VB.NET || How To Generate A Random String Of A Specified Length Using VB.NET
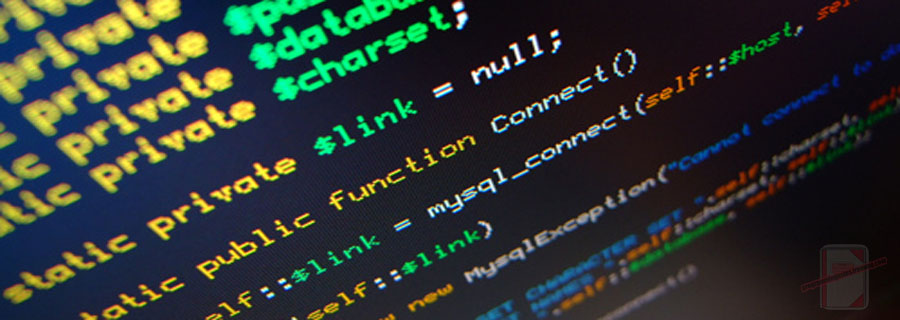
The following is a module with functions which demonstrates how to generate a random code of a specified length using VB.NET.
The function demonstrated on this page has the ability to generate random strings that contains only letters, only numerical digits, or alphanumeric strings.
1. Random Code – Alphabetical
The example below demonstrates the use of ‘Utils.GetRandomCode‘ to generate a code of a specified length that contains only letters.
The optional function parameter determines the type of code that is generated.
1 2 3 4 5 6 7 8 9 10 |
' Random Code - Alphabetical ' Generate code containing only letters Dim letters = Utils.GetRandomCode(5) ' Display the code Debug.Print($"Code: {letters}") ' example output: ' Code: DWhxO |
2. Random Code – Numeric
The example below demonstrates the use of ‘Utils.GetRandomCode‘ to generate a code of a specified length that contains only digits.
The optional function parameter determines the type of code that is generated.
1 2 3 4 5 6 7 8 9 10 |
' Random Code - Numeric ' Generate code containing only digits Dim numeric = Utils.GetRandomCode(7, Utils.CodeType.Numeric) ' Display the code Debug.Print($"Code: {numeric}") ' example output: ' Code: 6407422 |
3. Random Code – Alphanumeric
The example below demonstrates the use of ‘Utils.GetRandomCode‘ to generate a code of a specified length that is alphanumeric.
The optional function parameter determines the type of code that is generated.
1 2 3 4 5 6 7 8 9 10 |
' Random Code - Alphanumeric ' Generate alphanumeric code Dim alphaNumeric = Utils.GetRandomCode(10, Utils.CodeType.AlphaNumeric) ' Display the code Debug.Print($"Code: {alphaNumeric}") ' example output: ' Code: C3G9mt9wf8 |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 30, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils Public Enum CodeType ''' <summary> ''' Code contains only letters ''' </summary> Alphabetical ''' <summary> ''' Code contains only digits ''' </summary> Numeric ''' <summary> ''' Code contains letters and digits ''' </summary> AlphaNumeric End Enum ''' <summary> ''' Generates a random string of a specified length according to the type ''' </summary> ''' <param name="length">The length of the random string</param> ''' <param name="type">The type of string to generate</param> ''' <returns>The random string according to the type</returns> Public Function GetRandomCode(length As Integer _ , Optional type As CodeType = CodeType.Alphabetical) As String Dim digits = "0123456789" Dim alphabet = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ" Dim source = String.Empty Select Case type Case CodeType.Alphabetical source = alphabet Case CodeType.Numeric source = digits Case CodeType.AlphaNumeric source = alphabet + digits Case Else Throw New ArgumentException($"Unknown type: {type}", NameOf(type)) End Select Dim r = New Random Dim result = New System.Text.StringBuilder While result.Length < length result.Append(source(r.Next(0, source.Length))) End While Return result.ToString End Function End Module End Namespace ' http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 30, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ' Generate code containing only letters Dim letters = Utils.GetRandomCode(5) ' Display the code Display($"Code: {letters}") Display("") ' Generate code containing only digits Dim numeric = Utils.GetRandomCode(7, Utils.CodeType.Numeric) ' Display the code Display($"Code: {numeric}") Display("") ' Generate alphanumeric code Dim alphaNumeric = Utils.GetRandomCode(10, Utils.CodeType.AlphaNumeric) ' Display the code Display($"Code: {alphaNumeric}") Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply