Monthly Archives: April 2012
C++ || Snippet – How To Find The Minimum & Maximum Of 3 Numbers, Print In Ascending Order
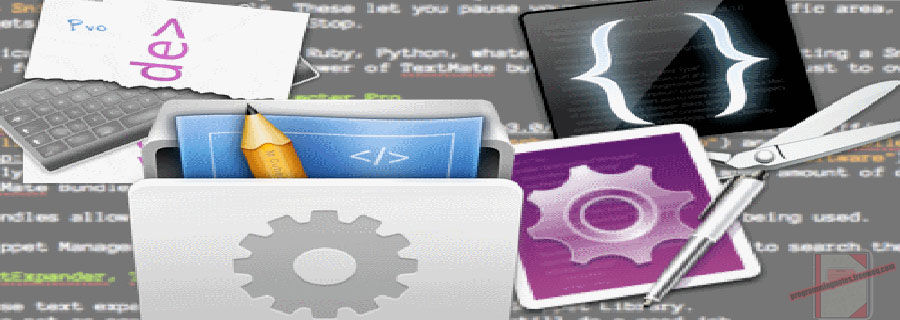
This page will demonstrate how to find the minimum and maximum of 3 numbers. After the maximum and minimum numbers are obtained, the 3 numbers are displayed to the screen in ascending order.
This program uses multiple if-statements to determine equality, and uses 3 seperate int varables to store its data. This program is very basic, so it does not utilize an integer array, or any sorting methods.
NOTE: If you want to find the Minimum & Maximum of numbers contained in an integer array, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
// ============================================================================ // Author: K Perkins // Date: Apr 29, 2012 // Taken From: http://programmingnotes.org/ // File: min_mid_max.cpp // Description: Demonstrates how to find the minimum & maximum of 3 numbers // ============================================================================ #include <iostream> using namespace std; int main() { // declare variables int a=0; int b=0; int c=0; int max=0; int min=0; int middle=0; // get data from user cout<<"Please enter 3 numbers: "; cin >> a >> b >> c; // display information back to the user cout <<"The numbers you just entered are: "<<a<<" "<<b<<" "<<c<<endl; // check for the highest number if(a > b && a > c) { max = a; } else if(b > a && b > c) { max = b; } else { max = c; } // check for the lowest number if(a < b && a < c) { min = a; } else if(b < a && b < c) { min = b; } else { min = c; } // use the 'min' and 'max' variables from above ^ to find // the 'middle' value if((max == a && min == b) || (max == b && min == a)) { middle = c; } else if((max == b && min == c) || (max == c && min == b)) { middle = a; } else { middle = b; } // display data to the screen cout<<"nThe maximum number is: "<<max; cout<<"nThe minimum number is: "<<min; cout<<"nThe numbers in order are: "<<min<<" "<<middle<< " "<<max<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Please enter 3 numbers: 89 56 1987
The numbers you just entered are: 89 56 1987The maximum number is: 1987
The minimum number is: 56
The numbers in order are: 56 89 1987
C++ || Snippet – How To Read & Write Data From A User Specified Text File
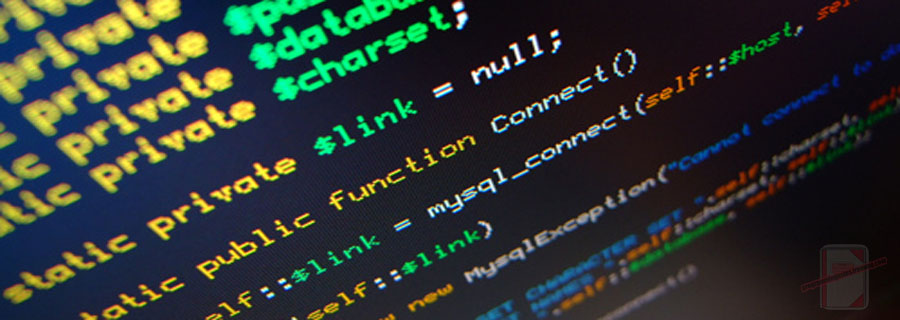
This page will consist of a demonstration of a simple quadratic formula program, which highlights the use of the input/output mechanisms of manipulating a text file. This program is very similar to an earlier snippet which was presented on this site, but in this example, the user has the option of choosing which file they want to manipulate. This program also demonstrates how to read in data from a file (numbers), manipulate that data, and output new data into a different text file.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Fstream
Ifstream
Ofstream
Working With Files
C_str() - Convert A String To Char Array Equivalent
Getline - String Version
Note: The data file that is used in this example can be downloaded here.
Also, in order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
#include <iostream> #include <fstream> #include <string> #include <cmath> #include <cstdlib> using namespace std; int main() { // declare variables // char fileName[80]; string fileName; ifstream infile; ofstream outfile; double a=0,b=0,c=0; double root1=0, root2=0; // get the name of the file from the user cout << "Please enter the name of the file: "; getline(cin, fileName); // ^ you could also use a character array instead // of a string. Your getline declaration would look // like this: // --------------------------------------------- // cin.getline(fileName,80); // this opens the input file // NOTE: you need to convert the string to a // char array using the function "c_str()" infile.open(fileName.c_str()); // ^ if you used a char array as the file name instead // of a string, the declaration to open the file // would look like this: // --------------------------------------------- // infile.open(fileName); // check to see if the file even exists, & if not then EXIT if(infile.fail()) { cout<<"nError, the input file could not be found!n"; exit(1); } // this opens the output file // if the file doesnt already exist, it will be created outfile.open("OUTPUT_Quadratic_programmingnotes_freeweq_com.txt"); // this loop reads in data until there is no more // data contained in the file while(infile.peek() != EOF) { // this assigns the incoming data to the // variables 'a', 'b' and 'c' // NOTE: it is just like a cin >> statement infile>> a >> b>> c; // NOTE: if you want to read data into an array // your declaration would be like this // ------------------------------------ // infile>> a[counter] >> b[counter] >> c[counter]; // ++counter; } // this does the quadratic formula calculations root1 = ((-b) + sqrt(pow(b,2) - (4*a*c)))/(2*a); root2 = ((-b) - sqrt(pow(b,2) - (4*a*c)))/(2*a); // this displays the numbers to screen via cout cout <<"For the numbersna = "<<a<<"nb = "<<b<<"nc = "<<c<<endl; cout <<"nroot 1 = "<<root1<<"nroot 2 = "<<root2<<endl; // this saves the data to the output file // NOTE: its almost exactly the same as the cout statement outfile <<"For the numbersna = "<<a<<"nb = "<<b<<"nc = "<<c<<endl; outfile <<"nroot 1 = "<<root1<<"nroot 2 = "<<root2<<endl; // close the input/output files once you are done using them infile.close(); outfile.close(); // stops the program from automatically closing cout<<"nPress ENTER to continue..."; cin.get(); return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Please enter the name of the file: INPUT_Quadratic_programmingnotes_freeweq_com.txt
For the numbers
a = 2
b = 4
c = -16root 1 = 2
root 2 = -4Press ENTER to continue...
C++ || Snippet – Custom Conio.h Sample Code For Linux
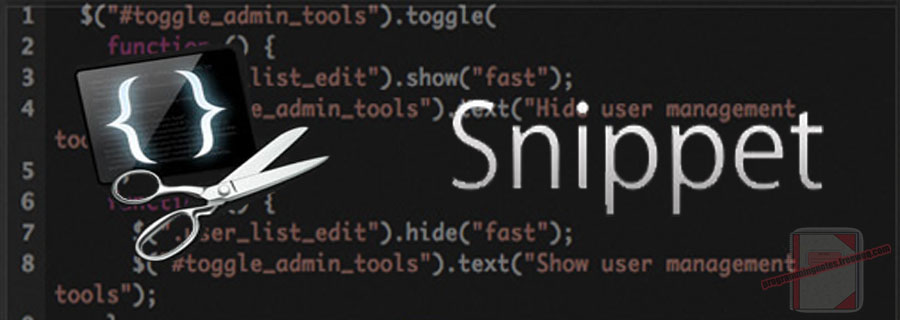
This page will consist of a brief implementation of “conio.h” for Linux.
Conio.h is a C header file used in old MS-DOS compilers to create text user interfaces. It is not part of the C standard library, ISO C, nor is it defined by POSIX. As a result, compilers that target Unix platforms do not contain this header file, and do not implement its library functions.
Included in the sample code are the following:
(1) getch() - Reads a character directly from the console without buffer, and without echo
(2) getche() - Reads a character directly from the console without buffer, but with echo.
(3) kbhit() - Determines if a keyboard key was pressed.
(4) clrscr() - Clears data from the console screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
#include <termios.h> #include <unistd.h> #include <stdio.h> #include <fcntl.h> /* Reads a character directly from the console without buffer, and without echo. */ int getch() { struct termios oldattr, newattr; int ch; tcgetattr( STDIN_FILENO, &oldattr ); newattr = oldattr; newattr.c_lflag &= ~( ICANON | ECHO ); tcsetattr( STDIN_FILENO, TCSANOW, &newattr ); ch = getchar(); tcsetattr( STDIN_FILENO, TCSANOW, &oldattr ); return ch; } /* Reads a character directly from the console without buffer, but with echo */ int getche() { struct termios oldattr, newattr; int ch; tcgetattr( STDIN_FILENO, &oldattr ); newattr = oldattr; newattr.c_lflag &= ~( ICANON ); tcsetattr( STDIN_FILENO, TCSANOW, &newattr ); ch = getchar(); tcsetattr( STDIN_FILENO, TCSANOW, &oldattr ); return ch; } /* Determines if a keyboard key was pressed */ int kbhit() { struct termios oldt, newt; int ch; int oldf; tcgetattr(STDIN_FILENO, &oldt); newt = oldt; newt.c_lflag &= ~(ICANON | ECHO); tcsetattr(STDIN_FILENO, TCSANOW, &newt); oldf = fcntl(STDIN_FILENO, F_GETFL, 0); fcntl(STDIN_FILENO, F_SETFL, oldf | O_NONBLOCK); ch = getchar(); tcsetattr(STDIN_FILENO, TCSANOW, &oldt); fcntl(STDIN_FILENO, F_SETFL, oldf); if(ch != EOF) { ungetc(ch, stdin); return 1; } return 0; } /* Clears data from the console screen */ void clrscr() { char str[] = " [H [2J"; str[3] = str[0] = 27; write(1, str, 7); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Note: Not all functions in conio.h are included in the above sample code.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
===== DEMONSTRATION HOW TO USE =====
Use of the above header file is the same as its DOS counterpart, and can be utilized by simply naming the above snippet as “conio.h.” Here is a sample program demonstrating its use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
#include <iostream> #include "conio.h" using namespace std; int main() { char userInput; while(!kbhit()) { cout<<"Press a key!n"; } userInput=getche(); cout<<"nYou pressed '"<<userInput<<"'!n"; cout<<"nThe screen will now clearn"; cout<<"Press any key to continue..n"; getch(); clrscr(); cout<<"The screen successfully clearedn"; cout<<"Press any key to continue..n"; getch(); return 0; }// http://programmingnotes.org/ |
Once compiled, you should get this as your output
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
Press a key!
p
You pressed 'p'!The screen will now clear
Press any key to continue..[ THE SCREEN CLEARS HERE ]
The screen successfully cleared
Press any key to continue..
C++ || Class & Input/Output – Display The Contents Of A User Specified Text File To The Screen
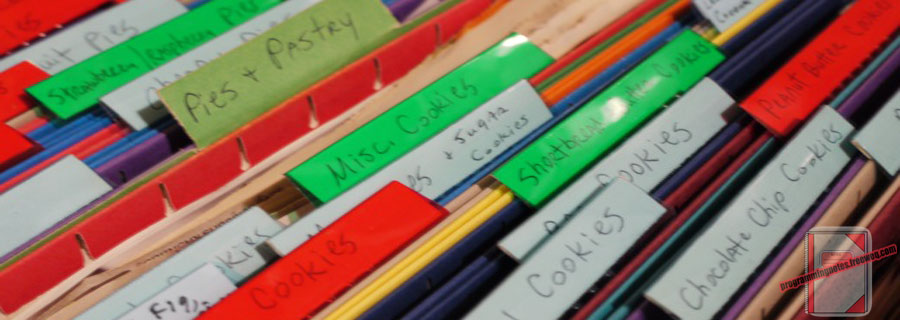
The following is another intermediate homework assignment which was presented in a C++ programming course. This program was assigned to introduce more practice using the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
How To Read Data From A File
String - Getline
Array - Cin.Getline
Strcpy - Copy Contents Of An Array
#Define
This program first prompts the user to input a file name. After it obtains a file name from the user, it then attempts to display the contents of the user specified file to the output screen. If the file could not be found, an error message appears. If the file is found, the program continues as normal. After the file contents finishes being displayed, a summary indicating the total number of lines which has been read is also shown to the screen.
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
Note: The data file that is used in this example can be downloaded here.
Also, in order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
======== FILE #1 – Main.cpp ========
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// ============================================================================ // File: Main.cpp // ============================================================================ // This program tests the "CFileDisp" object. It prompts the user for an input // filename, then attempts to display the contents of that file to stdout. // After the file contents have been displayed, a summary line indicating the // total number of lines that have been displayed is written to stdout. // ============================================================================ #include <iostream> #include <fstream> #include "CFileDisp.h" using namespace std; // ==== main ================================================================== // // ============================================================================ int main() { CFileDisp myFile; char fname[MAX_LENGTH]; int numLines; // get the filename from the user cout << "Enter a filename: "; cin.getline(fname, MAX_LENGTH); // copy the user's filename into the file object myFile.SetFilename(fname); // have the object open the file myFile.OpenFile(); // if all is good and well... if(myFile.IsValid()==true) { numLines = myFile.DisplayFileContents(); // close the file myFile.CloseFile(); // write how many lines were displayed to stdout cout << "nt*** Total lines displayed: " << numLines << endl; } return 0; }// http://programmingnotes.org/ |
======== FILE #2 – CFileDisp.h ========
Remember, you need to name the header file the same as the #include from the Main.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// ============================================================================ // File: CFileDisp.h // ============================================================================ // This is the header file which declares the objects which are used to open // a text file and display its contents to stdout. // ============================================================================ #ifndef CFILE_DISP_HEADER #define CFILE_DISP_HEADER #include <fstream> using namespace std; #define MAX_LENGTH 256 class CFileDisp { public: // constructor CFileDisp(); // member functions void SetFilename(char newFilename[]); /* Purpose: Copy the user's filename into the file object */ void OpenFile(); /* Purpose: Open's the file which is specified by the user */ bool IsValid(); /* Purpose: Checks if the file exists. Post: Returns false if file cannot be found */ int DisplayFileContents(); /* Purpose: Display's the file contents to stdout Post: Returns the total number of lines contained in the file */ void CloseFile(); /* Purpose: Closes the file */ // destructor ~CFileDisp(); private: bool m_bIsValid; char m_filename[MAX_LENGTH]; ifstream m_inFile; }; #endif // CFILE_DISP_HEADER // http://programmingnotes.org/ |
======== FILE #3 – CFileDisp.cpp ========
This is the function implementation file for the CFileDisp.h class. This file can be named anything you wish as long as you #include “CFileDisp.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
// ============================================================================ // File: CFileDisp.cpp // ============================================================================ // This file implements the functions which are declared in the CFileDisp // class, which is located in CFileDisp.h // ============================================================================ #include <iostream> #include <cstring> #include <string> #include "CFileDisp.h" using namespace std; CFileDisp::CFileDisp() { m_bIsValid = 0; }// end of CFileDisp void CFileDisp::SetFilename(char newFilename[]) { strcpy(m_filename,newFilename); }// end of SetFilename void CFileDisp::OpenFile() { m_inFile.open(m_filename); }// end of OpenFile bool CFileDisp::IsValid() { if (m_inFile.fail()) { cout << m_filename << " was not found...nn"; return false; } else { return true; } }// end of IsValid int CFileDisp::DisplayFileContents() { int total=0; string inLine; cout << endl; while(getline(m_inFile, inLine)) { cout << inLine << endl; ++total; } return total; }// end of DisplayFileContents void CFileDisp::CloseFile() { m_inFile.close(); }// end of CloseFile // this is the destructor CFileDisp::~CFileDisp() { m_bIsValid = 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Enter a filename: input_file_display_programmingnotes_freeweq_com.txt 346 130 982 90 656 117 595 415 948 126 4 558 571 87 42 360 412 721 463 47 119 441 190 985 214 509 2 571 77 81 681 651 995 93 74 310 9 995 561 92 14 288 466 664 892 8 766 34 639 151 64 98 813 67 834 369 This is a line of text! *** Total lines displayed: 10 |
C++ || Class – Roman Numeral To Integer & Integer To Roman Numeral Conversion
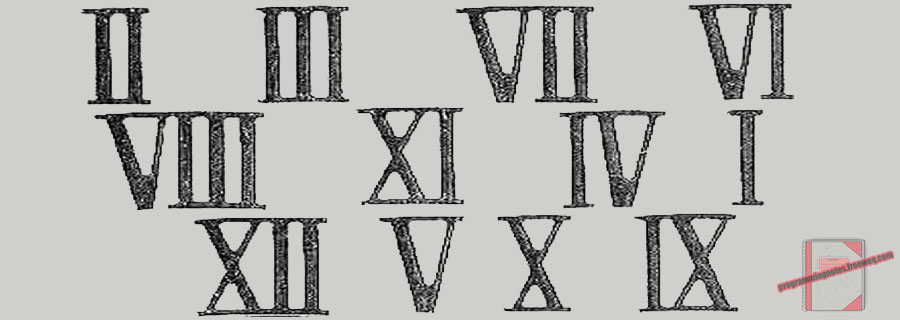
The following is another homework assignment which was presented in a C++ Data Structures course. This program was assigned in order to practice the use of the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
Do/While Loop
Passing a Value By Reference
Roman Numerals - How Do You Convert To Decimal?
Online Roman Numeral Converter - Check For Correct Results
This is an interactive program in which the user has the option of selecting from 7 modes of operation. Of those modes, the user has the option of entering in roman numerals for conversion, entering in decimal numbers for conversion, displaying the recently entered number, and finally converting between roman or decimal values.
A sample of the menu is as followed:
(Where the user would enter numbers 1-7 to select a choice)
1 2 3 4 5 6 7 8 9 10 11 |
From the following menu: 1. Enter a Decimal number 2. Enter a Roman Numeral 3. Convert from Decimal to Roman 4. Convert from Roman to Decimal 5. Print the current Decimal number 6. Print the current Roman Numeral 7. Quit Please enter a selection: |
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
======== FILE #1 – Menu.cpp ========
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
// ============================================================================ // File: Menu.cpp // Purpose: Converts a number enetered in Decimal to Roman Numeral and a // Roman Numeral to a Decimal Number // ============================================================================ #include <iostream> #include <iomanip> #include <string> #include "ClassRomanType.h" using namespace std; // function prototypes void DisplayMenu(); int GetCommand(int command); void RunChoice(int command, ClassRomanType& romanObject); int main() { int command=7; // Holds which action the user chooses to make ClassRomanType romanObject; // Gives access to the class do{ DisplayMenu(); command = GetCommand(command); RunChoice(command, romanObject); cout<<"n--------------------------------------------------------------------n"; }while(command!=7); cout<<"nBye!n"; return 0; }// End of main void RunChoice(int command, ClassRomanType& romanObject) {// Function: Execute the choice selected from the menu // declare private variables int decimalNumber=0; // Holds decimal value char romanNumeral[150]; //Holds value of roman numeral // execute the desired choice cout <<"ntYou selected choice #"<<command<<" which will: "; switch(command) { case 1: cout << "Get Decimal Numbernn"; cout <<"Enter a Decimal Number: "; cin >> decimalNumber; romanObject.GetDecimalNumber(decimalNumber); break; case 2: cout << "Get Roman Numeralnn"; cout <<"nEnter a Roman Numeral: "; cin >> romanNumeral; romanObject.GetRomanNumeral(romanNumeral); break; case 3: cout << "Convert from Decimal to Romannn"; romanObject.ConvertDecimalToRoman(); cout<<"Running....nComplete!n"; break; case 4: cout << "Convert from Roman to Decimalnn"; romanObject.ConvertRomanToDecimal(); cout<<"Running....nComplete!n"; break; case 5: cout << "Print the current Decimal numbernn"; cout<<"n The current Roman to Decimal Value is: "; cout << romanObject.ReturnDecimalNumber()<<endl; break; case 6: cout << "Print the current Roman Numeralnn"; cout<<"n The current Decimal to Roman Value is: "; cout << romanObject.ReturnRomanNumber()<<endl; break; case 7: cout << "Quitnn"; break; default: cout << "nError, you entered an invalid command!nPlease try again..."; break; } }//End of RunChoice int GetCommand(int command) {// Function: Gets choice from menu cout <<"nPlease enter a selection: "; cin>> command; return command; }// End of GetCommand void DisplayMenu() {// Function: Displays menu cout <<"From the following menu:n"<<endl; cout <<left<<setw(2)<<"1."<<left<<setw(15)<<" Enter a Decimal number"<<endl; cout <<left<<setw(2)<<"2."<<left<<setw(15)<<" Enter a Roman Numeral"<<endl; cout <<left<<setw(2)<<"3."<<left<<setw(15)<<" Convert from Decimal to Roman"<<endl; cout <<left<<setw(2)<<"4."<<left<<setw(15)<<" Convert from Roman to Decimal"<<endl; cout <<left<<setw(2)<<"5."<<left<<setw(15)<<" Print the current Decimal number"<<endl; cout <<left<<setw(2)<<"6."<<left<<setw(15)<<" Print the current Roman Numeral"<<endl; cout <<left<<setw(2)<<"7."<<left<<setw(15)<<" Quit"<<endl; }// http://programmingnotes.org/ |
======== FILE #2 – ClassRomanType.h ========
Remember, you need to name the header file the same as the #include from the Menu.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
// ============================================================================ // File: ClassRomanType.h // Purpose: Header file for Roman Numeral conversion. It contains all // declarations and prototypes for the class members to carry out the // conversion // ============================================================================ #include <string> using namespace std; class ClassRomanType { public: ClassRomanType(); /* Function: constructor initializes class variables to "0" Precondition: none Postcondition: defines private variables */ // member functions void GetDecimalNumber(int decimalNumber); /* Function: gets decimal number Precondition: none Postcondition: Gets arabic number from user */ void GetRomanNumeral(char romanNumeral[]); /* Function: gets roman numeral Precondition: none Postcondition: Gets roman numeral from user */ void ConvertDecimalToRoman(); /* Function: converts decimal number to ronman numeral Precondition: arabic and roman numeral characters should be known Postcondition: Gets roman numeral from user */ void ConvertRomanToDecimal(); /* Function: converts roman numeral to arabic number Precondition: arabic and roman numeral characters should be known Postcondition: Gets decimal number from user */ int ReturnDecimalNumber(); /* Function: displays converted decimal number Precondition: the converted roman numeral to decimal number Postcondition: displays decimal number to user */ string ReturnRomanNumber(); /* Function: displays converted roman numeral Precondition: the converted decimal number to roman numeral Postcondition: displays roman numeral to user */ ~ClassRomanType(); /* Function: destructor used to return memory to the system Precondition: the converted decimal number to roman numeral Postcondition: none */ private: char m_romanValue[150]; // An array holding the roman value within the class int m_decimalValue; // Holds the final output answer of the roman to decimal conversion }; // http://programmingnotes.org/ |
======== FILE #3 – RomanType.cpp ========
This is the function implementation file for the ClassRomanType.h class. This file can be named anything you wish as long as you #include “ClassRomanType.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 |
// ============================================================================ // File: RomanType.cpp // Purpose: Contains the implementation of the class members for the // roman numeral conversion // // *NOTE*: Uncomment cout statements to visualize whats happening // ============================================================================ #include <iostream> #include <string> #include <cstring> #include "ClassRomanType.h" using namespace std; ClassRomanType::ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// end of ClassRomanType void ClassRomanType:: GetDecimalNumber(int decimalNumber) { m_decimalValue = decimalNumber; }// end of GetDecimalNumber void ClassRomanType:: GetRomanNumeral(char romanNumeral[]) { strcpy(m_romanValue,romanNumeral); }// end of GetRomanNumeral void ClassRomanType:: ConvertDecimalToRoman() { strcpy(m_romanValue,""); // erases any previous data int numericValue[]={1000,900,500,400,100,90,50,40,10,9,5,4,1,0}; char *romanNumeral[]={"M","CM","D","CD","C","XC","L" ,"XL","X","IX","V","IV","I",0}; for (int x=0; numericValue[x] > 0; ++x) { //cout<<endl<<numericValue[x]<<endl; while (m_decimalValue >= numericValue[x]) { //cout<<"current m_decimalValue = " <<m_decimalValue<<endl; //cout<<"current numericValue = "<<numericValue[x]<<endl<<endl; if(strlen(m_romanValue)==0) { strcpy(m_romanValue,romanNumeral[x]); } else { strcat(m_romanValue,romanNumeral[x]); } m_decimalValue -= numericValue[x]; } } }// end of ConvertDecimalToRoman void ClassRomanType:: ConvertRomanToDecimal() { int currentNumber = 0; // Holds value of each letter numerical value int previousNumber = 0; // Holds the numerical value being compared m_decimalValue=0; // Resets the current 'romanToDecimalValue' to zero if(strcmp(m_romanValue,"Currently Undefined")!=0) { for (int counter = strlen(m_romanValue)-1; counter >= 0; counter--) { if ((m_romanValue[counter] == 'M') || (m_romanValue[counter] == 'm')) { currentNumber = 1000; } else if ((m_romanValue[counter] == 'D') || (m_romanValue[counter] == 'd')) { currentNumber = 500; } else if ((m_romanValue[counter] == 'C') || (m_romanValue[counter] == 'c')) { currentNumber = 100; } else if ((m_romanValue[counter] == 'L') || (m_romanValue[counter] == 'l')) { currentNumber = 50; } else if ((m_romanValue[counter] == 'X') || (m_romanValue[counter] == 'x')) { currentNumber = 10; } else if ((m_romanValue[counter] == 'V') || (m_romanValue[counter] == 'v')) { currentNumber = 5; } else if ((m_romanValue[counter] == 'I') || (m_romanValue[counter] == 'i')) { currentNumber = 1; } else { currentNumber = 0; } //cout<<"previousNumber = " <<previousNumber<<endl; //cout<<"currentNumber = " <<currentNumber<<endl; if (previousNumber > currentNumber) { m_decimalValue -= currentNumber; previousNumber = currentNumber; } else { m_decimalValue += currentNumber; previousNumber = currentNumber; } //cout<<"current m_decimalValue = " <<m_decimalValue<<endl<<endl; } } }// end of ConvertRomanToDecimal int ClassRomanType::ReturnDecimalNumber() { return m_decimalValue; }// end of ReturnDecimalNumber string ClassRomanType::ReturnRomanNumber() { return m_romanValue; }// end of ReturnRomanNumber ClassRomanType::~ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
From the following menu:
1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 9
You selected choice #9 which will:
Error, you entered an invalid command!
Please try again...
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 0
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: Currently Undefined
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 1
You selected choice #1 which will: Get Decimal Number
Enter a Decimal Number: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 3
You selected choice #3 which will: Convert from Decimal to Roman
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: MCMLXXXVII
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 2
You selected choice #2 which will: Get Roman Numeral
Enter a Roman Numeral: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 4
You selected choice #4 which will: Convert from Roman to Decimal
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 2012
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 7
You selected choice #7 which will: Quit
--------------------------------------------------------------------
Bye!
C++ || Snippet – Linked List Custom Template Stack Sample Code
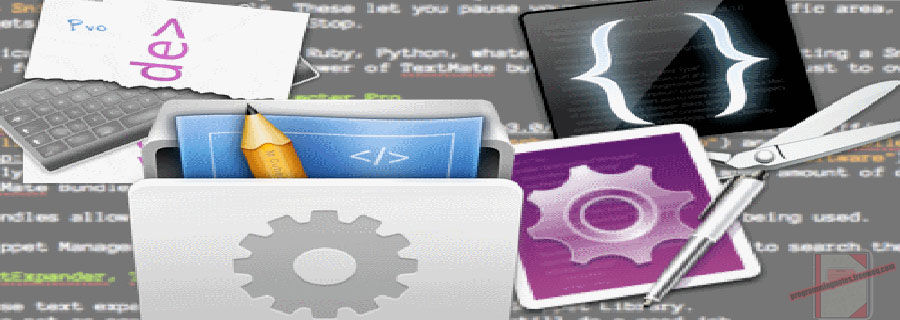
This page will consist of sample code for a custom linked list template stack. This page differs from the previously highlighted array based template stack in that this version uses a singly linked list to store data rather than using an array.
Looking for sample code for a queue? Click here.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Structs
Classes
Template Classes - What Are They?
Stacks
LIFO - What Is It?
#include < stack>
Linked Lists - How To Use
This template class is a custom duplication of the Standard Template Library (STL) stack class. Whether you like building your own data structures, you simply do not like to use any inbuilt functions, opting to build everything yourself, or your homework requires you make your own data structure, this sample code is really useful. I feel its beneficial building functions such as this, that way you better understand the behind the scene processes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 |
// ============================================================================ // Author: K Perkins // Date: Apr 9, 2012 // Taken From: http://programmingnotes.org/ // File: ClassStackListType.h // Description: This is a class which implements various functions // demonstrating the use of a stack. // ============================================================================ #include <iostream> template <class ItemType> class StackListType { public: StackListType(); /* Function: constructor initializes class variables Precondition: none Postcondition: defines private variables */ bool IsEmpty(); /* Function: Determines whether the stack is empty Precondition: Stack has been initialized Postcondition: Function value = (stack is empty) */ bool IsFull(); /* Function: Determines whether the stack is full Precondition: Stack has been initialized Postcondition: Function value = (stack is full) */ int Size(); /* Function: Return the current size of the stack Precondition: Stack has been initialized Postcondition: If (stack is full) exception FullStack is thrown else newItem is at the top of the stack */ void MakeEmpty(); /* Function: Empties the stack Precondition: Stack has been initialized Postcondition: Stack is empty */ void Push(ItemType newItem); /* Function: Adds newItem to the top of the stack Precondition: Stack has been initialized Postcondition: If (stack is full) exception FullStack is thrown else newItem is at the top of the stack */ ItemType Pop(); /* Function: Returns & then removes top item from the stack Precondition: Stack has been initialized Postcondition: If (stack is empty) exception EmptyStack is thrown else top element has been removed from the stack */ ItemType Top(); /* Function: Returns the top item from the stack Precondition: Stack has been initialized Postcondition: If (stack is empty) exception EmptyStack is thrown else top element has been removed from the stack */ ~StackListType(); /* Function: destructor deallocates class variables Precondition: none Postcondition: deallocates private variables */ private: struct NodeType { ItemType currentItem; // Variable which hold all the incoming currentItem NodeType* next; // Creates a pointer that points to the next node in the list. }; int size; // Indicates the size of the stack ItemType junk; NodeType* headPtr; // Creates a head pointer that will point to the begining of the list. }; //========================= Implementation ================================// template<class ItemType> StackListType<ItemType>::StackListType() { size = 0; headPtr = NULL; }// End of StackListType template<class ItemType> bool StackListType<ItemType>::IsEmpty() { return (headPtr == NULL); }// End of IsEmpty template<class ItemType> bool StackListType<ItemType>::IsFull() { try { NodeType* tempPtr = new NodeType; delete tempPtr; return false; } catch(std::bad_alloc&) { return true; } }// End of IsFull template<class ItemType> int StackListType<ItemType>::Size() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; } return size; }// End of Size template<class ItemType> void StackListType<ItemType>::MakeEmpty() { size = 0; if (!IsEmpty()) { std::cout << "Destroying nodes ...n"; while (!IsEmpty()) { NodeType* tempPtr = headPtr; //std::cout << tempPtr-> currentItem << 'n'; headPtr = headPtr-> next; delete tempPtr; } } }// End of MakeEmpty template<class ItemType> void StackListType<ItemType>::Push(ItemType newItem) { if(IsFull()) { std::cout<<"nSTACK FULLn"; return; } NodeType* tempPtr = new NodeType; tempPtr-> currentItem = newItem; tempPtr-> next = headPtr; headPtr = tempPtr; ++size; }// End of Push template<class ItemType> ItemType StackListType<ItemType>::Pop() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; return junk; } else { ItemType data = headPtr-> currentItem; NodeType* tempPtr = headPtr; headPtr = headPtr-> next; delete tempPtr; --size; return data; } }// End of Pop template<class ItemType> ItemType StackListType<ItemType>::Top() { if(IsEmpty()) { std::cout<<"nSTACK EMPTYn"; return junk; } else { return headPtr-> currentItem; } }// End of Top template<class ItemType> StackListType<ItemType>::~StackListType() { MakeEmpty(); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
===== DEMONSTRATION HOW TO USE =====
Use of the above template class is the same as its STL counterpart. Here is a sample program demonstrating its use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
#include <iostream> #include "ClassStackListType.h" using namespace std; int main() { // declare variables StackListType<char> charStack; StackListType<int> intStack; StackListType<float> floatStack; // ------ Char Example ------// char charArry[]="My Programming Notes Is Awesome"; int counter=0; while(charArry[counter]!='\0') { charStack.Push(charArry[counter]); ++counter; } cout<<"charStack has "<<charStack.Size()<<" items in itn" <<"and contains the text ""<<charArry<<"" backwards:n"; while(!charStack.IsEmpty()) { cout<<charStack.Pop(); } cout<<endl; // ------ Int Example ------// int intArry[]={1,22,3,46,5,66,7,8,1987}; counter=0; while(counter<9) { intStack.Push(intArry[counter]); ++counter; } cout<<"nintStack has "<<intStack.Size()<<" items in it.n" <<"The sum of the numbers in the stack is: "; counter=0; while(!intStack.IsEmpty()) { counter+=intStack.Pop(); } cout<<counter<<endl; // ------ Float Example ------// float floatArry[]={41.6,2.8,43.9,4.4,19.87,6.23,7.787,68.99,9.6,81.540}; float sum=0; counter=0; while(counter<10) { floatStack.Push(floatArry[counter]); ++counter; } cout<<"nfloatStack has "<<floatStack.Size()<<" items in it.n" <<"The sum of the numbers in the stack is: "; while(!floatStack.IsEmpty()) { sum+=floatStack.Pop(); } cout<<sum<<endl; }// http://programmingnotes.org/ |
Once compiled, you should get this as your output
charStack has 31 items in it
and contains the text "My Programming Notes Is Awesome" backwards:
emosewA sI setoN gnimmargorP yMintStack has 9 items in it.
The sum of the numbers in the stack is: 2145floatStack has 10 items in it.
The sum of the numbers in the stack is: 286.717
C++ || Printing Various Patterns Using Nested Loops
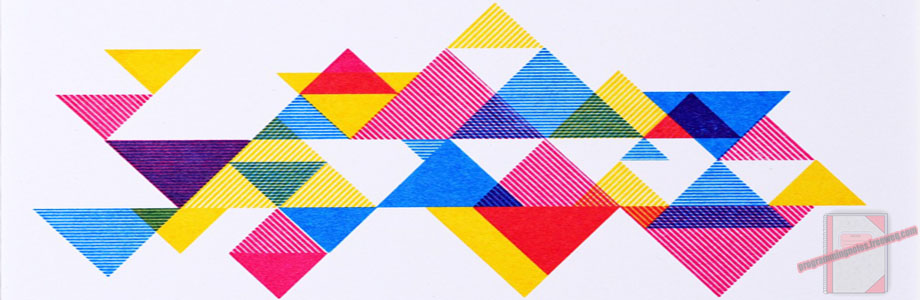
This page will demonstrate various programs which illustrates the use of nested loops to print selected patterns to the screen.
REQUIRED KNOWLEDGE FOR THIS PAGE
The following are famous homework assignments which are usually presented in an entry level programming course.
There are a total of ten (10) different patterns on this page, which is broken up into sections. This page will list:
(4) methods of printing a triangle
(4) methods of printing an upside down triangle
(1) method which prints a square
(1) method which prints a giant letter 'X'
======= PRINTING A TRIANGLE =======
This program prints a triangle shape to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; // get data from user cout<<"Enter a number: "; cin>>number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=0; x < counter; ++x) { cout<<"* "; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
In the above example, the user has a choice of entering the number of rows which will be displayed to the screen
SAMPLE OUTPUT:
Enter a number: 9
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
======= PRINTING A TRIANGLE WITH NUMBERS =======
The following program uses the same concept as above, but this time instead of using stars “*”, numbers will be printed to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=0; x < counter; ++x) { cout<<copy<<" "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9
8 8
7 7 7
6 6 6 6
5 5 5 5 5
4 4 4 4 4 4
3 3 3 3 3 3 3
2 2 2 2 2 2 2 2
1 1 1 1 1 1 1 1 1
======= PRINTING A TRIANGLE WITH NUMBERS IN-ORDER =======
The following program uses the same concept as above, but this time instead of using stars “*”, numbers will be printed to the screen in-order.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { copy=number; for(int x=0; x < counter; ++x) { cout<<copy-(counter-1)<<" "; ++copy; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9
8 9
7 8 9
6 7 8 9
5 6 7 8 9
4 5 6 7 8 9
3 4 5 6 7 8 9
2 3 4 5 6 7 8 9
1 2 3 4 5 6 7 8 9
======= PRINTING A TRIANGLE WITH NUMBERS USING MULTIPLICATION =======
This example demonstrates another triangle, this time printing a multiplication table.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; // get data from user cout<<"Enter a number: "; cin>>number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= counter; ++x) { cout<<number*x<<" "; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9
9 18
9 18 27
9 18 27 36
9 18 27 36 45
9 18 27 36 45 54
9 18 27 36 45 54 63
9 18 27 36 45 54 63 72
9 18 27 36 45 54 63 72 81
======= PRINTING AN UPSIDE-DOWN TRIANGLE =======
This program is similar to the first one, this time printing the triangle upside-down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=0; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= copy; ++x) { cout<<"* "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
* * * * * * * * *
* * * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
======= PRINTING AN UPSIDE-DOWN TRIANGLE WITH NUMBERS =======
This program is similar to the second one, this time printing the triangle upside-down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= copy; ++x) { cout<<copy<<" "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9 9 9 9 9 9 9 9 9
8 8 8 8 8 8 8 8
7 7 7 7 7 7 7
6 6 6 6 6 6
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1
======= PRINTING AN UPSIDE-DOWN TRIANGLE WITH NUMBERS IN-ORDER =======
The following program uses the same concept as above, but this time instead of using stars “*”, numbers will be printed to the screen in-order.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; copy=number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=counter; x <= copy; ++x) { cout<<x<<" "; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
1 2 3 4 5 6 7 8 9
2 3 4 5 6 7 8 9
3 4 5 6 7 8 9
4 5 6 7 8 9
5 6 7 8 9
6 7 8 9
7 8 9
8 9
9
===== PRINTING AN UPSIDE-DOWN TRIANGLE WITH NUMBERS USING MULTIPLICATION =====
This program is similar to the third one, this time printing the triangle upside-down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= copy; ++x) { cout<<number*x<<" "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9 18 27 36 45 54 63 72 81
9 18 27 36 45 54 63 72
9 18 27 36 45 54 63
9 18 27 36 45 54
9 18 27 36 45
9 18 27 36
9 18 27
9 18
9
======= PRINTING A SQUARE =======
This program prints a square to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
#include <iostream> using namespace std; int main() { // declare variables int row=0; int column=0; int number=0; cout<<"Enter the number of rows to be printed: "; cin >> number; row = number; // draw top while (row > 0) { cout<<"*"; --row; } cout<<endl; row = number - 2; column = number; // draw middle while (row > 0) { while (column > 0) { if ((column == number) || (column == 1)) { cout<<"*"; } else { cout<<" "; } --column; } cout<<endl; column = number; --row; } row = number; // draw bottom while (row > 0) { cout<<"*"; --row; } cout<<endl; return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
1 2 3 4 5 6 7 8 9 10 |
Enter the number of rows to be printed: 9 ********* * * * * * * * * * * * * * * ********* |
======= PRINTING THE LETTER “X” =======
The final program for this page will print a giant letter “X” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include <iostream> #include <cstdlib> using namespace std; int main() { // declare variables int number=0; int counter1=0; cout<<"Enter the size of the shape: "; cin >> number; // set 'counter1' equal to 'number' counter1=-number; // start of the nested loop while(counter1 <=number) { for (int counter2 = -number; counter2 <= number; ++counter2) { if (abs(counter1) == abs(counter2)) { cout<<"x"; } else { cout<<" "; } } ++counter1; cout<<endl; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
Enter the size of the shape: 9 x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x |
And there you have it. Simple shapes made possible in C++.
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || Savings Account Balance – Calculate The Balance Of A Savings Account At The End Of A Period
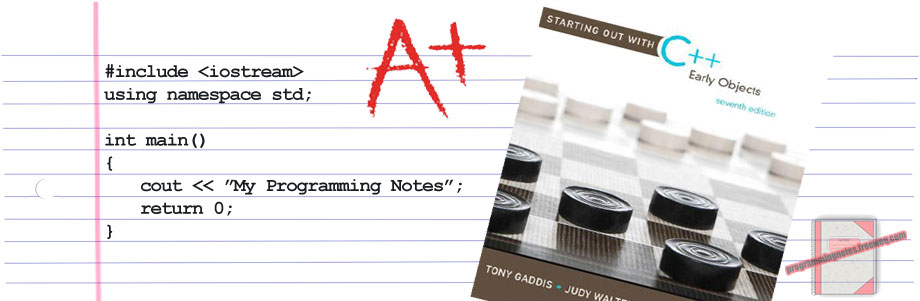
Here is another actual homework assignment which was presented in an intro to programming class. The following program was a question taken from the book “Starting Out with C++: Early Objects (7th Edition),” chapter 5, problem #16.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
For Loops - How To Use Them
Assignment Operators - What Are They?
Setprecision - What Is It?
Interest Rate
This program first prompts the user to enter the annual interest rate, starting balance, and the number of months which has passed since the account was established. Upon obtaining the information, a loop is then used to iterate through each month, performing the following:
• Ask the user for the amount deposited into the account during the month (positive values only). This amount is added to the balance.
• Ask the user for the amount withdrawn from the account during the month (positive values only). This is subtracted from the balance.
• Calculate the monthly interest. The monthly interest rate is the annual interest rate divided by 12. After each loop iteration, the monthly interest rate is multiplied by the current balance, which is then added to the total balance.
After the last iteration, the program displays the ending balance, the total amount of deposits, the total amount of withdrawals, and the total interest earned.
If a negative balance is calculated at any point, an erroneous message is displayed to the screen indicating that the account has been closed, and then the program terminates.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 |
// ============================================================================ // Author: Kenneth Perkins // Date: April 4, 2012 // Taken From: http://programmingnotes.org/ // File: SavingsAccount.cpp // Description: Demonstrates how to calculate the balance of a savings // account at the end of a period // ============================================================================ #include <iostream> #include <cstdlib> #include <iomanip> using namespace std; int main() { // declare variables double annInterestRate = 0; double startingBalance = 0; int monthsPassed = 0; double deposits = 0; double withdrawal = 0; double totDeposits = 0; double totWithdrawal = 0; double totInterest = 0; double monthlyInterestRate = 0; double totBalance = 0; // obtain data from user cout<<"Enter the annual interest rate on the account (e.g .04): "; cin >> annInterestRate; cout<<"Enter the starting balance: $"; cin >> startingBalance; cout<<"How many months have passed since the account was established? "; cin >> monthsPassed; // initialize variables with the required info totBalance = startingBalance; monthlyInterestRate = annInterestRate/12; // use a loop to obtain more data from user for(int x=1; x <= monthsPassed; ++x) { cout<<"\nMonth #"<<x<<endl; cout<<"\tTotal deposits for this month: $"; cin >> deposits; totDeposits += deposits; totBalance += deposits; cout<<"\tTotal withdrawal for this month: $"; cin >> withdrawal; totWithdrawal += withdrawal; totBalance -= withdrawal; totInterest += (totBalance*monthlyInterestRate); totBalance += (totBalance*monthlyInterestRate); // check for negative values // end loop if the values are negative if(deposits < 0 || withdrawal < 0 || totBalance < 0) { cout<< "\nPlease enter positive numbers only!"; cout<<"\nThe account has been closed..\n"; exit(1); } } // display results cout<<fixed<<setprecision(2); cout.fill('.'); cout<<"\nEnding balance:"<<left<<setw(20)<<right<<setw(20)<<" $ "<<totBalance; cout<<"\nAmount of deposits:"<<left<<setw(20)<<right<<setw(16)<<" $ "<<totDeposits; cout<<"\nAmount of withdrawals:"<<left<<setw(20)<<right<<setw(13)<<" $ "<<totWithdrawal; cout<<"\nAmount of interest earned:"<<left<<setw(20)<<right<<setw(9)<<" $ "<<totInterest<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter the annual interest rate on the account (e.g .04): .07
Enter the starting balance: $5000
How many months have passed since the account was established? 3Month #1
Total deposits for this month: $500
Total withdrawal for this month: $120Month #2
Total deposits for this month: $750
Total withdrawal for this month: $200Month #3
Total deposits for this month: $500
Total withdrawal for this month: $100Ending balance:................. $ 6433.47
Amount of deposits:............. $ 1750.00
Amount of withdrawals:.......... $ 420.00
Amount of interest earned:...... $ 103.47
C++ || “One Size Fits All” – BubbleSort Which Works For Integer, Float, & Char Arrays
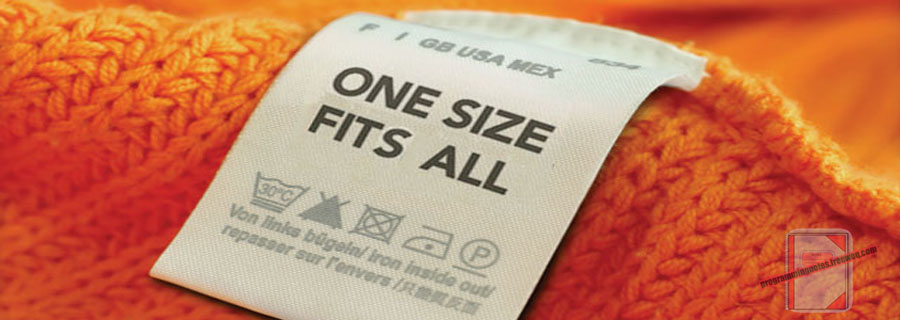
Here is another sorting algorithm, which sorts data that is contained in an array. The difference between this method, and the previous methods of sorting data is that this method works with multiple data types, all in one function. So for example, if you wanted to sort an integer and a character array within the same program, the code demonstrated on this page has the ability to sort both data types, eliminating the need to make two separate sorting functions for the two different data types.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Integer Arrays
Character Arrays
BubbleSort
Pointers
Function Pointers - What Are They?
Memcpy
Sizeof
Sizet
Malloc
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 |
#include <iostream> #include <cstdlib> #include <cctype> #include <iomanip> #include <cstring> #include <ctime> using namespace std; // function prototypes void BubbleSort(void* list, size_t nelm, size_t size, int (*cmp) (const void*, const void*)); void Swap(void* a, void* b, size_t width); int IntCompare (const void * a, const void * b); int StrCompare(const void *a, const void *b); int FloatCompare (const void * a, const void * b); // constant variable for int arrays const int NUM_INTS=14; int main() { // seed random number generator srand(time(NULL)); // =================== INT EXAMPLE =================== // int intNumbers[100]; int numInts=0; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { intNumbers[x] =rand()%100+1; } cout<<"Original values in the int array:n"; // show original data to the user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // this is the function call, which takes in an // integer array for sorting purposes // NOTICE: the function "IntCompare" is being used as a parameter BubbleSort(intNumbers,NUM_INTS,sizeof(int),IntCompare); cout<<"nnThe sorted values in the int array:n"; // display sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== FLOAT EXAMPLE =================== // float floatNumbers[100]; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { floatNumbers[x] =(rand()%100+1)+(0.5); } cout<<"Original values in the float array:n"; // show original array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // this is the function call, which takes in a // float array for sorting purposes // NOTICE: the function "FloatCompare" is being used as a parameter BubbleSort(floatNumbers,NUM_INTS,sizeof(float),FloatCompare); cout<<"nnThe sorted values in the float array:n"; // show sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== CHAR EXAMPLE =================== // char* names[100]={"This", "Is","Random","Text","Brought","To", "You","By","My","Programming","Notes"}; int numNames=11; cout<<"Original values in the char array:n"; // show original array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } // this is the function call, which takes in a // char array for sorting purposes // NOTICE: the function "StrCompare" is being used as a parameter BubbleSort(names,numNames,sizeof(char*),StrCompare); cout<<"nnThe sorted values in the char array:n"; // show sorted array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } cout<<endl; return 0; }// end of main void BubbleSort(void* arry, size_t nelm, size_t size, int (*cmp) (const void*, const void*)) {// this function sorts the received array bool sorted = false; do{ sorted = true; for(unsigned int x=0; x < nelm-1; ++x) { if(cmp((((char*)arry)+(x*size)), (((char*)arry)+((x+1)*size))) > 0) { Swap((((char*)arry)+((x)*size)), (((char*)arry)+((x+1)*size)),size); sorted=false; } } --nelm; }while(!sorted); }// end of BubbleSort void Swap(void* a, void* b, size_t width) {// this function swaps the values in the array void *tmp = malloc(width); memcpy(tmp, a, width); memcpy(a, b, width); memcpy(b, tmp, width); free(tmp); }// end of Swap int FloatCompare (const void * a, const void * b) {// this function compares two float values together return (*(float*)a - *(float*)b); }// end of FloatCompare int IntCompare (const void * a, const void * b) {// this function compares two int values together return (*(int*)a - *(int*)b); }// end of IntCompare int StrCompare(const void *a, const void *b) {// this function compares two char values together return(strcmp(*(const char **)a, *(const char **)b)); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Notice, the same function declaration is being used for all 3 different data types, with the only difference between each function call are the parameters which are being sent out.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: The function works for all three data types)
Original values in the int array:
44 91 43 22 20 100 77 80 84 60 47 91 51 81The sorted values in the int array:
20 22 43 44 47 51 60 77 80 81 84 91 91 100
---------------------------------------------------------------------------------------
Original values in the float array:
49.5 30.5 67.5 50.5 29.5 89.5 78.5 80.5 54.5 7.5 54.5 38.5 56.5 70.5The sorted values in the float array:
7.5 29.5 30.5 38.5 49.5 50.5 54.5 54.5 56.5 67.5 70.5 78.5 80.5 89.5
---------------------------------------------------------------------------------------
Original values in the char array:
This Is Random Text Brought To You By My Programming NotesThe sorted values in the char array:
Brought By Is My Notes Programming Random Text This To You