C# || Two Sum II – How To Get Two Numbers In Sorted Array Equal To Target Value Using C#
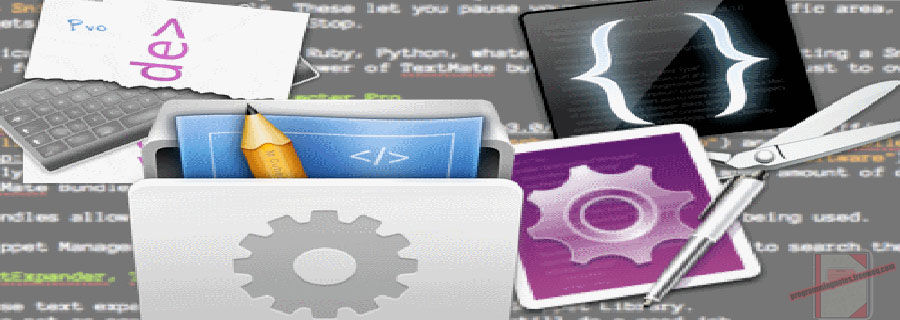
The following is a module with functions which demonstrates how to get two numbers in a sorted array equal to target value using C#.
1. Two Sum II – Problem Statement
Given a 1-indexed array of integers numbers that is already sorted in non-decreasing order, find two numbers such that they add up to a specific target number. Let these two numbers be numbers[index1] and numbers[index2] where 1 <= index1 < index2 <= numbers.length.
Return the indices of the two numbers, index1 and index2, added by one as an integer array [index1, index2] of length 2.
The tests are generated such that there is exactly one solution. You may not use the same element twice.
Example 1:
Input: numbers = [2,7,11,15], target = 9
Output: [1,2]
Explanation: The sum of 2 and 7 is 9. Therefore, index1 = 1, index2 = 2. We return [1, 2].
Example 2:
Input: numbers = [2,3,4], target = 6
Output: [1,3]
Explanation: The sum of 2 and 4 is 6. Therefore index1 = 1, index2 = 3. We return [1, 3].
Example 3:
Input: numbers = [-1,0], target = -1
Output: [1,2]
Explanation: The sum of -1 and 0 is -1. Therefore index1 = 1, index2 = 2. We return [1, 2].
2. Two Sum II – Solution
The following is a solution which demonstrates how to get two numbers in sorted array equal to target value.
In this solution, Binary Search is used to find the two numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 28, 2021 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to get two numbers equal to target value // ============================================================================ public class Solution { public int[] TwoSum(int[] numbers, int target) { var result = new int[2]; var lo = 0; var hi = numbers.Length - 1; while (lo < hi) { var m = lo + (hi - lo) / 2; var sum = numbers[lo] + numbers[hi]; if (sum == target) { result[0] = lo + 1; result[1] = hi + 1; break; } else if (sum < target) { // Determine if mid is less than the remaining value // Increase lo to equal mid if so if (numbers[m] < target - numbers[hi]) { lo = m + 1; } else { lo = lo + 1; } } else { // Determine if mid is greater than the remaining value // Decrease hi to equal mid if so if (numbers[m] > target - numbers[lo]) { hi = m - 1; } else { hi = hi - 1; } } } return result; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[1,2]
[1,3]
[1,2]
Leave a Reply