Tag Archives: functions
C++ || How To Find The Day Of The Week You Were Born Using C++
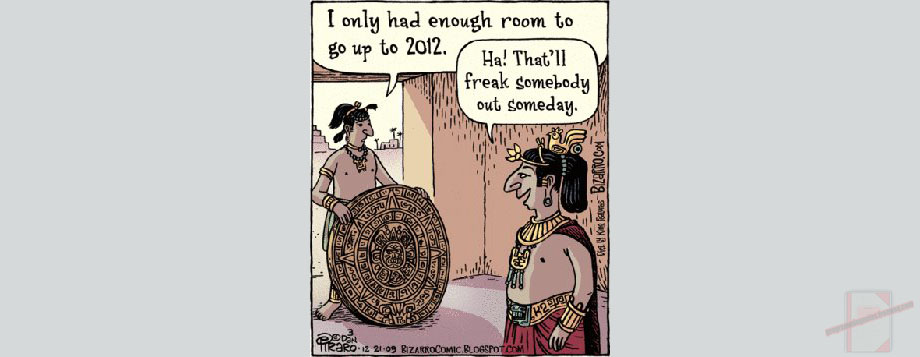
The following is a program which demonstrates how to find the day of week you were born using C++.
The program demonstrated on this page is an updated version of a previous program of the same type.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
1. Overview
This program prompts the user for their name, date of birth (month, day, year), and then displays information back to them via cout. Once the program obtains selected information from the user, it will use simple math to determine the day of the week in which the user was born, and determine the day of the week their current birthday will be for the current calendar year. The program will also display to the user their current age, along with re-displaying their name back to them.
2. When Were You Born?
Note: This program uses functions in a custom .h header file #include “Utils.h”. To obtain the code for that file, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 12, 2012 // Updated: Feb 6, 2021 // Taken From: http://programmingnotes.org/ // File: dateOfWeek.cpp // Description: Simple program to determine the day of week for a date // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" // Function prototypes std::string getMonthName(int month); void validate(int value, int lower, int upper, const std::string& desc); // A const variable containing the current year const int CURRENT_YEAR = 2021; int main() { try { // Declare variables std::string userName = ""; int month = 0; int day = 0; int year = 0; // Get user input std::cout << "Please enter your name: "; std::getline(std::cin, userName); // Get month born std::cout << "\nPlease enter the month in which you were born (between 1 and 12): "; std::cin >> month; // Validate correct month range validate(month, 1, 12, "month"); // Get day born std::cout << "\nPlease enter the day you were born (between 1 and 31): "; std::cin >> day; // Validate correct birth date range validate(day, 1, 31, "birth date"); // Get year born std::cout << "\nPlease enter the year you were born: "; std::cin >> year; // Get the month name auto monthName = getMonthName(month); // Get the week name auto weekdayName = Utils::getWeekdayName(day, month, year); // display data to user std::cout << "\nHello " << userName << ". Here are some facts about you!" << std::endl; std::cout << "You were born " << monthName << " " << day << " " << year << std::endl; std::cout << "Your birth took place on a " << weekdayName << std::endl; std::cout << "This year (" << CURRENT_YEAR << ") your birthday will take place on a " << Utils::getWeekdayName(day, month, CURRENT_YEAR) << std::endl; std::cout << "You currently are, or will be " << (CURRENT_YEAR - year) << " years old this year!" << std::endl; } catch (std::exception& e) { std::cout << "\nAn error occurred: " + std::string(e.what()) << std::endl; } std::cin.get(); return 0; } /** * FUNCTION: getMonthName * USE: Returns the name of the month for the given paremeters * (i.e month #1 = January) * @param month: The month * @return: The name of the month for the given parameters */ std::string getMonthName(int month) { std::string months[] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"}; return months[month - 1]; } void validate(int value, int lower, int upper, const std::string& desc) { if (value < lower || value > upper) { throw std::invalid_argument{ "Invalid " + desc + " entered: '" + std::to_string(value) + "'" }; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled four separate times to display the different outputs its able to produce
Please enter your name: My Programming Notes
Please enter the month in which you were born (between 1 and 12): 1
Please enter the day you were born (between 1 and 31): 1
Please enter the year you were born: 2012Hello My Programming Notes. Here are some facts about you!
You were born January 1 2012
Your birth took place on a Sunday
This year (2021) your birthday will take place on a Friday
You currently are, or will be 9 years old this year!---------------------------------------------------------------------
Please enter your name: Jennifer
Please enter the month in which you were born (between 1 and 12): 1
Please enter the day you were born (between 1 and 31): 27
Please enter the year you were born: 1991Hello Jennifer. Here are some facts about you!
You were born January 27 1991
Your birth took place on a Sunday
This year (2021) your birthday will take place on a Wednesday
You currently are, or will be 30 years old this year!---------------------------------------------------------------------
Please enter your name: Kenneth
Please enter the month in which you were born (between 1 and 12): 7
Please enter the day you were born (between 1 and 31): 28
Please enter the year you were born: 1987Hello Kenneth. Here are some facts about you!
You were born July 28 1987
Your birth took place on a Tuesday
This year (2021) your birthday will take place on a Wednesday
You currently are, or will be 34 years old this year!---------------------------------------------------------------------
Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 12
Please enter the day you were born (between 1 and 31): 35
An error occurred: Invalid birth date entered: '35'
Java || Modulus – Celsius To Fahrenheit Conversion Displaying Degrees Divisible By 10 Using Modulus
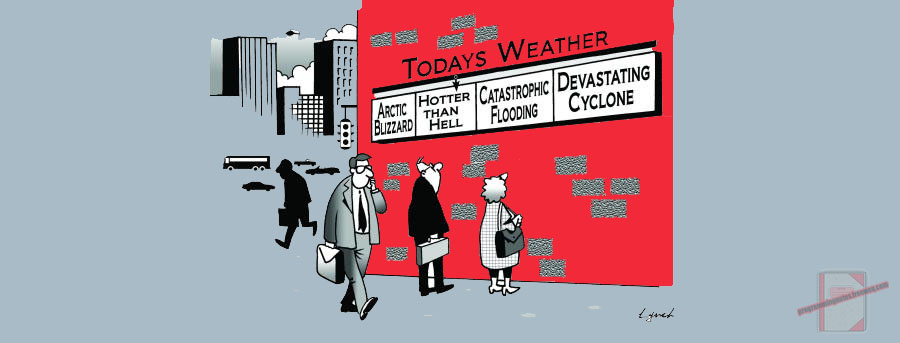
This page will consist of two simple programs which demonstrate the use of the modulus operator (%).
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Modulus
Do/While Loop
Methods (A.K.A "Functions") - What Are They?
Simple Math - Divisibility
Celsius to Fahrenheit Conversion
===== FINDING THE DIVISIBILITY OF A NUMBER =====
Take a simple arithmetic problem: what’s left over when you divide an odd number by an even number? The answer may not be easy to compute, but we know that it will most likely result in an answer which has a decimal remainder. How would we determine the divisibility of a number in a programming language like Java? That’s where the modulus operator comes in handy.
To have divisibility means that when you divide the first number by another number, the quotient (answer) is a whole number (i.e – no decimal values). Unlike the division operator, the modulus operator (‘%’), has the ability to give us the remainder of a given mathematical operation that results from performing integer division.
To illustrate this, here is a simple program which prompts the user to enter a number. Once the user enters a number, they are asked to enter in a divisor for the previous number. Using modulus, the program will determine if the second number is divisible by the first number. If the modulus result returns 0, the two numbers are divisible. If the modulus result does not return 0, the two numbers are not divisible. The program will keep re-prompting the user to enter in a correct choice until a correct result is obtained.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
import java.util.Scanner; public class Modulus { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare & initialize variables int numerator = 0; int denominator = 0; double multiple = 0; System.out.println("Welcome to My Programming Notes' Java Program.n"); // get first number System.out.print("Please enter a value: "); numerator = cin.nextInt(); // get second number System.out.print("nPlease enter a factor of "+numerator+": "); do{ // loop will keep going until the user enters a correct answer denominator = cin.nextInt(); // this is the modulus declaration, which will find the // remainder between the 2 numbers multiple = numerator % denominator; // if the modulus result returns 0, the 2 numbers // are divisible if(multiple == 0) { System.out.println("nCorrect! "+numerator+" is divisible by " +denominator); System.out.println("n("+numerator+"/"+denominator+") = " + ""+(numerator/denominator)); } // if the user entered an incorrect choice, promt an error message else { System.out.print("nIncorrect, "+numerator+" is not divisible by " +denominator); System.out.print(".nnPlease enter a new multiple integer for " +numerator+": "); } }while(multiple != 0); // ^ loop stops once user enters correct choice }// end of main }// http://programmingnotes.org/ |
The above program determines if number ‘A’ is divisible be number ‘B’ via modulus. Unlike the division operator, which does not return the remainder of a number, the modulus operator does, thus we are able to find divisibility between two numbers.
To demonstrate the above code, here is a sample run:
Welcome to My Programming Notes' Java Program.
Please enter a value: 21
Please enter a factor of 21: 5Incorrect, 21 is not divisible by 5.
Please enter a new multiple integer for 21: 7
Correct! 21 is divisible by 7(21/7) = 3
===== CELSIUS TO FAHRENHEIT CONVERSION DISPLAYING DEGREES DIVISIBLE BY 10 =====
Now that we understand how modulus works, the second program shouldn’t be too difficult. This function first prompts the user to enter in an initial (low) value. After the program obtains the low value from the user, the program will ask for another (high) value. After it obtains the needed information, it displays all the degrees, from the range of the low number to the high number, which are divisible by 10. So if the user enters a low value of 3 and a high value of 303, the program will display all of the Celsius to Fahrenheit degrees within that range which are divisible by 10.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
import java.util.Scanner; public class Temperature { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare & initialize variables int low = 0; int high = 0; double degreeFahrenhiet = 0; double multiple = 0; System.out.println("Welcome to My Programming Notes' Java Program.n"); // get data from user System.out.print("Enter a low number: "); low = cin.nextInt(); System.out.print("nEnter a high number: "); high = cin.nextInt(); // displays data back to user in table form System.out.print("nCelsius Fahrenheit:n"); // display the initial 'low' converted degrees to the user System.out.println(low+"t"+ConvertCelsiusToFahrenheit(low)); // this loop displays all the degrees that are divisible by 10 do{ // this increments the current degree number by 1 ++low; // this converts the current number from celsius to fahrenhiet degreeFahrenhiet = ConvertCelsiusToFahrenheit(low); // this is the modulus operation which finds the // numbers which are divisible by 10 multiple = low % 10; // the program will only display the degrees to the user via // cout which are divisible by 10 if(multiple == 0) { System.out.println(low+"t"+degreeFahrenhiet); } }while(low < high); // ^ loop stops once the 'low' variable reaches the 'high' variable // displays the 'high' converted degrees to the user System.out.println(high+"t"+ConvertCelsiusToFahrenheit(high)); }// end of main static double ConvertCelsiusToFahrenheit(int degree) { return ((1.8 * degree) + 32.0); }// end of ConvertCelsiusToFahrenheit }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Enter a low number: 3
Enter a high number: 303Celsius Fahrenheit:
3..........37.4
10.........50
20.........68
30.........86
40........104
50........122
60........140
70........158
80........176
90........194
100.......212
110.......230
120.......248
130.......266
140.......284
150.......302
160.......320
170.......338
180.......356
190.......374
200.......392
210.......410
220.......428
230.......446
240.......464
250.......482
260.......500
270.......518
280.......536
290.......554
300.......572
303.......577.4
C++ || Char Array – Palindrome Number Checker Using A Character Array, Strlen, Strcpy, & Strcmp
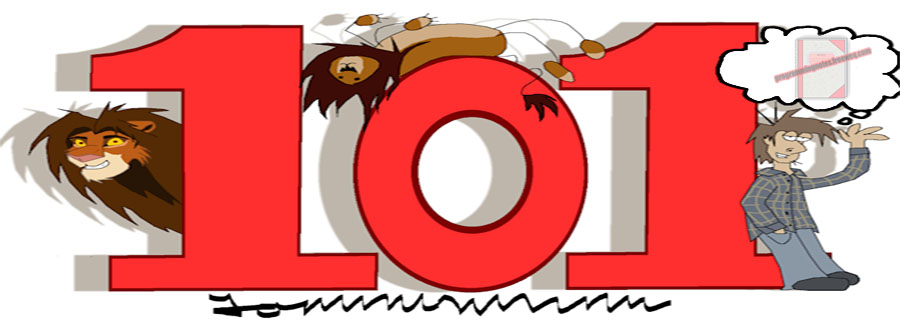
The following is a palindromic number checking program, which demonstrates more use of character array’s, Strlen, & Strcmp.
Want sample code for a palindrome checker which works for numbers and words? Click here.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Character Arrays
How to reverse a character array
Palindrome - What is it?
Strlen
Strcpy
Strcmp
Isdigit
Atoi - Convert a char array to a number
Do/While Loops
For Loops
This program first asks the user to enter a number that they wish to compare for similarity. If the number which was entered into the system is a palindrome, the program will prompt a message to the user via cout. This program determines similarity by using the strcmp function to compare two arrays together. Using a for loop, this program also demonstrates how to reverse a character array, aswell as demonstrates how to determine if the text contained in a character array is a number or not.
This program will repeatedly prompt the user for input until an “exit code” is obtained. The designated exit code in this program is the number 0 (zero). So the program will not stop asking for user input until the number 0 is entered into the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 10, 2012 // Taken From: http://programmingnotes.org/ // File: palindrome.cpp // Description: Palindrome number checker // ============================================================================ #include <iostream> #include <cctype> #include <cstring> #include <cstdlib> using namespace std; // function prototypes void Reverse(char arry[]); bool IsArryANum(char arry[]); // constant, which is the exit value const char* EXIT_VALUE = "0"; int main() { // declare variables char arry[80]; char arryReversed[80]; do{// get data from user using do/while loop cout<<"\nEnter a positive integer, or ("<<EXIT_VALUE<<") to exit: "; cin >> arry; if(atoi(arry) < 0) // check for negative numbers { cout <<"\n*** Error: "<<arry<<" must be greater than zero\n"; } else if(!IsArryANum(arry)) // check for any letters { cout <<"\n*** Error: "<<arry<<" is not an integer\n"; } else if(strcmp(EXIT_VALUE, arry) == 0) // check for "exit code" { cout <<"\nExiting program...\n"; } else // if all else is good, determine if number is a palindrome { // copy the user input from the first array (arry) // into the second array (arryReversed) strcpy(arryReversed, arry); // function call to reverse the contents inside the // "arryReversed" array to check for similarity Reverse(arryReversed); cout <<endl<<arry; // use strcmp to determine if the two arrays are the same if(strcmp(arryReversed, arry) == 0) { cout <<" is a Palindrome..\n"; } else { cout <<" is NOT a Palindrome!\n"; } } }while(strcmp(EXIT_VALUE, arry) != 0); // keep going until user enters the exit value cout <<"\nBYE!\n"; return 0; }// end of main void Reverse(char arry[]) { // get the length of the current word in the array index int length = strlen(arry)-1; // increment thru each letter within the current char array index // reversing the order of the array for (int currentChar=0; currentChar < length; --length, ++currentChar) { // copy 1st letter in the array index into temp char temp = arry[currentChar]; // copy last letter in the array index into the 1st array index arry[currentChar] = arry[length]; // copy temp into last array index arry[length] = temp; } }// end of Reverse bool IsArryANum(char arry[]) { // LOOP UNTIL U REACH THE NULL CHARACTER, // AKA THE END OF THE CHAR ARRAY for(int x=0; arry[x]!='\0'; ++x) { // if the current char isnt a number, // exit the loop & return false if(!isdigit(arry[x])) { return false; } } return true; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter a positive integer, or (0) to exit: L33T
*** error: "L33T" is not an integer
Enter a positive integer, or (0) to exit: -728
*** error: -728 must be greater than zero
Enter a positive integer, or (0) to exit: 1858
1858 is NOT a Palindrome!
Enter a positive integer, or (0) to exit: 7337
7337 is a Palindrome..
Enter a positive integer, or (0) to exit: 0
Exiting program...
BYE!
Java || Whats My Name? – Practice Using Strings, Methods & Switch Statemens
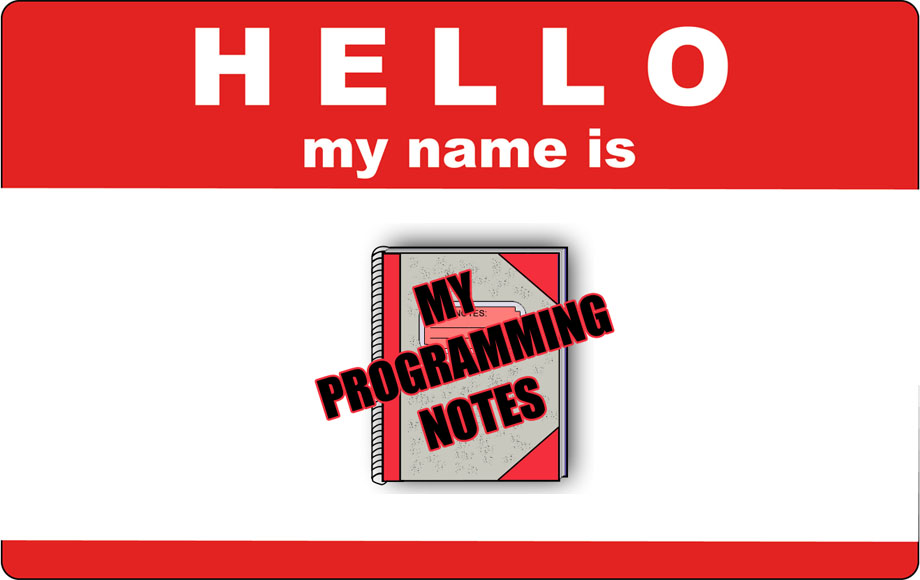
Here is another actual homework assignment which was presented in an intro to programming class. This program highlights more use using strings, modules, and switch statements.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Methods (A.K.A "Functions") - What Are They?
Switch Statements - How To Use Them
Equal - String Comparison
This program first prompts the user to enter their name. Upon receiving that information, the program saves input into a string called “firstName.” The program then asks if the user has a middle name. If they do, the user will enter a middle name. If they dont, the program proceeds to ask for a last name. Upon receiving the first, [middle], and last names, the program will append the first, [middle], and last names into a completely new string titled “fullName.” Lastly, if the users’ first, [middle], or last names are the same, the program will display that data to the screen via stdout. The program will also display to the user the number of characters their full name contains using the built in function “length.”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 |
import java.util.Scanner; public class NameString { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char response = ' '; String firstName= ""; String fullName=""; // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); // get first name from user System.out.print("Please enter your first name: "); firstName = cin.next(); System.out.print("nDo you have a middle name?(Y/N): "); String choice = cin.next(); choice = choice.toUpperCase(); response = choice.charAt(0); // use a switch statement to detrmine which function will be called switch(response) { case 'Y': fullName = FirstMiddleLast(firstName); // method declaration break; case 'N': fullName = FirstLast(firstName); // method declaration break; default: System.out.print("nPlease press either 'Y' or 'N'n" + "Program exiting...n"); System.exit(1); break; } System.out.println("And your full name is "+fullName); }// end of main // ==== FirstMiddleLast ==================================================== // // This module will take as input the first name, and prompt the user for // their middle and last name. Then it will display the total number of // characters in their name. It returns the full name back to main // // ========================================================================= private static String FirstMiddleLast(String firstName) { String middleName=""; String lastName=""; String fullName=""; System.out.print("nPlease enter your middle name: "); middleName = cin.next(); System.out.print("Please enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+middleName+" "+lastName; System.out.println(""); // check to see if the first, middle or last names are the same // if they are, display a message to the user if(firstName.equals(middleName)) { System.out.print("tYour first and middle name are the samen"); } if(middleName.equals(lastName)) { System.out.print("tYour middle and last name are the samen"); } if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the 2 white spaces System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-2)); // return the full name string to main return fullName; }// end of FirstMiddleLast // ==== FirstLast ========================================================== // // This module will take as input the first name, and prompt the user for // their last name. Then it will display the total number of characters in // their name. It returns the full name back to main // // ============================================================================ private static String FirstLast(String firstName) { String lastName=""; String fullName=""; System.out.print("nPlease enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+lastName; System.out.println(""); // check to see if the first or last names are the same // if they are, display a message to the user if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the white space System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-1)); // return the full name string to main return fullName; }// end of FirstLast }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: The code was compiled five separate times to display the different outputs its able to produce
====== RUN 1 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): y
Please enter your middle name: Programming
Please enter your last name: NotesThe total number of characters in your name is: 18
And your full name is My Programming Notes====== RUN 2 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Programming
Do you have a middle name?(Y/N): n
Please enter your last name: NotesThe total number of characters in your name is: 16
And your full name is Programming Notes====== RUN 3 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Notes
Do you have a middle name?(Y/N): y
Please enter your middle name: Notes
Please enter your last name: NotesYour first and middle name are the same
Your middle and last name are the same
Your first and last name are the sameThe total number of characters in your name is: 15
And your full name is Notes Notes Notes====== RUN 4 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): n
Please enter your last name: MyYour first and last name are the same
The total number of characters in your name is: 4
And your full name is My My====== RUN 5 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): zPlease press either 'Y' or 'N'
Program exiting...
Java || Count The Total Number Of Characters, Vowels, & UPPERCASE Letters Contained In A Sentence Using A ‘For Loop’
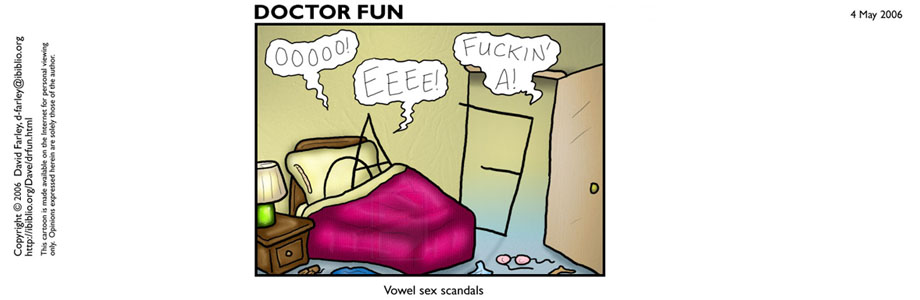
Here is a simple program, which demonstrates more practice using the input/output mechanisms which are available in Java.
This program will prompt the user to enter a sentence, then upon entering an “exit code,” will display the total number of uppercase letters, vowels and characters which are contained within that sentence. This program is very similar to an earlier project, this time, utilizing a for loop, strings, and user defined methods.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
For Loops
Methods (A.K.A "Functions") - What Are They?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
import java.util.Scanner; public class VowelCount { public static void main(String[] args) { // declare variables int numUpperCase = 0; int numVowel = 0; int numChars = 0; char character = ' '; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); System.out.print("Enter a sentence, ending with a period: "); userInput = cin.nextLine(); // loop thru the string until we reach the 'exit code' for(int index=0; userInput.charAt(index) != '.'; ++index) { character = userInput.charAt(index); // do nothing, we dont care about whitespace if(character == ' ') { continue; } // check to see if entered data is UPPERCASE if((character >= 'A')&&(character <= 'Z')) { ++numUpperCase; // Increments Total number of uppercase by one } // check to see if entered data is a vowel if((character == 'a')||(character == 'A')||(character == 'e')|| (character == 'E')||(character == 'i')||(character == 'I')|| (character == 'o')||(character == 'O')||(character == 'u')|| (character == 'U')||(character == 'y')||(character == 'Y')) { ++numVowel; // increment total number of vowels by one } ++numChars; // increment total number of chars by one } setwf("ntTotal number of upper case letters:", 45,'.'); System.out.println(numUpperCase); setwf("tTotal number of vowels:", 44,'.'); System.out.println(numVowel); setwf("tTotal number of characters:", 44,'.'); System.out.println(numChars); }// end of main public static void setwf(String str, int width, char fill) {// this mimics the C++ 'setw' function & formats string output to the screen System.out.print(str); for (int x = str.length(); x < width; ++x) { System.out.print(fill); } }// end of setwf }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted portions are areas of interest.
Notice line 22 contains the for loop declaration. The loop will continually loop thru the string, and will not stop doing so until it reaches an exit character, and the defined exit character in this program is a period (“.”). So, the program will not stop looping thru the string until it reaches a period.
Once compiling the above code, you should receive this as your output
Welcome to My Programming Notes' Java Program.
Enter a sentence, ending with a period:
My Programming Notes Is An Awesome Site.Total number of upper case letters:........7
Total number of vowels:....................14
Total number of characters:................33
Java || Compute The Sum From A String Of Integers & Display Each Number Individually
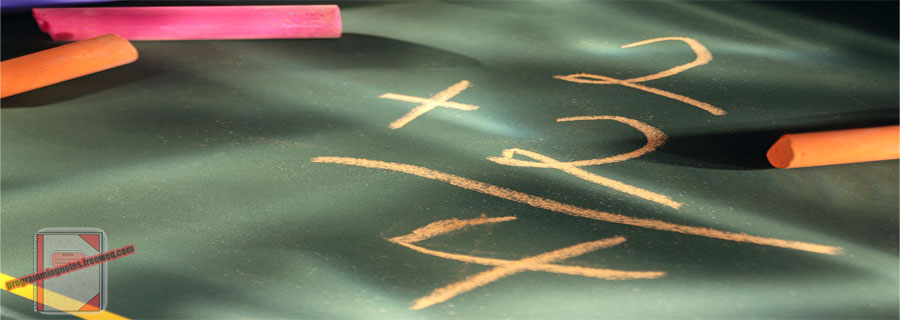
Here is a simple program, which was presented in a Java course. This program was used to introduce the input/output mechanisms which are available in Java. This assignment was modeled after Exercise 2.30, taken from the textbook “Java How to Program” (early objects) (9th Edition) (Deitel). It is the same exercise in both the 8th and 9th editions.
Our class was asked to make a program which prompts the user to enter a non-negative integer into the system. The program was supposed to then extract the digits from the inputted number, displaying them each individually, separated by a white space (” “). After the digits are displayed, the program was then supposed to display the sum of those digits to the screen. So for example, if the user inputted the number “39465,” the program would output the numbers 3 9 4 6 5 individually, and then the sum of 27.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Do/While Loops
For Loops
Methods (A.K.A "Functions") - What Are They?
ParseInt - Convert String To Integer
Substring
Try/Catch
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
import java.util.Scanner; import java.lang.Math; public class SumFromString { public static void main(String[] args) { // declare variables char question = 'n'; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); do{// this is the start of the do/while loop int sum = 0; // get data from the user System.out.print("nEnter a non negative integer: "); userInput = cin.nextLine(); System.out.println(""); // if the string isnt a number, dont compute the sum if(!IsNumeric(userInput)) { System.out.println(userInput+" is not a number..."); System.out.println("Please enter digits only!"); } // if the string is a negative number, dont compute the sum else if(IsNegative(userInput)) { System.out.println(userInput+" is a negative number..."); System.out.println("Please enter positive digits only!"); } // if the string is a decimal number (i.e 19.87), dont compute the sum else if(IsDecimal(userInput)) { System.out.println(userInput+" is a decimal number..."); System.out.println("Please enter positive whole numbers only!"); } // if everything else checks out OK, then compute the sum else { System.out.print("The digits are: "); sum = ComputeSum(userInput); System.out.println("and the sum is " + sum); } // ask user if they want to enter more data System.out.print("nDo you have more data for input? (Y/N): "); String response = cin.nextLine(); response=response.toLowerCase(); question = response.charAt(0); System.out.println("n------------------------------------------------"); }while(question == 'y'); System.out.print("nBYE!n"); }// end of main public static boolean IsNumeric(String str) {// checks to see if a string is a number try { Double.parseDouble(str); return true; } catch(NumberFormatException nfe) { return false; } }// end of IsNumeric public static boolean IsNegative(String str) {// checks to see if string is a negative number return Double.parseDouble(str) < 0; }// end of IsNegative public static boolean IsDecimal(String str) {// checks to see if string is a decimal number return (Math.floor(Double.parseDouble(str))) != Double.parseDouble(str); }// end of IsDecimal public static int ComputeSum(String userInput) {// displays each number individually & computes the sum of the string int sum = 0; String[] temp = new String[userInput.length()]; for(int counter=0; counter < userInput.length(); ++counter) { temp[counter] = userInput.substring(counter,counter+1); System.out.print(temp[counter] + " "); sum = sum + Integer.parseInt(temp[counter]); } return sum; }// end of ComputeSum }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Enter a non negative integer: 0
The digits are: 0 and the sum is 0
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 39465The digits are: 3 9 4 6 5 and the sum is 27
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: H3ll0 W0rldH3ll0 W0rld is not a number...
Please enter digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: -98-98 is a negative number...
Please enter positive digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 19.8719.87 is a decimal number...
Please enter positive whole numbers only!Do you have more data for input? (Y/N): n
------------------------------------------------
BYE!
C++ || Stack – Using A Stack, Determine If A Set Of Parentheses Is Well-Formed
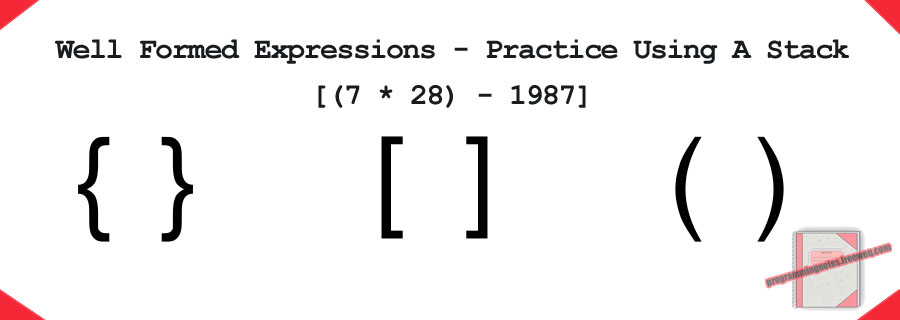
Here is another homework assignment which was presented in a C++ Data Structures course. This assignment was used to introduce the stack ADT, and helped prepare our class for two later assignments which required using a stack. Those assignments can be found here:
(1) Stack Based Infix To Postfix Conversion (Single Digit)
(2) Stack Based Postfix Evaluation (Single Digit)
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Stack Data Structure
Cin.getline
#include "ClassStackListType.h"
A simple exercise for testing a stack is determining whether a set of parenthesis is “well formed” or not. What exactly is meant by that? In the case of a pair of parenthesis, for an expression to be well formed, consider the following table.
1 2 3 4 5 6 |
Well-Formed Expressions | Ill-Formed Expressions ------------------------------------|-------------------------------------- ( a b c [ ] ) | ( a b c [ ) ( ) [ ] { } | ( ( { ( a b c d e ) ( ) } | [ a b c d e ) ( ) } ( a + [ b - c ] / d ) | ( a + [ b - c } / d ) |
Given an expression with characters and parenthesis, ( ), [ ], and { }, our class was asked to determine if an expression was well formed or not by using the following algorithm:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
// An algorithm for a Well Formed expression Set 'balanced' to true Set 'symbol' to the first character in the current expression while(there are more characters AND 'balanced' == true) { if('symbol' is an opening symbol) { Push 'symbol' onto the stack } else if('symbol' is a closing symbol) { if the stack is empty 'balanced' = false else Set the 'openSymbol' to the item at the top of the stack Pop the stack Check to see if 'symbol' matches 'openSymbol' (i.e - if openSymbol == '(' and symbol == ')' then 'balanced' = true) } Set 'symbol' to the next character in the current expression } if('balanced' == true AND stack is empty) { Expression is well formed } else { Expression is NOT well formed }// http://programmingnotes.org/ |
======= WELL-FORMED EXPRESSIONS =======
This program uses a custom template.h class. To obtain the code for that class, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2012 // Taken From: http://programmingnotes.org/ // File: wellFormed.cpp // Description: Demonstrates how to check if an expression is well formed // ============================================================================ #include <iostream> #include "ClassStackListType.h" using namespace std; // function prototypes bool IsOpen(char symbol); bool IsClosed(char symbol); bool IsWellFormed(char openSymbol, char closeSymbol); int main() { // declare variables char expression[120]; char openSymbol; int index=0; bool balanced = true; StackListType<char> stack; // this is the stack declaration // obtain data from the user using a char array cout <<"Enter an expression and press ENTER. "<<endl; cin.getline(expression,sizeof(expression)); cout << "\nThe expression: " << expression; // loop thru the char array until we reach the 'NULL' character // and while 'balanced' == true while (expression[index]!='\0' && balanced) { // if input is an "open bracket" push onto stack // else, process info if (IsOpen(expression[index])) { stack.Push(expression[index]); } else if (IsClosed(expression[index])) { if(stack.IsEmpty()) { balanced = false; } else { openSymbol = stack.Top(); stack.Pop(); balanced = IsWellFormed(openSymbol, expression[index]); } } ++index; } if (balanced && stack.IsEmpty()) { cout << " is well formed..." << endl; } else { cout << " is NOT well formed!!! " << endl; } }// End of Main bool IsOpen(char symbol) { if ((symbol == '(') || (symbol == '{') || (symbol == '[')) { return true; } else { return false; } }// End of IsOpen bool IsClosed(char symbol) { if ((symbol == ')') || (symbol == '}') || (symbol == ']')) { return true; } else { return false; } }// End of IsClosed bool IsWellFormed(char openSymbol, char closeSymbol) { return (((openSymbol == '(') && closeSymbol == ')') || ((openSymbol == '{') && closeSymbol == '}') || ((openSymbol == '[') && closeSymbol == ']')); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: the code was compile four separate times to display different output)
====== RUN 1 ======
Enter an expression and press ENTER.
((
The expression: (( is NOT well formed!!!====== RUN 2 ======
Enter an expression and press ENTER.
(a{b[]}c)The expression: (a{b[]}c) is well formed...
====== RUN 3 ======
Enter an expression and press ENTER.
[(7 * 28) - 1987]The expression: [(7 * 28) - 1987] is well formed...
====== RUN 4 ======
Enter an expression and press ENTER.
{3 + [2 / 3] - (9 + 18) * 12)The expression: {3 + [2 / 3] - (9 + 18) * 12) is NOT well formed!!!
C++ || Class & Input/Output – Display The Contents Of A User Specified Text File To The Screen
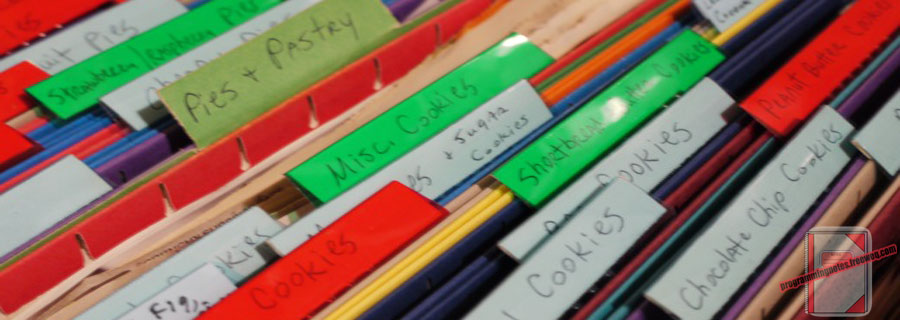
The following is another intermediate homework assignment which was presented in a C++ programming course. This program was assigned to introduce more practice using the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
How To Read Data From A File
String - Getline
Array - Cin.Getline
Strcpy - Copy Contents Of An Array
#Define
This program first prompts the user to input a file name. After it obtains a file name from the user, it then attempts to display the contents of the user specified file to the output screen. If the file could not be found, an error message appears. If the file is found, the program continues as normal. After the file contents finishes being displayed, a summary indicating the total number of lines which has been read is also shown to the screen.
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
Note: The data file that is used in this example can be downloaded here.
Also, in order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
======== FILE #1 – Main.cpp ========
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// ============================================================================ // File: Main.cpp // ============================================================================ // This program tests the "CFileDisp" object. It prompts the user for an input // filename, then attempts to display the contents of that file to stdout. // After the file contents have been displayed, a summary line indicating the // total number of lines that have been displayed is written to stdout. // ============================================================================ #include <iostream> #include <fstream> #include "CFileDisp.h" using namespace std; // ==== main ================================================================== // // ============================================================================ int main() { CFileDisp myFile; char fname[MAX_LENGTH]; int numLines; // get the filename from the user cout << "Enter a filename: "; cin.getline(fname, MAX_LENGTH); // copy the user's filename into the file object myFile.SetFilename(fname); // have the object open the file myFile.OpenFile(); // if all is good and well... if(myFile.IsValid()==true) { numLines = myFile.DisplayFileContents(); // close the file myFile.CloseFile(); // write how many lines were displayed to stdout cout << "nt*** Total lines displayed: " << numLines << endl; } return 0; }// http://programmingnotes.org/ |
======== FILE #2 – CFileDisp.h ========
Remember, you need to name the header file the same as the #include from the Main.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// ============================================================================ // File: CFileDisp.h // ============================================================================ // This is the header file which declares the objects which are used to open // a text file and display its contents to stdout. // ============================================================================ #ifndef CFILE_DISP_HEADER #define CFILE_DISP_HEADER #include <fstream> using namespace std; #define MAX_LENGTH 256 class CFileDisp { public: // constructor CFileDisp(); // member functions void SetFilename(char newFilename[]); /* Purpose: Copy the user's filename into the file object */ void OpenFile(); /* Purpose: Open's the file which is specified by the user */ bool IsValid(); /* Purpose: Checks if the file exists. Post: Returns false if file cannot be found */ int DisplayFileContents(); /* Purpose: Display's the file contents to stdout Post: Returns the total number of lines contained in the file */ void CloseFile(); /* Purpose: Closes the file */ // destructor ~CFileDisp(); private: bool m_bIsValid; char m_filename[MAX_LENGTH]; ifstream m_inFile; }; #endif // CFILE_DISP_HEADER // http://programmingnotes.org/ |
======== FILE #3 – CFileDisp.cpp ========
This is the function implementation file for the CFileDisp.h class. This file can be named anything you wish as long as you #include “CFileDisp.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
// ============================================================================ // File: CFileDisp.cpp // ============================================================================ // This file implements the functions which are declared in the CFileDisp // class, which is located in CFileDisp.h // ============================================================================ #include <iostream> #include <cstring> #include <string> #include "CFileDisp.h" using namespace std; CFileDisp::CFileDisp() { m_bIsValid = 0; }// end of CFileDisp void CFileDisp::SetFilename(char newFilename[]) { strcpy(m_filename,newFilename); }// end of SetFilename void CFileDisp::OpenFile() { m_inFile.open(m_filename); }// end of OpenFile bool CFileDisp::IsValid() { if (m_inFile.fail()) { cout << m_filename << " was not found...nn"; return false; } else { return true; } }// end of IsValid int CFileDisp::DisplayFileContents() { int total=0; string inLine; cout << endl; while(getline(m_inFile, inLine)) { cout << inLine << endl; ++total; } return total; }// end of DisplayFileContents void CFileDisp::CloseFile() { m_inFile.close(); }// end of CloseFile // this is the destructor CFileDisp::~CFileDisp() { m_bIsValid = 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Enter a filename: input_file_display_programmingnotes_freeweq_com.txt 346 130 982 90 656 117 595 415 948 126 4 558 571 87 42 360 412 721 463 47 119 441 190 985 214 509 2 571 77 81 681 651 995 93 74 310 9 995 561 92 14 288 466 664 892 8 766 34 639 151 64 98 813 67 834 369 This is a line of text! *** Total lines displayed: 10 |
C++ || Class – Roman Numeral To Integer & Integer To Roman Numeral Conversion
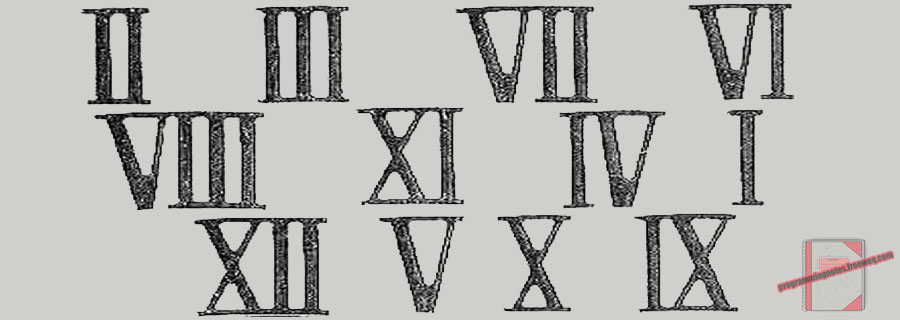
The following is another homework assignment which was presented in a C++ Data Structures course. This program was assigned in order to practice the use of the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
Do/While Loop
Passing a Value By Reference
Roman Numerals - How Do You Convert To Decimal?
Online Roman Numeral Converter - Check For Correct Results
This is an interactive program in which the user has the option of selecting from 7 modes of operation. Of those modes, the user has the option of entering in roman numerals for conversion, entering in decimal numbers for conversion, displaying the recently entered number, and finally converting between roman or decimal values.
A sample of the menu is as followed:
(Where the user would enter numbers 1-7 to select a choice)
1 2 3 4 5 6 7 8 9 10 11 |
From the following menu: 1. Enter a Decimal number 2. Enter a Roman Numeral 3. Convert from Decimal to Roman 4. Convert from Roman to Decimal 5. Print the current Decimal number 6. Print the current Roman Numeral 7. Quit Please enter a selection: |
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
======== FILE #1 – Menu.cpp ========
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
// ============================================================================ // File: Menu.cpp // Purpose: Converts a number enetered in Decimal to Roman Numeral and a // Roman Numeral to a Decimal Number // ============================================================================ #include <iostream> #include <iomanip> #include <string> #include "ClassRomanType.h" using namespace std; // function prototypes void DisplayMenu(); int GetCommand(int command); void RunChoice(int command, ClassRomanType& romanObject); int main() { int command=7; // Holds which action the user chooses to make ClassRomanType romanObject; // Gives access to the class do{ DisplayMenu(); command = GetCommand(command); RunChoice(command, romanObject); cout<<"n--------------------------------------------------------------------n"; }while(command!=7); cout<<"nBye!n"; return 0; }// End of main void RunChoice(int command, ClassRomanType& romanObject) {// Function: Execute the choice selected from the menu // declare private variables int decimalNumber=0; // Holds decimal value char romanNumeral[150]; //Holds value of roman numeral // execute the desired choice cout <<"ntYou selected choice #"<<command<<" which will: "; switch(command) { case 1: cout << "Get Decimal Numbernn"; cout <<"Enter a Decimal Number: "; cin >> decimalNumber; romanObject.GetDecimalNumber(decimalNumber); break; case 2: cout << "Get Roman Numeralnn"; cout <<"nEnter a Roman Numeral: "; cin >> romanNumeral; romanObject.GetRomanNumeral(romanNumeral); break; case 3: cout << "Convert from Decimal to Romannn"; romanObject.ConvertDecimalToRoman(); cout<<"Running....nComplete!n"; break; case 4: cout << "Convert from Roman to Decimalnn"; romanObject.ConvertRomanToDecimal(); cout<<"Running....nComplete!n"; break; case 5: cout << "Print the current Decimal numbernn"; cout<<"n The current Roman to Decimal Value is: "; cout << romanObject.ReturnDecimalNumber()<<endl; break; case 6: cout << "Print the current Roman Numeralnn"; cout<<"n The current Decimal to Roman Value is: "; cout << romanObject.ReturnRomanNumber()<<endl; break; case 7: cout << "Quitnn"; break; default: cout << "nError, you entered an invalid command!nPlease try again..."; break; } }//End of RunChoice int GetCommand(int command) {// Function: Gets choice from menu cout <<"nPlease enter a selection: "; cin>> command; return command; }// End of GetCommand void DisplayMenu() {// Function: Displays menu cout <<"From the following menu:n"<<endl; cout <<left<<setw(2)<<"1."<<left<<setw(15)<<" Enter a Decimal number"<<endl; cout <<left<<setw(2)<<"2."<<left<<setw(15)<<" Enter a Roman Numeral"<<endl; cout <<left<<setw(2)<<"3."<<left<<setw(15)<<" Convert from Decimal to Roman"<<endl; cout <<left<<setw(2)<<"4."<<left<<setw(15)<<" Convert from Roman to Decimal"<<endl; cout <<left<<setw(2)<<"5."<<left<<setw(15)<<" Print the current Decimal number"<<endl; cout <<left<<setw(2)<<"6."<<left<<setw(15)<<" Print the current Roman Numeral"<<endl; cout <<left<<setw(2)<<"7."<<left<<setw(15)<<" Quit"<<endl; }// http://programmingnotes.org/ |
======== FILE #2 – ClassRomanType.h ========
Remember, you need to name the header file the same as the #include from the Menu.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
// ============================================================================ // File: ClassRomanType.h // Purpose: Header file for Roman Numeral conversion. It contains all // declarations and prototypes for the class members to carry out the // conversion // ============================================================================ #include <string> using namespace std; class ClassRomanType { public: ClassRomanType(); /* Function: constructor initializes class variables to "0" Precondition: none Postcondition: defines private variables */ // member functions void GetDecimalNumber(int decimalNumber); /* Function: gets decimal number Precondition: none Postcondition: Gets arabic number from user */ void GetRomanNumeral(char romanNumeral[]); /* Function: gets roman numeral Precondition: none Postcondition: Gets roman numeral from user */ void ConvertDecimalToRoman(); /* Function: converts decimal number to ronman numeral Precondition: arabic and roman numeral characters should be known Postcondition: Gets roman numeral from user */ void ConvertRomanToDecimal(); /* Function: converts roman numeral to arabic number Precondition: arabic and roman numeral characters should be known Postcondition: Gets decimal number from user */ int ReturnDecimalNumber(); /* Function: displays converted decimal number Precondition: the converted roman numeral to decimal number Postcondition: displays decimal number to user */ string ReturnRomanNumber(); /* Function: displays converted roman numeral Precondition: the converted decimal number to roman numeral Postcondition: displays roman numeral to user */ ~ClassRomanType(); /* Function: destructor used to return memory to the system Precondition: the converted decimal number to roman numeral Postcondition: none */ private: char m_romanValue[150]; // An array holding the roman value within the class int m_decimalValue; // Holds the final output answer of the roman to decimal conversion }; // http://programmingnotes.org/ |
======== FILE #3 – RomanType.cpp ========
This is the function implementation file for the ClassRomanType.h class. This file can be named anything you wish as long as you #include “ClassRomanType.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 |
// ============================================================================ // File: RomanType.cpp // Purpose: Contains the implementation of the class members for the // roman numeral conversion // // *NOTE*: Uncomment cout statements to visualize whats happening // ============================================================================ #include <iostream> #include <string> #include <cstring> #include "ClassRomanType.h" using namespace std; ClassRomanType::ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// end of ClassRomanType void ClassRomanType:: GetDecimalNumber(int decimalNumber) { m_decimalValue = decimalNumber; }// end of GetDecimalNumber void ClassRomanType:: GetRomanNumeral(char romanNumeral[]) { strcpy(m_romanValue,romanNumeral); }// end of GetRomanNumeral void ClassRomanType:: ConvertDecimalToRoman() { strcpy(m_romanValue,""); // erases any previous data int numericValue[]={1000,900,500,400,100,90,50,40,10,9,5,4,1,0}; char *romanNumeral[]={"M","CM","D","CD","C","XC","L" ,"XL","X","IX","V","IV","I",0}; for (int x=0; numericValue[x] > 0; ++x) { //cout<<endl<<numericValue[x]<<endl; while (m_decimalValue >= numericValue[x]) { //cout<<"current m_decimalValue = " <<m_decimalValue<<endl; //cout<<"current numericValue = "<<numericValue[x]<<endl<<endl; if(strlen(m_romanValue)==0) { strcpy(m_romanValue,romanNumeral[x]); } else { strcat(m_romanValue,romanNumeral[x]); } m_decimalValue -= numericValue[x]; } } }// end of ConvertDecimalToRoman void ClassRomanType:: ConvertRomanToDecimal() { int currentNumber = 0; // Holds value of each letter numerical value int previousNumber = 0; // Holds the numerical value being compared m_decimalValue=0; // Resets the current 'romanToDecimalValue' to zero if(strcmp(m_romanValue,"Currently Undefined")!=0) { for (int counter = strlen(m_romanValue)-1; counter >= 0; counter--) { if ((m_romanValue[counter] == 'M') || (m_romanValue[counter] == 'm')) { currentNumber = 1000; } else if ((m_romanValue[counter] == 'D') || (m_romanValue[counter] == 'd')) { currentNumber = 500; } else if ((m_romanValue[counter] == 'C') || (m_romanValue[counter] == 'c')) { currentNumber = 100; } else if ((m_romanValue[counter] == 'L') || (m_romanValue[counter] == 'l')) { currentNumber = 50; } else if ((m_romanValue[counter] == 'X') || (m_romanValue[counter] == 'x')) { currentNumber = 10; } else if ((m_romanValue[counter] == 'V') || (m_romanValue[counter] == 'v')) { currentNumber = 5; } else if ((m_romanValue[counter] == 'I') || (m_romanValue[counter] == 'i')) { currentNumber = 1; } else { currentNumber = 0; } //cout<<"previousNumber = " <<previousNumber<<endl; //cout<<"currentNumber = " <<currentNumber<<endl; if (previousNumber > currentNumber) { m_decimalValue -= currentNumber; previousNumber = currentNumber; } else { m_decimalValue += currentNumber; previousNumber = currentNumber; } //cout<<"current m_decimalValue = " <<m_decimalValue<<endl<<endl; } } }// end of ConvertRomanToDecimal int ClassRomanType::ReturnDecimalNumber() { return m_decimalValue; }// end of ReturnDecimalNumber string ClassRomanType::ReturnRomanNumber() { return m_romanValue; }// end of ReturnRomanNumber ClassRomanType::~ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
From the following menu:
1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 9
You selected choice #9 which will:
Error, you entered an invalid command!
Please try again...
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 0
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: Currently Undefined
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 1
You selected choice #1 which will: Get Decimal Number
Enter a Decimal Number: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 3
You selected choice #3 which will: Convert from Decimal to Roman
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: MCMLXXXVII
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 2
You selected choice #2 which will: Get Roman Numeral
Enter a Roman Numeral: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 4
You selected choice #4 which will: Convert from Roman to Decimal
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 2012
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 7
You selected choice #7 which will: Quit
--------------------------------------------------------------------
Bye!
C++ || Snippet – Linked List Custom Template Stack Sample Code
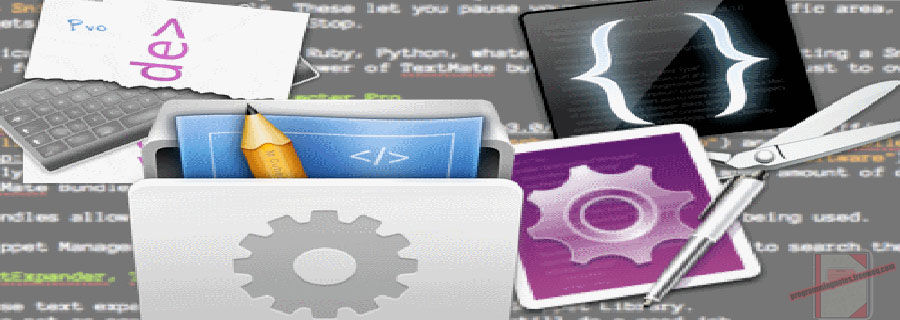
This page will consist of sample code for a custom linked list template stack. This page differs from the previously highlighted array based template stack in that this version uses a singly linked list to store data rather than using an array.
Looking for sample code for a queue? Click here.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Structs
Classes
Template Classes - What Are They?
Stacks
LIFO - What Is It?
#include < stack>
Linked Lists - How To Use
This template class is a custom duplication of the Standard Template Library (STL) stack class. Whether you like building your own data structures, you simply do not like to use any inbuilt functions, opting to build everything yourself, or your homework requires you make your own data structure, this sample code is really useful. I feel its beneficial building functions such as this, that way you better understand the behind the scene processes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 |
// ============================================================================ // Author: K Perkins // Date: Apr 9, 2012 // Taken From: http://programmingnotes.org/ // File: ClassStackListType.h // Description: This is a class which implements various functions // demonstrating the use of a stack. // ============================================================================ #include <iostream> template <class ItemType> class StackListType { public: StackListType(); /* Function: constructor initializes class variables Precondition: none Postcondition: defines private variables */ bool IsEmpty(); /* Function: Determines whether the stack is empty Precondition: Stack has been initialized Postcondition: Function value = (stack is empty) */ bool IsFull(); /* Function: Determines whether the stack is full Precondition: Stack has been initialized Postcondition: Function value = (stack is full) */ int Size(); /* Function: Return the current size of the stack Precondition: Stack has been initialized Postcondition: If (stack is full) exception FullStack is thrown else newItem is at the top of the stack */ void MakeEmpty(); /* Function: Empties the stack Precondition: Stack has been initialized Postcondition: Stack is empty */ void Push(ItemType newItem); /* Function: Adds newItem to the top of the stack Precondition: Stack has been initialized Postcondition: If (stack is full) exception FullStack is thrown else newItem is at the top of the stack */ ItemType Pop(); /* Function: Returns & then removes top item from the stack Precondition: Stack has been initialized Postcondition: If (stack is empty) exception EmptyStack is thrown else top element has been removed from the stack */ ItemType Top(); /* Function: Returns the top item from the stack Precondition: Stack has been initialized Postcondition: If (stack is empty) exception EmptyStack is thrown else top element has been removed from the stack */ ~StackListType(); /* Function: destructor deallocates class variables Precondition: none Postcondition: deallocates private variables */ private: struct NodeType { ItemType currentItem; // Variable which hold all the incoming currentItem NodeType* next; // Creates a pointer that points to the next node in the list. }; int size; // Indicates the size of the stack ItemType junk; NodeType* headPtr; // Creates a head pointer that will point to the begining of the list. }; //========================= Implementation ================================// template<class ItemType> StackListType<ItemType>::StackListType() { size = 0; headPtr = NULL; }// End of StackListType template<class ItemType> bool StackListType<ItemType>::IsEmpty() { return (headPtr == NULL); }// End of IsEmpty template<class ItemType> bool StackListType<ItemType>::IsFull() { try { NodeType* tempPtr = new NodeType; delete tempPtr; return false; } catch(std::bad_alloc&) { return true; } }// End of IsFull template<class ItemType> int StackListType<ItemType>::Size() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; } return size; }// End of Size template<class ItemType> void StackListType<ItemType>::MakeEmpty() { size = 0; if (!IsEmpty()) { std::cout << "Destroying nodes ...n"; while (!IsEmpty()) { NodeType* tempPtr = headPtr; //std::cout << tempPtr-> currentItem << 'n'; headPtr = headPtr-> next; delete tempPtr; } } }// End of MakeEmpty template<class ItemType> void StackListType<ItemType>::Push(ItemType newItem) { if(IsFull()) { std::cout<<"nSTACK FULLn"; return; } NodeType* tempPtr = new NodeType; tempPtr-> currentItem = newItem; tempPtr-> next = headPtr; headPtr = tempPtr; ++size; }// End of Push template<class ItemType> ItemType StackListType<ItemType>::Pop() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; return junk; } else { ItemType data = headPtr-> currentItem; NodeType* tempPtr = headPtr; headPtr = headPtr-> next; delete tempPtr; --size; return data; } }// End of Pop template<class ItemType> ItemType StackListType<ItemType>::Top() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; return junk; } else { return headPtr-> currentItem; } }// End of Top template<class ItemType> StackListType<ItemType>::~StackListType() { MakeEmpty(); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
===== DEMONSTRATION HOW TO USE =====
Use of the above template class is the same as its STL counterpart. Here is a sample program demonstrating its use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
#include <iostream> #include "ClassStackListType.h" using namespace std; int main() { // declare variables StackListType<char> charStack; StackListType<int> intStack; StackListType<float> floatStack; // ------ Char Example ------// char charArry[]="My Programming Notes Is Awesome"; int counter=0; while(charArry[counter]!='\0') { charStack.Push(charArry[counter]); ++counter; } cout<<"charStack has "<<charStack.Size()<<" items in itn" <<"and contains the text ""<<charArry<<"" backwards:n"; while(!charStack.IsEmpty()) { cout<<charStack.Pop(); } cout<<endl; // ------ Int Example ------// int intArry[]={1,22,3,46,5,66,7,8,1987}; counter=0; while(counter<9) { intStack.Push(intArry[counter]); ++counter; } cout<<"nintStack has "<<intStack.Size()<<" items in it.n" <<"The sum of the numbers in the stack is: "; counter=0; while(!intStack.IsEmpty()) { counter+=intStack.Pop(); } cout<<counter<<endl; // ------ Float Example ------// float floatArry[]={41.6,2.8,43.9,4.4,19.87,6.23,7.787,68.99,9.6,81.540}; float sum=0; counter=0; while(counter<10) { floatStack.Push(floatArry[counter]); ++counter; } cout<<"nfloatStack has "<<floatStack.Size()<<" items in it.n" <<"The sum of the numbers in the stack is: "; while(!floatStack.IsEmpty()) { sum+=floatStack.Pop(); } cout<<sum<<endl; }// http://programmingnotes.org/ |
Once compiled, you should get this as your output
charStack has 31 items in it
and contains the text "My Programming Notes Is Awesome" backwards:
emosewA sI setoN gnimmargorP yMintStack has 9 items in it.
The sum of the numbers in the stack is: 2145floatStack has 10 items in it.
The sum of the numbers in the stack is: 286.717
C++ || “One Size Fits All” – BubbleSort Which Works For Integer, Float, & Char Arrays
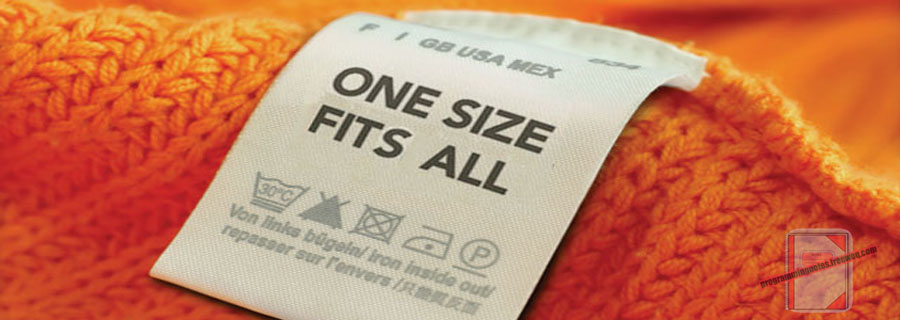
Here is another sorting algorithm, which sorts data that is contained in an array. The difference between this method, and the previous methods of sorting data is that this method works with multiple data types, all in one function. So for example, if you wanted to sort an integer and a character array within the same program, the code demonstrated on this page has the ability to sort both data types, eliminating the need to make two separate sorting functions for the two different data types.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Integer Arrays
Character Arrays
BubbleSort
Pointers
Function Pointers - What Are They?
Memcpy
Sizeof
Sizet
Malloc
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 |
#include <iostream> #include <cstdlib> #include <cctype> #include <iomanip> #include <cstring> #include <ctime> using namespace std; // function prototypes void BubbleSort(void* list, size_t nelm, size_t size, int (*cmp) (const void*, const void*)); void Swap(void* a, void* b, size_t width); int IntCompare (const void * a, const void * b); int StrCompare(const void *a, const void *b); int FloatCompare (const void * a, const void * b); // constant variable for int arrays const int NUM_INTS=14; int main() { // seed random number generator srand(time(NULL)); // =================== INT EXAMPLE =================== // int intNumbers[100]; int numInts=0; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { intNumbers[x] =rand()%100+1; } cout<<"Original values in the int array:n"; // show original data to the user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // this is the function call, which takes in an // integer array for sorting purposes // NOTICE: the function "IntCompare" is being used as a parameter BubbleSort(intNumbers,NUM_INTS,sizeof(int),IntCompare); cout<<"nnThe sorted values in the int array:n"; // display sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== FLOAT EXAMPLE =================== // float floatNumbers[100]; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { floatNumbers[x] =(rand()%100+1)+(0.5); } cout<<"Original values in the float array:n"; // show original array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // this is the function call, which takes in a // float array for sorting purposes // NOTICE: the function "FloatCompare" is being used as a parameter BubbleSort(floatNumbers,NUM_INTS,sizeof(float),FloatCompare); cout<<"nnThe sorted values in the float array:n"; // show sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== CHAR EXAMPLE =================== // char* names[100]={"This", "Is","Random","Text","Brought","To", "You","By","My","Programming","Notes"}; int numNames=11; cout<<"Original values in the char array:n"; // show original array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } // this is the function call, which takes in a // char array for sorting purposes // NOTICE: the function "StrCompare" is being used as a parameter BubbleSort(names,numNames,sizeof(char*),StrCompare); cout<<"nnThe sorted values in the char array:n"; // show sorted array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } cout<<endl; return 0; }// end of main void BubbleSort(void* arry, size_t nelm, size_t size, int (*cmp) (const void*, const void*)) {// this function sorts the received array bool sorted = false; do{ sorted = true; for(unsigned int x=0; x < nelm-1; ++x) { if(cmp((((char*)arry)+(x*size)), (((char*)arry)+((x+1)*size))) > 0) { Swap((((char*)arry)+((x)*size)), (((char*)arry)+((x+1)*size)),size); sorted=false; } } --nelm; }while(!sorted); }// end of BubbleSort void Swap(void* a, void* b, size_t width) {// this function swaps the values in the array void *tmp = malloc(width); memcpy(tmp, a, width); memcpy(a, b, width); memcpy(b, tmp, width); free(tmp); }// end of Swap int FloatCompare (const void * a, const void * b) {// this function compares two float values together return (*(float*)a - *(float*)b); }// end of FloatCompare int IntCompare (const void * a, const void * b) {// this function compares two int values together return (*(int*)a - *(int*)b); }// end of IntCompare int StrCompare(const void *a, const void *b) {// this function compares two char values together return(strcmp(*(const char **)a, *(const char **)b)); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Notice, the same function declaration is being used for all 3 different data types, with the only difference between each function call are the parameters which are being sent out.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: The function works for all three data types)
Original values in the int array:
44 91 43 22 20 100 77 80 84 60 47 91 51 81The sorted values in the int array:
20 22 43 44 47 51 60 77 80 81 84 91 91 100
---------------------------------------------------------------------------------------
Original values in the float array:
49.5 30.5 67.5 50.5 29.5 89.5 78.5 80.5 54.5 7.5 54.5 38.5 56.5 70.5The sorted values in the float array:
7.5 29.5 30.5 38.5 49.5 50.5 54.5 54.5 56.5 67.5 70.5 78.5 80.5 89.5
---------------------------------------------------------------------------------------
Original values in the char array:
This Is Random Text Brought To You By My Programming NotesThe sorted values in the char array:
Brought By Is My Notes Programming Random Text This To You
C++ || Snippet – Array Based Custom Template Stack Sample Code
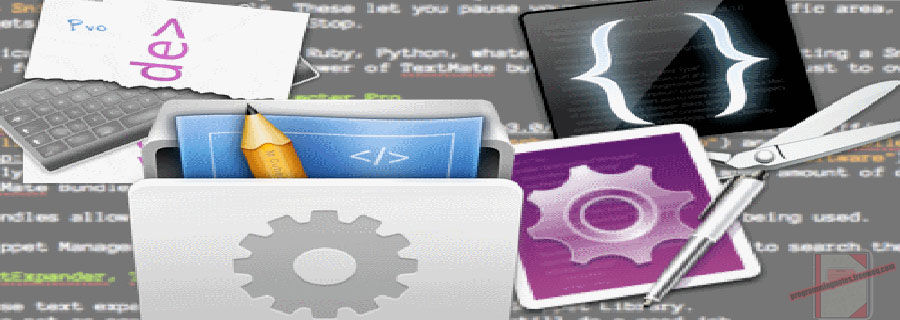
This page will consist of sample code for a custom array based template stack.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Classes
Template Classes - What Are They?
Stacks
LIFO - What Is It?
#include < stack>
This template class is a custom duplication of the Standard Template Library (STL) stack class. Whether you like building your own data structures, you simply do not like to use any inbuilt functions, opting to build everything yourself, or your homework requires you make your own data structure, this sample code is really useful. I feel its beneficial building functions such as this, that way you better understand the behind the scene processes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 |
// ============================================================================ // Author: K Perkins // Date: Mar 22, 2012 // Taken From: http://programmingnotes.org/ // File: ClassStackType.h // Description: This is a class which implements various functions // demonstrating the use of a stack. // ============================================================================ #include <iostream> template <class ItemType> class StackType { public: StackType(); /* Function: constructor initializes class variables Precondition: none Postcondition: defines private variables */ bool IsEmpty(); /* Function: Determines whether the stack is empty Precondition: Stack has been initialized Postcondition: Function value = (stack is empty) */ bool IsFull(); /* Function: Determines whether the stack is full Precondition: Stack has been initialized Postcondition: Function value = (stack is full) */ int Size(); /* Function: Return the current size of the stack Precondition: Stack has been initialized Postcondition: If (stack is full) exception FullStack is thrown else newItem is at the top of the stack */ void MakeEmpty(); /* Function: Empties the stack Precondition: Stack has been initialized Postcondition: Stack is empty */ void Push(ItemType newItem); /* Function: Adds newItem to the top of the stack Precondition: Stack has been initialized Postcondition: If (stack is full) exception FullStack is thrown else newItem is at the top of the stack */ ItemType Pop(); /* Function: Returns & then removes top item from the stack Precondition: Stack has been initialized Postcondition: If (stack is empty) exception EmptyStack is thrown else top element has been removed from the stack */ ItemType Top(); /* Function: Returns the top item from the stack Precondition: Stack has been initialized Postcondition: If (stack is empty) exception EmptyStack is thrown else top element has been removed from the stack */ ~StackType(); /* Function: destructor deallocates class variables Precondition: none Postcondition: deallocates private variables */ private: int top; // indicates which element is on top int MAX_ITEMS; // max number of items in the list ItemType stack[200]; // array holding the popped/pushed data }; //========================= Implementation ================================// template<class ItemType> StackType<ItemType>::StackType() { top = -1; MAX_ITEMS = 200; }// End of StackType template<class ItemType> bool StackType<ItemType>::IsEmpty() { return (top == -1); }// End of IsEmpty template<class ItemType> bool StackType<ItemType>::IsFull() { return (top==(MAX_ITEMS-1)); }// End of IsFull template<class ItemType> int StackType<ItemType>::Size() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; return('?'); } return top+1; }// End of Size template<class ItemType> void StackType<ItemType>::MakeEmpty() { top = -1; }// End of MakeEmpty template<class ItemType> void StackType<ItemType>::Push(ItemType newItem) { if(IsFull()) { std::cout<<"nSTACK FULLn"; return; } stack[++top]=newItem; }// End of Push template<class ItemType> ItemType StackType<ItemType>::Pop() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; return('?'); } return(stack[top--]); }// End of Pop template<class ItemType> ItemType StackType<ItemType>::Top() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; return('?'); } return(stack[top]); }// End of Top template<class ItemType> StackType<ItemType>::~StackType() { top = -1; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
===== DEMONSTRATION HOW TO USE =====
Use of the above template class is the same as its STL counterpart. Here is a sample program demonstrating its use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
#include <iostream> #include "ClassStackType.h" using namespace std; int main() { // declare variables StackType<char> charStack; StackType<int> intStack; StackType<float> floatStack; // ------ Char Example ------// char charArry[]="My Programming Notes"; int counter=0; while(charArry[counter]!=' ') { charStack.Push(charArry[counter]); ++counter; } cout<<"charStack has "<<charStack.Size()<<" items in itn" <<"and contains the text: "<<charArry<<" backwards "; while(!charStack.IsEmpty()) { cout<<charStack.Pop(); } cout<<endl; // ------ Int Example ------// int intArry[]={1,2,3,4,5,6,7,8,9}; counter=0; while(counter<9) { intStack.Push(intArry[counter]); ++counter; } cout<<"intStack has "<<intStack.Size()<<" items in it.n" <<"The sum of the numbers in the stack is: "; counter=0; while(!intStack.IsEmpty()) { counter+=intStack.Pop(); } cout<<counter<<endl; // ------ Float Example ------// float floatArry[]={1.6,2.8,3.9,4.4,5.987,6.23,7.787,8.99,9.6,1.540}; float sum=0; counter=0; while(counter<10) { floatStack.Push(floatArry[counter]); ++counter; } cout<<"floatStack has "<<floatStack.Size()<<" items in it.n" <<"The sum of the numbers in the stack is: "; while(!floatStack.IsEmpty()) { sum+=floatStack.Pop(); } cout<<sum<<endl; }// http://programmingnotes.org/ |
Once compiled, you should get this as your output
charStack has 20 items in it
and contains the text: My Programming Notes
backwards setoN gnimmargorP yMintStack has 9 items in it.
The sum of the numbers in the stack is: 45floatStack has 10 items in it.
The sum of the numbers in the stack is: 52.834
C++ || Snippet – How To Reverse An Integer Using Modulus & While Loop
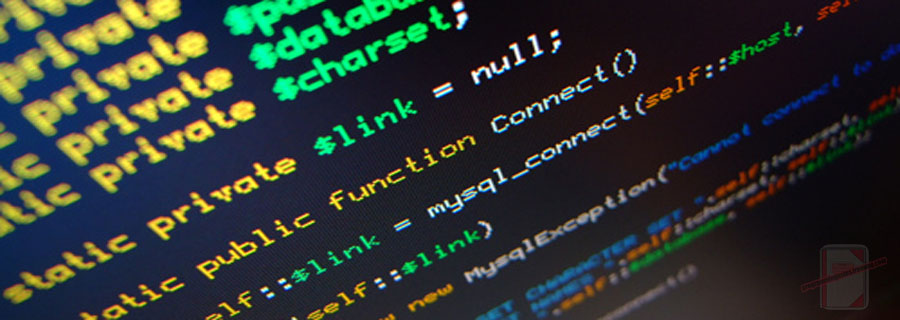
This page will consist of a simple program which demonstrates how to reverse an integer (not an int array) using modulus and a while loop.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
#include <iostream> using namespace std; // function prototype int ReverseNumber(int number); int main() { // declare variables int number = 0; int numReversed = 0; // get data from user cout << "Enter a number: "; cin >> number; // function call which will return the reversed number numReversed = ReverseNumber(number); // display new data to user cout <<endl<< number << " reversed is: "<< numReversed <<endl; return 0; }// end of main int ReverseNumber(int number) { // declare function variable int rev=0; while(number > 0) { rev = rev * 10 + (number % 10); number = number/10; } return rev; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: the code was compiled three separate times to display different output)
==== RUN #1 ====
Enter a number: 2012
2012 reversed is: 2102==== RUN #2 ====
Enter a number: 1987
1987 reversed is: 7891==== RUN #3 ====
Enter a number: 241
241 reversed is: 142
C++ || Char Array – Convert Text Contained In A Character Array From Lower To UPPERCASE
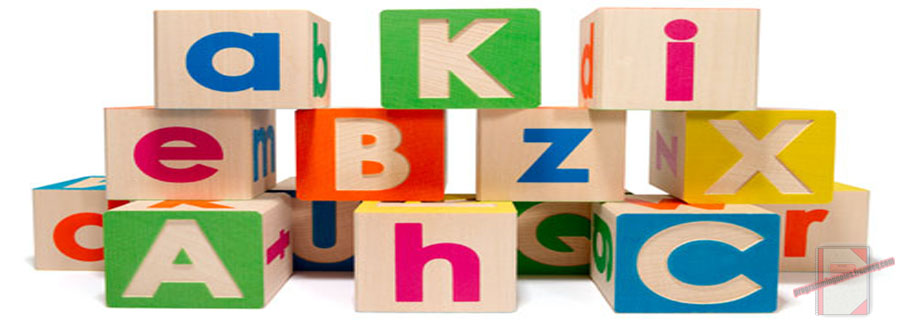
This program demonstrates how to switch text which is contained in a char array from lower to UPPERCASE. This program also demonstrates how to convert all of the text contained in a char array to lower/UPPERCASE.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Character Arrays
Cin.getline
Islower
Isupper
Tolower
Toupper
Strlen
While Loops
For Loops
Constant Variables
Setw
Using a constant integer value, this program first asks the user to enter in 3 lines of text they wish to convert from lower to UPPERCASE. Upon obtaining the information from the user, the program then converts all the text which was placed into the character array from lower to uppercase in the following order:
(1) Switches the text from lower to UPPERCASE
(2) Converts all the text to UPPERCASE
(3) Converts all the text to lowercase
After each conversion is complete, the program displays the updated information to the screen via cout.
NOTE: On some compilers, you may have to add #include < cstdlib>, #include < cctype>, and #include < cstring> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 |
#include <iostream> #include <iomanip> using namespace std; // constant value const int NUM_NAMES = 3; // function prototype void ConvertLowerToUpper(char names[][30]); void ConvertAllToUpper(char names[][30]); void ConvertAllToLower(char names[][30]); int main() { // declare variable // this is a 2-D char array, which by default, has // the ability to hold 3 names, each 30 characters long char names[NUM_NAMES][30]; // get data from user cout << "Please enter "<<NUM_NAMES<<" line(s) of text you wish to convert from lower to UPPERCASE:nt"; for(int index=0; index < NUM_NAMES; ++index) { cout<<"#"<< index+1 <<": "; cin.getline(names[index],30); cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // re-display the input to the screen cout << "nThis is what you entered into the system:nt"; for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // display the switched lower to UPPERCASE text to the user cout << "nThis is the information switched from lower to UPPERCASE:nt"; // function declaration ConvertLowerToUpper(names); for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // display the 'all UPPERCASE' converted text to the user cout << "nThis is the information converted to all UPPERCASE:nt"; // function declaration ConvertAllToUpper(names); for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // display the 'all lowercase' converted text to the user cout << "nThis is the information converted to all lowercase:nt"; // function declaration ConvertAllToLower(names); for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } return 0; }// end of main void ConvertLowerToUpper(char names[][30]) { int index=0; // increment thru the current char array index while(index < NUM_NAMES) { // increment thru each letter within the current char array index for(int currentChar=0; currentChar < strlen(names[index]); ++currentChar) { // checks each letter in the current array index // to see if its lower or UPPERCASE // if its lowercase, change to UPPERCASE if (islower(names[index][currentChar])) { names[index][currentChar] = toupper(names[index][currentChar]); } else // if its UPPERCASE, change to lowercase { names[index][currentChar] = tolower(names[index][currentChar]); } } ++index; } }// end of ConvertLowerToUpper void ConvertAllToUpper(char names[][30]) { int index=0; // increment thru the current char array index while(index < NUM_NAMES) { // increment thru each letter within the current char array index for(int currentChar=0; currentChar < strlen(names[index]); ++currentChar) { // checks each letter in the current array index // to see if its lower or UPPERCASE // if its lowercase, change to UPPERCASE if (islower(names[index][currentChar])) { names[index][currentChar] = toupper(names[index][currentChar]); } } ++index; } }// end of ConvertAllToUpper void ConvertAllToLower(char names[][30]) { int index=0; // increment thru the current char array index while(index < NUM_NAMES) { // increment thru each letter within the current char array index for(int currentChar=0; currentChar < strlen(names[index]); ++currentChar) { // checks each letter in the current array index // to see if its lower or UPPERCASE // if its UPPERCASE, change to lowercase if (isupper(names[index][currentChar])) { names[index][currentChar] = tolower(names[index][currentChar]); } } ++index; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Click here to see how cin.getline works.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Please enter 3 line(s) of text you wish to convert from lower to UPPERCASE:
#1: I StriKe hiM a heAVy bloW.
#2: When cAn the neRve ShinE?
#3: My Programming Notes.------------------------------------------------------------
This is what you entered into the system:
Text #1: I StriKe hiM a heAVy bloW.
Text #2: When cAn the neRve ShinE?
Text #3: My Programming Notes.------------------------------------------------------------
This is the information switched from lower to UPPERCASE:
Text #1: i sTRIkE HIm A HEavY BLOw.
Text #2: wHEN CaN THE NErVE sHINe?
Text #3: mY pROGRAMMING nOTES.------------------------------------------------------------
This is the information converted to all UPPERCASE:
Text #1: I STRIKE HIM A HEAVY BLOW.
Text #2: WHEN CAN THE NERVE SHINE?
Text #3: MY PROGRAMMING NOTES.------------------------------------------------------------
This is the information converted to all lowercase:
Text #1: i strike him a heavy blow.
Text #2: when can the nerve shine?
Text #3: my programming notes.