Tag Archives: for loop
C++ || Snippet – Bubble Sort, Selection Sort, Insertion Sort, Quick Sort & Merge Sort Sample Code For Integer Arrays
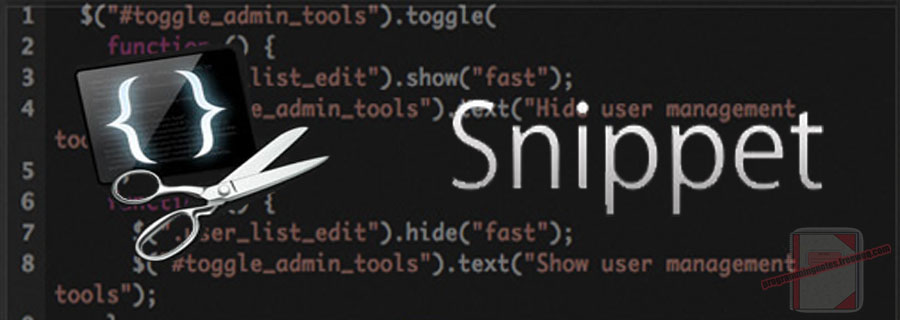
This page consists of algorithms for sorting integer arrays. Highlighted on this page are Bubble Sort, Selection Sort, Insertion Sort, Quick Sort, and Merge Sort.
In terms of performance and speed, the sorting algorithms on this page will be listed from the (on average) worst, to best case implementations.
Selection sort and Insertion sort are two simple sorting algorithms which are often more efficient than Bubble Sort, though all three techniques aren’t the top of the class algorithmically for sorting large data sets.
====== BUBBLE SORT ======
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 11, 2012 // Taken From: http://programmingnotes.org/ // File: bubbleSort.cpp // Description: Demonstrates how to sort an array using bubble sort // ============================================================================ #include <iostream> #include <iomanip> #include <ctime> #include <cstdlib> using namespace std; // const int const int NUM_INTS = 12; // function prototype void BubbleSort(int arry[], int arraySize); int main() { // variable declarations int arry[NUM_INTS]; srand(time(NULL)); // place random numbers into the array for (int x = 0; x < NUM_INTS; ++x) { arry[x] = rand() % 100 + 1; } cout << "Original array values" << endl; // output the original array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } // creates a line seperator cout << "\n--------------------------------------------------------\n"; BubbleSort(arry, NUM_INTS); cout << "The current sorted array" << endl; // output the current sorted array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } cout << endl; cin.get(); return 0; }// end of main void BubbleSort(int arry[], int arraySize) { bool sorted = false; do { sorted = true; for (int x = 0; x < arraySize - 1; ++x) { if (arry[x] > arry[x + 1]) { int temp = arry[x]; arry[x] = arry[x + 1]; arry[x + 1] = temp; sorted = false; } }// end of for loop --arraySize; } while (!sorted); }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Original array values
91 65 53 93 54 41 69 76 55 90 10 62
--------------------------------------------------------
The current sorted array
10 41 53 54 55 62 65 69 76 90 91 93
====== SELECTION SORT ======
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 11, 2012 // Taken From: http://programmingnotes.org/ // File: selectionSort.cpp // Description: Demonstrates how to sort an array using selection sort // ============================================================================ #include <iostream> #include <iomanip> #include <ctime> #include <cstdlib> using namespace std; // const int const int NUM_INTS = 12; // function prototype void SelectionSort(int arry[], int arraySize); int main() { // variable declarations int arry[NUM_INTS]; srand(time(NULL)); // place random numbers into the array for (int x = 0; x < NUM_INTS; ++x) { arry[x] = rand() % 100 + 1; } cout << "Original array values" << endl; // output the original array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } // creates a line seperator cout << "\n--------------------------------------------------------\n"; SelectionSort(arry, NUM_INTS); cout << "The current sorted array" << endl; // output the current sorted array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } cout << endl; cin.get(); return 0; }// end of main void SelectionSort(int arry[], int arraySize) { for (int currentNumber = 0; currentNumber < arraySize; ++currentNumber) { int index_of_min = currentNumber; for (int previousNumber = currentNumber + 1; previousNumber < arraySize; ++previousNumber) { if (arry[index_of_min] > arry[previousNumber]) { index_of_min = previousNumber; } } int temp = arry[currentNumber]; arry[currentNumber] = arry[index_of_min]; arry[index_of_min] = temp; } }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Original array values
87 74 58 64 4 43 23 16 3 93 9 80
--------------------------------------------------------
The current sorted array
3 4 9 16 23 43 58 64 74 80 87 93
====== INSERTION SORT ======
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 11, 2012 // Taken From: http://programmingnotes.org/ // File: insertionSort.cpp // Description: Demonstrates how to sort an array using insertion sort // ============================================================================ #include <iostream> #include <iomanip> #include <ctime> #include <cstdlib> using namespace std; // const int const int NUM_INTS = 12; // function prototype void InsertionSort(int arry[], int arraySize); int main() { // variable declarations int arry[NUM_INTS]; srand(time(NULL)); // place random numbers into the array for (int x = 0; x < NUM_INTS; ++x) { arry[x] = rand() % 100 + 1; } // output the original array values cout << "Original array values" << endl; for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } // creates a line seperator cout << "\n--------------------------------------------------------\n"; InsertionSort(arry, NUM_INTS); // display sorted values cout << "The current sorted array" << endl; // output the current sorted array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } cout << endl; cin.get(); return 0; }// end of main void InsertionSort(int arry[], int arraySize) { int previousIndex = 0; int currentValue = 0; // iterate through entire list for (int index = 1; index < arraySize; ++index) { currentValue = arry[index]; previousIndex = index - 1; while (previousIndex >= 0 && arry[previousIndex] > currentValue) { arry[previousIndex + 1] = arry[previousIndex]; previousIndex = previousIndex - 1; }// end while loop arry[previousIndex + 1] = currentValue; }// end for loop }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Original array values
97 80 94 74 10 38 87 7 87 14 3 97
--------------------------------------------------------
The current sorted array
3 7 10 14 38 74 80 87 87 94 97 97
====== QUICK SORT ======
Quicksort is one of the fastest sorting algorithms, and is often the best practical choice for sorting, as its average expected running time for large data sets is more efficient than the previously discussed methods.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 11, 2012 // Taken From: http://programmingnotes.org/ // File: quickSort.cpp // Description: Demonstrates how to sort an array using quick sort // ============================================================================ #include <iostream> #include <ctime> #include <iomanip> #include <cstdlib> using namespace std; // const int const int NUM_INTS = 12; // function prototypes void QuickSort(int arry[], int arraySize); void QuickSort(int arry[], int start, int end); int Partition(int arry[], int start, int end); int main() { // variable declarations int arry[NUM_INTS]; srand(time(NULL)); // place random numbers into the array for (int x = 0; x < NUM_INTS; ++x) { arry[x] = rand() % 100 + 1; } cout << "Original array values" << endl; // output the original array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } // creates a line seperator cout << "\n--------------------------------------------------------\n"; QuickSort(arry, NUM_INTS); cout << "The current sorted array" << endl; // output the current sorted array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } cout << endl; cin.get(); return 0; }// end of main // the initial function call and initiates the sort void QuickSort(int arry[], int arraySize) { QuickSort(arry, 0, arraySize - 1); }// end of QuickSort // recursive call that carries out the sort void QuickSort(int arry[], int start, int end) { if (start < end) { // partition the arry and get the new pivot position int newPiviotIndex = Partition(arry, start, end); // quick sort the first part QuickSort(arry, start, newPiviotIndex); // quick sort the second part QuickSort(arry, newPiviotIndex + 1, end); } }// end of QuickSort int Partition(int arry[], int start, int end) { // choose a random pivot int pivotIndex = start + rand() % (end - start + 1); std::swap(arry[end], arry[pivotIndex]); // swap pivot with last element int left = start; // left index int right = end; // right index // compare and select smallest from the subarray for (int index = start; index <= right; ++index) { if (arry[index] < arry[right]) { std::swap(arry[index], arry[left]); ++left; } } // move pivot to its final place std::swap(arry[right], arry[left]); return left; // return the position of the new pivot }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Original array values
50 94 1 16 51 63 41 17 70 28 6 34
--------------------------------------------------------
The current sorted array
1 6 16 17 28 34 41 50 51 63 70 94
====== MERGE SORT ======
Merge sort is a fast, stable sorting routine which, in the worst case, does about (on average) 39% fewer comparisons than quick sort.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 11, 2012 // Taken From: http://programmingnotes.org/ // File: mergeSort.cpp // Description: Demonstrates how to sort an array using merge sort // ============================================================================ #include <iostream> #include <iomanip> #include <ctime> #include <cstdlib> using namespace std; // const int const int NUM_INTS = 12; // function prototypes void MergeSort(int arry[], int arraySize); void MergeSort(int arry[], int start, int end); void Merge(int arry[], int start, int midPt, int end); int main() { // variable declarations int arry[NUM_INTS]; srand(time(NULL)); // place random numbers into the array for (int x = 0; x < NUM_INTS; ++x) { arry[x] = rand() % 100 + 1; } cout << "Original array values" << endl; // output the original array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } // creates a line seperator cout << "\n--------------------------------------------------------\n"; MergeSort(arry, NUM_INTS); cout << "The current sorted array" << endl; // output the current sorted for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } cout << endl; cin.get(); return 0; }// end of main // the initial function call and initiates the sort void MergeSort(int arry[], int arraySize) { MergeSort(arry, 0, arraySize - 1); }// end of MergeSort // recursive call that carries out the sort void MergeSort(int arry[], int start, int end) { // no significant comparisons are done during splitting if (start < end) { int midPt = (start + end) / 2; MergeSort(arry, start, midPt); MergeSort(arry, midPt + 1, end); Merge(arry, start, midPt, end); } }// end of MergeSort // sorts the sub array void Merge(int arry[], int start, int midPt, int end) { int leftFirst = start; int leftLast = midPt; int rightFirst = midPt + 1; int rightLast = end; int* tempArray = new int[rightLast + 1]; int index = leftFirst; int saveFirst = leftFirst; while ((leftFirst <= leftLast) && (rightFirst <= rightLast)) {// compare and select smallest from two subarrays if (arry[leftFirst] < arry[rightFirst]) { tempArray[index] = arry[leftFirst]; // smallest assigned to temp ++leftFirst; } else { tempArray[index] = arry[rightFirst]; ++rightFirst; } ++index; } while (leftFirst <= leftLast) { tempArray[index] = arry[leftFirst]; ++leftFirst; ++index; } while (rightFirst <= rightLast) { tempArray[index] = arry[rightFirst]; ++rightFirst; ++index; } for (index = saveFirst; index <= rightLast; ++index) {// copies from temp array to the initial array arry[index] = tempArray[index]; } delete[] tempArray; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Original array values
18 46 41 30 84 97 54 49 19 32 70 30
--------------------------------------------------------
The current sorted array
18 19 30 30 32 41 46 49 54 70 84 97
C++ || Dynamic Arrays – Create A Music Store Database Which Sorts CD Information & Display Grand Total
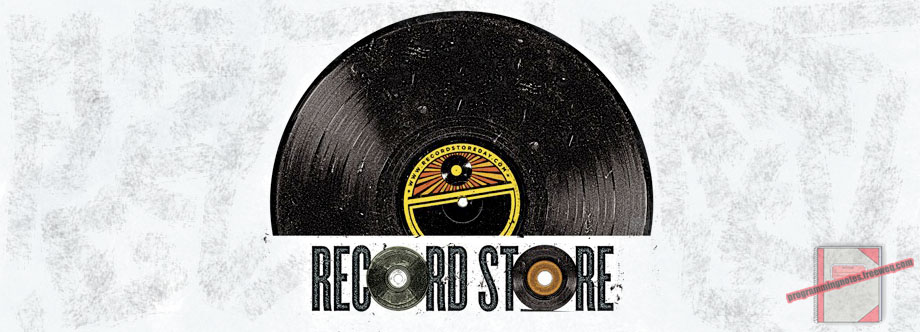
This program was presented as a homework assignment in a programming class to demonstrate the use of dynamic arrays, and pointer variables. The pointer variables which are displayed in this program are very excessive; and many are not needed, but it was good practice when trying to understand the logic behind it all.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Pointer Variables
Dynamic Arrays
2-D Dynamic Arrays - How To Declare
Bubble Sort
While Loops
For Loops
Constant Variables
Functions
Switch Statements
Toupper
Strcpy
Strcmp
This is an interactive program, which simulates a database for a music store, in which the user inputs data into the program (artist, CD title, genre, sales price, tax) and stores that information into multiple dynamic arrays (2-D and/or one dimensional dynamic arrays). The program will also apply any discounts that may currently be available for the selected CD genre, and applies that discount to the current CD information. When the user chooses to quit, the program will sort any data which is currently stored inside the array (by artist) in ascending order, and output the subtotal, tax applied, and grand total for all of the CD information which is entered into the array to the user.
After the program is complete, it will display a summary of the data which was stored into the array like so:
1 2 3 4 5 6 7 8 9 |
My Programming Notes Record Company Sales Report for 2/9/2012 Artist Title SalesPrice Genre DiscountRate SubTotal SalesTax GrandTotal Name1 CD1 12.99 Jazz 18.00% 10.65 1.26 11.91 Name2 CD2 12.99 Rap 7.00% 12.08 1.26 13.34 -------------------------------------------------------------------------------------------------------- Total (2 transactions) 22.73 2.52 25.25 |
NOTE: On some compilers, you may have to add #include < cstdlib> and #include < cstring> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 |
// ============================================================================= // This is an interactive program, which simulates a database for a music store // in which the user inputs data into the program (atrist, CD title, genre, // sales price, tax) and stores the data into a dynamic array. The program will // also apply any discounts that may currently be available to the selected CD // genre, and applies that discount to the current CD. When the user choses // to quit, the program will sort any data that is stored inside the array // (by artist) in ascending order and it will output the subtotal, tax applied, // and grand total for all of the CD information which is entered into the array // ============================================================================= #include <iostream> #include <iomanip> #include <string> #include <ctime> using namespace std; // function prototypes double* GetDiscounts(); void DisplayMenu(); void GetCDInfo(char** &artist, char** &CDTitle, char** &genre,double* &salesPrice, double* &taxRate, int* numItems); void GetDiscountRate(double* salesPrice,char** musicGenre, char** genre,int* numItems, double* &discountPercent,double* discountRate); void GetSubTotal(double* salesPrice, double* discountRate, int* numItems,double* subTotal); void GetTaxAmount(double* salesPrice, double* taxRate, int*numItems,double* salesTax); void GetGrandTotal(double* subTotal,double* salesTax,int* numItems,double* grandTotal); void SortData(char**artist, char**CDTitle, double*salesPrice, char**genre,double*discountPercent, double*subTotal, double*salesTax, double*grandTotal, int* numItems); void DisplayData(char**artist, char**CDTitle, double*salesPrice, char**genre,double*discountPercent, double*subTotal, double*salesTax, double*grandTotal, int* numItems); // constant discount values // these values are defines within the // 'GetDiscounts' function const double* discounts = GetDiscounts(); // ============================================================================= // main // ============================================================================= int main() { //-- Declare Variables START ---// // numItems for artist array int* numItems = new int(0); // 2-D Dynamic array for music genres char** musicGenre = new char*[7]; for(int index=0; index < 7; ++index) {musicGenre[index] = new char[13];} musicGenre[0]= "Classical"; musicGenre[1]= "Country"; musicGenre[2]= "International"; musicGenre[3]= "Jazz"; musicGenre[4]= "Pop"; musicGenre[5]= "Rock"; musicGenre[6]= "Rap"; // 2-D Dynamic Array for artist name char** artist = new char*[50]; for(int index=0; index < 50; ++index) {artist[index] = new char[20];} // 2-D Dynamic array for CD titles char** CDTitle = new char*[50]; for(int index=0; index < 50; ++index) {CDTitle[index] = new char[20];} // 2-D Dynamic array of music genre char** genre = new char*[50]; for(int index=0; index < 50; ++index) {genre[index] = new char[20];} // array for list price double* salesPrice = new double[20]; // array for tax rate double* taxRate = new double[20]; // array for discount amount double* discountRate= new double[20]; // array for sales price double* subTotal = new double[20]; // array for taxamount double* salesTax= new double[20]; // array for grandTotal double* grandTotal = new double[20]; // array for discount percent double* discountPercent = new double[20]; // variable for the while loop menu char* userInput = new char('n'); // variable if the user wants to enter more data char* enterMoreData = new char('y'); //-- Declare Variables END ---// // display menu DisplayMenu(); cin >> userInput; // loop thru menu options while((toupper(*userInput) !='Q')) { switch(toupper(*userInput)) { case 'E': // get artist info GetCDInfo(artist, CDTitle, genre, salesPrice, taxRate, numItems); // get discount GetDiscountRate(salesPrice, musicGenre, genre, numItems,discountPercent, discountRate); // get sub total GetSubTotal(salesPrice, discountRate, numItems,subTotal); // get tax amount GetTaxAmount(salesPrice, taxRate, numItems,salesTax); // get cash price GetGrandTotal(subTotal, salesTax, numItems,grandTotal); // ask if they want to enter more data cout << "nDo you want to enter more CD's? (y/n): "; cin >> *enterMoreData; if(toupper(*enterMoreData)=='Y') { (++*numItems); } else { (++*numItems); *userInput='q'; } break; case 'D': DisplayData(artist, CDTitle, salesPrice, genre, discountPercent,subTotal,salesTax, grandTotal, numItems); break; default: cout<<"nYou have selected an invalid command"; break; } //creates a line separator cout<<endl; cout<<setfill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // if user wants to enter more data, display menu to screen if(toupper(*enterMoreData)=='Y') { DisplayMenu(); cin >> userInput; } }// end of while loop // sort the current data inside the array SortData(artist, CDTitle, salesPrice, genre, discountPercent,subTotal,salesTax, grandTotal, numItems); // display the current data inside the array DisplayData(artist, CDTitle, salesPrice, genre, discountPercent,subTotal,salesTax, grandTotal, numItems); return 0; }// End of Main // ============================================================================= // DisplayMenu // displays the menu to the user // ============================================================================= void DisplayMenu() { cout<<"Welcome to the CD Management System!"; cout<<"nFrom the following menu, select an option"; cout<<"nnE - Enter new CD information into the database"; cout<<"nD - Display the current information in the database"; cout<<"nQ - Quit"; cout<<"nn>> "; }// End of DisplayMenu // ============================================================================= // DisplayData // displays the current contents in the array. If there is nothing in the array // the program promts a message to the user // ============================================================================= void DisplayData(char**artist, char**CDTitle, double*salesPrice, char**genre, double*discountPercent, double*subTotal, double*salesTax, double*grandTotal, int* numItems) { double* totSalePrice = new double(0); double* totSalesTax = new double(0); double* totgrandTotal = new double(0); // variables which will get the current date time_t t = time(0); // get current CPU time struct tm * now = localtime(& t); // if array is empty, display message if(*numItems ==0) { cout<<"nThe database is currently empty!n"; } else { // displays the company header to the user cout<<setfill(' '); cout << "nttttMy Programming Notes Record Company" << "ntttt Sales Report for "<<now->tm_mon + 1<<"/"<<now->tm_mday<<"/"<<now->tm_year + 1900<<"nn"; // displays the categories which will be shown to the user cout <<setw(1)<<right<<"Artist"<<setw(13)<<"Title"<<setw(13)<<"SalesPrice" <<setw(13)<<"Genre"<<setw(20)<<"DiscountRate"<<setw(13)<<"SubTotal" <<setw(13)<<"SalesTax"<<setw(13)<<"GrandTotal"; // displays the data which is currently inside the array's cout<<fixed<<setprecision(2); for(int* index = new int(0); *index < *numItems; ++*index) { cout << endl; cout <<setw(1)<<right<<artist[*index]<<setw(15)<<CDTitle[*index] <<setw(13)<<salesPrice[*index]<<setw(15)<<genre[*index]<<setw(13) <<discountPercent[*index]*100<<"%"<<setw(15)<<subTotal[*index]<<setw(16) <<salesTax[*index]<<setw(13)<<grandTotal[*index]; } // finds the total prices for the entire list of CD's for(int* index = new int(0); *index < *numItems; ++*index) { *totSalePrice+= subTotal[*index]; *totSalesTax+= salesTax[*index]; *totgrandTotal+= grandTotal[*index]; } // creates a line separator cout<<setfill('-'); cout<<endl<<endl<<left<<setw(52)<<""<<right<<setw(52)<<""<<endl; // displays the total to the user cout<< "Total ("<<*numItems<<" transactions)ttttttt" <<*totSalePrice<<"tt"<<*totSalesTax<<"t"<<*totgrandTotal<<endl; } }// End of DisplayData // ============================================================================= // SortData // sorts all the data which is currently present in the arrays via bubble sort // this function does not display any data to the user // ============================================================================= void SortData(char**artist, char**CDTitle, double*salesPrice, char**genre,double*discountPercent, double*subTotal, double*salesTax, double*grandTotal, int* numItems) { // bool which will tell us if sorting is complete bool sorted = false; // temporary variables for sorting purposes only char* tempArtist = new char[20]; char* tempCDTitle= new char[20]; char* tempGenre= new char[20]; double* tempListPrice= new double[20]; double* tempDiscountPercent = new double[20]; double* tempsubTotal = new double[20]; double* tempTaxAmt = new double[20]; double* tempgrandTotal = new double[20]; // this is the bubble sort // which sorts the entries by artist in ascending order while (sorted==false) { sorted=true; for(int* index= new int(0); *index < *numItems-1; ++*index) { // checks the artist to see if they are in the correct order if (strcmp(artist[*index],artist[*index+1]) > 0) { // swaps artist places strcpy(tempArtist, artist[*index]); strcpy(artist[*index], artist[*index+1]); strcpy(artist[*index+1], tempArtist); // swaps CD title strcpy(tempCDTitle, CDTitle[*index]); strcpy(CDTitle[*index], CDTitle[*index+1]); strcpy(CDTitle[*index+1], tempCDTitle); // swaps music genre strcpy(tempGenre, genre[*index]); strcpy(genre[*index], genre[*index+1]); strcpy(genre[*index+1], tempGenre); // swaps the CD price *tempgrandTotal = grandTotal[*index]; grandTotal[*index] = grandTotal[*index+1]; grandTotal[*index+1] = *tempgrandTotal; // swaps the tax amount *tempTaxAmt = salesTax[*index]; salesTax[*index] = salesTax[*index+1]; salesTax[*index+1] = *tempTaxAmt; // swaps the sales price *tempsubTotal = subTotal[*index]; subTotal[*index] = subTotal[*index+1]; subTotal[*index+1] = *tempsubTotal; // swaps the discount percent *tempDiscountPercent = discountPercent[*index]; discountPercent[*index] = discountPercent[*index+1]; discountPercent[*index+1] = *tempDiscountPercent; // swaps the list price *tempListPrice = salesPrice[*index]; salesPrice[*index] = salesPrice[*index+1]; salesPrice[*index+1] = *tempListPrice; // sets the 'sorted' variable to false sorted=false; }// end of if statement }// end for loop }// end while loop }// End of SortData // ============================================================================= // GetGrandTotal // calculates the grand total for the current transaction // ============================================================================= void GetGrandTotal(double* subTotal,double* salesTax,int* numItems,double* grandTotal) { grandTotal[*numItems]= (subTotal[*numItems])+(salesTax[*numItems]); }// End of GetgrandTotal // ============================================================================= // GetTaxAmount // calculates the sales tax for the current transaction // ============================================================================= void GetTaxAmount(double* salesPrice, double* taxRate, int*numItems,double* salesTax) { salesTax[*numItems] = (salesPrice[*numItems])* (taxRate[*numItems]); }// End of GetTaxAmount // ============================================================================= // GetSubTotal // gets the subtotal for the current transaction // ============================================================================= void GetSubTotal(double* salesPrice, double* discountRate, int* numItems,double* subTotal) { subTotal[*numItems] = (salesPrice[*numItems]) - (discountRate[*numItems]); }// End of GetsubTotal // ============================================================================= // GetDiscountRate // gets the discount rate for the currently selected CD // ============================================================================= void GetDiscountRate(double* salesPrice,char** musicGenre, char** genre,int* numItems, double* &discountPercent, double* discountRate) { // comapres the user inputted current genre to the pre-defined // genres as defined in the main function, then assigns a // discount to the current CD if(strcmp(genre[*numItems],musicGenre[0]) == 0) // if music genre is Classical {discountPercent[*numItems] = discounts[0];} else if(strcmp(genre[*numItems],musicGenre[1]) == 0) // if music genre is Country {discountPercent[*numItems] = discounts[1];} else if(strcmp(genre[*numItems],musicGenre[2]) == 0) // if music genre is International {discountPercent[*numItems] = discounts[2];} else if(strcmp(genre[*numItems],musicGenre[3]) == 0) // if music genre is Jazz {discountPercent[*numItems] = discounts[3];} else if(strcmp(genre[*numItems],musicGenre[4]) == 0) // if music genre is Pop {discountPercent[*numItems] = discounts[4];} else if(strcmp(genre[*numItems],musicGenre[5]) == 0) // if music genre is Rock {discountPercent[*numItems] = discounts[5];} else if(strcmp(genre[*numItems],musicGenre[6]) == 0) // if music genre is Rap {discountPercent[*numItems] = discounts[6];} else{discountPercent[*numItems] = discounts[4];} // if music genre is not any of these ^, then there is no discount // assign the discount rate to the current CD discountRate[*numItems] = (discountPercent[*numItems]) * (salesPrice[*numItems]); }// End of GetDiscountRate // ============================================================================= // GetCDInfo // obtains the CD information from the user // ============================================================================= void GetCDInfo(char** &artist, char** &CDTitle, char** &genre, double* &salesPrice, double* &taxRate, int* numItems) { cin.ignore(); cout << "nEnter the name of the artist: "; cin.getline(artist[*numItems],50, 'n'); cout << "nEnter the title of the CD: "; cin.getline(CDTitle[*numItems],50, 'n'); cout << "nEnter the genre: "; cin.getline(genre[*numItems], 20); cout << "nEnter the sales price $"; cin >> salesPrice[*numItems]; cout << "nEnter the sales tax: "; cin >> taxRate[*numItems]; }// End of GetInfo // ============================================================================= // GetDiscounts // returns the discount rate for the selected genre // ============================================================================= double* GetDiscounts() { double* temp = new double[7]; temp[0] = .09; // discount rate for Classical temp[1] = .03; // discount rate for Country temp[2] = .11; // discount rate for International temp[3] = .18; // discount rate for Jazz temp[4] = .00; // discount rate for Pop temp[5] = .10; // discount rate for Rock temp[6] = .07; // discount rate for Rap return temp; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm8
Enter the title of the CD: CD8
Enter the genre: Rock
Enter the sales price $12.99
Enter the sales tax: .098
Do you want to enter more CD's? (y/n): y------------------------------------------------------------
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm7
Enter the title of the CD: CD7
Enter the genre: Country
Enter the sales price $10.99
Enter the sales tax: .078
Do you want to enter more CD's? (y/n): y------------------------------------------------------------
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm6
Enter the title of the CD: CD6
Enter the genre: Pop
Enter the sales price $11.50
Enter the sales tax: .067
Do you want to enter more CD's? (y/n): y------------------------------------------------------------
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm5
Enter the title of the CD: CD5
Enter the genre: Jazz
Enter the sales price $12.24
Enter the sales tax: .045
Do you want to enter more CD's? (y/n): y------------------------------------------------------------
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm4
Enter the title of the CD: CD4
Enter the genre: Other
Enter the sales price $12.99
Enter the sales tax: .094
Do you want to enter more CD's? (y/n): y------------------------------------------------------------
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm3
Enter the title of the CD: CD3
Enter the genre: Classical
Enter the sales price $11.45
Enter the sales tax: .078
Do you want to enter more CD's? (y/n): y------------------------------------------------------------
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm2
Enter the title of the CD: CD2
Enter the genre: International
Enter the sales price $10.99
Enter the sales tax: .093
Do you want to enter more CD's? (y/n): y------------------------------------------------------------
Welcome to the CD Management System!
From the following menu, select an optionE - Enter new CD information into the database
D - Display the current information in the database
Q - Quit>> e
Enter the name of the artist: Nm1
Enter the title of the CD: CD1
Enter the genre: Rap
Enter the sales price $12.99
Enter the sales tax: .0975
Do you want to enter more CD's? (y/n): n
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
My Programming Notes Record Company Sales Report for 2/9/2012 Artist Title SalesPrice Genre DiscountRate SubTotal SalesTax GrandTotal Nm1 CD1 12.99 Rap 7.00% 12.08 1.27 13.35 Nm2 CD2 10.99 International 11.00% 9.78 1.02 10.80 Nm3 CD3 11.45 Classical 9.00% 10.42 0.89 11.31 Nm4 CD4 12.99 Other 0.00% 12.99 1.22 14.21 Nm5 CD5 12.24 Jazz 18.00% 10.04 0.55 10.59 Nm6 CD6 11.50 Pop 0.00% 11.50 0.77 12.27 Nm7 CD7 10.99 Country 3.00% 10.66 0.86 11.52 Nm8 CD8 12.99 Rock 10.00% 11.69 1.27 12.96 -------------------------------------------------------------------------------------------------------- Total (8 transactions) 89.16 7.85 97.01 |
C++ || Snippet – How To Input Numbers Into An Integer Array & Display Its Contents Back To User
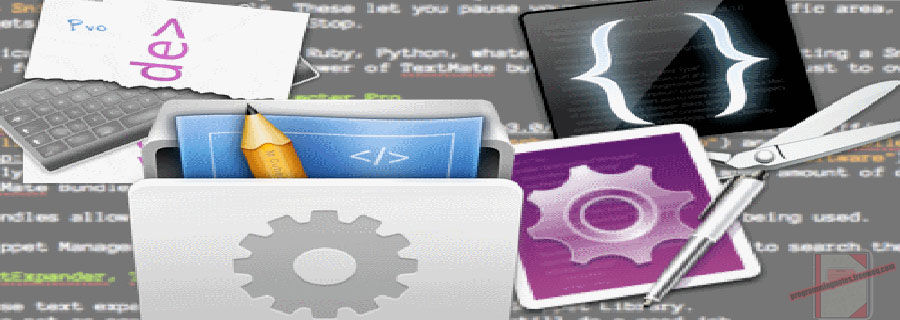
This snippet demonstrates how to place numbers into an integer array. It also shows how to display the contents of the array back to the user via cout.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
#include <iostream> using namespace std; // const int allocating space for the array const int ARRAY_SIZE = 100; int main() { // declare variables int numElems = 0; int myArray[ARRAY_SIZE]; // declare array which has the ability to hold 100 elements // ask user how many items they want to place in array cout << "How many items do you want to place into the array?: "; cin >> numElems; // user enters data into array using a for loop // you can also use a while loop, but for loops are more common // when dealing with arrays for(int index=0; index < numElems; ++index) { cout << "nEnter item #" << index +1<< " : "; cin >> myArray[index]; } // display data to user cout << "nThe current items inside the array are: "; // display contents inside array using a for loop for(int index=0; index < numElems; ++index) { cout << "nItem #" << index +1<< " : "; cout << myArray[index]; } cout<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
How many items do you want to place into the array?: 5
Enter item #1 : 45Enter item #2 : 7
Enter item #3 : 34
Enter item #4 : 8
Enter item #5 : 2
The current items inside the array are:
Item #1 : 45
Item #2 : 7
Item #3 : 34
Item #4 : 8
Item #5 : 2
C++ || Snippet – Sort An Integer Array Using Bubble Sort – Print Each Pass & Total Number Of Swaps
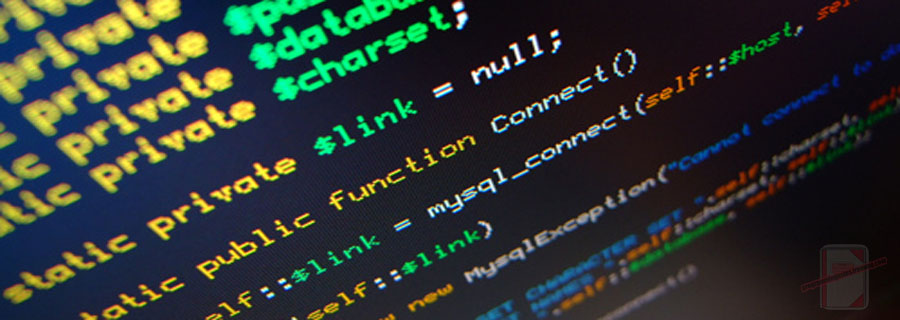
This is a program which has no functionality, but displays the sorting of an integer array through the use of the bubble sort algorithm.
This program sorts the values of a one-dimensional array in ascending order using bubble sort. It also prints the total number of passes thru each iteration
REQUIRED KNOWLEDGE FOR THIS SNIPPET
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 7, 2012 // Taken From: http://programmingnotes.org/ // File: bubbleSort.cpp // Description: This program sorts the values of a one-dimensional array // in ascending order using the Bubble Sort Algorithm. It also prints // the total number of passes thru each iteration // ============================================================================ #include <iostream> #include <iomanip> using namespace std; // const int const int NUM_INTS = 12; // function prototype void BubbleSort(int arry[], int &totalSwaps, int &totalPasses); int main() { // Declarations int arry[NUM_INTS] = {0, 1, 3, 95, 2, 4, 6, 10, 15, 4, 17, 35}; int totalSwaps = 0; int totalPasses = 0; cout << "Original array values" << endl; // Output the original array values for (int i = 0; i < NUM_INTS; ++i) { cout << setw(4) << arry[i]; } // creates a line separator cout << "\n---------------------------------------------------------------\n\n"; BubbleSort(arry,totalSwaps,totalPasses); // creates a line separator cout << "---------------------------------------------------------------\n"; cout << "The current sorted array" << endl; // Output the current sorted for (int i = 0; i < NUM_INTS; ++i) { cout << setw(4) << arry[i]; } // creates a line separator cout << "\n---------------------------------------------------------------\n"; // Display some statistics to the user cout << "Total Swaps: " << totalSwaps << endl; cout << "Total Passes: " << totalPasses << "\n\n"; return 0; }// end of main void BubbleSort(int arry[], int &totalSwaps, int &totalPasses) { int currentSwaps = 0; // Loop to determine amount of passes for (int iteration = 1; iteration < NUM_INTS; ++iteration) { // Reset variable swaps to zero currentSwaps = 0; // Bubble Sort Algorithm // Sort numbers in ascending order for (int p = 0; p < NUM_INTS - iteration; ++p) { // if the previous value is bigger than the next // then swap places if (arry[p]> arry[p+1]) { int temp = arry[p]; arry[p] = arry[p+1]; arry[p+1]= temp; ++currentSwaps; } } // If no swaps were made in the last pass, // no need to continue the loop. Sorting complete. if (currentSwaps == 0) { break; } cout << "Array after bubble sort pass #" << iteration << endl; // Display values after each pass for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } cout << "\n\n"; // Keeps track of the amount of swaps and passes totalSwaps += currentSwaps; ++totalPasses; }// end of for loop }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Original array values
0 1 3 95 2 4 6 10 15 4 17 35
---------------------------------------------------------------
Array after bubble sort pass #1
0 1 3 2 4 6 10 15 4 17 35 95Array after bubble sort pass #2
0 1 2 3 4 6 10 4 15 17 35 95Array after bubble sort pass #3
0 1 2 3 4 6 4 10 15 17 35 95Array after bubble sort pass #4
0 1 2 3 4 4 6 10 15 17 35 95
---------------------------------------------------------------
The current sorted array
0 1 2 3 4 4 6 10 15 17 35 95
---------------------------------------------------------------
Total Swaps: 12
Total Passes: 4
C++ || Input/Output – Using An Array, Sort Names From a Text File & Save The Sorted Names To A New Text File
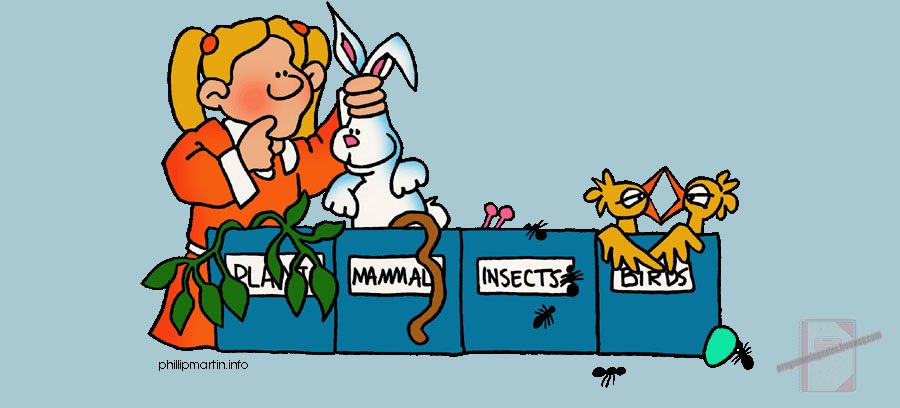
Since we previously discussed how to sort numbers which is contained in an integer array, it is only fitting that we display a program which sorts characters that are stored in a character array.
This is an interactive program which first displays a menu to the user, allowing them to choose from 6 different modes of operation. The 6 options are described as followed:
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit
From the available choices, the user has the option of reading in names from a file, manually entering in names themselves, displaying the current names in the array, sorting the current names in the array, clearing the current names in the array, and finally quitting the program. When the user chooses to quit the program, whatever data which is currently stored within the array will automatically be saved to the output text file.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Fstream
Ifstream
Ofstream
Character Arrays
2D Arrays
Working With Files
Pass By Reference
While Loops
For Loops
Bubble Sort
Functions
Switch Statements
Boolean Expressions
Toupper
Strcpy
Strcmp
The data file that is used in this example can be downloaded here.
Note: In order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
NOTE: On some compilers, you may have to add #include < cstdlib> and #include < cstring> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 |
#include <iostream> #include <fstream> #include <iomanip> using namespace std; // const variable indicating how many names the array can hold const int TOTALNAMES = 100; // function prototypes void DisplayMenu(); int ReadInData(char names[][50], ifstream &infile); void GetUserData(char names[][50], int numberOfNames); void ClearArray(char names[][50], int numberOfNames); void SortArray(char names[][50], int numNames); void DisplayArray(char names[][50], int numNames); void SaveArrayToFile(char names[][50], int numberOfNames, ofstream &outfile); int main() { // declare variables ifstream infile; ofstream outfile; char names[TOTALNAMES][50]; char userResponse = 'Q'; int numberOfNames = 0; // display menu to user DisplayMenu(); cin >> userResponse; // keep looping thru the menu until the user // selects Q (Quit) while(toupper(userResponse)!='Q') { // switch statement indicating the available choices // the user has to make switch(toupper(userResponse)) { case 'R': numberOfNames = ReadInData(names, infile); break; case 'E': cout << "nPlease enter the number of names you want to sort: "; cin >> numberOfNames; GetUserData(names,numberOfNames); break; case 'D': DisplayArray(names, numberOfNames); break; case 'C': ClearArray(names,numberOfNames); numberOfNames=0; break; case 'S': SortArray(names, numberOfNames); break; case 'Q': break; default: cout << "nThe selected option is not apart of the list!nPlease try again.."; break; } // creates a line seperator after each task is executed cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // re-display's the menu to the user DisplayMenu(); cin >> userResponse; } // after the user is finished manipulating the // data, save the names to the output file SaveArrayToFile(names, numberOfNames, outfile); return 0; }// end of main // ============================================================================ // displays options to user void DisplayMenu() { cout<<" Welcome to the name sorting program..."; cout<<"nFrom the following menu, select an option"; cout<<"nR - Read in names from a file for sorting"; cout<<"nE - Enter in names manually for sorting"; cout<<"nD - Display the current names in the array"; cout<<"nS - Sort the current names in the array"; cout<<"nC - Clear the current names in the array"; cout<<"nQ - Quitn"; cout<<"n>> "; }// end of DisplayMenu // ============================================================================ // reads in data from a file int ReadInData(char names[][50], ifstream &infile) { int numberOfNames=0; // open input file infile.open("INPUT_UNSORTED_NAMES_programmingnotes_freeweq_com.txt"); if(infile.fail()) { cout<<"Input file could not be found!n" <<"Please place the input file in the correct directory.."; return 0; } else { cout << "nReading in data from the file..."; while(infile.good()) { infile >> names[numberOfNames]; ++numberOfNames; } cout << "nSuccess!"; } infile.close(); // close the infile once we are done using it return numberOfNames; }// end of ReadInData // ============================================================================ // gets data from user (names) for direct input void GetUserData(char names[][50], int numberOfNames) { cout << "nPlease enter "<<numberOfNames<<" names" << endl; for(int index=0; index < numberOfNames; ++index) { cout<<"nName #"<<index+1<<": "; cin >> names[index]; } }// end of GetUserData // ============================================================================ // clears the data contained in the array void ClearArray(char names[][50], int numNames) { if(numNames==0) { cout<<"nThe array is currently empty!n"; } else { cout<<"nDeleting the data contained in the array..."; for(int index=0; index < numNames; ++index) { strcpy(names[index],""); } cout << "nClearing Complete!"; } }// end of ClearArray // ============================================================================ // sorts the array via 'bubble sort' void SortArray(char names[][50],int numNames) { bool sorted = false; char temp[50]; if(numNames==0) { cout<<"nThe array is currently empty!n"; } else { cout << "nSorting the names contained in the array..."; // this is the 'bubble sort' and will execute only // if there is more than 1 name contained within the array // If there is only one name contained in the array, // there is no need to sort anything while((sorted == false) && (numNames > 1)) { sorted = true; for (int index=0; index < numNames-1; ++index) { if (strcmp(names[index], names[index+1]) > 0) { strcpy(temp,names[index]); strcpy(names[index], names[index+1]); strcpy(names[index+1], temp); sorted = false; } } } cout << "nSuccess!"; } }// end of SortArray // ============================================================================ // saves the current data which is in the arrya to the output file void SaveArrayToFile(char names[][50], int numberOfNames, ofstream &outfile) { // open output file outfile.open("OUTPUT_SORTED_NAMES_programmingnotes_freeweq_com.txt"); if(outfile.fail()) { cout<<"Error creating output file!"; return; } else { if(numberOfNames==0) { cout<<"nThe array contained no names.nThere was no data to save to the output file...n"; outfile<<"The array contained no names.nThere was no data to save to the output file...n"; } else { cout<<"nSaving the current contents of the array to the ouptut file.."; outfile<<"Sorted items which were contained within the array..n"; for(int index=0; index < numberOfNames; ++index) { outfile <<"Name #"<<index+1<<": " << names[index]<<endl; } cout << "nSuccess!n"; } } outfile.close(); }// end of SaveArrayToFile // ============================================================================ // displays the current contents of the array to the user // via cout void DisplayArray(char names[][50], int numNames) { if(numNames==0) { cout<<"nThe array is currently empty!n"; } else { cout << "nThe values in the array are:n"; for (int index=0; index < numNames; ++index) { cout << names[index] << endl; } cout<<"nThere is currently "<<numNames<<" names in the array!n"; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Remember to include the input file)
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> d
The array is currently empty!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> q
The array contained no names.
There was no data to save to the output file...------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> e
Please enter the number of names you want to sort: 3
Please enter 3 names
Name #1: My
Name #2: Programming
Name #3: Notes------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> d
The values in the array are:
My
Programming
NotesThere is currently 3 names in the array!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> s
Sorting the names contained in the array...
Success!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> d
The values in the array are:
My
Notes
ProgrammingThere is currently 3 names in the array!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> c
Deleting the data contained in the array...
Clearing Complete!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> r
Reading in data from the file...
Success!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> d
The values in the array are:
My
Programming
Notes
C++
Java
Assembly
Lemon
Dark
Light
Black
High
Low
Cellphone
Cat
Dog
Penguin
Japan
Peace
Love
Color
White
One
Brain
Eggplant
Phalanx
Countenance
Crayons
Ben
Dover
Eileen
Bob
Downe
Justin
Elizebeth
Rick
Rolled
Sam
Widge
Liza
Destruction
Grove
Aardvark
Primal
Sushi
Victoria
Ostrich
Zebra
Scrumptious
Carbohydrate
Sulk
Ecstatic
Acrobat
Pneumonoultramicroscopicsilicovolcanoconiosis
English
Kenneth
Jessica
Pills
Pencil
Dragon
Mint
Chocolate
Temperature
Cheese
Rondo
Silicon
Scabbiest
Palpitate
Invariable
Henpecked
Titmouse
Canoodle
Boobies
Pressure
Density
Cards
Twiat
Tony
Pink
Green
Yellow
Duck
Dodge
Movie
Zoo
Xiomara
Eggs
Marshmallows
Umbrella
Apple
Panda
Brush
Handle
Door
Knob
Mask
Knife
Speaker
Wood
Orient
LoveThere is currently 100 names in the array!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> s
Sorting the names contained in the array...
Success!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> d
The values in the array are:
Aardvark
Acrobat
Apple
Assembly
Ben
Black
Bob
Boobies
Brain
Brush
C++
Canoodle
Carbohydrate
Cards
Cat
Cellphone
Cheese
Chocolate
Color
Countenance
Crayons
Dark
Density
Destruction
Dodge
Dog
Door
Dover
Downe
Dragon
Duck
Ecstatic
Eggplant
Eggs
Eileen
Elizebeth
English
Green
Grove
Handle
Henpecked
High
Invariable
Japan
Java
Jessica
Justin
Kenneth
Knife
Knob
Lemon
Light
Liza
Love
Love
Low
Marshmallows
Mask
Mint
Movie
My
Notes
One
Orient
Ostrich
Palpitate
Panda
Peace
Pencil
Penguin
Phalanx
Pills
Pink
Pneumonoultramicroscopicsilicovolcanoconiosis
Pressure
Primal
Programming
Rick
Rolled
Rondo
Sam
Scabbiest
Scrumptious
Silicon
Speaker
Sulk
Sushi
Temperature
Titmouse
Tony
Twiat
Umbrella
Victoria
White
Widge
Wood
Xiomara
Yellow
Zebra
ZooThere is currently 100 names in the array!
------------------------------------------------------------
Welcome to the name sorting program...
From the following menu, select an option
R - Read in names from a file for sorting
E - Enter in names manually for sorting
D - Display the current names in the array
S - Sort the current names in the array
C - Clear the current names in the array
Q - Quit>> q
Saving the current contents of the array to the ouptut file..
Success!
C++ || Searching An Integer Array For A Target Value
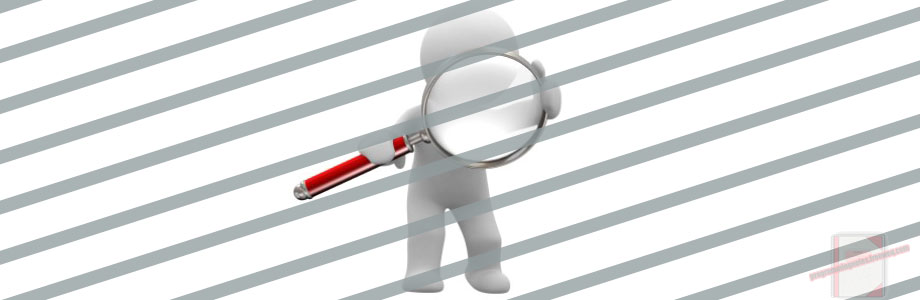
Here is another actual homework assignment which was presented in an intro to programming class.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
This is a small and simple program which demonstrates how to search for a target value that is stored in an integer array. This program prompts the user to enter five values into an int array. After the user has entered all the values, it displays a prompt asking the user for a search value. Once it has the search value, the program will search through the array looking for the target; wherever the value is found, it will display the index location. After it displays all the locations where the value is found, it will display the total number of occurrences the search value was found within the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // File: ArraySearch.cpp // ============================================================================ // // Description: // This program allows the user to populate an array of integers with five // values. After the user has entered all the values, it displays a prompt // asking the user for a search value. Once it has the search value, the // program will search through the array looking for the target; wherever the // value is found, it will display the index location. After it displays all // the locations where the value is found, it will display the total number // of occurrences the search value was found within the array. // // ============================================================================ #include <iostream> using namespace std; // constant variable const int NUM_INTS = 5; // function prototypes int SearchArray(int numValues[], int searchValue); // ==== main ================================================================== // // ============================================================================ int main() { // declare variables int searchValue; int numOccurences = 0; int numValues[NUM_INTS]; // int array size is initialized with a const value // get data from user via a 'for loop' cout << "Please enter "<< NUM_INTS <<" integer values:nn"; for (int index=0; index < NUM_INTS; ++index) { cout << "#" << index + 1 << ": "; cin >> numValues[index]; cout <<endl; } // get a search value from the user cout << "Please enter a search value: "; cin >> searchValue; cout <<endl; // finds the number of occurences the search value was found in the array numOccurences = SearchArray(numValues, searchValue); // display data to user cout << "nThe total occurrences of value " << searchValue << " within the array is: " << numOccurences; cout << endl << endl; return 0; }// end of main // ==== SearchArray =========================================================== // // This function will take as input the array, the number of array // elements, and the target value to search for. The function will traverse // the array looking for the target value, and when it finds it, display the // index location within the array. // // Input: // limit [IN] -- the array, the number of array // elements, and the target value // // Output: // The total number of occurrences of the target in the array // // ============================================================================ int SearchArray(int numValues[], int searchValue) { int numFound=0; for (int index=0; index < NUM_INTS; ++index) { if (numValues[index] == searchValue) { cout << "t" << searchValue << " was found at array index #" << index << endl; ++numFound; // if the search values was found, // increment the variable by 1 } } return numFound; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 3 separate times to display the different outputs its able to produce
Please enter 5 integer values:
#1: 12
#2: 12
#3: 12
#4: 12
#5: 12
Please enter a search value: 1212 was found at array index #0
12 was found at array index #1
12 was found at array index #2
12 was found at array index #3
12 was found at array index #4The total occurrences of value 12 within the array is: 5
-------------------------------------------------------------------------Please enter 5 integer values:
#1: 12
#2: 87
#3: 45
#4: 87
#5: 33
Please enter a search value: 8787 was found at array index #1
87 was found at array index #3The total occurrences of value 87 within the array is: 2
-------------------------------------------------------------------------Please enter 5 interger values:
#1: 54
#2: 67
#3: 98
#4: 45
#5: 98
Please enter a search value: 123The total occurrences of value 123 within the array is: 0
C++ || Find The Prime, Perfect & All Known Divisors Of A Number Using A For, While & Do/While Loop
This program was designed to better understand how to use different loops which are available in C++.
This program first asks the user to enter a non negative number. After it obtains a non negative integer from the user, the program will determine if the inputted number is a prime number or not, aswell as determine if the user inputted number is a perfect number or not. After it obtains its results, the program will display to the screen if the user inputted number is prime/perfect number or not. The program will also display a list of all the possible divisors of the user inputted number via cout.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Do/While Loop
While Loop
For Loop
Modulus
Basic Math - Prime Numbers
Basic Math - Perfect Numbers
Basic Math - Divisors
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
#include <iostream> #include <iomanip> using namespace std; int main () { int userInput = 0; int divisor = 0; int sumOfDivisors = 0; char response ='n'; do{ // this is the start of the do/while loop cout<<"Enter a number: "; cin >> userInput; // if the user inputs a negative number, do this code while(userInput < 0) { cout<<"ntaSorry, but the number entered is less than the allowable limit. Please try again"; cout<<"nnEnter an number: "; cin >> userInput; } cout << "nInput number: " << userInput; // for loop adds sum of all possible divisors for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0) { // this will repeatedly add the found divisors together sumOfDivisors += counter; } } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is prime if(userInput == (sumOfDivisors - 1)) { cout<<endl; cout << userInput << " is a prime number."; } else { cout<<endl; cout << userInput << " is not a prime number."; } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is a perfect number if (userInput == (sumOfDivisors - userInput)) { cout<<endl; cout << userInput << " is a perfect number."; } else { cout<<endl; cout << userInput << " is not a perfect number."; } cout << "nDivisors of " << userInput << " are: "; // for loop lists all the possible divisors for the // 'userInput' variable by using the modulus operator for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0 && counter !=userInput) { cout << counter << ", "; } } cout << "and "<< userInput; // asks user if they want to enter new data cout << "nntDo you want to input another number?(Y/N): "; cin >> response; // creates a line seperator if user wants to enter new data cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // resets variable back to zero if the user wants to enter new data sumOfDivisors=0; }while(response =='y' || response =='Y'); // ^ End of the do/while loop. As long as the user chooses // 'Y' the loop will keep going. // It stops when the user chooses the letter 'N' return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Enter a number: 8128
Input number: 8128
8128 is not a prime number.
8128 is a perfect number.
Divisors of 8128 are: 1, 2, 4, 8, 16, 32, 64, 127, 254, 508, 1016, 2032, 4064, and 8128Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 6Input number: 6
6 is not a prime number.
6 is a perfect number.
Divisors of 6 are: 1, 2, 3, and 6Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 241Input number: 241
241 is a prime number.
241 is not a perfect number.
Divisors of 241 are: 1, and 241Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 2012Input number: 2012
2012 is not a prime number.
2012 is not a perfect number.
Divisors of 2012 are: 1, 2, 4, 503, 1006, and 2012Do you want to input another number?(Y/N): n
------------------------------------------------------------
Press any key to continue . . .
C++ || Find The Average Using an Array – Omit Highest And Lowest Scores
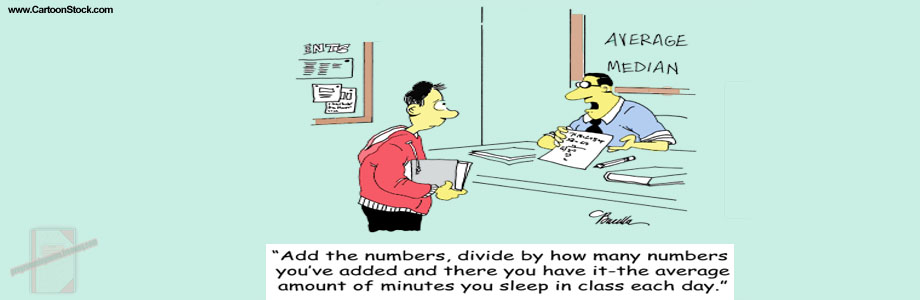
This page will consist of two programs which calculates the average of a specific amount of numbers using an array.
REQUIRED KNOWLEDGE FOR BOTH PROGRAMS
Float Data Type
Constant Values
Arrays
For Loops
Assignment Operators
Basic Math
====== FIND THE AVERAGE USING AN ARRAY ======
The first program is fairly simple, and it was used to introduce the array concept. The program prompts the user to enter the total amount of numbers they want to find the average for, then the program displays the answer to them via cout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
#include <iostream> using namespace std; int main() { // declare variables float total = 0; int numElems = 0; float average[100]; // declare array which has the ability to hold 100 elements cout << "How many numbers do you want to find the average for?: "; cin >> numElems; // user enters data into array using a for loop // you can also use a while loop, but for loops are more common // when dealing with arrays for(int index=0; index < numElems; ++index) { cout << "nEnter #" << index +1<< " : "; cin >> average[index]; total += average[index]; } // find the average. Note: // the expression below literally // means: total = total / numElems; total /= numElems; cout << "nThe average of the " << numElems << " numbers is " << total <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
ARRAY
Notice the array declaration on line #9. The type of array being used in this program is a static array, which has the ability to store up to 100 integer elements in the array. You can change the number of elements its able to store to a higher or lower number if you wish.
FOR LOOP
Notice line 17-22 contains a for loop, which is used to actually store the data inside of the array. Without some type of loop, it is virtually impossible for the user to input data into the array; that is, unless you want to add 100 different cout statements into your code asking the user to input data. Line 21 uses the assignment operator “+=” which gives us a running total of the data that is being inputted into the array. Note the loop only stores as many elements as the user so desires, so if the user only wants to input 3 numbers into the array, the for loop will only execute 3 times.
Once compiled, you should get this as your output:
How many numbers do you want to find the average for?: 5
Enter #1 : 23
Enter #2 : 17
Enter #3 : 29
Enter #4 : 14
Enter #5 : 16
The average of the 5 numbers is 19.8
====== FIND THE AVERAGE – OMIT HIGHEST AND LOWEST SCORES ======
The second program is really practical in a real world setting, specifically when a teacher records test scores into the computer. We were asked to create a program for a fictional competition which had 6 judges. The 6 judges each gave a score of the performance for a competitor in a competition, (i.e a score of 1-10), and we were asked to find the average of those scores, omitting the highest/lowest results. The program was to store the scores into an array, display the scores back to the user via cout, display the highest and lowest scores among the 6 obtained, display the average of the 6 scores, and finally display the average adjusted scores omitting the highest and lowest result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 |
#include <iostream> using namespace std; const int NUM_JUDGES = 6; int main() { int scores[NUM_JUDGES]; // array is initialized using a const variable int highestScore = -999999; int lowestScore = 999999; float sumOfScores = 0; float avgScores = 0; // use a for loop to obtain data from user using the const variable cout<< "Judges, enter one score each for the current competitor: "; for(int counter=0; counter < NUM_JUDGES; ++counter) { cin >> scores[counter]; } // use another for loop to redisplay the data back to the user via cout cout<<"nThese are the scores from the " << NUM_JUDGES << " judges: "; for(int index=0; index < NUM_JUDGES; ++index) { cout<<"nThe score for judge #"<<(index+1)<<" is: "<<scores[index]; } // use a for loop to go thru the array checking to see the highes/lowest element cout<<"nnThese are the highest and lowest scores: "; for(int index=0; index < NUM_JUDGES; ++index) { // if current score in the array is bigger than the current 'highestScore' // element, then set 'highestScore' equal to the current array element if(scores[index] > highestScore) { highestScore = scores[index]; } // if current 'lowestScore' element is bigger than the current array element, // then set 'lowestScore' equal to the current array element if(lowestScore > scores[index]) { lowestScore = scores[index]; } } cout << "ntHighest: "<< highestScore; cout << "ntLowest: "<< lowestScore; cout << "nThe average score is: "; // this calculates a running total, adding each element in the array together for(int counter=0; counter < NUM_JUDGES; ++counter) { sumOfScores += scores[counter]; } avgScores = sumOfScores/NUM_JUDGES; cout<< avgScores; // reset data back to 0 so we dont get wrong answers sumOfScores = 0; avgScores = 0; cout<<"nThe average adjusted score omitting the highest and lowest result is: "; // final loop, which calculates a running total, adding each element // in the array together, this time omitting the highest/lowest elements for(int counter=0; counter < NUM_JUDGES; ++counter) { // IF(current score isnt equal to the highest elem) AND (current score isnt equal lowest elem) // THEN create a running total if ((scores[counter] != highestScore) && (scores[counter] != lowestScore)) { sumOfScores += scores[counter]; } } avgScores = sumOfScores/(NUM_JUDGES-2); cout<< avgScores <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
CONST
A constant variable was declared and used to initialize the array (line 8). Note, when using static arrays, the program has to know how many elements to initialize the program with before the program starts, so creating a constant variable to do that for us is convenient.
FOR LOOPS
Once again loops were used to traverse the array, as noted on lines 16, 23, 30, 53, and 70. The const variable was also used within the for loops, making it easier to modify the code if its necessary to reduce or increase the number of available judges.
HIGHEST/LOWEST SCORES
This is noted on lines 34-44, and it is really simple to understand the process once you see the code.
OMITTING HIGHEST/LOWEST SCORE
Lines 70-78 highlight this process, and the loop basically traverses the array, skipping over the highest/lowest elements
Once compiled, you should get this as your output:
Judges, enter one score each for
the current competitor: 123 453 -789 2 23345 987These are the scores from the 6 judges:
The score for judge #1 is: 123
The score for judge #2 is: 453
The score for judge #3 is: -789
The score for judge #4 is: 2
The score for judge #5 is: 23345
The score for judge #6 is: 987These are the highest and lowest scores:
Highest: 23345
Lowest: -789
The average score is: 4020.17
The average adjusted score omitting the highest and lowest result is: 391.25