actually store the data inside of the array. Without some type of loop, it is virtually impossible for the user to input data into the array; that is, unless you want to add 100 different println statements into your code asking the user to input data. Line 31 uses the assignment operator “+=” which gives us a running total of the data that is being inputted into the array. Note the loop only stores as many elements as the user so desires, so if the user only wants to input 3 numbers into the array, the for loop will only execute 3 times.
Once compiled, you should get this as your output:
Welcome to My Programming Notes' Java Program.
How many numbers do you want to find the average for?: 4
Enter value #1: 21
Enter value #2: 24
Enter value #3: 19
Enter value #4: 17
The average of the 4 numbers is 20.25
====== FIND THE AVERAGE – OMIT HIGHEST AND LOWEST SCORES ======
The second program is really practical in a real world setting. We were asked to create a program for a fictional competition which had 6 judges. The 6 judges each gave a score of the performance for a competitor in a competition, (i.e a score of 1-10), and we were asked to find the average of those scores, omitting the highest/lowest results. The program was to store the scores into an array, display the scores back to the user via stdout, display the highest and lowest scores among the 6 obtained, display the average of the 6 scores, and finally display the average adjusted scores omitting the highest and lowest result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
import java.util.Scanner; public class FindOmittedAverage { // global variable declaration static Scanner cin = new Scanner(System.in); static final int NUM_JUDGES = 6; public static void main(String[] args) { // declare variables double highestScore = -999999; double lowestScore = 999999; double sumOfScores = 0; double avgScores = 0; double[] scores = new double[NUM_JUDGES]; // array is initialized using a final variable // display message to screen System.out.println("Welcome to My Programming Notes' Java Program.n"); System.out.print("Judges, enter one score each for the current competitor: "); // use a for loop to obtain data from user using the final variable for(int index=0; index < NUM_JUDGES; ++index) { // this puts data into the current array index scores[index] = cin.nextDouble(); // this calculates a running total of all the scores // adding each element in the array together sumOfScores += scores[index]; // if current score in the array index is bigger than the current 'highestScore' // value, then set 'highestScore' equal to the current value in the array if(scores[index] > highestScore) { highestScore = scores[index]; } // if current score in the array index is smaller than the current 'lowestScore' // value, then set 'lowestScore' equal to the current value in the array if(lowestScore > scores[index]) { lowestScore = scores[index]; } } System.out.print("nThese are the scores from the " + NUM_JUDGES + " judges: "); // use another for loop to redisplay the data back to the user via stdout for(int index=0; index < NUM_JUDGES; ++index) { System.out.print("nThe score for judge #"+(index+1)+" is: "+scores[index]); } // display the highest/lowest numbers to the screen System.out.print("nnThese are the highest and lowest scores: "); System.out.print("ntHighest: "+ highestScore); System.out.print("ntLowest: "+ lowestScore); // find the average avgScores = sumOfScores/NUM_JUDGES; System.out.print("nThe average score is: "+ avgScores); // reset data back to 0 so we can find the ommitted average sumOfScores = 0; avgScores = 0; System.out.print("nThe average adjusted score omitting the highest and lowest result is: "); // final loop, which calculates a running total, adding each element // in the array together, this time omitting the highest/lowest scores for(int index=0; index < NUM_JUDGES; ++index) { // IF(current score isnt equal to the highest elem) AND (current score isnt equal lowest elem) // THEN create a running total if((scores[index] != highestScore) && (scores[index] != lowestScore)) { sumOfScores += scores[index]; } } // find the average, minus the 2 scores avgScores = sumOfScores/(NUM_JUDGES-2); System.out.print(avgScores); }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
FINAL
A final variable was declared and used to initialize the array (line 7). This was used to initialize the size of the array.
FOR LOOPS
Once again loops were used to traverse the array, as noted on lines 24, 51 and 73. The final variable was also used within the for loops, making it easier to modify the code if its necessary to reduce or increase the number of available judges.
HIGHEST/LOWEST SCORES
This is noted on lines 35-45, and it is really simple to understand the process once you see the code.
OMITTING HIGHEST/LOWEST SCORE
Lines 73-81 highlights this process. The loop basically traverses the array, skipping over the highest/lowest elements.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Judges, enter one score each for
the current competitor: 123 453 -789 2 23345 987These are the scores from the 6 judges:
The score for judge #1 is: 123.0
The score for judge #2 is: 453.0
The score for judge #3 is: -789.0
The score for judge #4 is: 2.0
The score for judge #5 is: 23345.0
The score for judge #6 is: 987.0These are the highest and lowest scores:
Highest: 23345.0
Lowest: -789.0
The average score is: 4020.1666666666665
The average adjusted score omitting the highest and lowest result is: 391.25
Java || Whats My Name? – Practice Using Strings, Methods & Switch Statemens
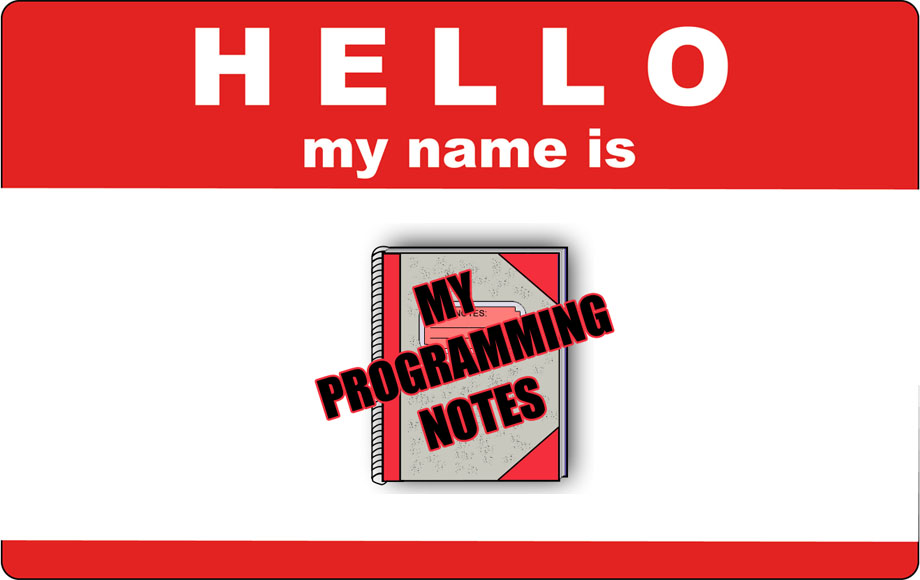
Here is another actual homework assignment which was presented in an intro to programming class. This program highlights more use using strings, modules, and switch statements.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Methods (A.K.A "Functions") - What Are They?
Switch Statements - How To Use Them
Equal - String Comparison
This program first prompts the user to enter their name. Upon receiving that information, the program saves input into a string called “firstName.” The program then asks if the user has a middle name. If they do, the user will enter a middle name. If they dont, the program proceeds to ask for a last name. Upon receiving the first, [middle], and last names, the program will append the first, [middle], and last names into a completely new string titled “fullName.” Lastly, if the users’ first, [middle], or last names are the same, the program will display that data to the screen via stdout. The program will also display to the user the number of characters their full name contains using the built in function “length.”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 |
import java.util.Scanner; public class NameString { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char response = ' '; String firstName= ""; String fullName=""; // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); // get first name from user System.out.print("Please enter your first name: "); firstName = cin.next(); System.out.print("nDo you have a middle name?(Y/N): "); String choice = cin.next(); choice = choice.toUpperCase(); response = choice.charAt(0); // use a switch statement to detrmine which function will be called switch(response) { case 'Y': fullName = FirstMiddleLast(firstName); // method declaration break; case 'N': fullName = FirstLast(firstName); // method declaration break; default: System.out.print("nPlease press either 'Y' or 'N'n" + "Program exiting...n"); System.exit(1); break; } System.out.println("And your full name is "+fullName); }// end of main // ==== FirstMiddleLast ==================================================== // // This module will take as input the first name, and prompt the user for // their middle and last name. Then it will display the total number of // characters in their name. It returns the full name back to main // // ========================================================================= private static String FirstMiddleLast(String firstName) { String middleName=""; String lastName=""; String fullName=""; System.out.print("nPlease enter your middle name: "); middleName = cin.next(); System.out.print("Please enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+middleName+" "+lastName; System.out.println(""); // check to see if the first, middle or last names are the same // if they are, display a message to the user if(firstName.equals(middleName)) { System.out.print("tYour first and middle name are the samen"); } if(middleName.equals(lastName)) { System.out.print("tYour middle and last name are the samen"); } if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the 2 white spaces System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-2)); // return the full name string to main return fullName; }// end of FirstMiddleLast // ==== FirstLast ========================================================== // // This module will take as input the first name, and prompt the user for // their last name. Then it will display the total number of characters in // their name. It returns the full name back to main // // ============================================================================ private static String FirstLast(String firstName) { String lastName=""; String fullName=""; System.out.print("nPlease enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+lastName; System.out.println(""); // check to see if the first or last names are the same // if they are, display a message to the user if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the white space System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-1)); // return the full name string to main return fullName; }// end of FirstLast }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: The code was compiled five separate times to display the different outputs its able to produce
====== RUN 1 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): y
Please enter your middle name: Programming
Please enter your last name: NotesThe total number of characters in your name is: 18
And your full name is My Programming Notes====== RUN 2 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Programming
Do you have a middle name?(Y/N): n
Please enter your last name: NotesThe total number of characters in your name is: 16
And your full name is Programming Notes====== RUN 3 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Notes
Do you have a middle name?(Y/N): y
Please enter your middle name: Notes
Please enter your last name: NotesYour first and middle name are the same
Your middle and last name are the same
Your first and last name are the sameThe total number of characters in your name is: 15
And your full name is Notes Notes Notes====== RUN 4 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): n
Please enter your last name: MyYour first and last name are the same
The total number of characters in your name is: 4
And your full name is My My====== RUN 5 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): zPlease press either 'Y' or 'N'
Program exiting...
Java || Count The Total Number Of Characters, Vowels, & UPPERCASE Letters Contained In A Sentence Using A ‘For Loop’
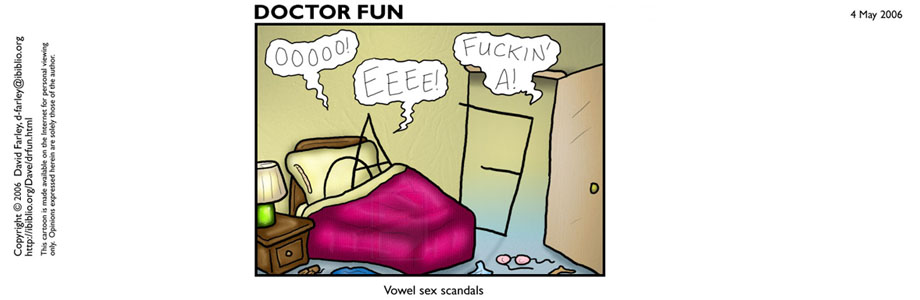
Here is a simple program, which demonstrates more practice using the input/output mechanisms which are available in Java.
This program will prompt the user to enter a sentence, then upon entering an “exit code,” will display the total number of uppercase letters, vowels and characters which are contained within that sentence. This program is very similar to an earlier project, this time, utilizing a for loop, strings, and user defined methods.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
For Loops
Methods (A.K.A "Functions") - What Are They?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
import java.util.Scanner; public class VowelCount { public static void main(String[] args) { // declare variables int numUpperCase = 0; int numVowel = 0; int numChars = 0; char character = ' '; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); System.out.print("Enter a sentence, ending with a period: "); userInput = cin.nextLine(); // loop thru the string until we reach the 'exit code' for(int index=0; userInput.charAt(index) != '.'; ++index) { character = userInput.charAt(index); // do nothing, we dont care about whitespace if(character == ' ') { continue; } // check to see if entered data is UPPERCASE if((character >= 'A')&&(character <= 'Z')) { ++numUpperCase; // Increments Total number of uppercase by one } // check to see if entered data is a vowel if((character == 'a')||(character == 'A')||(character == 'e')|| (character == 'E')||(character == 'i')||(character == 'I')|| (character == 'o')||(character == 'O')||(character == 'u')|| (character == 'U')||(character == 'y')||(character == 'Y')) { ++numVowel; // increment total number of vowels by one } ++numChars; // increment total number of chars by one } setwf("ntTotal number of upper case letters:", 45,'.'); System.out.println(numUpperCase); setwf("tTotal number of vowels:", 44,'.'); System.out.println(numVowel); setwf("tTotal number of characters:", 44,'.'); System.out.println(numChars); }// end of main public static void setwf(String str, int width, char fill) {// this mimics the C++ 'setw' function & formats string output to the screen System.out.print(str); for (int x = str.length(); x < width; ++x) { System.out.print(fill); } }// end of setwf }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted portions are areas of interest.
Notice line 22 contains the for loop declaration. The loop will continually loop thru the string, and will not stop doing so until it reaches an exit character, and the defined exit character in this program is a period (“.”). So, the program will not stop looping thru the string until it reaches a period.
Once compiling the above code, you should receive this as your output
Welcome to My Programming Notes' Java Program.
Enter a sentence, ending with a period:
My Programming Notes Is An Awesome Site.Total number of upper case letters:........7
Total number of vowels:....................14
Total number of characters:................33
C++ || Stack – Using A Stack, Determine If A Set Of Parentheses Is Well-Formed
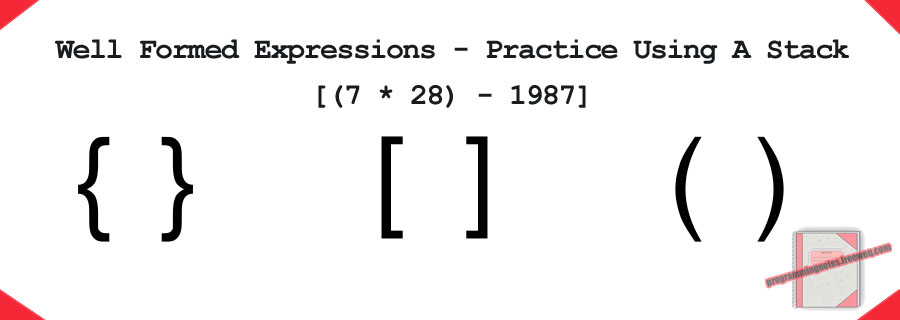
Here is another homework assignment which was presented in a C++ Data Structures course. This assignment was used to introduce the stack ADT, and helped prepare our class for two later assignments which required using a stack. Those assignments can be found here:
(1) Stack Based Infix To Postfix Conversion (Single Digit)
(2) Stack Based Postfix Evaluation (Single Digit)
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Stack Data Structure
Cin.getline
#include "ClassStackListType.h"
A simple exercise for testing a stack is determining whether a set of parenthesis is “well formed” or not. What exactly is meant by that? In the case of a pair of parenthesis, for an expression to be well formed, consider the following table.
1 2 3 4 5 6 |
Well-Formed Expressions | Ill-Formed Expressions ------------------------------------|-------------------------------------- ( a b c [ ] ) | ( a b c [ ) ( ) [ ] { } | ( ( { ( a b c d e ) ( ) } | [ a b c d e ) ( ) } ( a + [ b - c ] / d ) | ( a + [ b - c } / d ) |
Given an expression with characters and parenthesis, ( ), [ ], and { }, our class was asked to determine if an expression was well formed or not by using the following algorithm:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
// An algorithm for a Well Formed expression Set 'balanced' to true Set 'symbol' to the first character in the current expression while(there are more characters AND 'balanced' == true) { if('symbol' is an opening symbol) { Push 'symbol' onto the stack } else if('symbol' is a closing symbol) { if the stack is empty 'balanced' = false else Set the 'openSymbol' to the item at the top of the stack Pop the stack Check to see if 'symbol' matches 'openSymbol' (i.e - if openSymbol == '(' and symbol == ')' then 'balanced' = true) } Set 'symbol' to the next character in the current expression } if('balanced' == true AND stack is empty) { Expression is well formed } else { Expression is NOT well formed }// http://programmingnotes.org/ |
======= WELL-FORMED EXPRESSIONS =======
This program uses a custom template.h class. To obtain the code for that class, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2012 // Taken From: http://programmingnotes.org/ // File: wellFormed.cpp // Description: Demonstrates how to check if an expression is well formed // ============================================================================ #include <iostream> #include "ClassStackListType.h" using namespace std; // function prototypes bool IsOpen(char symbol); bool IsClosed(char symbol); bool IsWellFormed(char openSymbol, char closeSymbol); int main() { // declare variables char expression[120]; char openSymbol; int index=0; bool balanced = true; StackListType<char> stack; // this is the stack declaration // obtain data from the user using a char array cout <<"Enter an expression and press ENTER. "<<endl; cin.getline(expression,sizeof(expression)); cout << "\nThe expression: " << expression; // loop thru the char array until we reach the 'NULL' character // and while 'balanced' == true while (expression[index]!='\0' && balanced) { // if input is an "open bracket" push onto stack // else, process info if (IsOpen(expression[index])) { stack.Push(expression[index]); } else if (IsClosed(expression[index])) { if(stack.IsEmpty()) { balanced = false; } else { openSymbol = stack.Top(); stack.Pop(); balanced = IsWellFormed(openSymbol, expression[index]); } } ++index; } if (balanced && stack.IsEmpty()) { cout << " is well formed..." << endl; } else { cout << " is NOT well formed!!! " << endl; } }// End of Main bool IsOpen(char symbol) { if ((symbol == '(') || (symbol == '{') || (symbol == '[')) { return true; } else { return false; } }// End of IsOpen bool IsClosed(char symbol) { if ((symbol == ')') || (symbol == '}') || (symbol == ']')) { return true; } else { return false; } }// End of IsClosed bool IsWellFormed(char openSymbol, char closeSymbol) { return (((openSymbol == '(') && closeSymbol == ')') || ((openSymbol == '{') && closeSymbol == '}') || ((openSymbol == '[') && closeSymbol == ']')); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: the code was compile four separate times to display different output)
====== RUN 1 ======
Enter an expression and press ENTER.
((
The expression: (( is NOT well formed!!!====== RUN 2 ======
Enter an expression and press ENTER.
(a{b[]}c)The expression: (a{b[]}c) is well formed...
====== RUN 3 ======
Enter an expression and press ENTER.
[(7 * 28) - 1987]The expression: [(7 * 28) - 1987] is well formed...
====== RUN 4 ======
Enter an expression and press ENTER.
{3 + [2 / 3] - (9 + 18) * 12)The expression: {3 + [2 / 3] - (9 + 18) * 12) is NOT well formed!!!
C++ || FizzBuzz – Tackling The Fizz Buzz Test In C++
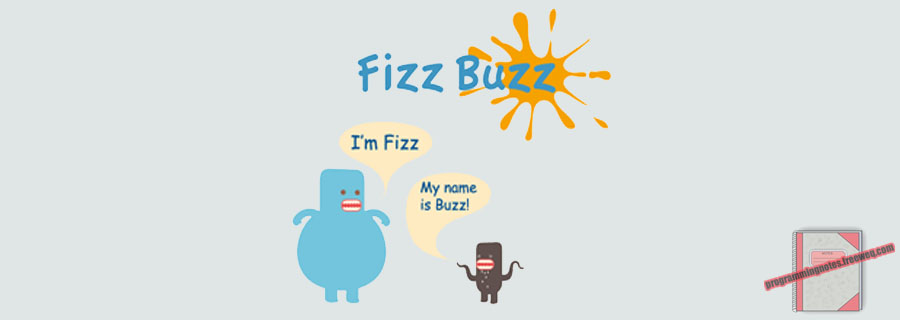
What is Fizz Buzz?
Simply put, a “Fizz-Buzz test” is a programming interview question designed to help filter out potential job prospects – those who can’t seem to program if their life depended on it.
An example of a typical Fizz-Buzz question is the following:
Write a program which prints the numbers from 1 to 100. But for multiples of three, print the word “Fizz” instead of the number, and for the multiples of five, print the word “Buzz”. For numbers which are multiples of both three and five, print the word “FizzBuzz”.
This seems easy enough, and many should be able to complete a program which carries out a solution in a few minutes. Though, after doing a little research, apparently that is not the case. This page will present one way to carry out a solution to this Fizz-Buzz problem.
There is a small “catch” that some may encounter when trying to solve this problem, and that is the fact that the conditional statement for the number divisible by 15 should come before each sequential conditional statement. Consider this pseudocode:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// A simple Fizz-Buzz algorithm using a while loop 'currentNumber' = 1 while('currentNumber' is less than or equal to 100) { if('currentNumber' is divisible by 3) AND ('currentNumber' is divisible by 5) print "FizzBuzz" else if('currentNumber' is divisible by 3) print "Fizz" else if('currentNumber' is divisible by 5) print "Buzz" else // 'currentNumber' is not divisible by 3 or 5 print 'currentNumber' increment 'currentNumber' by one }// http://programmingnotes.org/ |
The portion that may make this problem tricky for some is the fact that the conditional statement for the number divisible by 15 must be checked -before- the conditional statements which checks for numbers divisible by 3 and 5. If the conditional statements are placed in any other order, the end result will not be correct, which is what can make the problem difficult for many.
======= THE FIZZ BUZZ TEST =======
So building upon the pseudocode found from above, utilizing the modulus operator, here is a simple solution to the Fizz Buzz Test
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2012 // Taken From: http://programmingnotes.org/ // File: fizzbuzz.cpp // Description: Demonstrates the fizz buzz test // ============================================================================ #include <iostream> using namespace std; int main() { // declare variables int fizz = 3; int buzz = 5; int endNumber = 100; int fizzBuzz = fizz * buzz; // ^ numbers divisible by 3 and 5 are also divisible by 3 * 5 // start the loop, continue until the counter // reaches the 'end' for (int currentNumber = 1; currentNumber <= endNumber; ++currentNumber) { if (currentNumber % fizzBuzz == 0) // divisible by 3 and 5 { cout<<"FIZZ BUZZ!!\n"; } else if (currentNumber % fizz == 0) // divisible by 3 { cout<<"FIZZ\n"; } else if (currentNumber % buzz == 0)// divisible by 5 { cout<<"BUZZ\n"; } else // not divisible by 3 or 5 { cout<<currentNumber<<endl; } } return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
1
2
FIZZ
4
BUZZ
FIZZ
7
8
FIZZ
BUZZ
11
FIZZ
13
14
FIZZ BUZZ!!
16
17
FIZZ
19
BUZZ
FIZZ
22
23
FIZZ
BUZZ
26
FIZZ
28
29
FIZZ BUZZ!!
31
32
FIZZ
34
BUZZ
FIZZ
37
38
FIZZ
BUZZ
41
FIZZ
43
44
FIZZ BUZZ!!
46
47
FIZZ
49
BUZZ
FIZZ
52
53
FIZZ
BUZZ
56
FIZZ
58
59
FIZZ BUZZ!!
61
62
FIZZ
64
BUZZ
FIZZ
67
68
FIZZ
BUZZ
71
FIZZ
73
74
FIZZ BUZZ!!
76
77
FIZZ
79
BUZZ
FIZZ
82
83
FIZZ
BUZZ
86
FIZZ
88
89
FIZZ BUZZ!!
91
92
FIZZ
94
BUZZ
FIZZ
97
98
FIZZ
BUZZ
C++ || Snippet – Round A Number To The Nearest Whole Number
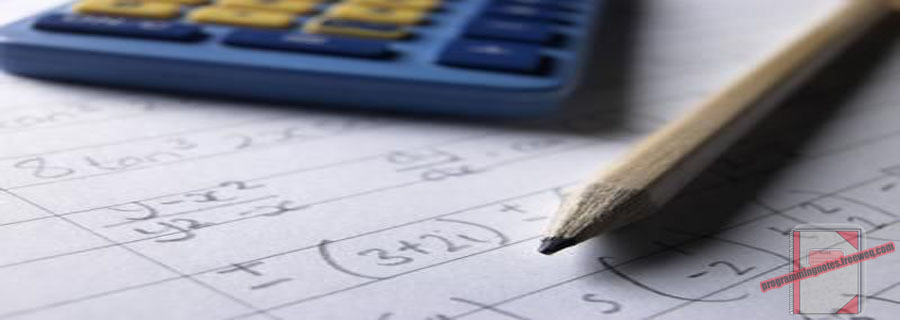
This page will display a simple implementation of a function which rounds a floating point number to the nearest whole number. So for example, if the number 12.34542 was sent to the function, it would return the rounded value of 12.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
#include <iostream> #include <cmath> using namespace std; // function prototype double RoundNumber(double floatNumber); int main() { // declare variables double floatNumber = 0; cout<<"Enter in a floating point number to round: "; cin >> floatNumber; cout<<endl<<floatNumber<<" rounded to the nearest " "whole number is: "<< RoundNumber(floatNumber)<<endl; return 0; } double RoundNumber(double floatNumber) { // declare variables double intNumber = 0; // perform modulus (floatNumber % intNumber) // to render the decimal portion of the floatNumber double fraction = modf(floatNumber,&intNumber); // we round up if fraction >=.50 if(fraction >=.50) { ++intNumber; } return intNumber; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: the code was compile three separate times to display different output)
====== RUN 1 ======
Enter in a floating point number to round: 1.3333
1.3333 rounded to the nearest whole number is: 1
====== RUN 2 ======
Enter in a floating point number to round: 35.56
35.56 rounded to the nearest whole number is: 36
====== RUN 3 ======
Enter in a floating point number to round: 19.8728
19.8728 rounded to the nearest whole number is: 20
C++ || Snippet – How To Find The Minimum & Maximum Of 3 Numbers, Print In Ascending Order
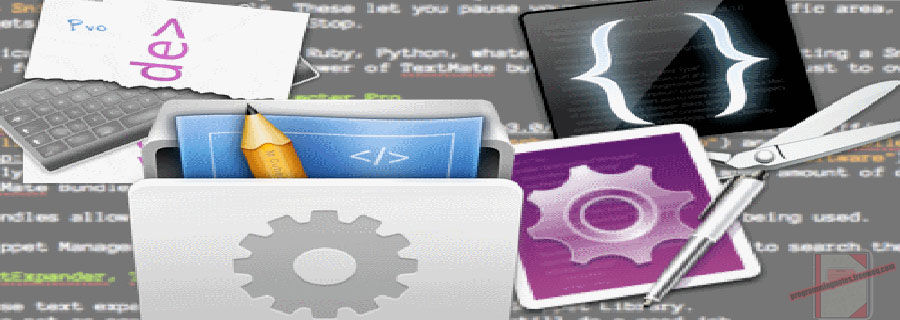
This page will demonstrate how to find the minimum and maximum of 3 numbers. After the maximum and minimum numbers are obtained, the 3 numbers are displayed to the screen in ascending order.
This program uses multiple if-statements to determine equality, and uses 3 seperate int varables to store its data. This program is very basic, so it does not utilize an integer array, or any sorting methods.
NOTE: If you want to find the Minimum & Maximum of numbers contained in an integer array, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
// ============================================================================ // Author: K Perkins // Date: Apr 29, 2012 // Taken From: http://programmingnotes.org/ // File: min_mid_max.cpp // Description: Demonstrates how to find the minimum & maximum of 3 numbers // ============================================================================ #include <iostream> using namespace std; int main() { // declare variables int a=0; int b=0; int c=0; int max=0; int min=0; int middle=0; // get data from user cout<<"Please enter 3 numbers: "; cin >> a >> b >> c; // display information back to the user cout <<"The numbers you just entered are: "<<a<<" "<<b<<" "<<c<<endl; // check for the highest number if(a > b && a > c) { max = a; } else if(b > a && b > c) { max = b; } else { max = c; } // check for the lowest number if(a < b && a < c) { min = a; } else if(b < a && b < c) { min = b; } else { min = c; } // use the 'min' and 'max' variables from above ^ to find // the 'middle' value if((max == a && min == b) || (max == b && min == a)) { middle = c; } else if((max == b && min == c) || (max == c && min == b)) { middle = a; } else { middle = b; } // display data to the screen cout<<"nThe maximum number is: "<<max; cout<<"nThe minimum number is: "<<min; cout<<"nThe numbers in order are: "<<min<<" "<<middle<< " "<<max<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Please enter 3 numbers: 89 56 1987
The numbers you just entered are: 89 56 1987The maximum number is: 1987
The minimum number is: 56
The numbers in order are: 56 89 1987
C++ || Class & Input/Output – Display The Contents Of A User Specified Text File To The Screen
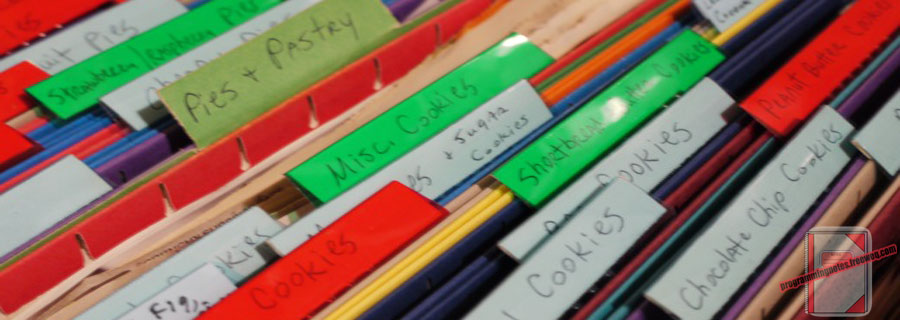
The following is another intermediate homework assignment which was presented in a C++ programming course. This program was assigned to introduce more practice using the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
How To Read Data From A File
String - Getline
Array - Cin.Getline
Strcpy - Copy Contents Of An Array
#Define
This program first prompts the user to input a file name. After it obtains a file name from the user, it then attempts to display the contents of the user specified file to the output screen. If the file could not be found, an error message appears. If the file is found, the program continues as normal. After the file contents finishes being displayed, a summary indicating the total number of lines which has been read is also shown to the screen.
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
Note: The data file that is used in this example can be downloaded here.
Also, in order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
======== FILE #1 – Main.cpp ========
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// ============================================================================ // File: Main.cpp // ============================================================================ // This program tests the "CFileDisp" object. It prompts the user for an input // filename, then attempts to display the contents of that file to stdout. // After the file contents have been displayed, a summary line indicating the // total number of lines that have been displayed is written to stdout. // ============================================================================ #include <iostream> #include <fstream> #include "CFileDisp.h" using namespace std; // ==== main ================================================================== // // ============================================================================ int main() { CFileDisp myFile; char fname[MAX_LENGTH]; int numLines; // get the filename from the user cout << "Enter a filename: "; cin.getline(fname, MAX_LENGTH); // copy the user's filename into the file object myFile.SetFilename(fname); // have the object open the file myFile.OpenFile(); // if all is good and well... if(myFile.IsValid()==true) { numLines = myFile.DisplayFileContents(); // close the file myFile.CloseFile(); // write how many lines were displayed to stdout cout << "nt*** Total lines displayed: " << numLines << endl; } return 0; }// http://programmingnotes.org/ |
======== FILE #2 – CFileDisp.h ========
Remember, you need to name the header file the same as the #include from the Main.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// ============================================================================ // File: CFileDisp.h // ============================================================================ // This is the header file which declares the objects which are used to open // a text file and display its contents to stdout. // ============================================================================ #ifndef CFILE_DISP_HEADER #define CFILE_DISP_HEADER #include <fstream> using namespace std; #define MAX_LENGTH 256 class CFileDisp { public: // constructor CFileDisp(); // member functions void SetFilename(char newFilename[]); /* Purpose: Copy the user's filename into the file object */ void OpenFile(); /* Purpose: Open's the file which is specified by the user */ bool IsValid(); /* Purpose: Checks if the file exists. Post: Returns false if file cannot be found */ int DisplayFileContents(); /* Purpose: Display's the file contents to stdout Post: Returns the total number of lines contained in the file */ void CloseFile(); /* Purpose: Closes the file */ // destructor ~CFileDisp(); private: bool m_bIsValid; char m_filename[MAX_LENGTH]; ifstream m_inFile; }; #endif // CFILE_DISP_HEADER // http://programmingnotes.org/ |
======== FILE #3 – CFileDisp.cpp ========
This is the function implementation file for the CFileDisp.h class. This file can be named anything you wish as long as you #include “CFileDisp.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
// ============================================================================ // File: CFileDisp.cpp // ============================================================================ // This file implements the functions which are declared in the CFileDisp // class, which is located in CFileDisp.h // ============================================================================ #include <iostream> #include <cstring> #include <string> #include "CFileDisp.h" using namespace std; CFileDisp::CFileDisp() { m_bIsValid = 0; }// end of CFileDisp void CFileDisp::SetFilename(char newFilename[]) { strcpy(m_filename,newFilename); }// end of SetFilename void CFileDisp::OpenFile() { m_inFile.open(m_filename); }// end of OpenFile bool CFileDisp::IsValid() { if (m_inFile.fail()) { cout << m_filename << " was not found...nn"; return false; } else { return true; } }// end of IsValid int CFileDisp::DisplayFileContents() { int total=0; string inLine; cout << endl; while(getline(m_inFile, inLine)) { cout << inLine << endl; ++total; } return total; }// end of DisplayFileContents void CFileDisp::CloseFile() { m_inFile.close(); }// end of CloseFile // this is the destructor CFileDisp::~CFileDisp() { m_bIsValid = 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Enter a filename: input_file_display_programmingnotes_freeweq_com.txt 346 130 982 90 656 117 595 415 948 126 4 558 571 87 42 360 412 721 463 47 119 441 190 985 214 509 2 571 77 81 681 651 995 93 74 310 9 995 561 92 14 288 466 664 892 8 766 34 639 151 64 98 813 67 834 369 This is a line of text! *** Total lines displayed: 10 |
C++ || Class – Roman Numeral To Integer & Integer To Roman Numeral Conversion
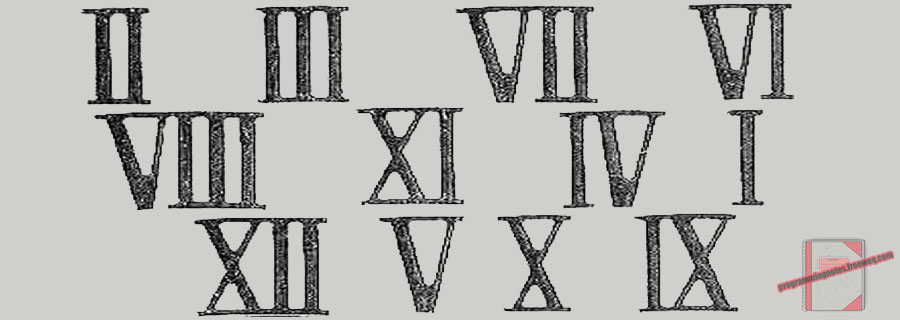
The following is another homework assignment which was presented in a C++ Data Structures course. This program was assigned in order to practice the use of the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
Do/While Loop
Passing a Value By Reference
Roman Numerals - How Do You Convert To Decimal?
Online Roman Numeral Converter - Check For Correct Results
This is an interactive program in which the user has the option of selecting from 7 modes of operation. Of those modes, the user has the option of entering in roman numerals for conversion, entering in decimal numbers for conversion, displaying the recently entered number, and finally converting between roman or decimal values.
A sample of the menu is as followed:
(Where the user would enter numbers 1-7 to select a choice)
1 2 3 4 5 6 7 8 9 10 11 |
From the following menu: 1. Enter a Decimal number 2. Enter a Roman Numeral 3. Convert from Decimal to Roman 4. Convert from Roman to Decimal 5. Print the current Decimal number 6. Print the current Roman Numeral 7. Quit Please enter a selection: |
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
======== FILE #1 – Menu.cpp ========
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
// ============================================================================ // File: Menu.cpp // Purpose: Converts a number enetered in Decimal to Roman Numeral and a // Roman Numeral to a Decimal Number // ============================================================================ #include <iostream> #include <iomanip> #include <string> #include "ClassRomanType.h" using namespace std; // function prototypes void DisplayMenu(); int GetCommand(int command); void RunChoice(int command, ClassRomanType& romanObject); int main() { int command=7; // Holds which action the user chooses to make ClassRomanType romanObject; // Gives access to the class do{ DisplayMenu(); command = GetCommand(command); RunChoice(command, romanObject); cout<<"n--------------------------------------------------------------------n"; }while(command!=7); cout<<"nBye!n"; return 0; }// End of main void RunChoice(int command, ClassRomanType& romanObject) {// Function: Execute the choice selected from the menu // declare private variables int decimalNumber=0; // Holds decimal value char romanNumeral[150]; //Holds value of roman numeral // execute the desired choice cout <<"ntYou selected choice #"<<command<<" which will: "; switch(command) { case 1: cout << "Get Decimal Numbernn"; cout <<"Enter a Decimal Number: "; cin >> decimalNumber; romanObject.GetDecimalNumber(decimalNumber); break; case 2: cout << "Get Roman Numeralnn"; cout <<"nEnter a Roman Numeral: "; cin >> romanNumeral; romanObject.GetRomanNumeral(romanNumeral); break; case 3: cout << "Convert from Decimal to Romannn"; romanObject.ConvertDecimalToRoman(); cout<<"Running....nComplete!n"; break; case 4: cout << "Convert from Roman to Decimalnn"; romanObject.ConvertRomanToDecimal(); cout<<"Running....nComplete!n"; break; case 5: cout << "Print the current Decimal numbernn"; cout<<"n The current Roman to Decimal Value is: "; cout << romanObject.ReturnDecimalNumber()<<endl; break; case 6: cout << "Print the current Roman Numeralnn"; cout<<"n The current Decimal to Roman Value is: "; cout << romanObject.ReturnRomanNumber()<<endl; break; case 7: cout << "Quitnn"; break; default: cout << "nError, you entered an invalid command!nPlease try again..."; break; } }//End of RunChoice int GetCommand(int command) {// Function: Gets choice from menu cout <<"nPlease enter a selection: "; cin>> command; return command; }// End of GetCommand void DisplayMenu() {// Function: Displays menu cout <<"From the following menu:n"<<endl; cout <<left<<setw(2)<<"1."<<left<<setw(15)<<" Enter a Decimal number"<<endl; cout <<left<<setw(2)<<"2."<<left<<setw(15)<<" Enter a Roman Numeral"<<endl; cout <<left<<setw(2)<<"3."<<left<<setw(15)<<" Convert from Decimal to Roman"<<endl; cout <<left<<setw(2)<<"4."<<left<<setw(15)<<" Convert from Roman to Decimal"<<endl; cout <<left<<setw(2)<<"5."<<left<<setw(15)<<" Print the current Decimal number"<<endl; cout <<left<<setw(2)<<"6."<<left<<setw(15)<<" Print the current Roman Numeral"<<endl; cout <<left<<setw(2)<<"7."<<left<<setw(15)<<" Quit"<<endl; }// http://programmingnotes.org/ |
======== FILE #2 – ClassRomanType.h ========
Remember, you need to name the header file the same as the #include from the Menu.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
// ============================================================================ // File: ClassRomanType.h // Purpose: Header file for Roman Numeral conversion. It contains all // declarations and prototypes for the class members to carry out the // conversion // ============================================================================ #include <string> using namespace std; class ClassRomanType { public: ClassRomanType(); /* Function: constructor initializes class variables to "0" Precondition: none Postcondition: defines private variables */ // member functions void GetDecimalNumber(int decimalNumber); /* Function: gets decimal number Precondition: none Postcondition: Gets arabic number from user */ void GetRomanNumeral(char romanNumeral[]); /* Function: gets roman numeral Precondition: none Postcondition: Gets roman numeral from user */ void ConvertDecimalToRoman(); /* Function: converts decimal number to ronman numeral Precondition: arabic and roman numeral characters should be known Postcondition: Gets roman numeral from user */ void ConvertRomanToDecimal(); /* Function: converts roman numeral to arabic number Precondition: arabic and roman numeral characters should be known Postcondition: Gets decimal number from user */ int ReturnDecimalNumber(); /* Function: displays converted decimal number Precondition: the converted roman numeral to decimal number Postcondition: displays decimal number to user */ string ReturnRomanNumber(); /* Function: displays converted roman numeral Precondition: the converted decimal number to roman numeral Postcondition: displays roman numeral to user */ ~ClassRomanType(); /* Function: destructor used to return memory to the system Precondition: the converted decimal number to roman numeral Postcondition: none */ private: char m_romanValue[150]; // An array holding the roman value within the class int m_decimalValue; // Holds the final output answer of the roman to decimal conversion }; // http://programmingnotes.org/ |
======== FILE #3 – RomanType.cpp ========
This is the function implementation file for the ClassRomanType.h class. This file can be named anything you wish as long as you #include “ClassRomanType.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 |
// ============================================================================ // File: RomanType.cpp // Purpose: Contains the implementation of the class members for the // roman numeral conversion // // *NOTE*: Uncomment cout statements to visualize whats happening // ============================================================================ #include <iostream> #include <string> #include <cstring> #include "ClassRomanType.h" using namespace std; ClassRomanType::ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// end of ClassRomanType void ClassRomanType:: GetDecimalNumber(int decimalNumber) { m_decimalValue = decimalNumber; }// end of GetDecimalNumber void ClassRomanType:: GetRomanNumeral(char romanNumeral[]) { strcpy(m_romanValue,romanNumeral); }// end of GetRomanNumeral void ClassRomanType:: ConvertDecimalToRoman() { strcpy(m_romanValue,""); // erases any previous data int numericValue[]={1000,900,500,400,100,90,50,40,10,9,5,4,1,0}; char *romanNumeral[]={"M","CM","D","CD","C","XC","L" ,"XL","X","IX","V","IV","I",0}; for (int x=0; numericValue[x] > 0; ++x) { //cout<<endl<<numericValue[x]<<endl; while (m_decimalValue >= numericValue[x]) { //cout<<"current m_decimalValue = " <<m_decimalValue<<endl; //cout<<"current numericValue = "<<numericValue[x]<<endl<<endl; if(strlen(m_romanValue)==0) { strcpy(m_romanValue,romanNumeral[x]); } else { strcat(m_romanValue,romanNumeral[x]); } m_decimalValue -= numericValue[x]; } } }// end of ConvertDecimalToRoman void ClassRomanType:: ConvertRomanToDecimal() { int currentNumber = 0; // Holds value of each letter numerical value int previousNumber = 0; // Holds the numerical value being compared m_decimalValue=0; // Resets the current 'romanToDecimalValue' to zero if(strcmp(m_romanValue,"Currently Undefined")!=0) { for (int counter = strlen(m_romanValue)-1; counter >= 0; counter--) { if ((m_romanValue[counter] == 'M') || (m_romanValue[counter] == 'm')) { currentNumber = 1000; } else if ((m_romanValue[counter] == 'D') || (m_romanValue[counter] == 'd')) { currentNumber = 500; } else if ((m_romanValue[counter] == 'C') || (m_romanValue[counter] == 'c')) { currentNumber = 100; } else if ((m_romanValue[counter] == 'L') || (m_romanValue[counter] == 'l')) { currentNumber = 50; } else if ((m_romanValue[counter] == 'X') || (m_romanValue[counter] == 'x')) { currentNumber = 10; } else if ((m_romanValue[counter] == 'V') || (m_romanValue[counter] == 'v')) { currentNumber = 5; } else if ((m_romanValue[counter] == 'I') || (m_romanValue[counter] == 'i')) { currentNumber = 1; } else { currentNumber = 0; } //cout<<"previousNumber = " <<previousNumber<<endl; //cout<<"currentNumber = " <<currentNumber<<endl; if (previousNumber > currentNumber) { m_decimalValue -= currentNumber; previousNumber = currentNumber; } else { m_decimalValue += currentNumber; previousNumber = currentNumber; } //cout<<"current m_decimalValue = " <<m_decimalValue<<endl<<endl; } } }// end of ConvertRomanToDecimal int ClassRomanType::ReturnDecimalNumber() { return m_decimalValue; }// end of ReturnDecimalNumber string ClassRomanType::ReturnRomanNumber() { return m_romanValue; }// end of ReturnRomanNumber ClassRomanType::~ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
From the following menu:
1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 9
You selected choice #9 which will:
Error, you entered an invalid command!
Please try again...
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 0
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: Currently Undefined
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 1
You selected choice #1 which will: Get Decimal Number
Enter a Decimal Number: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 3
You selected choice #3 which will: Convert from Decimal to Roman
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: MCMLXXXVII
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 2
You selected choice #2 which will: Get Roman Numeral
Enter a Roman Numeral: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 4
You selected choice #4 which will: Convert from Roman to Decimal
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 2012
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 7
You selected choice #7 which will: Quit
--------------------------------------------------------------------
Bye!
C++ || Savings Account Balance – Calculate The Balance Of A Savings Account At The End Of A Period
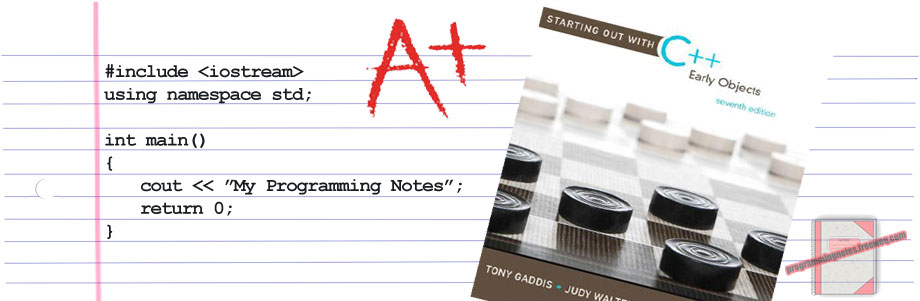
Here is another actual homework assignment which was presented in an intro to programming class. The following program was a question taken from the book “Starting Out with C++: Early Objects (7th Edition),” chapter 5, problem #16.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
For Loops - How To Use Them
Assignment Operators - What Are They?
Setprecision - What Is It?
Interest Rate
This program first prompts the user to enter the annual interest rate, starting balance, and the number of months which has passed since the account was established. Upon obtaining the information, a loop is then used to iterate through each month, performing the following:
• Ask the user for the amount deposited into the account during the month (positive values only). This amount is added to the balance.
• Ask the user for the amount withdrawn from the account during the month (positive values only). This is subtracted from the balance.
• Calculate the monthly interest. The monthly interest rate is the annual interest rate divided by 12. After each loop iteration, the monthly interest rate is multiplied by the current balance, which is then added to the total balance.
After the last iteration, the program displays the ending balance, the total amount of deposits, the total amount of withdrawals, and the total interest earned.
If a negative balance is calculated at any point, an erroneous message is displayed to the screen indicating that the account has been closed, and then the program terminates.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 |
// ============================================================================ // Author: Kenneth Perkins // Date: April 4, 2012 // Taken From: http://programmingnotes.org/ // File: SavingsAccount.cpp // Description: Demonstrates how to calculate the balance of a savings // account at the end of a period // ============================================================================ #include <iostream> #include <cstdlib> #include <iomanip> using namespace std; int main() { // declare variables double annInterestRate = 0; double startingBalance = 0; int monthsPassed = 0; double deposits = 0; double withdrawal = 0; double totDeposits = 0; double totWithdrawal = 0; double totInterest = 0; double monthlyInterestRate = 0; double totBalance = 0; // obtain data from user cout<<"Enter the annual interest rate on the account (e.g .04): "; cin >> annInterestRate; cout<<"Enter the starting balance: $"; cin >> startingBalance; cout<<"How many months have passed since the account was established? "; cin >> monthsPassed; // initialize variables with the required info totBalance = startingBalance; monthlyInterestRate = annInterestRate/12; // use a loop to obtain more data from user for(int x=1; x <= monthsPassed; ++x) { cout<<"\nMonth #"<<x<<endl; cout<<"\tTotal deposits for this month: $"; cin >> deposits; totDeposits += deposits; totBalance += deposits; cout<<"\tTotal withdrawal for this month: $"; cin >> withdrawal; totWithdrawal += withdrawal; totBalance -= withdrawal; totInterest += (totBalance*monthlyInterestRate); totBalance += (totBalance*monthlyInterestRate); // check for negative values // end loop if the values are negative if(deposits < 0 || withdrawal < 0 || totBalance < 0) { cout<< "\nPlease enter positive numbers only!"; cout<<"\nThe account has been closed..\n"; exit(1); } } // display results cout<<fixed<<setprecision(2); cout.fill('.'); cout<<"\nEnding balance:"<<left<<setw(20)<<right<<setw(20)<<" $ "<<totBalance; cout<<"\nAmount of deposits:"<<left<<setw(20)<<right<<setw(16)<<" $ "<<totDeposits; cout<<"\nAmount of withdrawals:"<<left<<setw(20)<<right<<setw(13)<<" $ "<<totWithdrawal; cout<<"\nAmount of interest earned:"<<left<<setw(20)<<right<<setw(9)<<" $ "<<totInterest<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter the annual interest rate on the account (e.g .04): .07
Enter the starting balance: $5000
How many months have passed since the account was established? 3Month #1
Total deposits for this month: $500
Total withdrawal for this month: $120Month #2
Total deposits for this month: $750
Total withdrawal for this month: $200Month #3
Total deposits for this month: $500
Total withdrawal for this month: $100Ending balance:................. $ 6433.47
Amount of deposits:............. $ 1750.00
Amount of withdrawals:.......... $ 420.00
Amount of interest earned:...... $ 103.47
C++ || Stack Based Infix To Postfix Conversion (Single Digit)
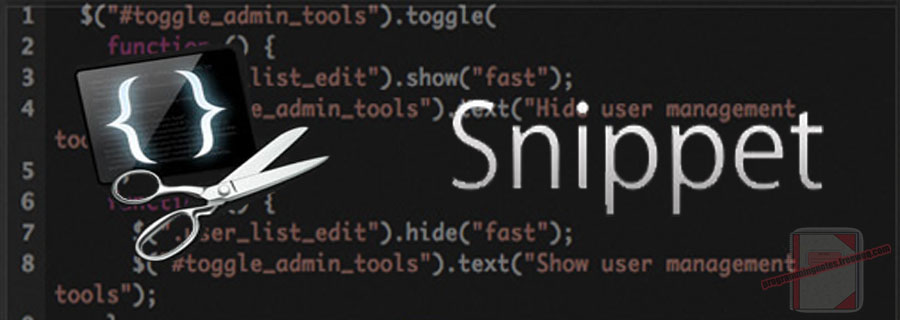
This page consists of another homework assignment which was presented in a C++ Data Structures course. No matter which institution you attend, it seems every instructor assigns a program similar to this at one time or another.
Want to evaluate a postfix expression? Click here.
Want to convert & evaluate multi digit, decimal, and negative numbers? Click here!
REQUIRED KNOWLEDGE FOR THIS PROGRAM
What Is Infix?
What Is Postfix?
Stack Data Structure
Cin.getline
How To Convert To Postfix
The Order Of Operations
#include "ClassStackType.h"
The program demonstrated on this page has the ability to convert a normal infix equation to postfix equation, so for example, if the user enters the infix equation of (1*2)+3, the program will display the postfix result of 12*3+.
Before we get into things, here is a helpful algorithm for converting from infix to postfix in pseudo code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// An algorithm for infix to postfix expression conversion. // For example, a + b - c translates to a b + c - // a + b * c translates to a b c * + // (1 + 2) / (5 + 6) goes to 1 2 + 5 6 + / // Valid operands are single digits: 0-9, a-z, A-Z // Valid operators are: +, -, *, /, (, ), ^, $ // Highest precedence: ^, $ // Lowest precedence: +,- // ) never goes on stack. // ( has lowest precedence on the stack and highest precedence outside of stack. // Bottom of the stack has the lowest precedence than any operator. // Use a prec() function to compare the precedence of the operators based on the above rules. // Note there is little error checking in the algorithm! void ConvertInfixToPostfix(string infix) { while there is input { if input is a number or a letter place onto postfix string else if input is '(' // '(' has lowest precedence in the stack, highest outside push input on stack else if input is ')' while stack is not empty and top of stack is not '(' place item from top of stack onto postfix string pop stack if stack is not empty // pops '(' off the stack pop stack else error // no matching '(' else if input is a math operator if stack is empty push input on stack else if prec(top of stack) >= prec(current math operator) while stack is not empty and prec(top of stack) >= prec(current math operator) place item from top of stack onto postfix string pop stack push current math operator on stack else error } while stack is not empty { place item from top of stack onto postfix string pop stack } }// http://programmingnotes.org/ |
======= INFIX TO POSTFIX CONVERSION =======
This program uses a custom template.h class. To obtain the code for that class, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 |
// ============================================================================ // Author: Kenneth Perkins // Date: Mar 23, 2012 // Taken From: http://programmingnotes.org/ // File: PostfixConversion.cpp // Description: Demonstrate the use of a stack based infix to // postfix conversion. // ============================================================================ #include <iostream> #include <cctype> #include <cstdlib> #include <cstring> #include "ClassStackType.h" using namespace std; // function prototypes void DisplayDirections(); void ConvertInfixToPostfix(char* infix); int OrderOfOperations(char token); bool IsMathOperator(char token); int main() { // declare variables char expression[50]; // array holding the infix data // display directions to user DisplayDirections(); // get data from user cout<<"\nPlease enter an infix expression: "; cin.getline(expression, sizeof(expression)); cout <<"\nThe Infix expression = "<<expression<<endl; ConvertInfixToPostfix(expression); cout<<"The Postfix expression = "<<expression<<endl; return 0; }// end of main void DisplayDirections() { cout << "\n==== Infix to Postfix Conversion ====\n" <<"\nMath Operators:\n" <<"+ || Addition\n" <<"- || Subtraction\n" <<"* || Multiplication\n" <<"/ || Division\n" <<"% || Modulus\n" <<"^ || Power\n" <<"$ || Square Root\n" <<"Sample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)\n"; }// end of DisplayDirections void ConvertInfixToPostfix(char* infix) { // declare function variables int infixCounter = 0; int postfixCounter = 0; char token = 'a'; char postfix[50]; StackType<char> charStack; // loop thru array until there is no more data while(infix[infixCounter] != '\0') { // push numbers/letters onto 'postfix' array if(isdigit(infix[infixCounter]) || isalpha(infix[infixCounter])) { postfix[postfixCounter] = infix[infixCounter]; ++postfixCounter; } else if(isspace(infix[infixCounter])) { // DO NOTHING } else if(IsMathOperator(infix[infixCounter])) { // if stack is empty, place first math operator onto stack token = infix[infixCounter]; if(charStack.IsEmpty()) { charStack.Push(token); } else { // get the current math operator from the top of the stack token = charStack.Top(); charStack.Pop(); // use the 'OrderOfOperations' function to check equality // of the math operators while(OrderOfOperations(token) >= OrderOfOperations(infix[infixCounter])) { // if stack is empty, do nothing if(charStack.IsEmpty()) { break; } // place the popped math operator from above ^ // onto the postfix array else { postfix[postfixCounter] = token; ++postfixCounter; // pop the next operator from the stack and // continue the process until complete token = charStack.Top(); charStack.Pop(); } } // push any remainding math operators onto the stack charStack.Push(token); charStack.Push(infix[infixCounter]); } } // push outer parentheses onto stack else if(infix[infixCounter] == '(') { charStack.Push(infix[infixCounter]); } else if(infix[infixCounter] == ')') { // pop the current math operator from the stack token = charStack.Top(); charStack.Pop(); while(token != '(' && !charStack.IsEmpty()) { // place the math operator onto the postfix array postfix[postfixCounter] = token; ++postfixCounter; // pop the next operator from the stack and // continue the process until complete token = charStack.Top(); charStack.Pop(); } } else { cout<<"\nINVALID INPUT\n"; exit(1); } ++infixCounter; } // place any remaining math operators from the stack onto // the postfix array while(!charStack.IsEmpty()) { postfix[postfixCounter] = charStack.Top(); ++postfixCounter; charStack.Pop(); } postfix[postfixCounter] = '\0'; // copy the data from the postfix array into the infix array // the data in the infix array gets sent back to main // since the array is passed by reference strcpy(infix,postfix); }// end of ConvertInfixToPostfix int OrderOfOperations(char token) {// this function checks priority of each math operator int priority = 0; if(token == '^'|| token == '$') { priority = 4; } else if(token == '*' || token == '/' || token == '%') { priority = 3; } else if(token == '-') { priority = 2; } else if(token == '+') { priority = 1; } return priority; }// end of OrderOfOperations bool IsMathOperator(char token) {// this function checks if operand is a math operator switch(token) { case '+': return true; break; case '-': return true; break; case '*': return true; break; case '/': return true; break; case '%': return true; break; case '^': return true; break; case '$': return true; break; default: return false; break; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Want to convert & evaluate multi digit, decimal, and negative numbers? Click here!
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Want to evaluate a postfix expression? Click here for sample code.
Once compiled, you should get this as your output
(Note: the code was compile three separate times to display different output)
====== RUN 1 ======
==== Infix to Postfix Conversion ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
Please enter an infix expression: ((a+b)+c)/(d^e)
The Infix expression = ((a+b)+c)/(d^e)
The Postfix expression = ab+c+de^/====== RUN 2 ======
==== Infix to Postfix Conversion ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
Please enter an infix expression: (3*5)+(7^6)
The Infix expression = (3*5)+(7^6)
The Postfix expression = 35*76^+====== RUN 3 ======
==== Infix to Postfix Conversion ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
Please enter an infix expression: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
The Infix expression = (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
The Postfix expression = 45^14*232+$2-/12%24*/*
Java || Using If Statements, Char & String Variables
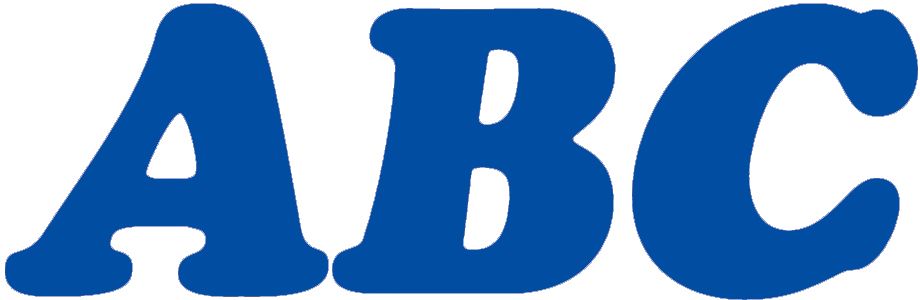
As previously mentioned, you can use the “int/float/double” data type to store numbers. But what if you want to store letters? Char and Strings help you do that.
===== SINGLE CHAR =====
This example will demonstrate a simple program using char, which checks to see if you entered the correctly predefined letter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: GuessALetter.java // Description: Demonstrates using char variables // ============================================================================ import java.util.Scanner; public class GuessALetter { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char userInput = ' '; System.out.print("Please try to guess the letter I am thinking of: "); // get a single character from the user data input userInput = cin.next().charAt(0); // Use an If Statement to check equality if (userInput == 'a' || userInput == 'A') { System.out.println("You have Guessed correctly!"); } else { System.out.println("Sorry, that was not the correct letter I was thinking of"); } }// end main }// http://programmingnotes.org/ |
Notice in line 19 I declare the char data type, naming it “userInput.” I also initialized it as an empty variable. In line 26 I used an “If/Else Statement” to determine if the user inputted value matches the predefined letter within the program. I also used the “OR” operator in line 26 to determine if the letter the user inputted was lower or uppercase. Try compiling the program simply using this
if (userInput == 'a')
as your if statement, and notice the difference.
The resulting code should give this as output
Please try to guess the letter I am thinking of: A
You have Guessed correctly!
===== CHECK IF LETTER IS UPPER CASE =====
This example is similar to the previous one, and will check if a letter is uppercase
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: CheckIfUppercase.java // Description: Demonstrates checking if a char variable is uppercase // ============================================================================ import java.util.Scanner; public class CheckIfUppercase { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char userInput = ' '; System.out.print("Please enter an UPPERCASE letter: "); // get a single character from the user data input userInput = cin.next().charAt(0); // Checks to see if inputted data falls between uppercase values if ((userInput >= 'A') && (userInput <= 'Z')) { System.out.println(userInput + " is an uppercase letter"); } else { System.out.println(userInput + " is not an uppercase letter"); } }// end main }// http://programmingnotes.org/ |
Notice in line 26, an If statement was used, which checked to see if the user inputted data fell between letter A and letter Z. We did that by using the “AND” operator. So that IF statement is basically saying (in plain english)
IF ('userInput' is equal to or greater than 'A') AND ('userInput' is equal to or less than 'Z')
THEN it is an uppercase letter
The resulting code should give this as output
Please enter an UPPERCASE letter: p
p is not an uppercase letter
===== CHECK IF LETTER IS A VOWEL =====
This example will utilize more if statements, checking to see if the user inputted data is a vowel or not. This will be very similar to the previous example, utilizing the OR operator once again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: CheckIfVowel.java // Description: Demonstrates checking if a char variable is a vowel // ============================================================================ import java.util.Scanner; public class CheckIfVowel { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char userInput = ' '; System.out.print("Please enter a vowel: "); // get a single character from the user data input userInput = cin.next().charAt(0); // Checks to see if entered data is A,E,I,O,U,Y if ((userInput == 'a')||(userInput == 'A')||(userInput == 'e')|| (userInput == 'E')||(userInput == 'i')||(userInput == 'I')|| (userInput == 'o')||(userInput == 'O')||(userInput == 'u')|| (userInput == 'U')||(userInput == 'y')||(userInput == 'Y')) { System.out.println("Correct, " + userInput + " is a vowel!"); } else { System.out.println("Sorry, " + userInput + " is not a vowel"); } }// end main }// http://programmingnotes.org/ |
This program should be very straight forward, and its basically checking to see if the user entered data is the letter A, E, I, O, U or Y.
The resulting code should give the following output
Please enter a vowel: o
Correct, o is a vowel!
===== HELLO WORLD v2 =====
This last example will demonstrate using the string data type to print the line “Hello World!” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: HelloWorldString.java // Description: Demonstrates using string variables // ============================================================================ import java.util.Scanner; public class HelloWorldString { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables String userInput = ""; System.out.print("Please enter a sentence: "); // get a string from the user userInput = cin.nextLine(); // display message to user System.out.println("You entered: " + userInput); }// end main }// http://programmingnotes.org/ |
The resulting code should give following output
Please enter a sentence: Hello World!
You entered: Hello World!
===== HOW TO COMPILE CODE USING THE TERMINAL =====
*** This can be achieved by typing the following command:
(Notice the .java source file is named exactly the same as the class header)
javac YOUR_CLASS_NAME.java
*** To run the compiled program, simply type this command:
java YOUR_CLASS_NAME
C++ || Snippet – How To Find The Highest & Lowest Numbers Contained In An Integer Array
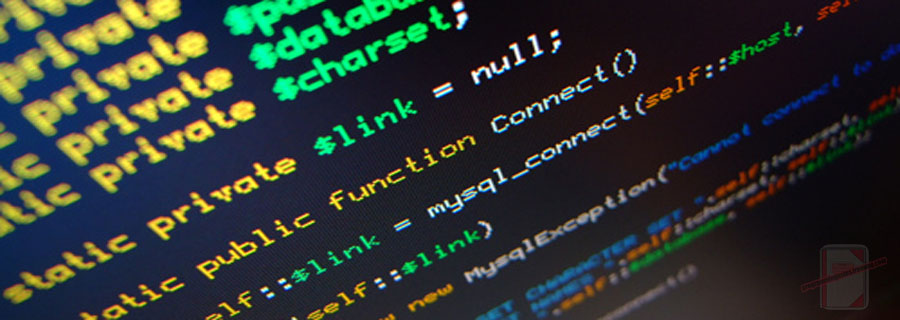
This page will consist of a simple demonstration for finding the highest and lowest numbers contained in an integer array.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Finding the highest/lowest values in an array can be found in one or two ways. The first way would be via a sort, which would render the highest/lowest numbers contained in the array because the values would be sorted in order from highest to lowest. But a sort may not always be practical, especially when you want to keep the array values in the same order that they originally came in.
The second method of finding the highest/lowest values is by traversing through the array, checking each value it contains one by one to determine if the current number which is being compared truly is a target value or not. That method will be displayed below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 12, 2012 // Taken From: http://programmingnotes.org/ // File: arrayMinMax.cpp // Description: Determines the highest and lowest value in an array // ============================================================================ #include <iostream> #include <iomanip> #include <ctime> using namespace std; const int NUM_INTS = 14; int main() { // declare variables int arry[NUM_INTS]; // array is initialized using a const variable srand(time(NULL)); // place random numbers into the array for (int x = 0; x < NUM_INTS; ++x) { arry[x] = rand() % 100 + 1; } cout << "Original array values" << endl; // Output the original array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } // creates a line separator cout << "\n--------------------------------------------------------"; // use a for loop to go thru the array checking to see the highest/lowest element cout << "\nThese are the highest and lowest array values: "; // set the lowest/highest to the first array item (if any) int lowestScore = NUM_INTS > 0 ? arry[0] : 0; int highestScore = lowestScore; for (int index = 1; index < NUM_INTS; ++index) { // if current score in the array is bigger than the current 'highestScore' // element, then set 'highestScore' equal to the current array element if (arry[index] > highestScore) { highestScore = arry[index]; } // if current score in the array is smaller than the current 'lowestScore' // element, then set 'lowestScore' equal to the current array element if (arry[index] < lowestScore) { lowestScore = arry[index]; } }// end for loop // display the results to the user cout << "\n\tHighest: " << highestScore; cout << "\n\tLowest: " << lowestScore; cout << endl; cin.get(); return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Original array values
10 14 1 94 29 25 7 95 11 17 6 71 100 59
--------------------------------------------------------
These are the highest and lowest array values:
Highest: 100
Lowest: 1