VB.NET || How To Get First/Last Day Of The Week And The First/Last Day Of The Month
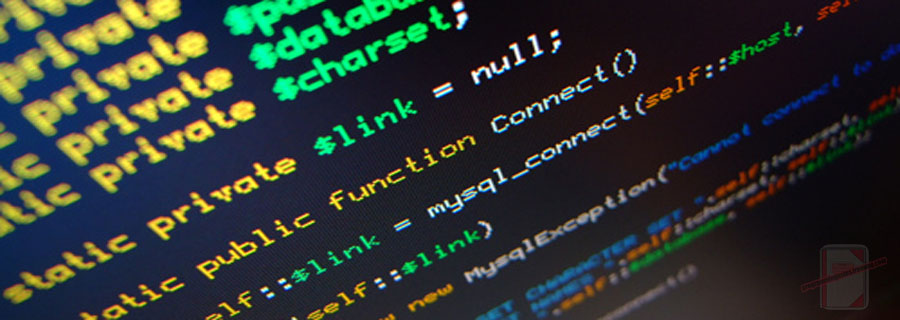
The following is a module with functions which demonstrates how to get the first and last day of the week, as well as how to get the first and last day of the month using VB.NET.
1. First & Last Day Of Week
The example below demonstrates the use of ‘Utils.DateRange.GetWeekStartAndEnd‘ to get the first and last day of the week.
By default, the start of the week is set on Monday. This can be changed by setting the second parameter to any valid property contained in the Microsoft.VisualBasic.FirstDayOfWeek enum.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
' First & Last Day Of Week Dim weekResult = Utils.DateRange.GetWeekStartAndEnd(Date.Today) Debug.Print($" Today = {Date.Today.ToShortDateString} Week Start = {weekResult.StartDate.ToShortDateString} Week End = {weekResult.EndDate.ToShortDateString} ") ' expected output: ' Today = 11/2/2020 ' Week Start = 11/2/2020 ' Week End = 11/8/2020 |
2. First & Last Day Of Month
The example below demonstrates the use of ‘Utils.DateRange.GetMonthStartAndEnd‘ to get the first and last day of the week.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
' First & Last Day Of Month Dim monthResult = Utils.DateRange.GetMonthStartAndEnd(Date.Today) Debug.Print($" Today = {Date.Today.ToShortDateString} Month Start = {monthResult.StartDate.ToShortDateString} Month End = {monthResult.EndDate.ToShortDateString} ") ' expected output: ' Today = 11/2/2020 ' Month Start = 11/1/2020 ' Month End = 11/30/2020 |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 2, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils Public Class DateRange Public Property StartDate As Date Public Property EndDate As Date ''' <summary> ''' Takes in a date and returns the first and last day of the week ''' </summary> ''' <param name="date">The reference date in question</param> ''' <param name="firstDayOfWeek">The day the week starts on</param> ''' <returns>An object that contains the date range result</returns> Public Shared Function GetWeekStartAndEnd([date] As Date, Optional firstDayOfWeek As Microsoft.VisualBasic.FirstDayOfWeek = Microsoft.VisualBasic.FirstDayOfWeek.Monday) As DateRange Dim result = New DateRange result.StartDate = [date].AddDays(1 - Weekday([date], firstDayOfWeek)).Date result.EndDate = result.StartDate.AddDays(6).Date Return result End Function ''' <summary> ''' Takes in a date and returns the first and last day of the month ''' </summary> ''' <param name="date">The reference date in question</param> ''' <returns>An object that contains the date range result</returns> Public Shared Function GetMonthStartAndEnd([date] As Date) As DateRange Dim result = New DateRange result.StartDate = New Date([date].Year, [date].Month, 1).Date result.EndDate = result.StartDate.AddMonths(1).AddDays(-1).Date Return result End Function End Class End Module End Namespace ' http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 2, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Module Program Sub Main(args As String()) Try Dim weekResult = Utils.DateRange.GetWeekStartAndEnd(Date.Today) Display($" Today = {Date.Today.ToShortDateString} Week Start = {weekResult.StartDate.ToShortDateString} Week End = {weekResult.EndDate.ToShortDateString} ") Dim monthResult = Utils.DateRange.GetMonthStartAndEnd(Date.Today) Display($" Today = {Date.Today.ToShortDateString} Month Start = {monthResult.StartDate.ToShortDateString} Month End = {monthResult.EndDate.ToShortDateString} ") Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply