C++ || Snippet – How To Send Text Over A Network Using A UDP Connection
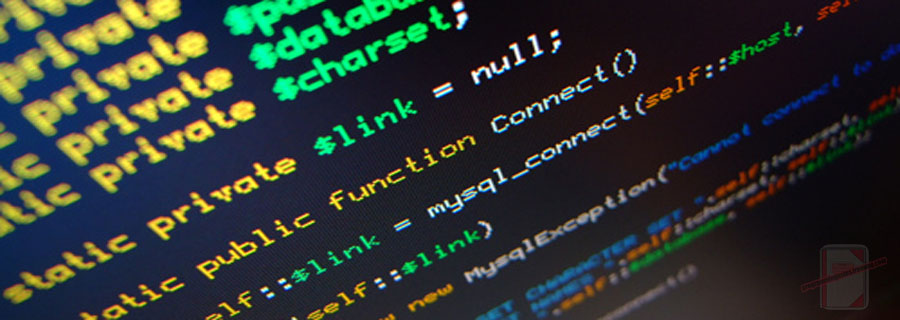
The following is sample code which demonstrates the use of the “socket“, “bind“, “recvfrom“, and “sendto” function calls for interprocess communication over a network on Unix based systems.
The following example demonstrates a simple network application in which a client sends text to a server, and the server replies (sends text) back to the client. This program demonstrates communication between two programs using a UDP connection.
Click here to examine the TCP version.
Note: The server program must be ran before the client program!
=== 1. SERVER ===
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 |
// ============================================================================ // Author: K Perkins // Date: Jan 8, 2014 // Taken From: http://programmingnotes.org/ // File: ServerUDP.cpp // Description: This program implements a simple network application in // which a client sends text to a server and the server replies back to the // client. This is the server program, and has the following functionality: // 1. Listen for incoming connections on a specified port. // 2. When a client connects, text is received from the client. // 3. Text is sent back to the client from the server. // 4. Go back to waiting for more connections. // ============================================================================ #include <iostream> #include <cstdio> #include <cstdlib> #include <cstring> #include <ctime> #include <sys/socket.h> #include <netinet/in.h> using namespace std; // Compile & Run // g++ ServerUDP.cpp -o ServerUDP // ./ServerUDP 1234 // the maximum size for sending/receiving text const int MSG_SIZE = 100; int main(int argc, char* argv[]) { // declare variables // to store the port number int port = -1; // to store the socket file descriptor int socketfd = -1; // the address structures for the server and the client sockaddr_in servaddr, cliaddr; // the size of sockaddr_in structure socklen_t sockaddr_in_len = 0; // the number of bytes received and sent int numRead = -1, numSent = -1; // the buffer to store the string to send/receive char data[MSG_SIZE]; // check the command line if(argc < 2) { cerr<<"n** ERROR NOT ENOUGH ARGS!n" <<"USAGE: "<<argv[0]<<" <SERVER PORT #>n"; exit(1); } // convert the port number to an integer port = atoi(argv[1]); // make sure the port range is valid if(port < 0 || port > 65535) { cerr<<"nInvalid port numbern"; exit(1); } // create a UDP socket if((socketfd = socket(AF_INET, SOCK_DGRAM, 0)) < 0) { perror("socket"); exit(1); } // clear the structure for the server address memset(&servaddr, 0, sizeof(servaddr)); // populate the server address structure servaddr.sin_family = AF_INET; servaddr.sin_addr.s_addr = htonl(INADDR_ANY); servaddr.sin_port = htons(port); // bind the socket to the port if(bind(socketfd,(sockaddr *)&servaddr, sizeof(servaddr)) < 0) { perror("bind"); exit(1); } cerr<<"nServer started!n"; // keep receiving forever while(true) { cerr<<"nWaiting for someone to connect..n"; // get the size of the client address data structure sockaddr_in_len = sizeof(cliaddr); // get something from the client; Will block until the client sends. if((numRead = recvfrom(socketfd, data, sizeof(data), 0, (sockaddr *)&cliaddr, &sockaddr_in_len)) < 0) { perror("recvfrom"); exit(1); } cerr<<"nRECEIVED: ""<<data<<"" from the clientn"; //cerr<<"Bytes read: "<<numRead<<endl; // set the array to all zeros memset(&data, 0, sizeof(data)); // retrieve the time time_t rawtime; time(&rawtime); struct tm* timeinfo; timeinfo = localtime(&rawtime); strftime(data, sizeof(data),"%a, %b %d %Y, %I:%M:%S %p", timeinfo); cerr<<"nSENDING: ""<<data<<"" to the clientn"; // send the time to the client if((numSent = sendto(socketfd, data, strlen(data)+1, 0, (sockaddr *)&cliaddr,sizeof(cliaddr))) < 0) { perror("sendto"); exit(1); } } return 0; }// http://programmingnotes.org/ |
=== 2. CLIENT ===
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 |
// ============================================================================ // Author: K Perkins // Date: Jan 8, 2014 // Taken From: http://programmingnotes.org/ // File: ClientUDP.cpp // Description: This program implements a simple network application in // which a client sends text to a server and the server replies back to the // client. This is the client program, and has the following functionality: // 1. Send text to the server on a specified port. // 2. Recieve text from the server and exit. // ============================================================================ #include <iostream> #include <cstdio> #include <cstdlib> #include <cstring> #include <ctime> #include <unistd.h> #include <sys/socket.h> #include <netinet/in.h> #include <arpa/inet.h> using namespace std; // Compile & Run // g++ ClientUDP.cpp -o ClientUDP // ./ClientUDP 127.0.0.1 1234 // the maximum size for sending/receiving text const int MSG_SIZE = 100; int main(int argc, char* argv[]) { // declare variables // to store the port number int port = -1; // to store the socket file descriptor int socketfd = -1; // the address structures for the server and the client sockaddr_in servaddr, cliaddr; // the size of sockaddr_in structure socklen_t sockaddr_in_len = 0; // the number of bytes received and sent int numRead = -1, numSent = -1; // the buffer to store the string to send/receive char data[MSG_SIZE] = "Server, what time is it?"; // check the command line if(argc < 3) { cerr<<"n** ERROR NOT ENOUGH ARGS!n" <<"USAGE: "<<argv[0]<<" <SERVER IP> <SERVER PORT #>n"; exit(1); } // convert the port number to an integer port = atoi(argv[2]); // make sure the port range is valid if(port < 0 || port > 65535) { cerr<<"nInvalid port numbern"; exit(1); } // create a UDP socket if((socketfd = socket(AF_INET, SOCK_DGRAM, 0)) < 0) { perror("socket"); exit(1); } // clear the structure for the server address memset(&servaddr, 0, sizeof(servaddr)); // populate the server address structure servaddr.sin_family = AF_INET; // initialize the IP address of the server if(!inet_pton(AF_INET, argv[1], &servaddr.sin_addr.s_addr)) { perror("inet_pton"); exit(1); } // set the port servaddr.sin_port = htons(port); // get the size of the client address data structure sockaddr_in_len = sizeof(cliaddr); cerr<<"nSENDING: ""<<data<<"" to the servern"; // send the time to the client if((numSent = sendto(socketfd, data, strlen(data)+1, 0, (sockaddr *)&servaddr, sizeof(servaddr))) < 0) { perror("sendto"); exit(1); } // get something from the client; Will block until the client sends. if((numRead = recvfrom(socketfd, data, sizeof(data), 0, (sockaddr *)&cliaddr, &sockaddr_in_len)) < 0) { perror("recvfrom"); exit(1); } // NULL terminate the received string data[numRead] = ' '; cerr<<"nRECEIVED: ""<<data<<"" from the servern"; //cerr<<"Bytes read: "<<numRead<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
The following is sample output.
SERVER OUTPUT:
Server started!
Waiting for someone to connect..
RECEIVED: "Server, what time is it?" from the client
SENDING: "Wed, Jan 08 2014, 11:47:12 PM" to the client
CLIENT OUTPUT:
SENDING: "Server, what time is it?" to the server
RECEIVED: "Wed, Jan 08 2014, 11:47:12 PM" from the server
Leave a Reply