Assembly || Hello World!
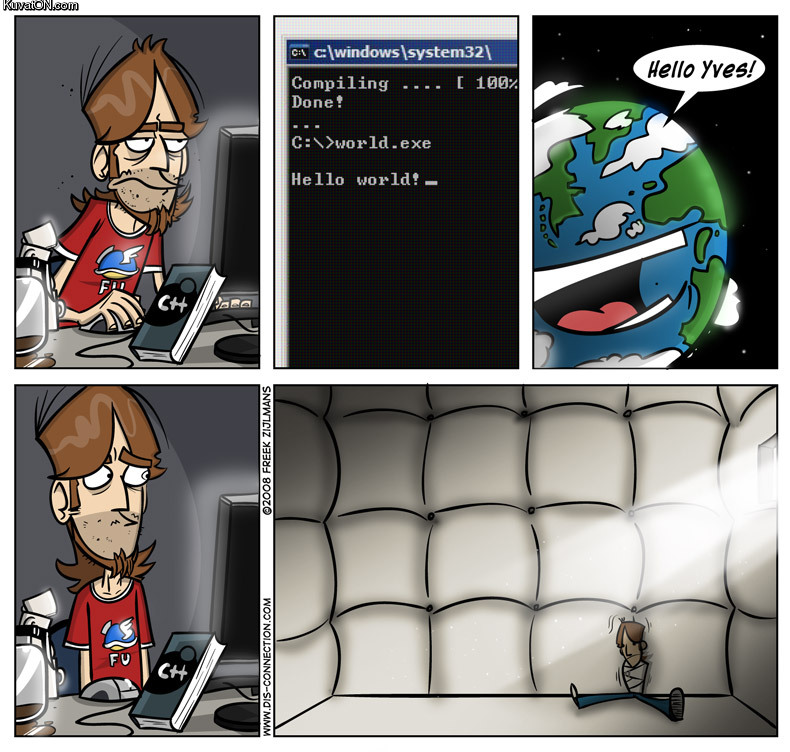
This page will consist of creating the typical “hello world!” application. if you have never programmed in assembly before, this will be very interesting as the syntax is very different from most high level programming languages.
As noted on the introductory page, the assembly code presented on this site (X86-64) was assembled using The Netwide Assembler (NASM) under the Unix platform (Ubuntu) in association with C/C++ files. The purpose of combining Assembly code in association with C/C++ files is to demonstrate how each language “talks” to each other. Also, more importantly it is because today, it is unusual to create a stand alone program written completely in assembly language. Why is that the case? Because It is much easier and faster to program in a high level language than it is in assembly. So why should you learn assembly? Learning assembly can be most useful to help one gain a deeper understanding of how computers work, aswell as helping one to better understand how compilers and higher level languages like C work.
==== HELLO WORLD ====
All of the programs presented on this site will start with a simple C or C++ driver program like so:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
// =================================================================================== // Author: K Perkins // Date: Jul 9, 2012 // Program: Hello World // Taken From: http://programmingnotes.org/ // File: driver.c // // Purpose: This is the driver to the Hello World program. Driver.c only calls // helloWorld.asm. This file is used just as a "driver" and demostrates how to use a // C file along with an Assembly file to create one working program. Once the Assembly // file displays "Hello World" to the screen, control of the program is passed // back to the driver.c file, then the program closes // // ----- These are the commands to link all the files together ------- // // (1) Compile driver.c file: gcc -c -Wall -m64 -std=c99 -l driver.lis -o driver.o driver.c // (2) helloWorld.asm assembler file nasm -f elf64 -l helloWorld.lis -o helloWorld.o helloWorld.asm // (3) Link all files: gcc -m64 -o helloWorld.out driver.o helloWorld.o // (4) Execute in 64-bit protected mode: ./helloWorld.out // // ===== Begin code area ============================================================== #include <stdio.h> // external function prototype extern unsigned long int DisplayHelloWorld(); int main() { // declare variable unsigned long int returnCode = 1987; // display message to the screen printf("nnWelcome to My Programming Notes' Assembly Program.n"); printf("nControl will now be passed to the Assembly file...nn"); // here is a function call to the assembly file, where the asm file passes // back a return code to this current file, which will be displayed below returnCode = DisplayHelloWorld(); printf("nControl has now been passed back from the Assembly file to the C file!n"); printf("nThe return code is: %lu", returnCode); printf("nnBYE!n"); return returnCode; }// http://programmingnotes.org/ |
The “driver” file really only has one task, and that is simply to call the assembly function named ‘DisplayHelloWorld()’ as noted on line 39. This is a routine that will be present among all the code on this site.
There are several advantages in using the C driver routine. First, this lets the C system set up the program to run correctly in protected mode. All the segments and their corresponding segment registers will be initialized by C. The assembly code doesn’t need to worry about any of this. Secondly, the C library will also be available to be used by the assembly code.
The following shows a simple assembly program utilizing the C function “printf” to display ‘Hello World’ to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 |
;===================================================================================== ; Author: K Perkins ; Date: Jul 9, 2012 ; Program: Hello World ; Taken From: http://programmingnotes.org/ ; File: helloWorld.asm ; ; Purpose: This is the helloWorld.asm file which demonstrates how to display text to ; the screen in assembly using C libraries. ; ;===== Begin code area =============================================================== extern printf ;External function printf taken ;from the C library which will be ;used to display output to the screen ; segment .data ;Place initialized data here ; ;======== Text declarations & variables which will be displayed to the user ========== displayMessage db "Hello World!",10, 0 specifierForStringData db "%s", 0 displayLineSeperator db "--------------------------------------------",10,0 ; ;;========= End of text declarations which will be displayed to the user ============= ; segment .bss ;Place un-initialized data here ; segment .text ;Place instruction code here ; global DisplayHelloWorld ;DisplayHelloWorld- the declaration ;that is visible for other programs ;to link to it ; DisplayHelloWorld: ;This is an entry point. Execution ;will begin here. ; ;============= Push registers to the stack =========================================== ;safe programming which pushes all registers to the stack so data doesnt get corrupted push rbp push rbx push rcx push rdx push rdi push rsi push rbp push r8 push r9 push r10 push r11 push r12 push r13 push r14 push r15 ; ;================ End of Push Registers ============================================== ; ; Left side: X86 instructions ;Right side: the narrative 'A.K.A' ;the story about this program. ; ;===== #1 Display Line Seperator ===================================================== mov qword rdi, specifierForStringData ;Prepare printf for string output mov qword rsi, displayLineSeperator ;The 'line seperator' is displayed mov qword rax, 0 ;No vector registers used call printf ;printf is going to output the data ; ;===== #2 Display Hello World ======================================================== mov qword rdi, specifierForStringData ;Prepare printf for string output mov qword rsi, displayMessage ;Hello World will be displayed mov qword rax, 0 ;No vector registers used call printf ;printf is going to output the data ; ;===== #3 Display Line Seperator ===================================================== mov qword rdi, specifierForStringData ;Prepare printf for string output mov qword rsi, displayLineSeperator ;The 'line seperator' is displayed mov qword rax, 0 ;No vector registers used call printf ;printf is going to output the data ; ; END CODE EXECUTION FOR HELLOWORLD.ASM ; ;====== pop the registers back from the stack in reverse order ======================== pop r15 pop r14 pop r13 pop r12 pop r11 pop r10 pop r9 pop r8 pop rbp pop rsi pop rdi pop rdx pop rcx pop rbx pop rbp ; ;===== END - RETURN TO CALLED FUNCTION =============================================== mov rax, 0 ;return 0 to the called function ret ;ret pops the stack taking away 8 bytes ; ; http://programmingnotes.org/ ; ;===== End of DisplayHelloWorld subprogram =========================================== |
Line 16 of the program defines a section that specifies memory to be stored in the data segment (whose name is .data). Only initialized data should be defined in this segment. On lines 19 to 21, several strings are declared. They will be printed with the C library, so they must be terminated with a null character (ASCII code 0). Remember there is a big difference between 0 and ’0’. Note, the number 10 is the ASCII code for a newline.
Uninitialized data should be declared in the .bss segment (named .bss on line 25). This segment gets its name from an early UNIX-based assembler operator that meant “block started by symbol.”
The code segment named .text is where instructions are placed. Note that if you are using Windows, the code label for the main routine (line 29 and 33) should have an underscore prefix, so it would be _DisplayHelloWorld. You would also need to do the same for printf (so it would be _printf). This is part of the C calling convention. This convention specifies the rules C uses when compiling code. It is very important to know this convention when interfacing C and assembly. (Note: This rule is specifically for DOS/Windows, the Linux C compiler does not prepend anything to C symbol names.)
The global directive on line 29 tells the assembler to make the asm main label global. Unlike in C, labels have internal scope by default. This means that only code in the same module can use the label. The global directive gives the specified label (or labels) external scope.
And there you have it! After you assemble the above code (see below), you should get this as your output
Welcome to My Programming Notes' Assembly Program.
Control will now be passed to the Assembly file...
--------------------------------------------
Hello World!
--------------------------------------------Control has now been passed back from the Assembly file to the C file!
The return code is: 0
BYE!
==== ASSEMBLING THE CODE ====
This can be achieved by simply opening the teminal, and doing a copy/paste of the commands listed on the ‘driver.c’ file, lines 16 thru 19. Make sure to compile them in order for the sake of continuity.
1 2 3 4 |
(1) Compile driver.c file: gcc -c -Wall -m64 -std=c99 -l driver.lis -o driver.o driver.c (2) helloWorld.asm assembler file: nasm -f elf64 -l helloWorld.lis -o helloWorld.o helloWorld.asm (3) Link all files: gcc -m64 -o helloWorld.out driver.o helloWorld.o (4) Execute in 64-bit protected mode: ./helloWorld.out |
Be advised, that the commands to assemble the code is designed to run in 64-bit mode. If you are not running a 64-bit machine, the commands will most likely fail to assemble.
If you are running a Windows computer and would like to assemble the code, look here or here for information.
You will need to change the 64-bit registers to 32-bit registers in the “helloWorld.asm” file, aswell as removing lines 38-52 and lines 80-94 respectively in order to run the program successfully.
Leave a Reply