JavaScript || How To Get All Unique Values In An Array & Remove Duplicates Using Vanilla JavaScript
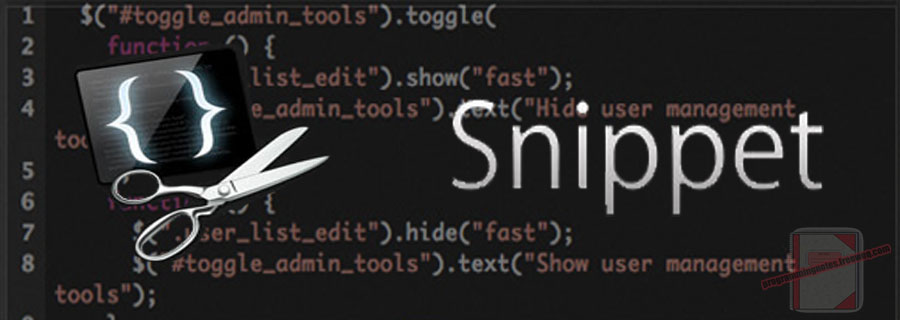
The following is a module with functions which demonstrates how to get all distinct values in an array & remove duplicates from a simple and object array.
The functions on this page allows for an optional selector function as a parameter, which makes them general enough to work on arrays of any type.
1. Simple Array
The example below demonstrates the use of ‘Utils.arrayUnique‘ to get unique values from a simple array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
// Simple Array <script> (() => { // Simple array let fruits = [ 'apple', 'orange', 'apple', 'watermelon', 'apple', 'peach', 'orange', 'watermelon', 'grape' ]; // Get unique items from an array let result = Utils.arrayUnique(fruits); result.forEach(item => { console.log(item); }); })(); </script> // expected output: /* apple orange watermelon peach grape */ |
2. Object Array
The example below demonstrates the use of ‘Utils.arrayUnique‘ to get unique values from an object array.
In this example, a selector is used, which extracts the value to be used in the evaluation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// Object Array <script> (() => { // Object array let products = [{ 'id': 1, 'title': 'iPad 4 Mini', 'price': 500.01, 'inventory': 2, 'categories': ['Electronics'], }, { 'id': 2, 'title': 'H&M T-Shirt White', 'price': 10.99, 'inventory': 10, 'categories': ['Clothing'], }, { 'id': 3, 'title': 'Charli XCX - Sucker CD', 'price': 19.99, 'inventory': 5, 'categories': ['Music', 'Electronics'] } ]; // Get unique items from an object array let result = Utils.arrayUnique(products, (product) => product.categories); result.forEach(item => { console.log(item); }); })(); </script> // expected output: /* Electronics Clothing Music */ |
3. Mixed Array
The example below demonstrates the use of ‘Utils.arrayUnique‘ to get unique values from a mixed array.
In this example, a selector is used, which extracts the value to be used in the evaluation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// Mixed Array <script> (() => { // Mixed array let mixedArray = [ [0, 'Aluminium', 0, 'Francis'], [1, 'Argon', 1, 'Ada'], [2, 'Brom', 2, 'John'], [3, 'Cadmium', 9, 'Marie'], [4, 'Fluor', 12, 'Marie'], [5, 'Gold', 1, 'Ada'], [6, 'Kupfer', 4, 'Ines'], [7, 'Krypton', 4, 'Joe'], [8, 'Sauerstoff', 0, 'Marie'], [9, 'Zink', 5, 'Max'] ]; // Get unique items from an array at index 2 let result = Utils.arrayUnique(mixedArray, (item) => item[2]); result.forEach(item => { console.log(item); }); })(); </script> // expected output: /* 0 1 2 9 12 4 5 */ |
4. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 19, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: arrayUnique * USE: Returns distinct elements from a sequence. * @param array: The array to remove duplicate elements from. * @param selector: Optional. A transform function to extract values from each element. * @return: An array that contains distinct elements from the source sequence. */ exposed.arrayUnique = (array, selector = null) => { if (typeof selector !== 'function') { selector = (item) => item; } let value = array .reduce((acc, item) => acc.concat(selector.call(array, item)), []) .filter((value, index, self) => self.indexOf(value) === index); return value; } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply