C++ || Modulus – Celsius To Fahrenheit Conversion Displaying Degrees Divisible By 10 Using Modulus
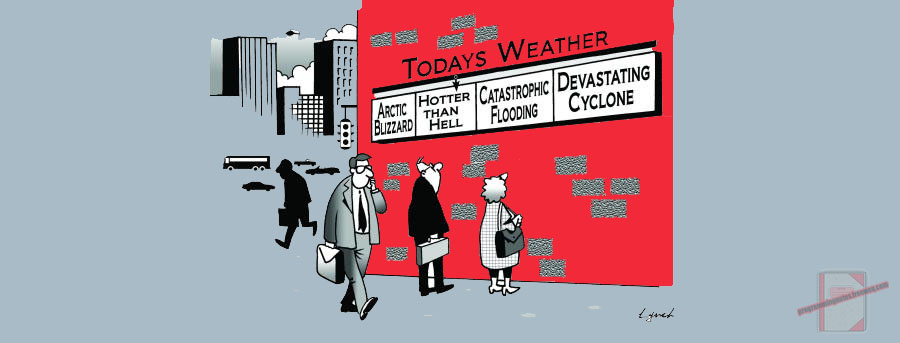
This page will consist of two simple programs which demonstrate the use of the modulus operator (%).
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Modulus
Do/While Loop
Functions
Simple Math - Divisibility
Celsius to Fahrenheit Conversion
===== FINDING THE DIVISIBILITY OF A NUMBER =====
Take a simple arithmetic problem: what’s left over when you divide an odd number by an even number? The answer may not be easy to compute, but we know that it will most likely result in an answer which has a decimal remainder. How would we determine the divisibility of a number in a programming language like C++? That’s where the modulus operator comes in handy.
To have divisibility means that when you divide the first number by another number, the quotient (answer) is a whole number (i.e – no decimal values). Unlike the division operator, the modulus operator (‘%’), has the ability to give us the remainder of a given mathematical operation that results from performing integer division.
To illustrate this, here is a simple program which prompts the user to enter a number. Once the user enters a number, they are asked to enter in a divisor for the previous number. Using modulus, the program will determine if the second number is divisible by the first number. If the modulus result returns 0, the two numbers are divisible. If the modulus result does not return 0, the two numbers are not divisible. The program will keep re-prompting the user to enter in a correct choice until a correct result is obtained.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
#include <iostream> using namespace std; int main() { // declare variables int base=0; int userInput=0; double multiple =0; // get first number cout <<"Please enter a value: "; cin >> base; // get second number cout << "nPlease enter a factor of "<<base<<": "; cin >> userInput; do{ // loop will keep going until the user enters a correct answer // this is the modulus declaration, which will find the // remainder between the 2 numbers multiple = base % userInput; // if the modulus result returns 0, the 2 numbers // are divisible if (multiple == 0) { cout <<"nCorrect! " << base << " is divisible by " << userInput <<endl; cout <<"n("<< base << "/" << userInput <<") = "<<(base/userInput)<<endl; } // if the user entered an incorrect choice, promt an error message else { cout << "nIncorrect, " << base <<" is not divisible by " << userInput <<".nPlease enter a new multiple integer for that value: "; cin >> userInput; } }while(multiple != 0); // ^ loop stops once user enters correct choice return 0; }// http://programmingnotes.org/ |
The above program determines if number ‘A’ is divisible be number ‘B’ via modulus. Unlike the division operator, which does not return the remainder of a number, the modulus operator does, thus we are able to find divisibility between two numbers.
To demonstrate the above code, here is a sample run:
Please enter a value: 21
Please enter a factor of 21: 5Incorrect, 21 is not divisible by 5.
Please enter a new multiple integer for that value: 7Correct! 21 is divisible by 7
(21/7) = 3
===== CELSIUS TO FAHRENHEIT CONVERSION =====
Now that we understand how modulus works, the second program shouldn’t be too difficult. This function first prompts the user to enter in an initial (low) value. After the program obtains the low value from the user, the program will ask for another (high) value. After it obtains the needed information, it displays all the degrees, from the range of the low number to the high number, which are divisible by 10. So if the user enters a low value of 3 and a high value of 303, the program will display all of the Celsius to Fahrenheit degrees within that range which are divisible by 10.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
#include <iostream> using namespace std; // function prototype double ConvertCelsiusToFahrenheit(int degree); int main() { // declare variables int low=0; int high=0; double degreeFahrenhiet =0; double multiple =0; // get data from user cout << "Enter a low number: "; cin >> low; cout << "nEnter a high number: "; cin >> high; // displays data back to user in table form cout <<"nCelsius Fahrenheit:n"; cout << low << "t" << ConvertCelsiusToFahrenheit(low)<<endl; ++low; // this loop displays all the degrees that are divisible by 10 do{ // this converts the current number from celsius to fahrenhiet degreeFahrenhiet = ConvertCelsiusToFahrenheit(low); // this is the modulus operation which finds the // numbers which are divisible by 10 multiple = low % 10; // the program will only display the degrees to the user via // cout which are divisible by 10 if(multiple ==0) { cout << low << "t" << degreeFahrenhiet<<endl; } // this increments the current degree number by 1 ++low; }while(low < high); // ^ loop stops once the 'low' variable reaches the 'high' variable // displays the 'high' converted degrees to the user cout << high << "t" << ConvertCelsiusToFahrenheit(high)<<endl; return 0; }// end of main // function declaration which returns the converted celsius to fahrenhiet value double ConvertCelsiusToFahrenheit(int degree) { return ((1.8 * degree) + 32.0); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter a low number: 3
Enter a high number: 303Celsius Fahrenheit:
3..........37.4
10.........50
20.........68
30.........86
40........104
50........122
60........140
70........158
80........176
90........194
100.......212
110.......230
120.......248
130.......266
140.......284
150.......302
160.......320
170.......338
180.......356
190.......374
200.......392
210.......410
220.......428
230.......446
240.......464
250.......482
260.......500
270.......518
280.......536
290.......554
300.......572
303.......577.4
Leave a Reply