C# || How To Validate A Phone Number Using C#
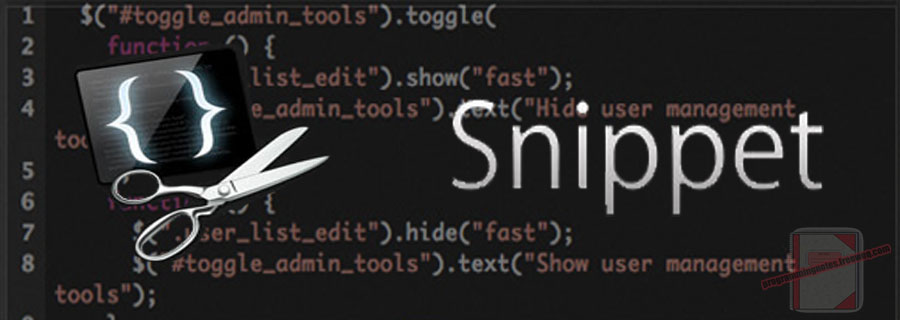
The following is a module with functions which demonstrates how to validate a phone number using C#.
Not only does this function check formatting, but follows NANP numbering rules by ensuring that the first digit in the area code and the first digit in the second section are 2-9.
1. Validate Phone Number
The example below demonstrates the use of ‘Utils.Methods.IsValidPhoneNumber‘ to determine if a phone number is valid.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
// Validate Phone Number var numbers = new List<string>() { "(123) 456-7890", "(123)456-7890", "123-456-7890", "123.456.7890", "1234567890", "+31636363634", "075-63546725", "(657) 278-2011", "(657)278-2011", "(657)2782011", "6572782011", "+6572782011", "657-278-2011", "657 278 2011", "657.278.2011", "1657.278.2011", "+6572782011", "16572782011", "657-2782011" }; // Display results foreach (var number in numbers) { Console.WriteLine($"Number: {number}, Is Valid: {Utils.Methods.IsValidPhoneNumber(number)}"); } // expected output: /* Number: (123) 456-7890, Is Valid: False Number: (123)456-7890, Is Valid: False Number: 123-456-7890, Is Valid: False Number: 123.456.7890, Is Valid: False Number: 1234567890, Is Valid: False Number: +31636363634, Is Valid: False Number: 075-63546725, Is Valid: False Number: (657) 278-2011, Is Valid: True Number: (657)278-2011, Is Valid: True Number: (657)2782011, Is Valid: True Number: 6572782011, Is Valid: True Number: +6572782011, Is Valid: True Number: 657-278-2011, Is Valid: True Number: 657 278 2011, Is Valid: True Number: 657.278.2011, Is Valid: True Number: 1657.278.2011, Is Valid: True Number: +6572782011, Is Valid: True Number: 16572782011, Is Valid: True Number: 657-2782011, Is Valid: True */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 8, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Methods { /// <summary> /// Not only checks formatting, but follows NANP numbering rules /// by ensuring that the first digit in the area code and the first /// digit in the second section are 2-9. /// </summary> /// <param name="phoneNumber">The phone number to verify</param> /// <returns>True if the phone number is valid, false otherwise</returns> public static bool IsValidPhoneNumber(string phoneNumber) { if (string.IsNullOrWhiteSpace(phoneNumber)) { return false; } var pattern = @"^[\+]?[{1}]?[(]?[2-9]\d{2}[)]?[-\s\.]?[2-9]\d{2}[-\s\.]?[0-9]{4}$"; var options = System.Text.RegularExpressions.RegexOptions.Compiled | System.Text.RegularExpressions.RegexOptions.IgnoreCase; return System.Text.RegularExpressions.Regex.IsMatch(phoneNumber, pattern, options); } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 8, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { static void Main(string[] args) { try { var numbers = new List<string>() { "(123) 456-7890", "(123)456-7890", "123-456-7890", "123.456.7890", "1234567890", "+31636363634", "075-63546725", "(657) 278-2011", "(657)278-2011", "(657)2782011", "6572782011", "+6572782011", "657-278-2011", "657 278 2011", "657.278.2011", "1657.278.2011", "+6572782011", "16572782011", "657-2782011" }; foreach (var number in numbers) { Display($"Number: {number}, Is Valid: {Utils.Methods.IsValidPhoneNumber(number)}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Love this man! Really useful 🙂