C++ || Find The Average Using an Array – Omit Highest And Lowest Scores
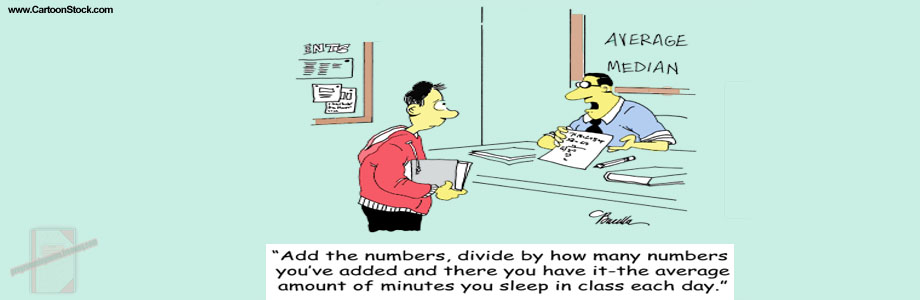
This page will consist of two programs which calculates the average of a specific amount of numbers using an array.
REQUIRED KNOWLEDGE FOR BOTH PROGRAMS
Float Data Type
Constant Values
Arrays
For Loops
Assignment Operators
Basic Math
====== FIND THE AVERAGE USING AN ARRAY ======
The first program is fairly simple, and it was used to introduce the array concept. The program prompts the user to enter the total amount of numbers they want to find the average for, then the program displays the answer to them via cout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
#include <iostream> using namespace std; int main() { // declare variables float total = 0; int numElems = 0; float average[100]; // declare array which has the ability to hold 100 elements cout << "How many numbers do you want to find the average for?: "; cin >> numElems; // user enters data into array using a for loop // you can also use a while loop, but for loops are more common // when dealing with arrays for(int index=0; index < numElems; ++index) { cout << "nEnter #" << index +1<< " : "; cin >> average[index]; total += average[index]; } // find the average. Note: // the expression below literally // means: total = total / numElems; total /= numElems; cout << "nThe average of the " << numElems << " numbers is " << total <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
ARRAY
Notice the array declaration on line #9. The type of array being used in this program is a static array, which has the ability to store up to 100 integer elements in the array. You can change the number of elements its able to store to a higher or lower number if you wish.
FOR LOOP
Notice line 17-22 contains a for loop, which is used to actually store the data inside of the array. Without some type of loop, it is virtually impossible for the user to input data into the array; that is, unless you want to add 100 different cout statements into your code asking the user to input data. Line 21 uses the assignment operator “+=” which gives us a running total of the data that is being inputted into the array. Note the loop only stores as many elements as the user so desires, so if the user only wants to input 3 numbers into the array, the for loop will only execute 3 times.
Once compiled, you should get this as your output:
How many numbers do you want to find the average for?: 5
Enter #1 : 23
Enter #2 : 17
Enter #3 : 29
Enter #4 : 14
Enter #5 : 16
The average of the 5 numbers is 19.8
====== FIND THE AVERAGE – OMIT HIGHEST AND LOWEST SCORES ======
The second program is really practical in a real world setting, specifically when a teacher records test scores into the computer. We were asked to create a program for a fictional competition which had 6 judges. The 6 judges each gave a score of the performance for a competitor in a competition, (i.e a score of 1-10), and we were asked to find the average of those scores, omitting the highest/lowest results. The program was to store the scores into an array, display the scores back to the user via cout, display the highest and lowest scores among the 6 obtained, display the average of the 6 scores, and finally display the average adjusted scores omitting the highest and lowest result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 |
#include <iostream> using namespace std; const int NUM_JUDGES = 6; int main() { int scores[NUM_JUDGES]; // array is initialized using a const variable int highestScore = -999999; int lowestScore = 999999; float sumOfScores = 0; float avgScores = 0; // use a for loop to obtain data from user using the const variable cout<< "Judges, enter one score each for the current competitor: "; for(int counter=0; counter < NUM_JUDGES; ++counter) { cin >> scores[counter]; } // use another for loop to redisplay the data back to the user via cout cout<<"nThese are the scores from the " << NUM_JUDGES << " judges: "; for(int index=0; index < NUM_JUDGES; ++index) { cout<<"nThe score for judge #"<<(index+1)<<" is: "<<scores[index]; } // use a for loop to go thru the array checking to see the highes/lowest element cout<<"nnThese are the highest and lowest scores: "; for(int index=0; index < NUM_JUDGES; ++index) { // if current score in the array is bigger than the current 'highestScore' // element, then set 'highestScore' equal to the current array element if(scores[index] > highestScore) { highestScore = scores[index]; } // if current 'lowestScore' element is bigger than the current array element, // then set 'lowestScore' equal to the current array element if(lowestScore > scores[index]) { lowestScore = scores[index]; } } cout << "ntHighest: "<< highestScore; cout << "ntLowest: "<< lowestScore; cout << "nThe average score is: "; // this calculates a running total, adding each element in the array together for(int counter=0; counter < NUM_JUDGES; ++counter) { sumOfScores += scores[counter]; } avgScores = sumOfScores/NUM_JUDGES; cout<< avgScores; // reset data back to 0 so we dont get wrong answers sumOfScores = 0; avgScores = 0; cout<<"nThe average adjusted score omitting the highest and lowest result is: "; // final loop, which calculates a running total, adding each element // in the array together, this time omitting the highest/lowest elements for(int counter=0; counter < NUM_JUDGES; ++counter) { // IF(current score isnt equal to the highest elem) AND (current score isnt equal lowest elem) // THEN create a running total if ((scores[counter] != highestScore) && (scores[counter] != lowestScore)) { sumOfScores += scores[counter]; } } avgScores = sumOfScores/(NUM_JUDGES-2); cout<< avgScores <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
CONST
A constant variable was declared and used to initialize the array (line 8). Note, when using static arrays, the program has to know how many elements to initialize the program with before the program starts, so creating a constant variable to do that for us is convenient.
FOR LOOPS
Once again loops were used to traverse the array, as noted on lines 16, 23, 30, 53, and 70. The const variable was also used within the for loops, making it easier to modify the code if its necessary to reduce or increase the number of available judges.
HIGHEST/LOWEST SCORES
This is noted on lines 34-44, and it is really simple to understand the process once you see the code.
OMITTING HIGHEST/LOWEST SCORE
Lines 70-78 highlight this process, and the loop basically traverses the array, skipping over the highest/lowest elements
Once compiled, you should get this as your output:
Judges, enter one score each for
the current competitor: 123 453 -789 2 23345 987These are the scores from the 6 judges:
The score for judge #1 is: 123
The score for judge #2 is: 453
The score for judge #3 is: -789
The score for judge #4 is: 2
The score for judge #5 is: 23345
The score for judge #6 is: 987These are the highest and lowest scores:
Highest: 23345
Lowest: -789
The average score is: 4020.17
The average adjusted score omitting the highest and lowest result is: 391.25
Leave a Reply