Tag Archives: pad center
C++ || How To Pad Left, Pad Right & Pad Center A String Of Fixed Length Using C++
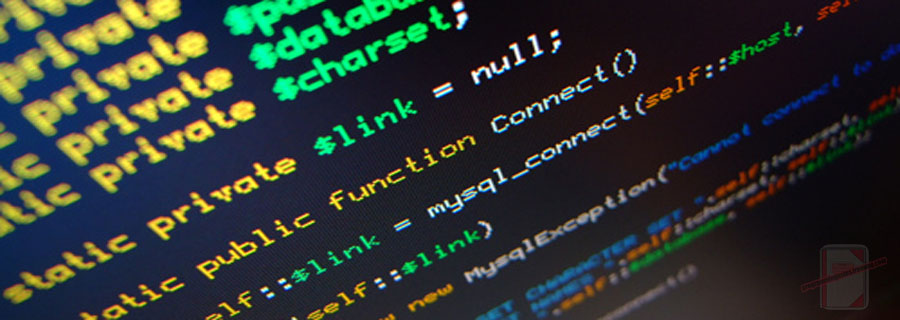
The following is a module with functions which demonstrates how to pad left, pad right, and pad center a string of a fixed length using C++.
The functions demonstrated on this page left, right, and center aligns the characters in a string by padding them on the left and right with a specified character, of a specified total length.
The returned string is padded with as many padding characters needed to reach a length of the specified total width.
The padding character is user defined, but if no padding character is specified, the string is padded using a whitespace (‘ ‘).
1. Pad Left
The example below demonstrates the use of ‘Utils::padLeft‘ to right align the characters in a string by padding them on the left with a specified character, of a specified total length.
In this example, the default padding character is used to pad the string, which is a whitespace (‘ ‘).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Pad Left // Declare string std::string original = "<-Kenneth->"; // Get padding width int width = 15; // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Pad Left: '" << Utils::padLeft(original, width) << "'"; // expected output: /* Original: '<-Kenneth->' Pad Left: ' <-Kenneth->' */ |
2. Pad Right
The example below demonstrates the use of ‘Utils::padRight‘ to left align the characters in a string by padding them on the right with a specified character, of a specified total length.
In this example, the default padding character is used to pad the string, which is a whitespace (‘ ‘).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Pad Right // Declare string std::string original = "<-Kenneth->"; // Get padding width int width = 15; // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Pad Right: '" << Utils::padRight(original, width) << "'"; // expected output: /* Original: '<-Kenneth->' Pad Right: '<-Kenneth-> ' */ |
3. Pad Center
The example below demonstrates the use of ‘Utils::padCenter‘ to center align the characters in a string by padding them on the left and right with a specified character, of a specified total length.
In this example, the default padding character is used to pad the string, which is a whitespace (‘ ‘).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Pad Center // Declare string std::string original = "<-Kenneth->"; // Get padding width int width = 15; // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Pad Center: '" << Utils::padCenter(original, width) << "'"; // expected output: /* Original: '<-Kenneth->' Pad Center: ' <-Kenneth-> ' */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 2, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> namespace Utils { /** * FUNCTION: padLeft * USE: Returns a new string that right aligns the characters in a * string by padding them on the left with a specified * character, of a specified total length * @param source: The source string * @param totalWidth: The number of characters to pad the source string * @param paddingChar: The padding character * @return: The modified source string left padded with as many paddingChar * characters needed to create a length of totalWidth */ std::string padLeft(std::string source, std::size_t totalWidth, char paddingChar = ' ') { if (totalWidth > source.size()) { source.insert(0, totalWidth - source.size(), paddingChar); } return source; } /** * FUNCTION: padRight * USE: Returns a new string that left aligns the characters in a * string by padding them on the right with a specified * character, of a specified total length * @param source: The source string * @param totalWidth: The number of characters to pad the source string * @param paddingChar: The padding character * @return: The modified source string right padded with as many paddingChar * characters needed to create a length of totalWidth */ std::string padRight(std::string source, std::size_t totalWidth, char paddingChar = ' ') { if (totalWidth > source.size()) { source.insert(source.size(), totalWidth - source.size(), paddingChar); } return source; } /** * FUNCTION: padCenter * USE: Returns a new string that center aligns the characters in a * string by padding them on the left and right with a specified * character, of a specified total length * @param source: The source string * @param totalWidth: The number of characters to pad the source string * @param paddingChar: The padding character * @return: The modified source string padded with as many paddingChar * characters needed to create a length of totalWidth */ std::string padCenter(std::string source, std::size_t totalWidth, char paddingChar = ' ') { if (totalWidth > source.size()) { std::size_t totalPadWidth = totalWidth - source.size(); std::size_t leftPadWidth = (totalPadWidth / 2) + source.size(); source = padRight(padLeft(source, leftPadWidth, paddingChar), totalWidth, paddingChar); } return source; } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 2, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare string std::string original = "<-Kenneth->"; // Get padding width int width = 15; // Display result display("Original: '" + original + "'"); display("Pad Center: '" + Utils::padCenter(original, width) + "'"); display("Pad Right: '" + Utils::padRight(original, width) + "'"); display("Pad Left: '" + Utils::padLeft(original, width) + "'"); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Pad Center & Center Align A String Of Fixed Length Using C#
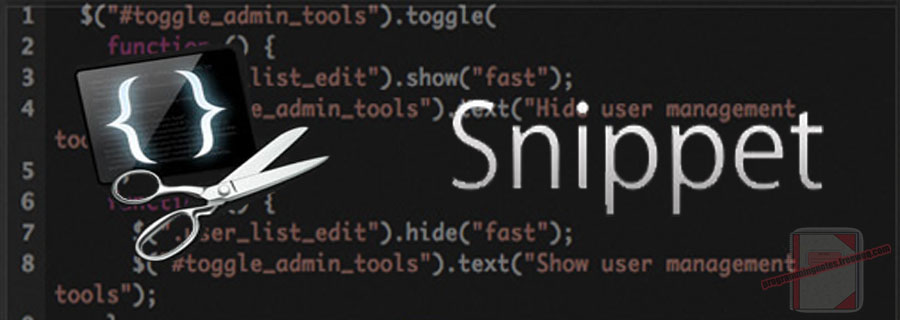
The following is a module with functions which demonstrates how to pad center and center align a string of a fixed length using C#.
The function demonstrated on this page center aligns the characters in a string by padding them on the left and right with a specified character, of a specified total length.
The returned string is padded with as many padding characters needed to reach a length of the specified total width.
The padding character is user defined, but if no padding character is specified, the string is padded using a whitespace (‘ ‘).
1. Pad Center
The example below demonstrates the use of ‘Utils.Extensions.PadCenter‘ to center align a string of a fixed length.
In this example, the default padding character is used to pad the string, which is a whitespace (‘ ‘).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Pad Center using Utils; // Declare string string original = "<-Kenneth->"; // Get padding width int width = 15; // Display result Console.WriteLine("Original: '" + original + "'"); Console.WriteLine("Pad Center: '" + original.PadCenter(width) + "'"); Console.WriteLine("Pad Right: '" + original.PadRight(width) + "'"); Console.WriteLine("Pad Left: '" + original.PadLeft(width) + "'"); // expected output: /* Original: '<-Kenneth->' Pad Center: ' <-Kenneth-> ' Pad Right: '<-Kenneth-> ' Pad Left: ' <-Kenneth->' */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 28, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Returns a new string that center aligns the characters in a /// string by padding them on the left and right with a specified /// character, of a specified total length /// </summary> /// <param name="source">The source string</param> /// <param name="totalWidth">The number of characters to pad the source string</param> /// <param name="paddingChar">The padding character</param> /// <returns>The modified source string padded with as many paddingChar /// characters needed to create a length of totalWidth</returns> public static string PadCenter(this string source, int totalWidth, char paddingChar = ' ') { int spaces = totalWidth - source.Length; int padLeft = spaces / 2 + source.Length; return source.PadLeft(padLeft, paddingChar).PadRight(totalWidth, paddingChar); } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jul 28, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { // Declare string string original = "<-Kenneth->"; // Get padding width int width = 15; // Display result Display("Original: '" + original + "'"); Display("Pad Center: '" + original.PadCenter(width) + "'"); Display("Pad Right: '" + original.PadRight(width) + "'"); Display("Pad Left: '" + original.PadLeft(width) + "'"); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Pad Center & Center Align A String Of Fixed Length Using VB.NET
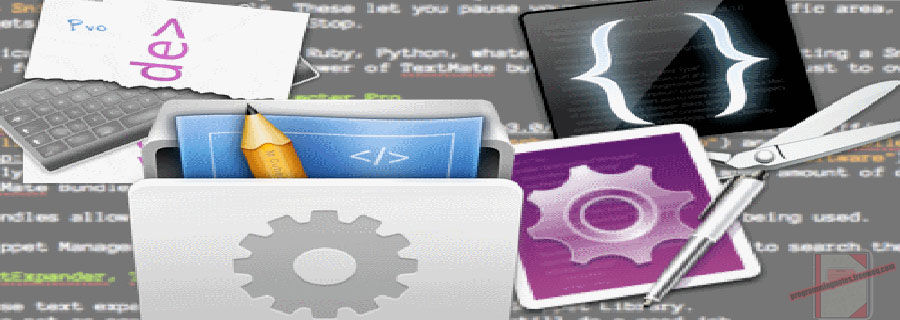
The following is a module with functions which demonstrates how to pad center and center align a string of a fixed length using VB.NET.
The function demonstrated on this page center aligns the characters in a string by padding them on the left and right with a specified character, of a specified total length.
The returned string is padded with as many padding characters needed to reach a length of the specified total width.
The padding character is user defined, but if no padding character is specified, the string is padded using a whitespace (‘ ‘).
1. Pad Center
The example below demonstrates the use of ‘Utils.PadCenter‘ to center align a string of a fixed length.
In this example, the default padding character is used to pad the string, which is a whitespace (‘ ‘).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
' Pad Center Imports Utils ' Declare string Dim original As String = "<-Kenneth->" ' Get padding width Dim width As Integer = 15 ' Display result Debug.Print("Original: '" & original & "'") Debug.Print("Pad Center: '" & original.PadCenter(width) & "'") Debug.Print("Pad Right: '" & original.PadRight(width) & "'") Debug.Print("Pad Left: '" & original.PadLeft(width) & "'") ' expected output: ' Original: '<-Kenneth->' ' Pad Center: ' <-Kenneth-> ' ' Pad Right: '<-Kenneth-> ' ' Pad Left: ' <-Kenneth->' |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Jul 18, 2021 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Returns a new string that center aligns the characters in a ''' string by padding them on the left and right with a specified ''' character, of a specified total length ''' </summary> ''' <param name="source">The source string</param> ''' <param name="totalWidth">The number of characters to pad the source string</param> ''' <param name="paddingChar">The padding character</param> ''' <returns>The modified source string padded with as many paddingChar ''' characters needed to create a length of totalWidth</returns> <Runtime.CompilerServices.Extension()> Public Function PadCenter(source As String, totalWidth As Integer, Optional paddingChar As Char = " "c) As String Dim spaces = totalWidth - source.Length Dim padLeft = CInt(spaces / 2 + source.Length) Return source.PadLeft(padLeft, paddingChar).PadRight(totalWidth, paddingChar) End Function End Module End Namespace ' http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Jul 18, 2021 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Imports Utils Public Module Program Sub Main(args As String()) Try ' Declare string Dim original As String = "<-Kenneth->" ' Get padding width Dim width As Integer = 15 ' Display result Display("Original: '" & original & "'") Display("Pad Center: '" & original.PadCenter(width) & "'") Display("Pad Right: '" & original.PadRight(width) & "'") Display("Pad Left: '" & original.PadLeft(width) & "'") Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.