C# || How To Generate Permutations From Array With Distinct Values Using C#
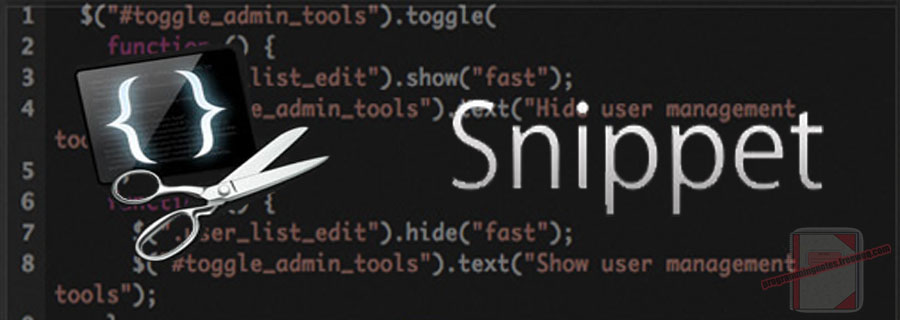
The following is a module with functions which demonstrates how to generate permutations from an array with distinct values using C#.
1. Permute – Problem Statement
Given an array nums of distinct integers, return all the possible permutations. You can return the answer in any order.
Example 1:
Input: nums = [1,2,3]
Output: [[1,2,3],[1,3,2],[2,1,3],[2,3,1],[3,1,2],[3,2,1]]
Example 2:
Input: nums = [0,1]
Output: [[0,1],[1,0]]
Example 3:
Input: nums = [1]
Output: [[1]]
2. Permute – Solution
The following is a solution which demonstrates how to generate permutations from an array with distinct values.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 27, 2021 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to generate permutations // ============================================================================ public class Solution { private List<IList<int>> result = new List<IList<int>>(); public IList<IList<int>> Permute(int[] nums) { Generate(nums, new List<int>(), new bool[nums.Length]); return result; } private void Generate(int[] nums, List<int> combination, bool[] visited) { if (combination.Count == nums.Length) { result.Add(new List<int>(combination)); return; } for (int index = 0; index < nums.Length; ++index) { // Check to see if this index has been visited if (visited[index]) { continue; } // Set that this index has been visited visited[index] = true; // Add this number to the combination combination.Add(nums[index]); // Keep generating permutations Generate(nums, combination, visited); // Unset that this index has been visited visited[index] = false; // Remove last item as its already been explored combination.RemoveAt(combination.Count - 1); } } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[[1,2,3],[1,3,2],[2,1,3],[2,3,1],[3,1,2],[3,2,1]]
[[0,1],[1,0]]
[[1]]
Leave a Reply