Tag Archives: do/while loop
Java || Modulus – Celsius To Fahrenheit Conversion Displaying Degrees Divisible By 10 Using Modulus
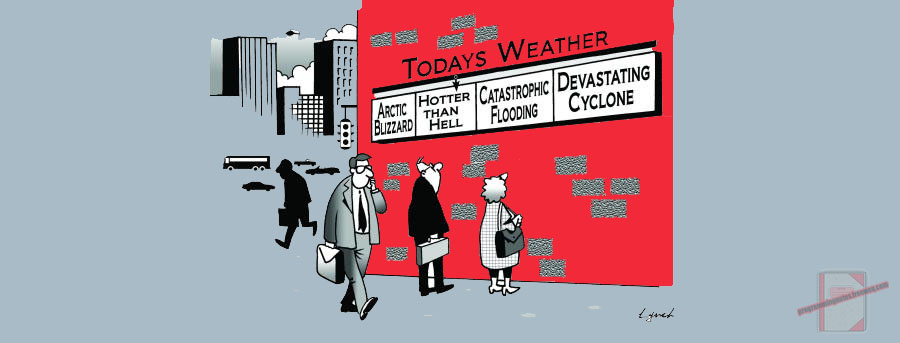
This page will consist of two simple programs which demonstrate the use of the modulus operator (%).
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Modulus
Do/While Loop
Methods (A.K.A "Functions") - What Are They?
Simple Math - Divisibility
Celsius to Fahrenheit Conversion
===== FINDING THE DIVISIBILITY OF A NUMBER =====
Take a simple arithmetic problem: what’s left over when you divide an odd number by an even number? The answer may not be easy to compute, but we know that it will most likely result in an answer which has a decimal remainder. How would we determine the divisibility of a number in a programming language like Java? That’s where the modulus operator comes in handy.
To have divisibility means that when you divide the first number by another number, the quotient (answer) is a whole number (i.e – no decimal values). Unlike the division operator, the modulus operator (‘%’), has the ability to give us the remainder of a given mathematical operation that results from performing integer division.
To illustrate this, here is a simple program which prompts the user to enter a number. Once the user enters a number, they are asked to enter in a divisor for the previous number. Using modulus, the program will determine if the second number is divisible by the first number. If the modulus result returns 0, the two numbers are divisible. If the modulus result does not return 0, the two numbers are not divisible. The program will keep re-prompting the user to enter in a correct choice until a correct result is obtained.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
import java.util.Scanner; public class Modulus { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare & initialize variables int numerator = 0; int denominator = 0; double multiple = 0; System.out.println("Welcome to My Programming Notes' Java Program.n"); // get first number System.out.print("Please enter a value: "); numerator = cin.nextInt(); // get second number System.out.print("nPlease enter a factor of "+numerator+": "); do{ // loop will keep going until the user enters a correct answer denominator = cin.nextInt(); // this is the modulus declaration, which will find the // remainder between the 2 numbers multiple = numerator % denominator; // if the modulus result returns 0, the 2 numbers // are divisible if(multiple == 0) { System.out.println("nCorrect! "+numerator+" is divisible by " +denominator); System.out.println("n("+numerator+"/"+denominator+") = " + ""+(numerator/denominator)); } // if the user entered an incorrect choice, promt an error message else { System.out.print("nIncorrect, "+numerator+" is not divisible by " +denominator); System.out.print(".nnPlease enter a new multiple integer for " +numerator+": "); } }while(multiple != 0); // ^ loop stops once user enters correct choice }// end of main }// http://programmingnotes.org/ |
The above program determines if number ‘A’ is divisible be number ‘B’ via modulus. Unlike the division operator, which does not return the remainder of a number, the modulus operator does, thus we are able to find divisibility between two numbers.
To demonstrate the above code, here is a sample run:
Welcome to My Programming Notes' Java Program.
Please enter a value: 21
Please enter a factor of 21: 5Incorrect, 21 is not divisible by 5.
Please enter a new multiple integer for 21: 7
Correct! 21 is divisible by 7(21/7) = 3
===== CELSIUS TO FAHRENHEIT CONVERSION DISPLAYING DEGREES DIVISIBLE BY 10 =====
Now that we understand how modulus works, the second program shouldn’t be too difficult. This function first prompts the user to enter in an initial (low) value. After the program obtains the low value from the user, the program will ask for another (high) value. After it obtains the needed information, it displays all the degrees, from the range of the low number to the high number, which are divisible by 10. So if the user enters a low value of 3 and a high value of 303, the program will display all of the Celsius to Fahrenheit degrees within that range which are divisible by 10.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
import java.util.Scanner; public class Temperature { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare & initialize variables int low = 0; int high = 0; double degreeFahrenhiet = 0; double multiple = 0; System.out.println("Welcome to My Programming Notes' Java Program.n"); // get data from user System.out.print("Enter a low number: "); low = cin.nextInt(); System.out.print("nEnter a high number: "); high = cin.nextInt(); // displays data back to user in table form System.out.print("nCelsius Fahrenheit:n"); // display the initial 'low' converted degrees to the user System.out.println(low+"t"+ConvertCelsiusToFahrenheit(low)); // this loop displays all the degrees that are divisible by 10 do{ // this increments the current degree number by 1 ++low; // this converts the current number from celsius to fahrenhiet degreeFahrenhiet = ConvertCelsiusToFahrenheit(low); // this is the modulus operation which finds the // numbers which are divisible by 10 multiple = low % 10; // the program will only display the degrees to the user via // cout which are divisible by 10 if(multiple == 0) { System.out.println(low+"t"+degreeFahrenhiet); } }while(low < high); // ^ loop stops once the 'low' variable reaches the 'high' variable // displays the 'high' converted degrees to the user System.out.println(high+"t"+ConvertCelsiusToFahrenheit(high)); }// end of main static double ConvertCelsiusToFahrenheit(int degree) { return ((1.8 * degree) + 32.0); }// end of ConvertCelsiusToFahrenheit }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Enter a low number: 3
Enter a high number: 303Celsius Fahrenheit:
3..........37.4
10.........50
20.........68
30.........86
40........104
50........122
60........140
70........158
80........176
90........194
100.......212
110.......230
120.......248
130.......266
140.......284
150.......302
160.......320
170.......338
180.......356
190.......374
200.......392
210.......410
220.......428
230.......446
240.......464
250.......482
260.......500
270.......518
280.......536
290.......554
300.......572
303.......577.4
Java || Random Number Guessing Game Using Random & Do/While Loop
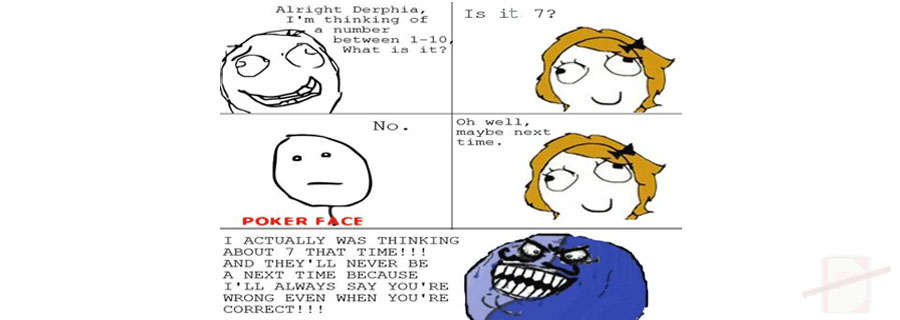
This is a simple guessing game, which demonstrates the use of the “Random” class to generate random numbers. This program first prompts the user to enter a number between 1 and 1000. Using if/else statements, the program will check to see if the user obtained number is higher/lower than the pre defined random number which is generated by the program. If the user makes a wrong guess, the program will re prompt the user to enter in a new number, where they will have a chance to enter in a new guess. Once the user finally guesses the correct answer, using a do/while loop, the program will ask if they want to play again. If the user selects yes, the game will start over, and a new random number will be generated. If the user selects no, the game will end.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
The "Random" Class
Do/While Loop
How To get Character Input
Custom Setw/Setfill In Java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
// ============================================================================ // Author: Kenneth Perkins // Date: Nov 19, 2012 // Taken From: http://programmingnotes.org/ // File: GuessingGame.java // Description: Demonstrates a simple random number guessing game // ============================================================================ import java.util.*; public class GuessingGame { // global variable declarations static Scanner cin = new Scanner(System.in); static Random rand = new Random(); // this is the call to the "Random" class public static void main(String[] args) { // declare & initialize variables char playAgain = 'y'; int userInput = 0; int numGuesses = 0; int randomNumber = rand.nextInt(1000)+1; // ^ get a number from the random generator in the range of 1 - 1000 System.out.println("Welcome to My Programming Notes' Java Program.\n"); // display directions to user System.out.println("I'm thinking of a number between 1 and 1000. Go " + "ahead and make your first guess.\n"); do { // this is the start of the do/while loop System.out.print(">> "); // get data from user userInput = cin.nextInt(); System.out.println(""); // increments the 'numGuesses' variable each time the user // gets the guess wrong ++numGuesses; // if user guess is too high, do this code if (userInput > randomNumber) { System.out.println("Too high! Think lower."); } // if user guess is too low, do this code else if (userInput < randomNumber) { System.out.println("Too low! Think higher."); } // if user guess is correct, do this code else { // display data to user, prompt if user wants to play again System.out.print("You got it, and it only took you " +numGuesses+" trys!\nWould you like to play again (y/n)? "); playAgain = cin.next().charAt(0); // if user wants to play again then re initialize the variables if (playAgain == 'y'|| playAgain == 'Y') { // creates a line seperator if user wants to enter new data System.out.println(""); setwRF("", 60, '-'); numGuesses = 0; System.out.println("\n\nMake a guess (between 1-1000):\n"); // generate a new random number for the user to try & guess randomNumber = rand.nextInt(1000)+1; } } System.out.println(""); } while (playAgain == 'y' || playAgain == 'Y'); // ^ do/while loop ends when user doesnt select 'Y' // display data to user System.out.println("Thanks for playing!!"); }// end of main public static void setwRF(String str, int width, char fill) { System.out.print(str); for (int x = str.length(); x < width; ++x) { System.out.print(fill); } }// end of setwRF }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Welcome to My Programming Notes' Java Program.
I'm thinking of a number between 1 and 1000. Go ahead and make your first guess.
>> 900
Too high! Think lower.
>> 300
Too high! Think lower.
>> 100
Too low! Think higher.
>> 200
Too low! Think higher.
>> 350
You got it, and it only took you 5 trys!
Would you like to play again (y/n)? y------------------------------------------------------------
Make a guess (between 1-1000):
>> 300
Too low! Think higher.
>> 600
Too high! Think lower.
>> 500
Too high! Think lower.
>> 400
You got it, and it only took you 4 trys!
Would you like to play again (y/n)? nThanks for playing!!
C++ || Char Array – Palindrome Number Checker Using A Character Array, Strlen, Strcpy, & Strcmp
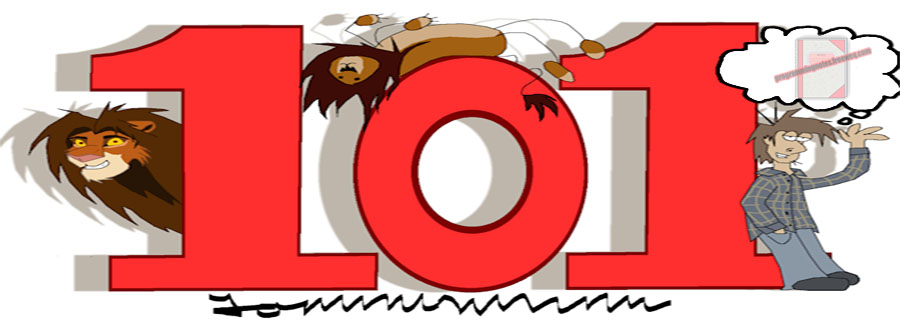
The following is a palindromic number checking program, which demonstrates more use of character array’s, Strlen, & Strcmp.
Want sample code for a palindrome checker which works for numbers and words? Click here.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Character Arrays
How to reverse a character array
Palindrome - What is it?
Strlen
Strcpy
Strcmp
Isdigit
Atoi - Convert a char array to a number
Do/While Loops
For Loops
This program first asks the user to enter a number that they wish to compare for similarity. If the number which was entered into the system is a palindrome, the program will prompt a message to the user via cout. This program determines similarity by using the strcmp function to compare two arrays together. Using a for loop, this program also demonstrates how to reverse a character array, aswell as demonstrates how to determine if the text contained in a character array is a number or not.
This program will repeatedly prompt the user for input until an “exit code” is obtained. The designated exit code in this program is the number 0 (zero). So the program will not stop asking for user input until the number 0 is entered into the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 10, 2012 // Taken From: http://programmingnotes.org/ // File: palindrome.cpp // Description: Palindrome number checker // ============================================================================ #include <iostream> #include <cctype> #include <cstring> #include <cstdlib> using namespace std; // function prototypes void Reverse(char arry[]); bool IsArryANum(char arry[]); // constant, which is the exit value const char* EXIT_VALUE = "0"; int main() { // declare variables char arry[80]; char arryReversed[80]; do{// get data from user using do/while loop cout<<"\nEnter a positive integer, or ("<<EXIT_VALUE<<") to exit: "; cin >> arry; if(atoi(arry) < 0) // check for negative numbers { cout <<"\n*** Error: "<<arry<<" must be greater than zero\n"; } else if(!IsArryANum(arry)) // check for any letters { cout <<"\n*** Error: "<<arry<<" is not an integer\n"; } else if(strcmp(EXIT_VALUE, arry) == 0) // check for "exit code" { cout <<"\nExiting program...\n"; } else // if all else is good, determine if number is a palindrome { // copy the user input from the first array (arry) // into the second array (arryReversed) strcpy(arryReversed, arry); // function call to reverse the contents inside the // "arryReversed" array to check for similarity Reverse(arryReversed); cout <<endl<<arry; // use strcmp to determine if the two arrays are the same if(strcmp(arryReversed, arry) == 0) { cout <<" is a Palindrome..\n"; } else { cout <<" is NOT a Palindrome!\n"; } } }while(strcmp(EXIT_VALUE, arry) != 0); // keep going until user enters the exit value cout <<"\nBYE!\n"; return 0; }// end of main void Reverse(char arry[]) { // get the length of the current word in the array index int length = strlen(arry)-1; // increment thru each letter within the current char array index // reversing the order of the array for (int currentChar=0; currentChar < length; --length, ++currentChar) { // copy 1st letter in the array index into temp char temp = arry[currentChar]; // copy last letter in the array index into the 1st array index arry[currentChar] = arry[length]; // copy temp into last array index arry[length] = temp; } }// end of Reverse bool IsArryANum(char arry[]) { // LOOP UNTIL U REACH THE NULL CHARACTER, // AKA THE END OF THE CHAR ARRAY for(int x=0; arry[x]!='\0'; ++x) { // if the current char isnt a number, // exit the loop & return false if(!isdigit(arry[x])) { return false; } } return true; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter a positive integer, or (0) to exit: L33T
*** error: "L33T" is not an integer
Enter a positive integer, or (0) to exit: -728
*** error: -728 must be greater than zero
Enter a positive integer, or (0) to exit: 1858
1858 is NOT a Palindrome!
Enter a positive integer, or (0) to exit: 7337
7337 is a Palindrome..
Enter a positive integer, or (0) to exit: 0
Exiting program...
BYE!
Java || Find The Prime, Perfect & All Known Divisors Of A Number Using A For, While & Do/While Loop
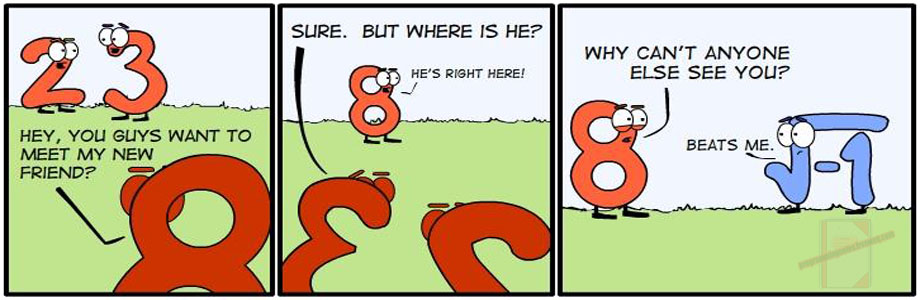
This program was designed to better understand how to use different loops which are available in Java, as well as more practice using objects with classes.
This program first asks the user to enter a non negative number. After it obtains a non negative integer from the user, the program determines if the user obtained number is a prime number or not, aswell as determining if the user obtained number is a perfect number or not. After it obtains its results, the program will display to the screen if the user inputted number is prime/perfect number or not. The program will also display a list of all the possible divisors of the user obtained number via stdout.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Class Objects - How TO Use
Constructors - What Are They?
Do/While Loop
While Loop
For Loop
Modulus
Basic Math - Prime Numbers
Basic Math - Perfect Numbers
Basic Math - Divisors
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 |
import java.util.Scanner; public class PrimePerfectNums { // global variable declaration int userInput = 0; static Scanner cin = new Scanner(System.in); public PrimePerfectNums(int input) {// this is the constructor // give the global variable a value userInput = input; }// end of PrimePerfectNums public void CalcPrimePerfect() { // declare variables int divisor = 0; int sumOfDivisors = 0; System.out.print("nInput number: " + userInput); // for loop adds sum of all possible divisors for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0) { // this will repeatedly add the found divisors together sumOfDivisors += counter; } } System.out.println(""); // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is prime if(userInput == (sumOfDivisors - 1)) { System.out.print(userInput + " is a prime number."); } else { System.out.print(userInput + " is not a prime number."); } System.out.println(""); // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is a perfect number if (userInput == (sumOfDivisors - userInput)) { System.out.print(userInput + " is a perfect number."); } else { System.out.print(userInput + " is not a perfect number."); } System.out.print("nDivisors of " + userInput + " are: "); // for loop lists all the possible divisors for the // 'userInput' variable by using the modulus operator for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0 && counter !=userInput) { System.out.print(counter + ", "); } } System.out.print("and " + userInput); }// end of CalcPrimePerfect public static void main(String[] args) { // declare variables int input = 0; char response ='n'; do{ // this is the start of the do/while loop System.out.print("Enter a number: "); input = cin.nextInt(); // if the user inputs a negative number, do this code while(input < 0) { System.out.print("ntSorry, but the number entered is less " + "than the allowable limit.ntPlease try again....."); System.out.print("nnEnter an number: "); input = cin.nextInt(); } // entry point to class declaration PrimePerfectNums myClass = new PrimePerfectNums(input); // function call to obtain data using the number // which was passed from main to the constructor myClass.CalcPrimePerfect(); // asks user if they want to enter new data System.out.print("nntDo you want to input another number?(Y/N): "); response = cin.next().toLowerCase().charAt(0); System.out.println("---------------------------" + "---------------------------------"); }while(response =='y'); // ^ End of the do/while loop. As long as the user chooses // 'Y' the loop will keep going. // It stops when the user chooses the letter 'N' System.out.println("BYE!"); }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Enter a number: 41
Input number: 41
41 is a prime number.
41 is not a perfect number.
Divisors of 41 are: 1, and 41Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 496Input number: 496
496 is not a prime number.
496 is a perfect number.
Divisors of 496 are: 1, 2, 4, 8, 16, 31, 62, 124, 248, and 496Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 1858Input number: 1858
1858 is not a prime number.
1858 is not a perfect number.
Divisors of 1858 are: 1, 2, 929, and 1858Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: -9Sorry, but the number entered is less than the allowable limit.
Please try again.....Enter an number: 12
Input number: 12
12 is not a prime number.
12 is not a perfect number.
Divisors of 12 are: 1, 2, 3, 4, 6, and 12Do you want to input another number?(Y/N): n
------------------------------------------------------------
BYE!
Java || Compute The Sum From A String Of Integers & Display Each Number Individually
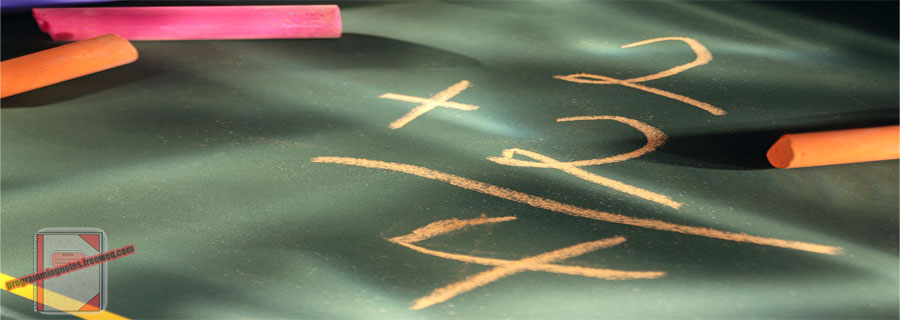
Here is a simple program, which was presented in a Java course. This program was used to introduce the input/output mechanisms which are available in Java. This assignment was modeled after Exercise 2.30, taken from the textbook “Java How to Program” (early objects) (9th Edition) (Deitel). It is the same exercise in both the 8th and 9th editions.
Our class was asked to make a program which prompts the user to enter a non-negative integer into the system. The program was supposed to then extract the digits from the inputted number, displaying them each individually, separated by a white space (” “). After the digits are displayed, the program was then supposed to display the sum of those digits to the screen. So for example, if the user inputted the number “39465,” the program would output the numbers 3 9 4 6 5 individually, and then the sum of 27.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Do/While Loops
For Loops
Methods (A.K.A "Functions") - What Are They?
ParseInt - Convert String To Integer
Substring
Try/Catch
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
import java.util.Scanner; import java.lang.Math; public class SumFromString { public static void main(String[] args) { // declare variables char question = 'n'; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); do{// this is the start of the do/while loop int sum = 0; // get data from the user System.out.print("nEnter a non negative integer: "); userInput = cin.nextLine(); System.out.println(""); // if the string isnt a number, dont compute the sum if(!IsNumeric(userInput)) { System.out.println(userInput+" is not a number..."); System.out.println("Please enter digits only!"); } // if the string is a negative number, dont compute the sum else if(IsNegative(userInput)) { System.out.println(userInput+" is a negative number..."); System.out.println("Please enter positive digits only!"); } // if the string is a decimal number (i.e 19.87), dont compute the sum else if(IsDecimal(userInput)) { System.out.println(userInput+" is a decimal number..."); System.out.println("Please enter positive whole numbers only!"); } // if everything else checks out OK, then compute the sum else { System.out.print("The digits are: "); sum = ComputeSum(userInput); System.out.println("and the sum is " + sum); } // ask user if they want to enter more data System.out.print("nDo you have more data for input? (Y/N): "); String response = cin.nextLine(); response=response.toLowerCase(); question = response.charAt(0); System.out.println("n------------------------------------------------"); }while(question == 'y'); System.out.print("nBYE!n"); }// end of main public static boolean IsNumeric(String str) {// checks to see if a string is a number try { Double.parseDouble(str); return true; } catch(NumberFormatException nfe) { return false; } }// end of IsNumeric public static boolean IsNegative(String str) {// checks to see if string is a negative number return Double.parseDouble(str) < 0; }// end of IsNegative public static boolean IsDecimal(String str) {// checks to see if string is a decimal number return (Math.floor(Double.parseDouble(str))) != Double.parseDouble(str); }// end of IsDecimal public static int ComputeSum(String userInput) {// displays each number individually & computes the sum of the string int sum = 0; String[] temp = new String[userInput.length()]; for(int counter=0; counter < userInput.length(); ++counter) { temp[counter] = userInput.substring(counter,counter+1); System.out.print(temp[counter] + " "); sum = sum + Integer.parseInt(temp[counter]); } return sum; }// end of ComputeSum }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Enter a non negative integer: 0
The digits are: 0 and the sum is 0
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 39465The digits are: 3 9 4 6 5 and the sum is 27
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: H3ll0 W0rldH3ll0 W0rld is not a number...
Please enter digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: -98-98 is a negative number...
Please enter positive digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 19.8719.87 is a decimal number...
Please enter positive whole numbers only!Do you have more data for input? (Y/N): n
------------------------------------------------
BYE!
C++ || Class – Roman Numeral To Integer & Integer To Roman Numeral Conversion
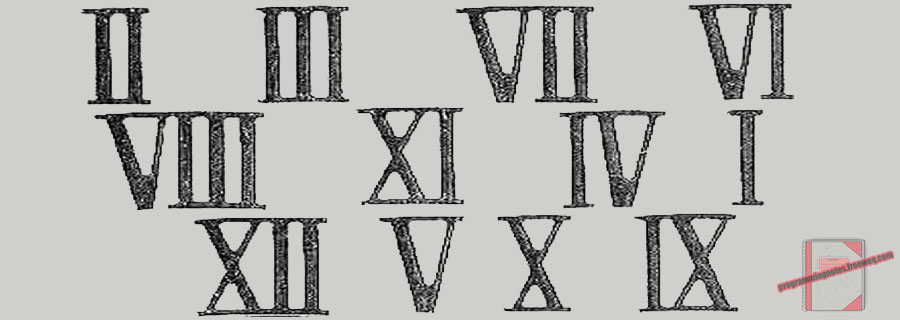
The following is another homework assignment which was presented in a C++ Data Structures course. This program was assigned in order to practice the use of the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
Do/While Loop
Passing a Value By Reference
Roman Numerals - How Do You Convert To Decimal?
Online Roman Numeral Converter - Check For Correct Results
This is an interactive program in which the user has the option of selecting from 7 modes of operation. Of those modes, the user has the option of entering in roman numerals for conversion, entering in decimal numbers for conversion, displaying the recently entered number, and finally converting between roman or decimal values.
A sample of the menu is as followed:
(Where the user would enter numbers 1-7 to select a choice)
1 2 3 4 5 6 7 8 9 10 11 |
From the following menu: 1. Enter a Decimal number 2. Enter a Roman Numeral 3. Convert from Decimal to Roman 4. Convert from Roman to Decimal 5. Print the current Decimal number 6. Print the current Roman Numeral 7. Quit Please enter a selection: |
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
======== FILE #1 – Menu.cpp ========
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
// ============================================================================ // File: Menu.cpp // Purpose: Converts a number enetered in Decimal to Roman Numeral and a // Roman Numeral to a Decimal Number // ============================================================================ #include <iostream> #include <iomanip> #include <string> #include "ClassRomanType.h" using namespace std; // function prototypes void DisplayMenu(); int GetCommand(int command); void RunChoice(int command, ClassRomanType& romanObject); int main() { int command=7; // Holds which action the user chooses to make ClassRomanType romanObject; // Gives access to the class do{ DisplayMenu(); command = GetCommand(command); RunChoice(command, romanObject); cout<<"n--------------------------------------------------------------------n"; }while(command!=7); cout<<"nBye!n"; return 0; }// End of main void RunChoice(int command, ClassRomanType& romanObject) {// Function: Execute the choice selected from the menu // declare private variables int decimalNumber=0; // Holds decimal value char romanNumeral[150]; //Holds value of roman numeral // execute the desired choice cout <<"ntYou selected choice #"<<command<<" which will: "; switch(command) { case 1: cout << "Get Decimal Numbernn"; cout <<"Enter a Decimal Number: "; cin >> decimalNumber; romanObject.GetDecimalNumber(decimalNumber); break; case 2: cout << "Get Roman Numeralnn"; cout <<"nEnter a Roman Numeral: "; cin >> romanNumeral; romanObject.GetRomanNumeral(romanNumeral); break; case 3: cout << "Convert from Decimal to Romannn"; romanObject.ConvertDecimalToRoman(); cout<<"Running....nComplete!n"; break; case 4: cout << "Convert from Roman to Decimalnn"; romanObject.ConvertRomanToDecimal(); cout<<"Running....nComplete!n"; break; case 5: cout << "Print the current Decimal numbernn"; cout<<"n The current Roman to Decimal Value is: "; cout << romanObject.ReturnDecimalNumber()<<endl; break; case 6: cout << "Print the current Roman Numeralnn"; cout<<"n The current Decimal to Roman Value is: "; cout << romanObject.ReturnRomanNumber()<<endl; break; case 7: cout << "Quitnn"; break; default: cout << "nError, you entered an invalid command!nPlease try again..."; break; } }//End of RunChoice int GetCommand(int command) {// Function: Gets choice from menu cout <<"nPlease enter a selection: "; cin>> command; return command; }// End of GetCommand void DisplayMenu() {// Function: Displays menu cout <<"From the following menu:n"<<endl; cout <<left<<setw(2)<<"1."<<left<<setw(15)<<" Enter a Decimal number"<<endl; cout <<left<<setw(2)<<"2."<<left<<setw(15)<<" Enter a Roman Numeral"<<endl; cout <<left<<setw(2)<<"3."<<left<<setw(15)<<" Convert from Decimal to Roman"<<endl; cout <<left<<setw(2)<<"4."<<left<<setw(15)<<" Convert from Roman to Decimal"<<endl; cout <<left<<setw(2)<<"5."<<left<<setw(15)<<" Print the current Decimal number"<<endl; cout <<left<<setw(2)<<"6."<<left<<setw(15)<<" Print the current Roman Numeral"<<endl; cout <<left<<setw(2)<<"7."<<left<<setw(15)<<" Quit"<<endl; }// http://programmingnotes.org/ |
======== FILE #2 – ClassRomanType.h ========
Remember, you need to name the header file the same as the #include from the Menu.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
// ============================================================================ // File: ClassRomanType.h // Purpose: Header file for Roman Numeral conversion. It contains all // declarations and prototypes for the class members to carry out the // conversion // ============================================================================ #include <string> using namespace std; class ClassRomanType { public: ClassRomanType(); /* Function: constructor initializes class variables to "0" Precondition: none Postcondition: defines private variables */ // member functions void GetDecimalNumber(int decimalNumber); /* Function: gets decimal number Precondition: none Postcondition: Gets arabic number from user */ void GetRomanNumeral(char romanNumeral[]); /* Function: gets roman numeral Precondition: none Postcondition: Gets roman numeral from user */ void ConvertDecimalToRoman(); /* Function: converts decimal number to ronman numeral Precondition: arabic and roman numeral characters should be known Postcondition: Gets roman numeral from user */ void ConvertRomanToDecimal(); /* Function: converts roman numeral to arabic number Precondition: arabic and roman numeral characters should be known Postcondition: Gets decimal number from user */ int ReturnDecimalNumber(); /* Function: displays converted decimal number Precondition: the converted roman numeral to decimal number Postcondition: displays decimal number to user */ string ReturnRomanNumber(); /* Function: displays converted roman numeral Precondition: the converted decimal number to roman numeral Postcondition: displays roman numeral to user */ ~ClassRomanType(); /* Function: destructor used to return memory to the system Precondition: the converted decimal number to roman numeral Postcondition: none */ private: char m_romanValue[150]; // An array holding the roman value within the class int m_decimalValue; // Holds the final output answer of the roman to decimal conversion }; // http://programmingnotes.org/ |
======== FILE #3 – RomanType.cpp ========
This is the function implementation file for the ClassRomanType.h class. This file can be named anything you wish as long as you #include “ClassRomanType.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 |
// ============================================================================ // File: RomanType.cpp // Purpose: Contains the implementation of the class members for the // roman numeral conversion // // *NOTE*: Uncomment cout statements to visualize whats happening // ============================================================================ #include <iostream> #include <string> #include <cstring> #include "ClassRomanType.h" using namespace std; ClassRomanType::ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// end of ClassRomanType void ClassRomanType:: GetDecimalNumber(int decimalNumber) { m_decimalValue = decimalNumber; }// end of GetDecimalNumber void ClassRomanType:: GetRomanNumeral(char romanNumeral[]) { strcpy(m_romanValue,romanNumeral); }// end of GetRomanNumeral void ClassRomanType:: ConvertDecimalToRoman() { strcpy(m_romanValue,""); // erases any previous data int numericValue[]={1000,900,500,400,100,90,50,40,10,9,5,4,1,0}; char *romanNumeral[]={"M","CM","D","CD","C","XC","L" ,"XL","X","IX","V","IV","I",0}; for (int x=0; numericValue[x] > 0; ++x) { //cout<<endl<<numericValue[x]<<endl; while (m_decimalValue >= numericValue[x]) { //cout<<"current m_decimalValue = " <<m_decimalValue<<endl; //cout<<"current numericValue = "<<numericValue[x]<<endl<<endl; if(strlen(m_romanValue)==0) { strcpy(m_romanValue,romanNumeral[x]); } else { strcat(m_romanValue,romanNumeral[x]); } m_decimalValue -= numericValue[x]; } } }// end of ConvertDecimalToRoman void ClassRomanType:: ConvertRomanToDecimal() { int currentNumber = 0; // Holds value of each letter numerical value int previousNumber = 0; // Holds the numerical value being compared m_decimalValue=0; // Resets the current 'romanToDecimalValue' to zero if(strcmp(m_romanValue,"Currently Undefined")!=0) { for (int counter = strlen(m_romanValue)-1; counter >= 0; counter--) { if ((m_romanValue[counter] == 'M') || (m_romanValue[counter] == 'm')) { currentNumber = 1000; } else if ((m_romanValue[counter] == 'D') || (m_romanValue[counter] == 'd')) { currentNumber = 500; } else if ((m_romanValue[counter] == 'C') || (m_romanValue[counter] == 'c')) { currentNumber = 100; } else if ((m_romanValue[counter] == 'L') || (m_romanValue[counter] == 'l')) { currentNumber = 50; } else if ((m_romanValue[counter] == 'X') || (m_romanValue[counter] == 'x')) { currentNumber = 10; } else if ((m_romanValue[counter] == 'V') || (m_romanValue[counter] == 'v')) { currentNumber = 5; } else if ((m_romanValue[counter] == 'I') || (m_romanValue[counter] == 'i')) { currentNumber = 1; } else { currentNumber = 0; } //cout<<"previousNumber = " <<previousNumber<<endl; //cout<<"currentNumber = " <<currentNumber<<endl; if (previousNumber > currentNumber) { m_decimalValue -= currentNumber; previousNumber = currentNumber; } else { m_decimalValue += currentNumber; previousNumber = currentNumber; } //cout<<"current m_decimalValue = " <<m_decimalValue<<endl<<endl; } } }// end of ConvertRomanToDecimal int ClassRomanType::ReturnDecimalNumber() { return m_decimalValue; }// end of ReturnDecimalNumber string ClassRomanType::ReturnRomanNumber() { return m_romanValue; }// end of ReturnRomanNumber ClassRomanType::~ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
From the following menu:
1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 9
You selected choice #9 which will:
Error, you entered an invalid command!
Please try again...
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 0
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: Currently Undefined
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 1
You selected choice #1 which will: Get Decimal Number
Enter a Decimal Number: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 3
You selected choice #3 which will: Convert from Decimal to Roman
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: MCMLXXXVII
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 2
You selected choice #2 which will: Get Roman Numeral
Enter a Roman Numeral: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 4
You selected choice #4 which will: Convert from Roman to Decimal
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 2012
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 7
You selected choice #7 which will: Quit
--------------------------------------------------------------------
Bye!
C++ || Class – A Simple Calculator Implementation Using A Class, Enum List, Typedef & Header Files
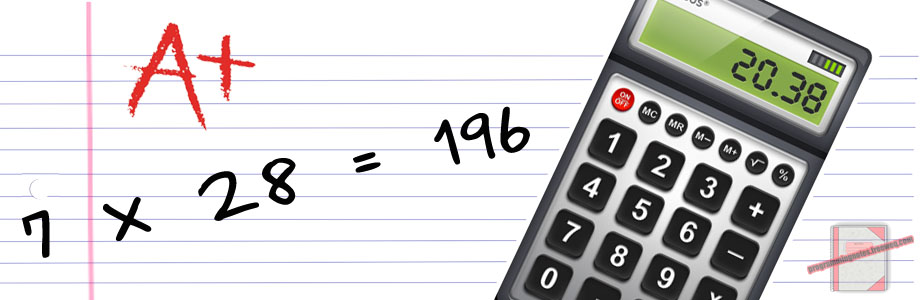
The following is another homework assignment which was presented in a programming class, that was used to introduce the concept of the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - Data Structure
Enumerated List
Typedef
Do/While Loop
Passing a Value By Reference
Constant Variables
Atoi - Convert String To Int Value
This is an interactive program which simulates a basic arithmetic calculator, where the user has the option of selecting from 9 modes of operation. Of those modes, the user has the option of adding, subtracting, multiplying, and dividing two numbers together. Also included in the calculator is a mode which deducts percentages from any given variable the user desires, so for example, if the user wanted to deduct 23% from the number 87, the program would display the reduced value of 66.99.
A sample of the menu is as followed:
(Where the user would enter numbers 1-9 to select a choice)
1 2 3 4 5 6 7 8 9 10 11 12 |
Calculator Options: 1) Clear Calculator 2) Set Initial Calculator Value 3) Display The Current Value 4) Add 5) Subtract 6) Divide 7) Multiply 8) Percentage Calculator 9) Quit Please enter a selection: |
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
======== File #1 Main.cpp ========
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 |
// ============================================================================ // This program tests the CCalc class. A loop is entered where the user is // prompted for a simple mathematical operation to be performed by the CCalc // class. The user is allowed to manipulate the calculator until they // indicate they wish to quit. // ============================================================================ #include <iostream> #include <iomanip> #include "CCalc.h" using namespace std; // defined constants const int LENGTH = 81; // function prototypes void DisplayMenu(); void HandleMenuSelection(CCalc &calcObject, int menuItem); // enumerated list enum MenuChoice { ITEM_CLEAR = 1 , ITEM_SET_VALUE = 2 , ITEM_DISPLAY_VALUE = 3 , ITEM_ADD = 4 , ITEM_SUBTRACT = 5 , ITEM_DIVIDE = 6 , ITEM_MULTIPLY = 7 , ITEM_PERCENT = 8 , ITEM_QUIT = 9}; // ==== main ================================================================== // // ============================================================================ int main() { // variable declarations CCalc calculator; // 'calculator' is an accessor to the 'CCalc' class char myString[LENGTH]; int menuItem=0; // initialize the calculator calculator.SetCurrentValue(0); // loop and let the user manipulate the calculator do{ // display the menu and get the user selection DisplayMenu(); cout << "nPlease enter a selection: "; cin.getline(myString, LENGTH); // convert the ascii string into an integer menuItem = atoi(myString); // creates a line seperator after each task is executed cout<<setfill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // handle the menu selection HandleMenuSelection(calculator, menuItem); cout <<endl; // creates a line seperator after each task is executed cout<<setfill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // ensures the menu isnt displayed twice on the screen if(menuItem!=1 && menuItem!=3 && menuItem!=9) { cin.ignore(); } }while(menuItem!=ITEM_QUIT); cout << "nBye....n"; return 0; } // end of "main" // ==== DisplayMenu =========================================================== // // This function displays the menu of options to stdout. // // Input: nothing // // Output: nothing // // ============================================================================ void DisplayMenu() { cout << "Calculator Options:" << endl; cout << " 1) Clear Calculator" << endl; cout << " 2) Set Initial Calculator Value" << endl; cout << " 3) Display The Current Value" << endl; cout << " 4) Add" << endl; cout << " 5) Subtract" << endl; cout << " 6) Divide" << endl; cout << " 7) Multiply" << endl; cout << " 8) Percentage Calculator" << endl; cout << " 9) Quit" << endl; } // end of "DisplayMenu" // ==== HandleMenuSelection =================================================== // // This function handles the menu selection by examining the input integer // value and calling the appropriate function. // // Input: // calculator -- a reference to the CCalc class // // menuItem -- an integer representing the current menu selection // // Output: // The desired user selection // // ============================================================================ void HandleMenuSelection(CCalc &calculator, int menuItem) { double currentValue = 0; double prevNum = calculator.GetPreviousValue(); // checks to see which user defined selection the calculator will execute // as defined in the enum list located above the main function. // A switch would be more practical to be used here, but i wasnt // comfortable yet using switches when this program was initially made if (menuItem == ITEM_CLEAR) { cout << "nThe current value is " << calculator.Clear() << endl; } // set a initial value else if (menuItem == ITEM_SET_VALUE) { cout << "nPlease enter a new value to assign: "; cin >> currentValue; calculator.SetCurrentValue(currentValue); } // display current value else if (menuItem == ITEM_DISPLAY_VALUE) { cout << "nThe current value is: "<< calculator.DisplayCurrentValue() <<endl; } // add current number by another number else if (menuItem == ITEM_ADD) { cout << "nThe current value is: "<< prevNum <<endl; cout << "nPlease enter a number to be added to "<<prevNum<<": "; cin >> currentValue; calculator.Add(currentValue); cout << "n"<<prevNum<<" + "<<currentValue<<" = "<< calculator.DisplayCurrentValue() <<endl; } // subtract current number by another number else if (menuItem == ITEM_SUBTRACT) { cout << "nThe current value is: "<< prevNum <<endl; cout << "nPlease enter a number to be subtracted from "<<prevNum<<": "; cin >> currentValue; calculator.Subtract(currentValue); cout << "n"<<prevNum<<" - "<<currentValue<<" = " << calculator.DisplayCurrentValue() <<endl; } // divide current value by another number else if (menuItem == ITEM_DIVIDE) { cout << "nThe current value is: "<< prevNum <<endl; cout << "nPlease enter a divisor to be divided by "<<prevNum<<": "; cin >> currentValue; if (currentValue == 0) { cout << "nSorry, division by zero is not allowed...nPlease press ENTER to continue..."; cin.get(); } else { calculator.Divide(currentValue); cout << "n"<<prevNum<<" "<<char(246)<<" "<<currentValue<<" = " << calculator.DisplayCurrentValue() <<endl; } } // multiply current value by another number else if (menuItem == ITEM_MULTIPLY) { cout << "nThe current value is: "<< prevNum <<endl; cout << "nPlease enter a number to be multiplied by "<<prevNum<<": "; cin >> currentValue; calculator.Multiply(currentValue); cout << "n"<<prevNum<<" x "<<currentValue<<" = " << calculator.DisplayCurrentValue() <<endl; } // convert value to decimal percent else if (menuItem == ITEM_PERCENT) { cout << "nThe current value is: "<< prevNum <<endl; cout << "nPlease enter a percentage to be deducted from "<<prevNum<<": "; cin >> currentValue; cout << "n"<<currentValue<<"% off of "<<prevNum<<" is " << calculator.GetPercentage(currentValue) <<endl; } // quit program else if (menuItem == ITEM_QUIT) { cout << "nCalculator will now quit"; } // user entered an invalid choice else { cout << "nYou have entered an invalid command...nPlease press ENTER to try again."; } } // http://programmingnotes.org/ |
======== File #2 CCalc.h. ========
Remember, you need to name the header file the same as the #include from the main.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
// ============================================================================ // File: CCalc.h // ============================================================================ // This is the header file for the CCalc class. // ============================================================================ #ifndef CCALC_HEADER #define CCALC_HEADER // a typedef statement to create a synonym for // the 'double' data type named "CCalcType" typedef double CCalcType; class CCalc // this is the 'CCalc' class declaration { public: // constructor CCalc(); // initializes variables // member functions void Add(CCalcType value); // adds numbers together CCalcType Clear(); // clears the current number in the calculator void Divide(CCalcType value); // divides numbers together CCalcType DisplayCurrentValue(); // displays the current value in the calculator CCalcType GetPreviousValue(); // returns the previous value in the calculator CCalcType GetPercentage(CCalcType value); // deducts a user specified percentage of a given value void Multiply(CCalcType value); // multiplies numbers together void SetCurrentValue(CCalcType value); // set a current value in the calculator void Subtract(CCalcType value); // subtracts two numbers together // destructor ~CCalc(); // deletes any variables contained in the class private: CCalcType m_total; // private variable used within the program }; #endif // CCALC_HEADER -- http://programmingnotes.org/ |
======== File #3 CCalc.cpp. ========
This is the function implementation file for the CCalc.h class. This file can be named anything you wish as long as you #include “CCalc.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
// ============================================================================ // File: CCalc.cpp // ============================================================================ // This is the function implementation file for the CCalc.h class. // ============================================================================ #include <iostream> #include "CCalc.h" using namespace std; CCalc::CCalc() // this is the constructor { m_total = 0; }// end of CCalc void CCalc::Add(CCalcType value) { m_total += value; }// end of Add CCalcType CCalc::Clear() { m_total = 0; return m_total; }// end of Clear void CCalc::Divide(CCalcType value) { m_total /= value; }// end of Divide CCalcType CCalc::DisplayCurrentValue() { return m_total; }// end of DisplayCurrentValue CCalcType CCalc::GetPreviousValue() { return m_total; }// end of GetPreviousValue void CCalc::Multiply(CCalcType value) { m_total *= value; }// end if Multiply void CCalc::SetCurrentValue(CCalcType value) { m_total = value; }// end of SetValue void CCalc::Subtract(CCalcType value) { m_total -= value; }// end of Subtract CCalcType CCalc::GetPercentage(CCalcType value) { CCalcType percent= value/100; CCalcType tempTotal = m_total; tempTotal *= percent; m_total -= tempTotal; return m_total; }// end of GetPercentage CCalc::~CCalc() // this is the destructor { m_total = 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 0
------------------------------------------------------------You have entered an invalid command...
Please ENTER to try again.
------------------------------------------------------------Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 2
------------------------------------------------------------Please enter a new value to assign: 2
------------------------------------------------------------
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 3
------------------------------------------------------------The current value is: 2
------------------------------------------------------------
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 4
------------------------------------------------------------The current value is: 2
Please enter a number to be added to 2: 2
2 + 2 = 4
------------------------------------------------------------
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 5
------------------------------------------------------------The current value is: 4
Please enter a number to be subtracted from 4: 6
4 - 6 = -2
------------------------------------------------------------
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 6
------------------------------------------------------------The current value is: -2
Please enter a divisor to be divided by -2: -2
-2 ÷ -2 = 1
------------------------------------------------------------
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 7
------------------------------------------------------------The current value is: 1
Please enter a number to be multiplied by 1: 87
1 x 87 = 87
------------------------------------------------------------
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 8
------------------------------------------------------------The current value is: 87
Please enter a percentage to be deducted from 87: 23
23% off of 87 is 66.99
------------------------------------------------------------
Calculator Options:
1) Clear Calculator
2) Set Initial Calculator Value
3) Display The Current Value
4) Add
5) Subtract
6) Divide
7) Multiply
8.) Percentage Calculator
9) QuitPlease enter a selection: 9
------------------------------------------------------------Calculator will now quit
------------------------------------------------------------Bye....
C++ || Modulus – Celsius To Fahrenheit Conversion Displaying Degrees Divisible By 10 Using Modulus
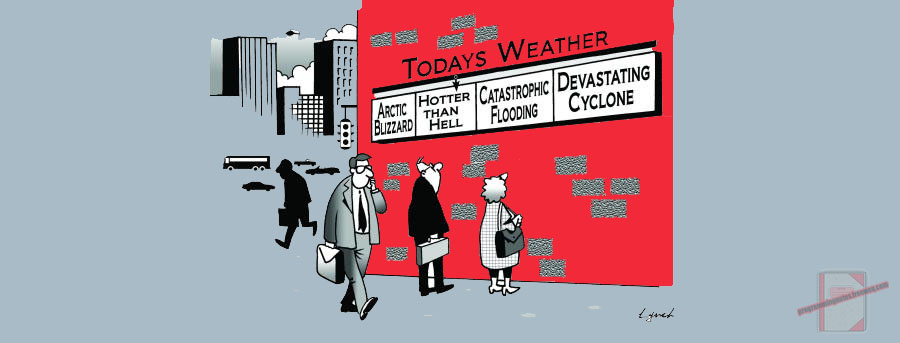
This page will consist of two simple programs which demonstrate the use of the modulus operator (%).
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Modulus
Do/While Loop
Functions
Simple Math - Divisibility
Celsius to Fahrenheit Conversion
===== FINDING THE DIVISIBILITY OF A NUMBER =====
Take a simple arithmetic problem: what’s left over when you divide an odd number by an even number? The answer may not be easy to compute, but we know that it will most likely result in an answer which has a decimal remainder. How would we determine the divisibility of a number in a programming language like C++? That’s where the modulus operator comes in handy.
To have divisibility means that when you divide the first number by another number, the quotient (answer) is a whole number (i.e – no decimal values). Unlike the division operator, the modulus operator (‘%’), has the ability to give us the remainder of a given mathematical operation that results from performing integer division.
To illustrate this, here is a simple program which prompts the user to enter a number. Once the user enters a number, they are asked to enter in a divisor for the previous number. Using modulus, the program will determine if the second number is divisible by the first number. If the modulus result returns 0, the two numbers are divisible. If the modulus result does not return 0, the two numbers are not divisible. The program will keep re-prompting the user to enter in a correct choice until a correct result is obtained.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
#include <iostream> using namespace std; int main() { // declare variables int base=0; int userInput=0; double multiple =0; // get first number cout <<"Please enter a value: "; cin >> base; // get second number cout << "nPlease enter a factor of "<<base<<": "; cin >> userInput; do{ // loop will keep going until the user enters a correct answer // this is the modulus declaration, which will find the // remainder between the 2 numbers multiple = base % userInput; // if the modulus result returns 0, the 2 numbers // are divisible if (multiple == 0) { cout <<"nCorrect! " << base << " is divisible by " << userInput <<endl; cout <<"n("<< base << "/" << userInput <<") = "<<(base/userInput)<<endl; } // if the user entered an incorrect choice, promt an error message else { cout << "nIncorrect, " << base <<" is not divisible by " << userInput <<".nPlease enter a new multiple integer for that value: "; cin >> userInput; } }while(multiple != 0); // ^ loop stops once user enters correct choice return 0; }// http://programmingnotes.org/ |
The above program determines if number ‘A’ is divisible be number ‘B’ via modulus. Unlike the division operator, which does not return the remainder of a number, the modulus operator does, thus we are able to find divisibility between two numbers.
To demonstrate the above code, here is a sample run:
Please enter a value: 21
Please enter a factor of 21: 5Incorrect, 21 is not divisible by 5.
Please enter a new multiple integer for that value: 7Correct! 21 is divisible by 7
(21/7) = 3
===== CELSIUS TO FAHRENHEIT CONVERSION =====
Now that we understand how modulus works, the second program shouldn’t be too difficult. This function first prompts the user to enter in an initial (low) value. After the program obtains the low value from the user, the program will ask for another (high) value. After it obtains the needed information, it displays all the degrees, from the range of the low number to the high number, which are divisible by 10. So if the user enters a low value of 3 and a high value of 303, the program will display all of the Celsius to Fahrenheit degrees within that range which are divisible by 10.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
#include <iostream> using namespace std; // function prototype double ConvertCelsiusToFahrenheit(int degree); int main() { // declare variables int low=0; int high=0; double degreeFahrenhiet =0; double multiple =0; // get data from user cout << "Enter a low number: "; cin >> low; cout << "nEnter a high number: "; cin >> high; // displays data back to user in table form cout <<"nCelsius Fahrenheit:n"; cout << low << "t" << ConvertCelsiusToFahrenheit(low)<<endl; ++low; // this loop displays all the degrees that are divisible by 10 do{ // this converts the current number from celsius to fahrenhiet degreeFahrenhiet = ConvertCelsiusToFahrenheit(low); // this is the modulus operation which finds the // numbers which are divisible by 10 multiple = low % 10; // the program will only display the degrees to the user via // cout which are divisible by 10 if(multiple ==0) { cout << low << "t" << degreeFahrenhiet<<endl; } // this increments the current degree number by 1 ++low; }while(low < high); // ^ loop stops once the 'low' variable reaches the 'high' variable // displays the 'high' converted degrees to the user cout << high << "t" << ConvertCelsiusToFahrenheit(high)<<endl; return 0; }// end of main // function declaration which returns the converted celsius to fahrenhiet value double ConvertCelsiusToFahrenheit(int degree) { return ((1.8 * degree) + 32.0); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter a low number: 3
Enter a high number: 303Celsius Fahrenheit:
3..........37.4
10.........50
20.........68
30.........86
40........104
50........122
60........140
70........158
80........176
90........194
100.......212
110.......230
120.......248
130.......266
140.......284
150.......302
160.......320
170.......338
180.......356
190.......374
200.......392
210.......410
220.......428
230.......446
240.......464
250.......482
260.......500
270.......518
280.......536
290.......554
300.......572
303.......577.4
C++ || Random Number Guessing Game Using Srand, Rand, & Do/While Loop
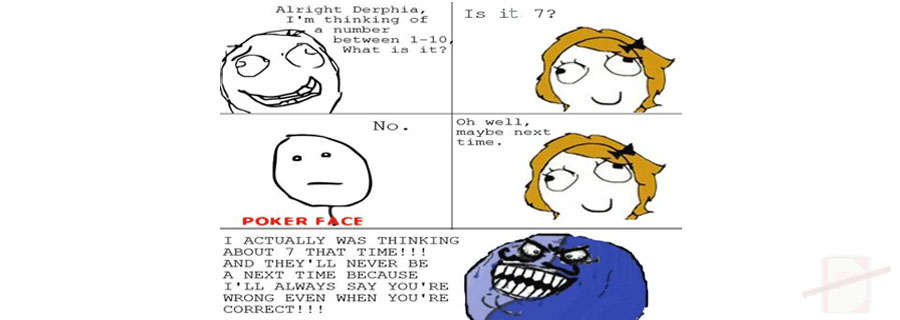
This is a simple guessing game, which demonstrates the use of srand and rand to generate random numbers. This program first prompts the user to enter a number between 1 and 1000. Using if/else statements, the program will then check to see if the user inputted number is higher/lower than the pre defined random number which is generated by the program. If the user makes a wrong guess, the program will re prompt the user to enter in a new number, where they will have a chance to enter in a new guess. Once the user finally guesses the correct answer, using a do/while loop, the program will ask if they want to play again. If the user selects yes, the game will start over, and a new random number will be generated. If the user selects no, the game will end.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 20, 2012 // Taken From: http://programmingnotes.org/ // File: guessingGame.cpp // Description: Demonstrates a simple random number guessing game // ============================================================================ #include <iostream> #include <ctime> #include <iomanip> using namespace std; int main() { // declare & initialize variables char playAgain = 'y'; int userInput = 0; int numGuesses = 0; // Seed the random number generator with the current time so // the numbers will be different every time the program runs srand(time(NULL)); int randomNumber = rand() % 1000+1; // display directions to user cout << "I'm thinking of a number between 1 and 1000. Go ahead and make your first guess.\n\n"; do{ // this is the start of the do/while loop // get data from user cin >> userInput; // increments the 'numGuesses' variable each time the user // gets the guess wrong ++numGuesses; // if user guess is too high, do this code if(userInput > randomNumber) { cout << "Too high! Think lower.\n"; } // if user guess is too low, do this code else if(userInput < randomNumber) { cout << "Too low! Think higher.\n"; } // if user guess is correct, do this code else { // display data to user, prompt if user wants to play again cout << "You got it, and it only took you " << numGuesses <<" trys!\nWould you like to play again (y/n)? "; cin >> playAgain; // if user wants to play again then re initialize the variables if(playAgain == 'y'|| playAgain =='Y') { // creates a line seperator if user wants to enter new data cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; numGuesses = 0; cout << "\n\nMake a guess (between 1-1000):\n\n"; // generate a new random number for the user to try & guess randomNumber = rand() % 1000+1; } } }while(playAgain =='y' || playAgain =='Y'); // ^ do/while loop ends when user doesnt select 'Y' // display data to user cout<<"\n\nThanks for playing!!"<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
I'm thinking of a number between 1 and 1000. Go ahead and make your first guess.
67
Too low! Think higher.
500
Too low! Think higher.
700
Too high! Think lower.
600
Too low! Think higher.
680
Too high! Think lower.
650
Too low! Think higher.
660
Too low! Think higher.
670
You got it, and it only took you 8 trys!
Would you like to play again (y/n)? y------------------------------------------------------------
Make a guess (between 1-1000):
500
Too low! Think higher.
600
Too low! Think higher.
700
Too low! Think higher.
900
Too high! Think lower.
800
Too high! Think lower.
760
Too high! Think lower.
740
Too high! Think lower.
720
Too high! Think lower.
700
Too low! Think higher.
710
Too high! Think lower.
705
Too high! Think lower.
701
Too low! Think higher.
702
Too low! Think higher.
703
Too low! Think higher.
704
You got it, and it only took you 15 trys!
Would you like to play again (y/n)? nThanks for playing!!
C++ || Find The Prime, Perfect & All Known Divisors Of A Number Using A For, While & Do/While Loop
This program was designed to better understand how to use different loops which are available in C++.
This program first asks the user to enter a non negative number. After it obtains a non negative integer from the user, the program will determine if the inputted number is a prime number or not, aswell as determine if the user inputted number is a perfect number or not. After it obtains its results, the program will display to the screen if the user inputted number is prime/perfect number or not. The program will also display a list of all the possible divisors of the user inputted number via cout.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Do/While Loop
While Loop
For Loop
Modulus
Basic Math - Prime Numbers
Basic Math - Perfect Numbers
Basic Math - Divisors
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
#include <iostream> #include <iomanip> using namespace std; int main () { int userInput = 0; int divisor = 0; int sumOfDivisors = 0; char response ='n'; do{ // this is the start of the do/while loop cout<<"Enter a number: "; cin >> userInput; // if the user inputs a negative number, do this code while(userInput < 0) { cout<<"ntaSorry, but the number entered is less than the allowable limit. Please try again"; cout<<"nnEnter an number: "; cin >> userInput; } cout << "nInput number: " << userInput; // for loop adds sum of all possible divisors for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0) { // this will repeatedly add the found divisors together sumOfDivisors += counter; } } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is prime if(userInput == (sumOfDivisors - 1)) { cout<<endl; cout << userInput << " is a prime number."; } else { cout<<endl; cout << userInput << " is not a prime number."; } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is a perfect number if (userInput == (sumOfDivisors - userInput)) { cout<<endl; cout << userInput << " is a perfect number."; } else { cout<<endl; cout << userInput << " is not a perfect number."; } cout << "nDivisors of " << userInput << " are: "; // for loop lists all the possible divisors for the // 'userInput' variable by using the modulus operator for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0 && counter !=userInput) { cout << counter << ", "; } } cout << "and "<< userInput; // asks user if they want to enter new data cout << "nntDo you want to input another number?(Y/N): "; cin >> response; // creates a line seperator if user wants to enter new data cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // resets variable back to zero if the user wants to enter new data sumOfDivisors=0; }while(response =='y' || response =='Y'); // ^ End of the do/while loop. As long as the user chooses // 'Y' the loop will keep going. // It stops when the user chooses the letter 'N' return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Enter a number: 8128
Input number: 8128
8128 is not a prime number.
8128 is a perfect number.
Divisors of 8128 are: 1, 2, 4, 8, 16, 32, 64, 127, 254, 508, 1016, 2032, 4064, and 8128Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 6Input number: 6
6 is not a prime number.
6 is a perfect number.
Divisors of 6 are: 1, 2, 3, and 6Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 241Input number: 241
241 is a prime number.
241 is not a perfect number.
Divisors of 241 are: 1, and 241Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 2012Input number: 2012
2012 is not a prime number.
2012 is not a perfect number.
Divisors of 2012 are: 1, 2, 4, 503, 1006, and 2012Do you want to input another number?(Y/N): n
------------------------------------------------------------
Press any key to continue . . .