C# || How To Remove Excess Whitespace From A String Using C#
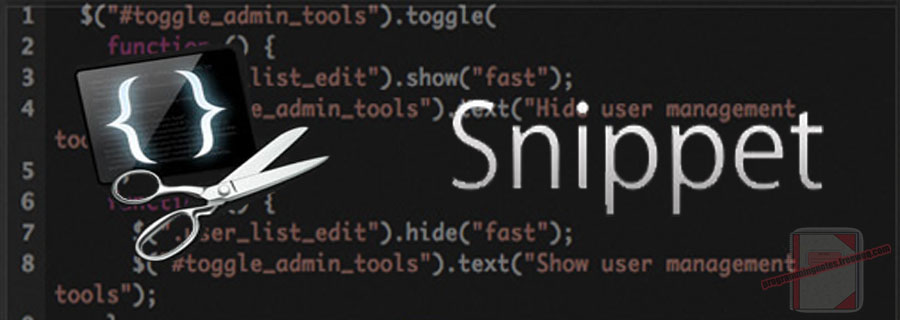
The following is a module with functions which demonstrates how to remove excess whitespace from a string, replacing multiple spaces into just one using C#.
1. Remove Excess Whitespace
The example below demonstrates the use of ‘Utils.Extensions.ToSingleSpace‘ to remove excess whitespace from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Remove Excess Whitespace using Utils; var word = @" This document uses 3 other documents "; // Creates single space string var result = word.ToSingleSpace(); Console.WriteLine(result); // expected output: /* This document uses 3 other documents */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Removes excess whitespace from a string /// </summary> /// <param name="source">The source string</param> /// <returns>The modified source string</returns> public static string ToSingleSpace(this string source) { var pattern = @"\s\s+"; var result = System.Text.RegularExpressions.Regex.Replace(source, pattern, " ").Trim(); return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { var word = @" This document uses 3 other documents "; // Creates single space string var result = word.ToSingleSpace(); Display(result); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply