C++ || Savings Account Balance – Calculate The Balance Of A Savings Account At The End Of A Period
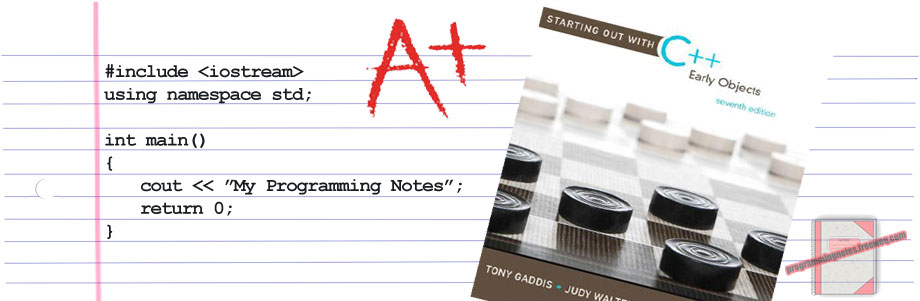
Here is another actual homework assignment which was presented in an intro to programming class. The following program was a question taken from the book “Starting Out with C++: Early Objects (7th Edition),” chapter 5, problem #16.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
For Loops - How To Use Them
Assignment Operators - What Are They?
Setprecision - What Is It?
Interest Rate
This program first prompts the user to enter the annual interest rate, starting balance, and the number of months which has passed since the account was established. Upon obtaining the information, a loop is then used to iterate through each month, performing the following:
• Ask the user for the amount deposited into the account during the month (positive values only). This amount is added to the balance.
• Ask the user for the amount withdrawn from the account during the month (positive values only). This is subtracted from the balance.
• Calculate the monthly interest. The monthly interest rate is the annual interest rate divided by 12. After each loop iteration, the monthly interest rate is multiplied by the current balance, which is then added to the total balance.
After the last iteration, the program displays the ending balance, the total amount of deposits, the total amount of withdrawals, and the total interest earned.
If a negative balance is calculated at any point, an erroneous message is displayed to the screen indicating that the account has been closed, and then the program terminates.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 |
// ============================================================================ // Author: Kenneth Perkins // Date: April 4, 2012 // Taken From: http://programmingnotes.org/ // File: SavingsAccount.cpp // Description: Demonstrates how to calculate the balance of a savings // account at the end of a period // ============================================================================ #include <iostream> #include <cstdlib> #include <iomanip> using namespace std; int main() { // declare variables double annInterestRate = 0; double startingBalance = 0; int monthsPassed = 0; double deposits = 0; double withdrawal = 0; double totDeposits = 0; double totWithdrawal = 0; double totInterest = 0; double monthlyInterestRate = 0; double totBalance = 0; // obtain data from user cout<<"Enter the annual interest rate on the account (e.g .04): "; cin >> annInterestRate; cout<<"Enter the starting balance: $"; cin >> startingBalance; cout<<"How many months have passed since the account was established? "; cin >> monthsPassed; // initialize variables with the required info totBalance = startingBalance; monthlyInterestRate = annInterestRate/12; // use a loop to obtain more data from user for(int x=1; x <= monthsPassed; ++x) { cout<<"\nMonth #"<<x<<endl; cout<<"\tTotal deposits for this month: $"; cin >> deposits; totDeposits += deposits; totBalance += deposits; cout<<"\tTotal withdrawal for this month: $"; cin >> withdrawal; totWithdrawal += withdrawal; totBalance -= withdrawal; totInterest += (totBalance*monthlyInterestRate); totBalance += (totBalance*monthlyInterestRate); // check for negative values // end loop if the values are negative if(deposits < 0 || withdrawal < 0 || totBalance < 0) { cout<< "\nPlease enter positive numbers only!"; cout<<"\nThe account has been closed..\n"; exit(1); } } // display results cout<<fixed<<setprecision(2); cout.fill('.'); cout<<"\nEnding balance:"<<left<<setw(20)<<right<<setw(20)<<" $ "<<totBalance; cout<<"\nAmount of deposits:"<<left<<setw(20)<<right<<setw(16)<<" $ "<<totDeposits; cout<<"\nAmount of withdrawals:"<<left<<setw(20)<<right<<setw(13)<<" $ "<<totWithdrawal; cout<<"\nAmount of interest earned:"<<left<<setw(20)<<right<<setw(9)<<" $ "<<totInterest<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter the annual interest rate on the account (e.g .04): .07
Enter the starting balance: $5000
How many months have passed since the account was established? 3Month #1
Total deposits for this month: $500
Total withdrawal for this month: $120Month #2
Total deposits for this month: $750
Total withdrawal for this month: $200Month #3
Total deposits for this month: $500
Total withdrawal for this month: $100Ending balance:................. $ 6433.47
Amount of deposits:............. $ 1750.00
Amount of withdrawals:.......... $ 420.00
Amount of interest earned:...... $ 103.47
What is the use of those highlighted with yellow? Sorry, Im a beginner that’s why I dont know much about c++
Hello, thanks for visiting!
The highlighted lines merely represent the code that is essential to completing the task of this program
hello can you do th flowchart please
Can you please explain this: totBalance+=(totBalance*monthlyInterestRate);
That expression (located on line #50 in the code) literally means:
totBalance = totBalance + (totBalance*monthlyInterestRate);
This operation is taking your current total balance, and adding to it the calculated interest received for that given month.
This specification can be found in the following program requirements listed above the source code.
Hope this helps.
why you don’t put the first formula of interest after the second formula of balance ?
totBalance += (totBalance*monthlyInterestRate);
totInterest += (totBalance*monthlyInterestRate);
I’m working on the exact same program yet my results are wildly incorrect, can I show my code and get some help fixing it?
This is hard my interest one asks me to average the beginning and end of the month totals. I’m very confused